Using Multiple Variables in for or while Loops in Bash
Last updated: March 18, 2024
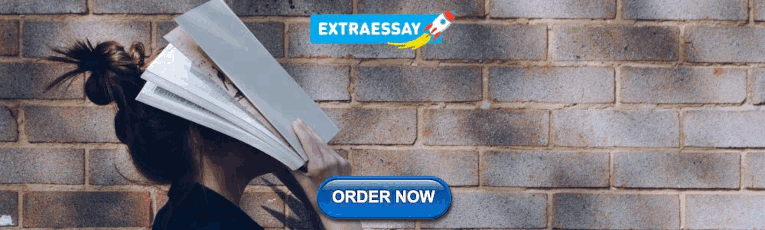
1. Introduction
Using multiple variables within a loop is particularly beneficial when dealing with array -structured data. Additionally, it proves advantageous when using a C-style syntax for iterative processing.
In this tutorial, we’ll discuss how to use multiple variables in a for loop in the shell .
First, we’ll discuss the classic C-style syntax method. After that, we’ll explore the nested loop method to use multiple variables in a for loop, followed by a discussion on custom delimiter approach with a for loop. Lastly, we’ll look into the use of the read command with while .
2. Using the C-Style for Loop
To begin with, we’ll use multiple variables in a C-style loop. The C-style syntax is often used in the context of the for loop for complex scenarios . Notably, we employ this type of loop in situations requiring more precise control over variables and conditions relative to a simple for loop. The syntax is similar to the C programming language.
Not all shells support C-style for loops, so using multiple variables in such loops may not be universally applicable across different shell environments.
First, let’s consider a simple shell for loop:
We use the simple shell for loop to iterate over a list and print each of the numbers. Notably, this loop type has a fairly simple syntax, making it easy to use for basic iteration tasks.
On the other hand, let’s check the C_style.sh script:
Now, we make the file executable using the chmod command, and run the script:
In the above code, the initial loop header action involves initializing two variables . Here, we initialize i to 1 and j to 10 . Next, we place a condition that the loop should continue as long as i is less than or equal to 5 . After each iteration, i is incremented by 1 , and j is decremented by 1 . Upon satisfying the condition, the loop terminates automatically.
Within the loop body, we utilize the echo command to display the values of variables i and j .
In summary, the code utilizes two variables in the for loop and displays the output of both variables at each step.
3. Using Nested for Loops
In the same way, we can utilize a nested for loop to use multiple variables for iterating over different types of elements .
Nested loops are convenient for performing operations on combinations of elements from various sets or generating patterns and grids. Also, they provide a structured way to traverse and manipulate data.
For instance, to iterate through two different text files, we’ll use nested for loops.
3.1. Example Script
In this approach, we use two files num.txt and char.txt , and read data from these files. Next, we store the filenames in the num and ch variables respectively:
After that, we initialize a counter variable int1 to 0 . Moreover, the outer for loop iterates over each value in num.txt . Also, the int1 counter increments by 1 after each iteration using parameter expansion .
Subsequently, the inner for loop iterates over each value in char.txt , starting from the line number specified by the current value of int1 :
- cat $ch outputs the contents of the file specified by the variable $ch
- tail -n +$int1 outputs all lines starting from line number $int1 onwards
- $(…) performs command substitution, replacing the expression with the output of the enclosed commands
To elaborate, for j in $(cat $ch | tail -n +$int1) sets up a loop where j takes on each value (word) in the output of cat $ch | tail -n +$int1 . The loop iterates over each word in the lines of char.txt starting from the line specified by int1 .
Lastly, we use the echo command to output the values of $i and $j .
The break statement exits the inner loop after processing the first value from char.txt . Additionally, the done command ends the outer loop, and the script continues to the next iteration of the outer loop with the subsequent value from num.txt .
So, let’s test the script.
3.2. Demonstration
First, let’s check our two sample files:
Moreover, we’ll make the script executable and run it:
In summary, the script reads numbers from num.txt in the outer loop and values from char.txt in the inner loop. For each value, it outputs the pair of values.
4. Using a Custom Delimiter With a for Loop
An alternative approach for utilizing multiple variables involves employing IFS with a for loop . Thus, we use multiple variables within the loop body.
In practice, the IFS variable is used to tokenize a string into fields based on the specified delimiter. To elaborate, this special shell variable determines how Bash recognizes word boundaries in strings.
In this method, we’ll use IFS with a for loop to iterate over different variables.
Now, let’s view the script:
The data file consists of some sample data:
Let’s make the script executable and run it:
In this script, we see that the IFS is set to the colon : character. Next, the for loop iterates over each line in the data.txt file. Each line is then assigned to the n variable.
Moreover, fields=($n) splits the value of n into an array called fields using the specified IFS . Then, first=”${fields[0]}” and second=”${fields[1]}” assigns the first and second fields of the array fields to the variables first and second , respectively.
Lastly, we use the echo command to print the output.
5. Using Multiple Variables in a while Loop With read
Similarly, we can use multiple variables with a while loop by combining it with the read command .
First, we’ll take a look at the sample file we’re working with:
Next, let’s view the whileloop.sh script:
Now, let’s execute and check the output of the script:
The above code uses a while loop with IFS to process the input from < “file.txt” . Here, IFS is set to the pipe character ( | ), indicating that fields within each line should be separated by this particular character.
Next, read -r i j uses the read command to get each line from the input, i.e., the data.txt contents. After that, it assigns the first field to variable i and the second field to variable j .
Furthermore, inside the loop, the echo command prints the values of i and j , separated by a colon.
Hence, this script reads each line from file.txt , and uses the pipe character as a field separator. Next, it outputs the first and second field values separated by a colon.
6. Conclusion
In this article, we gained insights into utilizing multiple variables within a shell script for loop .
First, we explored the C-style for loops, characterized by their resemblance to the syntax used in the C programming language. This method is for managing more intricate loop control structures.
After that, we discussed the nested loop method to use multiple variables in the for loop. Lastly, we discussed employing a read command with a while loop to use multiple variables.
How-To Geek
9 examples of for loops in linux bash scripts.

Your changes have been saved
Email Is sent
Please verify your email address.
You’ve reached your account maximum for followed topics.
How to Use Your Apple Watch for Sleep Tracking
Microsoft excel’s web app just got a massive overhaul, the beauty of local backups, quick links, the for loop, simple for loops, c-like for loops, for loops using word arrays, for loops using associative arrays, iterating over the output of commands, the dazzling for loop, key takeaways.
- The Bash for loop is a versatile tool that can work with numbers, words, arrays, command line variables, or the output of other commands.
- Simple for loops can be used with numerical lists or word lists, allowing you to repeat a section of code for each value in the list.
- The for loop can also be used with number ranges, filenames, command line parameters, word arrays, and associative arrays, providing flexibility in scripting tasks.
The versatile Bash for loop does much more than loop around a set number of times. We describe its many variants so you can use them successfully in your own Linux scripts.
All scripting and programming languages have some way of handling loops. A loop is a section of code that you want to have executed repeatedly. Rather than type the same set of instructions into your script , again and again, a loop will repeat one section of code over and over for you.
The Bash for loop is very flexible. It can work with numbers, words, arrays, command line variables, or the output of other commands. These are used in the header of the loop. The header dictates what the loop is working with---numbers or strings, for example---and what the end condition is that will stop the looping.
The body of the loop contains the code that you want to have repeated. It holds what you want the loop to do. The loop body can contain any valid script command.
A variable called the loop counter or iterator is used to step through a range of values or a list of data items. For each loop, the iterator takes on the value of the next number, string, or whatever data type the loop is iterating over. This allows the loop to work with the values of each of the data items in turn, or even in some cases to manipulate the data items themselves.
If you're looking to write your first for loop, these simple examples will get you started.
for Loops using Numerical Lists
You can run a for loop on the command line. This command creates and executes a simple for loop. The iterator is a variable called i . We're going to assign i to be each of the values in the list of numbers, in turn. The body of the loop is going to print that value to the terminal window. The condition that ends this loop is when i has iterated across the entire list of numbers.
for i in 1 2 3 4 5; do echo $i; done
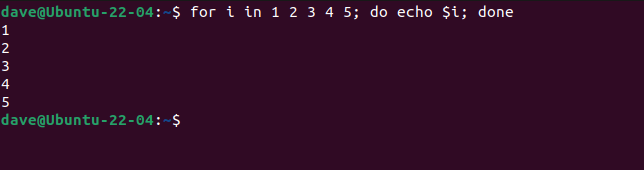
It's important to note here that the variable i is increased by one each time the loop spins round, but that's because the list of numbers goes up by one each time.
This list of numbers starts at 3 and goes up in steps of two, then arbitrarily leaps to 44.
for i in 3 5 7 9 11 44; do echo $i; done
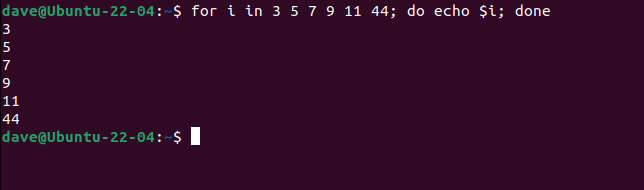
It makes no difference to the for loop. It starts at one end of the list and uses each value in turn, until all the values in the list have been used.
Nor do the numbers need to be in ascending order. They can be in any order.
for i in 3 43 44 11 9; do echo $i; done
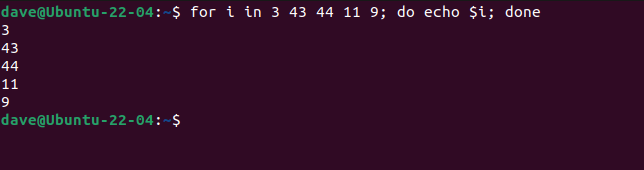
for Loops Using Word Lists
We can just as easily do the same with words. Copy the text of the script into an editor and save it as "word-list.sh."
You'll need to use chmod to make the script executable, and any other script you copy out of this article. Just substitute the name of the script each time you use the chmod command.
chmod +x word-list.sh

Let's run the script.
./word-list.sh
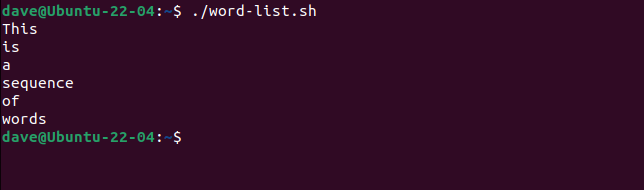
Just as it did with the numbers, the iterator---in this example, the variable word ---works its way through the list of data items until it reaches the end of the list. The loop body accesses the value in the word variable and so each word in the list gets processed.
for Loops with Number Ranges
If you wanted a for loop to run 100 times it would be a pretty tiresome affair to have to type in a sequence of 100 numbers in the loop header. Number ranges let you specify the first and last number only.
This script is "number-range.sh."
The number range is defined within curly brackets " {} " with two periods " .. " separating the numbers that start and end the range. Make sure you don't include any whitespace in the range definition.
This is how it runs:
./number-range.sh
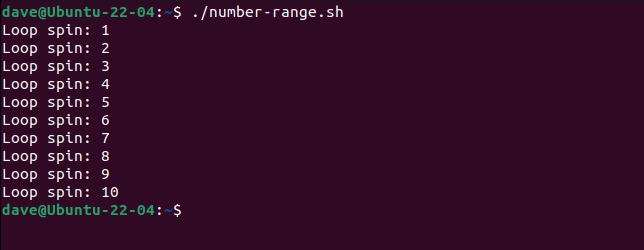
You can include another number that defines the step size the iterator should use to walk through the numbers in the range. This script, "number-range2.sh" will use a range of 0 to 32, and a step size of 4.
The iterator steps through the number range in jumps of four.
./number-range2.sh
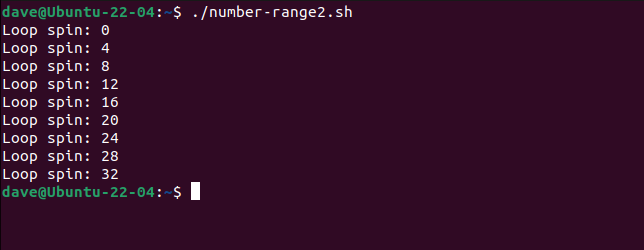
for Loops Using Filenames
Because we can process lists of words, we can get our scripts to work with filenames. This script is called "filenames.sh."
It would be pretty pointless to have a script that only does what ls can do, but it does demonstrate how to access filenames inside the loop body.
./filenames.sh
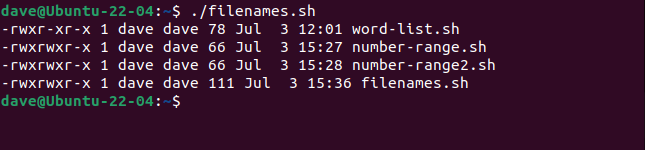
In a similar way to using the number range, we can use a file pattern in the loop header to specify the files we want to process. This avoids a lot of typing and means we don't need to know in advance the names of the files.
This script is called "filenames2.sh." We've replaced the list of filenames with the filename pattern "*.sh" to have the script report on all script files in the current directory.
Here's the output.
./filenames2.sh
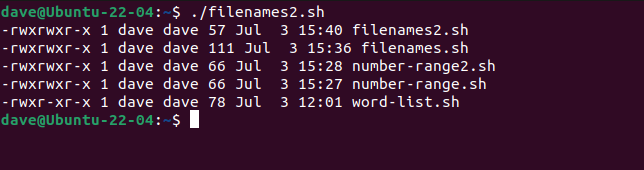
for Loops Using Command Line Parameters
We can add some more flexibility by passing in the filename pattern on the command line. The $* variable represents all of the command line parameters passed to the script.
This is "filenames3.sh."
We'll ask for filenames that begin with "n" and have an SH extension.
./filenames3.sh n*.sh

We can also pass in more than one pattern at a time.
./filenames3.sh n*.sh .bashrc
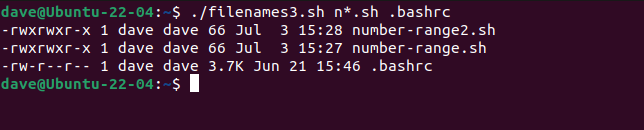
The iterator variable file takes on the value of each of the command line parameters. Filename patterns are expanded, and all of the filenames are processed in the loop body.
Bash supports the classic three-term for loop, such as those found in the C programming language. They're called three-term for loops because there are three terms in the loop header.
- The initial value of the loop iterator.
- The test for whether the loop continues or ends.
- The incrementing---or decrementing---of the iterator.
This script is "c-like.sh."
The iterator I is set to 1 at the start of the loop, and the loop will run for as long as the statement " i<=10 " is true. As soon as i reaches 11, the for loop will stop. The iterator is being increased by one, every revolution of the loop.
Let's run this script.
./c-like.sh
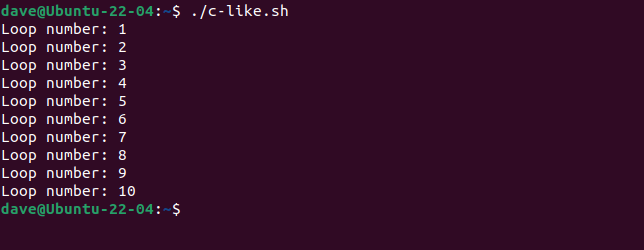
The C-like for loop permits the easy creation of for loops that have slightly odd requirements. This loop starts at 15, and counts backward in steps of 3. This is "c-like2.sh"
When we run it, it should jump backward in steps of three.
./c-like2.sh
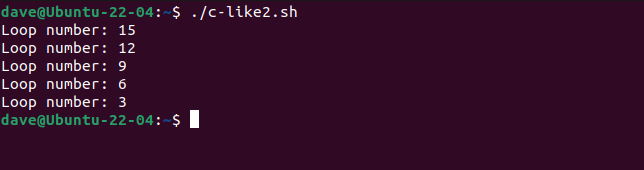
Infinite for Loops
You can also use this format of for loop to create an infinite loop. All you need do is remove all of the elements from the loop header, like this. This is "infinite.sh."
You'll need to hit Ctrl+C to stop the loop.
./infinite.sh
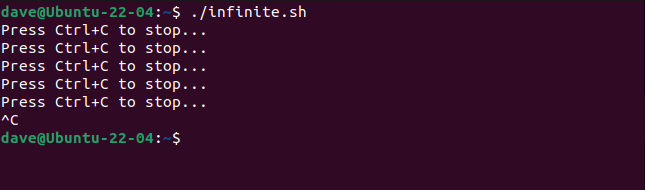
We can easily iterate through an array of words. We need to provide the name of the array in the loop header, and the iterator will walk through all entries in the array. This is "word-array.sh."
All the distributions are listed for us.
./word-array.sh
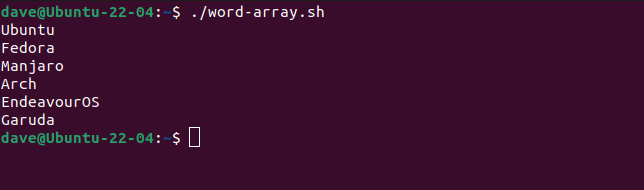
The continue Command
If you want the loop to step over a particular entry, test whether the iterator matches that entry and use the continue command. The continue command abandons the current spin of the loop. It increments the iterator and starts the next spin of the loop---assuming the entry you want to skip over isn't the last item in the list.
This is "word-array2.sh." It steps over the "Arch" array entry but processes all other array members.
"Arch" doesn't appear in the terminal window.
./word-array2.sh
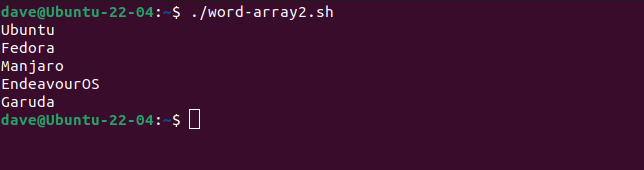
The break Command
The break command breaks out of the loop and prevents any more processing.
This is "word-array3.sh." It's the same as the previous script with continue replaced by break .
When the iterator contains "Arch" the for loop abandons any more processing.
./word-array3.sh
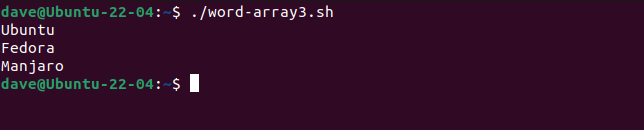
In Bash 4 and higher, associative arrays allow you to create lists of key-value pairs that can be searched by the key or by the value. Because of the two-way relationship between the key and the value, they're also called data dictionaries.
We can iterate through an associative array using a for loop. This script is "associative.sh." It defines an associative array with four entries in it, one for each of "dog", "cat", "robin" , and "human." These are the keys. The values are the (default) number of legs they each have.
The iterator is called legs . Note that the loop header contains an " ! " exclamation point. This is not acting as the logical NOT operator, it's part of the associative array syntax. It is required to search through the array.
The body of the loop performs a string comparison test. If the value of the array member is "Two-legged", it prints the key value to the terminal window. When we run it, the script prints the two-legged creatures.
./associative.sh

If you have a command or sequence of commands that produce a list of something, such as filenames, you can iterate through them with a for loop. You need to watch out for unexpected filename expansions, but in simple cases it is fine.
This script is "command.sh." it uses ls and wc to provide a sorted list of script file names, together with their line, word, and byte counts.
When we run it we get the statistics for each file, with the files listed in alphabetical order.
./command.sh
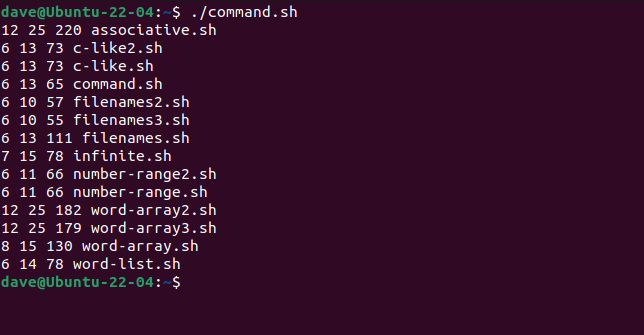
The for loop is a versatile and easily understood scripting tool. But as flexible as it is, don't forget that other loops exist for a reason. Don't be dazzled into thinking the for loop is all you'll ever need.
Related: How to Process a File Line by Line in a Linux Bash Script
The while loop, for example, is much better suited for certain things than the for loop, such as reading lines from a file .
Writing good scripts means using the most suitable tool for the task at hand. The for loop is a great tool to have in your toolbox of tricks.
- Linux & macOS Terminal
The Ultimate Guide To Using Bash For Loop Between Two Variables
What Is a Bash for Loop?
A Bash for loop is a looping construct that is used in Bash scripting. It allows the user to execute a set of commands for a specified number of times. The for loop is a very powerful tool that can be used to automate tasks and make them more efficient. It’s commonly used for programming tasks such as creating interactive scripts, automating system administration tasks, and more. In this article, we’ll take a look at how to use a Bash for loop between two variables.
How to Use a Bash for Loop Between Two Variables
Using a Bash for loop between two variables is easy. All you need to do is use the syntax for (variable_name; condition; increment_or_decrement) { } The variable_name is the name of the variable you want to use in the for loop. The condition is the condition you want to use to determine when the loop should stop. The increment_or_decrement is the amount by which you want to increment or decrement the variable_name on each iteration. For example, if you wanted to loop from 0 to 10, you would use the syntax for (i=0; i <= 10; i++){ } This would loop from 0 to 10, with the variable i being incremented by 1 each iteration.
Examples of Bash for Loop Between Two Variables
Here are some examples of how a Bash for loop between two variables can be used. The first example is a simple loop that will print out the numbers from 0 to 10.
for (i=0; i <= 10; i++){ echo $i } This will print out 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, and 10.
The next example is a loop that will print out the even numbers from 0 to 10.
for (i=0; i <= 10; i+=2){ echo $i } This will print out 0, 2, 4, 6, 8, and 10.
Using Arrays in Bash for Loop Between Two Variables
You can also use arrays in a Bash for loop between two variables. An array is a data structure that allows you to store multiple values in a single variable. To use an array in a Bash for loop, you need to use the syntax for (variable_name in array_name) { } The variable_name is the name of the variable you want to use in the for loop. The array_name is the name of the array you want to loop through. For example, if you had an array called colors that contained the values red, blue, and yellow, you could use the following syntax to print out the values in the array.
for (color in colors){ echo $color } This will print out red, blue, and yellow.
In this article, we’ve looked at how to use a Bash for loop between two variables. We’ve discussed the syntax for using a Bash for loop and given some examples of how it can be used. We’ve also looked at how to use arrays in a Bash for loop. With this knowledge, you should be able to use Bash for loops to automate tasks and make them more efficient.
See author's posts
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
- Articles Automation Career Cloud Containers Kubernetes Linux Programming Security
Introduction to Linux Bash programming: 5 `for` loop tips
%t min read | by Nathan Lager (Sudoer, Red Hat) , Ricardo Gerardi (Editorial Team, Sudoer alumni, Red Hat)

Every sysadmin probably has some skill they've learned over the years that they can point at and say, "That changed my world." That skill, or that bit of information, or that technique just changed how I do things. For many of us, that thing is looping in Bash. There are other approaches to automation that are certainly more robust or scalable. Most of them do not compare to the simplicity and ready usability of the for loop, though.
If you want to automate the configuration of thousands of systems, you should probably use Ansible. However, if you're trying to rename a thousand files, or execute the same command several times, then the for loop is definitely the right tool for the job.
[ You might also like: Mastering loops with Jinja templates in Ansible ]
If you already have a programming or scripting background, you're probably familiar with what for loops do. If you're not, I'll try to break it down in plain English for you.
The basic concept is: FOR a given set of items, DO a thing.
The given set of items can be a literal set of objects or anything that Bash can extrapolate to a list. For example, text pulled from a file, the output of another Bash command, or parameters passed via the command line. Converting this loop structure into a Bash script is also trivial. In this article, we show you some examples of how a for loop can make you look like a command line hero, and then we take some of those examples and put them inside a more structured Bash script.
Basic structure of the for loop
First, let's talk about the basic structure of a for loop, and then we'll get into some examples.
The basic syntax of a for loop is:
The variable name will be the variable you specify in the do section and will contain the item in the loop that you're on.
The list of items can be anything that returns a space or newline-separated list.
Here's an example:
That's about as simple as it gets and there isn't a whole lot going on there, but it gets you started. The variable $name will contain the item in the list that the loop is currently operating on, and once the command (or commands) in the do section are carried out, the loop will move to the next item. You can also perform more than one action per loop. Anything between do and done will be executed. New commands just need a ; delimiting them.
Now for some real examples.
Renaming files
This loop takes the output of the Bash command ls *.pdf and performs an action on each returned file name. In this case, we're adding today's date to the end of the file name (but before the file extension).
To illustrate, run this loop in a directory containing these files:
The files will be renamed like this:
In a directory with hundreds of files, this loop saves you a considerable amount of time in renaming all of them.
Extrapolating lists of items
Imagine that you have a file that you want to scp to several servers. Remember that you can combine the for loop with other Bash features, such as shell expansion, which allows Bash to expand a list of items that are in a series. This can work for letters and numbers. For example:
Assuming your servers are named in some sort of pattern like, web0 , web1 , web2 , web3 , you can have Bash iterate the series of numbers like this:
This will iterate through web0 , web1 , web2 , web3 , and so forth, executing your command on each item.
You can also define a few iterations. For example:
You can also combine iterations. Imagine that you have two data centers, one in the United States, another in Canada, and the server's naming convention identifies which data center a server HA pair lived in. For example, web-us-0 would be the first web server in the US data center, while web-ca-0 would be web 0's counterpart in the CA data center. To execute something on both systems, you can use a sequence like this:
In case your server names are not easy to iterate through, you can provide a list of names to the for loop:
You can also combine some of these ideas for more complex use cases. For example, imagine that you want to copy a list of files to your web servers that follow the numbered naming convention you used in the previous example.
You can accomplish that by iterating a second list based on your first list through nested loops. This gets a little hard to follow when you're doing it as a one-liner, but it can definitely be done. Your nested for loop gets executed on every iteration of the parent for loop. Be sure to specify different variable names for each loop.
To copy the list of files file1.txt, file2.txt, and file3.txt to the web servers, use this nested loop:
More creative renaming
There might be other ways to get this done, but remember, this is just an example of things you can do with a for loop. What if you have a mountain of files named something like FILE002.txt , and you want to replace FILE with something like TEXT . Remember that in addition to Bash itself, you also have other open source tools at your disposal, like sed , grep , and more. You can combine those tools with the for loop, like this:
Adding a for loop to a Bash script
Running for loops directly on the command line is great and saves you a considerable amount of time for some tasks. In addition, you can include for loops as part of your Bash scripts for increased power, readability, and flexibility.
For example, you can add the nested loop example to a Bash script to improve its readability, like this:
When you save and execute this script, the result is the same as running the nested loop example above, but it's more readable, plus it's easier to change and maintain.
You can also increase the flexibility and reusability of your for loops by including them in Bash scripts that allow parameter input. For example, to rename files like the example More creative renaming above allowing the user to specify the name suffix, use this script:
In this script, the user provides the source file's prefix as the first parameter, the file suffix as the second, and the new prefix as the third parameter. For example, to rename all files starting with FILE , of type .txt to TEXT , execute the script like this :
This is similar to the original example, but now your users can specify other parameters to change the script behavior. For example, to rename all files now starting with TEXT to NEW , use the following:
[ A free course for you: Virtualization and Infrastructure Migration Technical Overview . ]
Hopefully, these examples have demonstrated the power of a for loop at the Bash command line. You really can save a lot of time and perform tasks in a less error-prone way with loops. Just be careful. Your loops will do what you ask them to, even if you ask them to do something destructive by accident, like creating (or deleting) logical volumes or virtual disks.
We hope that Bash for loops change your world the same way they changed ours.

Nathan Lager
Nate is a Technical Account Manager with Red Hat and an experienced sysadmin with 20 years in the industry. He first encountered Linux (Red Hat 5.0) as a teenager, after deciding that software licensing was too expensive for a kid with no income, in the late 90’s. Since then he’s run More about me

Ricardo Gerardi
Ricardo Gerardi is Technical Community Advocate for Enable Sysadmin and Enable Architect. He was previously a senior consultant at Red Hat Canada, where he specialized in IT automation with Ansible and OpenShift. More about me
Try Red Hat Enterprise Linux
Download it at no charge from the red hat developer program., related content.
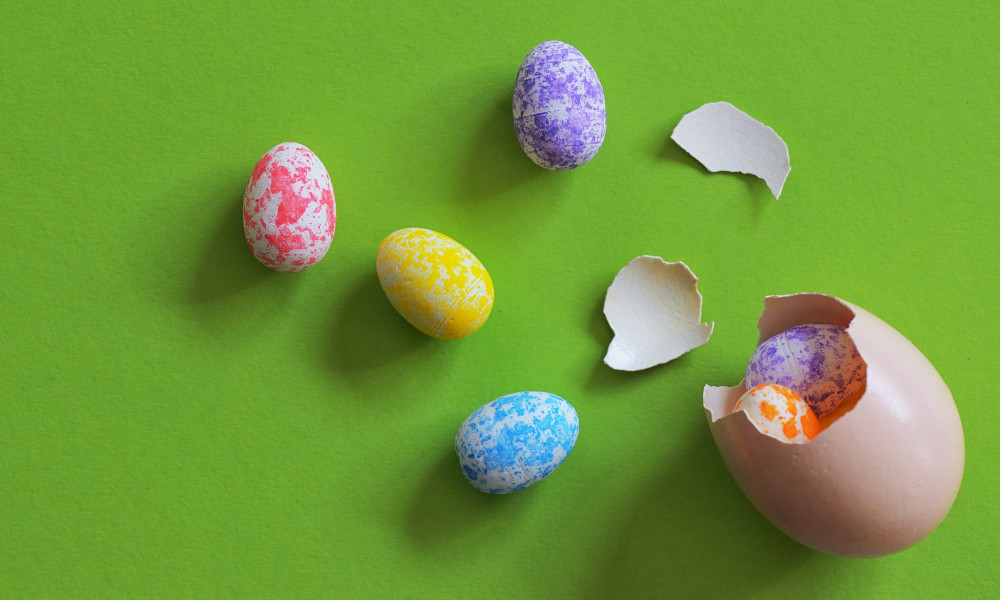

Home > Bash Scripting Tutorial > Loops in Bash > For Loop in Bash > 10 Common Bash “for” Loop Examples [Basic to Intermediate]
10 Common Bash “for” Loop Examples [Basic to Intermediate]

The Bash for loop is more than just a tool. It is a gateway to efficient scripting and streamlined automation. Its simplicity, which seems contradictory to its potential, offers scriptwriters a robust mechanism for streamlining operations. This article will go through some of the common bash scripts where for loop is used.
Table of Contents
10 Practical Bash “for” Loop Examples
The for loop in Bash is a fundamental control structure used for iterating over a list of items. It allows you to execute a sequence of commands repeatedly for each item in the list. It can be used to create patterns, create mathematical sequences, managing files etc. This article will explore 10 practical examples demonstrating the versatility of Bash for loop.
1. Chessboard Pattern Generation
The uses of nested for loops in Bash offer insight into advanced looping techniques for generating complex patterns or iterating through multidimensional data structures. In the following script, a chessboard pattern was created using nested for loop:
Here, the outer loop iterates over the rows of the chessboard, while the inner loop iterates over the columns. The script calculates the sum of the row and column numbers within each iteration. It then checks if the sum is even or odd by finding the remainder when divided by 2. Based on the parity of the sum, it alternates between printing white and black squares using ASCII color codes . Finally, after each row is processed, the script moves to the next row of the chessboard.
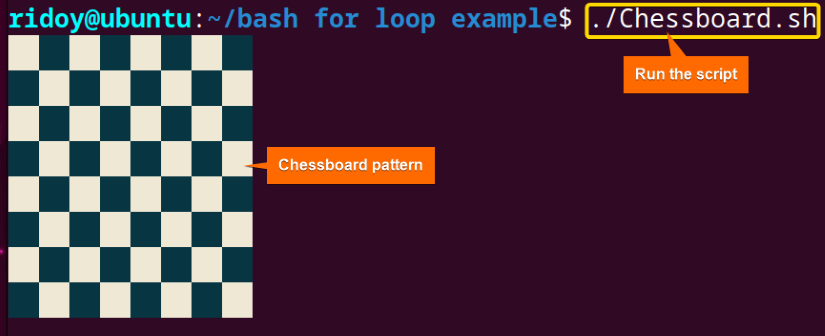
The chessboard pattern is displayed in the terminal.
2. Isosceles Triangle Pattern Generation
Using the versatility of the for loop in Bash, a pattern like isosceles triangle or pyramid-like pattern can be created. The following script employs two nested for loops to construct a triangular shape using asterisks * . Here is the script to do so:
This Bash script consists of two nested for loops. The outer loop iterates through numbers from 1 to 10, representing the rows of the triangle. Inside this loop, there are two inner loops. The first inner loop generates spaces before each row, with the number of spaces decreasing as the row number increases. The second inner loop prints asterisks, forming the triangular pattern, with the number of asterisks increasing as the row number increases.
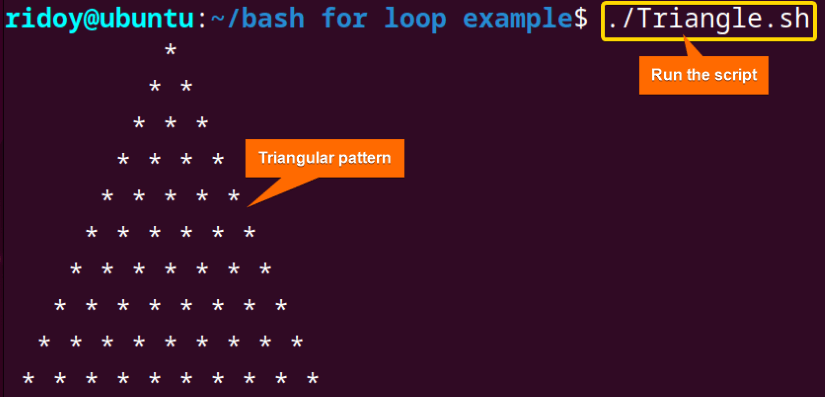
Here, the script displays a formatted multiplication table in the terminal.
3. Multiplication Table (5×10) Creation
The functionality of the for loop can be demonstrated by creating a concise multiplication table. This script creates a structured tabular multiplication table using a C style for loop and the printf command. Execute the bash script below to create a multiplication table of dimension 5 by 10:
This Bash script generates a multiplication table from 1 to 5, each row containing multiples up to 10. It begins by displaying a header with numbers from 1 to 10. After printing a separator line, it enters a loop to generate the table. Within this loop, for each row number, it calculates and prints the product of that number with numbers ranging from 1 to 10. The script utilizes nested loops to iterate through the rows and columns.
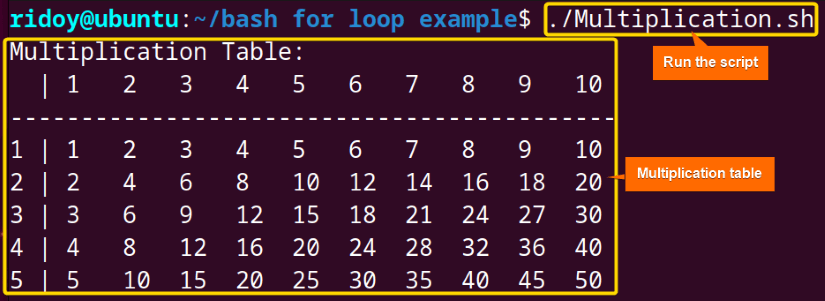
The script displays a formatted multiplication table in the terminal.
4. Prime Number Generation
The seq command in Bash is a utility used to generate sequences of numbers. It allows users to specify starting and ending values, along with an optional increment or decrement step. Execute this Bash script to print all prime numbers in a specific range using the seq command and nested “for” loop:
This Bash script generates prime numbers from 2 to 50 using nested loops. It begins by iterating through numbers from 2 to 50, considering each as a potential prime candidate. Within the outer loop, an inner loop tests divisibility from 2 up to half of the number’s value. If the number is not divisible by any integer within this range, it’s marked as prime . The script then checks the prime status and prints the number if it’s determined to be prime.
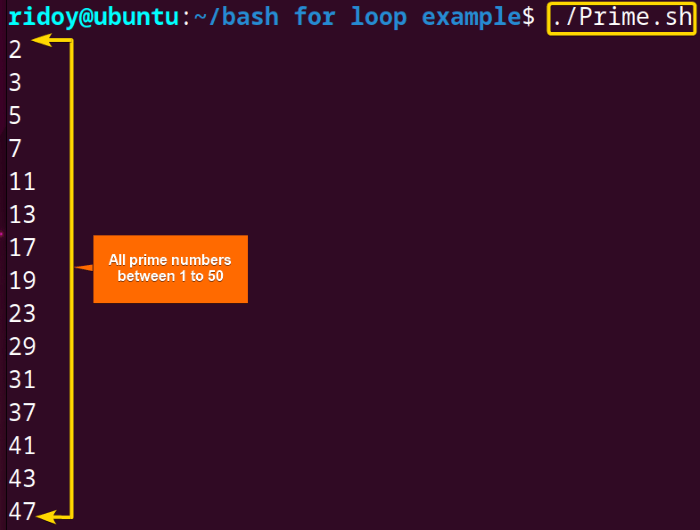
The script echoes all prime numbers from 1 to 50 in the terminal.
5. Palindrome Checking
A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. In other words, it remains unchanged when its characters are reversed. Checking for palindromes is a common task in programming. The following bash script checks if a given number is palindrome using the for loop and conditional statement:
This Bash script prompts the user to input a number and reads the input using the read command. It then initializes variables to store the reversed number and the original input. The script uses a for loop to reverse the digits of the number by repeatedly finding the remainder when divided by 10 and updating the reversed number variable.
After the loop, it compares the reversed number with the original input to determine if the number is a palindrome. Finally, it prints either “Palindrome” or “Not a palindrome” based on the comparison result.
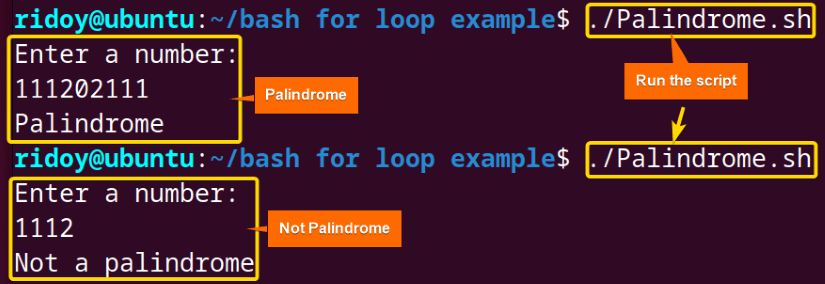
Here the 1st input is a palindrome. And the 2nd one is not palindrome.
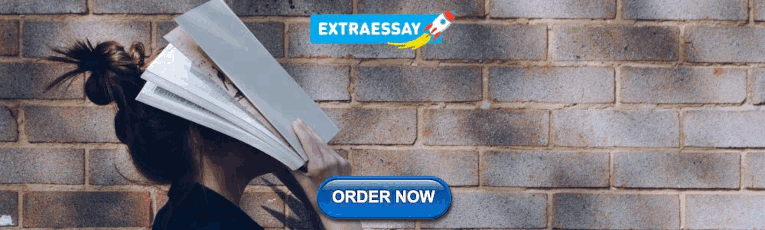
6. Fibonacci Calculation
Fibonacci numbers are a series where each number is the sum of the two preceding ones, typically starting with 0 and 1. The Fibonacci sequence is widely used in various mathematical and computational contexts. This Bash script takes a number as input and calculates the corresponding Fibonacci number using a for loop and a user-defined function:
This Bash script calculates the Fibonacci number for a given input. It defines a function fibonacci that iteratively computes the Fibonacci sequence up to the input number. The function initializes variables a and b as the first two Fibonacci numbers. It then uses a for loop to calculate subsequent Fibonacci numbers using the formula where each number is the sum of the two preceding numbers. Finally, it displays the Fibonacci number corresponding to the input.

The script echoes the 51st number of the Fibonacci sequence in the terminal.
7. Factorial Calculation
Factorial is a mathematical operation that calculates the product of all positive integers up to a given number. It is denoted by the symbol ! . The seq command in Bash is used to generate sequences of numbers. It allows users to specify starting and ending values, along with an optional increment or decrement step. Execute the following Bash script to calculate the factorial of a given number using the seq command within a “for” loop:
This Bash script calculates the factorial of a given number provided by the user. It prompts the user to enter a number using the read command and stores it in the variable num . Then, it initializes the variable fact to 1, which will store the factorial value. Using a for loop, it iterates from 1 to the input number and multiplies each number to calculate the factorial. Finally, it displays the factorial of the input number.

Here, the script echoes the factorial of 15 in the terminal.
8. Sorting Number
Sorting arrays is a fundamental task in programming to arrange elements in a specific order. Common sorting algorithms include bubble sort, insertion sort, quicksort, etc. In this Bash script, a simple bubble sort algorithm is utilized to arrange an array of integers in ascending order. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The process continues until it sorts the entire array.
Here is the script to implement this algorithm using nested for loop and conditional statements:
This Bash script sorts an array of integers. First, it declares an array named numbers containing unsorted integers. The script then iterates through the array using nested for loops, comparing adjacent elements and swapping them if they are in the wrong order. The process continues until it sorts the entire array. Finally, it prints the sorted array to the console.

Here, the script uses bubble sort to sort 6 elements of the array.
9. Password Generator
In the digital age, where cybersecurity is paramount, the need for robust password-generation methods is undeniable. Passwords serve as the first line of defense against unauthorized access to sensitive information. While creating strong passwords manually can be challenging, Bash scripting offers a practical solution. By combining character sets, numerical values, and special symbols, the following Bash script can generate secure passwords tailored to user specifications:
This script generates a random password based on user-defined criteria. It first defines character sets for lowercase letters, uppercase letters, numbers, and symbols. The user specifies the desired length of the password, which determines the number of characters the script will generate. Using a for loop, the script iterates through each position in the password length, randomly selecting characters from the concatenated character sets and appending them to the password string. Then it prints the generated password to the console for the user.

Here, a 10-digit password is generated automatically.
10. Image Converter
Image format conversion is a fundamental task in managing digital media. From JPEG to PNG, GIF to TIFF, the ability to seamlessly convert between formats ensures accessibility and usability across diverse platforms and devices. Execute the following script to convert all JPEG files in the current directory to PNG format concurrently :
This Bash script concurrently converts JPEG files to PNG format. It begins by echoing a message indicating the conversion process has started. The convert_image function is defined to convert each JPEG file to PNG using the ImageMagick package’s convert command. Within a for loop, it iterates through all JPEG files in the current directory, invoking the convert_image function for each file in the background using & . The wait command ensures all background processes are complete before echoing a message indicating that the format conversion is finished.
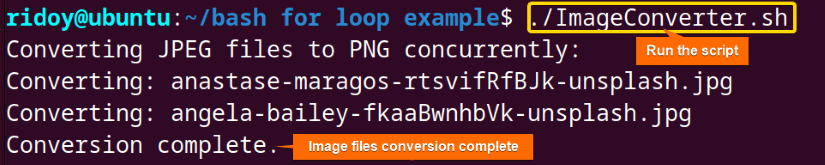
The script converts 2 images in JPEG format from the current directory to PNG format.
Note : If your system does not have the convert command, it’s likely that the command-line tool provided by the ImageMagick package, which includes the convert command, is not installed. Use your system’s package manager to install ImageMagick. Here is how you can do it in Ubuntu:
Practice Tasks on Bash “for” Loop
If you aim to be better at using the “for” loop in the Bash scripting, then you can try solving the following problems using the concept discussed in this article:
- Create a Bash script that renames all files in a directory with a specific extension (e.g., .txt) to include a timestamp using a for loop.
- Develop a Bash script that generates Pascal’s triangle, using the for loop.
- Develop a script that takes an array of strings as input and prints the elements in reverse order using a for loop.
- Write a Bash script that calculates the total size of all files in a directory and its subdirectories using a for loop.
- Create a script that periodically checks network connectivity by pinging remote servers. Log connection status and generate reports indicating downtime periods.
Mastering the for loop in Bash scripting opens doors to automating routine tasks effortlessly. Whether you are a Linux enthusiast or a scripting novice, I hope the examples presented in this article will sharpen your bash scripting skills.
People Also Ask
What is a loop in bash.
A loop in Bash is a programming construct used to execute a set of commands repeatedly based on certain conditions. It helps automate tasks by repeating actions without manual intervention. In Bash scripting, loops are essential for performing repetitive tasks efficiently. They enable the execution of commands or operations on multiple items or data sets, enhancing the efficiency of scripts and programs.
How to print a string list using bash for loop?
To print a list of strings using a Bash for loop, you can iterate through each string in the list and print it individually. First, define an array containing the strings you want to print using string_list=("string1" "string2" "string3" ... "stringN") . Then, use a for loop to iterate through each element of the array using for item in "${string_list[@]}" . Within the loop, access each element using array indexing and print it to the console with echo "$item" .
How do I run a for loop in Linux?
To run a for loop in Linux, you can use the Bash shell scripting language. First, you need to define the structure of your loop, typically using the syntax: for variable in list; do command; done . Here, for each iteration of the loop, the “ variable ” takes on the value of each item in the “ list “, and the associated “ command ” is executed. This structure allows you to automate repetitive tasks efficiently in a Linux environment.
Can you nest for loops in Bash?
Yes , you can nest for loops in Bash to perform more complex iterations. The basic syntax for nesting for loops in Bash is as follows:
In this structure, the outer loop iterates over the elements of the outer list, while the inner loop iterates over the elements of the inner list for each iteration of the outer loop. This allows for executing commands or actions for each combination of elements from both lists.
What is the structure of the for loop in Bash?
The structure of a for loop in Bash consists of defining a variable that iterates through a list of values. The basic syntax for a for loop in Bash is as follows:
In this structure, the “ variable ” represents the variable that changes with each iteration, while the “ list ” is the list of values over which the variable iterates. Within the loop body, commands to execute for each value in the list can be added.
Related Articles
- How to Iterate Through List Using “for” Loop in Bash
- How to Use Bash “for” Loop with Variable [12 Examples]
- Bash Increment and Decrement Variable in “for” Loop
- How to Use Bash Parallel “for” Loop [7 Examples]
- How to Loop Through Array Using “for” Loop in Bash
- How to Use Bash “for” Loop with Range [5 Methods]
- How to Use Bash “for” Loop with “seq” Command [10 Examples]
- How to Use “for” Loop in Bash Files [9 Practical Examples]
- Usage of “for” Loop in Bash Directory [5 Examples]
- How to Use “for” Loop in One Line in Bash [7 Examples]
- How to Create Infinite Loop in Bash [7 Cases]
- How to Use Bash Continue with “for” Loop [9 Examples]
<< Go Back to For Loop in Bash | Loops in Bash | Bash Scripting Tutorial

Ridoy Chandra Shil
Hello everyone. I am Ridoy Chandra Shil, currently working as a Linux Content Developer Executive at SOFTEKO. I am a Biomedical Engineering graduate from Bangladesh University of Engineering and Technology. I am a science and tech enthusiast. In my free time, I read articles about new tech and watch documentaries on science-related topics. I am also a big fan of “The Big Bang Theory”. Read Full Bio
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.

Get In Touch!
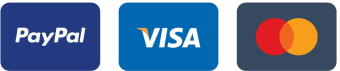
Legal Corner
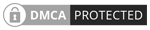
Copyright © 2024 LinuxSimply | All Rights Reserved.
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Using multiple variables in a for loop
I want to use two variables in a for loop like this (This is for example, I'm not going to execute seq like operations)
Expected output is
1 www.google.com 2 www.yahoo.com
- command-line

3 Answers 3
If i was to just be a number that incremented with each string, you could try a for loop and increment i with each iteration.
For example:

- why did you, use double braces in $((i++)) . I think one braces is enought for this. Please correct me , If i said wrong. – SuperKrish Commented Nov 5, 2016 at 8:32
- 1 @SuperKrish $(( . . .)) is arithmetic expansion, which is what makes i++ work. With single braces that's $(. . .) command substitution , so i++ won't work there. If you were trying to catch output of another program, say $( df ) for example, you could use single braces. If you need calculation, then you use $(( . . . )) structure – Sergiy Kolodyazhnyy Commented Nov 5, 2016 at 10:30
- Great! @jake My doubt is clear – SuperKrish Commented Nov 5, 2016 at 10:47
Lets create variable to point to the file location
FILE="/home/user/myfile"
The file content:
To get the output of:
It can be done by one of the following methods below:
Using counter variable:
Using cat -n (number all output lines)
Using array:
If your file already with line numbers, example:
Loop and split every line to array:
For more info:
How to increment a variable in bash?
Loop Through Array of Strings in Bash Script
Arrays Syntax

Here are some alternative ways, two short and simple ones:
which both output:
and the slightly more complex:
that outputs:
In that second suggestion, I'm using an array named s created with the line s=(xx yy)
The ${#s[@]} syntax is the number of elements in the array, here 2 and the ${s[i-1]} is element at offset i-1 from the beginning of the array, thus ${s[1-1]} is ${s[0]} and then is www.google.com , etc.

- I dont know what is means ${#s[@]} `${s[i-1]}1. Here s is the variable loaded with some values.Can you clear my doubt? – SuperKrish Commented Nov 5, 2016 at 14:15
- Answer edited with explanations added. – jlliagre Commented Nov 5, 2016 at 15:26
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged command-line bash ..
- The Overflow Blog
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- The meaning of "奪耳" in 《說文解字》
- How to photograph the lettering on a bronze plaque?
- Tour de France General Classification time
- Why do Electric Aircraft Seem to Eschew Photovoltaics?
- Job talk Q&A: handling the room vs. being respectful
- Air magic only used to decrease humidity and improve living conditions?
- Optimizing Pi Estimation Code
- Simulate slow disks in KVM to see effect of LVM cache in test setup
- What spells can I cast while swallowed?
- Is a desert planet with a small habitable area possible?
- Can the US president legally kill at will?
- ForeignFunctionLoad / RawMemoryAllocate and c-struct that includes an array
- A manifold whose tangent space of a sum of line bundles and higher rank vector bundles
- pdfgrep How to locate the pages that contain multiple strings and print the page numbers?
- Why did Nigel Farage choose Clacton as the constituency to campaign in?
- Plastic plugs used to fasten cover over radiator
- Is it well defined to cast to an identical layout with const members?
- Improve spacing around equality = and other math relation symbols
- Weather on a Flat, Infinite Sea
- Why is there not a test for diagonalizability of a matrix
- PowerShell manipulation of `for` results
- When selling a machine with proprietary software that links against an LGPLv3 library, do I need to give the customer root access?
- How do we define addition?
- Center Set of Equations Using Align
- Mobile Site
- Staff Directory
- Advertise with Ars
Filter by topic
- Biz & IT
- Gaming & Culture
Front page layout
for comment in $comments ; upvote $comment ; done —
Command line wizardry, part two: variables and loops in bash, learn to process thousands of items reliably and repeatably in this installment..
Jim Salter - Sep 20, 2021 11:15 am UTC
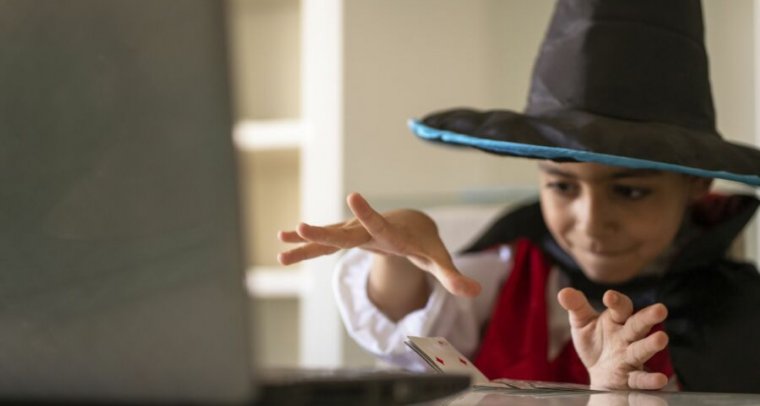
In our first tutorial on command line wizardry, we covered simple redirection and the basics of sed, awk, and grep. Today, we're going to introduce the concepts of simple variable substitution and loops—again, with a specific focus on the Bash command line itself, rather than Bash scripting.
If you need to write a script for repeated use—particularly one with significant logical branching and evaluation—I strongly recommend a "real language" instead of Bash. Luckily, there are plenty of options. I'm personally a big fan of Perl, in part because it's available on pretty much any *nix system you'll ever encounter. Others might reasonably choose, say, Python or Go instead, and I wouldn't judge.
The real point is that we're focusing on the command line itself. Everything below is something you can easily learn to think in and use in real time with a little practice.
Setting and getting values in Bash
Bash handles variables a bit oddly compared to any of the "real" languages I've used. In particular, you must refer to the same variable with different syntax when setting its value versus when retrieving it.
Let's take a look at a very simple example:
If you've never worked with variables in Bash before, you might think there's a typo in the above example. We set the value of hello but read back its value as $hello . That's not a mistake—you don't use the leading $ when defining/setting a variable, but you must use it when reading from the same variable later.
Destroying variables
If we need to clear that variable, we can use the unset command—but, again, we must refer to hello instead of $hello :
Thinking about the error messages we get when trying incorrectly to unset $hello should make the problem clear. When we unset $hello , we're passing the value of hello to unset rather than passing the variable name itself. That means exactly what you think it does:
Although the switch back and forth between referencing hello and $hello is deeply unsettling if you're only familiar with "real" languages, Bash is at least relatively consistent about when it uses each style. With a little practice, you'll get used to this—even if you never feel truly comfortable about it.
Variable scope
Before we move on from the basics of variables, we need to talk about variable scope. Basically, a Bash variable is limited in scope to only the current process. It won't pass your variables on to any child processes you initiate. We can see this in action most easily by calling bash from bash :
In our original Bash session, we set the variable hello to equal "Hello, world!" and access it successfully with the echo command. But when we start a new bash session, hello is not passed on to that child session. So when we again try to echo $hello , we get nothing. But after exiting the child shell, we can once again successfully echo $hello and receive the value we originally set.
If you need to carry variables set in one session into a child process, you need to use the export command:
As you can see, export successfully flagged our variable hello for passing down to child processes—in this case, another instance of bash . But the same technique works, in the same way, for calling any child process that references environment variables. We can see this in action by writing a very small Perl script:
Just like our earlier example of a child session of bash , our Perl script can't read our hello environment variable unless we first export it.
The last thing we'll say about export is that it can be used to set the value of the variable it's exporting, in one step:
Now that we understand how to set and read values of variables on the command line, let's move on to a very useful operator: $() .
Capturing command output with $()
The $() command substitution operator captures the output of a command. Sounds simple enough, right? In the above example, we demonstrate that the output of the command echo test is, of course, "test"—so when we echo $(echo test) we get the same result.
Backticks can provide the same result—eg, echo `echo test` —but $() is generally preferred, since unlike backticks, the $() operator can be nested.
We can take this a step further and instead assign the output of a command into a variable:
Finally, we can combine this with the lessons from our first tutorial and strip the first column from du 's output to assign to our variable:
At this point, we understand how variables and the $() operator work. So let's talk about simple looping structures.
For loops in Bash
The above example concisely demonstrates the way a simple for loop works in Bash. It iterates through a supplied sequence of items, assigning the current item from the list to a loop variable. We used semicolons to separate the for loop itself, the do command that marks the interior of the loop, and the done , which lets us know the loop is over. Alternatively, we could also enter them as technically separate lines.
In this example, the leading angle brackets aren't something you type—they're a prompt supplied by Bash itself. This lets you know you're still inside a loop despite having hit Enter . And that's important, because you can have as many commands as you'd like inside the loop itself!
As you can see, we can string as many commands as we'd like together inside our for loop. Within the loop, each command may refer to the loop variable if it likes—or it can ignore the loop variable entirely.
So far, we've only looked at numbers in sequences, but the for loop doesn't care about that a bit. Any sequence of items, in any order, will work:
Finally, you don't have to supply the list with a variable; you can also embed it directly in the loop... but be careful about how you use quotes, because bash considers a quoted "list" to be a single item:
reader comments
Channel ars technica.

- Search forums
- General Linux Forums
- Command Line
Bash Scripting FOR loop with 2 variables
- Thread starter Neuls
- Start date Nov 15, 2016
- Tags bash;for;loop
- Nov 15, 2016
Hi all, I'm not pretty sure if this post is placed in the right forum.. I'd like to write a script which does the following idea: #!/bin/bash CODESEC=`cut -c 1-5 Data.txt` CODEORD=`cut -c 7-21 Data.txt` for i in ${CODESEC} AND j in ${CODEORD} do echo $i $j done I have googled this concept several times but i cant find a way which solves this problem, mainly because both variables are extracted from the same database .txt file and must be utilized at the same time Thank you for yr help
Try this: Save this as whatever you want to call it. I've just called it cutfile! Code: #!/bin/bash if [ $# -eq 1 ] && [ -f $1 ]; then while read line do CODESEC=`echo ${line} | cut -c 1-5` CODEORD=`echo ${line} | cut -c 7-21` echo "${CODESEC} ${CODEORD}" done < ${1} else echo "Usage: ${0} {filename}" fi Run it using: ./cutfile /path/to/data.txt What it does: - Checks that a single parameter was passed to the script and the parameter is a valid file - If no parameter is passed, or the parameter is not a valid file - some usage information is displayed. - Assuming we have a valid file, we keep reading lines from the file, extracting the information we require into variables which are then echoed to the screen. Is that close to what you were trying to do?
- Nov 17, 2016
JasKinasis said: Try this: Save this as whatever you want to call it. I've just called it cutfile! Code: #!/bin/bash if [ $# -eq 1 ] && [ -f $1 ]; then while read line do CODESEC=`echo ${line} | cut -c 1-5` CODEORD=`echo ${line} | cut -c 7-21` echo "${CODESEC} ${CODEORD}" done < ${1} else echo "Usage: ${0} {filename}" fi Run it using: ./cutfile /path/to/data.txt What it does: - Checks that a single parameter was passed to the script and the parameter is a valid file - If no parameter is passed, or the parameter is not a valid file - some usage information is displayed. - Assuming we have a valid file, we keep reading lines from the file, extracting the information we require into variables which are then echoed to the screen. Is that close to what you were trying to do? Click to expand...
- Nov 18, 2016
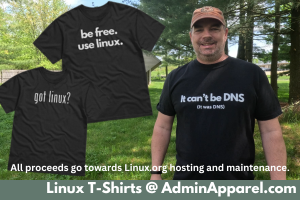
Staff online

Members online
- wizardfromoz
- Sherri is a Cat
Latest posts

- Latest: f33dm3bits
- 10 minutes ago
- Latest: PunchFox
- Today at 5:48 AM
- Latest: wizardfromoz
- Today at 5:38 AM
- Today at 5:34 AM

- Latest: wildman
- Today at 5:27 AM
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Can I do a for loop over variables in the bash shell?
I'm learning the shell, and I want to be able to loop over some variables. I can't seem to find anywhere where anyone has done this so I'm not sure it's even possible.
Basically I just want to save myself trouble by using the same sed command on each of these variables. However the code obviously doesn't work. My question is, is it possible to loop over variables and if not how should I be doing this?
here is the error:
- What's the error when you run the code? (Assuming you're referring to something that the syntax highlighting didn't already point out to you...) – user541686 Commented Jun 18, 2011 at 8:00
- Another common way to do this type of problem is to do something like: – William Pursell Commented Jun 19, 2011 at 9:42
Your problem isn't with the loop, it's with the assignment. The variable name needs to be literal in an assignment, i.e. you can write title=some_value but not $arg=some_value .
A portable way to assign to a variably-named variable is to use eval . You also need to obtain the value of $arg (not just the value of arg , which is $arg ), which again requires using eval .
Another way to assign to a variably-named variable that's specific to bash/ksh/zsh but won't work in plain sh is to use the typeset built-in. In bash, if you do this in a function, this makes the assignment local to the function. To obtain the value of the variably-named variable, you can use ${!arg} ; this is specific to bash.
Other problems with your snippet:
- title="$(echo string1)" is a complicated way to write title="string1" , which furthermore may mangle string1 if it contains backslashes or begins with - .
- You need a command terminator ( ; or newline) before the do keyword.
If you're relying on bash/ksh/zsh, you can make the replacements inside the shell with the ${VARIABLE//PATTERN/REPLACEMENT} construct.

- this is fantastic. I tried it out and actually it didn't quite work (I'm trying the portable route). Here's what I came up with: new_value="$(eval printf %s \"\$$arg\" | sed -e 's/&/\\&/g' -e 's/</\\</g' -e 's/>/\\>/g')" eval arg=\$new_value I had to add a second $ to the printf statement or else new_value would equal "title" "album" or "artist". I also removed the $ from the second eval statement or else that would equal "title" etc.. oh and for curiosity's sake why does there have to be a \$ before new_value on the second line as opposed to just $? – Dennis Commented Jun 18, 2011 at 9:59
- @Dennis: Sorry, the missing $ in the printf call was a typo. I don't see anything wrong with any of the others, which one did you mean? In eval $arg=\$new_value , the string to evaluate is something like title=$new_value , which assigns the value of new_value to the variable title . If you wrote eval $arg=$new_value , the string to evaluate would be e.g. title=Apostrophe (') : the new value would be used as shell code, not as a literal string. – Gilles 'SO- stop being evil' Commented Jun 18, 2011 at 13:51
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged linux shell variables or ask your own question .
- The Overflow Blog
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
- The [lib] tag is being burninated
- What makes a homepage useful for logged-in users
Hot Network Questions
- Minimum number of select-all/copy/paste steps for a string containing n copies of the original
- Tikz: specific tool for exploded-view drawings?
- How do I drill a 60cm hole in a tree stump, 4.4 cm wide?
- Is "necesse est tibi esse placidus" valid classical Latin?
- Reversing vowels in a string
- Job talk Q&A: handling the room vs. being respectful
- Why are responses to an attack in a cycling race immediate?
- Souls originating from Adam HaRishon
- How to solve the intersection truncation problem of multiple \draw[thick, color=xxx] commands by color?
- How to optimize performance with DeleteDuplicates?
- Why did Nigel Farage choose Clacton as the constituency to campaign in?
- Would this telescope be capable to detect Middle Ages Civilization?
- Vacuum region in SIESTA
- Why does the length of an antenna matter when electromagnetic waves propagate perpendicular to the antenna?
- Challenge the appointment of the prime minister
- Could two moons orbit each other around a planet?
- Where is the pentagon in the Fibonacci sequence?
- What does "that" in "No one ever meant that, Drax" refer to?
- ForeignFunctionLoad / RawMemoryAllocate and c-struct that includes an array
- Manga/manhua/manhwa where the female lead is a princess who is reincarnated by the guard who loved her
- Why not build smaller Ringworlds?
- It was the second, but we were told it was the fifth
- 130 TIF DEM file (total size 3 GB) become 7.4 GB TIF file after merging. Why?
- How to see what statement (memory address) my program is currently running: the program counter register, through Linux commands?
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Loop through variable in bash using "2" variables
So I know how to loop through 1 variable:
But how to do it when they are 2?
So script should loop each folder with correct mount added.
At the end, execution should be like this:
- shell-script
- How to iterate two variables in a sh script? – kaylum Commented Jan 12, 2020 at 1:05
- Done. Final version added of explanation. – Viktor Commented Jan 12, 2020 at 1:06
You need to iterate over the array index number and not the value. This is done using the ${!folder[@]} syntax.
In that way you can access both arrays via the same index inside the loop
e.g. (putting echo in front for this example)
Will output
You must log in to answer this question.
Not the answer you're looking for browse other questions tagged bash shell-script ..
- The Overflow Blog
- Community Products Roadmap Update, July 2024
- Featured on Meta
- We spent a sprint addressing your requests — here’s how it went
- Upcoming initiatives on Stack Overflow and across the Stack Exchange network...
Hot Network Questions
- 130 TIF DEM file (total size 3 GB) become 7.4 GB TIF file after merging. Why?
- Does installing Ubuntu Deskto on Xubuntu LTS adopt the longer Ubuntu support period
- Why did the main wire to my apartment burn before the breaker tripped?
- Why do Electric Aircraft Seem to Eschew Photovoltaics?
- pdfgrep How to locate the pages that contain multiple strings and print the page numbers?
- Capture multiple errors before raising an exception
- Are there dedicated research facilities in the USA?
- Vacuum region in SIESTA
- When do people say "Toiletten" in the plural?
- What is the reason for using decibels to measure sound?
- Is "necesse est tibi esse placidus" valid classical Latin?
- Minimum number of select-all/copy/paste steps for a string containing n copies of the original
- Can you be charged with breaking and entering if the door was open, and the owner of the property is deceased?
- Transferring at JFK: How is passport checked if flights arrive at/depart from adjacent gates?
- Level shift a digital 1.8V signal to split supply rail, using BJT
- Confusion on defining uniform distribution on hypersphere and its sampling problem
- Can player build dungeons in D&D? I thought that was just a job for the DM
- Can the differential be unitless while the variable have an unit in integration?
- Segments of a string, doubling in length
- How to photograph the lettering on a bronze plaque?
- How to see what statement (memory address) my program is currently running: the program counter register, through Linux commands?
- Souls originating from Adam HaRishon
- Are there rules for gender of durations?
- Is there a way to change a cantrip spell type to Necromancy in order to fulfil the requirement of the Death Domain Reaper ability for Clerics?
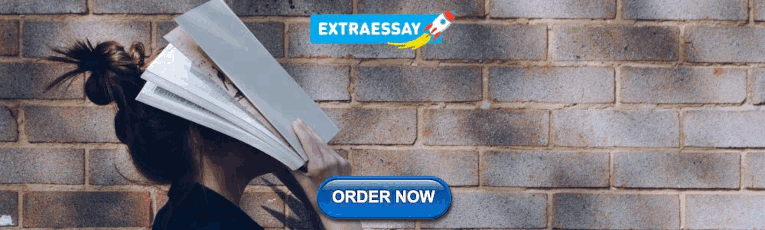
IMAGES
VIDEO
COMMENTS
This works fine. It loops thru 4 times, 0 to 3 inclusive, printing my message and putting the count at the end. do some stuff 0 do some stuff 1 do some stuff 2 do some stuff 3 When I try the same thing with the following for loop, it seems to equal a string, which is not what i want.
First, we'll discuss the classic C-style syntax method. After that, we'll explore the nested loop method to use multiple variables in a for loop, followed by a discussion on custom delimiter approach with a for loop. Lastly, we'll look into the use of the read command with while. 2. Using the C-Style for Loop.
Here, all array elements are echoed on separate lines. Example 2: Calculate the Sum of Numbers. Calculating the sum of some predefined numbers is a very common task in Bash scripting. The script uses a "for" loop to iterate through the numbers of a list and adds them to a running total.After the loop is complete, the total sum is echoed.
That's stdin, stdout and stderr and the commands inside the loop may need to use them (in the case of printf only 1 and possibly 2 for errors). 3<&- is the syntax to close a fd. We're closing 3 for the commands inside the loop as they don't need access to (and shouldn't use) that resource.
The continue Command If you want the loop to step over a particular entry, test whether the iterator matches that entry and use the continue command. The continue command abandons the current spin of the loop. It increments the iterator and starts the next spin of the loop---assuming the entry you want to skip over isn't the last item in the list.
Using a Bash for loop between two variables is easy. All you need to do is use the syntax for (variable_name; condition; increment_or_decrement) {. The variable_name is the name of the variable you want to use in the for loop. The condition is the condition you want to use to determine when the loop should stop.
A for loop is classified as an iteration statement i.e. it is the repetition of a process within a bash script. For example, you can run UNIX command or task 5 times or read and process list of files using a for loop. A for loop can be used at a shell prompt or within a shell script itself. Tutorial requirements.
Loop through variable in bash using "2" variables. Related. 2. How to iterate a command with two different variables? 2. How to iterate through a variable while skipping one of the values? 1. How to extract and concatenate variable values into another variable? 2.
The basic syntax of a for loop is: for <variable name> in <a list of items>;do <some command> $<variable name>;done; The variable name will be the variable you specify in the do section and will contain the item in the loop that you're on. The list of items can be anything that returns a space or newline-separated list.
Extending my answer based on the OP's clarification. Again, you can use an array: $ cat /tmp/foo.sh #/bin/bash # Sample values from OP export MASTER_CLUSTER_1="MASTER1 MASTER2" export MASTER_CLUSTER_2="MASTER3 MASTER4" # (edited to be unique) export SLAVE_CLUSTER_1="SLAVE1 SLAVE2 SLAVE3 SLAVE4" export SLAVE_CLUSTER_2="SLAVE5 SLAVE6 SLAVE7 SLAVE8" # (edited to be unique) # Create two arrays ...
It then loops over this list, setting the variable name to the value of the current filename. In the loop, for each filename, the first positional parameter is shifted off of the list of positional parameters. ... Create variables and assign values via loop (bash) 2. Need to iterate through subdirectories, concatenating files, with an iterative ...
This Bash script consists of two nested for loops. The outer loop iterates through numbers from 1 to 10, representing the rows of the triangle. Inside this loop, there are two inner loops. ... The structure of a for loop in Bash consists of defining a variable that iterates through a list of values. The basic syntax for a for loop in Bash is as ...
Tested on Mac OSX 10.6.8, Bash 3.2.48. Share. Improve this answer. Follow edited Dec 22, 2020 at 8:23. answered Jun 18, 2019 at 16:54. Zimba Zimba. 3,387 23 23 ... Bash for loop on variable numbers. 1. How to loop through mixed numbers in Bash. 3. Bash: iterate in a list of variables. 1.
I commonly like to use a slight variant on the standard for loop. I often use this to run a command on a series of remote hosts. I take advantage of Bash's brace expansion to create for loops that allow me to create non-numerical for loops. Example: I want to run the uptime command on frontend hosts 1-5 and backend hosts 1-3:
The while loop is another popular and intuitive loop you can use in bash scripts. The general syntax for a bash while loop is as follows: while [ condition ]; do. [COMMANDS] done. For example, the following 3x10.sh script uses a while loop that will print the first ten multiples of the number three: #!/bin/bash.
Using kernel 2.6.x. GNU bash, version 4.3.42(1)-release (arm-openwrt-linux-gnu) In a bash script, how would you write a for loop that produces the following result with the variables below ?
Stack Exchange Network. Stack Exchange network consists of 183 Q&A communities including Stack Overflow, the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.. Visit Stack Exchange
reader comments 291 Command Line Wizardry. Command line wizardry, part two: Variables and loops in Bash; Linux/BSD command line wizardry: Learn to think in sed, awk, and grep
Going back to the parameters: If you want to be able to pass the script a list of files to operate on, you could always check the number of parameters ($#), and then use a loop to check that each parameter is a valid file. - If it is a file - process it using your "while read" loop.
Two variables in for loop in bash. Hot Network Questions Capture multiple errors before raising an exception How do I drill a 60cm hole in a tree stump, 4.4 cm wide? What spells can I cast while swallowed? How to manage talkover in meetings? Sci-fi book series about a teenage detective, possibly named Diamond, with a floating AI assistant ...
Another way to assign to a variably-named variable that's specific to bash/ksh/zsh but won't work in plain sh is to use the typeset built-in. In bash, if you do this in a function, this makes the assignment local to the function. To obtain the value of the variably-named variable, you can use ${!arg}; this is specific to bash.
You need to iterate over the array index number and not the value. This is done using the ${!folder[@]} syntax. In that way you can access both arrays via the same index inside the loop. e.g. (putting echo in front for this example) folder=(first second third) mount=(something1 something2 something3) for i in "${!folder[@]}"; do. echo rclone ...