
- Forum Listing
- Advanced Search
- Software & Hardware
- Software Development
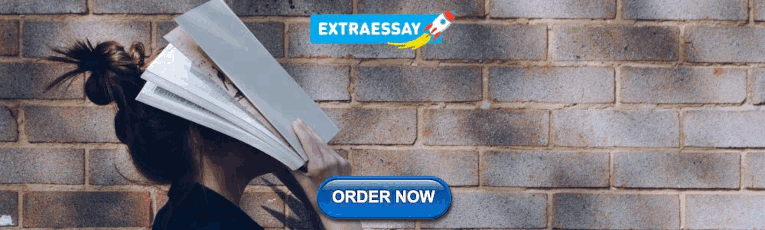
Solved: Assign Numbers to Letters

- Add to quote
Hey, Question-what would be the easiest way to code a program in c/c++ that would output a number according to its neumeric postition in the alphabet without a lot of nested if statements e.g: #include <iostream> void main() { char letterin == 'A'; \\Stuff goes here that changes letterin into 1 (i.e. A = 1, B = 2, Z = 26) } Thanks Doctorzeus

Hi, you could use the following method: Code: #include <iostream> using namespace std; char letters[27] = "abcdefghijklmnopqrstuvwxyz"; void main() { while(true) { cout << "Enter a letter: "; char letterIn; cin >> letterIn; for(int i = 1; i <= 26; i++) { if(letterIn == letters[i-1]) { cout << "Letter #" << i << "\r\n\r\n"; break; } } } } There I just have a char array with all the letters of the alphabet in, and a loop matches the letter entered by the user, and prints out the index+1 of the array, i.e the position of that letter in the alphabet. Andy
You could also use the ASCII (or ANSI) equivalent. I'm not familiar enough with C/C++ to write the code, but VB has an ASC function that returns the decimal equivalent of a character. Then you'd need two checks since upper & lower case characters fall into different ranges. (The below assumes you've already checked to ensure x is a valid letter of the alphabet.) If ASC(x) < 97 then ' lowercase a = Char(97) so x is uppercase, which starts at Char(41) y = ASC(x) - 40 Else y = ASC(x) - 96 End If
andythepandy said: Hi, you could use the following method: Code: #include <iostream> using namespace std; char letters[27] = "abcdefghijklmnopqrstuvwxyz"; void main() { while(true) { cout << "Enter a letter: "; char letterIn; cin >> letterIn; for(int i = 1; i <= 26; i++) { if(letterIn == letters[i-1]) { cout << "Letter #" << i << "\r\n\r\n"; break; } } } } There I just have a char array with all the letters of the alphabet in, and a loop matches the letter entered by the user, and prints out the index+1 of the array, i.e the position of that letter in the alphabet. Andy Click to expand...
calvin-c said: You could also use the ASCII (or ANSI) equivalent. I'm not familiar enough with C/C++ to write the code, but VB has an ASC function that returns the decimal equivalent of a character. Then you'd need two checks since upper & lower case characters fall into different ranges. (The below assumes you've already checked to ensure x is a valid letter of the alphabet.) If ASC(x) < 97 then ' lowercase a = Char(97) so x is uppercase, which starts at Char(41) y = ASC(x) - 40 Else y = ASC(x) - 96 End If Click to expand...
You don't need to call any functions to use calvin-c's approach: Code: char letterIn; int letterNum = 0; cout << "Enter a letter> "; cin >> letterIn; if(letterIn >= 'A' && letterIn <= 'Z') { letterNum = letterIn - 'A' + 1; } else if(letterIn >= 'a' && letterIn <= 'z') { letterNum = letterIn - 'a' + 1; } if(letterNum != 0) { cout << "Letter # " << letterNum; } else { cout << "Not a letter."; }
JimmySeal said: You don't need to call any functions to use calvin-c's approach: Code: char letterIn; int letterNum = 0; cout << "Enter a letter> "; cin >> letterIn; if(letterIn >= 'A' && letterIn <= 'Z') { letterNum = letterIn - 'A' + 1; } else if(letterIn > 'a' && letterIn <= 'z') { letterNum = letterIn - 'a' + 1; } if(letterNum != 0) { cout << "Letter # " << letterNum; } else { cout << "Not a letter."; } Click to expand...
Well spotted. Fixed it!
Umm, you realize that you actually are calling the function, right? All you've done is make the call implicit rather than explicit. There are arguments either way, of course, but I hate to see people fooling themselves by thinking that if they don't explicitly code it then it isn't happening.
calvin-c said: Umm, you realize that you actually are calling the function, right? All you've done is make the call implicit rather than explicit. There are arguments either way, of course, but I hate to see people fooling themselves by thinking that if they don't explicitly code it then it isn't happening. Click to expand...
letterIn - 'a' + 1 won't work unless you convert the character 'a' into its numeric equivalent-which requires calling a function. I'll note that the same function occurs when the string is assigned to a variable since it's stored in memory as its numeric equivalent-but that's what I mean about people fooling themselves into thinking that they aren't calling functions just because they don't call them explicitly.
doctorzeus said: I refined it to create this version: Code: omitted} I do a lot of C++ quizes where turning letters into their assigned number comes up VERY frequently so it can be used in various circumstances, just created a header file with it in so I can use it in the future and call the function which should be usefull Thanks Doctorzeus Click to expand...
calvin-c said: letterIn - 'a' + 1 won't work unless you convert the character 'a' into its numeric equivalent-which requires calling a function. I'll note that the same function occurs when the string is assigned to a variable since it's stored in memory as its numeric equivalent-but that's what I mean about people fooling themselves into thinking that they aren't calling functions just because they don't call them explicitly. Click to expand...
doctorzeus said: Looks like it will take up less resources and easier to write although calling functions like this in c/c++ is usually a lot more difficult Click to expand...
JimmySeal said: When I said that the code didn't need any functions, I was referring to doctorzeus' comment: From that perspective, the code does, for all intents and purposes, not have any function calls so your nitpicking is quite irrelevant. Aside from that, your comment isn't even correct. In C, and C-based languages, char s are numeric types that get special treatment in certain situations. The 'a' token would be converted to its equivalent numeric value at compile time, so there is no implicit function call at runtime to convert it to a number. And unlike VB, C/C++ don't even have functions for converting between chars and ints, though cast operators can be used to convert between them. When I said your approach didn't need any functions, I was actually defending your comment against doctorzeus' notion that it was "a lot more difficult." I don't know what you're trying to get at, but I do suggest that you have some idea what you're talking about before making any more obnozious, condescending remarks. Click to expand...
JimmySeal said: Not that it will impact your programs' performance terribly, but using a for loop to do this is quite silly and it doesn't even address capital letters. I would suggest using the approach that Calvin-c suggested and I demonstrated, as it is a lot more straightforward. Click to expand...
User logic or logic circuits? Note that Jimmy's and my approaches differ mostly in how easily a programmer can understand what they do. I learned my programming in strongly-typed languages with little implicit conversion capability-that's why I prefer explicit conversions over implicit. These days, with object-oriented programming, it doesn't make all that much difference. Put the function into a library (or header file) and the programmer that uses it really doesn't need to know how it works, only what it does.
calvin-c said: User logic or logic circuits? Note that Jimmy's and my approaches differ mostly in how easily a programmer can understand what they do. I learned my programming in strongly-typed languages with little implicit conversion capability-that's why I prefer explicit conversions over implicit. These days, with object-oriented programming, it doesn't make all that much difference. Put the function into a library (or header file) and the programmer that uses it really doesn't need to know how it works, only what it does. Click to expand...
doctorzeus said: I meant functions in the included library headers...you know like writeprocessmemory() which took me a while to get my head round if you know what I mean..I know that I'm already calling a function Clearly I misspoke, Doctorzeus Click to expand...
Heated internal dispute? Not on my part.
doctorzeus said: Hey, Question-what would be the easiest way to code a program in c/c++ that would output a number according to its neumeric postition in the alphabet without a lot of nested if statements e.g: Thanks Doctorzeus Click to expand...
- ?
- 861.3K members
Top Contributors this Month

- C++ Tutorial
- Java Tutorial
- Python Tutorial
- HTML Tutorial
- CSS Tutorial
- C Introduction
- C Data Types and Modifiers
- C Naming Conventions
- C Integer Variable
- C Float and Double Variables
- C Character Variable
- C Constant Variable
- C Typecasting
- C Operators
- C Assignment Operators
- C Arithmetic Operators
- C Relational Operators
- C Logical Operators
- C Operator Precedence
- C Environment Setup
- C Program Basic Structure
- C printf() Function
- C scanf() Function
- C Decision Making
- C if Statement
- C if else Statement
- C if else if Statement
- C Conditional Operator
- C switch case Statement
- C Mathematical Functions
- C while Loop
- C do while Loop
- C break and continue
- C One Dimensional Array
- C Two Dimensional Array
- C String Methods
- C Bubble Sort
- C Selection Sort
- C Insertion Sort
- C Linear Search
- C Binary Search
- C Dynamic Memory Allocation
- C Structure
- C Recursive Function
- C Circular Queue
- C Double Ended Queue
- C Singly Linked List
- C Doubly Linked List
- C Stack using Linked List
- C Queue using Linked List
- C Text File Handling
- C Binary File Handling
How to Declare and Use Character Variables in C Programming
C basic concepts.
In this lesson, we will discover how to declare and use character variables in C programming with this practical guide. Get hands-on with examples and take a quiz to reinforce your understanding.
What is Character Variable
In C programming, a character variable can hold a single character enclosed within single quotes. To declare a variable of this type, we use the keyword char , which is pronounced as kar . With a char variable, we can store any character, including letters, numbers, and symbols.
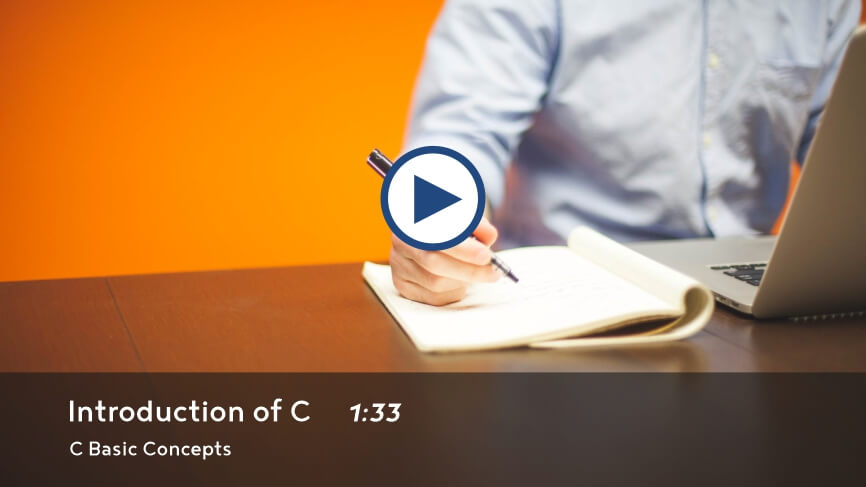
Syntax of Declaring Character Variable in C
Here char is used for declaring Character data type and variable_name is the name of variable (you can use any name of your choice for example: a, b, c, alpha, etc.) and ; is used for line terminator (end of line).
Now let's see some examples for more understanding.
Declare a character variable x .
Declare 3 character variables x , y , and z to assign 3 different characters in it.
Note: A character can be anything, it can be an alphabet or digit or any other symbol, but it must be single in quantity.
Declare a character variable x and assign the character '$' and change it value to @ in the next line.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
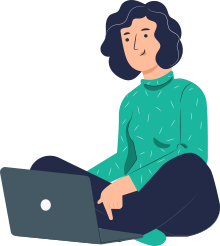
For over a decade, Dremendo has been recognized for providing quality education. We proudly introduce our newly open online learning platform, which offers free access to popular computer courses.
Our Courses
News updates.
- Refund and Cancellation
- Privacy Policy
C String – How to Declare Strings in the C Programming Language
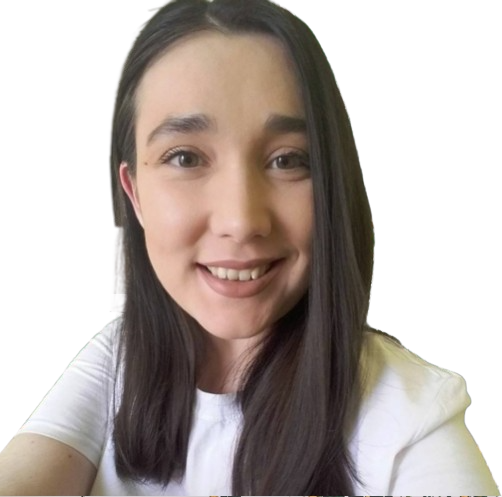
Computers store and process all kinds of data.
Strings are just one of the many forms in which information is presented and gets processed by computers.
Strings in the C programming language work differently than in other modern programming languages.
In this article, you'll learn how to declare strings in C.
Before doing so, you'll go through a basic overview of what data types, variables, and arrays are in C. This way, you'll understand how these are all connected to one another when it comes to working with strings in C.
Knowing the basics of those concepts will then help you better understand how to declare and work with strings in C.
Let's get started!
Data types in C
C has a few built-in data types.
They are int , short , long , float , double , long double and char .
As you see, there is no built-in string or str (short for string) data type.
The char data type in C
From those types you just saw, the only way to use and present characters in C is by using the char data type.
Using char , you are able to to represent a single character – out of the 256 that your computer recognises. It is most commonly used to represent the characters from the ASCII chart.
The single characters are surrounded by single quotation marks .
The examples below are all char s – even a number surrounded by single quoation marks and a single space is a char in C:
Every single letter, symbol, number and space surrounded by single quotation marks is a single piece of character data in C.
What if you want to present more than one single character?
The following is not a valid char – despite being surrounded by single quotation marks. This is because it doesn't include only a single character inside the single quotation marks:
'freeCodeCamp is awesome'
When many single characters are strung together in a group, like the sentence you see above, a string is created. In that case, when you are using strings, instead of single quotation marks you should only use double quotation marks.
"freeCodeCamp is awesome"
How to declare variables in C
So far you've seen how text is presented in C.
What happens, though, if you want to store text somewhere? After all, computers are really good at saving information to memory for later retrieval and use.
The way you store data in C, and in most programming languages, is in variables.
Essentially, you can think of variables as boxes that hold a value which can change throughout the life of a program. Variables allocate space in the computer's memory and let C know that you want some space reserved.
C is a statically typed language, meaning that when you create a variable you have to specify what data type that variable will be.
There are many different variable types in C, since there are many different kinds of data.
Every variable has an associated data type.
When you create a variable, you first mention the type of the variable (wether it will hold integer, float, char or any other data values), its name, and then optionally, assign it a value:
Be careful not to mix data types when working with variables in C, as that will cause errors.
For intance, if you try to change the example from above to use double quotation marks (remember that chars only use single quotation marks), you'll get an error when you compile the code:
As mentioned earlier on, C doesn't have a built-in string data type. That also means that C doesn't have string variables!
How to create arrays in C
An array is essentially a variable that stores multiple values. It's a collection of many items of the same type.
As with regular variables, there are many different types of arrays because arrays can hold only items of the same data type. There are arrays that hold only int s, only float s, and so on.
This is how you define an array of ints s for example:
First you specify the data type of the items the array will hold. Then you give it a name and immediately after the name you also include a pair of square brackets with an integer. The integer number speficies the length of the array.
In the example above, the array can hold 3 values.
After defining the array, you can assign values individually, with square bracket notation, using indexing. Indexing in C (and most programming languages) starts at 0 .
You reference and fetch an item from an array by using the name of the array and the item's index in square brackets, like so:
What are character arrays in C?
So, how does everything mentioned so far fit together, and what does it have to do with initializing strings in C and saving them to memory?
Well, strings in C are actually a type of array – specifically, they are a character array . Strings are a collection of char values.
How strings work in C
In C, all strings end in a 0 . That 0 lets C know where a string ends.
That string-terminating zero is called a string terminator . You may also see the term null zero used for this, which has the same meaning.
Don't confuse this final zero with the numeric integer 0 or even the character '0' - they are not the same thing.
The string terminator is added automatically at the end of each string in C. But it is not visible to us – it's just always there.
The string terminator is represented like this: '\0' . What sets it apart from the character '0' is the backslash it has.
When working with strings in C, it's helpful to picture them always ending in null zero and having that extra byte at the end.
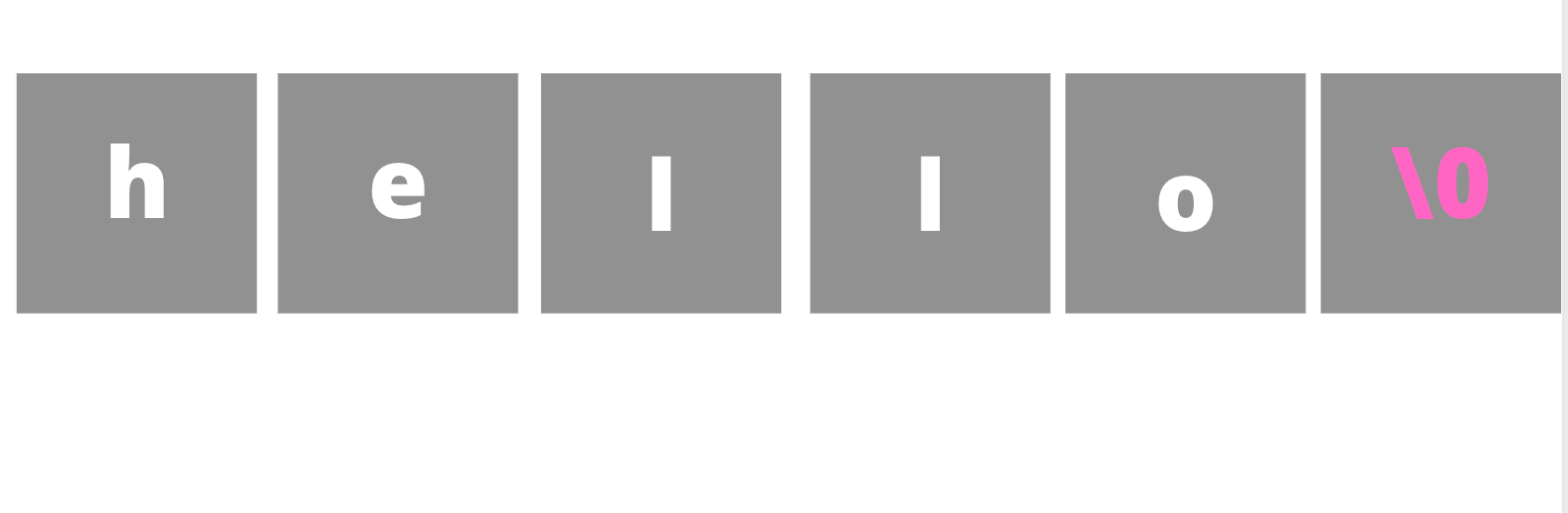
Each character takes up one byte in memory.
The string "hello" , in the picture above, takes up 6 bytes .
"Hello" has five letters, each one taking up 1 byte of space, and then the null zero takes up one byte also.
The length of strings in C
The length of a string in C is just the number of characters in a word, without including the string terminator (despite it always being used to terminate strings).
The string terminator is not accounted for when you want to find the length of a string.
For example, the string freeCodeCamp has a length of 12 characters.
But when counting the length of a string, you must always count any blank spaces too.
For example, the string I code has a length of 6 characters. I is 1 character, code has 4 characters, and then there is 1 blank space.
So the length of a string is not the same number as the number of bytes that it has and the amount of memory space it takes up.
How to create character arrays and initialize strings in C
The first step is to use the char data type. This lets C know that you want to create an array that will hold characters.
Then you give the array a name, and immediatelly after that you include a pair of opening and closing square brackets.
Inside the square brackets you'll include an integer. This integer will be the largest number of characters you want your string to be including the string terminator.
You can initialise a string one character at a time like so:
But this is quite time-consuming. Instead, when you first define the character array, you have the option to assign it a value directly using a string literal in double quotes:
If you want, istead of including the number in the square brackets, you can only assign the character array a value.
It works exactly the same as the example above. It will count the number of characters in the value you provide and automatically add the null zero character at the end:
Remember, you always need to reserve enough space for the longest string you want to include plus the string terminator.
If you want more room, need more memory, and plan on changing the value later on, include a larger number in the square brackets:
How to change the contents of a character array
So, you know how to initialize strings in C. What if you want to change that string though?
You cannot simply use the assignment operator ( = ) and assign it a new value. You can only do that when you first define the character array.
As seen earlier on, the way to access an item from an array is by referencing the array's name and the item's index number.
So to change a string, you can change each character individually, one by one:
That method is quite cumbersome, time-consuming, and error-prone, though. It definitely is not the preferred way.
You can instead use the strcpy() function, which stands for string copy .
To use this function, you have to include the #include <string.h> line after the #include <stdio.h> line at the top of your file.
The <string.h> file offers the strcpy() function.
When using strcpy() , you first include the name of the character array and then the new value you want to assign. The strcpy() function automatically add the string terminator on the new string that is created:
And there you have it. Now you know how to declare strings in C.
To summarize:
- C does not have a built-in string function.
- To work with strings, you have to use character arrays.
- When creating character arrays, leave enough space for the longest string you'll want to store plus account for the string terminator that is included at the end of each string in C.
- Define the array and then assign each individual character element one at a time.
- OR define the array and initialize a value at the same time.
- When changing the value of the string, you can use the strcpy() function after you've included the <string.h> header file.
If you want to learn more about C, I've written a guide for beginners taking their first steps in the language.
It is based on the first couple of weeks of CS50's Introduction to Computer Science course and I explain some fundamental concepts and go over how the language works at a high level.
You can also watch the C Programming Tutorial for Beginners on freeCodeCamp's YouTube channel.
Thanks for reading and happy learning :)
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Search anything:
Working with character (char) in C
Software engineering c programming.
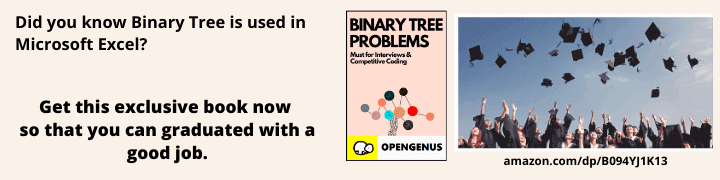
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
C uses char type to store characters and letters. However, the char type is integer type because underneath C stores integer numbers instead of characters.In C, char values are stored in 1 byte in memory,and value range from -128 to 127 or 0 to 255. In order to represent characters, the computer has to map each integer with a corresponding character using a numerical code. The most common numerical code is ASCII, which stands for American Standard Code for Information Interchange.
How to declare characters?
To declare a character in C, the syntax:
Complete Example in C:
C library functions for characters
The Standard C library #include <ctype.h> has functions you can use for manipulating and testing character values:
How to convert character to lower case?
- int islower(ch)
Returns value different from zero (i.e., true) if indeed c is a lowercase alphabetic letter. Zero (i.e., false) otherwise.
How to convert character to upper case?
- int isupper(ch)
A value different from zero (i.e., true) if indeed c is an uppercase alphabetic letter. Zero (i.e., false) otherwise.
Check if character is an alphabet?
- isalpha(ch)
Returns value different from zero (i.e., true) if indeed c is an alphabetic letter. Zero (i.e., false) otherwise.
Check if character is a digit
- int isdigit(ch);
Returns value different from zero (i.e., true) if indeed c is a decimal digit. Zero (i.e., false) otherwise.
Check if character is a digit or alphabet
- int isalnum(ch);
Returns value different from zero (i.e., true) if indeed c is either a digit or a letter. Zero (i.e., false) otherwise.
Check if character is a punctuation
- int ispunct(ch)
Returns value different from zero (i.e., true) if indeed c is a punctuation character. Zero (i.e., false) otherwise.
Check if character is a space
- int isspace(ch)
Retuns value different from zero (i.e., true) if indeed c is a white-space character. Zero (i.e., false) otherwise.
- char tolower(ch) & char toupper(ch)
The value of the character is checked other if the vlaue is lower or upper case otherwise it is change and value is returned as an int value that can be implicitly casted to char.
Find size of character using Sizeof()?
To get the exact size of a type or a variable on a particular platform, you can use the sizeof operator. The expressions sizeof(type) yields the storage size of the object or type in bytes.
In the below example the size of char is 1 byte, but the type of a character constant like 'a' is actually an int, with size of 4.
- All the function in Ctype work under constant time
What are the different characters supported?
The characters supported by a computing system depends on the encoding supported by the system. Different encoding supports different character ranges.
Different encoding are:
ASCII encoding has most characters in English while UTF has characters from different languages.
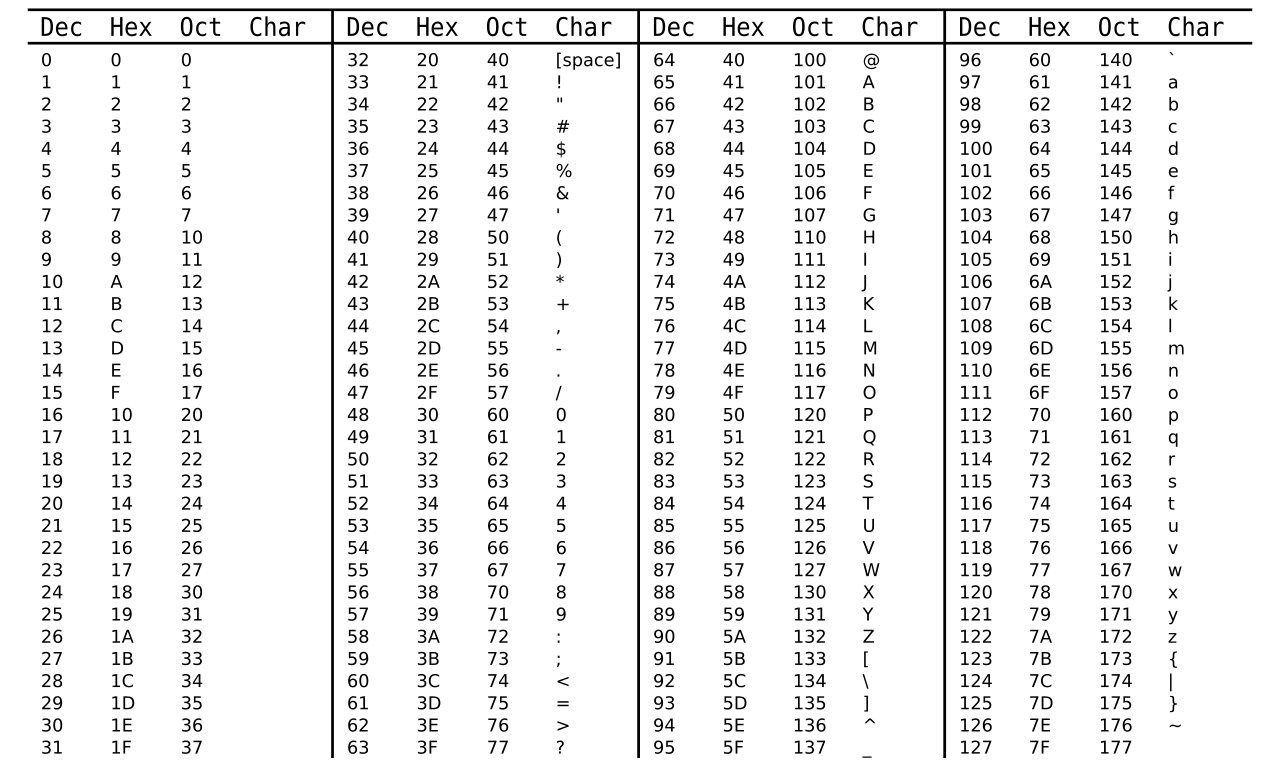
Consider the following C++ code:
What will be the output of the above code?
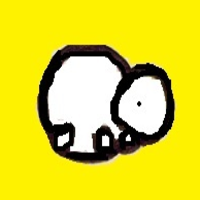
C Functions
C structures, c variables.
Variables are containers for storing data values, like numbers and characters.
In C, there are different types of variables (defined with different keywords), for example:
- int - stores integers (whole numbers), without decimals, such as 123 or -123
- float - stores floating point numbers, with decimals, such as 19.99 or -19.99
- char - stores single characters, such as 'a' or 'B' . Characters are surrounded by single quotes
Declaring (Creating) Variables
To create a variable, specify the type and assign it a value :
Where type is one of C types (such as int ), and variableName is the name of the variable (such as x or myName ). The equal sign is used to assign a value to the variable.
So, to create a variable that should store a number , look at the following example:
Create a variable called myNum of type int and assign the value 15 to it:
You can also declare a variable without assigning the value, and assign the value later:
Output Variables
You learned from the output chapter that you can output values/print text with the printf() function:
In many other programming languages (like Python , Java , and C++ ), you would normally use a print function to display the value of a variable. However, this is not possible in C:
To output variables in C, you must get familiar with something called " format specifiers ", which you will learn about in the next chapter.
C Exercises
Test yourself with exercises.
Create a variable named myNum and assign the value 50 to it.
Start the Exercise

COLOR PICKER

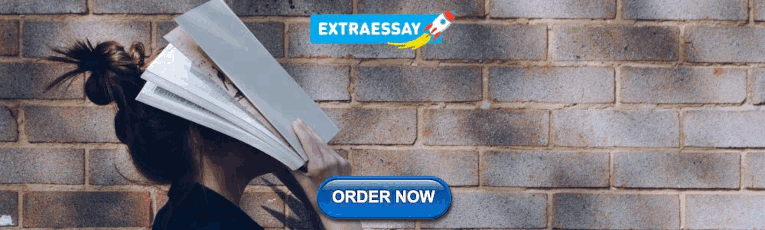
Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Contribute to the Microsoft 365 and Office forum! Click here to learn more 💡
April 9, 2024
Contribute to the Microsoft 365 and Office forum!
Click here to learn more 💡
Excel Forum Top Contributors: HansV MVP - Ashish Mathur - Andreas Killer - Jim_ Gordon - Riny_van_Eekelen ✅
May 10, 2024
Excel Forum Top Contributors:
HansV MVP - Ashish Mathur - Andreas Killer - Jim_ Gordon - Riny_van_Eekelen ✅
- Search the community and support articles
- Microsoft 365 and Office
- Search Community member
Ask a new question
How do I assign a numerical value to a letter that can be used in a formula?
Report abuse
Reported content has been submitted
Replies (3)
- Article Author
3 people found this reply helpful
Was this reply helpful? Yes No
Sorry this didn't help.
Great! Thanks for your feedback.
How satisfied are you with this reply?
Thanks for your feedback, it helps us improve the site.
Thanks for your feedback.
try and this sample..
assuming that you assign number 10 to A, 50 to B and 1 to H
(say that in cell A1 is data validation list... A,B,H)
in cell D1 write:
=LOOKUP($A$1,{"A","B","H"},{10,50,1})*$B$2
always, A, B, H by Ascending order
x1, x2, x10
x1, x10, x2
=LOOKUP($A$1,{"x1","x10","x2"},{10,50,1})*$B$2
Question Info
- Norsk Bokmål
- Ελληνικά
- Русский
- עברית
- العربية
- ไทย
- 한국어
- 中文(简体)
- 中文(繁體)
- 日本語
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
C++ Data Types
- C++ Type Modifiers
- C++ Constants
C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
- C++ if, if...else and Nested if...else
- C++ for Loop
- C++ while and do...while Loop
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
- C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
- C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
- C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
- C++ Classes and Objects
- C++ Constructors
- C++ Constructor Overloading
- C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
- C++ Inheritance
- C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
- C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
- C++ Standard Template Library
- C++ STL Containers
- C++ std::array
- C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
- C++ Iterators
- C++ Algorithm
- C++ Functor
Additional Topics
- C++ Exceptions Handling
- C++ File Handling
- C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
- C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
C++ istream
- C++ ostream
C++ Tutorials
- Find ASCII Value of a Character
- C++ tolower()
- C++ toupper()
- C++ ispunct()
- C++ isspace()
In C++, the char keyword is used to declare character type variables. A character variable can store only a single character.
Example 1: Printing a char variable
In the example above, we have declared a character type variable named ch . We then assigned the character h to it.
Note: In C and C++, a character should be inside single quotation marks. If we use, double quotation marks, it's a string.
- ASCII Value
In C and C++, an integer (ASCII value) is stored in char variables rather than the character itself. For example, if we assign 'h' to a char variable, 104 is stored in the variable rather than the character itself. It's because the ASCII value of 'h' is 104.
Here is a table showing the ASCII values of characters A , Z , a , z and 5 .
To learn more about ASCII code , visit the ASCII Chart .
Example 2: Get ASCII Value of a Character
We can get the corresponding ASCII value of a character by using int() when we print it.
We can assign an ASCII value (from 0 to 127 ) to the char variable rather than the character itself.
Example 3: Print Character Using ASCII Value
Note: If we assign '5' (quotation marks) to a char variable, we are storing 53 (its ASCII value). However, if we assign 5 (without quotation marks) to a char variable, we are storing the ASCII value 5 .
- C++ Escape Sequences
Some characters have special meaning in C++, such as single quote ' , double quote " , backslash \ and so on. We cannot use these characters directly in our program. For example,
Here, we are trying to store a single quote character ' in a variable. But this code shows a compilation error.
So how can we use those special characters?
To solve this issue, C++ provides special codes known as escape sequences. Now with the help of escape sequences, we can write those special characters as they are. For example,
Here, \' is an escape sequence that allows us to store a single quote in the variable.
The table below lists escape sequences of C++.
Example 4: Using C++ Escape Sequences
In the above program, we have used two escape sequences: the horizontal tab \t and the new line \n .
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
C++ Tutorial
C++ Strings
- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- assign letters to numbers
assign letters to numbers

- Standard Template Library
- STL Priority Queue
- STL Interview Questions
- STL Cheatsheet
- C++ Templates
- C++ Functors
- C++ Iterators
- Vector in C++ STL
- Initialize a vector in C++ (7 different ways)
Commonly Used Methods
- vector::begin() and vector::end() in C++ STL
- vector::empty() and vector::size() in C++ STL
- vector::operator= and vector::operator[ ] in C++ STL
- vector::front() and vector::back() in C++ STL
- vector::push_back() and vector::pop_back() in C++ STL
- vector insert() Function in C++ STL
- vector emplace() function in C++ STL
vector :: assign() in C++ STL
- vector erase() and clear() in C++
Other Member Methods
- vector max_size() function in C++ STL
- vector capacity() function in C++ STL
- vector rbegin() and rend() function in C++ STL
- vector :: cbegin() and vector :: cend() in C++ STL
- vector::crend() & vector::crbegin() with example
- vector : : resize() in C++ STL
- vector shrink_to_fit() function in C++ STL
- Using std::vector::reserve whenever possible
- vector data() function in C++ STL
- 2D Vector In C++ With User Defined Size
- Passing Vector to a Function in C++
- How does a vector work in C++?
- How to implement our own Vector Class in C++?
- Advantages of vector over array in C++
Common Vector Programs
- Sorting a vector in C++
- How to reverse a Vector using STL in C++?
- How to find the minimum and maximum element of a Vector using STL in C++?
- How to find index of a given element in a Vector in C++
vector:: assign() is an STL in C++ which assigns new values to the vector elements by replacing old ones. It can also modify the size of the vector if necessary.
The syntax for assigning constant values:
Program 1: The program below shows how to assign constant values to a vector
The syntax for assigning values from an array or list:
Program 2: The program below shows how to assign values from an array or list
The syntax for modifying values from a vector
Program 3: The program below shows how to modify the vector
Time Complexity – Linear O(N)
Syntax for assigning values with initializer list:
Program 4:The program below shows how to assign a vector with an initializer list.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
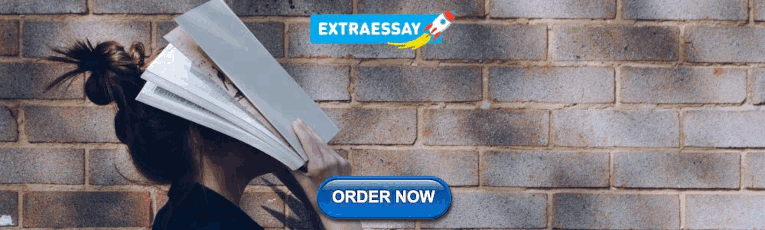
IMAGES
VIDEO
COMMENTS
Here's the code. char letter = 'E'; // could be any upper or lower case letter. char str[2] = { letter }; // make a string out of the letter. int num = strtol( str, NULL, 36 ) - 10; // convert the letter to a number. The reason this works can be found in the man page for strtol which states:
You can get the number associated with c by doing: int n = static_cast<int>(c); Then, add some offset to 'n': n += 10; ...and cast it back to a char: c = static_cast<char>(n); Note: The above assumes that characters are consecutive, i.e. the number corresponding to 'a' is equal to the one corresponding to 'z' minus the amount of letters between ...
Solved: Assign Numbers to Letters. ... Question-what would be the easiest way to code a program in c/c++ that would output a number according to its neumeric postition in the alphabet without a lot of nested if statements e.g: #include <iostream> void main() {char letterin == 'A';
We can initialize a C string in 4 different ways which are as follows: 1. Assigning a String Literal without Size. String literals can be assigned without size. Here, the name of the string str acts as a pointer because it is an array. char str[] = "GeeksforGeeks"; 2. Assigning a String Literal with a Predefined Size.
Syntax of Declaring Character Variable in C. char variable_name; Here char is used for declaring Character data type and variable_name is the name of variable (you can use any name of your choice for example: a, b, c, alpha, etc.) and ; is used for line terminator (end of line).
A variable name can only have letters (both uppercase and lowercase letters), digits and underscore. ... 2.5 and 'c' are literals. Why? You cannot assign different values to these terms. 1. Integers ... (associated with numbers) without any fractional or exponential part. There are three types of integer literals in C programming: decimal (base 10)
"Hello" has five letters, each one taking up 1 byte of space, and then the null zero takes up one byte also. The length of strings in C. The length of a string in C is just the number of characters in a word, without including the string terminator (despite it always being used to terminate strings).
In C programming, a string is a sequence of characters terminated with a null character \0. For example: char c[] = "c string"; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default. Memory Diagram.
First of all, you don't need to hardcode the letters and their positions in the alphabet - you can use the string.ascii_lowercase.. Also, you don't have to call list() on a new_text - you can just iterate over it character by character.. Then, what if we would construct a mapping between letters and letter indexes in the alphabet (with the help of enumerate()).
Reading time: 30 minutes. C uses char type to store characters and letters. However, the char type is integer type because underneath C stores integer numbers instead of characters.In C, char values are stored in 1 byte in memory,and value range from -128 to 127 or 0 to 255.
In character arithmetic character converts into an integer value to perform the task. For this ASCII value is used. It is used to perform actions on the strings. To understand better let's take an example. Example 1. C. // C program to demonstrate character arithmetic. #include <stdio.h>. int main()
Reading a Character in C. Problem Statement#1: Write a C program to read a single character as input in C. Syntax-. scanf("%c", &charVariable); Approach-. scanf () needs to know the memory location of a variable in order to store the input from the user. So, the ampersand will be used in front of the variable (here ch) to know the address of a ...
Variables are containers for storing data values, like numbers and characters. In C, there are different types of variables (defined with different keywords), for example:. int - stores integers (whole numbers), without decimals, such as 123 or -123; float - stores floating point numbers, with decimals, such as 19.99 or -19.99; char - stores single characters, such as 'a' or 'B'.
I've assign the number '1' to the letter 'H'. When using the the formula in another cell though, I keep coming up with an error, that as far as I can tell, is because of the letter, even though the letter has a numerical value assigned. I'll attach a screen shot. I NEED to have the letter showing, not the number that it's assigned to. Please help.
I need a way to assign numbers to letters in C++, for example, '$' would represent the number 1. I obviously need to be able to obtain the number from the character with something like a function, e.g. getNumFromChar('$') would return 1 and getNumFromChar('#') would return 2.
ASCII Value. In C and C++, an integer (ASCII value) is stored in char variables rather than the character itself. For example, if we assign 'h' to a char variable, 104 is stored in the variable rather than the character itself. It's because the ASCII value of 'h' is 104.. Here is a table showing the ASCII values of characters A, Z, a, z and 5.
send + more = money. then I will extract the unique characters from this string: s, e, n, d, m, o, r, y. I have programmed up to this point and it works fine. Now I want to give each letter a unique number. Next, I will use next_permutation () to give me all the possible permutations of those numbers.
Well, the best advice for this problem is to create a dictionary associating letter to numbers: d = {'A':1, 'B':2 ... and changing your if else nightmare with this: tempSum += d[letter] Now, by looking detailed at your example and the organization, it seems to be that the value to be summed is the position of upper case letters in the alphabet ...
The member function assign () is used for the assignments, it assigns a new value to the string, replacing its current contents. Syntax 1: Assign the value of string str. string& string::assign (const string& str) str : is the string to be assigned. Returns : *this. CPP.
The reason is, arrays are not initiated by a default value (like 0) in C, so when you input a string which does not cover whole the array, rest of elements don't have any default value and they have some garbage value from the memory. You can initiate the word array like this: for(i = 0; i < 10; i++) word1[i] = 0;
vector:: assign () is an STL in C++ which assigns new values to the vector elements by replacing old ones. It can also modify the size of the vector if necessary. The syntax for assigning constant values: vectorname.assign(int size, int value) Parameters: size - number of values to be assigned.