How to Create Array of Specific Length in JavaScript
- JavaScript Howtos
- How to Create Array of Specific Length …
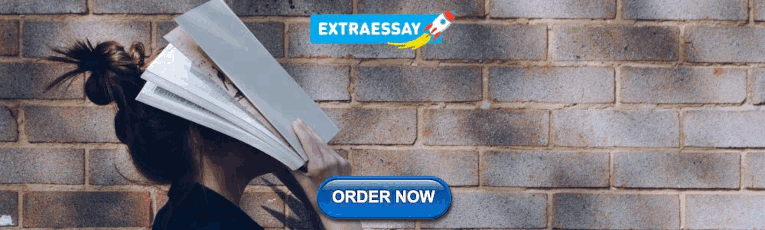
Create an Array of Length Using Array() Constructor in JavaScript
Create an array of lengths using the apply() method in javascript, create an array of lengths using the map() method in javascript, creates an array of length using the fill() method in javascript.
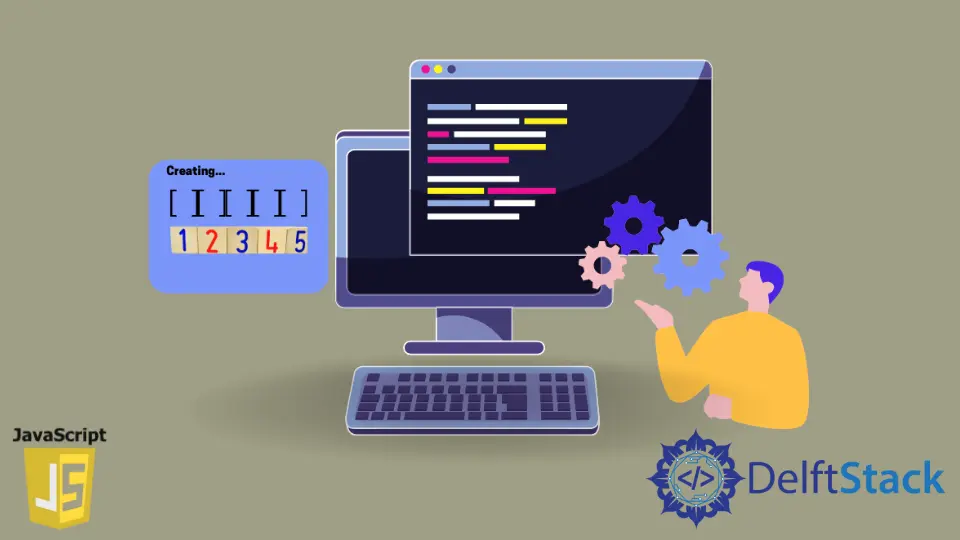
In JavaScript, you might need to create or declare an array of specific lengths and insert values you want inside the array. It can be achieved using different ways.
Below are some of the most ways to create an array of specific lengths in JavaScript. Now only use this in case you think there is a need for this because most of the time, there is no need to create or declare an array of specific lengths; you can dynamically make an array and use it.
The first way of creating an empty array of specific lengths is by using the Array() constructor and passing an integer as an argument to it. Since we are calling a constructor, we will be using the new keyword.
This way of creating an array of specific lengths is not recommended if you are using JSlint, as it might cause issues. Since this way is ambiguous and it does not just create an array of a particular length, it can also be used to do other things like creating an array containing a value as follows.
The Array constructor provides a method called apply() . Using this apply() method, you can provide arguments to a method in the form of an array. The apply() method takes two arguments, the first is the reference to this argument, and the second is the array.
To create an empty array of a specific length, let’s say 5 , we will pass null as the first argument, and the second is the array Array(5) . This will create an array of length 5 and initialize each element with an undefined value.
Alternatively, you can also do the following. Here, you can pass an array object as the second parameter and then inside it define the length of the array that you want to create, in this case, 5 .
Another way of creating an array of specific lengths is to use the map() method in JavaScript. Here, the Array(5) constructor will create an empty array of length 5. This is similar to what we saw previously. Then using the spread operator ... , we will spread every element of the array and enclose this inside a square bracket like this [...Array(5)] . It will create an array of length 5 where the value of each element will be undefine . Then to initialize this array, we will make use of the map() method.
Using the map() method, we will take every element inside the x variable and then add value zero to it. This will initialize all the elements of the array to zero.
The fill() method also works the same way as map() does; the only thing is that the fill() does not take any function as a parameter. It directly takes a value as an input. You can create an array of specific length Array(5) and then fill the array with some integer value, as shown below.
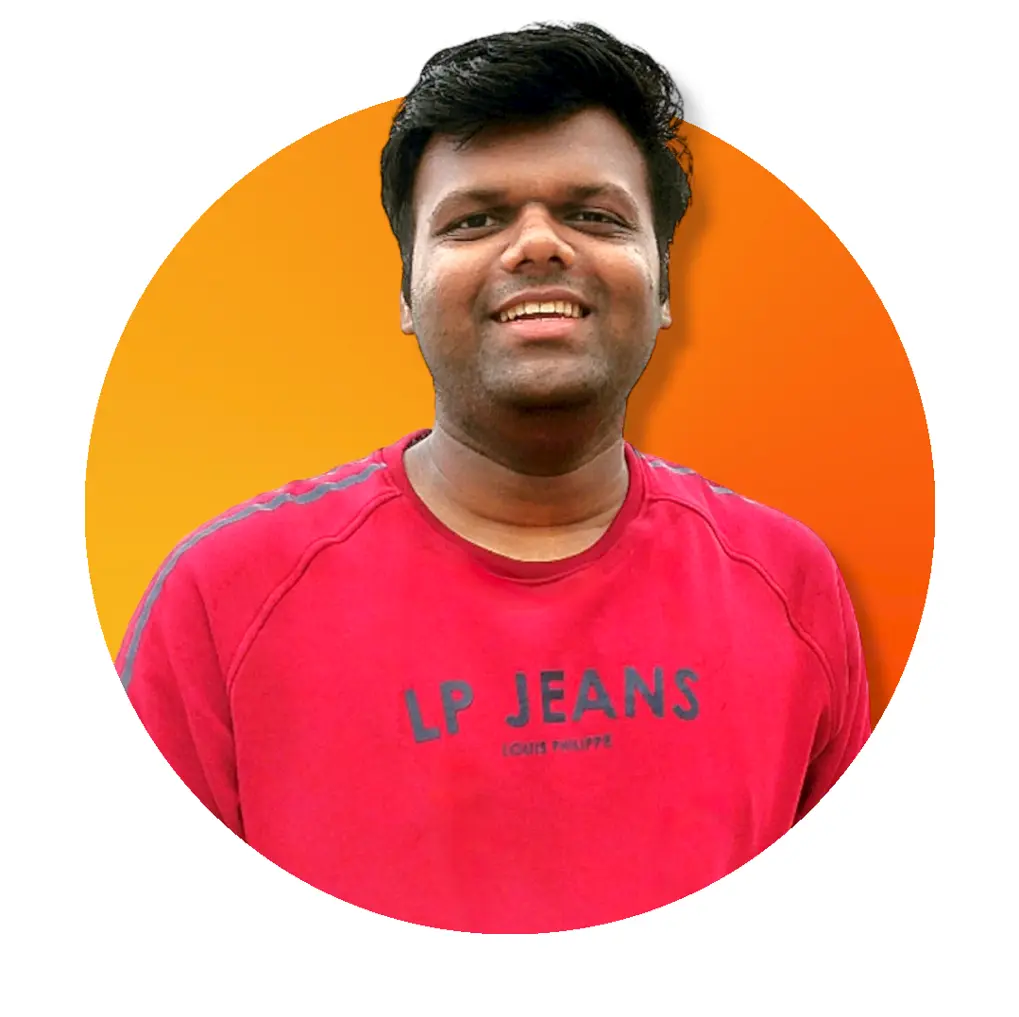
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript
- Read Tutorial
- Watch Guide Video
- Complete the Exercise
In this guide, we're going to start working through what arrays are in JavaScript and how we can work with them.
There are two main ways that you can create an array and we're going to use the first syntax which is essentially a generated syntax where we create a new array object. And so I'm going to store it in a variable called generatedArray and the syntax for this is new which is a special keyword and then array. Now there are a couple of ways to do this. Say that you want an array with three elements inside of it, we can pass it in just like this.
And this will create the array for us.
If I say generatedArray now you can see that we have 3 items. They're all undefined. But we do have a collection. We could even call generatedArray length and you can see that we have three items in this array. Now having 3 undefine items may not seem very helpful. So let's go and let's create a new array with some names so I can create some baseball player names here.
And now if I run
this generatedArray you can see that we have a collection of three individuals and three strings. So that is one way that you can generate an array. I don't use that way too often and the only time I'll usually use it is when I want to create an array and I don't know what the values are going to be, but I happen to know how many elements are going to be inside and then I use exactly what I did up here.
The more common way that I create an array is by creating what is called the array literal syntax . And so here is a VAR literal array. Obviously you could call it anything you want and here it is simply going to be using the brackets. And so I can put inside of these three items and run it. And now I have a literal array just like that.
Now I could obviously mix these. So those are using integers but if I wanted to I could put strings inside of it. So if I go literal array again I can reassign it to these values. And now if I call literal array it has Altuve, Correa, and Spring which is supposed to be Springer the baseball player.
I can also do just like before, I can call link that has that attribute associated with it so that those are the two most common ways.
Now I've shown you how you can have integers and I've shown you how you can have strings. But JavaScript is incredibly flexible and you can mix and match the elements in the data types inside of your arrays. So let's create a new one called mixed array. And with this mixed array you'll see we can combine anything that we want. For the most part. So I can say hi and put a integer. I can't even put another array. So I could but the ABC's is right here and this is going to be a nested array inside of it.
Make sure you separate each one with the comma and then from there I can even put in objects. Here I can put an object with a name, close the object out and the last one we're going to do may seem a little bit tricky but I can actually put a function in here as well. I could say function greeting and then inside of it console log 'hey there' and then also close it off obviously.
And we have our mixedArray, so that should show you could put literally anything you want. It's a collection of data but JavaScript is a much more flexible with what you can put inside of your arrays than many other languages. Many languages like C or Java in those ones you have to declare the type of elements that are going to be inside of an array and that's because they have very strict compiler requirements. whereas, the JavaScript engine is much more flexible and what it allows. So that's how you can create arrays.
Now let's talk about how we can actually get things out of the arrays. So let's start with our most basic one. If we go back to literal array here the syntax for getting items out is what's called the bracket syntax so you put two brackets right here. And then inside of it you call the index of the item that you want to pull out. Early on in this course I talked about indexes in computer science starting with 0 instead of 1. We discussed that when we're talking about how we could count the characters and grab characters from a string.
We can follow the same pattern when working with arrays so right here if we want Altuve, we can pass in zero and remember it's because arrays and indexes in general start with zero.
If I hit return, that pulls out Altuve and now I can use it however I want. So a very common pattern you'll see is to do something like this. I can say player name and then call the literalArray and this time let's go with the next item which is going to be 1. So I store it and now if I call player name you can see it's stored with Correa and that is very common kind of pattern that you will see in development.
Now that is helpful but you may still kind of be wondering when in the world am I going to use this? That's a very common question to ask especially if you are new to development. Arrays are incredibly handy for a number of scenarios. One of the most common that I use them for is with database queries. Usually when you're building an application and you make a query to a database or to an API when you receive that data back there has to be a standard representation for how that data is sent back to you. One of the most common is to have data sent as an array and you can then loop over that data show it on the screen. And so that's one of the reasons why it's so important to understand the way that arrays and collections work.
Now let's move down the list a little bit. So we talked about how we can grab a single element. Let's also talk about how we can work with some of those more complex ones.
Remember we have our mixedArray and if I open this up you can see all of the different things that we have inside it. In the zero index, we have "Hi" in the first index, we have the integer 1 then we have an array that has an array inside of it. Then we have a object with a key value pair of name and then Kristine and then we have a function called greeting.
So how exactly can we call some of these other items. Well we already talked about how we could call the first two. Those are pretty basic where we in a call just mixed array and do something like that with the bracket syntax with the next one it may seem like this would be more tricky when you have a nested array. So what we're going to do is pass in the index 2 and whenever you want to reference that that's one thing I love about the javascript console is it gives you a really nice reference point for your indexes and different things like that.
So here I want the array but let's say that I want the "C" in the array and I know it's at the second index, I can pass in a second set of brackets so whenever you have something nested like this then you can do your very first call your very first query and then use the bracket syntax again and chain these together. Now run this and it returns "C" and you could store that in a variable or whatever you want to do with it. When you're working with nested arrays.
Now let's talk about our object. We know it's in the index of 3, and because of that we're going to receive just a plain object back. So if I just say 3, this gives us an object back so don't let the end of the bracket syntax intimidate you because all you need to do is treat it like we've been treating objects this entire course. You can use the dot syntax and chain it together. Now you can have access to that name.
Now let's talk about the last one because this one I've seen trip people up a little bit. So we have our greeting and if you just call 4 that is only going to return the function greeting. But what if we actually want to call the function which is a more standard thing. Well it's going to be the exact same way that we had call a regular function. We've queried it and now we just put our nice parends at the end and it prints out 'hey there' and the function is executed.
Now go a little bit further if you want to do things like be able to have methods inside of objects that is a very common thing to do and you can have those include it and arrays. So one of the easiest ways of understanding the way arrays work in JavaScript is just think of them as a collection of all the regular items that you've been using this entire course. So there's nothing more special about them than that. They collect everything you can put things inside of it.
It's just a way of storing it, when you want to store multiple things inside of the same variable you can. But beyond that you can treat them exactly the same way as when they were just kind of one off items stored inside of variables. So the main thing to understand is how you can properly query each one of those items just like we walk through right here.
- Source code
devCamp does not support ancient browsers. Install a modern version for best experience.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Array.from()
Baseline widely available.
This feature is well established and works across many devices and browser versions. It’s been available across browsers since September 2015 .
- See full compatibility
- Report feedback
The Array.from() static method creates a new, shallow-copied Array instance from an iterable or array-like object.
An iterable or array-like object to convert to an array.
A function to call on every element of the array. If provided, every value to be added to the array is first passed through this function, and mapFn 's return value is added to the array instead. The function is called with the following arguments:
The current element being processed in the array.
The index of the current element being processed in the array.
Value to use as this when executing mapFn .
Return value
A new Array instance.
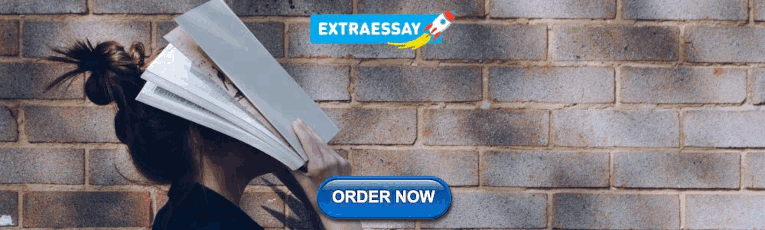
Description
Array.from() lets you create Array s from:
- iterable objects (objects such as Map and Set ); or, if the object is not iterable,
- array-like objects (objects with a length property and indexed elements).
To convert an ordinary object that's not iterable or array-like to an array (by enumerating its property keys, values, or both), use Object.keys() , Object.values() , or Object.entries() . To convert an async iterable to an array, use Array.fromAsync() .
Array.from() never creates a sparse array. If the arrayLike object is missing some index properties, they become undefined in the new array.
Array.from() has an optional parameter mapFn , which allows you to execute a function on each element of the array being created, similar to map() . More clearly, Array.from(obj, mapFn, thisArg) has the same result as Array.from(obj).map(mapFn, thisArg) , except that it does not create an intermediate array, and mapFn only receives two arguments ( element , index ) without the whole array, because the array is still under construction.
Note: This behavior is more important for typed arrays , since the intermediate array would necessarily have values truncated to fit into the appropriate type. Array.from() is implemented to have the same signature as TypedArray.from() .
The Array.from() method is a generic factory method. For example, if a subclass of Array inherits the from() method, the inherited from() method will return new instances of the subclass instead of Array instances. In fact, the this value can be any constructor function that accepts a single argument representing the length of the new array. When an iterable is passed as arrayLike , the constructor is called with no arguments; when an array-like object is passed, the constructor is called with the normalized length of the array-like object. The final length will be set again when iteration finishes. If the this value is not a constructor function, the plain Array constructor is used instead.
Array from a String
Array from a set, array from a map, array from a nodelist, array from an array-like object (arguments), using arrow functions and array.from(), sequence generator (range), calling from() on non-array constructors.
The from() method can be called on any constructor function that accepts a single argument representing the length of the new array.
When the this value is not a constructor, a plain Array object is returned.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Polyfill of Array.from in core-js
- Indexed collections guide
- Array.fromAsync()
- Array.prototype.map()
- TypedArray.from()
How to make a fixed length array type?
Basically I want to make a type that is just a fixed size array with the same type. Like in C i would do int[4] to make a array of 4 ints. Is there any way to do this with the luau type system? I already tried something like
but that doesnt’ work.
To my knowledge Roblox’s implementation of Luau doesn’t support the ability to create fixed-length arrays.
I dont actually want a fixed size array but just a way to make the typechecker only allow a certain array size
I’d say for you to index the length of the array with “false” statements. Then everytime you want to Index something inside it, you can check if the value at that index equals to false, meaning that the slot is available, if it returns nil then it means it’s out of the range
You can use table.create :
Here’s a function to verify if a table only has 4 elements that are all number values:
And to typecheck you can use the {number} typecheck that means “An array of numbers” although it wont typecheck if the array has exactly 4 elements in it.
yea i guess its not possible to do it with the typechecker then thanks for tryin tho
I never said it’s not possible, I just don’t know how to do it.

An official website of the United States government
Here’s how you know
Official websites use .gov A .gov website belongs to an official government organization in the United States.
Secure .gov websites use HTTPS A lock ( Lock A locked padlock ) or https:// means you’ve safely connected to the .gov website. Share sensitive information only on official, secure websites.
Biden-Harris Administration Invests in Clean Energy to Strengthen North Carolina Farms and Businesses as Part of Investing in America Agenda
Projects Funded by President Biden’s Inflation Reduction Act Will Lower Energy Costs and Create Revenue for Rural Business Owners and Farms
RALEIGH, North Carolina., March 28, 2024 – U.S. Department of Agriculture (USDA) Secretary Tom Vilsack today announced USDA is investing $1.3 million in renewable energy projects in North Carolina to lower energy costs, generate new income and create jobs for farmers, ranchers, agricultural producers and rural small businesses in the Tar Heel State. The projects announced today are being funded by President Biden’s Inflation Reduction Act, the nation’s largest-ever investment in combating the climate crisis, through the Rural Energy for America Program (REAP).
This funding advances the President’s Investing in America agenda to grow the nation’s economy from the middle out and bottom up by increasing competition in agricultural markets, lowering costs and expanding clean energy.
“Under the Biden-Harris Administration, USDA is committed to ensuring farmers, ranchers and small businesses are directly benefitting from both a clean energy economy and a strong U.S. supply chain,” North Carolina Rural Development State Director Reginald Speight said. “The investments announced today will expand access to renewable energy systems while creating good-paying jobs and saving people money that they can then invest back into their businesses and communities.”
Rural Clean Energy Production
Through the REAP program, USDA provides grants and loans to help ag producers and rural small business owners expand their use of wind, solar and other forms of clean energy and make energy efficiency improvements. These innovations help them increase their income, grow their businesses, address climate change and lower energy costs.
The REAP program is part of the President’s Justice40 Initiative , which set a goal to deliver 40% of the overall benefits of certain federal investments to disadvantaged communities that are marginalized by underinvestment and overburdened by pollution.
These investments will cut energy costs for farmers and ag producers that can instead be used to create jobs and new revenue streams for people in their communities.
In North Carolina today’s announcement will benefit 12 producers in 10 counties.
For example:
- In Alexander County Danny Millsaps will use a $69 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 93.5-kilowatt solar array. This poultry farming operation will realize $9,537 per year in savings, and will save 119 thousand kilowatt hours per year, which is save enough electricity to power eleven homes.
- In Alexander County Icenhour Poultry and Beef LLC will use a $154 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 179-kilowatt solar array. This agricultural producer will save 263 thousand kilowatt hours per year, which is enough electricity to power 25 homes.
- In Buncombe County Vargas Vineyard Ventures LLC will use a $63 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 22.1-kilowatt solar array. This project will save 27 thousand kilowatt hours per year.
- In Cherokee County Rare Bird Emporium LLC will use a $81 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 18.72 kilowatt-solar array which will be connected to a battery backup energy storage system to ensure the business never loses power. This project will save 25 thousand kilowatt hours per year, which is enough electricity to power two homes.
- In Clay County Incredible Storage Hayesville LLC will use a $100 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 46.41-kilowatt solar array which will be connected to a battery backup energy storage system to ensure the business never loses power. This project will save 61 thousand kilowatt hours per year, which is enough electricity to power five homes.
- In Forsyth County Heritage Harvest LTD will use a $16 thousand USDA REAP Renewable Energy and Efficiency Grant to make energy-efficiency improvements to their farm operations. Funds will be used to purchase and install improvements to their cooler and HVAC system. This project will save the store $919 per year and will save 25 thousand kilowatt hours per year, which is enough electricity to power two homes.
- In Guilford County Charles Gary Cobb will use a $82 thousand USDA REAP Renewable Energy and Efficiency Grant to make energy-efficiency improvements to his farming operations. Funds will be used to purchase and install a grain dryer, which will save the farm $13,827 per year and will save 184 thousand kilowatt hours per year, which is enough electricity to power 17 homes.
- In Madison County Madison Branch & Bloom DBA Good Fight will use a $70 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 18.27-kilowatt solar array which will be connected to a battery backup energy storage system to ensure the business never loses power. This project will generate 21 thousand kilowatt hours per year, which is enough electricity to power two homes.
- In Madison County Lightshed LLC will use a $16 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 12-kilowatt solar array. This project will generate 16 thousand hours per year.
- In Polk County Pure Country Inc. will use a $257 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 264-kilowatt solar array. This project will save 889 thousand kilowatt hours per year, which is enough electricity to power 33 homes.
- In Surry County Christopher Andrew Collins will use a $96 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 84.5-kilowatt solar array. This project will save 127 thousand kilowatt hours per year, which is enough electricity to power 12 homes.
- In Wilkes County Speaks Poultry will use a $332 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 386-kilowatt solar array. This project will generate 587,102 kilowatt hours per year, which is enough electricity to power 53 homes.
Nationwide today’s announcement includes an investment of over $120 million in 541 Rural Energy for America Program (REAP) projects across 44 states.
USDA is making the REAP awards in Alabama, Alaska, Arkansas, California, Colorado, Connecticut, Delaware, Florida, Georgia, Hawaii, Idaho, Illinois, Indiana, Iowa, Kansas, Kentucky, Louisiana, Maine, Maryland, Massachusetts, Michigan, Minnesota, Mississippi, Missouri, Montana, Nebraska, New Hampshire, New Jersey, New Mexico, North Carolina, North Dakota, Ohio, Oklahoma, Pennsylvania, Rhode Island, South Carolina, South Dakota, Tennessee, Utah, Vermont, Virginia, Washington, Wisconsin and Wyoming.
Since the start of the Biden-Harris Administration, USDA has invested more than $1.8 billion through REAP in over 6,000 renewable energy and energy efficiency improvements that will help rural business owners' lower energy costs, generate new income, and strengthen their resiliency of operations.
USDA continues to accept REAP applications and will hold funding competitions quarterly through September 30, 2024. The funding includes a dedicated portion for underutilized renewable energy technologies. For additional information on application deadlines and submission details, see page 19239 of the March 31 Federal Register .
USDA Rural Development provides loans and grants to help expand economic opportunities, create jobs and improve the quality of life for millions of Americans in rural areas. This assistance supports infrastructure improvements; business development; housing; community facilities such as schools, public safety and health care; and high-speed internet access in rural, tribal and high-poverty areas. Visit the Rural Data Gateway to learn how and where these investments are impacting rural America. To subscribe to USDA Rural Development updates, visit the GovDelivery Subscriber Page .
USDA touches the lives of all Americans each day in so many positive ways. Under the Biden-Harris Administration, USDA is transforming America’s food system with a greater focus on more resilient local and regional food production, fairer markets for all producers, ensuring access to safe, healthy and nutritious food in all communities, building new markets and streams of income for farmers and producers using climate-smart food and forestry practices, making historic investments in infrastructure and clean energy capabilities in rural America, and committing to equity across the Department by removing systemic barriers and building a workforce more representative of America. To learn more, visit www.usda.gov .
USDA is an equal opportunity provider, employer, and lender.
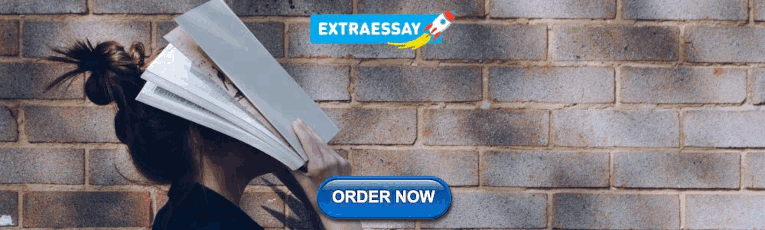
IMAGES
VIDEO
COMMENTS
@wdanxna when Array is given multiple arguments, it iterates over the arguments object and explicitly applies each value to the new array. When you call Array.apply with an array or an object with a length property Array is going to use the length to explicitly set each value of the new array. This is why Array(5) gives an array of 5 elisions, while Array.apply(null, Array(5)) gives an array ...
Create an Array of Length Using Array() Constructor in JavaScript. The first way of creating an empty array of specific lengths is by using the Array() constructor and passing an integer as an argument to it. Since we are calling a constructor, we will be using the new keyword. var arr = new Array(5);
Both create a new Array instance. ... elementN. A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to ... only argument passed to the Array constructor is an integer between 0 and 2 32 - 1 (inclusive), this returns a new JavaScript array with its length property set to that ...
var foo = new Array(N); // where N is a positive integer /* this will create an array of size, N, primarily for memory allocation, but does not create any defined values foo.length // size of Array foo[ Math.floor(foo.length/2) ] = 'value' // places value in the middle of the array */ ES6 Spread
The array object observes the length property, and automatically syncs the length value with the array's content. This means: Setting length to a value smaller than the current length truncates the array — elements beyond the new length are deleted.; Setting any array index (a nonnegative integer smaller than 2 32) beyond the current length extends the array — the length property is ...
The .fill () method changes an existing Array and fills it with a specified value. That helps with initializing an Array after creating it via new Array (): const arr = new Array(LEN).fill(0); Caveat: If you .fill () an Array with an object, all elements refer to the same instance (i.e., the object isn't cloned):
Creating an Array. Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [ item1, item2, ... ]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const.
Another way to create an array is to use the new keyword with the Array constructor. Here is the basic syntax: new Array(); If a number parameter is passed into the parenthesis, that will set the length for the new array. In this example, we are creating an array with a length of 3 empty slots. new Array(3)
This example shows three ways to create new array: first using array literal notation, then using the Array() constructor, and finally using String.prototype.split() to build the array from a string. js. // 'fruits' array created using array literal notation. const fruits = ["Apple", "Banana"];
Summary: in this tutorial, you'll learn about the JavaScript Array length property and how to handle it correctly.. What exactly is the JavaScript Array length property. By definition, the length property of an array is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array.. The value of the length is 2 32.It means that an array can hold up to ...
Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements, both to/from the beginning or the end. ... Create an array styles with items "Jazz" and "Blues". ... In other words, if we increase the array size 2 times, the algorithm will work 4 times longer. For big arrays (1000, 10000 or more items ...
In JavaScript, an array is an object constituted by a group of items having a specific order. Arrays can hold values of mixed data types and their size is not fixed. How to Create an Array in JavaScript. You can create an array using a literal syntax - specifying its content inside square brackets, with each item separated by a comma.
Since the array index starts from 0, you can start the for loop at 0 too by using let i = 0;. Set the length property manually. The array length property can also be used to set the size of an array by assigning a new number to it. Consider the following example:
Say that you want an array with three elements inside of it, we can pass it in just like this. var generatedArray = new Array( 3 ); And this will create the array for us. If I say generatedArray now you can see that we have 3 items. They're all undefined. But we do have a collection.
The fill() method fills empty slots in sparse arrays with value as well. The fill() method is generic. It only expects the this value to have a length property. Although strings are also array-like, this method is not suitable to be applied on them, as strings are immutable. Note: Using Array.prototype.fill() on an empty array ( length = 0 ...
Create your own server using Python, PHP, React.js, Node.js, Java, C#, etc. How To's. Large collection of code snippets for HTML, CSS and JavaScript. CSS Framework. Build fast and responsive sites using our free W3.CSS framework ... JavaScript Array length
Using the <.length> property. Javascript has a <.length> property that returns the size of an array as a number (integer). Here's an example of how to use it: let numbers = [12,13,14,25] let numberSize = numbers.length. console.log(numberSize) # Output. # 4. In the above code, a variable with the name numbers stores an array of numbers, while ...
Javascript create array of objects of length n [duplicate] Ask Question Asked 4 years, 6 months ago. Modified 1 year, 6 months ago. Viewed 14k times 16 This question already has answers here: ... Is there a one-liner to create an array of objects of length n?
Description. Array.from() lets you create Array s from: iterable objects (objects such as Map and Set ); or, if the object is not iterable, array-like objects (objects with a length property and indexed elements). To convert an ordinary object that's not iterable or array-like to an array (by enumerating its property keys, values, or both), use ...
I already tried something like. type Sizes = {number,number,number,number} but that doesnt' work. Basically I want to make a type that is just a fixed size array with the same type. Like in C i would do int [4] to make a array of 4 ints.
There's something else going on I don't understand. I'm creating a new array with new Array(2) and what I get back is [ <2 empty items> ] not [undefined, undefined]. Using .map on the former has no effect. However, I can iterate over it using a for...of loop. If I create a new array using literal notation a = [undefined, undefined] then I can ...
In Alexander County Danny Millsaps will use a $69 thousand USDA REAP Renewable Energy and Efficiency Grant to purchase and install a 93.5-kilowatt solar array. This poultry farming operation will realize $9,537 per year in savings, and will save 119 thousand kilowatt hours per year, which is save enough electricity to power eleven homes.
313. You can use Array.sort method to sort the array. The callback function should use the length of item as the sorting criteria: // sort ascending - shorter items first. arr.sort((a, b) => a.length - b.length); // sort descending - longer items first. arr.sort((a, b) => b.length - a.length); You can specify additional criteria if length of ...