A Complete Guide to Indexing and Accessing Elements in Python Lists
In Python, lists are mutable sequences that can store elements of different data types. Lists are one of the most commonly used data structures in Python. They allow you to store and access ordered collections of data in an efficient and organized manner.
One of the fundamental operations we perform on lists is accessing or indexing individual elements. Python provides a simple syntax and built-in methods to retrieve, modify or delete elements from a list based on their indices. This comprehensive guide will walk you through the indexing and accessing of list elements in Python.
We will cover the following topics in detail with example code snippets:
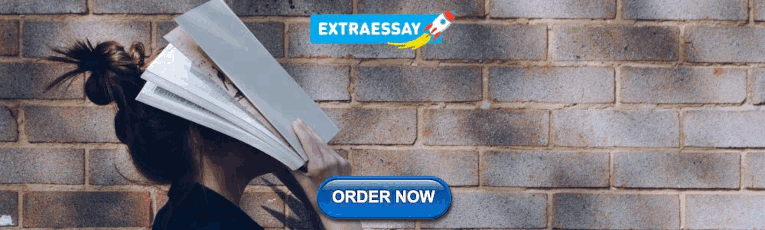
Table of Contents
What is indexing, accessing the first and last elements, accessing elements using negative indexes, slicing syntax, omitting start, stop, or step parameters, checking if an element exists, modifying elements, deleting elements, iterating through list elements, things to remember.
Indexing allows you to access individual elements in a list by referring to an index number. In Python lists, indexes start at 0 for the first element, 1 for the second element, and so on. This is known as zero-based indexing.
For example, consider the following simple list with 4 elements:
To access the first element ‘apple’, we use index 0:
The index numbers from left to right are 0, 1, 2, 3 respectively.
Indexing syntax in Python is simple - specify the list name followed by the index number enclosed in square brackets.
Now let’s look at the different ways we can index lists in Python.
Accessing Elements Using Positive Indexes
We can access any element in a list using its positive index. Positive indexes start from 0 from the left and increment by 1.
To retrieve the 2nd element ‘banana’ from the fruits list, use index 1:
Similarly, we can access the 3rd and 4th elements ‘mango’ and ‘orange’ using indexes 2 and 3 respectively.
We can also assign new values to elements based on their index:
This mutable nature allows us to modify lists easily.
We can use index 0 to get the first element and index -1 to get the last element of a list quickly:
Accessing the first and last elements is a common operation, so Python makes it easier with these shorthands.
Python allows negative indexes that start from the end of the list. The index -1 refers to the last element, -2 refers to the second last element and so on.

Here are some examples:
We can use negative indexes to modify elements as well:
Negative indexes provide an easy way to access elements from the end of the list.
Slicing a List
Slicing allows us to retrieve a subset of elements from a list. It creates a new list containing elements from the start index to stop index.
The basic slicing syntax is:
start is the index to start slicing (inclusive). Defaults to 0 if omitted.
stop is the index to end slicing (exclusive). Defaults to the length of the list if omitted.
step is the increment between indexes. Defaults to 1 if omitted.
Let’s slice our fruits list:
We can also slice from the beginning or end by omitting start and stop indexes:
Use negative indexes to slice backwards from the end:
We can add a step to skip elements:
Slicing is a very convenient way to extract sections from a list.
Python allows us to omit one or more slicing parameters for convenience:
- Omitting start will start from index 0
- Omitting stop will slice to the end of the list
- Omitting step will use the default step of 1
The last example shows reverse slicing by setting step to -1.
We can check if a list contains a particular element by using in operator:
This returns True if the element exists and False if not.
To check based on index, we can use:
Here we compare the index against the valid range of indexes calculated using range() and len() .
These membership tests help avoid errors while indexing elements.
We can modify one or more elements in a list by assigning new values.
To change the second element to ‘strawberry’:
We can also assign an entirely new list to slice and replace elements:
Here’s how to insert a new element without replacing any existing element:
The insert() method inserts the element at the specified index.
To delete an element from a list, use the del keyword by specifying index or slice to remove:
The remove() method can delete the first matching element by value:
Similarly, pop() removes and returns the element at a given index:
All these methods modify the list in-place and reduce the length.
We can loop over the elements of a list using for loop:
The loop iterates through each element in order starting from index 0.
We can also iterate over indexes and access elements:
This allows us to perform any operations on the elements inside the loop.
List comprehension is another concise way to iterate through elements:
Indexes start from 0 for the first element and increase by 1 for subsequent elements.
Use positive indexes to access elements from the start, and negative indexes to access elements from the end.
Slicing extracts sections of a list into a new list.
Check if an element exists using in operator before accessing it.
Modifying lists by assigning to indexes or slices changes the original list in-place.
Delete individual elements or slices using del , pop() or remove() methods.
Iterate through lists using for loops, range() or list comprehensions.
And there you have it - a comprehensive guide to indexing, slicing, modifying and accessing elements in Python lists through various examples. Python’s list indexing and slicing operations provide a convenient and efficient way to work with ordered data. Mastering element access in lists is fundamental to Python programming.
- python
- data-structures
How to Add an Element to a Python List at an Index?
To add an element to a given Python list, you can use either of the three following methods:
- Use the list insert method list.insert(index, element) .
- Use slice assignment lst[index:index] = [element] to overwrite the empty slice with a list of one element.
- Use list concatenation with slicing lst[:2] + ['Alice'] + lst[2:] to create a new list object.

In the following, you’ll learn about all three methods in greater detail. But before that, feel free to test those yourself in our interactive Python shell (just click “Run” to see the output):
Method 1: insert(index, element)
The list.insert(i, element) method adds an element element to an existing list at position i . All elements j>i will be moved by one index position to the right.
Here’s an example with comments:
Check out the objects in memory while executing this code snippet (in comparison to the other methods discussed in this article):
Click “Next” to move on in the code and observe the memory objects creation.
Related article : Python List insert() Method
Method 2: Slice Assignment
Slice assignment is a little-used, beautiful Python feature to replace a slice with another sequence.
Simply select the slice you want to replace on the left and the values to replace it on the right side of the equation.
For example, the slice assignment list[2:4] = [42, 42] replaces the list elements with index 2 and 3 with the value 42 .
Here’s another example that shows you how to insert the string 'Alice' into a list with four integers.
Let’s have a look at the code:
Related article : Python Slice Assignment
Method 3: List Concatenation
If you use the + operator on two integers, you’ll get the sum of those integers. But if you use the + operator on two lists, you’ll get a new list that is the concatenation of those lists.
Here’s the same example, you’ve already seen in the previous sections:
Related article : How to Concatenate Lists in Python? [Interactive Guide]
Where to Go From Here?
Enough theory. Let’s get some practice!
Coders get paid six figures and more because they can solve problems more effectively using machine intelligence and automation.
To become more successful in coding, solve more real problems for real people. That’s how you polish the skills you really need in practice. After all, what’s the use of learning theory that nobody ever needs?
You build high-value coding skills by working on practical coding projects!
Do you want to stop learning with toy projects and focus on practical code projects that earn you money and solve real problems for people?
🚀 If your answer is YES! , consider becoming a Python freelance developer ! It’s the best way of approaching the task of improving your Python skills—even if you are a complete beginner.
If you just want to learn about the freelancing opportunity, feel free to watch my free webinar “How to Build Your High-Income Skill Python” and learn how I grew my coding business online and how you can, too—from the comfort of your own home.
Join the free webinar now!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
Indexing in Python – A Complete Beginners Guide
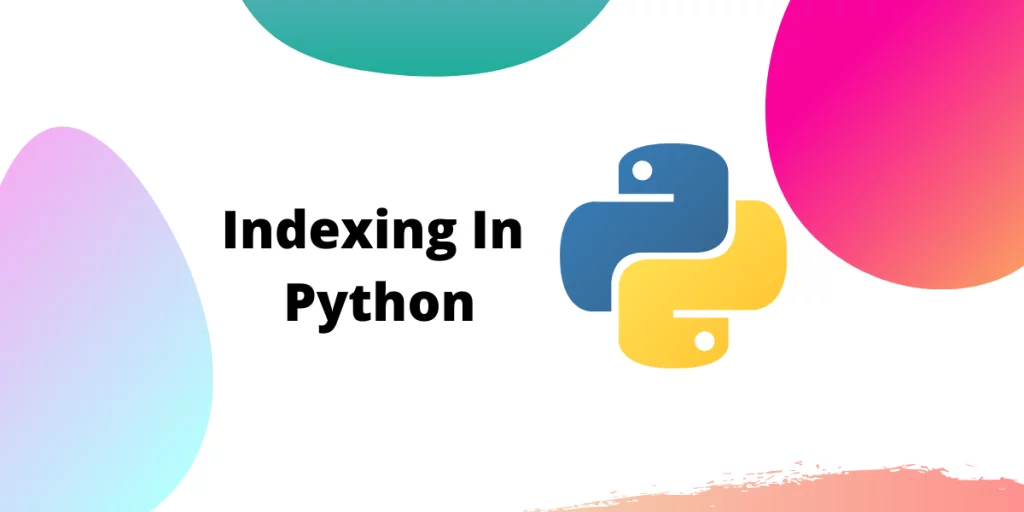
Data structures in Python include lists, tuples, etc. These data structures can have multiple elements in them, each having some different properties but the problem is how to refer to a particular element from the hundreds of elements they contain. Here indexing comes into action. Indexing is a simple but fundamental concept that is important to learn before further processing with Python data structures.
This tutorial will explain everything you need to know about indexing in Python. But first, let’s take a quick look at iterables.
Prerequisite – What are Iterables?
Before we get started with indexing, let’s understand what iterables are and what is their main function. The knowledge of iterables is much needed to go behind indexing.
Iterables in Python
Iterables are a special type of objects in Python that you can iterate over. Meaning you can traverse through all the different elements or entities contained within the object. It can be easily achieved using the for loops .
Under the hood, what all these iterable items carry are two unique methods called __iter__() or __getitem__() that implement the Sequence Semantics .
Besides lists in Python, strings and tuples are also iterable.
Now that we know what Iterables are in Python. How is this related to indexing?
What is Indexing in Python?
Indexing in Python is a way to refer to the individual items within an iterable by their position. In other words, you can directly access your elements of choice within an iterable and do various operations depending on your needs.
Before we get into examples of Indexing in Python, there’s an important thing to Note:
In Python, objects are “zero-indexed” meaning the position count starts at zero. Many other programming languages follow the same pattern. So, if there are 5 elements present within a list. Then the first element (i.e. the leftmost element) holds the “zeroth” position, followed by the elements in the first, second, third, and fourth positions.
Python Index Method
The index of a specific item within a list can be revealed when the index() method is called on the list with the item name passed as an argument.
Where item is an element that index value we want to get.
Python Index Operator
The Python Index Operator is represented by opening and closing square brackets: []. The syntax, however, requires you to put a number inside the brackets.
Where n is just an integer number representing the position of the element we want to access.
We can see how our print function accesses different elements within our string object to get the specific characters we want.
Negative Indexing in Python
We’ve recently learned how to use indexing in Lists and Strings to get the specific items of our interest. Although in all our previous cases we’ve used a positive integer inside our index operator (the square brackets), it’s not necessarily needed to be that way.
Often, if we are interested in the last few elements of a list or maybe we just want to index the list from the opposite end, we can use negative integers. This process of indexing from the opposite end is called Negative Indexing.
Note: In negative Indexing, the last element is represented by -1 and not -0.
In this tutorial, we’ve learned that indexing is just a way of referencing the elements of an iterable. We have used the Python index() method to get the index of a particular element. We’ve also looked at the Python index operator and what negative indexing is. Hope you enjoyed the tutorial and learned how to implement indexing in your next project.
https://docs.python.org/3/tutorial/datastructures.html
- Google for Education
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Python Lists
Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len() function and square brackets [ ] to access data, with the first element at index 0. (See the official python.org list docs .)

Assignment with an = on lists does not make a copy. Instead, assignment makes the two variables point to the one list in memory.

The "empty list" is just an empty pair of brackets [ ]. The '+' works to append two lists, so [1, 2] + [3, 4] yields [1, 2, 3, 4] (this is just like + with strings).
Python's *for* and *in* constructs are extremely useful, and the first use of them we'll see is with lists. The *for* construct -- for var in list -- is an easy way to look at each element in a list (or other collection). Do not add or remove from the list during iteration.
If you know what sort of thing is in the list, use a variable name in the loop that captures that information such as "num", or "name", or "url". Since Python code does not have other syntax to remind you of types, your variable names are a key way for you to keep straight what is going on. (This is a little misleading. As you gain more exposure to python, you'll see references to type hints which allow you to add typing information to your function definitions. Python doesn't use these type hints when it runs your programs. They are used by other programs such as IDEs (integrated development environments) and static analysis tools like linters/type checkers to validate if your functions are called with compatible arguments.)
The *in* construct on its own is an easy way to test if an element appears in a list (or other collection) -- value in collection -- tests if the value is in the collection, returning True/False.
The for/in constructs are very commonly used in Python code and work on data types other than list, so you should just memorize their syntax. You may have habits from other languages where you start manually iterating over a collection, where in Python you should just use for/in.
You can also use for/in to work on a string. The string acts like a list of its chars, so for ch in s: print(ch) prints all the chars in a string.
The range(n) function yields the numbers 0, 1, ... n-1, and range(a, b) returns a, a+1, ... b-1 -- up to but not including the last number. The combination of the for-loop and the range() function allow you to build a traditional numeric for loop:
There is a variant xrange() which avoids the cost of building the whole list for performance sensitive cases (in Python 3, range() will have the good performance behavior and you can forget about xrange()).
Python also has the standard while-loop, and the *break* and *continue* statements work as in C++ and Java, altering the course of the innermost loop. The above for/in loops solves the common case of iterating over every element in a list, but the while loop gives you total control over the index numbers. Here's a while loop which accesses every 3rd element in a list:
List Methods
Here are some other common list methods.
- list.append(elem) -- adds a single element to the end of the list. Common error: does not return the new list, just modifies the original.
- list.insert(index, elem) -- inserts the element at the given index, shifting elements to the right.
- list.extend(list2) adds the elements in list2 to the end of the list. Using + or += on a list is similar to using extend().
- list.index(elem) -- searches for the given element from the start of the list and returns its index. Throws a ValueError if the element does not appear (use "in" to check without a ValueError).
- list.remove(elem) -- searches for the first instance of the given element and removes it (throws ValueError if not present)
- list.sort() -- sorts the list in place (does not return it). (The sorted() function shown later is preferred.)
- list.reverse() -- reverses the list in place (does not return it)
- list.pop(index) -- removes and returns the element at the given index. Returns the rightmost element if index is omitted (roughly the opposite of append()).
Notice that these are *methods* on a list object, while len() is a function that takes the list (or string or whatever) as an argument.
Common error: note that the above methods do not *return* the modified list, they just modify the original list.
List Build Up
One common pattern is to start a list as the empty list [], then use append() or extend() to add elements to it:
List Slices
Slices work on lists just as with strings, and can also be used to change sub-parts of the list.
Exercise: list1.py
To practice the material in this section, try the problems in list1.py that do not use sorting (in the Basic Exercises ).
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2023-09-05 UTC.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Python List Index Out of Range - How to Fix IndexError
- Python | List comprehension vs * operator
- Print lists in Python (6 Different Ways)
- Python | Creating a 3D List
- Apply function to each element of a list - Python
- Reducing Execution time in Python using List Comprehensions
- Python List Comprehensions vs Generator Expressions
- Python | Iterate over multiple lists simultaneously
- Sum of list (with string types) in Python
- Python List Comprehension | Segregate 0's and 1's in an array list
- Use of slice() in Python
- Extending a list in Python (5 different ways)
- Python - Change List Item
- Python | Ways to initialize list with alphabets
- Python | Find maximum value in each sublist
- Python | Adding two list elements
- Python | Filter even values from a list
- Python | Check if one list is subset of other
- Python | Make a list of intervals with sequential numbers
Python Indexerror: list assignment index out of range Solution
In python, lists are mutable as the elements of a list can be modified. But if you try to modify a value whose index is greater than or equal to the length of the list then you will encounter an Indexerror: list assignment index out of range.
Python Indexerror: list assignment index out of range Example
If ‘fruits’ is a list, fruits=[‘Apple’,’ Banana’,’ Guava’]and you try to modify fruits[5] then you will get an index error since the length of fruits list=3 which is less than index asked to modify for which is 5.
So, as you can see in the above example, we get an error when we try to modify an index that is not present in the list of fruits.
Method 1: Using insert() function
The insert(index, element) function takes two arguments, index and element, and adds a new element at the specified index.
Let’s see how you can add Mango to the list of fruits on index 1.
It is necessary to specify the index in the insert(index, element) function, otherwise, you will an error that the insert(index, element) function needed two arguments.
Method 2: Using append()
The append(element) function takes one argument element and adds a new element at the end of the list.
Let’s see how you can add Mango to the end of the list using the append(element) function.
Python IndexError FAQ
Q: what is an indexerror in python.
A: An IndexError is a common error that occurs when you try to access an element in a list, tuple, or other sequence using an index that is out of range. It means that the index you provided is either negative or greater than or equal to the length of the sequence.
Q: How can I fix an IndexError in Python?
A: To fix an IndexError, you can take the following steps:
- Check the index value: Make sure the index you’re using is within the valid range for the sequence. Remember that indexing starts from 0, so the first element is at index 0, the second at index 1, and so on.
- Verify the sequence length: Ensure that the sequence you’re working with has enough elements. If the sequence is empty, trying to access any index will result in an IndexError.
- Review loop conditions: If the IndexError occurs within a loop, check the loop conditions to ensure they are correctly set. Make sure the loop is not running more times than expected or trying to access an element beyond the sequence’s length.
- Use try-except: Wrap the code block that might raise an IndexError within a try-except block. This allows you to catch the exception and handle it gracefully, preventing your program from crashing.
Please Login to comment...
Similar reads.
- Python How-to-fix
- python-list
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python list methods, python list index().
- Python List append()
- Python List extend()
Python List insert()
Python List remove()
Python List count()
Python List pop()
- Python List reverse()
- Python List sort()
- Python List copy()
- Python List clear()
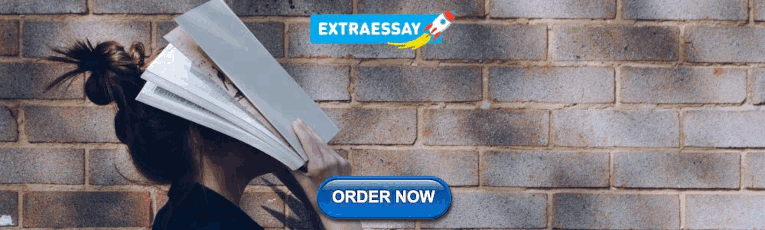
Python Tutorials
- Python Tuple index()
- Python String index()
- Python String rindex()
- Python Tuple count()
The index() method returns the index of the specified element in the list .
Syntax of List index()
The syntax of the list index() method is:
list index() parameters
The list index() method can take a maximum of three arguments:
- element - the element to be searched
- start (optional) - start searching from this index
- end (optional) - search the element up to this index
Return Value from List index()
- The index() method returns the index of the given element in the list.
- If the element is not found, a ValueError exception is raised.
Note: The index() method only returns the first occurrence of the matching element.
Example 1: Find the index of the element
Example 2: index of the element not present in the list, example 3: working of index() with start and end parameters.
Also Read: Python Program to Access Index of a List Using for Loop
Sorry about that.
Python References
Python Library

- Online Python Compiler
- Hello World
- Console Operations
- Conditional Statements
- Loop Statements
- Builtin Functions
- Type Conversion
Collections
- Classes and Objects
- File Operations
- Global Variables
- Regular Expressions
- Multi-threading
- phonenumbers
- Breadcrumbs
- ► Python Examples
- ► ► List Operations
- ► ► ► Python List index()
- Python Strings
- Python List Tutorials
- Python Lists
- Python List Operations
- Create Lists
- Python – Create an empty list
- Python – Create a list of size n
- Python – Create a list of numbers from 1 to n
- Python – Create a list of strings
- Python – Create a list of objects
- Python – Create a list of empty lists
- Access Lists
- Python List – Access elements
- Python List – Get item at specific index
- Python List – Get first element
- Python List – Get last element
- Python List – Iterate with index
- Python List – While loop
- Python List – For loop
- Python List – Loop through items
- Python – Unpack List
- List Properties
- Python – List length
- List Methods
- Python List Methods
- Python List append()
- Python List clear()
- Python List copy()
- Python List count()
- Python List extend()
Python List index()
- Python List insert()
- Python List pop()
- Python List remove()
- Python List reverse()
- Python List sort()
- Update / Transform Lists
- Python – Add item to list
- Python – Remove specific item from list
- Python – Remove item at specific index from list
- Python – Remove all occurrences of an item from list
- Python – Remove duplicates from list
- Python – Append a list to another list
- Python – Reverse list
- Python – Sort list
- Python – Sort list in descending order
- Python – Shuffle list
- Python – Convert list into list of lists
- Checking Operations on Lists
- Python - Check if list is empty
- Python - Check if element is present in list
- Python - Check if value is in list using "in" operator
- Python - Check if list contains all elements of another list
- List Finding Operations
- Python – Count the occurrences of items in a List with a specific value
- Python – Find duplicates in list
- Python – Find unique elements in list
- Python – Find index of specific item in list
- Python – Insert item at specific position in list
- Python – Find element with most number of occurrences in the list
- Python – Find element with least number of occurrences in the list
- List Comprehension Operations
- Python List Comprehension
- Python List Comprehension with two lists
- Python List Comprehension with If condition
- Python List Comprehension with multiple conditions
- Python List Comprehension with List of Lists
- Python List Comprehension with nested For loops
- List Filtering
- Python – Filter list
- Python – Filter list of strings that start with specific prefix string
- Python – Filter list based on datatype
- Python – Get list without last element
- Python – Traverse list except last element
- Python List – Get first N elements
- Python List – Get last N elements
- Python List – Slice
- Python List – Slice first N elements
- Python List – Slice last N elements
- Python List – Slice all but last
- Python List – Slice reverse
- List Conversions to other Types
- Python – Convert List to Dictionary
- Python – Convert dictionary keys to list
- Python – Convert dictionary values to list
- Python – Convert list to tuple
- Python – Convert tuple to list
- Python – Convert list to set
- Python – Convert set to list
- Python – Convert list to string
- Python – Convert CSV string to list
- Python – Convert list to CSV string
- Python – Convert string (numeric range) to a list
- Python – Convert list of lists to a flat list
- Python - Convert list of strings into list of integers
- Python - Convert list of strings into list of floats
- Python - Convert list of integers into list of strings
- Python - Convert list of floats into list of strings
- Numeric Lists
- Python – Find average of numbers in list
- Python – Find largest number in list
- Python – Find smallest number in list
- Python – Find sum of all numbers in numeric list
- Python – Find product of all numbers in numeric list
- Python – Sort numbers
- String Lists
- Python – Compare lists lexicographically
- Python – Find shortest string in list
- Python – Find longest string in list
- Python – Find most occurred string in a list
- Python – Find least occurred string in a list
- Python – Sort list of strings based on length
- Python – Sort a list alphabetically
- Python - Join list with new line
- Python - Join list with comma
- Python - Join list with space
- Printing Lists
- Python - Print list
- Python - Print list without brackets
- Python - Print list without commas
- Python - Print list without quotes
- Python - Print list without spaces
- Python - Print list without brackets and commas
- Python - Print list without brackets and quotes
- Python - Print list without quotes and commas
- Python - Print list in reverse order
- Python - Print list in JSON format
- Python - Print list with double quotes
- Python - Print list with index
- Python - Print list as column
- Python - Print list as lines
- Python - Print elements at even indices in List
- Python - Print elements at odd indices in List
- Exceptions with Lists
- Python List ValueError: too many values to unpack
- Python List ValueError: not enough values to unpack
- List Other Topics
- Python List of Lists
- Python – Print a list
- Python List vs Set
- Python Dictionary
Syntax of List index()
- Get the index of value ‘cherry’ in the list in Python
- Get the index of value ‘cherry’ in the list with search from a specific start index
- Get the index of value ‘cherry’ in the list with search in [start, stop) indices of list
- List index() method when the value is not present in list
Python List index() method
Python List index() method is used to find the index (position) of the specified value in the list.
The search for the specified value happens from starting off the list to the ending of the list. This is the direction of search.
The syntax of List index() method is
index() method can take three parameters. Let us see those parameters, and their description.
Return Value
index() method returns an integer value representing the index of the specified value in the list.
index() method can raise ValueError exception.
If the specified value is not present in the list, then index() method raises ValueError.
1. Get the index of value ‘cherry’ in the list in Python
In the following program, we define a list my_list with four string values. We have to find the index of the value 'cherry' in this list.
Call index() method on the list, and pass the value as argument.
Python Program
Explanation
2. Get the index of value ‘cherry’ in the list with search from a specific start index
In the following program, we define a list my_list with five string values. We have to find the index of the value 'cherry' in this list with search happening from a start index of 3 .
Call index() method on the list, and pass the search value 'cherry' and start index 3 as arguments.
3. Get the index of value ‘cherry’ in the list with search in [start, stop) indices of list
In the following program, we define a list my_list with five string values. We have to find the index of the value 'cherry' in this list with search happening from a start index of 1 , and stoping at an index of 3 .
Call index() method on the list, and pass the search value 'cherry' , start index 1 , and stop index 3 as arguments.
4. List index() method when the value is not present in list
If the search value is not present in the list, then the index() method throws ValueError exception, as shown in the following program.
In this tutorial of Python Examples , we learned about List index() method, how to use index() method to find the index of a value in the list, with syntax and examples.
Related Tutorials
Python Find in List – How to Find the Index of an Item or Element in a List
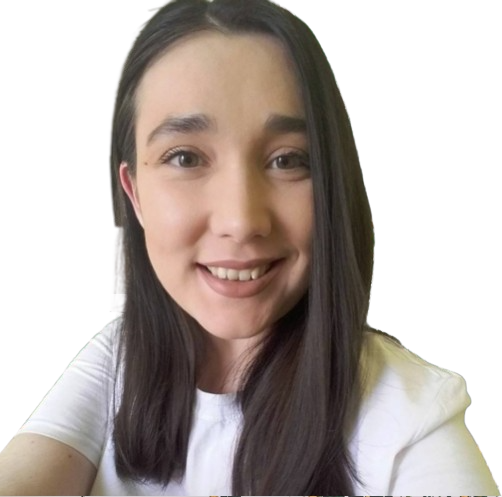
In this article you will learn how to find the index of an element contained in a list in the Python programming language.
There are a few ways to achieve this, and in this article you will learn three of the different techniques used to find the index of a list element in Python.
The three techniques used are:
- finding the index using the index() list method,
- using a for-loop ,
- and finally, using list comprehension and the enumerate() function.
Specifically, here is what we will cover in depth:
- How indexing works
- Use the index() method to find the index of an item 1. Use optional parameters with the index() method
- Use a for-loop to get indices of all occurrences of an item in a list
- Use list comprehension and the enumerate() function to get indices of all occurrences of an item in a list
What are Lists in Python?
Lists are a built-in data type in Python, and one of the most powerful data structures.
They act as containers and store multiple, typically related, items under the same variable name.
Items are placed and enclosed inside square brackets, [] . Each item inside the square brackets is separated by a comma, , .
From the example above, you can see that lists can contain items that are of any data type, meaning list elements can be heterogeneous.
Unlike arrays that only store items that are of the same type, lists allow for more flexibility.
Lists are also mutable , which means they are changeable and dynamic. List items can be updated, new items can be added to the list, and any item can be removed at any time throughout the life of the program.
An Overview of Indexing in Python
As mentioned, lists are a collection of items. Specifically, they are an ordered collection of items and they preserve that set and defined order for the most part.
Each element inside a list will have a unique position that identifies it.
That position is called the element's index .
Indices in Python, and in all programming languages, start at 0 and not 1 .
Let's take a look at the list that was used in the previous section:
The list is zero-indexed and counting starts at 0 .
The first list element, "John Doe" , has an index of 0 . The second list element, 34 , has an index of 1 . The third list element, "London" , has an index of 2 . The forth list element, 1.76 , has an index of 3 .
Indices come in useful for accessing specific list items whose position (index) you know.
So, you can grab any list element you want by using its index.
To access an item, first include the name of the list and then in square brackets include the integer that corresponds to the index for the item you want to access.
Here is how you would access each item using its index:
But what about finding the index of a list item in Python?
In the sections that follow you will see some of the ways you can find the index of list elements.
Find the Index of an Item using the List index() Method in Python
So far you've seen how to access a value by referencing its index number.
What happens though when you don't know the index number and you're working with a large list?
You can give a value and find its index and in that way check the position it has within the list.
For that, Python's built-in index() method is used as a search tool.
The syntax of the index() method looks like this:
Let's break it down:
- my_list is the name of the list you are searching through.
- .index() is the search method which takes three parameters. One parameter is required and the other two are optional.
- item is the required parameter. It's the element whose index you are searching for.
- start is the first optional parameter. It's the index where you will start your search from.
- end the second optional parameter. It's the index where you will end your search.
Let's see an example using only the required parameter:
In the example above, the index() method only takes one argument which is the element whose index you are looking for.
Keep in mind that the argument you pass is case-sensitive . This means that if you had passed "python", and not "Python", you would have received an error as "python" with a lowercase "p" is not part of the list.
The return value is an integer, which is the index number of the list item that was passed as an argument to the method.
Let's look at another example:
If you try and search for an item but there is no match in the list you're searching through, Python will throw an error as the return value - specifically it will return a ValueError .
This means that the item you're searching for doesn't exist in the list.
A way to prevent this from happening, is to wrap the call to the index() method in a try/except block.
If the value does not exist, there will be a message to the console saying it is not stored in the list and therefore doesn't exist.
Another way would be to check to see if the item is inside the list in the first place, before looking for its index number. The output will be a Boolean value - it will be either True or False.
How to Use the Optional Parameters with the index() Method
Let's take a look at the following example:
In the list programming_languages there are three instances of the "Python" string that is being searched.
As a way to test, you could work backwards as in this case the list is small.
You could count and figure out their index numbers and then reference them like you've seen in previous sections:
There is one at position 1 , another one at position 3 and the last one is at position 5 .
Why aren't they showing in the output when the index() method is used?
When the index() method is used, the return value is only the first occurence of the item in the list. The rest of the occurrences are not returned.
The index() method returns only the index of the position where the item appears the first time.
You could try passing the optional start and end parameters to the index() method.
You already know that the first occurence starts at index 1 , so that could be the value of the start parameter.
For the end parameter you could first find the length of the list.
To find the length, use the len() function:
The value for end parameter would then be the length of the list minus 1. The index of the last item in a list is always one less than the length of the list.
So, putting all that together, here is how you could try to get all three instances of the item:
The output still returns only the first instance!
Although the start and end parameters provide a range of positions for your search, the return value when using the index() method is still only the first occurence of the item in the list.
How to Get the Indices of All Occurrences of an Item in A List
Use a for-loop to get the indices of all occurrences of an item in a list.
Let's take the same example that we've used so far.
That list has three occurrences of the string "Python".
First, create a new, empty list.
This will be the list where all indices of "Python" will be stored.
Next, use a for-loop . This is a way to iterate (or loop) through the list, and get each item in the original list. Specifically, we loop over each item's index number.
You first use the for keyword.
Then create a variable, in this case programming_language , which will act as a placeholder for the position of each item in the original list, during the iterating process.
Next, you need to specify the set amount of iterations the for-loop should perform.
In this case, the loop will iterate through the full length of the list, from start to finish. The syntax range(len(programming_languages)) is a way to access all items in the list programming_languages .
The range() function takes a sequence of numbers that specify the number it should start counting from and the number it should end the counting with.
The len() function calculates the length of the list, so in this case counting would start at 0 and end at - but not include - 6 , which is the end of the list.
Lastly, you need to set a logical condition.
Essentially, you want to say: "If during the iteration, the value at the given position is equal to 'Python', add that position to the new list I created earlier".
You use the append() method for adding an element to a list.
Use List Comprehension and the enumerate() Function to Get the Indices of All Occurrences of an Item in A List
Another way to find the indices of all the occurrences of a particular item is to use list comprehension.
List comprehension is a way to create a new list based on an existing list.
Here is how you would get all indices of each occurrence of the string "Python", using list comprehension:
With the enumerate() function you can store the indices of the items that meet the condition you set.
It first provides a pair ( index, item ) for each element in the list ( programming_languages ) that is passed as the argument to the function.
index is for the index number of the list item and item is for the list item itself.
Then, it acts as a counter which starts counting from 0 and increments each time the condition you set is met, selecting and moving the indices of the items that meet your criteria.
Paired with the list comprehension, a new list is created with all indices of the string "Python".
And there you have it! You now know some of the ways to find the index of an item, and ways to find the indices of multiple occurrences of an item, in a list in Python.
I hope you found this article useful.
To learn more about the Python programming language, check out freeCodeCamp's Scientific Computing with Python Certification .
You'll start from the basics and learn in an interacitve and beginner-friendly way. You'll also build five projects at the end to put into practice and help reinforce what you've learned.
Thanks for reading and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python lists.
Lists are used to store multiple items in a single variable.
Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple , Set , and Dictionary , all with different qualities and usage.
Lists are created using square brackets:
Create a List:
List items are ordered, changeable, and allow duplicate values.
List items are indexed, the first item has index [0] , the second item has index [1] etc.
When we say that lists are ordered, it means that the items have a defined order, and that order will not change.
If you add new items to a list, the new items will be placed at the end of the list.
Note: There are some list methods that will change the order, but in general: the order of the items will not change.
The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
Allow Duplicates
Since lists are indexed, lists can have items with the same value:
Lists allow duplicate values:
Advertisement
List Length
To determine how many items a list has, use the len() function:
Print the number of items in the list:
List Items - Data Types
List items can be of any data type:
String, int and boolean data types:
A list can contain different data types:
A list with strings, integers and boolean values:
From Python's perspective, lists are defined as objects with the data type 'list':
What is the data type of a list?
The list() Constructor
It is also possible to use the list() constructor when creating a new list.
Using the list() constructor to make a List:
Python Collections (Arrays)
There are four collection data types in the Python programming language:
- List is a collection which is ordered and changeable. Allows duplicate members.
- Tuple is a collection which is ordered and unchangeable. Allows duplicate members.
- Set is a collection which is unordered, unchangeable*, and unindexed. No duplicate members.
- Dictionary is a collection which is ordered** and changeable. No duplicate members.
*Set items are unchangeable, but you can remove and/or add items whenever you like.
**As of Python version 3.7, dictionaries are ordered . In Python 3.6 and earlier, dictionaries are unordered .
When choosing a collection type, it is useful to understand the properties of that type. Choosing the right type for a particular data set could mean retention of meaning, and, it could mean an increase in efficiency or security.

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
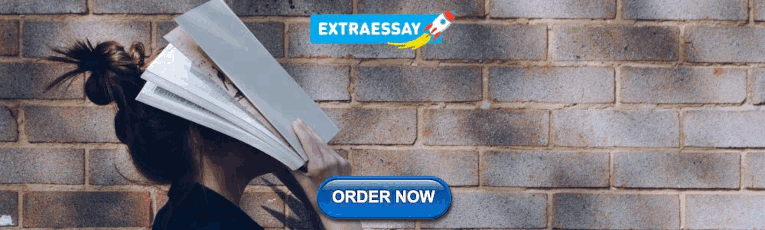
IMAGES
VIDEO
COMMENTS
Just in case someone needs, I figured out a soluction for my problem, I needed to calc a lot of factorials, some of them could be repeated, so here is my solution:. factorials = {} def calcFact(v): try: return factorials[v] except KeyError: factorials[v] = math.factorial(v) return factorials[v]
Python list index() method is very useful when searching for an element in a list. Python list index() function works best in a list where every element is unique. Hope you learnt about how to use index() function in Python? after reading this article. Also Read: Python Program to Accessing index and value in list
Indexing allows you to access individual elements in a list by referring to an index number. In Python lists, indexes start at 0 for the first element, 1 for the second element, and so on. This is known as zero-based indexing. ... Modifying lists by assigning to indexes or slices changes the original list in-place.
Related article: Python Slice Assignment. Method 3: List Concatenation. If you use the + operator on two integers, you'll get the sum of those integers. But if you use the + operator on two lists, you'll get a new list that is the concatenation of those lists. Here's the same example, you've already seen in the previous sections:
Data structures in Python include lists, tuples, etc. These data structures can have multiple elements in them, each having some different properties but the problem is how to refer to a particular element from the hundreds of elements they contain. ... Python Index Method. The index of a specific item within a list can be revealed when the ...
Python - Insert Item at Specific Position in List - To insert of add an item at specific position or index in a list, you can use insert() method of List class. The syntax of insert() method is: mylist.insert(index, item)
In Python, assigning a new value to an existing variable doesn't modify the variable's current value. Instead, ... You can use the assignment operator to mutate the value stored at a given index in a Python list. The operator also works with list slices. The syntax to write these types of assignment statements is the following: Python.
The general approach we follow is to extract each list element by its index and then assign it to variables. This approach requires more line of code. ... Python Program to assign each list element value equal to its magnitude order Assigning multiple variables in one line in Python How to check multiple variables against a value in Python? ...
pt = list() pt.append(raw_input()) pt.append(raw_input()) print pt. You now have two elements in your list. Once you are more familiar with python syntax, you might write this as: pt = [raw_input(), raw_input()] Also, note that lists are not to be confused with arrays in Java or C: Lists grow dynamically. You don't have to declare the size when ...
In this tutorial, we will learn about Python lists (creating lists, changing list items, removing items, and other list operations) with the help of examples. ... The first element of a list is at index 0, the second element is at index 1, and so on. Index of List Elements ... We can change the items of a list by assigning new values using the ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
Python's *for* and *in* constructs are extremely useful, and the first use of them we'll see is with lists. The *for* construct -- for var in list -- is an easy way to look at each element in a list (or other collection). Do not add or remove from the list during iteration. squares = [1, 4, 9, 16] sum = 0. for num in squares: sum += num.
A: To fix an IndexError, you can take the following steps: Check the index value: Make sure the index you're using is within the valid range for the sequence. Remember that indexing starts from 0, so the first element is at index 0, the second at index 1, and so on. Verify the sequence length: Ensure that the sequence you're working with ...
The index of e: 1 The index of i: 6 Traceback (most recent call last): File "*lt;string>", line 13, in ValueError: 'i' is not in list Also Read: Python Program to Access Index of a List Using for Loop
Indices in Python begin at 0 not 1.You can do what you want by using the built-in zip() function along with the count() generator function in the itertools module.. It's also necessary to explicitly convert zip()'s result to a list if that's what you want (which is why I changed the name of your variable to my_list to prevent it from "hiding" the built-in class with the same name — a good ...
Python List index() method. Python List index() method is used to find the index (position) of the specified value in the list. The search for the specified value happens from starting off the list to the ending of the list. This is the direction of search. Syntax of List index() The syntax of List index() method is. list.index(value, start, stop)
If you need an array... you should use an array. However, you can do things like for index in range(10): do_something(l[index]) after it's populated - i.e. even though it doesn't look like an array, it acts like an array for most uses. you will need to define what it means if l[i] doesn't exist though - this will create a empty defaultdict object, but likely if you're seeing that, there's some ...
For the end parameter you could first find the length of the list. To find the length, use the len() function: print(len(programming_languages)) #output is 6. The value for end parameter would then be the length of the list minus 1. The index of the last item in a list is always one less than the length of the list.
Python Variables Variable Names Assign Multiple Values Output Variables Global Variables Variable Exercises. ... the first item has index [0], the second item has index [1] etc. ... There are four collection data types in the Python programming language: List is a collection which is ordered and changeable. Allows duplicate members.
Note: you can also do this with map (which is a little cleaner): df.index = df.index.map(lambda x: 'row%s' % x) ...though I should say that usually this isn't something you usually need to do, keeping integer index is A Good Thing TM. answered Oct 9, 2015 at 23:17. Andy Hayden.