
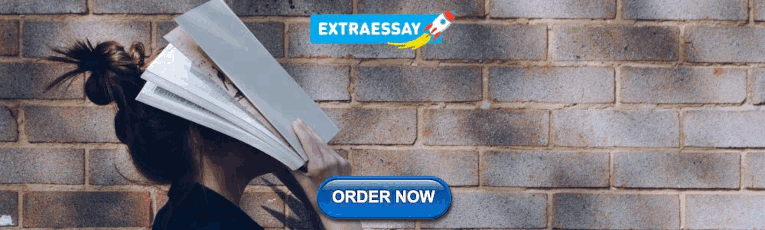
Verilog Conditional Operator
Just what the heck is that question mark doing.
Have you ever come across a strange looking piece of Verilog code that has a question mark in the middle of it? A question mark in the middle of a line of code looks so bizarre; they’re supposed to go at the end of sentences! However in Verilog the ? operator is a very useful one, but it does take a bit of getting used to.
The question mark is known in Verilog as a conditional operator though in other programming languages it also is referred to as a ternary operator , an inline if , or a ternary if . It is used as a short-hand way to write a conditional expression in Verilog (rather than using if/else statements). Let’s look at how it is used:
Here, condition is the check that the code is performing. This condition might be things like, “Is the value in A greater than the value in B?” or “Is A=1?”. Depending on if this condition evaluates to true, the first expression is chosen. If the condition evaluates to false, the part after the colon is chosen. I wrote an example of this. The code below is really elegant stuff. The way I look at the question mark operator is I say to myself, “Tell me about the value in r_Check. If it’s true, then return “HI THERE” if it’s false, then return “POTATO”. You can also use the conditional operator to assign signals , as shown with the signal w_Test1 in the example below. Assigning signals with the conditional operator is useful!
Nested Conditional Operators
There are examples in which it might be useful to combine two or more conditional operators in a single assignment. Consider the truth table below. The truth table shows a 2-input truth table. You need to know the value of both r_Sel[1] and r_Sel[0] to determine the value of the output w_Out. This could be achieved with a bunch of if-else if-else if combinations, or a case statement, but it’s much cleaner and simpler to use the conditional operator to achieve the same goal.
Learn Verilog
Leave A Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Verilog Conditional Statements Tutorial
Conditional statements are crucial in Verilog as they enable you to make decisions and create conditional behaviors in your designs. They allow you to execute specific blocks of code based on certain conditions. In this tutorial, we will explore different types of conditional statements in Verilog and learn how to use them effectively.
Introduction to Conditional Statements
Conditional statements in Verilog provide a way to control the flow of your code based on certain conditions. They are essential for implementing decision-making logic and creating complex behaviors in digital designs. The three primary conditional statements in Verilog are if , else if , and case .
Verilog if Statement
The if statement is used to check a single condition and execute a block of code if the condition evaluates to true. If the condition is false, the code inside the if block is skipped. The syntax of the if statement is as follows:
Verilog else if Statement
The else if statement allows you to check additional conditions if the previous conditions in the if and else if blocks are false. The code inside the first matching condition block is executed, and the rest are skipped. The syntax of the else if statement is as follows:
Verilog case Statement
The case statement is used to perform multi-way decisions based on the value of an expression. It is similar to the switch-case statement in many programming languages. The syntax of the case statement is as follows:
Common Mistakes with Verilog Conditional Statements
- Using blocking assignments inside the conditional blocks, leading to incorrect behavior.
- Not considering all possible cases in the case statement, causing incomplete behavior.
- Mixing different data types in conditionals without proper typecasting.
- Using the assignment operator "=" instead of the equality operator "==" in conditions.
- Overlooking operator precedence in complex conditions, leading to unexpected results.
Frequently Asked Questions (FAQs)
- Q: Can I have nested conditional statements in Verilog? A: Yes, you can nest conditional statements (e.g., if inside else or case inside case ) to create complex decision structures.
- Q: Can I use non-constant expressions in case statements? A: No, Verilog requires constant expressions in case statements, so each case value must be a constant or a constant expression.
- Q: Is the case statement only for sequential logic? A: No, the case statement can be used for both combinational and sequential logic depending on how it is used in the code.
- Q: How does Verilog handle multiple matching cases in a case statement? A: Verilog executes the first matching case it encounters in a case statement and skips the rest.
- Q: Can I use multiple conditions in an if statement? A: Yes, you can use logical operators (e.g., &&, ||) to combine multiple conditions in an if statement.
Conditional statements in Verilog are essential for implementing decision-making logic and creating complex behaviors in digital designs. The if , else if , and case statements enable you to control the flow of your code based on specific conditions. By understanding how to use these conditional statements correctly, you can create efficient and reliable Verilog designs for various applications.
- Verilog tutorial
- ANN tutorial
- CSS tutorial
- Go Lang tutorial
- Azure Resource Manager (ARM) Templates tutorial
- JAXB tutorial
- Expressjs tutorial
- Grafana tutorial
?: conditional operator in Verilog
Compact conditional operators.
Many Verilog designs make use of a compact conditional operator:
A comman example, shown below, is an “enable” mask. Suppose there is some internal signal named a . When enabled by en== 1 , the module assigns q = a , otherwise it assigns q = 0 :
The syntax is also permitted in always blocks:
Assigned Tasks
This assignment uses only a testbench simulation, with no module to implement. Open the file src/testbench.v and examine how it is organized. It uses the conditional operator in an always block to assign q = a^b (XOR) when enabled, else q= 0 .
Run make simulate to test the operation. Verify that the console output is correct. Then modify the testbench to use an assign statement instead of an always block . Change the type of q as appropriate for the assign statement.
Turn in your work using git :
Indicate on Canvas that your assignment is done.
Modeling Concurrent Functionality in Verilog
- First Online: 01 March 2019
Cite this chapter
- Brock J. LaMeres 2
84k Accesses
This chapter presents a set of built-in operators that will allow basic logic expressions to be modeled within a Verilog module. This chapter then presents a series of combinational logic model examples.
This is a preview of subscription content, log in via an institution to check access.
Access this chapter
- Available as PDF
- Read on any device
- Instant download
- Own it forever
- Available as EPUB and PDF
Tax calculation will be finalised at checkout
Purchases are for personal use only
Institutional subscriptions
Author information
Authors and affiliations.
Department of Electrical & Computer Engineering, Montana State University, Bozeman, MT, USA
Brock J. LaMeres
You can also search for this author in PubMed Google Scholar
Rights and permissions
Reprints and permissions
Copyright information
© 2019 Springer Nature Switzerland AG
About this chapter
LaMeres, B.J. (2019). Modeling Concurrent Functionality in Verilog. In: Quick Start Guide to Verilog. Springer, Cham. https://doi.org/10.1007/978-3-030-10552-5_3
Download citation
DOI : https://doi.org/10.1007/978-3-030-10552-5_3
Published : 01 March 2019
Publisher Name : Springer, Cham
Print ISBN : 978-3-030-10551-8
Online ISBN : 978-3-030-10552-5
eBook Packages : Engineering Engineering (R0)
Share this chapter
Anyone you share the following link with will be able to read this content:
Sorry, a shareable link is not currently available for this article.
Provided by the Springer Nature SharedIt content-sharing initiative
- Publish with us
Policies and ethics
- Find a journal
- Track your research
- The Verilog-AMS Language
- Initial and Always Processes
- Assignment Statements
Assignment Statements
Blocking assignment .
A blocking assignment evaluates the expression on its right hand side and then immediately assigns the value to the variable on its left hand side:
It is also possible to add delay to a blocking assignment. For example:
In this case, the expression on the right hand side is evaluated and the value is held for 10 units of time. During this time, the execution of the code is blocked in the middle of the assignment statement. After the 10 units of time, the value is stored in the variable on the left
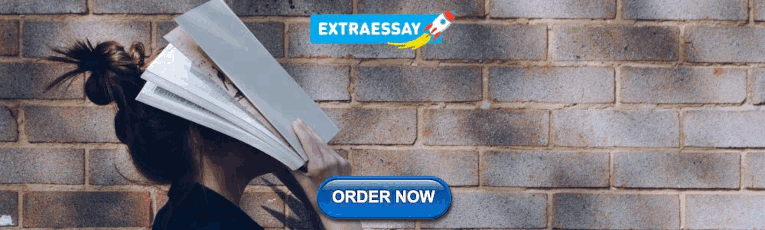
Nonblocking Assignment
A nonblocking assignment evaluates the expression on its right hand side without immediately assigning the value to the variable on the left. Instead the value is cached and execution is allowed to continue onto the next statement without performing the assignment. The assignment is deferred until the next blocking statement is encountered. In the example below, on the positive edge of clk the right-hand side of the first nonblocking assignment is evaluated and the value cached without changing a. Then the right-hand side of the second nonblocking assignment statement is evaluated is also cached without changing b. Execution continues until it returns to the event statement, once there the execution of the process blocks until the next positive edge of the clk. Just before the process blocks, the cached values finally assigned to the target variables. In this way, the following code swaps the values in a and b on every positive edge of clk:
Adding delay to nonblocking assignments is done as follows:
Using nonblocking assignment with delay in this manner is a way of implementing transport delay , as shown below:
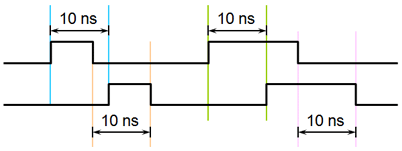
Blocking versus Nonblocking Assignment
Nonblocking statements allow you to schedule assignments without blocking the procedural flow. You can use the nonblocking procedural statement whenever you want to make several register assignments within the same time step without regard to order or dependence upon each other. It means that nonblocking statements resemble actual hardware more than blocking assignments.
Generally you would use nonblocking assignment whenever assigning to variables that are shared between multiple initial or always processes if the statements that access the variable could execute at the same time. Doing so resolves race conditions.
Blocking assignment is used to assign to temporary variables when breaking up large calculations into multiple assignment statements. For example:
Procedural Continuous Assignment
Two types of continuous assignment are available in initial and always processes: assign and force .
The target of an assign statement must be a register or a concatenation of registers. The value is continuously driven onto its target and that value takes priority over values assigned in procedural assignments. Once a value is assigned with an assign statement, it can only be changed with another assign statement or with a force statement. Execution of deassign releases the continuous assignment, meaning that the value of the register can once again be changed with procedural assignments. For example, the following implements a D-type flip-flop with set and reset:
Assign statements are used to implement set and reset because they dominate over the non-blocking assignment used to update q upon positive edges of the clock c . If instead a simple procedural assignment were used instead, then a positive edge on the clock could change q even if r or s were high.
A force statement is similar to assign , except that it can be applied to both registers and nets. It overrides all other assignments until the release statement is executed. Force is often used in testbenches to eliminate initial x-values in the DUT or to place it in a particular state. For example:
Reference Designer
- Tutorials Home
Verilog Basic Tutorial
- Verilog Introduction
- Installing Verilog and Hello World
- Simple comparator Example
- Code Verification
- Simulating with verilog
- Verilog Language and Syntax
- Verilog Syntax Contd..
- Verilog Syntax - Vector Data
- Verilog $monitor
- Verilog Gate Level Modeling
- Verilog UDP
- Verilog Bitwise Operator
- Viewing Waveforms
- Full Adder Example
- Multiplexer Example
- Always Block for Combinational ckt
- if statement for Combinational ckt
- Case statement for Combinational ckt
- Hex to 7 Segment Display
- casez and casex
- full case and parallel case
- Verilog for loop
- Verilog localparam and parameter
Sequential Ckt Tutorial
- Sequential Circuits
- Serial Shift Register
- Binary Counters
- Ring Counter
Misc Verilog Topics
- Setting Path Variable
- Verilog Tutorial Videos
- Verilog Interview questions #1
- Verilog Interview questions #2
- Verilog Interview questions #3
- Verilog Books
- Synchronous and Asynchronous Reset
- Left and Right shift >
- Negative Numbers
- Blocking Vs Non Blocking
- wand and wor
- delay in verilog
- $dumpfile and $dumpvars
- Useful Resources
- Verilog Examples
Verilog Quizs
- Verilog Quiz # 1
- Verilog Quiz # 2
- Verilog Quiz # 3
- Verilog Quiz # 4
- Verilog Quiz # 5
- Verilog Quiz # 6
- Verilog Quiz # 7
- Verilog Quiz # 8
- Verilog Quiz # 9
Other Tutorials
- Verilog Simulation with Xilinx ISE
- VHDL Tutorial
Verilog Multiplexer
Copyright © 2009 Reference Designer
Tutorials | Products | Services | Contact Us
Verilog Tutorial
Verilog code example, what is verilog .
Verilog is a hardware description language (HDL) that is used to describe digital systems and circuits in the form of code. It was developed by Gateway Design Automation in the mid-1980s and later acquired by Cadence Design Systems.
Verilog is widely used for design and verification of digital and mixed-signal systems, including both application-specific integrated circuits (ASICs) and field-programmable gate arrays (FPGAs). It supports a range of levels of abstraction, from structural to behavioral, and is used for both simulation-based design and synthesis-based design.
The language is used to describe digital circuits hierarchically, starting with the most basic elements such as logic gates and flip-flops and building up to more complex functional blocks and systems. It also supports a range of modeling techniques, including gate-level, RTL-level, and behavioral-level modeling.
What was used before Verilog ?
Before the development of Verilog, the primary hardware description language (HDL) used for digital circuit design and verification was VHDL (VHSIC Hardware Description Language). VHDL was developed in the 1980s by the U.S. Department of Defense as part of the Very High-Speed Integrated Circuit (VHSIC) program to design and test high-speed digital circuits.
VHDL is a complex language that enables designers to describe digital systems using a range of abstraction levels, from the low-level transistor and gate levels up to complex hierarchical systems. It was designed to be more descriptive and flexible than earlier HDLs, such as ABEL (Advanced Boolean Expression Language), ISP (Integrated System Synthesis Procedure), and CUPL (Compiler for Universal Programmable Logic).
Despite the development of Verilog and its increasing popularity since the 1980s, VHDL remains a widely used HDL, particularly in Europe and in the military and aerospace industries. Today, both Verilog and VHDL are widely used in digital circuit design and verification, with many companies and organizations using a combination of the two languages.
Why is Verilog better than its predecessor languages ?
Verilog introduced several important improvements over its predecessor languages, which helped make it a more popular and effective HDL for digital circuit design and verification. Here are a few reasons why Verilog is considered better than its predecessor HDLs:
How is Verilog useful ?
Verilog creates a level of abstraction that helps hide away the details of its implementation and technology.
For example, the design of a D flip-flop would require the knowledge of how the transistors need to be arranged to achieve a positive-edge triggered FF and what the rise, fall and clk-Q times required to latch the value onto a flop among many other technology oriented details. Power dissipation, timing and the ability to drive nets and other flops would also require a more thorough understanding of the physical characteristics of a transistor.
Verilog helps us to focus on the behavior and leave the rest to be sorted out later.
The following Verilog code describes the behavior of a counter. The counter counts up if the up_down signal is 1, and down if its value is 0. It also resets the counter if the signal rstn becomes 0, making it an active-low reset.
The simple example shown above illustrates how all the physical implementation details (interconnection of underlying logic gates like NAND and NOR) have been hidden while still providing a clear idea of how the counter functions.
ctr is a module that represents an up/down counter, and it is possible to choose the actual physical implementation of the design from a wide variety of different styles of flops optimized for area, power and performance. They are usually compiled into libraries and will be available for us to select within EDA tools at a later stage in the design process.
How is Verilog different from software languages like C and Java ?
Verilog is a hardware description language (HDL) used to describe digital circuits and systems, while C and Java are software programming languages used to write code that runs on general-purpose computers. Here are some of the main differences between Verilog and programming languages like C and Java:
Overall, Verilog is a specialized language designed specifically for digital circuit design and isn't used for general-purpose programming like C and Java. While there are some similarities in syntax and programming concepts between these languages, the primary focus and application of Verilog is on the design, simulation, and implementation of digital circuits and systems.
What may replace Verilog in the future ?
It's difficult to predict exactly what may replace Verilog in the future, but there are several emerging technologies and languages that may have an impact on the future of digital system design and verification.
One technology that may affect the future of digital system design is High-Level Synthesis (HLS), which is a technique for automatically generating hardware designs from high-level descriptions in languages like C, C++, and SystemC. HLS allows designers to express their design intents and functionality at a higher level of abstraction, rather than specifying the details of logic gates and register transfers in Verilog or VHDL. This could enable more efficient and rapid design of digital systems, and allow designers to explore more design space in a shorter period of time.
Another technology that may impact the future of digital system design is machine learning and artificial intelligence (AI), which have the potential to significantly streamline the design and verification process of digital systems. For example, machine learning algorithms can be used to automatically optimize and generate hardware designs, reducing the need for manual design efforts.
There are also emerging HDLs that are trying to address some of the limitations of Verilog and VHDL, such as Chisel and MyHDL, which are based on more modern programming concepts and provide higher-level abstractions.
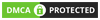
Using Continuous Assignment to Model Combinational Logic in Verilog
In this post, we talk about continuous assignment in verilog using the assign keyword. We then look at how we can model basic logic gates and multiplexors in verilog using continuous assignment.
There are two main classes of digital circuit which we can model in verilog – combinational and sequential .
Combinational logic is the simplest of the two, consisting solely of basic logic gates, such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
In contrast, sequential circuits use a clock and require storage elements such as flip flops . As a result, output changes are synchronized to the circuit clock and are not immediate.
In this post, we talk about the techniques we can use to design combinational logic circuits in verilog. In the next post, we will discuss the techniques we use to model basic sequential circuits .
Continuous Assignment in Verilog
We use continuous assignment to drive data onto verilog net types in our designs. As a result of this, we often use continuous assignment to model combinational logic circuits.
We can actually use two different methods to implement continuous assignment in verilog.
The first of these is known as explicit continuous assignment. This is the most commonly used method for continuous assignment in verilog.
In addition, we can also use implicit continuous assignment, or net declaration assignment as it is also known. This method is less common but it can allow us to write less code.
Let's look at both of these techniques in more detail.
- Explicit Continuous Assignment
We normally use the assign keyword when we want to use continuous assignment in verilog. This approach is known as explicit continuous assignment.
The verilog code below shows the general syntax for continuous assignment using the assign keyword.
The <variable> field in the code above is the name of the signal which we are assigning data to. We can only use continuous assignment to assign data to net type variables.
The <value> field can be a fixed value or we can create an expression using the verilog operators we discussed in a previous post. We can use either variable or net types in this expression.
When we use continuous assignment, the <variable> value changes whenever one of the signals in the <value> field changes state.
The code snippet below shows the most basic example of continuous assignment in verilog. In this case, whenever the b signal changes states, the value of a is updated so that it is equal to b.
- Net Declaration Assignment
We can also use implicit continuous assignment in our verilog designs. This approach is also commonly known as net declaration assignment in verilog.
When we use net declaration assignment, we place a continuous assignment in the statement which declares our signal. This can allow us to reduce the amount of code we have to write.
To use net declaration assignment in verilog, we use the = symbol to assign a value to a signal when we declare it.
The code snippet below shows the general syntax we use for net declaration assignment.
The variable and value fields have the same function for both explicit continuous assignment and net declaration assignment.
As an example, the verilog code below shows how we would use net declaration assignment to assign the value of b to signal a.
Modelling Combinational Logic Circuits in Verilog
We use continuous assignment and the verilog operators to model basic combinational logic circuits in verilog.
To show we would do this, let's look at the very basic example of a three input and gate as shown below.
To model this circuit in verilog, we use the assign keyword to drive the data on to the and_out output. This means that the and_out signal must be declared as a net type variable, such as a wire.
We can then use the bit wise and operator (&) to model the behavior of the and gate.
The code snippet below shows how we would model this three input and gate in verilog.
This example shows how simple it is to design basic combinational logic circuits in verilog. If we need to change the functionality of the logic gate, we can simply use a different verilog bit wise operator .
If we need to build a more complex combinational logic circuit, it is also possible for us to use a mixture of different bit wise operators.
To demonstrate this, let's consider the basic circuit shown below as an example.
To model this circuit in verilog, we need to use a mixture of the bit wise and (&) and or (|) operators. The code snippet below shows how we would implement this circuit in verilog.
Again, this code is relatively straight forward to understand as it makes use of the verilog bit wise operators which we discussed in the last post.
However, we need to make sure that we use brackets to model more complex logic circuit. Not only does this ensure that the circuit operates properly, it also makes our code easier to read and maintain.
Modelling Multiplexors in Verilog
Multiplexors are another component which are commonly used in combinational logic circuits.
In verilog, there are a number of ways we can model these components.
One of these methods uses a construct known as an always block . We normally use this construct to model sequential logic circuits, which is the topic of the next post in this series. Therefore, we will look at this approach in more detail the next blog post.
In the rest of this post, we will look at the other methods we can use to model multiplexors.
- Verilog Conditional Operator
As we talked about in a previous blog, there is a conditional operator in verilog . This functions in the same way as the conditional operator in the C programming language.
To use the conditional operator, we write a logical expression before the ? operator which is then evaluated to see if it is true or false.
The output is assigned to one of two values depending on whether the expression is true or false.
The verilog code below shows the general syntax which the conditional operator uses.
From this example, it is clear how we can create a basic two to one multiplexor using this operator.
However, let's look at the example of a simple 2 to 1 multiplexor as shown in the circuit diagram below.
The code snippet below shows how we would use the conditional operator to model this multiplexor in verilog.
- Nested Conditional Operators
Although this is not common, we can also write code to build larger multiplexors by nesting conditional operators.
To show how this is done, let's consider a basic 4 to 1 multiplexor as shown in the circuit below.
To model this in verilog using the conditional operator, we treat the multiplexor circuit as if it were a pair of two input multiplexors.
This means one multiplexor will select between inputs A and B whilst the other selects between C and D. Both of these multiplexors use the LSB of the address signal as the address pin.
To create the full four input multiplexor, we would then need another multiplexor.
This takes the outputs from the first two multiplexors and uses the MSB of the address signal to select between them.
The code snippet below shows the simplest way to do this. This code uses the signals mux1 and mux2 which we defined in the last example.
However, we could easily remove the mux1 and mux2 signals from this code and instead use nested conditional operators.
This reduces the amount of code that we would have to write without affecting the functionality.
The code snippet below shows how we would do this.
As we can see from this example, when we use conditional operators to model multiplexors in verilog, the code can quickly become difficult to understand. Therefore, we should only use this method to model small multiplexors.
- Arrays as Multiplexors
It is also possible for us to use verilog arrays to build simple multiplexors.
To do this we combine all of the multiplexor inputs into a single array type and use the address to point at an element in the array.
To get a better idea of how this works in practise, let's consider a basic four to one multiplexor as an example.
The first thing we must do is combine our input signals into an array. There are two ways in which we can do this.
Firstly, we can declare an array and then assign all of the individual bits, as shown in the verilog code below.
Alternatively we can use the verilog concatenation operator , which allows us to assign the entire array in one line of code.
To do this, we use a pair of curly braces - { } - and list the elements we wish to include in the array inside of them.
When we use the concatenation operator we can also declare and assign the variable in one statement, as long as we use a net type.
The verilog code below shows how we can use the concatenation operator to populate an array.
As verilog is a loosely typed language , we can use the two bit addr signal as if it were an integer type. This signal then acts as a pointer that determines which of the four elements to select.
The code snippet below demonstrates this method in practise. As the mux output is a wire, we must use continuous assignment in this instance.
What is the difference between implicit and explicit continuous assignment?
When we use implicit continuous assignment we assign the variable a value when we declare. When we use explicit continuous assignment we use the assign keyword to assign a value.
Write the code for a 2 to 1 multiplexor using any of the methods discussed we discussed.
Write the code for circuit below using both implicit and explicit continuous assignment.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
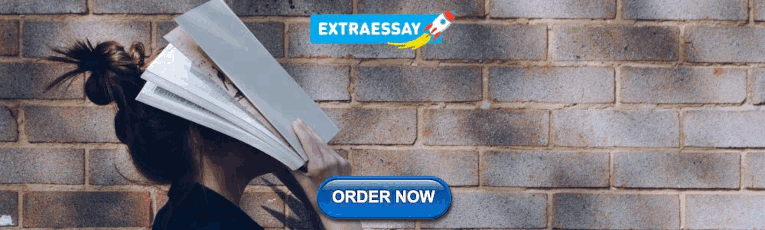
COMMENTS
The question mark is known in Verilog as a conditional operator though in other programming languages it also is referred to as a ternary operator, an inline if, ... There are examples in which it might be useful to combine two or more conditional operators in a single assignment. Consider the truth table below.
In Verilog, conditional statements are used to control the flow of execution based on certain conditions. There are several types of conditional statements in Verilog listed below. ... Readability: The conditional operator can enhance code readability, especially for simple conditional assignments. It clearly expresses the intent of assigning ...
The assign statement serves as a conditional block like an if statement you are probably used to in popular programming languages such as C or C++. The assign operator works as such: Assign my value to other values based upon if certain conditions are true. The above assign operator works as follows: If val == 2'b00, assign x to the value of a.
A case statement tests and expression and then enumerates what actions should be taken for the various values that expression can take. For example: case (sel) 0: out = in0; 1: out = in1; 2: out = in2; 3: out = in3; endcase. If the needed case is not found, then no statements are executed.
Introduction to Conditional Statements. Conditional statements in Verilog provide a way to control the flow of your code based on certain conditions. They are essential for implementing decision-making logic and creating complex behaviors in digital designs. The three primary conditional statements in Verilog are if, else if, and case .
This is used to assign values onto scalar and vector nets and happens whenever there is a change in the RHS. It provides a way to model combinational logic without specifying an interconnection of gates and makes it easier to drive the net with logical expressions. // Example model of an AND gate. wire a, b, c;
Assigned Tasks. This assignment uses only a testbench simulation, with no module to implement. Open the file src/testbench.v and examine how it is organized. It uses the conditional operator in an always block to assign q = a^b (XOR) when enabled, else q= 0.. Run make simulate to test the operation. Verify that the console output is correct.
It is also possible to specify a default case: case (sel) 0: out = in0; 1: out = in1; 2: out = in2; 3: out = in3; default: out = 'bx; endcase. The are two special versions of the case statement available: casez and casex. casex treats an x or a z in either the case expression or the case items as don't cares whereas casez only treats a z as a ...
It's much more readable your version. If you're not used to the ternary conditional operator, well you just have to get used to it. It's part of C and many C-like languages (including Perl) but it's very widely used in Verilog since it's a natural multiplex operator, and it can be used in the middle of complex expressions.
The conditional assignment allows us to have a more abstract description of certain circuits because it has the functionality of an "if" statement found in traditional computer programming languages. The conditional operator can be used in a nested form to implement more complex circuits. ... we got familiar with the Verilog conditional ...
Use continuous assignment and conditional operators. Declare your module and ports to match the block diagram provided. Use the type wire for your ports. 3.3.6. Design a Verilog model to implement the behavior described by the 4-input truth table shown in Fig. 3.6. Use continuous assignment and conditional operators. Declare your module and ...
The conditional operator (?:) is 2:1 mono-directional mux. The assign statements also mono-directional. Therefore the assignments are putting additional drivers on signals matching *_output*. The multiple conflicting drivers values are the cause of the unknown values.
Blocking Assignment. A blocking assignment evaluates the expression on its right hand side and then immediately assigns the value to the variable on its left hand side: a = b + c; It is also possible to add delay to a blocking assignment. For example: a = #10 b + c; In this case, the expression on the right hand side is evaluated and the value ...
We can extend this idea to increase the number of the control bits to 2. This 2 bit multiplexer will connect one of the 4 inputs to the out put. We will now write verilog code for a single bit multiplexer. mux.v. module Mux2_1 ( out, cntrl, in1, in2); input cntrl, in1, in2; output out; assign out = cntrl ? in1 : in2; endmodule.
@newbie: I don't think if-else versus conditional assignment affect synthesis. When it comes to debugging, it is much easier to set breakpoints on different sections of a nested if-else statement, but a conditional assignment is usually considered a single break point. ... Generate Conditional Assignment Statements in Verilog. 2. Assign vs if ...
Purpose: Verilog is used to describe digital circuits and systems, while C and Java are used to write software programs that run on computers. Syntax: Verilog has a different syntax than C and Java, as it is designed to describe the behavior of digital circuits rather than the execution of software instructions. For example, Verilog describes ...
Non-blocking statements in Verilog work in the following fashion: The expressions on the right-hand side get evaluated sequentially but they do not get assigned immediately. The assignment takes place at the end of the time step. In your example, clk_counter + 1 is evaluated but not assigned to clk_counter right away.
The verilog code below shows the general syntax for continuous assignment using the assign keyword. assign <variable> = <value>; The <variable> field in the code above is the name of the signal which we are assigning data to. We can only use continuous assignment to assign data to net type variables.
In VHDL-2008 it is allowed to be a boolean, std_logic, or bit. The condition operator (9.2.9) which can be applied implicitly (after the when in a conditional assignment statement) is predefined in package standard for type BIT. Absent declarations (or an entity header) the type type of tone is not known.
For example, in this code, when you're using a non-blocking assignment, its action won't be registered until the next clock cycle. This means that the order of the assignments is irrelevant and will produce the same result. The other assignment operator, '=', is referred to as a blocking assignment. When '=' assignment is used, for the purposes ...