Advisory boards aren’t only for executives. Join the LogRocket Content Advisory Board today →
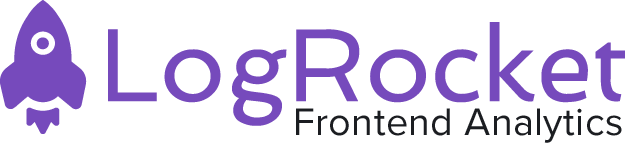
- Product Management
- Solve User-Reported Issues
- Find Issues Faster
- Optimize Conversion and Adoption
- Start Monitoring for Free
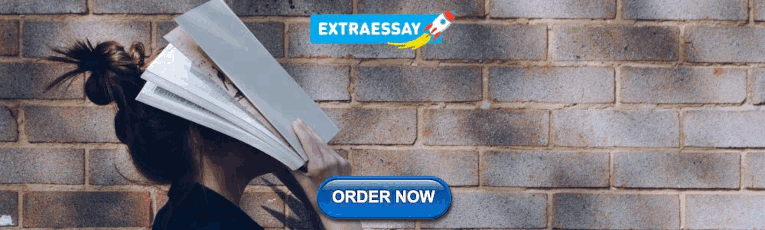
How to copy objects in JavaScript: A complete guide
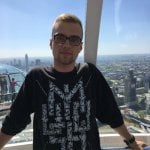
Editor’s note: This post was updated on 23 March 2022 to include updated information for copying objects in JavaScript and TypeScript, including the structured cloning technique.
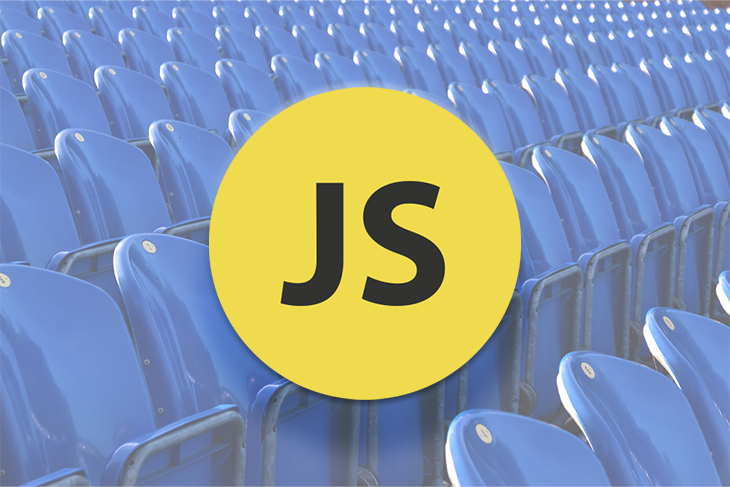
When working with functional programming, a good rule of thumb is to always create new objects instead of changing old ones. In doing so we can be sure that our meddling with the object’s structure won’t affect some seemingly unrelated part of the application, which in turn makes the entire code more predictable.
How exactly can we be sure that the changes we make to an object do not affect the code elsewhere? Removing the unwanted references altogether seems like a good idea. To get rid of a reference we need to copy all of the object’s properties to a new object. In this article, we’ll examine five techniques we can use to copy objects in JavaScript, as well as when to use each technique. Where applicable, we’ll also demonstrate how to use each technique to copy objects in TypeScript. TypeScript is basically a subset of JavaScript with static typing, but it is the preferred option for some developers. Compared to JavaScript, Typescript is generally easier to read, understand, and debug.
Here are the five JavaScript copy methods that we’ll review:
Shallow copy
- Merging with the spread operator or Object.assign() function
Structured cloning
A shallow copy of an object will have the same references as the source object from which the copy was made. As a result, when we modify either the source or the copy, we may also cause the other object to change. In other words, we may unintentionally create unexpected changes in the source or copy. It is critical to grasp the difference between selectively modifying the value of a shared property of an existing element and assigning a completely new value to an existing element.
JavaScript offers standard inbuilt object-copy operations for creating shallow copies: Array.from() , Array.prototype.concat() , Array.prototype.slice() , Object.assign() , and Object.create() , spread syntax .
Here’s an example of shallow copy in JavaScript:
Here’s an example of shallow copy in TypeScript. In this example, we copy the object using the spread operator ( … ).
Here’s another example of shallow copy in TypeScript. In this example, we create a new object and copy every property from the source object:
When to use shallow copy
Shallow copy can be used when we’re dealing with an object that only has properties with primitive data types (for example, strings or numbers). If our object contains non-primitive data types (for example, functions or arrays), it may disrupt our program.
A deep copy of an object will have properties that do not share the same references as the source object from which the copy was made. As a result, we can alter either the source or the copy without changing the other object. In other words, making a change to one object will not cause unexpected changes to either the source or copy.
To make deep copies in JavaScript, we use the JSON.stringify() and JSON.parse() methods. First, we convert the object to a JSON string using the JSON.stringify() function. Then, we parse the string with the JSON.parse() method to create a new JavaScript object:
Now, let’s look at how to make a deep copy of an object in TypeScript.
Our first example works recursively. We write a deep function, which checks the type of the argument sent to it and either calls an appropriate function for the argument (if it is an array or an object) or simply returns the value of the argument (if it is neither an array nor an object).
The deepObject function takes all of the keys of an object and iterates over them, recursively calling the deep function for each value.
So, deepArray iterates over the provided array, calling deep for every value in it.
Over 200k developers use LogRocket to create better digital experiences

Now, let’s look at another TypeScript example taking a different approach. Our goal is to create a new object without any reference to the previous one, right? Why don’t we use the JSON object then? First, we stringify the object, then parse the resulting string. What we get is a new object that is totally unaware of its origin.
It’s important to note that in the prior example the methods of the object are retained, but here they are not. Since JSON format does not support functions, they are removed altogether.
When to use deep copy
Deep copy may be used when your object contains both primitive and non-primitive data types. It can also be used anytime you feel the need to update nested objects or arrays.
The Object.assign() function can be used to copy all enumerable own properties from one or more source objects to a target object. This function returns the target object to the newObject variable.
Here’s an example of copying with the Object.assign() function in JavaScript:
Here’s an example of copying by assigning in TypeScript. Here, we just take each source object and copy its properties to the target , which we normally pass as {} in order to prevent mutation.
Here’s another example of copying by assigning in TypeScript. This example is a safe version in which, instead of mutating the target object, we create an entirely new one that we later assign to a variable. This means we don’t need to pass the target argument at all. Unfortunately, this version does not work with the keyword this because this can’t be reassigned.
When to use assigning
The Object.assign() function may be used to replicate an object that is not modified and assign some new properties to an existing object. In the above sample code, we created an empty object, {} , called target , and assigned the properties from the source object.
More great articles from LogRocket:
- Don't miss a moment with The Replay , a curated newsletter from LogRocket
- Learn how LogRocket's Galileo cuts through the noise to proactively resolve issues in your app
- Use React's useEffect to optimize your application's performance
- Switch between multiple versions of Node
- Discover how to use the React children prop with TypeScript
- Explore creating a custom mouse cursor with CSS
- Advisory boards aren’t just for executives. Join LogRocket’s Content Advisory Board. You’ll help inform the type of content we create and get access to exclusive meetups, social accreditation, and swag.
The merge method is similar to the assign method, but instead of altering properties in the target, it joins them together. If a value is an array or an object, this function merges the attributes in a recursive manner. There are two ways to merge objects in JavaScript: using the spread operator or the Object.assign() method.
Spread operator
The spread operator, ... , was implemented in ES6 and can be used to merge two or more objects into one new object that will have the properties of the merged objects. If two objects have the same property name, the latter object property will overwrite the former.
Here’s an example of merging with the spread operator in JavaScript:
Now, let’s look at an example of merging in TypeScript.
The function mergeValues accepts two arguments: target and source . If both values are objects we call and return mergeObjects with the aforementioned target and source as arguments. Analogically, when both values are arrays we call and return mergeArrays . If the source is undefined we just keep whatever value was previously there which means we return the target argument. If none of the above applies we just return the source argument.
Both mergeArrays and mergeObjects work the same way: we take the source properties and set them under the same key in the target .
Now all that is left to do is to create a TypeScript merge function:
Object.assign() method
The Object.assign() method can be used to merge two objects and copy the result to a new target. Just like the spread operator, If the source objects have the same property name, the latter object will replace the preceding object.
Here’s an example:
Now, let’s look at another example of merging in Typescript. With this approach, we want to first get all the properties of the source object , even if they are nested three objects deep , and save a path to the properties. This will later allow us to set the value at the proper path inside the target object.
A path is an array of strings that looks something like this: [‘firstObject’,‘secondObject’, ‘propertyName’] .
Here’s an example of how this works:
We call the getValue function to get an array of objects that contain paths and values of the properties. If the argument value is null or is not object-like, we can’t go any deeper so we return an object containing the argument value and its path.
Otherwise, if the argument is object-like and not null , we can be sure it is either an array or an object. If it is an array, we call getArrayValues . If it is an object , we call getObjectValues .
Both getArrayValues and getObjectValues iterate over properties calling getValue for each with the current index / key now appended to the path .
After getting the paths and values of an entire source object we can see that they are deeply nested. Still, we’d like to keep all of them in a single array. This means that we need to flatten the array.
Flattening an array boils down to iterating over each item to check if it is an array. If it is we flatten it and then concat the value to the result array.
Now that we’ve covered how to get the path , let’s consider how to set all these properties in the target object.
Let’s talk about the setAtPath function that we are going to use to set the values at their respective paths. We want to get access to the last property of the path to set the value. To do so, we need to go over the path’s items, its properties’ names, and each time get the property’s value. We start the reduce function with the target object which is then available as the result argument.
Each time we return the value under result[key] it becomes the result argument in the next iteration. This way, when we get to the last item of the path the result argument is the object or array where we set the value.
In our example the result argument, for each iteration, would be: target -> firstObject -> secondObject .
We have to keep in mind that the target might be an empty object whereas sources can be many levels deep. This means we might have to recreate an object’s or an array’s structure ourselves before setting a value.
We set the value at the last item of the path and return the object we started with.
If inside the firstObject there were no secondObject , we would get undefined and then an error if we tried to set undefined[‘property’] . To prevent this, we first check if result[key] exists. If it does not exist, we’ll need to create it as either an object or as an array. If the type of the next item is a 'number' (effectively an index), then we’ll need to create an array. If it is a string, we’ll create an object.
Now, all that is left to do is to create the merge function which ties everything together.
When to use merging
Merging objects is not a typical practice in JavaScript, but this method enables us to combine object properties, even with very deeply nested objects.
Structured cloning is a new technique for copying objects in JavaScript. It is a global method that uses the structured clone algorithm to create a deep copy of a specified item. Rather than cloning objects, it transfers objects from their original source to a new source where they are no longer accessible in the original source.
This technique may be used with transferable objects , which is a type of object that owns resources. These objects can only be transferred using the original parameter’s transfer value. As a result of the transfer, the original object will be rendered unusable.
In the below example, the code would would transfer Pascal from the passed in value, but not Akunne :
When to use structured cloning
Structured cloning can be useful for cases when you need to asynchronously validate data in a buffer before saving the data. To avoid the buffer being modified before the data is saved, you can clone the buffer and validate that data. This technique can also be useful if you are transferring the data. With structured cloning, any attempts to modify the original buffer will fail, preventing its accidental misuse.
In this article, we discussed five useful techniques to copy an object in JavaScript as well as TypeScript. We use shallow copy when dealing with an object that only has properties with primitive data types (strings or numbers). Deep copy ensures that there are no references to the source object or any of its properties. Assign is a great way to replicate an object or just to assign some new properties to an existing object. Merge allows us to merge properties of objects, even if the objects are deeply nested. Finally, structured cloning allows us to asynchronously validate and transfer object data, which then renders the original object unusable.
Objects are the basic method by which we organize and transmit data in JavaScript. They are represented in TypeScript via object types ( result: object ). Whether you choose to copy objects in JavaScript or TypeScript, hopefully, this guide has provided you with options to consider for multiple use cases. If you are familiar with other techniques for copying objects in JavaScript, please share them in the comments section.
LogRocket : Debug JavaScript errors more easily by understanding the context
Debugging code is always a tedious task. But the more you understand your errors, the easier it is to fix them.
LogRocket allows you to understand these errors in new and unique ways. Our frontend monitoring solution tracks user engagement with your JavaScript frontends to give you the ability to see exactly what the user did that led to an error.
LogRocket records console logs, page load times, stack traces, slow network requests/responses with headers + bodies, browser metadata, and custom logs. Understanding the impact of your JavaScript code will never be easier!
Try it for free .
Share this:
- Click to share on Twitter (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Facebook (Opens in new window)
- #vanilla javascript
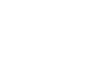
Stop guessing about your digital experience with LogRocket
Recent posts:.
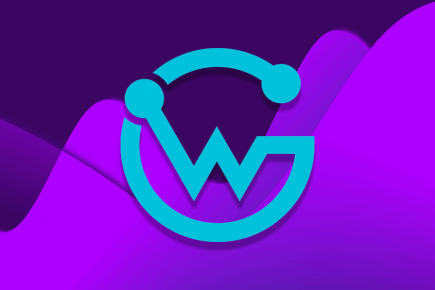
How to integrate WunderGraph with your frontend application
Unify and simplify APIs using WunderGraph to integrate REST, GraphQL, and databases in a single endpoint.
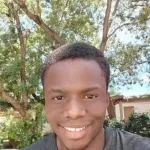
Understanding the latest Webkit features in Safari 17.4
The Safari 17.4 update brought in many modern features and bug fixes. Explore the major development-specific updates you should be aware of.
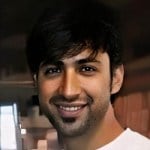
Using WebRTC to implement P2P video streaming
Explore one of WebRTC’s major use cases in this step-by-step tutorial: live peer-to-peer audio and video streaming between systems.
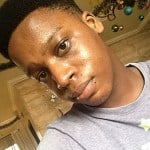
htmx vs. React: Choosing the right library for your project
Both htmx and React provide powerful tools for building web apps, but in different ways that are suited to different types of projects.
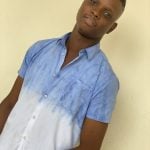
One Reply to "How to copy objects in JavaScript: A complete guide"
How is spread syntax. is a shallow copy!
Leave a Reply Cancel reply
Cloning Arrays in JavaScript
- Post author By John Au-Yeung
- Post date December 31, 2019
- No Comments on Cloning Arrays in JavaScript

There are a few ways to clone an array in JavaScript,
Object.assign
Object.assign allows us to make a shallow copy of any kind of object including arrays.
For example:
Array.slice
The Array.slice function returns a copy of the original array.
The Array.slice function returns a copy of the original array. It takes array like objects like Set and it also takes an array as an argument.
Spread Operator
The fastest way to copy an array, which is available with ES6 or later, is the spread operator.
JSON.parse and JSON.stringify
This allows for deep copy of an array and only works if the objects in the array are plain objects. It can be used like this:
Related Posts
Iterating Array For Loop The for loop is one of the most basic loops for…
There are a few simple ways to check if an object is an array. Array.isArray…
By John Au-Yeung
Web developer specializing in React, Vue, and front end development.
Object references and copying
One of the fundamental differences of objects versus primitives is that objects are stored and copied “by reference”, whereas primitive values: strings, numbers, booleans, etc – are always copied “as a whole value”.
That’s easy to understand if we look a bit under the hood of what happens when we copy a value.
Let’s start with a primitive, such as a string.
Here we put a copy of message into phrase :
As a result we have two independent variables, each one storing the string "Hello!" .
Quite an obvious result, right?
Objects are not like that.
A variable assigned to an object stores not the object itself, but its “address in memory” – in other words “a reference” to it.
Let’s look at an example of such a variable:
And here’s how it’s actually stored in memory:
The object is stored somewhere in memory (at the right of the picture), while the user variable (at the left) has a “reference” to it.
We may think of an object variable, such as user , like a sheet of paper with the address of the object on it.
When we perform actions with the object, e.g. take a property user.name , the JavaScript engine looks at what’s at that address and performs the operation on the actual object.
Now here’s why it’s important.
When an object variable is copied, the reference is copied, but the object itself is not duplicated.
For instance:
Now we have two variables, each storing a reference to the same object:
As you can see, there’s still one object, but now with two variables that reference it.
We can use either variable to access the object and modify its contents:
It’s as if we had a cabinet with two keys and used one of them ( admin ) to get into it and make changes. Then, if we later use another key ( user ), we are still opening the same cabinet and can access the changed contents.
Comparison by reference
Two objects are equal only if they are the same object.
For instance, here a and b reference the same object, thus they are equal:
And here two independent objects are not equal, even though they look alike (both are empty):
For comparisons like obj1 > obj2 or for a comparison against a primitive obj == 5 , objects are converted to primitives. We’ll study how object conversions work very soon, but to tell the truth, such comparisons are needed very rarely – usually they appear as a result of a programming mistake.
An important side effect of storing objects as references is that an object declared as const can be modified.
It might seem that the line (*) would cause an error, but it does not. The value of user is constant, it must always reference the same object, but properties of that object are free to change.
In other words, the const user gives an error only if we try to set user=... as a whole.
That said, if we really need to make constant object properties, it’s also possible, but using totally different methods. We’ll mention that in the chapter Property flags and descriptors .
Cloning and merging, Object.assign
So, copying an object variable creates one more reference to the same object.
But what if we need to duplicate an object?
We can create a new object and replicate the structure of the existing one, by iterating over its properties and copying them on the primitive level.
We can also use the method Object.assign .
The syntax is:
- The first argument dest is a target object.
- Further arguments is a list of source objects.
It copies the properties of all source objects into the target dest , and then returns it as the result.
For example, we have user object, let’s add a couple of permissions to it:
If the copied property name already exists, it gets overwritten:
We also can use Object.assign to perform a simple object cloning:
Here it copies all properties of user into the empty object and returns it.
There are also other methods of cloning an object, e.g. using the spread syntax clone = {...user} , covered later in the tutorial.
Nested cloning
Until now we assumed that all properties of user are primitive. But properties can be references to other objects.
Now it’s not enough to copy clone.sizes = user.sizes , because user.sizes is an object, and will be copied by reference, so clone and user will share the same sizes:
To fix that and make user and clone truly separate objects, we should use a cloning loop that examines each value of user[key] and, if it’s an object, then replicate its structure as well. That is called a “deep cloning” or “structured cloning”. There’s structuredClone method that implements deep cloning.
structuredClone
The call structuredClone(object) clones the object with all nested properties.
Here’s how we can use it in our example:
The structuredClone method can clone most data types, such as objects, arrays, primitive values.
It also supports circular references, when an object property references the object itself (directly or via a chain or references).
As you can see, clone.me references the clone , not the user ! So the circular reference was cloned correctly as well.
Although, there are cases when structuredClone fails.
For instance, when an object has a function property:
Function properties aren’t supported.
To handle such complex cases we may need to use a combination of cloning methods, write custom code or, to not reinvent the wheel, take an existing implementation, for instance _.cloneDeep(obj) from the JavaScript library lodash .
Objects are assigned and copied by reference. In other words, a variable stores not the “object value”, but a “reference” (address in memory) for the value. So copying such a variable or passing it as a function argument copies that reference, not the object itself.
All operations via copied references (like adding/removing properties) are performed on the same single object.
To make a “real copy” (a clone) we can use Object.assign for the so-called “shallow copy” (nested objects are copied by reference) or a “deep cloning” function structuredClone or use a custom cloning implementation, such as _.cloneDeep(obj) .
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Understanding Object.assign() Method in JavaScript
Cloning an object, merging objects, converting an array to an object, browser compatibility.
The Object.assign() method was introduced in ES6 that copies all enumerable own properties from one or more source objects to a target object, and returns the target object .
The Object.assign() method invokes the getters on the source objects and setters on the target object. It assigns properties only, not copying or defining new properties.
The properties in the target object are overwritten by the properties in source objects if they have the same key. Similarly, the later source objects' properties are overwritten by the earlier ones.
The Object.assign() method handles null and undefined source values gracefully, and doesn't throw any exception.
Here is how the syntax of Object.assign() looks like:
- target — The target object that is modified and returned after applying the sources' properties.
- sources — The source object(s) containing the properties you want to apply to the target object.
The Object.assign() method is one of the four ways, I explained earlier, to clone an object in JavaScript.
The following example demonstrates how you can use Object.assign() to clone an object:
Remember that Object.assign() only creates a shallow clone of the object and not a deep clone.
To create a deep clone, you can either use JSON methods or a 3rd-party library like Lodash .
The Object.assign() method can also merge multiple source objects into a target object. If you do not want to modify the target object, just pass an empty ( {} ) object as target as shown below:
If the source objects have same properties , they are overwritten by the properties of the objects later in the parameters order:
Lastly, you could also use the Object.assign() method to convert an array to an object in JavaScript. The array indexes are converted to object keys, and array values are converted to object values:
As Object.assign() is part of ES6, it only works in modern browsers. To support older browsers like IE, you can use a polyfill available on MDN.
To learn more about JavaScript objects, prototypes, and classes, take a look at this guide .
Read Next: Understanding Array.from() Method in JavaScript
✌️ Like this article? Follow me on Twitter and LinkedIn . You can also subscribe to RSS Feed .
You might also like...
- Get the length of a Map in JavaScript
- Delete an element from a Map in JavaScript
- Get the first element of a Map in JavaScript
- Get an element from a Map using JavaScript
- Update an element in a Map using JavaScript
- Add an element to a Map in JavaScript
The simplest cloud platform for developers & teams. Start with a $200 free credit.
Buy me a coffee ☕
If you enjoy reading my articles and want to help me out paying bills, please consider buying me a coffee ($5) or two ($10). I will be highly grateful to you ✌️
Enter the number of coffees below:
✨ Learn to build modern web applications using JavaScript and Spring Boot
I started this blog as a place to share everything I have learned in the last decade. I write about modern JavaScript, Node.js, Spring Boot, core Java, RESTful APIs, and all things web development.
The newsletter is sent every week and includes early access to clear, concise, and easy-to-follow tutorials, and other stuff I think you'd enjoy! No spam ever, unsubscribe at any time.
- JavaScript, Node.js & Spring Boot
- In-depth tutorials
- Super-handy protips
- Cool stuff around the web
- 1-click unsubscribe
- No spam, free-forever!
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
Create shallow and deep copy of an array in JavaScript
This post will discuss how to create a shallow and deep copy of an array in JavaScript.
With a shallow copy, the values are copied by reference when the array is nested. This means that if the referenced object is changed, the changes are reflected in both the original array and its copy. There are several ways to create a shallow and deep copy of an array in JavaScript. All the functions below copies any nested objects or arrays by reference except the JSON functions, jQuery’s $.extend() function with the first argument set to true , and the Lodash’s _.cloneDeep() function.
1. Using Array.slice() function
The slice() is commonly used built-in function that returns a shallow copy of a portion of an array into a new array object. We can use this function to copy the whole array by omitting the start and end arguments, or specify a range of elements to copy.
Download Run Code
2. Using Spread operator
The spread operator (…) is a feature of ES6 syntax that allows us to expand an iterable object into individual elements. We can use this operator inside an array literal ([]) to create a new array with the same elements as the original array, while offering the best performance.
Instead of using the array literal, we can use the spread operator with the Array.of() function, which creates a new Array instance from the expanded sequence of elements.
3. Using Array.from() function
The Array.from() is a built-in function that allows us to create a new array from an array-like or iterable object. We can use this function to copy an array by passing it as the first argument. We can also optionally provide a map() function as the second argument to modify the elements of the new array.
4. Using Array.concat() function
The Array.concat() is a built-in function that returns a new array that is the result of merging two or more arrays. We can use this function to copy an array by passing an empty array as the first argument and the original array as the second argument.
We can also use the concat() function in another way. If no parameter is specified, the concat() function returns a shallow copy of the calling array.
5. Using Object.assign() or Object.values() function
The Object.assign() is a built-in function that copies the values of all enumerable own properties from one or more source objects to a target object. We can use this function to copy an array by passing an empty array as the target object and the original array as the source object.
The Object.values() is a built-in function that returns an array of the values of the enumerable own properties of an object. We can use this function to extract the values of the array object.
6. Using Array.map() function
The Array.map() is a built-in function that creates a new array with the results of calling a callback function on every element in the original array. We can use this function to copy the elements of an array by passing an identity function as a callback.
7. Using JSON
The JSON.parse() and JSON.stringify() are the built-in functions that convert an object or an array to a JSON string and vice versa. We can use these functions to create a deep copy of an array by first converting it to a JSON string and then parsing it back to an array. This works with any type of value, but it will lose any functions or symbols in the array:
8. Using jQuery
The $.extend() function from the jQuery library copies the values of all enumerable own properties from one or more source objects to a target object. We can use this function to copy an array by passing an empty array as the target object and the original array as the source object. We can also pass a true value as the first argument to perform a deep copy of nested arrays or objects.
Download Code
9. Using Lodash/Underscore Library
With Lodash library, we can use the _.extend() or _.assign() or _.clone() function to get a shallow copy of an array. These functions work with any type of value, and they can preserve functions and symbols in the array. To duplicate any nested objects or arrays, we should use the _.cloneDeep function instead, which returns a deep copy.
The underscore library also offers the _.extend() , and _.clone() function, to create a shallow-copy of the specified object. However, the underscore library currently does not have any function that can perform a deep copy operation on an array.
That’s all about creating a shallow and deep copy of an array in JavaScript.
Create a shallow and deep copy of object array in JavaScript
Get a deep copy of an array in JavaScript
Copy a 2-dimensional array in JavaScript
Rate this post
Average rating 4.88 /5. Vote count: 25
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
Home » JavaScript Object Methods » JavaScript Object.assign()
JavaScript Object.assign()
Summary : in this tutorial, you will learn how to use the JavaScript Object.assign() method in ES6.
The following shows the syntax of the Object.assign() method:
The Object.assign() copies all enumerable and own properties from the source objects to the target object. It returns the target object.
The Object.assign() invokes the getters on the source objects and setters on the target. It assigns properties only, not copying or defining new properties.
Using JavaScript Object.assign() to clone an object
The following example uses the Object.assign() method to clone an object .
Note that the Object.assign() only carries a shallow clone, not a deep clone.
Using JavaScript Object.assign() to merge objects
The Object.assign() can merge source objects into a target object which has properties consisting of all the properties of the source objects. For example:
If the source objects have the same property, the property of the later object overwrites the earlier one:
- Object.assign() assigns enumerable and own properties from a source object to a target object.
- Object.assign() can be used to clone an object or merge objects .
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Baseline Widely available
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The Array object, as with arrays in other programming languages, enables storing a collection of multiple items under a single variable name , and has members for performing common array operations .
Description
In JavaScript, arrays aren't primitives but are instead Array objects with the following core characteristics:
- JavaScript arrays are resizable and can contain a mix of different data types . (When those characteristics are undesirable, use typed arrays instead.)
- JavaScript arrays are not associative arrays and so, array elements cannot be accessed using arbitrary strings as indexes, but must be accessed using nonnegative integers (or their respective string form) as indexes.
- JavaScript arrays are zero-indexed : the first element of an array is at index 0 , the second is at index 1 , and so on — and the last element is at the value of the array's length property minus 1 .
- JavaScript array-copy operations create shallow copies . (All standard built-in copy operations with any JavaScript objects create shallow copies, rather than deep copies ).
Array indices
Array objects cannot use arbitrary strings as element indexes (as in an associative array ) but must use nonnegative integers (or their respective string form). Setting or accessing via non-integers will not set or retrieve an element from the array list itself, but will set or access a variable associated with that array's object property collection . The array's object properties and list of array elements are separate, and the array's traversal and mutation operations cannot be applied to these named properties.
Array elements are object properties in the same way that toString is a property (to be specific, however, toString() is a method). Nevertheless, trying to access an element of an array as follows throws a syntax error because the property name is not valid:
JavaScript syntax requires properties beginning with a digit to be accessed using bracket notation instead of dot notation . It's also possible to quote the array indices (e.g., years['2'] instead of years[2] ), although usually not necessary.
The 2 in years[2] is coerced into a string by the JavaScript engine through an implicit toString conversion. As a result, '2' and '02' would refer to two different slots on the years object, and the following example could be true :
Only years['2'] is an actual array index. years['02'] is an arbitrary string property that will not be visited in array iteration.
Relationship between length and numerical properties
A JavaScript array's length property and numerical properties are connected.
Several of the built-in array methods (e.g., join() , slice() , indexOf() , etc.) take into account the value of an array's length property when they're called.
Other methods (e.g., push() , splice() , etc.) also result in updates to an array's length property.
When setting a property on a JavaScript array when the property is a valid array index and that index is outside the current bounds of the array, the engine will update the array's length property accordingly:
Increasing the length extends the array by adding empty slots without creating any new elements — not even undefined .
Decreasing the length property does, however, delete elements.
This is explained further on the length page.
Array methods and empty slots
Array methods have different behaviors when encountering empty slots in sparse arrays . In general, older methods (e.g. forEach ) treat empty slots differently from indices that contain undefined .
Methods that have special treatment for empty slots include the following: concat() , copyWithin() , every() , filter() , flat() , flatMap() , forEach() , indexOf() , lastIndexOf() , map() , reduce() , reduceRight() , reverse() , slice() , some() , sort() , and splice() . Iteration methods such as forEach don't visit empty slots at all. Other methods, such as concat , copyWithin , etc., preserve empty slots when doing the copying, so in the end the array is still sparse.
Newer methods (e.g. keys ) do not treat empty slots specially and treat them as if they contain undefined . Methods that conflate empty slots with undefined elements include the following: entries() , fill() , find() , findIndex() , findLast() , findLastIndex() , includes() , join() , keys() , toLocaleString() , toReversed() , toSorted() , toSpliced() , values() , and with() .
Copying methods and mutating methods
Some methods do not mutate the existing array that the method was called on, but instead return a new array. They do so by first constructing a new array and then populating it with elements. The copy always happens shallowly — the method never copies anything beyond the initially created array. Elements of the original array(s) are copied into the new array as follows:
- Objects: the object reference is copied into the new array. Both the original and new array refer to the same object. That is, if a referenced object is modified, the changes are visible to both the new and original arrays.
- Primitive types such as strings, numbers and booleans (not String , Number , and Boolean objects): their values are copied into the new array.
Other methods mutate the array that the method was called on, in which case their return value differs depending on the method: sometimes a reference to the same array, sometimes the length of the new array.
The following methods create new arrays by accessing this.constructor[Symbol.species] to determine the constructor to use: concat() , filter() , flat() , flatMap() , map() , slice() , and splice() (to construct the array of removed elements that's returned).
The following methods always create new arrays with the Array base constructor: toReversed() , toSorted() , toSpliced() , and with() .
The following table lists the methods that mutate the original array, and the corresponding non-mutating alternative:
An easy way to change a mutating method into a non-mutating alternative is to use the spread syntax or slice() to create a copy first:
Iterative methods
Many array methods take a callback function as an argument. The callback function is called sequentially and at most once for each element in the array, and the return value of the callback function is used to determine the return value of the method. They all share the same signature:
Where callbackFn takes three arguments:
The current element being processed in the array.
The index of the current element being processed in the array.
The array that the method was called upon.
What callbackFn is expected to return depends on the array method that was called.
The thisArg argument (defaults to undefined ) will be used as the this value when calling callbackFn . The this value ultimately observable by callbackFn is determined according to the usual rules : if callbackFn is non-strict , primitive this values are wrapped into objects, and undefined / null is substituted with globalThis . The thisArg argument is irrelevant for any callbackFn defined with an arrow function , as arrow functions don't have their own this binding .
The array argument passed to callbackFn is most useful if you want to read another index during iteration, because you may not always have an existing variable that refers to the current array. You should generally not mutate the array during iteration (see mutating initial array in iterative methods ), but you can also use this argument to do so. The array argument is not the array that is being built, in the case of methods like map() , filter() , and flatMap() — there is no way to access the array being built from the callback function.
All iterative methods are copying and generic , although they behave differently with empty slots .
The following methods are iterative: every() , filter() , find() , findIndex() , findLast() , findLastIndex() , flatMap() , forEach() , map() , and some() .
In particular, every() , find() , findIndex() , findLast() , findLastIndex() , and some() do not always invoke callbackFn on every element — they stop iteration as soon as the return value is determined.
The reduce() and reduceRight() methods also take a callback function and run it at most once for each element in the array, but they have slightly different signatures from typical iterative methods (for example, they don't accept thisArg ).
The sort() method also takes a callback function, but it is not an iterative method. It mutates the array in-place, doesn't accept thisArg , and may invoke the callback multiple times on an index.
Iterative methods iterate the array like the following (with a lot of technical details omitted):
Note the following:
- Not all methods do the i in this test. The find , findIndex , findLast , and findLastIndex methods do not, but other methods do.
- The length is memorized before the loop starts. This affects how insertions and deletions during iteration are handled (see mutating initial array in iterative methods ).
- The method doesn't memorize the array contents, so if any index is modified during iteration, the new value might be observed.
- The code above iterates the array in ascending order of index. Some methods iterate in descending order of index ( for (let i = length - 1; i >= 0; i--) ): reduceRight() , findLast() , and findLastIndex() .
- reduce and reduceRight have slightly different signatures and do not always start at the first/last element.
Generic array methods
Array methods are always generic — they don't access any internal data of the array object. They only access the array elements through the length property and the indexed elements. This means that they can be called on array-like objects as well.
Normalization of the length property
The length property is converted to an integer and then clamped to the range between 0 and 2 53 - 1. NaN becomes 0 , so even when length is not present or is undefined , it behaves as if it has value 0 .
The language avoids setting length to an unsafe integer . All built-in methods will throw a TypeError if length will be set to a number greater than 2 53 - 1. However, because the length property of arrays throws an error if it's set to greater than 2 32 - 1, the safe integer threshold is usually not reached unless the method is called on a non-array object.
Some array methods set the length property of the array object. They always set the value after normalization, so length always ends as an integer.
Array-like objects
The term array-like object refers to any object that doesn't throw during the length conversion process described above. In practice, such object is expected to actually have a length property and to have indexed elements in the range 0 to length - 1 . (If it doesn't have all indices, it will be functionally equivalent to a sparse array .) Any integer index less than zero or greater than length - 1 is ignored when an array method operates on an array-like object.
Many DOM objects are array-like — for example, NodeList and HTMLCollection . The arguments object is also array-like. You can call array methods on them even if they don't have these methods themselves.
Constructor
Creates a new Array object.
Static properties
Returns the Array constructor.
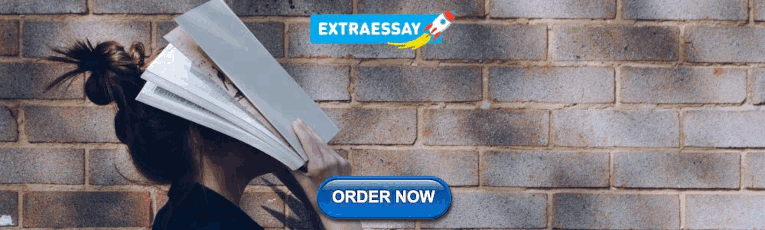
Static methods
Creates a new Array instance from an iterable or array-like object.
Creates a new Array instance from an async iterable, iterable, or array-like object.
Returns true if the argument is an array, or false otherwise.
Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments.
Instance properties
These properties are defined on Array.prototype and shared by all Array instances.
The constructor function that created the instance object. For Array instances, the initial value is the Array constructor.
Contains property names that were not included in the ECMAScript standard prior to the ES2015 version and that are ignored for with statement-binding purposes.
These properties are own properties of each Array instance.
Reflects the number of elements in an array.
Instance methods
Returns the array item at the given index. Accepts negative integers, which count back from the last item.
Returns a new array that is the calling array joined with other array(s) and/or value(s).
Copies a sequence of array elements within an array.
Returns a new array iterator object that contains the key/value pairs for each index in an array.
Returns true if every element in the calling array satisfies the testing function.
Fills all the elements of an array from a start index to an end index with a static value.
Returns a new array containing all elements of the calling array for which the provided filtering function returns true .
Returns the value of the first element in the array that satisfies the provided testing function, or undefined if no appropriate element is found.
Returns the index of the first element in the array that satisfies the provided testing function, or -1 if no appropriate element was found.
Returns the value of the last element in the array that satisfies the provided testing function, or undefined if no appropriate element is found.
Returns the index of the last element in the array that satisfies the provided testing function, or -1 if no appropriate element was found.
Returns a new array with all sub-array elements concatenated into it recursively up to the specified depth.
Returns a new array formed by applying a given callback function to each element of the calling array, and then flattening the result by one level.
Calls a function for each element in the calling array.
Determines whether the calling array contains a value, returning true or false as appropriate.
Returns the first (least) index at which a given element can be found in the calling array.
Joins all elements of an array into a string.
Returns a new array iterator that contains the keys for each index in the calling array.
Returns the last (greatest) index at which a given element can be found in the calling array, or -1 if none is found.
Returns a new array containing the results of invoking a function on every element in the calling array.
Removes the last element from an array and returns that element.
Adds one or more elements to the end of an array, and returns the new length of the array.
Executes a user-supplied "reducer" callback function on each element of the array (from left to right), to reduce it to a single value.
Executes a user-supplied "reducer" callback function on each element of the array (from right to left), to reduce it to a single value.
Reverses the order of the elements of an array in place . (First becomes the last, last becomes first.)
Removes the first element from an array and returns that element.
Extracts a section of the calling array and returns a new array.
Returns true if at least one element in the calling array satisfies the provided testing function.
Sorts the elements of an array in place and returns the array.
Adds and/or removes elements from an array.
Returns a localized string representing the calling array and its elements. Overrides the Object.prototype.toLocaleString() method.
Returns a new array with the elements in reversed order, without modifying the original array.
Returns a new array with the elements sorted in ascending order, without modifying the original array.
Returns a new array with some elements removed and/or replaced at a given index, without modifying the original array.
Returns a string representing the calling array and its elements. Overrides the Object.prototype.toString() method.
Adds one or more elements to the front of an array, and returns the new length of the array.
Returns a new array iterator object that contains the values for each index in the array.
Returns a new array with the element at the given index replaced with the given value, without modifying the original array.
An alias for the values() method by default.
This section provides some examples of common array operations in JavaScript.
Note: If you're not yet familiar with array basics, consider first reading JavaScript First Steps: Arrays , which explains what arrays are , and includes other examples of common array operations.
Create an array
This example shows three ways to create new array: first using array literal notation , then using the Array() constructor, and finally using String.prototype.split() to build the array from a string.
Create a string from an array
This example uses the join() method to create a string from the fruits array.
Access an array item by its index
This example shows how to access items in the fruits array by specifying the index number of their position in the array.
Find the index of an item in an array
This example uses the indexOf() method to find the position (index) of the string "Banana" in the fruits array.
Check if an array contains a certain item
This example shows two ways to check if the fruits array contains "Banana" and "Cherry" : first with the includes() method, and then with the indexOf() method to test for an index value that's not -1 .
Append an item to an array
This example uses the push() method to append a new string to the fruits array.
Remove the last item from an array
This example uses the pop() method to remove the last item from the fruits array.
Note: pop() can only be used to remove the last item from an array. To remove multiple items from the end of an array, see the next example.
Remove multiple items from the end of an array
This example uses the splice() method to remove the last 3 items from the fruits array.
Truncate an array down to just its first N items
This example uses the splice() method to truncate the fruits array down to just its first 2 items.
Remove the first item from an array
This example uses the shift() method to remove the first item from the fruits array.
Note: shift() can only be used to remove the first item from an array. To remove multiple items from the beginning of an array, see the next example.
Remove multiple items from the beginning of an array
This example uses the splice() method to remove the first 3 items from the fruits array.
Add a new first item to an array
This example uses the unshift() method to add, at index 0 , a new item to the fruits array — making it the new first item in the array.
Remove a single item by index
This example uses the splice() method to remove the string "Banana" from the fruits array — by specifying the index position of "Banana" .
Remove multiple items by index
This example uses the splice() method to remove the strings "Banana" and "Strawberry" from the fruits array — by specifying the index position of "Banana" , along with a count of the number of total items to remove.
Replace multiple items in an array
This example uses the splice() method to replace the last 2 items in the fruits array with new items.
Iterate over an array
This example uses a for...of loop to iterate over the fruits array, logging each item to the console.
But for...of is just one of many ways to iterate over any array; for more ways, see Loops and iteration , and see the documentation for the every() , filter() , flatMap() , map() , reduce() , and reduceRight() methods — and see the next example, which uses the forEach() method.
Call a function on each element in an array
This example uses the forEach() method to call a function on each element in the fruits array; the function causes each item to be logged to the console, along with the item's index number.
Merge multiple arrays together
This example uses the concat() method to merge the fruits array with a moreFruits array, to produce a new combinedFruits array. Notice that fruits and moreFruits remain unchanged.
Copy an array
This example shows three ways to create a new array from the existing fruits array: first by using spread syntax , then by using the from() method, and then by using the slice() method.
All built-in array-copy operations ( spread syntax , Array.from() , Array.prototype.slice() , and Array.prototype.concat() ) create shallow copies . If you instead want a deep copy of an array, you can use JSON.stringify() to convert the array to a JSON string, and then JSON.parse() to convert the string back into a new array that's completely independent from the original array.
You can also create deep copies using the structuredClone() method, which has the advantage of allowing transferable objects in the source to be transferred to the new copy, rather than just cloned.
Finally, it's important to understand that assigning an existing array to a new variable doesn't create a copy of either the array or its elements. Instead the new variable is just a reference, or alias, to the original array; that is, the original array's name and the new variable name are just two names for the exact same object (and so will always evaluate as strictly equivalent ). Therefore, if you make any changes at all either to the value of the original array or to the value of the new variable, the other will change, too:
Creating a two-dimensional array
The following creates a chessboard as a two-dimensional array of strings. The first move is made by copying the 'p' in board[6][4] to board[4][4] . The old position at [6][4] is made blank.
Here is the output:
Using an array to tabulate a set of values
Creating an array using the result of a match.
The result of a match between a RegExp and a string can create a JavaScript array that has properties and elements which provide information about the match. Such an array is returned by RegExp.prototype.exec() and String.prototype.match() .
For example:
For more information about the result of a match, see the RegExp.prototype.exec() and String.prototype.match() pages.
Mutating initial array in iterative methods
Iterative methods do not mutate the array on which it is called, but the function provided as callbackFn can. The key principle to remember is that only indexes between 0 and arrayLength - 1 are visited, where arrayLength is the length of the array at the time the array method was first called, but the element passed to the callback is the value at the time the index is visited. Therefore:
- callbackFn will not visit any elements added beyond the array's initial length when the call to the iterative method began.
- Changes to already-visited indexes do not cause callbackFn to be invoked on them again.
- If an existing, yet-unvisited element of the array is changed by callbackFn , its value passed to the callbackFn will be the value at the time that element gets visited. Removed elements are not visited.
Warning: Concurrent modifications of the kind described above frequently lead to hard-to-understand code and are generally to be avoided (except in special cases).
The following examples use the forEach method as an example, but other methods that visit indexes in ascending order work in the same way. We will first define a helper function:
Modification to indexes not visited yet will be visible once the index is reached:
Modification to already visited indexes does not change iteration behavior, although the array will be different afterwards:
Inserting n elements at unvisited indexes that are less than the initial array length will make them be visited. The last n elements in the original array that now have index greater than the initial array length will not be visited:
Inserting n elements with index greater than the initial array length will not make them be visited:
Inserting n elements at already visited indexes will not make them be visited, but it shifts remaining elements back by n , so the current index and the n - 1 elements before it are visited again:
Deleting n elements at unvisited indexes will make them not be visited anymore. Because the array has shrunk, the last n iterations will visit out-of-bounds indexes. If the method ignores non-existent indexes (see array methods and empty slots ), the last n iterations will be skipped; otherwise, they will receive undefined :
Deleting n elements at already visited indexes does not change the fact that they were visited before they get deleted. Because the array has shrunk, the next n elements after the current index are skipped. If the method ignores non-existent indexes, the last n iterations will be skipped; otherwise, they will receive undefined :
For methods that iterate in descending order of index, insertion causes elements to be skipped, and deletion causes elements to be visited multiple times. Adjust the code above yourself to see the effects.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Indexed collections guide
- ArrayBuffer
JS Copy an Object – How to Clone an Obj in JavaScript

A JavaScript object is a collection of key-value pairs. It is a non-primitive data type that can contain various data types. For example:
When working with objects in JavaScript, you may sometimes want to change the value or add a new property to the object.
In some scenarios, before you update or add new properties, you will want to create a new object and copy or clone the value of the original one.
For example, if you want to copy the value of the userDetails object and then change the name to something different. In the typical sense, you will want to use the equality (=) operator.
Everything still seems to be fine, but let's see what happens if we edit our second object:
Everything is fine with the new object, but if you try to check your original object's values, you will notice it is affected. Why? How?
This is the issue. The original object is affected because objects are reference types . This means any value you store either in the clone or original object points to the same object.
This is not what you want. You want to store an object's value in a new object and manipulate the value in the new object without affecting the original array.
In this article, you will learn three methods that you can use to do this. You will also learn what deep and shallow clones mean and how they work.
In case you are in a rush, here are the three methods and an example of how they work.
If you are not in a rush, let's get started.🚀
How to Clone an Object in JavaScript With the Spread Operator
The spread operator was introduced in ES6 and can spread values into an object with the three dots in front.
This is no longer referenced, meaning changing the object's value will not affect the original object.
When you check the name value in the original object or the entire object, you will notice it is not affected.
Note: You can only use the spread syntax to make a shallow copy of an object while deeper objects are referenced. You will understand when we get to the last section of this article.
How to Clone an Object in JavaScript With Object.assign()
An alternative to the spread operator is the Object.assign() method. You use this method to copy the values and properties from one or more source objects to a target object.
Note: You can only use the Object.assign() method to make a shallow copy of an object while deeper objects are referenced. You will understand when we get to the last section of this article.
How to Clone an Object With JSON.parse()
The final method is JSON.parse(). You will use this method alongside JSON.stringify() . You can use this to deeply clone, but it has some downsides. First, let’s see how it works.
Also, just like the previous methods, this is no longer referenced. This means that you can change a value in the new object without affecting the original object.
Note: This method can be used for deep cloning but will not be the best option because it does not work with function or symbol properties.
Let’s now explore shallow and deep cloning and how you can use the JSON.parse() method to perform deep cloning. You will also learn why it is not the best option.
Shallow Clone vs. Deep Clone
So far, the example used in this article is a basic object with only one level. This means that we have only performed shallow clone(s). But when an object has more than one level, then you will be required to perform a deep clone.
Notice that the deep object has more than one level because there is another object in the userDetails object. A deep object can have as many levels as you want.
Note: When you use the spread operator or Object.assign() method to clone a deep object, the deeper objects will be referenced.
You will notice that both the original and new objects are affected because when you use either the spread operator or Object.assign() method to clone a deep object, the deeper objects will be referenced.
How can you fix this issue
You can use the JSON.parse() method, and everything will work fine.
But there is an issue with this method. The issue is that you can lose your data. How?
JSON.stringify() works very well with primitive data types like numbers, strings, or Booleans, and that is what you have seen in our previous examples. But sometimes, JSON.stringify() is unpredictable if you are not aware of some values and how it handles them.
For example, it does not work with functions, symbols, or undefined values. It also changes other values like Nan and Infinity to null , breaking your code. When you have a function, symbol, or undefined value, it will return an empty key-value pair and skip it.
Everything seems to work fine, but for the new object, JSON.stringify() will return no key-value pair for the undefined and symbol values.
This means you need to be careful. The best option to implement deep cloning will be to use Lodash . You can then be sure that none of your data will be lost.
Wrapping Up!
This article has taught you how to clone an object in JavaScript using three major methods. You've seen how those methods work, and when to use each one. You also learned about deep cloning.
You can read this article to understand why JSON.parse(JSON.stringify()) is a bad practice to clone an object in JavaScript.
Have fun coding!
Frontend Developer & Technical Writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
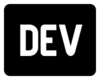
DEV Community

Posted on Mar 16
Copy Objects Ways in JavaScript
To copy objects in JavaScript, you typically have three options: using the assignment operator (=) for reference copying, performing a shallow copy using methods like Object.assign() or the spread operator (...) , and creating a deep copy using a combination of JSON.parse() and JSON.stringify() .
1. Reference Copy (Assignment Operator =):
In this method, referenceCopy now points to the same object as originalObject. Changes made to one will affect the other.This method creates a reference to the original object. Changes made to the original object will be reflected in the copied object, and vice versa. This is not a true copy; rather, both variables point to the same object.
2. Shallow Copy:
a. Using Object.assign():
Object.assign() creates a shallow copy of the object. It copies the enumerable properties from one or more source objects to a target object. In the example above, an empty object {} serves as the target, and originalObject is the source. Note that nested objects are still referenced, so changes to nested objects in the original will affect the copied object.
b. Using Spread Operator (...):
The spread operator is a concise way to create shallow copies of objects. Like Object.assign() , it only creates a copy of the top-level properties. Nested objects are still referenced, so changes to nested objects in the original will affect the copied object.
Both of these methods create a shallow copy, copying only the top-level properties of the object. Changes to nested objects will be reflected in both the original and the copy.
3. Deep Copy:
Using JSON.parse() and JSON.stringify()
This method creates a deep copy of the object, including nested structures. Keep in mind that this approach has limitations, such as not preserving non-JSON-safe data and being less efficient for large or complex objects.
Select the suitable approach depending on your particular circumstances:
- Reference Copy (=): Use when you want both variables to reference the same object.
- Shallow Copy (Object.assign() or Spread Operator): Use when you need a copy of the top-level properties and are not concerned about nested objects being shared.
- Deep Copy (JSON.parse() and JSON.stringify()): Use when you need a completely independent copy, including nested structures. Be cautious about limitations with non-JSON-safe data.
A glance at examples
Object.assign()
How do I correctly clone a JavaScript object?
Top comments (17)
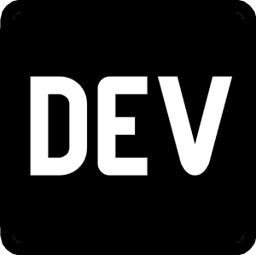
Templates let you quickly answer FAQs or store snippets for re-use.

- Location Bangkok 🇹🇭
- Joined Jun 1, 2018
You missed structuredClone .

- Email [email protected]
- Location Berlin, Germany
- Education shiraz univesity
- Work Aviv Group
- Joined Dec 11, 2022
In JavaScript, the structuredClone() method is used to create a deep copy of a JavaScript object, including nested objects and arrays. This method is similar to JSON.parse(JSON.stringify(object)), but it can handle more complex data types such as Date, RegExp, Map, Set, and cyclic object references, which are not supported by JSON.stringify().

- Joined Nov 26, 2019
⚠️ Moreover JSON.parse and stringify are very bad for performance. (Incorrect. See discussion below)
Totally agree with the first comments that structuredClone should be the only way in modern codebases to deep clone objects. If you need alternatives, you can use libraries for that (e.g. _.cloneDeep() )

- Location Würzburg, Germany
- Work Usability Engineer & Frontend Developer
- Joined Dec 4, 2019
Performance of JSON.parse(JSON.stringify(obj)) vs. structuredClone(obj) depends on the kind of data you want to clone.
While for more (almost) shallow data the JSON approach is faster , for deeply nested data structuredClone(obj) becomes more performant:
measurethat.net/Benchmarks/Show/30...
The test is very deeply nested, but plausible.
As annoying as this is - there is no silver bullet (performance-wise). You need to know what kind of data your application will handle, you need to test your solution based on your knowledge about the target audience and their hardware.

- Location Berlin
- Joined Apr 14, 2023
I guess you are right @htho , structuredClone(obj) seems faster in almost all cases unless the data was utterly nested (an edge case).
However, for edge cases I wouldn't use JSON.parse(JSON.stringify(obj)) either. I'd rather stay with structuredClone(obj) for a safer copy option.
jsbm 100 copies jsbm 1 copy

- Location Bristol, UK
- Work Chief Technology Officer
- Joined May 18, 2020
Are you sure? Last time I checked structuredClone was dramatically slower than parse and stringify.
measurethat.net/Benchmarks/Show/18...
Yup, structuredClone does seem much slower .
You are correct, I'm sorry (comment above edited). I remembered a discussion where I heard that JSON.stringify was significantly a slow approach to deep clone objects. That misled me doing the comparison with structuredClone . Thank you for the rectification.
Same thing bit me, I presumed it would be faster and wasted a couple of days refactoring some things (across workers etc) ended up with the "dance" just being way faster, even coping with circular references etc. Frustrating and I was pretty surprised.

- Joined Mar 17, 2024
Honestly very odd that this isn't edited in the main article as the main option now. It should completely replace the JSON.stringify dance. That can be completely forgotten for this purpose. Not for serialization of course, but in this context it shouldn't be recommended anymore.
I abandoned it because it's way slower. It has some useful other capabilities, but I find I don't need them. In my tests, structured clone was way too slow.
Structured clone is way slower than stringify? I mean it should be somewhat slower because it's more complete and has more logic. How much we talking? A benchmark coverage would be interesting to read. 👍
Jon and I linked benchmarks in other comments. For me it is between 42% and 45% slower. I copy a decent number of simple objects, 1/2 the speed is impactful. I do have cases with circular references etc., these are a reasonable candidate for structured clone, I find that an adapted stringify is more handy though, I can then store things and restore them from persistent storage.
Mine , Jon's

- Location Brazil
- Pronouns He / Him
- Work Full Stack Developer at Midas Solutions
- Joined Oct 8, 2019
Hi Fatemeh Paghar, Your tips are very useful Thanks for sharing

- Location Ha Noi, Viet Nam
- Education Information Technology
- Work Software development freelancer
- Joined Jan 13, 2019
With nested object, we can use recursion and Object.assign() or Spread Operator to make deep copy too :)

- Joined Feb 26, 2024
Thank you for all the posts and discussions! While I was already familiar with shallow copying an object, I hadn't known about creating deep copies until now. I've learned several approaches, along with their pros and cons. Your post is a lot helpful! 👍🏻

- Joined Mar 5, 2024
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Get 100 lighthouse performance score with a React app
Mladen Stojanovic - May 7

useEffect() - What You Need to Know for Acing Tech Interviews!
Alberto Cubeddu - May 7

Benefits Of Using An IDE For React Native Development
Saumya - May 7
ReactJS onClick Event on Child Components
Paulund - May 7
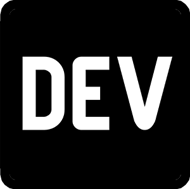
We're a place where coders share, stay up-to-date and grow their careers.
JS Reference
Html events, html objects, other references, javascript object.assign(), description.
The Object.assign() method copies properties from one or more source objects to a target object.
Related Methods:
Object.assign() copies properties from a source object to a target object.
Object.create() creates an object from an existing object.
Object.fromEntries() creates an object from a list of keys/values.
Return Value
Advertisement
Browser Support
Object.assign() is an ECMAScript6 (ES6) feature.
ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
Object.assign() is not supported in Internet Explorer.
Object Tutorials
JavaScript Objects
JavaScript Object Definition
JavaScript Object Methods
JavaScript Object Properties

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- Add Elements to a JavaScript Array
- Create an array of given size in JavaScript
- Add new elements at the beginning of an array using JavaScript
- Remove elements from a JavaScript Array
- Remove Duplicate Elements from JavaScript Array
- How to remove multiple elements from array in JavaScript ?
- Reverse an array in JavaScript
- Remove the last Item From an Array in JavaScript
- Remove empty elements from an array in JavaScript
- Count occurrences of all items in an array in JavaScript
- Add an Object to an Array in JavaScript
- How to append an element in an array in JavaScript ?
- How to select a random element from array in JavaScript ?
- How to remove duplicates from an array of objects using JavaScript ?
- Split an array into chunks in JavaScript
- How to find the sum of all elements of a given array in JavaScript ?
- Find the min/max element of an Array using JavaScript
- Get the first and last item in an array using JavaScript
- How to Add Elements to the End of an Array in JavaScript ?
- How to add elements to an existing array dynamically in JavaScript ?
- How to move an array element from one array position to another in JavaScript?
- How to insert an item into array at specific index in JavaScript ?
Copy Array Items into Another Array in JavaScript
- How to remove n elements from the end of a given array in JavaScript ?
- How to clone an array in JavaScript ?
- How to find the index of all occurrence of elements in an array using JavaScript ?
- Check if an array is empty or not in JavaScript
We will see how to copy array items into another array in JavaScript . This operation is valuable when you need to generate a new array containing either identical or a subset of elements from an original array.
These are the following ways to solve this problem:
Table of Content
- Using the Spread Operator (…)
- Using slice() Method
- Using apply() Method
- Using Array.concat() Method
- Using push() Method
- Using map() Method
Approaches 1: Using the Spread Operator (…)
The spread operator (…) is a concise syntax introduced in ES6 that allows us to expand iterable objects, like arrays, into individual elements. To copy an array using the spread operator, you simply spread the elements of the original array into a new array literal.
Example: In this example, we will see the use of the spread operator.
Approach 2: Using slice() Method
The slice() method is an array method in JavaScript that returns a shallow copy of a portion of an array into a new array. When called without any arguments, it can be used to copy the entire array.
Example: In this example, we will see the use of the slice() Method.
Approach 3: Using apply() Method
The apply() method is an older approach to copying an array, but it is less commonly used compared to the spread operator and slice() method. The apply() method calls a function with a given value and an array of arguments. To copy an array using apply(), you can use the concat() method along with apply() to concatenate the elements of the original array into a new array.
Example: In this example, we will see the use of apply() Method.
Approach 4: Using Array.concat() Method
This approach concatenates the source array with an empty array using the Array.prototype.concat() method , which returns a new array with all the elements.
Example: In this example, we will see the use of Array.concat() Method.
Approach 5: Using push() Method
In JavaScript, to copy array items into another array using the push method , iterate over the given array and use push to add each item to our result array
Example: In this example, we use the push() method to copy array items into another array.
Approach 6: Using map() Method
JavaScript map method can be used to copy array items into another array. By applying a callback function that returns the original item, a new array is created with copied items,
Example: In this example, we are using the map() method to copy an array item into another array.
Please Login to comment...
Similar reads.
- javascript-array
- JavaScript-DSA
- JavaScript-Questions
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
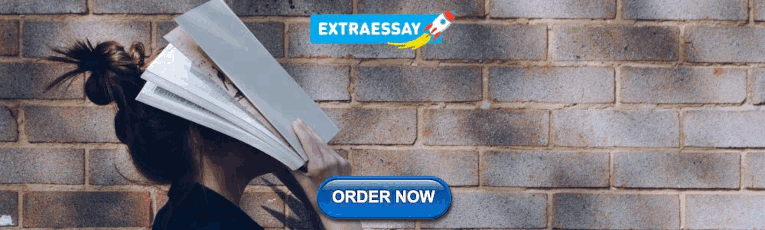
IMAGES
VIDEO
COMMENTS
Map will create a new array from the old one (without reference to old one) and inside the map you create a new object and iterate over properties (keys) and assign values from the old Array object to corresponding properties to the new object.. This will create exactly the same array of objects. let newArray = oldArray.map(a => { let newObject = {}; Object.keys(a).forEach(propertyKey ...
There are at least 6 (!) ways to clone an array:. loop; slice; Array.from() concat; spread syntax (FASTEST) map A.map(function(e){return e;});; There has been a huuuge BENCHMARKS thread, providing following information:. for blink browsers slice() is the fastest method, concat() is a bit slower, and while loop is 2.4x slower.. for other browsers while loop is the fastest method, since those ...
The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties.
JavaScript has many ways to do anything. I've written on 10 Ways to Write pipe/compose in JavaScript [/news/10-ways-to-write-pipe-compose-in-javascript-f6d54c575616/], and now we're doing arrays. Here's an interactive scrim that shows various ways to clone arrays in JavaScript: 1. Spread Operator (Shallow copy) Ever since ES6 dropped, this has been the most
JavaScript offers standard inbuilt object-copy operations for creating shallow copies: Array.from(), Array.prototype.concat(), Array.prototype.slice(), Object.assign(), and Object.create(), spread syntax. Here's an example of shallow copy in JavaScript: Here's an example of shallow copy in TypeScript.
There are a few ways to clone an array in JavaScript, Object.assign. Object.assign allows us to make a shallow copy of any kind of object including arrays. For example: const a = [1,2,3]; const b = Object.assign([], a); // [1,2,3] Array.slice. The Array.slice function returns a copy of the original array.
) and Object.assign() perform a shallow copy while the JSON methods carry a deep copy. Shallow copy vs. deep copy. In JavaScript, you use variables to store values that can be primitive or references. When you make a copy of a value stored in a variable, you create a new variable with the same value. For a primitive value, you just simply use a ...
When an object variable is copied, the reference is copied, but the object itself is not duplicated. For instance: let user = { name: "John" }; let admin = user; Now we have two variables, each storing a reference to the same object: As you can see, there's still one object, but now with two variables that reference it.
The Object.assign() method was introduced in ES6 that copies all enumerable own properties from one or more source objects to a target object, and returns the target object. The Object.assign() method invokes the getters on the source objects and setters on the target object. It assigns properties only, not copying or defining new properties.
Download Run Code. 3. Using Array.from() function. The Array.from() is a built-in function that allows us to create a new array from an array-like or iterable object. We can use this function to copy an array by passing it as the first argument. We can also optionally provide a map() function as the second argument to modify the elements of the new array.
The following shows the syntax of the Object.assign () method: The Object.assign () copies all enumerable and own properties from the source objects to the target object. It returns the target object. The Object.assign () invokes the getters on the source objects and setters on the target. It assigns properties only, not copying or defining new ...
This example shows three ways to create new array: first using array literal notation, then using the Array() constructor, and finally using String.prototype.split() to build the array from a string. js. // 'fruits' array created using array literal notation. const fruits = ["Apple", "Banana"];
Using the Array.map () method is another way to clone an array in JavaScript. This method creates a new array by mapping each element from the original array to a new value. Example: This example shows the implementation of the above-explained approach. Javascript. const originalArray = [1, 2, 3];
You use this method to copy the values and properties from one or more source objects to a target object. // Declaring Object const userDetails = { name: "John Doe", age: 14, verified: false }; // Cloning the Object with Object.assign() Method let cloneUser = Object.assign({}, userDetails);
You assign a new value to copyOfMyNumber. If you assign a new value to copyOfMyArray it will not change myArray either. You can create a copy of an array using slice[docs]: var copyOfMyArray = myArray.slice (0); But note that this only returns a shallow copy, i.e. objects inside the array will not be cloned.
To copy objects in JavaScript, you typically have three options: using the assignment operator (=) for reference copying, performing a shallow copy using methods like Object.assign() or the spread operator (...), and creating a deep copy using a combination of JSON.parse() and JSON.stringify(). 1. Reference Copy (Assignment Operator =): In this ...
In JavaScript, a two-dimensional array is just an array of arrays. Therefore, cloning one dimension is not enough. We also need to clone all the sub-dimension arrays.
Description. The Object.assign() method copies properties from one or more source objects to a target object. Object.assign () copies properties from a source object to a target object. Object.create () creates an object from an existing object. Object.fromEntries () creates an object from a list of keys/values.
const copy = Object.assign([], original); copy.forEach(obj => obj.value = obj.value + 1); I do not understand why this is the case. In the MDN web docs, the following statements can be found in the deep copy warning section: For deep cloning, we need to use alternatives, because Object.assign() copies property values.
To copy an array using apply (), you can use the concat () method along with apply () to concatenate the elements of the original array into a new array. Syntax: const copiedArray = Array.apply(null, originalArray); Example: In this example, we will see the use of apply () Method. Javascript.
So if you do something like newArray1 = oldArray.slice(0); newArray2 = oldArray.slice(0), the two new arrays will reference to just 1 array and changing one will affect the other. Alternatively, using newArray1 = JSON.parse(JSON.stringify(old array)) will only copy the value, thus it creates a new array each time.