Verilog Assignments
Variable declaration assignment, net declaration assignment, assign deassign, force release.
- Procedural continuous
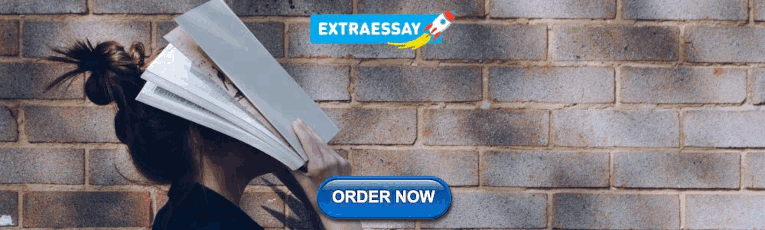
Legal LHS values
An assignment has two parts - right-hand side (RHS) and left-hand side (LHS) with an equal symbol (=) or a less than-equal symbol (<=) in between.
The RHS can contain any expression that evaluates to a final value while the LHS indicates a net or a variable to which the value in RHS is being assigned.
Procedural Assignment
Procedural assignments occur within procedures such as always , initial , task and functions and are used to place values onto variables. The variable will hold the value until the next assignment to the same variable.
The value will be placed onto the variable when the simulation executes this statement at some point during simulation time. This can be controlled and modified the way we want by the use of control flow statements such as if-else-if , case statement and looping mechanisms.
An initial value can be placed onto a variable at the time of its declaration as shown next. The assignment does not have a duration and holds the value until the next assignment to the same variable happens. Note that variable declaration assignments to an array are not allowed.
If the variable is initialized during declaration and at time 0 in an initial block as shown below, the order of evaluation is not guaranteed, and hence can have either 8'h05 or 8'hee.
Procedural blocks and assignments will be covered in more detail in a later section.
Continuous Assignment
This is used to assign values onto scalar and vector nets and happens whenever there is a change in the RHS. It provides a way to model combinational logic without specifying an interconnection of gates and makes it easier to drive the net with logical expressions.
Whenever b or c changes its value, then the whole expression in RHS will be evaluated and a will be updated with the new value.
This allows us to place a continuous assignment on the same statement that declares the net. Note that because a net can be declared only once, only one declaration assignment is possible for a net.
Procedural Continuous Assignment
- assign ... deassign
- force ... release
This will override all procedural assignments to a variable and is deactivated by using the same signal with deassign . The value of the variable will remain same until the variable gets a new value through a procedural or procedural continuous assignment. The LHS of an assign statement cannot be a bit-select, part-select or an array reference but can be a variable or a concatenation of variables.
These are similar to the assign - deassign statements but can also be applied to nets and variables. The LHS can be a bit-select of a net, part-select of a net, variable or a net but cannot be the reference to an array and bit/part select of a variable. The force statment will override all other assignments made to the variable until it is released using the release keyword.
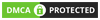
404 Not found
Assigning an Initial Value to a Register - 2023.2 English
Vivado design suite user guide: synthesis (ug901).
Assign a set/reset (initial) value to a register.
- Assign the value to the register when the register reset line goes to the appropriate value. See the following coding example.
- The value is implemented as a Flip-Flop, the output of which is controlled by a local reset.
- The value is carried in the Verilog file as an FDP or FDC Flip-Flop.
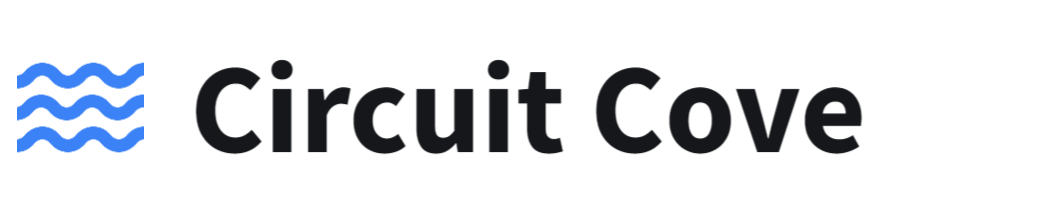
Designing a Register File in Verilog and SystemVerilog
A register file is a collection of registers that can be read and written by a digital circuit. Register files are a fundamental building block in many digital designs, such as microprocessors and digital signal processors. In this tutorial, we will discuss how to design a register file in Verilog and SystemVerilog.
Overview of Register Files
A register file is typically implemented as an array of registers, where each register is a collection of storage elements. The register file has two major operations: read and write. During a read operation, the contents of a register are transferred to an output port. During a write operation, the contents of an input port are transferred to a register.
Handling Read/Write Conflicts
When the read index and write index are the same, the read data will be the current register data, not the updating data. This behavior is important to keep in mind when designing a register file, especially in applications where multiple instructions may attempt to read and write to the same register at the same time.
One potential solution to this problem is to build a bypassing version of the register file, which allows the processor to read the result of the current instruction before it has been written to the register file. However, this solution can be complex and may not be necessary if the control logic for the CPU is carefully designed.
The following code shows an example implementation of a register file in Verilog and SystemVerilog:
The RegisterFile module has seven ports: clk , writeEn , readAddr1 , readAddr2 , writeAddr , writeData , readData1 , and readData2 . The clk port is the clock input, the writeEn port is the write enable input, the readAddr1 and readAddr2 ports are the read address inputs, the writeAddr port is the write address input, the writeData port is the write data input, and the readData1 and readData2 ports are the read data outputs.
The regs signal is an array of registers that stores the register file contents. The always_ff block is used to describe the behavior of the register file during a write operation. When the write enable signal writeEn is high, the contents of the register specified by the writeAddr input are updated with the data specified by the writeData input.
The assign statements are used to describe the behavior of the register file during a read operation. The contents of the registers specified by the readAddr1 and readAddr2 inputs are transferred to the readData1 and readData2 outputs, respectively. If the read address matches the write address, the read data is set to the current register data, rather than the updating data.
In this tutorial, we discussed how to design a register file in Verilog and SystemVerilog, including handling read/write conflicts. By understanding the principles behind register files and their implementation in Verilog and SystemVerilog, you can design custom register file circuits for your specific application. With the addition of proper control logic, the register file design presented here can handle most common read/write conflict scenarios.
whitehorsesoft (Member) asked a question.
- reg [ 3 : 0 ] r1 = 4 'h0; // initial value
- r1 = 15; // assign as this, rather than 4' hf ?
- Simulation & Verification
Related Questions
Community Feedback?

- Search forums
Follow along with the video below to see how to install our site as a web app on your home screen.
Note: This feature may not be available in some browsers.
Welcome to EDAboard.com
Welcome to our site edaboard.com is an international electronics discussion forum focused on eda software, circuits, schematics, books, theory, papers, asic, pld, 8051, dsp, network, rf, analog design, pcb, service manuals... and a whole lot more to participate you need to register. registration is free. click here to register now..
- Digital Design and Embedded Programming
- PLD, SPLD, GAL, CPLD, FPGA Design
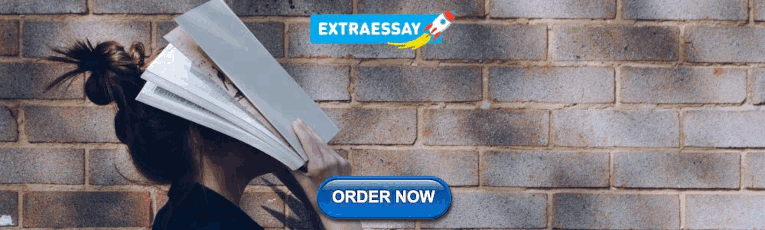
Verilog: assign register outputs using wire
- Thread starter Buriedcode
- Start date Jan 6, 2012
- Jan 6, 2012

Full Member level 6
Trickydicky, advanced member level 7.
Have a play with the synthesis translate off directive around the cntout assignment. You say its only for simulation, so to avoid any implementation on a real CPLD put the directives around it: Code: module LCDcolfmat(clkin, // synthesis translate_off cntout, // synthesis translate_on dout,R,G,B,reset); // synthesis translate_off assign cntout = counter; // synthesis translate_on Im a big fat FPGA person, but given its only a wire, I dont see why it should take anything more than routing resource (and pins!). The above removal should get rid. Any reason you need to route it out to the top anyway for simulation? simulators can monitor internal signals. You shouldnt need the output at all (and should just be able to see counter). PS. Im a VHDL person, but the directive is the same for both languages.
- Jan 7, 2012
Full Member level 4
if reduction of pld resources is the main goal, try such approach, quartus reported slightly less usage then your numbers; I believe the idea is quite simple, if not I can add an explanation; Code: module lcd ( input clk, input reset, input R, G, B, output [7:0] dout ); reg [11:0] rgb; // shift-in register // rgb[8:0] hold r,g,b data; rgb[11:0] control always @(posedge clk) if ( reset ) rgb <= 12'h1; else if ( rgb[11] ) //last byte of three ready rgb <= {5'h0,1'b1,rgb[2:0],R,G,B}; else if ( rgb[10] ) //second byte ready rgb <= {6'h0,1'b1,rgb[1:0],R,G,B}; else if ( rgb[9] ) //first byte ready rgb <= {7'h0, 1'b1,rgb[0], R,G,B}; else // shifting data in rgb <= {rgb[8:0],R,G,B}; assign dout = rgb[11] ? rgb[10:3] : rgb[10] ? rgb[ 9:2] : rgb[ 8:1]; endmodule --- have fun, J.A

Super Moderator
Comparing macrocells utilization without looking at the respective logic capabilties, e.g. number of available terms and input connectivity is like compare apples to oranges. One thing is to reduce the macrocell requirements by finding an optimal problem description, as discussed by j_andr, the other point is to find out which logic family is best suited for the application.
Anyways, seems the software I use (Quartus, ispLEVER, and ISEwebpack) are all pretty sensitive to verilog coding style. Click to expand...
Similar threads
- Started by mahesh_namboodiri
- Jan 31, 2024
- Started by keikaku
- Mar 5, 2024
- Replies: 10
- Started by EDA_hg81
- Sep 8, 2023
- Started by Lightning89
- Feb 14, 2024
- Started by Xenon02
- Oct 2, 2023
Part and Inventory Search
Welcome to edaboard.com.
- This site uses cookies to help personalise content, tailor your experience and to keep you logged in if you register. By continuing to use this site, you are consenting to our use of cookies. Accept Learn more…
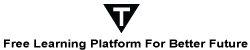
- Interview Q
Verilog Tutorial
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

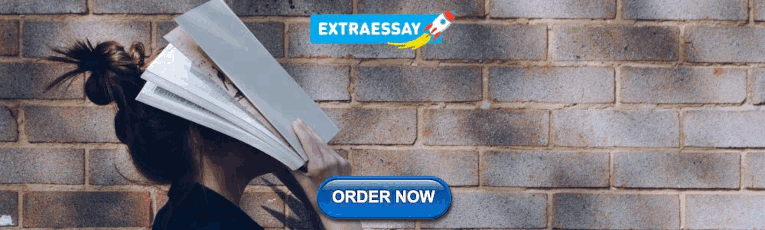
IMAGES
VIDEO
COMMENTS
What you want is a continuous assignment, which is what assign does. That should get you to the point where B is the value you expect. Though I should add that this doesn't mean you are assigning an actual hardware register. EDIT: You also need to remove the reg part of the declaration of outout reg [15:0] B on the copy module.
Verilog assign statement. Signals of type wire or a similar wire like data type requires the continuous assignment of a value. For example, consider an electrical wire used to connect pieces on a breadboard. As long as the +5V battery is applied to one end of the wire, the component connected to the other end of the wire will get the required ...
I want to declare a reg of 8 bits and set the value for each one of the bits separately (based on another "counter" reg) inside an always block using Verilog. Here is what I thought: module readv...
The LHS of an assign statement cannot be a bit-select, part-select or an array reference but can be a variable or a concatenation of variables. reg q; initial begin assign q = 0; #10 deassign q; end force release. These are similar to the assign - deassign statements but can also be applied to nets and variables. The LHS can be a bit-select of ...
This code does not properly represent a register with RESET. A RESET input (aka PRESET/CLEAR) clears the output to a fixed value, either 1 or 0, every time it is used. Your code sets the output to equal an input value which might change each time the reset signal is seen. Your code could also be written as
Assigning Specific Input Bits to Separate Registers in Verilog. Ask Question Asked 2 years, 7 months ago. Modified 2 years, 7 months ago. ... In the test-bench, after you set a non zero value to input, give some delay before stopping the simulation. \$\endgroup ... You are using assign statements, which are continuous assignments. So signals A ...
I have a data set consisting of 30 values and each of 16 bit wide. I tried to add these values as an input in my Verilog code in the following way: `timescale 1ns / 1ps module com (inp,clk,out ...
Signals of type wire or a similar wiring liked data type requirement the uninterrupted assignment of a value. For model, consider a electrical lead used to couple pieces on an breadboard. As long as the +5V backup is applied to one end of the wiring, the compone ... Verilog assign explanations Verilog assign examples Verilog Operators Verilog ...
This enables us to remember your preferences (for example, your choice of language or region) or when you register on areas of the Sites, such as our web programs or extranets. These cookies store data such as online identifiers (including IP address and device identifiers) along with the information used to provide the function.
In this tutorial, we will discuss how to design a register file in Verilog and SystemVerilog. Overview of Register Files. A register file is typically implemented as an array of registers, where each register is a collection of storage elements. The register file has two major operations: read and write.
If the signal is driven by continuous assignment (an assign statement) or is the output of a module instance, then it must be declared as wire (or one of its variants like wor or wand ), or as an output without the reg qualifier. A reg signal might be physically the output of either a latch or a flip-flop or of combinatorial logic (for example ...
Solved: Hi, is it possible to initialize a register value in PSoC verilog implementation? I tried "reg A = 1'b1;" on register definition, Announcements. Help us improve the Power & Sensing Selection Guide. ... You can assign register some value only inside 'always' block. You can refer to the section 2.3.2 (Register) of the Warp_verilog ...
For example (greatly pared down and simplified for clarity): reg [3:0] r1 = 4'h0; // initial value. ... r1 = 15; // assign as this, rather than 4'hf? Apologies for the learner question. From here it seems that integers are a 32-bit signed 4-state variable. Also on page 234 of UG901 ("Vivado Synthesis") there is some info about integer handling ...
I searched the web for an answer and tried all kinds of things but still cannot get the output assigned. Here's the code: input CLK, input En, input CLR, input [7:0] in, output [7:0] out. ); reg[7:0] temp;
assign dout = b[15:8]; assign cntout = counter; endmodule. The counter is sent to an output purely for simulation so I can see what value the counter is at a particular time. It is quite a complicated idea but its the only way I can format the input data for a colour-STN LCD.
In Verilog a wire cannot store a value, it can only be used to connect two parts of a circuit together. In order to be used in a procedural block (such as an always block, or initial block, etc.) a variable must be able to store a value, even if it is only during the processing of the block.
Verilog assign Statement. Assign statements are used to drive values on the net. And it is also used in Data Flow Modeling. Signals of type wire or a data type require the continuous assignment of a value. As long as the +5V battery is applied to one end of the wire, the component connected to the other end of the wire will get the required ...
Verilog - Assigning value to a reg twice in a single always block. 0. Verilog: assigning value to reg. Hot Network Questions What could cause a society to collectively forget about an event? Force non-interactive `apt-get install` to fail on config file conflict how much the pickup can influence the 'quality' of a pinch hamonic ...
In one always block, one bit register variable is flipping and in other always block, a counter variable is running. Now when counter will reach to a specific value I want to read the status of another loop variable. But couldn't understand the correct way to do it. Please help. Thank you for your time. Verilog code: