
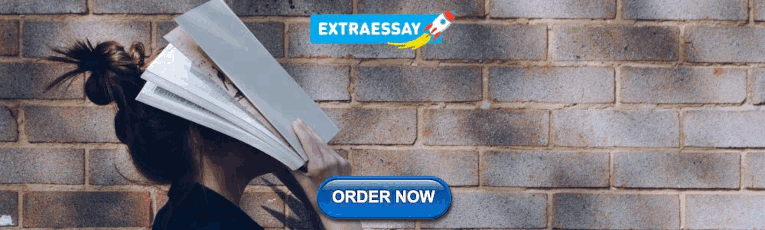
Files and Directories
The member functions presented with boost::filesystem::path simply process strings. They access individual components of a path, append paths to one another, and so on.
In order to work with physical files and directories on the hard drive, several free-standing functions are provided. They expect one or more parameters of type boost::filesystem::path and call operating system functions internally.
Prior to introducing the various functions, it is important to understand what happens in case of an error. All of the functions call operating system functions that may fail. Therefore, Boost.Filesystem provides two variants of the functions that behave differently in case of an error:
The first variant throws an exception of type boost::filesystem::filesystem_error . This class is derived from boost::system::system_error and thus fits into the Boost.System framework.
The second variant expects an object of type boost::system::error_code as an additional parameter. This object is passed by reference and can be examined after the function call. In case of a failure, the object stores the corresponding error code.
boost::system::system_error and boost::system::error_code are presented in Chapter 55 . In addition to the inherited interface from boost::system::system_error , boost::filesystem::filesystem_error provides two member functions called path1() and path2() , both of which return an object of type boost::filesystem::path . Since there are functions that expect two parameters of type boost::filesystem::path , these two member functions provide an easy way to retrieve the corresponding paths in case of a failure.
Example 35.10 introduces boost::filesystem::status() , which queries the status of a file or directory. This function returns an object of type boost::filesystem::file_status , which can be passed to additional helper functions for evaluation. For example, boost::filesystem::is_directory() returns true if the status for a directory was queried. Besides boost::filesystem::is_directory() , other functions are available, including boost::filesystem::is_regular_file() , boost::filesystem::is_symlink() , and boost::filesystem::exists() , all of which return a value of type bool .
The function boost::filesystem::symlink_status() queries the status of a symbolic link. With boost::filesystem::status() the status of the file referred to by the symbolic link is queried. On Windows, symbolic links are identified by the file extension lnk .
A different category of functions makes it possible to query attributes. The function boost::filesystem::file_size() returns the size of a file in bytes. The return value is of type boost::uintmax_t , which is a type definition for unsigned long long . The type is provided by Boost.Integer.
Example 35.11 uses an object of type boost::system::error_code , which needs to be evaluated explicitly to determine whether the call to boost::filesystem::file_size() was successful.
To determine the time a file was modified last, boost::filesystem::last_write_time() can be used (see Example 35.12 ).
boost::filesystem::space() retrieves the total and remaining disk space (see Example 35.13 ). It returns an object of type boost::filesystem::space_info , which provides three public member variables: capacity , free , and available , all of type boost::uintmax_t . The disk space is in bytes.
While the functions presented so far leave files and directories untouched, there are several functions that can be used to create, rename, or delete files and directories.
Example 35.14 should be self-explanatory. Looking closely, one can see that it’s not always an object of type boost::filesystem::path that is passed to functions, but rather a simple string. This is possible because boost::filesystem::path provides a non-explicit constructor that will convert strings to objects of type boost::filesystem::path . This makes it easy to use Boost.Filesystem since it’s not required to create paths explicitly.
In Example 35.14 , boost::filesystem::remove() is explicitly called using its namespace. Otherwise, Visual C++ 2013 would confuse the function with remove() from the header file stdio.h .
Additional functions such as create_symlink() to create symbolic links or copy_file() and copy_directory() to copy files and directories are available as well.
Example 35.15 presents a function that creates an absolute path based on a file name or section of a path. The path displayed depends on which directory the program is started in. For example, if the program was started in C:\ , the output would be "C:\photo.jpg" .
To retrieve an absolute path relative to a different directory, a second parameter can be passed to boost::filesystem::absolute() .
Example 35.16 displays "D:\photo.jpg" .
The last example in this section, Example 35.17 , introduces a useful helper function to retrieve the current working directory.
Example 35.17 calls boost::filesystem::current_path() multiple times. If the function is called without parameters, the current working directory is returned. If an object of type boost::filesystem::path is passed, the current working directory is set.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Windows permission documentation #95
redlars commented Dec 19, 2018
Lastique commented May 3, 2020
Sorry, something went wrong.
No branches or pull requests
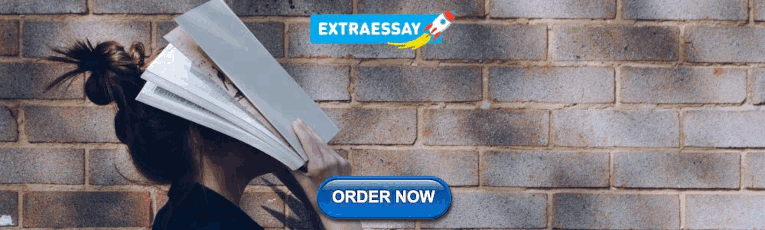
Boost C++ Libraries
...one of the most highly regarded and expertly designed C++ library projects in the world. — Herb Sutter and Andrei Alexandrescu , C++ Coding Standards
boost/filesystem/file_status.hpp
Revised $Date$
Copyright Beman Dawes, David Abrahams, 1998-2005.
Copyright Rene Rivera 2004-2008.
Distributed under the Boost Software License, Version 1.0 .
OSI Certified
cppreference.com
Std::filesystem:: perm_options.
This type represents available options that control the behavior of the function std::filesystem::permissions() .
perm_options satisfies the requirements of BitmaskType (which means the bitwise operators operator & , operator | , operator ^ , operator~ , operator & = , operator | = , and operator ^ = are defined for this type).
[ edit ] Member constants
At most one of add , remove , replace may be present, otherwise the behavior of the permissions function is undefined.
[ edit ] Example
Possible output:
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 6 September 2023, at 10:30.
- This page has been accessed 27,199 times.
- Privacy policy
- About cppreference.com
- Disclaimers

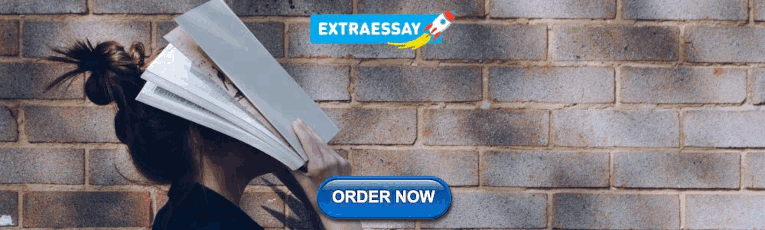
COMMENTS
How can I use the Boost library to change the permissions of a file to read-only? There are some questions that I have already seen, such as this and this , but I still don't know how to do it, I have tried doing
File: An object that can be written to, or read from, or both. A file has certain attributes, including type. File types include regular files and directories. Other types of files, such as symbolic links, may be supported by the implementation. File system: A collection of files and certain of their attributes.
The Boost.Filesystem file_size function returns a uintmax_t containing the size of the file named by the argument. The declaration looks like this: uintmax_t file_size(const path& p); For now, all you need to know is that class path has constructors that take const char * and other string types. (If you can't wait to find out more, skip ahead to the class path section of the tutorial.)
If opts is perm_options:: remove, the file permissions are set to exactly status (p). permissions & ~ (prms & perms:: mask) (meaning, any valid bit that is clear in prms, but set in the file's current permissions is cleared in the file's permissions). opts is required to have only one of replace, add, or remove to be set.
Hi, I'm using 1.65.1.0ubuntu1. I want to change the permissions of directories and set permissions when I create directory: boost::filesystem::create_direcory () boost::filesystem::create_directories() //all the directories recursively. As far I know boost::filesystem::permissions() works just for files. I don't file to use system and exec ...
boost::filesystem::space() retrieves the total and remaining disk space (see Example 35.13).It returns an object of type boost::filesystem::space_info, which provides three public member variables: capacity, free, and available, all of type boost::uintmax_t.The disk space is in bytes. While the functions presented so far leave files and directories untouched, there are several functions that ...
The boost::filesystem interface doesn't use the new types directly. It does use u16string and u32string in namespace boost. These are typedefs to std:: ... There are no permissions set for the file. Note: file_not_found is no_perms rather than perms_not_known: owner_read: 0400: S_IRUSR: Read permission, owner: owner_write: 0200:
We found the description of filesystem perms enumerator [1] confussing and started a discussion on boost mailinglist [2]. It appears the filesystem uses the readonly attribute to set permissions and does not use Windows ACL at all. We believe that the current docs should be updated to give a better description of the permissions functionality.
Access permissions model POSIX permission bits, and any individual file permissions (as reported by filesystem::status) are a combination of some of the following bits: Contents. 1 Member constants; 2 Notes; 3 Example; 4 See also Member constants. ... Set group ID to file's user group ID on execution sticky_bit: 01000: S_ISVTX:
Created file with permissions: rw-r--r-- After adding u+rwx and g+wrx: rwxrwxr--
3. So, boost::filesystem lets you access a file's permissions in the sense of which permissions the owner has, which permissions the group has and which all users have. That's nice, but I don't want to start checking who I am, what my group is etc - I just want to check if I can, say, recurse some directory or not (and not by trying to do so ...
The Boost.Filesystem file_size function returns a uintmax_t containing the size of the file named by the argument. The declaration looks like this: uintmax_t file_size(const path& p);. For now, all you need to know is that class path has constructors that take const char * and many other useful types. (If you can't wait to find out more, skip ahead to the class path section of the tutorial.)
The Boost.Filesystem library provides facilities to manipulate files and directories, and the paths that identify them. The features of the library include: A modern C++ interface, highly compatible with the C++ standard library. Many users say the interface is their primary motivation for using Boost.Filesystem.
I was getting this issue with all directory permissions set to 775 and cache/.boost set to 644 during a server migration from RHEL to CentOS. The Plesk panel owned the cache directory (psacln) so all that was needed was a recursive chown call from the Drupal root directory to assign the owner as apache apache:
void set_unrestricted noexcept; Sets permissions to unrestricted access: A null DACL for windows or 0666 for UNIX. void set_permissions (os_permissions_type perm) noexcept; Sets permissions from a user provided os-dependent permissions. os_permissions_type get_permissions const noexcept; Returns stored os-dependent permissions
My storage HDD is located within the media folder, which I changed permissions on for any user to use. Occasionally bitcoind will start and simply just stay at block 0.... Really frustrated and quite frankly im on the verge of moving on to other projects.
std::filesystem:: This type represents available options that control the behavior of the directory_iterator and recursive_directory_iterator . directory_options satisfies the requirements of BitmaskType (which means the bitwise operators operator&, operator|, operator^, operator~, operator&=, operator|=, and operator^= are defined for this ...
BOOST_CONSTEXPR file_status(file_type v, perms prms) BOOST_NOEXCEPT : m_value(v), m_perms(prms) {. } // As of October 2015 the interaction between noexcept and =default is so troublesome. // for VC++, GCC, and probably other compilers, that =default is not used with noexcept. // functions.
This type represents available options that control the behavior of the function std::filesystem::permissions().. perm_options satisfies the requirements of BitmaskType (which means the bitwise operators operator &, operator |, operator ^, operator~, operator & =, operator | =, and operator ^ = are defined for this type). [] Member constantAt most one of add, remove, replace may be present ...