cppreference.com
Std:: vector.
The elements are stored contiguously, which means that elements can be accessed not only through iterators, but also using offsets to regular pointers to elements. This means that a pointer to an element of a vector may be passed to any function that expects a pointer to an element of an array.
The storage of the vector is handled automatically, being expanded as needed. Vectors usually occupy more space than static arrays, because more memory is allocated to handle future growth. This way a vector does not need to reallocate each time an element is inserted, but only when the additional memory is exhausted. The total amount of allocated memory can be queried using capacity() function. Extra memory can be returned to the system via a call to shrink_to_fit() [1] .
Reallocations are usually costly operations in terms of performance. The reserve() function can be used to eliminate reallocations if the number of elements is known beforehand.
The complexity (efficiency) of common operations on vectors is as follows:
- Random access - constant 𝓞(1) .
- Insertion or removal of elements at the end - amortized constant 𝓞(1) .
- Insertion or removal of elements - linear in the distance to the end of the vector 𝓞(n) .
std::vector (for T other than bool ) meets the requirements of Container , AllocatorAwareContainer (since C++11) , SequenceContainer , ContiguousContainer (since C++17) and ReversibleContainer .
- ↑ In libstdc++, shrink_to_fit() is not available in C++98 mode.
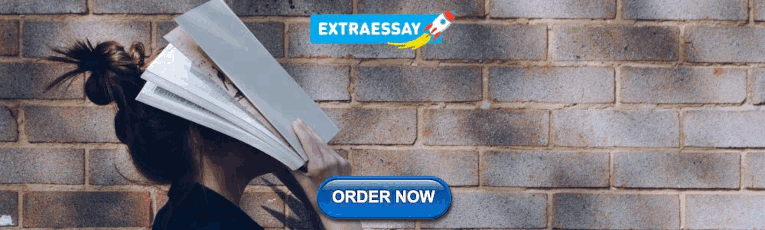
[ edit ] Template parameters
[edit]
[ edit ] Specializations
The standard library provides a specialization of std::vector for the type bool , which may be optimized for space efficiency.
[ edit ] Iterator invalidation
[ edit ] member types, [ edit ] member functions, [ edit ] non-member functions, [ edit ] notes, [ edit ] example, [ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 13 November 2023, at 23:49.
- This page has been accessed 14,709,782 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
- C++ Data Types
- C++ Type Modifiers
- C++ Constants
- C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
- C++ if, if...else and Nested if...else
- C++ for Loop
- C++ while and do...while Loop
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
- C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
- C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
- C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
- C++ Classes and Objects
- C++ Constructors
- C++ Constructor Overloading
- C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
- C++ Inheritance
- C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
- C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
C++ Standard Template Library
- C++ STL Containers
- C++ std::array
C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
C++ Iterators
C++ Algorithm
- C++ Functor
Additional Topics
- C++ Exceptions Handling
- C++ File Handling
C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
- C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
- C++ istream
- C++ ostream
C++ Tutorials
In C++, vectors are used to store elements of similar data types. However, unlike arrays , the size of a vector can grow dynamically.
That is, we can change the size of the vector during the execution of a program as per our requirements.
Vectors are part of the C++ Standard Template Library . To use vectors, we need to include the vector header file in our program.
- C++ Vector Declaration
Once we include the header file, here's how we can declare a vector in C++:
The type parameter <T> specifies the type of the vector. It can be any primitive data type such as int , char , float , etc. For example,
Here, num is the name of the vector.
Notice that we have not specified the size of the vector during the declaration. This is because the size of a vector can grow dynamically, so it is not necessary to define it.
- C++ Vector Initialization
There are different ways to initialize a vector in C++.
Here, we are initializing the vector by providing values directly to the vector. Now, both vector1 and vector2 are initialized with values 1 , 2 , 3 , 4 , 5 .
Here, 5 is the size of the vector and 12 is the value.
This code creates an int vector with size 5 and initializes the vector with the value of 12 . So, the vector is equivalent to
Example: C++ Vector Initialization
Here, we have declared and initialized three different vectors using three different initialization methods and displayed their contents.
Basic Vector Operations
The vector class provides various methods to perform different operations on vectors. We will look at some commonly used vector operations in this tutorial:
- Add elements
- Access elements
- Change elements
- Remove elements
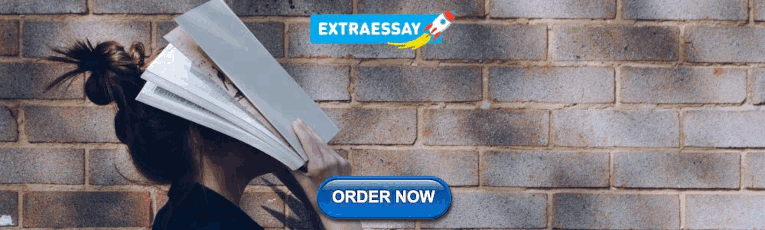
1. Add Elements to a Vector
To add a single element into a vector, we use the push_back() function. It inserts an element into the end of the vector. For example,
Here, we have initialized an int vector num with the elements {1, 2, 3, 4, 5} . Notice the statements
Here, the push_back() function adds elements 6 and 7 to the vector.
Note : We can also use the insert() and emplace() functions to add elements to a vector.
2. Access Elements of a Vector
In C++, we use the index number to access the vector elements. Here, we use the at() function to access the element from the specified index. For example,
- num.at(0) - access element at index 0
- num.at(2) - access element at index 2
- num.at(4) - access element at index 4
Note: Like an array, we can also use the square brackets [] to access vector elements. For example,
However, the at() function is preferred over [] because at() throws an exception whenever the vector is out of bound, while [] gives a garbage value.
3. Change Vector Element
We can change an element of the vector using the same at() function. For example,
In the above example, notice the statements,
Here, we have assigned new values to indexes 1 and 4 . So the value at index 1 is changed to 9 and the value at index 4 is changed to 7 .
4. Delete Elements from C++ Vectors
To delete a single element from a vector, we use the pop_back() function. For example,
In the above example, notice the statement,
Here, we have removed the last element ( 7 ) from the vector.
- C++ Vector Functions
In C++, the vector header file provides various functions that can be used to perform different operations on a vector.
- C++ Vector Iterators
Vector iterators are used to point to the memory address of a vector element. In some ways, they act like pointers in C++.
We can create vector iterators with the syntax
For example, if we have 2 vectors of int and double types, then we will need 2 different iterators corresponding to their types:
Initialize Vector Iterators
We can initialize vector iterators using the begin() and end() functions.
1. begin() function
The begin() function returns an iterator that points to the first element of the vector. For example,
2. end() function
The end() function points to the theoretical element that comes after the final element of the vector. For example,
Here, due to the nature of the end() function, we have used the code num.end() - 1 to point to the last element of the num vector i.e. num[2] .
Example: C++ Vector Iterators
In this program, we have declared an int vector iterator iter to use it with the vector num .
Then, we initialized the iterator to the first element of the vector using the begin() function.
Then, we printed the vector element by dereferencing the iterator:
Then, we printed the 3rd element of the vector by changing the value of iter to num.begin() + 2 .
Finally, we printed the last element of the vector using the end() function.
- Example: Iterate Through Vector Using Iterators
Here, we have used a for loop to initialize and iterate the iterator iter from the beginning of the vector to the end of the vector using the begin() and end() functions.
Also Read :
Table of Contents
- Introduction
- Example: C++ Vector
- Add Elements to a Vector
- Access Elements of a Vector
- Change Vector Element
- Delete Elements from C++ Vectors
Sorry about that.
Related Tutorials
C++ Tutorial
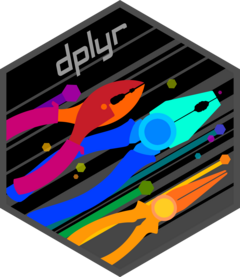
Extract the first, last, or nth value from a vector
These are useful helpers for extracting a single value from a vector. They are guaranteed to return a meaningful value, even when the input is shorter than expected. You can also provide an optional secondary vector that defines the ordering.
For nth() , a single integer specifying the position. Negative integers index from the end (i.e. -1L will return the last value in the vector).
An optional vector the same size as x used to determine the order.
A default value to use if the position does not exist in x .
If NULL , the default, a missing value is used.
If supplied, this must be a single value, which will be cast to the type of x .
When x is a list , default is allowed to be any value. There are no type or size restrictions in this case.
Should missing values in x be removed before extracting the value?
If x is a list, a single element from that list. Otherwise, a vector the same type as x with size 1.
For most vector types, first(x) , last(x) , and nth(x, n) work like x[[1]] , x[[length(x)] , and x[[n]] , respectively. The primary exception is data frames, where they instead retrieve rows, i.e. x[1, ] , x[nrow(x), ] , and x[n, ] . This is consistent with the tidyverse/vctrs principle which treats data frames as a vector of rows, rather than a vector of columns.
- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
- vector<bool>
- vector::~vector
- vector::vector
member functions
- vector::assign
- vector::back
- vector::begin
- vector::capacity
- C++11 vector::cbegin
- C++11 vector::cend
- vector::clear
- C++11 vector::crbegin
- C++11 vector::crend
- C++11 vector::data
- C++11 vector::emplace
- C++11 vector::emplace_back
- vector::empty
- vector::end
- vector::erase
- vector::front
- vector::get_allocator
- vector::insert
- vector::max_size
- vector::operator[]
- vector::operator=
- vector::pop_back
- vector::push_back
- vector::rbegin
- vector::rend
- vector::reserve
- vector::resize
- C++11 vector::shrink_to_fit
- vector::size
- vector::swap
non-member overloads
- relational operators (vector)
- swap (vector)
std:: vector ::erase
Return value, iterator validity, exception safety.
- Standard Template Library
- STL Priority Queue
- STL Interview Questions
- STL Cheatsheet
- C++ Templates
- C++ Functors
- C++ Iterators
How to Change an Element by Index in a Vector in C++?
- How to Access an Element in a Vector Using Index in C++?
- How to copy elements of an Array in a Vector in C++
- How to find index of a given element in a Vector in C++
- How to Access Elements in Set by Index in C++?
- How to Find the Index of an Element in an Array in C++?
- How to Access the Last Element in a Vector in C++?
- Find index of an element in a Set in C++
- How to Check if a Vector is Empty in C++?
- How to Find Minimum Element in a Vector in C++?
- How to Add Element at the End of a Vector in C++?
- How to find Index of Element in Vector in R ?
- How to Find All Indexes of an Element in an Array in C++?
- How to Check if a Vector Contains a Given Element in C++?
- How to Create, Access, and Modify Vector Elements in R ?
- How to Convert a Vector to a List Without Losing Element Order in C++?
- How to Access an Element in Set in C++?
- How to Access the First Element of a Vector in C++?
- How to Convert an Array to a Vector in C++?
- How to Remove an Element from Vector in C++?
In C++, vectors are dynamic containers that store the data in a contiguous memory location but unlike arrays , they can resize themselves to store more elements. In this article, we will learn how to change a specific element by its index in a vector in C++.
Change a Specific Element in a Vector by Index
To replace a specific element in a vector using its index, we can use the [] array subscript operator . It is used with the index and the vector name and we directly assign the new value using the assignment operator.
C++ Program to Change a Specific Element in a Vector by Index
Time Complexity : O(1) Auxilary Space: O(1)
Please Login to comment...
Similar reads.
- CPP Examples
- C++ Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
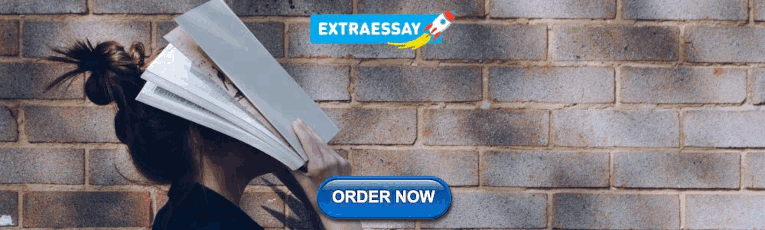
IMAGES
VIDEO
COMMENTS
Now, for example, how do I change the 5th element of the vector to -1? I tried l.assign(4, -1); It is not behaving as expected. None of the other vector methods seem to fit. I have used vector as I need random access functionality in my code (using l.at(i)). c++; stl; vector; Share. Follow
Last element of vector in C++ (Accessing and updating) In C++ vectors, we can access last element using size of vector using following ways. 1) Using size () Output : 2) Using back () We can access and modify last value using back (). Output : A Computer Science portal for geeks. It contains well written, well thought and well explained ...
Returns a reference to the last element in the vector. Unlike member vector::end, which returns an iterator just past this element, this function returns a direct reference. Calling this function on an empty container causes undefined behavior. Parameters none Return value A reference to the last element in the vector. If the vector object is const-qualified, the function returns a const ...
Let us see the differences in a tabular form -: vector::front () vector::back () 1. It is used to return a reference to the first element in the vector. It is used to return a reference to the last element in the vector. 2. Its syntax is -: vectorName.front ();
That is a valid way to change the last element. We need more context. vector.back() is a simpler way to do the same thing: VarNames.back().Type = "test"; If VarNames.size() == 0, you are trying to access VarNames[-1], which becomes VarNames[maximum size_t value], which is going out of bounds of your vector.
The last element is modified. Concurrently accessing or modifying other elements is safe, although iterating ranges that include the removed element is not. Exception safety If the container is not empty, the function never throws exceptions (no-throw guarantee). Otherwise, it causes undefined behavior. See also vector::push_back
namespace pmr {. template<class T > using vector = std ::vector< T, std::pmr::polymorphic_allocator< T >>; } (2) (since C++17) 1)std::vector is a sequence container that encapsulates dynamic size arrays. 2)std::pmr::vector is an alias template that uses a polymorphic allocator. The elements are stored contiguously, which means that elements can ...
The end() function points to the theoretical element that comes after the final element of the vector. For example, // iter points to the last element of num iter = num.end() - 1; Here, due to the nature of the end() function, we have used the code num.end() - 1 to point to the last element of the num vector i.e. num[2].
Access the Last Element in a Vector in C++. The simplest and most efficient way to access the last element of a vector is by using the std::vector::back () member function. This function returns a reference to the last element in the vector.
Arguments x. A vector. n. For nth(), a single integer specifying the position.Negative integers index from the end (i.e. -1L will return the last value in the vector). order_by. An optional vector the same size as x used to determine the order.. default. A default value to use if the position does not exist in x.. If NULL, the default, a missing value is used.. If supplied, this must be a ...
Removes from the vector either a single element (position) or a range of elements ([first,last)). This effectively reduces the container size by the number of elements removed, which are destroyed. Because vectors use an array as their underlying storage, erasing elements in positions other than the vector end causes the container to relocate all the elements after the segment erased to their ...
Pushing 10 or fewer elements onto the vector will not change its capacity or cause reallocation to occur. However, if the vector's length is increased to 11, it will have to reallocate, which can be slow. ... The removed element is replaced by the last element of the vector.
The vector containers have the std::vector::pop_back() function which provides a simple and efficient way to remove the last element from a vector in C++ STL. It is a member function of the std::vector class and can be used as shown. Syntax of std::vector::pop_back()
Unlike last from the pastecs package, head and tail (from utils) work not only on vectors but also on data frames etc., and also can return data "without first/last n elements", e.g. but.last <- function(x) { head(x, n = -1) } (Note that you have to use head for this, instead of tail.)
Hello, I am trying to replace an element in a vector with a new element which can only be constructed after getting ownership of the old element. An example could look like this: #[derive(Debug)] struct Foo(Box<i32>); …
Syntax to Declare Vector in C++. std::vector<dataType> vectorName; where the data type is the type of data of each element of the vector. You can remove the std:: if you have already used the std namespace. Initialization of Vector in C++. We can initialize a vector in the following ways: 1. Initialization Using List.
4 Answers. Sorted by: 132. You can use negative offsets in head (or tail ), so head (x, -1) removes the last element: R> head ( 1:4, -1) [1] 1 2 3 R>. This also saves an additional call to length (). Edit: As pointed out by Jason, this approach is actually not faster. Can't argue with empirics. On my machine:
Replace a Particular Element in a Vector in C++. To replace a specific element in a std::vector in C++, we can use the std::replace () function provided by the STL. The std::replace () function replaces all occurrences of a specific value in a range with another value.
To replace a specific element in a vector using its index, we can use the [] array subscript operator. It is used with the index and the vector name and we directly assign the new value using the assignment operator. C++ Program to Change a Specific Element in a Vector by Index. cout << "Index out of range!" << endl;