Learn C practically and Get Certified .
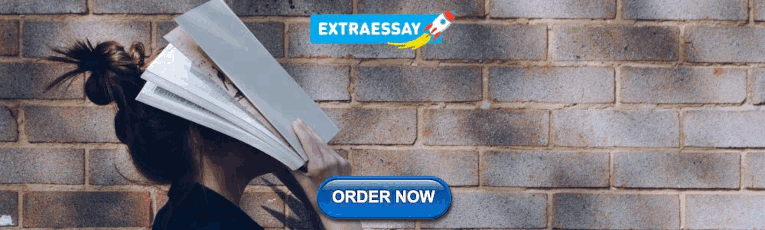
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
C Multidimensional Arrays
Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find Largest Element in an Array
- Calculate Average Using Arrays
- Access Array Elements Using Pointer
- Add Two Matrices Using Multi-dimensional Arrays
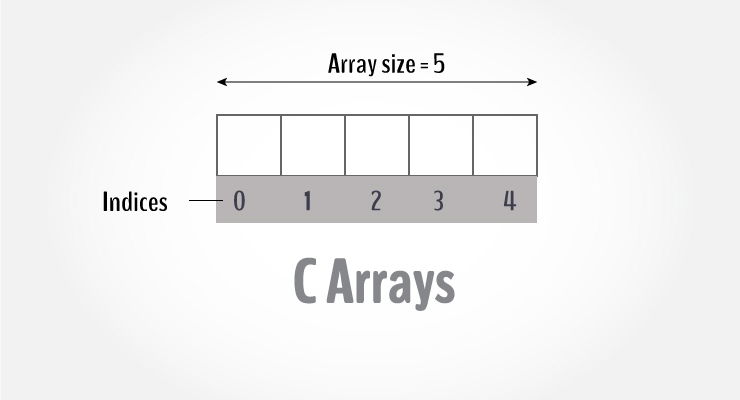
An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it.
How to declare an array?
For example,
Here, we declared an array, mark , of floating-point type. And its size is 5. Meaning, it can hold 5 floating-point values.
It's important to note that the size and type of an array cannot be changed once it is declared.
Access Array Elements
You can access elements of an array by indices.
Suppose you declared an array mark as above. The first element is mark[0] , the second element is mark[1] and so on.
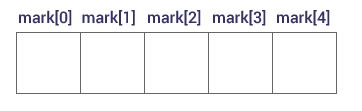
Few keynotes :
- Arrays have 0 as the first index, not 1. In this example, mark[0] is the first element.
- If the size of an array is n , to access the last element, the n-1 index is used. In this example, mark[4]
- Suppose the starting address of mark[0] is 2120d . Then, the address of the mark[1] will be 2124d . Similarly, the address of mark[2] will be 2128d and so on. This is because the size of a float is 4 bytes.
How to initialize an array?
It is possible to initialize an array during declaration. For example,
You can also initialize an array like this.
Here, we haven't specified the size. However, the compiler knows its size is 5 as we are initializing it with 5 elements.
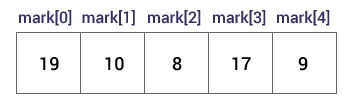
Change Value of Array elements
Input and output array elements.
Here's how you can take input from the user and store it in an array element.
Here's how you can print an individual element of an array.
Example 1: Array Input/Output
Here, we have used a for loop to take 5 inputs from the user and store them in an array. Then, using another for loop, these elements are displayed on the screen.
Example 2: Calculate Average
Here, we have computed the average of n numbers entered by the user.
Access elements out of its bound!
Suppose you declared an array of 10 elements. Let's say,
You can access the array elements from testArray[0] to testArray[9] .
Now let's say if you try to access testArray[12] . The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might get an error and some other time your program may run correctly.
Hence, you should never access elements of an array outside of its bound.
Multidimensional arrays
In this tutorial, you learned about arrays. These arrays are called one-dimensional arrays.
In the next tutorial, you will learn about multidimensional arrays (array of an array) .
Table of Contents
- C Arrays (Introduction)
- Declaring an Array
- Access array elements
- Initializing an array
- Change Value of Array Elements
- Array Input/Output
- Example: Calculate Average
- Array Elements Out of its Bound
Video: C Arrays
Sorry about that.
Related Tutorials
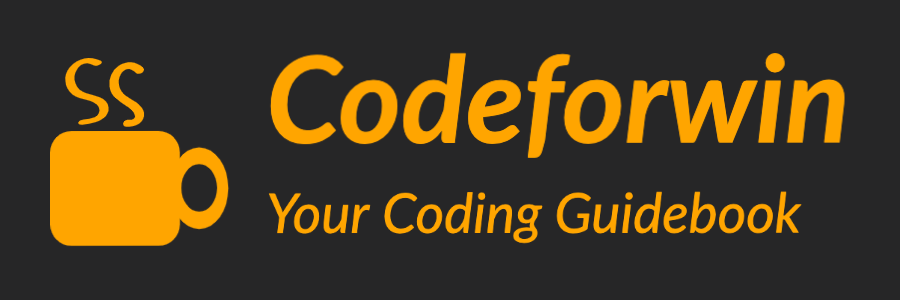
Arrays in C – Declare, initialize and access
Array is a data structure that hold finite sequential collection of homogeneous data .
To make it simple let’s break the words.
- Array is a collection – Array is a container that can hold a collection of data.
- Array is finite – The collection of data in array is always finite, which is determined prior to its use.
- Array is sequential – Array stores collection of data sequentially in memory.
- Array contains homogeneous data – The collection of data in array must share a same data type .
We can divide arrays in two categories.
- One-dimensional array (Or single-dimensional array)
- Multi-dimensional array
Why we need arrays?
Let us understand the significance of arrays through an example.
Suppose, I asked you to write a program to input 1000 students marks from user. Finally print average of their marks .
To solve the problem you will declare 1000 integer variable to input marks. Call I/O functions to input marks in 1000 variables and finally find the average.
Think for a while how tedious will be to code if solved using above approach. Declare 1000 variables, take input in all variables, then find average and finally print its average. The above increases length of code, complexity and degrades performance. If you don’t believe, try to code solution using above approach.
To solve above problem efficiently we use arrays. Arrays are good at handling collection of data (collection of 1000 student marks). Mind that in programming, we will always use some data structure (array in our case) to handle a collection of data efficiently.
How to use arrays?

Array in memory is stored as a continuous sequence of bytes. Like variables we give name to an array. However unlike variables, arrays are multi-valued they contain multiple values. Hence you cannot access specific array element directly.
For example, you can write sum = 432; to access sum . But, you cannot access specific array element directly by using array variable name. You cannot write marks to access 4 th student marks.
In array, we use an integer value called index to refer at any element of array. Array index starts from 0 and goes till N - 1 (where N is size of the array). In above case array index ranges from 0 to 4 .
To access individual array element we use array variable name with index enclosed within square brackets [ and ] . To access first element of marks array, we use marks[0] . Similarly to access third element we use marks[2] .
How to declare an array?
Syntax to declare an array.
- data_type is a valid C data type that must be common to all array elements.
- array_name is name given to array and must be a valid C identifier .
- SIZE is a constant value that defines array maximum capacity.
Example to declare an array
How to initialize an array.
There are two ways to initialize an array.
- Static array initialization – Initializes all elements of array during its declaration.
- Dynamic array initialization – The declared array is initialized some time later during execution of program.
Static initialization of array
We define value of all array elements within a pair of curly braces { and } during its declaration. Values are separated using comma , and must be of same type.
Example of static array initialization
Note: Size of array is optional when declaring and initializing array at once. The C compiler automatically determines array size using number of array elements. Hence, you can write above array initialization as.
Dynamic initialization of array
You can assign values to an array element dynamically during execution of program. First declare array with a fixed size. Then use the following syntax to assign values to an element dynamically.
Example to initialize an array dynamically
Instead of hard-coding marks values, you can ask user to input values to array using scanf() function.
The array index is an integer value, so instead of hard-coding you can wrap array input code inside a loop .
The above code will run 5 times from 0 to 4 . In each iteration it ask user to input an integer and stores it in successive elements of marks array.
Example program to implement one-dimensional array
Let us write a C program to declare an array capable of storing 10 student marks. Input marks of all 10 students and find their average.
Output –
Array best practices
- Arrays are fixed size, hence always be cautious while accessing arrays. Accessing an element that does not exists is undefined. You may get a valid value or the program may crash.For example, consider the below program. int array[5]; // Declare an integer array of size 5 /* Accessing sixth element of array which is undefined. */ array[5] = 50; /* Accessing -1th element of array is undefined */ array[-1] = 10;
- Always keep in mind that all array element store a value of similar type.
Recommended array example programs
- Program to read and print array element.
- Program to find maximum and minimum element in array.
- Program to insert a new element in array.
- Program to search an element in array.
- Program to sort array elements.
Practice more array programming exercises to learn more.

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Arrays in C
Arrays in C are a kind of data structure that can store a fixed-size sequential collection of elements of the same data type . Arrays are used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
What is an Array in C?
An array in C is a collection of data items of similar data type. One or more values same data type, which may be primary data types (int, float, char), or user-defined types such as struct or pointers can be stored in an array. In C, the type of elements in the array should match with the data type of the array itself.
The size of the array, also called the length of the array, must be specified in the declaration itself. Once declared, the size of a C array cannot be changed. When an array is declared, the compiler allocates a continuous block of memory required to store the declared number of elements.
Why Do We Use Arrays in C?
Arrays are used to store and manipulate the similar type of data.
Suppose we want to store the marks of 10 students and find the average. We declare 10 different variables to store 10 different values as follows −
These variables will be scattered in the memory with no relation between them. Importantly, if we want to extend the problem of finding the average of 100 (or more) students, then it becomes impractical to declare so many individual variables.
Arrays offer a compact and memory-efficient solution. Since the elements in an array are stored in adjacent locations, we can easily access any element in relation to the current element. As each element has an index, it can be directly manipulated.
Example: Use of an Array in C
To go back to the problem of storing the marks of 10 students and find the average, the solution with the use of array would be −
Run the code and check its output −
Array elements are stored in contiguous memory locations. Each element is identified by an index starting with "0". The lowest address corresponds to the first element and the highest address to the last element.
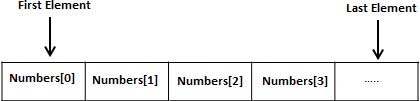
Declaration of an Array in C
To declare an array in C, you need to specify the type of the elements and the number of elements to be stored in it.
Syntax to Declare an Array
The "size" must be an integer constant greater than zero and its "type" can be any valid C data type. There are different ways in which an array is declared in C.
Declaring an Uninitialized Array
In such type of declaration, the uninitialized elements in the array may show certain random garbage values.
Example: Declaring an Array in C
In the following example, we are declaring an array of 5 integers and printing the indexes and values of all array elements −
Initialization of an Array in C
At the time of declaring an array, you can initialize it by providing the set of comma-separated values enclosed within the curly braces {}.
Syntax to Initialize an Array
If a set of comma-separated sequence values put inside curly brackets is assigned in the declaration, the array is created with each element initialized with their corresponding value.
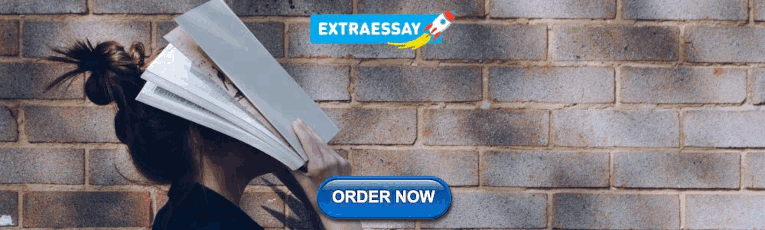
Example to Initialize an Array
The following example demonstrates the initialization of an integer array:
Example of Initializing all Array Elements to 0
To initialize all elements to 0, put it inside curly brackets
When you run this code, it will produce the following output −
Example of Partial Initialization of an Array
If the list of values is less than the size of the array, the rest of the elements are initialized with "0".
Example of Partial and Specific Elements Initialization
If an array is partially initialized, you can specify the element in the square brackets.
On execution, it will produce the following output −
Getting Size of an Array in C
The compiler allocates a continuous block of memory. The size of the allocated memory depends on the data type of the array.
Example 1: Size of Integer Array
If an integer array of 5 elements is declared, the array size in number of bytes would be "sizeof(int) x 5"
On execution, you will get the following output −
The sizeof operator returns the number of bytes occupied by the variable.
Example 2: Adjacent Address of Array Elements
The size of each int is 4 bytes. The compiler allocates adjacent locations to each element.
In this array, each element is of int type. Hence, the 0th element occupies the first 4 bytes 642016 to 19. The element at the next subscript occupies the next 4 bytes and so on.
Example 3: Array of Double Type
If we have the array type of double type, then the element at each subscript occupies 8 bytes
Example 4: Size of Character Array
The length of a "char" variable is 1 byte. Hence, a char array length will be equal to the array size.
Accessing Array Elements in C
Each element in an array is identified by a unique incrementing index, stating with "0". To access the element by its index, this is done by placing the index of the element within square brackets after the name of the array.
The elements of an array are accessed by specifying the index (offset) of the desired element within the square brackets after the array name. For example −
The above statement will take the 10th element from the array and assign the value to the "salary".
Example to Access Array Elements in C
The following example shows how to use all the three above-mentioned concepts viz. declaration, assignment, and accessing arrays.
On running this code, you will get the following output −
The index gives random access to the array elements. An array may consist of struct variables, pointers and even other arrays as its elements.
More on C Arrays
Arrays, being an important concept in C, need a lot more attention. The following important concepts related to arrays should be clear to a C programmer −
To Continue Learning Please Login

Welcome.please sign up.
By signing up or logging in, you agree to our Terms of service and confirm that you have read our Privacy Policy .
Already a member? Go to Log In
Welcome.please login.
Forgot your password
Not registered yet? Go to Sign Up
- Introduction
- Let's start
- Decide if/else
- Loop and loop
- Decide and loop
- My functions
- Pre-processor
- Enjoy with files
- Dynamic memory
- Storage classes
Arrays in C
In simple English, an array is a collection.
In C also, it is a collection of similar type of data which can be either of int, float, double, char (String), etc. All the data types must be same. For example, we can't have an array in which some of the data are integer and some are float.

Suppose we need to store marks of 50 students in a class and calculate the average marks. So, declaring 50 separate variables will do the job but no programmer would like to do so. And there comes array in action.
How to declare an array
datatype array_name [ array_size ] ;
For example, take an array of integers 'n'.
n[ ] is used to denote an array 'n'. It means that 'n' is an array.
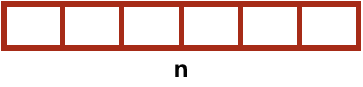
We need to give the size of the array because the complier needs to allocate space in the memory which is not possible without knowing the size. Compiler determines the size required for an array with the help of the number of elements of an array and the size of the data type present in the array.
Here 'int n[6]' will allocate space to 6 integers.
We can also declare an array by another method.
int n[ ] = {2, 3, 15, 8, 48, 13};
In this case, we are declaring and assigning values to the array at the same time. Here, there is no need to specify the array size because compiler gets it from { 2,3,15,8,48,13 } .
Index of an Array
Every element of an array has its index. We access any element of an array using its index.
Pictorial view of the above mentioned array is:
0, 1, 2, 3, 4 and 5 are indices. It is like they are identity of 6 different elements of an array. Index always starts from 0. So, the first element of an array has a index of 0.
We access any element of an array using its index and the syntax to do so is:
array_name[index]
For example, if the name of an array is 'n', then to access the first element (which is at 0 index), we write n[0] .
Here, n[0] is 2 n[1] is 3 n[2] is 15 n[3] is 8 n[4] is 48 n[5] is 13

n[0] , n[1] , etc. are like any other variables we were using till now i.e., we can set there value as n[0] = 5; like we do with any other variables ( x = 5; , y = 6; , etc.).
Assigning Values to Array
By writing int n[ ]={ 2,4,8 }; , we are declaring and assigning values to the array at the same time, thus initializing it.
But when we declare an array like int n[3]; , we need to assign values to it separately. Because 'int n[3];' will definitely allocate space of 3 integers in memory but there are no integers in that space.
To initialize it, assign a value to each of the elements of the array.
n[0] = 2; n[1] = 4; n[2] = 8;
It is just like we are declaring some variables and then assigning values to them.
int x,y,z; x=2; y=4; z=8;
Thus, the first way of assigning values to the elements of an array is by doing so at the time of its declaration.
int n[ ]={ 2,4,8 };
And the second method is declaring the array first and then assigning values to its elements.
int n[3]; n[0] = 2; n[1] = 4; n[2] = 8;
You can understand this by treating n[0] , n[1] and n[2] as similar to different variables you used before.
Just like variable, array can be of any other data type also.
float f[ ]= { 1.1, 1.4, 1.5};
Here, 'f' is an array of floats.
First, let's see the example to calculate the average of the marks of 3 students. Here, marks[0] represents the marks of the first student, marks[1] represents marks of the second and marks[2] represents marks of the third student.
Here you just saw a working example of array, we treated elements of the array in an exactly similar way as we had treated normal variables. &marks[0], &marks[1] and &marks[2] represent the addresses of marks[0], marks[1] and marks[2] respectively.
We can also use for loop as done in the next example.
The above code was just to make you familiar with using loops with an array because you will be doing this many times later.
The code is simple, 'i' and 'j' start from 0 because the index of an array starts from 0 and goes up to 9 (for 10 elements). So, 'i' and 'j' goes up to 9 and not 10 ( i<10 and j<10 ). So, in the code n[i] will be n[1], n[2], ...., n[9] and things will go accordingly.
Suppose we declare and initialize an array as
int n[5] = { 12, 13, 5 };
This means that n[0]=12, n[1]=13 and n[2]=5 and rest all elements are zero i.e. n[3]=0 and n[4]=0.
int n[5]; n[0] = 12; n[1] = 13; n[2] = 5;
In the above code, n[0], n[1] and n[2] are initialized to 12, 13 and 5 respectively. Therefore, n[4] and n[5] are both 0.
Pointer to Arrays
Till now, we have seen how to declare and initialize an array. Now, we will see how we can have pointers to arrays too. But before starting, we are assuming that you have gone through Pointers from the topic Point Me . If not, then first read the topic Pointers and practice some problems from the Practice section .
As we all know that pointer is a variable whose value is the address of some other variable i.e., if a variable 'y' points to another variable 'x', it means that the value of the variable 'y' is the address of 'x'.
Similarly, if we say that a variable 'y' points to an array 'n', it would mean that the value of 'y' is the address of the first element of the array i.e. n[0]. It means that the pointer of an array is the pointer of its first element.
If 'p' is a pointer to array 'age', means that p (or age) points to age[0].
int age[50]; int *p; p = age;
The above code assigns 'p' the address of the first element of the array 'age'.
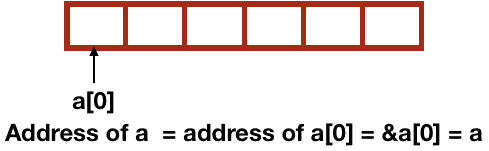
Now, since 'p' points to the first element of array 'age', '*p' is the value of the first element of the array.
So, *p is age[0], *(p+1) is age[1], *(p+2) is age[2].
Similarly, *age is age[0] (value at age), *(age+1) is age[1] (value at age+1), *(age+2) is age[2] (value at age+2) and so on.
That's all in pointer to arrays.
Now let's see some examples.
As 'p' is pointing to the first element of array, so, *p or *(p+0) represents the value at p[0] or the value at the first element of 'p'. Similarly, *(p+1) represents value at p[1]. And *(p+3) and *(p+4) represents p[3] and p[4] respectively. So accordingly, things were printed.
The above example sums up the above concepts. Now, let's print the address of the array and also individual elements of the array.
As you have noticed that the address of the first element of n and p are same and this means I was not lying! You can print other elements' addresses by using (p+1), (p+2) and (p+3) also.
Let's pass whole Array in Function
In C, we can pass an element of an array or the full array as an argument to a function.
Let's first pass a single array element to function.
Passing an entire Array in a Function
We can also pass the entire array to a function by passing array name as the argument. Yes, the trick is that we will pass the address of an array, that is the address of the first element of the array. Thus by having the pointer of the first element, we can get the entire array as we have done in examples above.

Let's see an example to understand it.
average(float a[]) → It is the function that is taking an array of float. And rest of the body of the function is performing accordingly.
b = average(n) → One thing you should note here is that we passed 'n'. And as discussed earlier, 'n' is the pointer to the first element or pointer to the array n[] . So, we have actually passed the pointer.
In the above example in which we calculated the average of the values of the elements of an array, we already knew the size of the array i.e. 8.
Suppose we are taking the size of the array from the user. In that case, the size of the array is not fixed. Here, we need to pass the size of array as the second argument to the function.
The code is similar to the previous one except that we passed the size of array explicitly - float average(float a[], int size ) .
We can also pass an array to a function using pointers. Let's see how.
In the above example, the address of the array i.e. address of n[0] is passed to the formal parameters of the function.
display(int *p) → This means that function 'display' is taking a pointer to an integer.
Now we passed the pointer of an integer i.e. pointer of the array n[] - 'n' as per the demand of our function 'display'.
Since 'p' is the address of the array n[] in the function 'display' i.e., address of the first element of the array (n[0]), therefore *p represents the value of n[0]. In the for loop in function, p++ increases the value of p by 1. So, when i=0, the value of *p gets printed. Then p++ increases *p to *(p+1) and in the second loop, the value of *(p+1) i.e. n[1] also gets printed. This loop continues till i=7 when the value of *(p+7) i.e. n[7] gets printed.

What if arrays are 2 dimensional?
Yes, 2-dimensional arrays also exist and are generally known as matrix . These consist of rows and columns.
Before going into its application, let's first see how to declare and initialize a 2D array.
Declaration of 2D Array
Similar to one-dimensional array, we define a 2-dimensional array as below.
int a[2][4];
Here, 'a' is a 2D array of integers which consists of 2 rows and 4 columns .
Now let's see how to initialize a 2-dimensional array.
Assigning Values to a 2 D Array
Same as in one-dimensional array, we can assign values to the elements of a 2-dimensional array in 2 ways as well.
In the first method, just assign a value to the elements of the array. If no value is assigned to any element, then its value is assumed to be zero.
Suppose we declared a 2-dimensional array a[2][2] . Now, we need to assign values to its elements.
int a[2][2]; a[0][0]=1; a[0][1]=2; a[1][0]=3; a[1][1]=4;
The second way is to declare and assign values at the same time as we did in one-dimensional array.
int a[2][3] = { 1, 2, 3, 4, 5, 6 };
Here, value of a[0][0] is 1, a[0][1] is 2, a[0][2] is 3, a[1][0] is 4, a[1][1] is 5 and a[1][2] is 6.
We can also write the above code as:
int a[2][3] = { {1, 2, 3}, {4, 5, 6 } };
Let's consider different cases of assigning values to an array at the time of declaration.
int a[2][2] = { 1, 2, 3, 4 }; /* valid */ int a[ ][2] = { 1, 2, 3, 4 }; /* valid */ int a[2][ ] = { 1, 2, 3, 4 }; /* invalid */ int a[ ][ ] = { 1, 2, 3, 4 }; /* invalid */
Why use of 2 D Array
Suppose we have 3 students each studying 2 subjects (subject 1 and subject 2) and we have to display the marks in both the subjects of the 3 students. Let's input the marks from the user.
This is something like
- Array vs Linked list in C
- Prime numbers using Sieve Algorithm in C
- Sorting an array using bubble sort in C
- Sorting an array using selection sort in C
- Sorting an array using insertion sort in C
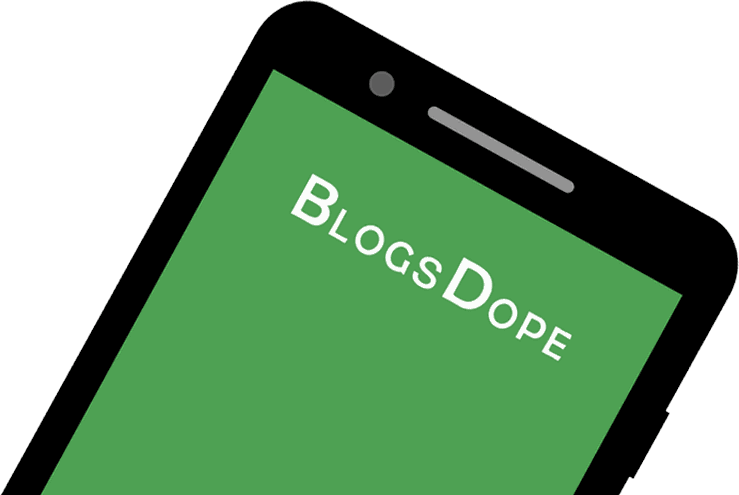
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- Properties of Array in C
- Length of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Jagged Array or Array of Arrays in C with Examples
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- How to pass an array by value in C ?
- Variable Length Arrays (VLAs) in C
- What are the data types for which it is not possible to create an array?
- Strings in C
Array of Strings in C
- C Library - <string.h>
- C String Functions
- What is the difference between single quoted and double quoted declaration of char array?
- Array C/C++ Programs
- String C/C++ Programs
In C programming String is a 1-D array of characters and is defined as an array of characters. But an array of strings in C is a two-dimensional array of character types. Each String is terminated with a null character (\0). It is an application of a 2d array.
- var_name is the name of the variable in C.
- r is the maximum number of string values that can be stored in a string array.
- c is the maximum number of character values that can be stored in each string array.
Example:
Below is the Representation of the above program
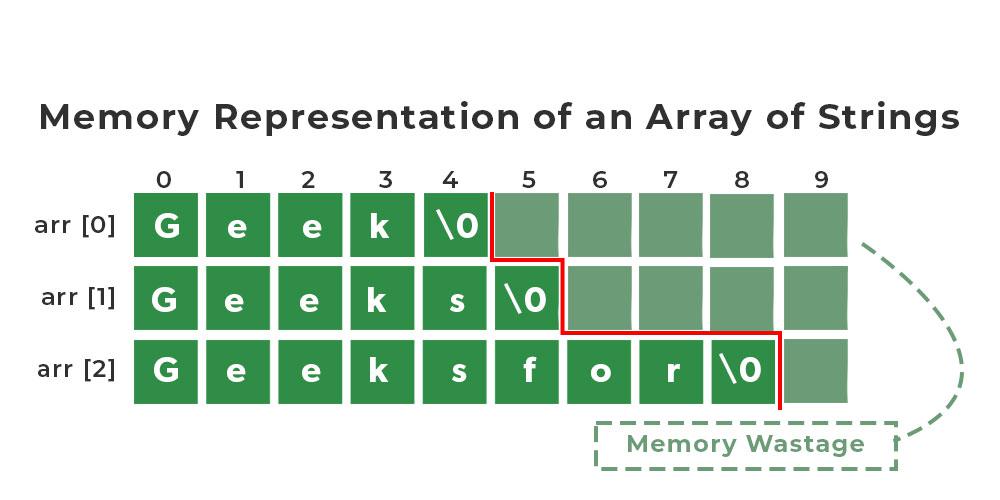
We have 3 rows and 10 columns specified in our Array of String but because of prespecifying, the size of the array of strings the space consumption is high. So, to avoid high space consumption in our program we can use an Array of Pointers in C.
Invalid Operations in Arrays of Strings
We can’t directly change or assign the values to an array of strings in C.
Here, arr[0] = “GFG”; // This will give an Error that says assignment to expression with an array type.
To change values we can use strcpy() function in C
Array of Pointers of Strings
In C we can use an Array of pointers. Instead of having a 2-Dimensional character array, we can have a single-dimensional array of Pointers. Here pointer to the first character of the string literal is stored.
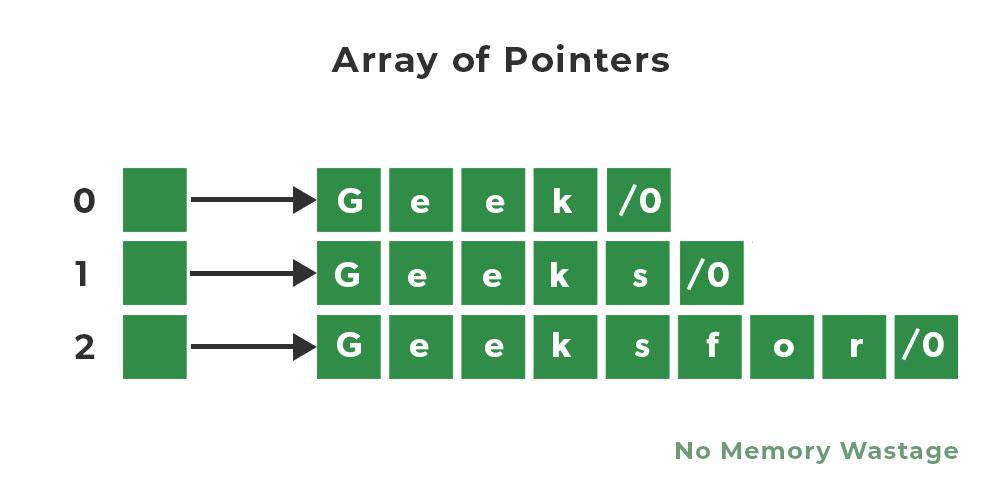
Below is the C program to print an array of pointers:
Please Login to comment...
Similar reads.
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Std:: array.
std::array is a container that encapsulates fixed size arrays.
This container is an aggregate type with the same semantics as a struct holding a C-style array T [ N ] as its only non-static data member. Unlike a C-style array, it doesn't decay to T * automatically. As an aggregate type, it can be initialized with aggregate-initialization given at most N initializers that are convertible to T : std :: array < int , 3 > a = { 1 , 2 , 3 } ; .
The struct combines the performance and accessibility of a C-style array with the benefits of a standard container, such as knowing its own size, supporting assignment, random access iterators, etc.
std::array satisfies the requirements of Container and ReversibleContainer except that default-constructed array is not empty and that the complexity of swapping is linear, satisfies the requirements of ContiguousContainer , (since C++17) and partially satisfies the requirements of SequenceContainer .
There is a special case for a zero-length array ( N == 0 ). In that case, array. begin ( ) == array. end ( ) , which is some unique value. The effect of calling front ( ) or back ( ) on a zero-sized array is undefined.
An array can also be used as a tuple of N elements of the same type.
[ edit ] Iterator invalidation
As a rule, iterators to an array are never invalidated throughout the lifetime of the array. One should take note, however, that during swap , the iterator will continue to point to the same array element, and will thus change its value.
[ edit ] Template parameters
[ edit ] member types, [ edit ] member functions, [ edit ] non-member functions, [ edit ] helper classes, [ edit ] example, [ edit ] see also.
- Todo with reason
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 22 December 2023, at 23:28.
- This page has been accessed 4,735,567 times.
- Privacy policy
- About cppreference.com
- Disclaimers

C String – How to Declare Strings in the C Programming Language
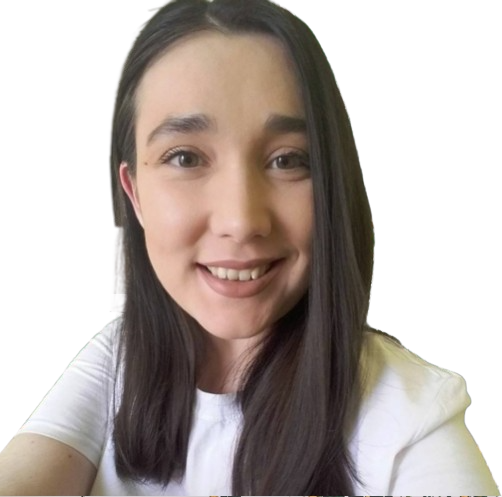
Computers store and process all kinds of data.
Strings are just one of the many forms in which information is presented and gets processed by computers.
Strings in the C programming language work differently than in other modern programming languages.
In this article, you'll learn how to declare strings in C.
Before doing so, you'll go through a basic overview of what data types, variables, and arrays are in C. This way, you'll understand how these are all connected to one another when it comes to working with strings in C.
Knowing the basics of those concepts will then help you better understand how to declare and work with strings in C.
Let's get started!
Data types in C
C has a few built-in data types.
They are int , short , long , float , double , long double and char .
As you see, there is no built-in string or str (short for string) data type.
The char data type in C
From those types you just saw, the only way to use and present characters in C is by using the char data type.
Using char , you are able to to represent a single character – out of the 256 that your computer recognises. It is most commonly used to represent the characters from the ASCII chart.
The single characters are surrounded by single quotation marks .
The examples below are all char s – even a number surrounded by single quoation marks and a single space is a char in C:
Every single letter, symbol, number and space surrounded by single quotation marks is a single piece of character data in C.
What if you want to present more than one single character?
The following is not a valid char – despite being surrounded by single quotation marks. This is because it doesn't include only a single character inside the single quotation marks:
'freeCodeCamp is awesome'
When many single characters are strung together in a group, like the sentence you see above, a string is created. In that case, when you are using strings, instead of single quotation marks you should only use double quotation marks.
"freeCodeCamp is awesome"
How to declare variables in C
So far you've seen how text is presented in C.
What happens, though, if you want to store text somewhere? After all, computers are really good at saving information to memory for later retrieval and use.
The way you store data in C, and in most programming languages, is in variables.
Essentially, you can think of variables as boxes that hold a value which can change throughout the life of a program. Variables allocate space in the computer's memory and let C know that you want some space reserved.
C is a statically typed language, meaning that when you create a variable you have to specify what data type that variable will be.
There are many different variable types in C, since there are many different kinds of data.
Every variable has an associated data type.
When you create a variable, you first mention the type of the variable (wether it will hold integer, float, char or any other data values), its name, and then optionally, assign it a value:
Be careful not to mix data types when working with variables in C, as that will cause errors.
For intance, if you try to change the example from above to use double quotation marks (remember that chars only use single quotation marks), you'll get an error when you compile the code:
As mentioned earlier on, C doesn't have a built-in string data type. That also means that C doesn't have string variables!
How to create arrays in C
An array is essentially a variable that stores multiple values. It's a collection of many items of the same type.
As with regular variables, there are many different types of arrays because arrays can hold only items of the same data type. There are arrays that hold only int s, only float s, and so on.
This is how you define an array of ints s for example:
First you specify the data type of the items the array will hold. Then you give it a name and immediately after the name you also include a pair of square brackets with an integer. The integer number speficies the length of the array.
In the example above, the array can hold 3 values.
After defining the array, you can assign values individually, with square bracket notation, using indexing. Indexing in C (and most programming languages) starts at 0 .
You reference and fetch an item from an array by using the name of the array and the item's index in square brackets, like so:
What are character arrays in C?
So, how does everything mentioned so far fit together, and what does it have to do with initializing strings in C and saving them to memory?
Well, strings in C are actually a type of array – specifically, they are a character array . Strings are a collection of char values.
How strings work in C
In C, all strings end in a 0 . That 0 lets C know where a string ends.
That string-terminating zero is called a string terminator . You may also see the term null zero used for this, which has the same meaning.
Don't confuse this final zero with the numeric integer 0 or even the character '0' - they are not the same thing.
The string terminator is added automatically at the end of each string in C. But it is not visible to us – it's just always there.
The string terminator is represented like this: '\0' . What sets it apart from the character '0' is the backslash it has.
When working with strings in C, it's helpful to picture them always ending in null zero and having that extra byte at the end.
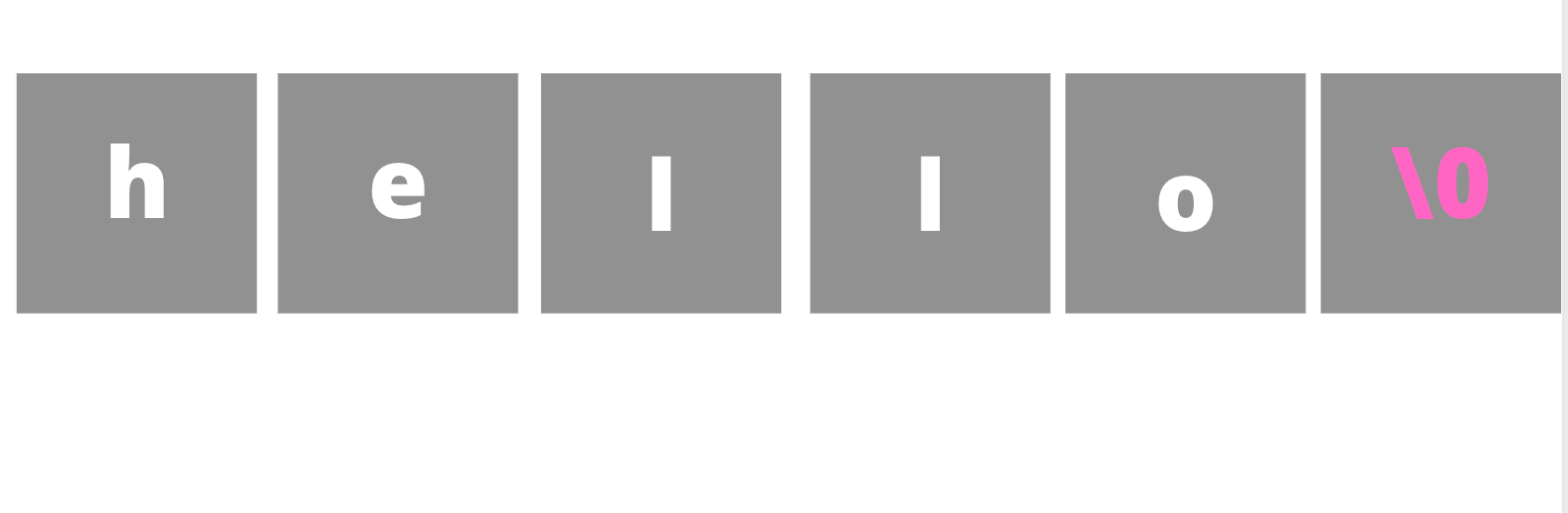
Each character takes up one byte in memory.
The string "hello" , in the picture above, takes up 6 bytes .
"Hello" has five letters, each one taking up 1 byte of space, and then the null zero takes up one byte also.
The length of strings in C
The length of a string in C is just the number of characters in a word, without including the string terminator (despite it always being used to terminate strings).
The string terminator is not accounted for when you want to find the length of a string.
For example, the string freeCodeCamp has a length of 12 characters.
But when counting the length of a string, you must always count any blank spaces too.
For example, the string I code has a length of 6 characters. I is 1 character, code has 4 characters, and then there is 1 blank space.
So the length of a string is not the same number as the number of bytes that it has and the amount of memory space it takes up.
How to create character arrays and initialize strings in C
The first step is to use the char data type. This lets C know that you want to create an array that will hold characters.
Then you give the array a name, and immediatelly after that you include a pair of opening and closing square brackets.
Inside the square brackets you'll include an integer. This integer will be the largest number of characters you want your string to be including the string terminator.
You can initialise a string one character at a time like so:
But this is quite time-consuming. Instead, when you first define the character array, you have the option to assign it a value directly using a string literal in double quotes:
If you want, istead of including the number in the square brackets, you can only assign the character array a value.
It works exactly the same as the example above. It will count the number of characters in the value you provide and automatically add the null zero character at the end:
Remember, you always need to reserve enough space for the longest string you want to include plus the string terminator.
If you want more room, need more memory, and plan on changing the value later on, include a larger number in the square brackets:
How to change the contents of a character array
So, you know how to initialize strings in C. What if you want to change that string though?
You cannot simply use the assignment operator ( = ) and assign it a new value. You can only do that when you first define the character array.
As seen earlier on, the way to access an item from an array is by referencing the array's name and the item's index number.
So to change a string, you can change each character individually, one by one:
That method is quite cumbersome, time-consuming, and error-prone, though. It definitely is not the preferred way.
You can instead use the strcpy() function, which stands for string copy .
To use this function, you have to include the #include <string.h> line after the #include <stdio.h> line at the top of your file.
The <string.h> file offers the strcpy() function.
When using strcpy() , you first include the name of the character array and then the new value you want to assign. The strcpy() function automatically add the string terminator on the new string that is created:
And there you have it. Now you know how to declare strings in C.
To summarize:
- C does not have a built-in string function.
- To work with strings, you have to use character arrays.
- When creating character arrays, leave enough space for the longest string you'll want to store plus account for the string terminator that is included at the end of each string in C.
- Define the array and then assign each individual character element one at a time.
- OR define the array and initialize a value at the same time.
- When changing the value of the string, you can use the strcpy() function after you've included the <string.h> header file.
If you want to learn more about C, I've written a guide for beginners taking their first steps in the language.
It is based on the first couple of weeks of CS50's Introduction to Computer Science course and I explain some fundamental concepts and go over how the language works at a high level.
You can also watch the C Programming Tutorial for Beginners on freeCodeCamp's YouTube channel.
Thanks for reading and happy learning :)
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Apache Arrow 16.1.0 Release
Published 14 May 2024 By The Apache Arrow PMC (pmc)
The Apache Arrow team is pleased to announce the 16.1.0 release. This is a minor release that includes 34 resolved issues from 16 distinct contributors . See the Install Page to learn how to get the libraries for your platform.
The release notes below are not exhaustive and only expose selected highlights of the release. Other bugfixes and improvements have been made: we refer you to the complete changelog .
The scratch space required by some Scalar subclasses is now immutable after initialization ( GH-40069 ). This fixes thread-safety bugs when this scratch space was lazily initialized, but introduces an API incompatibility because writing to the value member of some concrete Scalar subclasses is not allowed anymore. Affected classes include BaseBinaryScalar , BaseListScalar , SparseUnionScalar , DenseUnionScalar and RunEndEncodedScalar .
The bit_width and byte_width methods on ExtensionType now return the corresponding value for the underlying storage type ( GH-41353 ).
A regression that prevented reading BYTE_STREAM_SPLIT columns with null values was fixed ( GH-41562 ).
- Recompute a sliced array’s null count on demand when it is unknown ( GH-41136 )
- Support writing sliced arrays in the Arrow IPC format ( GH-40517 , GH-41225 , GH-41231 )
- Bug fixes for union array behaviour ( GH-41137 , GH-41140 )
- Enable support for reading date64 from CSV ( GH-41594 )
- Update MarshalJSON() for Float32 and Float64 to be able to handle NaN, +Inf and -Inf values ( GH-40563 )
JavaScript notes
- Store Timestamps in 64 bits ( GH-40959 )
- Update JS dependencies ( GH-40989 )
- Add at() for array like types ( GH-39131 )
- Refactor imports ( GH-39482 )
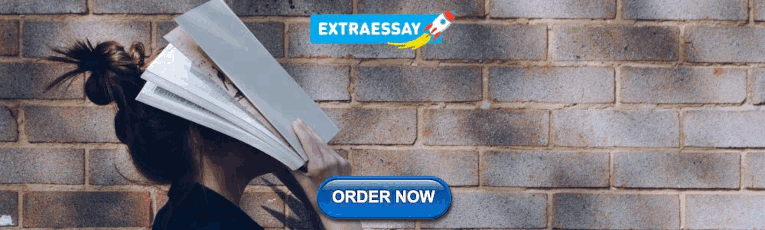
IMAGES
VIDEO
COMMENTS
Array is not a pointer. Arrays only decay to pointers causing a lots of confusion when people learn C language. You cant assign arrays in C. You only assign scalar types (integers, pointers, structs and unions) but not the arrays. Structs can be used as a workaround if you want to copy the array
Why does C++ support memberwise assignment of arrays within structs, but not generally? Arrays are not pointers. x here does refer to an array, though in many circumstances this "decays" (is implicitly converted) to a pointer to its first element. Likewise, y too is the name of an array, not a pointer. You can do array assignment within structs:
Array in C is one of the most used data structures in C programming. It is a simple and fast way of storing multiple values under a single name. In this article, we will study the different aspects of array in C language such as array declaration, definition, initialization, types of arrays, array syntax, advantages and disadvantages, and many more.
C Arrays; C Multidimensional Arrays; Pass arrays to a function in C; C Programming Pointers. C Pointers; Relationship Between Arrays and Pointers; ... It's important to note that the size and type of an array cannot be changed once it is declared. Access Array Elements.
Array is a type consisting of a contiguously allocated nonempty sequence of objects with a particular element type. The number of those objects (the array size) never changes during the array lifetime. ... Assignment. Objects of array type are not modifiable lvalues, and although their address may be taken, they cannot appear on the left hand ...
A string in C is a special case of array. • Array Type Designators : Referring to a specific array type. • Incomplete Array Types : Naming, but not allocating, a new array. • Limitations of C Arrays : Arrays are not first-class objects. • Multidimensional Arrays : Arrays of arrays. • Constructing Array Values : Assigning values to an ...
data_type array_name[SIZE]; data_type is a valid C data type that must be common to all array elements. ... Dynamic initialization of array. You can assign values to an array element dynamically during execution of program. First declare array with a fixed size. Then use the following syntax to assign values to an element dynamically.
An array in C is a collection of data items of similar data type. One or more values same data type, which may be primary data types (int, float, char), or user-defined types such as struct or pointers can be stored in an array. In C, the type of elements in the array should match with the data type of the array itself.
The general syntax for declaring an array in C looks as you can see it in the code snippet below: data_type array_name[array_size]; Let's take the following example: int my_numbers[5]; Let's break it down: I first defined the data type of the array, int. I then specified the name, my_numbers, followed by a pair of opening and closing square ...
In simple English, an array is a collection. In C also, it is a collection of similar type of data which can be either of int, float, double, char (String), etc. All the data types must be same. For example, we can't have an array in which some of the data are integer and some are float.
A C array is a data structure that stores a sequential collection of elements that are of a fixed size and the same type under a single name. It is used to hold a collection of primitive data, such as float, char, int, etc., in contiguous memory locations. The lowest address relates to the first element and the highest address to the last.
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
In C++, we can declare an array by simply specifying the data type first and then the name of an array with its size. data_type array_name[Size_of_array]; Example. int arr[5]; Here, int: It is the type of data to be stored in the array. We can also use other data types such as char, float, and double.
In C you cannot assign arrays directly. At first I thought this might because the C facilities were supposed to be implementable with a single or a few instructions and more complicated functionality was offloaded to standard library functions. After all using memcpy() is not that hard. However, one can directly assign structures/unions which ...
A declaration of the form T a [N];, declares a as an array object that consists of N contiguously allocated objects of type T.The elements of an array are numbered 0 , …, N -1, and may be accessed with the subscript operator [], as in a [0], …, a [N -1].. Arrays can be constructed from any fundamental type (except void), pointers, pointers to members, classes, enumerations, or from other ...
You can assign it in the same way: a = {1, 2, 3}. But that's actually C++0x ("extended initializer lists"). If you need to reassign the array in each loop iteration, you can still use memcpy. @archer: then you're almost surely wrong. Array allocation on the stack in just a stack pointer addition/subtraction.
But an array of strings in C is a two-dimensional array of character types. Each String is terminated with a null character (\0). It is an application of a 2d array. Syntax: char variable_name[r] = {list of string}; Here, var_name is the name of the variable in C. r is the maximum number of string values that can be stored in a string array.
std::array is a container that encapsulates fixed size arrays.. This container is an aggregate type with the same semantics as a struct holding a C-style array T [N] as its only non-static data member. Unlike a C-style array, it doesn't decay to T * automatically. As an aggregate type, it can be initialized with aggregate-initialization given at most N initializers that are convertible to T ...
How to create arrays in C. An array is essentially a variable that stores multiple values. It's a collection of many items of the same type. As with regular variables, there are many different types of arrays because arrays can hold only items of the same data type. There are arrays that hold only ints, only floats, and so on.
It modifies the length of the value stored. With %f you are attempting to store a 32-bit floating point number in a 64-bit variable. Since the sign-bit, biased exponent and mantissa are encoded in different bits depending on the size, using %f to attempt to store a double always fails` (e.g. the sign-bit is still the MSB for both, but e.g. a float has an 8-bit exponent, while a double has 11 ...
The bit_width and byte_width methods on ExtensionType now return the corresponding value for the underlying storage type . Parquet. A regression that prevented reading BYTE_STREAM_SPLIT columns with null values was fixed . C# notes. Recompute a sliced array's null count on demand when it is unknown
Then, correcting the data type, considering the char array is used, In the first case, arr = "Hello"; is an assignment, which is not allowed with an array type as LHS of assignment. OTOH, char arr[10] = "Hello"; is an initialization statement, which is perfectly valid statement. edited Oct 28, 2022 at 14:48. knittl.