4 Easy Ways to Copy a Dictionary in Python
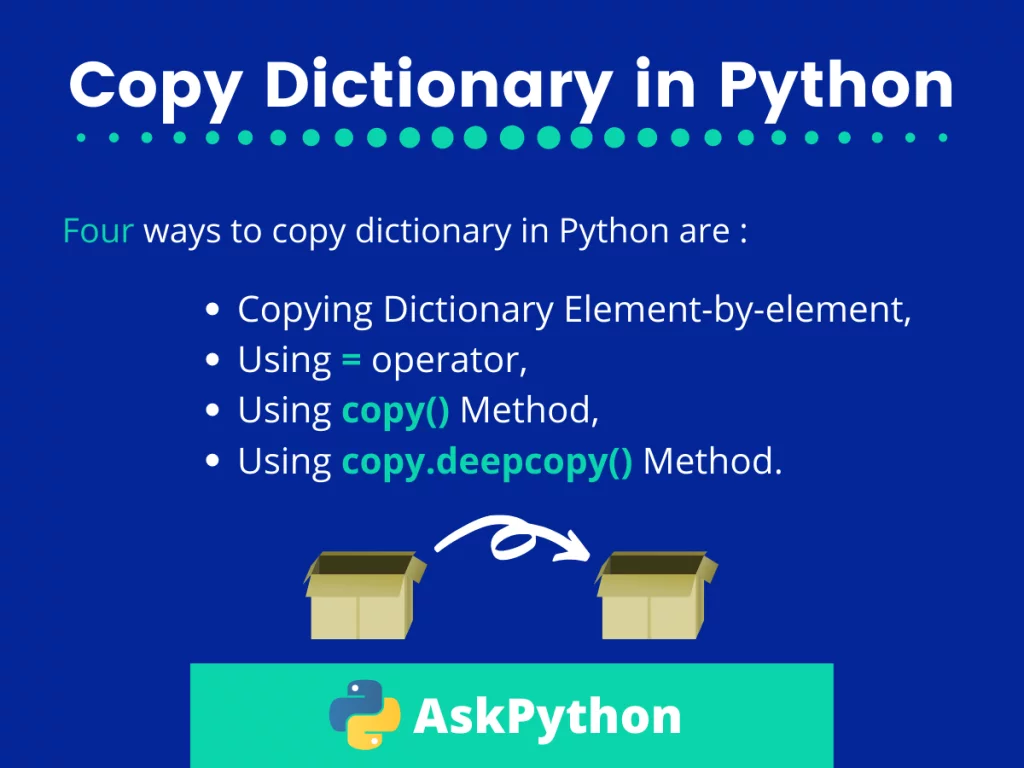
A dictionary in Python is an unordered collection of data that stores data in the form of key-value pair where each value can be accessed using its corresponding key.
A dictionary can contain large sets of key-value pairs, manually copying this dictionary can be hard and time-consuming. In Python, we have various ways to copy a dictionary using a few lines of code.
This tutorial will discuss the different methods or techniques to copy dictionaries in Python with examples.
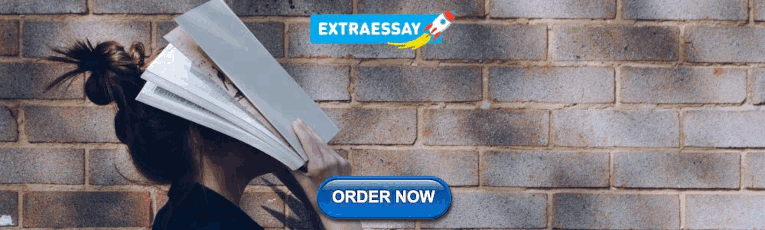
Methods to Copy a Dictionary in Python
In this section, we are going to detail the 4 different methods by that one can copy a dictionary in Python. Let’s learn about them one by one.
1. Copying Dictionary Element-by-element
In this technique, we loop through the entire dictionary and copy each element by its key into a new dictionary declared earlier.
In the above code:
- We initialized a dictionary dict1 and print it,
- Then we declare an empty dictionary dict2 where we are going to copy dict1,
- Next, we traverse through the dict1 using a for loop . And using the operation dict2[i]=dict1[i] , we copy each and every element from dict1 to dict2.
Note: The = operator creates a reference to iterable objects in the dictionary. Therefore, if a non-iterable element in dict2 is updated, the corresponding element in dict1 is unchanged. Whereas, if an iterable element like a list item is changed, we see it modify the original dictionary dict1 list as well. The second part of the above code explains it. After updating try to compare both dict1 and dict2 results. We see that the above statement is true.
2. Using the = operator to Copy a Dictionary in Python
We have a quick way to copy a dictionary in Python using the ‘=’ operator.
Let us see with an example how we can directly copy a dictionary in Python using an ‘=’ operator.
- Firstly we initialize a dictionary dict1 and directly copy it to a new object dict2 by the code line dict2=dict1 ,
- This operation copies references of each object present in dict1 to the new dictionary dict2,
- Hence, updating any element of the dict2 will result in a change in dict1 and vice versa,
- This is clear from the above code that when we update any (iterable or non-iterable) object in dict2, we also see the same change in dict1.
3. Using copy() Method
copy() is a Python copy dictionary method returns a shallow copy of the given dictionary. It is similar to what we saw previously in the case of copying elements by traversing through a dictionary, that is, references of the dictionary elements are inserted into the new dictionary(Shallow copy). Look at the below example:
- We initialize a dictionary dict1 with some values. And use the copy() method on it to create a shallow copy,
- After the copy has been made, we update the new elements and see the corresponding change in the original dictionary,
- Similar to the case of the element-by-element copying technique, here too, change in non-iterable elements of dict2 does not have any effect on the original dictionary,
- Whereas for iterable ones like lists, the change is reflected in the given dictionary dict1 too
4. Using copy.deepcopy() Method to Copy a Dictionary in Python
We can use another Python dictionary copy method deepcopy() to copy a dictionary in Python. The deepcopy() method in Python is a member of the copy module. It returns a new dictionary with copied elements of the passed dictionary. Note, this method copies all the elements of the given dictionary in a recursive manner. Let us see how can use it,
- In the first line, we import the copy module,
- Then, we initialize the original dictionary dict1,
- We use the copy.deepcopy() method to copy dict1 elements in the new dictionary dict2,
- After successful copying, we update the new copy and see the changes in the original dictionary,
- As we can see from the output, any change in dict2 is not reflected in dict1. Hence, this method is useful when we need to change the new dictionary in our code while keeping the original one intact.
In this tutorial, we have learned about the four different ways to copy a dictionary in Python: 1. Copying Dictionary Element-by-element , 2. Using = operator to Copy a Dictionary in Python , 3. Using copy() Method , and 4. Using copy.deepcopy() Method to Copy a Dictionary in Python . The best way to choose any one of the above methods is to try it yourself and find out what works best for you. Hope you enjoyed reading this tutorial.
- https://docs.python.org/3/library/copy.html
- https://stackoverflow.com/questions/5861498/fast-way-to-copy-dictionary-in-python
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
Add contents of a Dictionary to another Dictionary in C#
This post will discuss how to add the contents of a Dictionary to another Dictionary in C#. The solution should add key-value pairs present in the given dictionary into the source dictionary.
1. Using List<T>.ForEach() method
The idea is to convert the second dictionary into a List of KeyValuePair<K,V> Then, insert each entry into the first dictionary using the ForEach() method.
Download Run Code
The above code throws an ArgumentException when attempting to add a duplicate key.
To overwrite the value of a key already present in the dictionary, we can use dict[key] = value syntax instead of the Add() method. This is demonstrated below:
2. Using foreach loop
We can also iterate over the dictionary using a regular foreach loop and append all the second dictionary entries into the first dictionary. This solution handles duplicate keys as it uses the dict[key] property instead of the Add() method.
That’s all about adding contents of a Dictionary to another Dictionary in C#.
Initialize a Dictionary in C#
Add values to a Dictionary in C#
Get corresponding key from a given value in Dictionary in C#
Rate this post
Average rating 4.11 /5. Vote count: 9
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
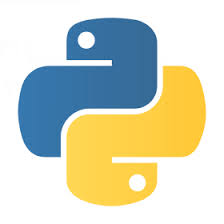
- Listing a directory using Python
How to insert a dictionary in another dictionary in Python (How to merge two dictionaries)
- range vs. xrange in Python
- List Comprehension vs Generator Expressions in Python
- Plain function or Callback - An example in Python
- Callback or Iterator in Python
- Function vs Generator in Python
- urllib vs urllib2 in Python - fetch the content of 404 or raise exception?
- Print HTML links using Python HTML Parser
- Extract HTML links using Python HTML Parser
- Creating an Iterator in Python
- Python Weekly statistics (using urllib2, HTMLParser and pickle)
- Solution: Number guessing game in Python
- Show Emoji in Python code
- for-else in Python indicating "value not found"
- Create your own interactive shell with cmd in Python
- Traversing directory tree using walk in Python - skipping .git directory
- Python: avoid importing everything using a star: *
- Type checking of Python code using mypy
- Python package dependency management - pip freeze - requirements.txt and constraints.txt
- Create images with Python PIL and Pillow and write text on them
- Python: get size of image using PIL or Pillow
- Write text on existing image using Python PIL - Pillow
- Crop images using Python PIL - Pillow
- Resize images using Python PIL Pillow
- Showing speed improvement using a GPU with CUDA and Python with numpy on Nvidia Quadro 2000D
- Send HTTP Requests in Python
- Command-line counter in Python
- Never use input() in Python 2
- Parallel processing in Python using fork
- Static web server in Python
- How to serialize a datetime object as JSON using Python?
- Skeleton: A minimal example generating HTML with Python Jinja
- Simple logging in Python
- Logging in Python
- Python argparse to process command line arguments
- Using the Open Weather Map API with Python
- Python: seek - move around in a file and tell the current location
- Python: Capture standard output, standard error, and the exit code of a subprocess
- Python: Iterate over list of tuples
- Print version number of Python module
- Python: Repeat the same random numbers using seed
- Python: split command line into pieces as the shell does - shlex.split()
- Python context tools - cwd, tmpdir
- Python and PostgreSQL
- RPC with Python using RPyC
- Python: Fermat primality test and generating co-primes
- Python UUID - Universally unique identifier
- Time left in process (progress bar) in Python
- Counter with Python and MongoDB
- qx or backticks in python - capture the output of external programs
- Calling Java from Python
- Python and ElasticSearch
- Python daemon (background service)
- Python: print stack trace after catching exception
- Python: logging in a library even before enabling logging
- Python atexit exit handle - like the END block of Perl
- Python: traversing dependency tree
- Creating PDF files using Python and reportlab
- Show file modification time in Python
- Static code analysis for Python code - PEP8, FLAKE8, pytest
- Python timeout on a function call or any code-snippet.
- Compile Python from source code
- Introduction to Python unittest
- Doctest in Python
- Testing Python: Getting started with Pytest
- Python testing with Pytest: Order of test functions - fixtures
- Python Pytest assertion error reporting
- Python: Temporary files and directory for Pytest
- Mocking input and output for Python testing
- Testing random numbers in Python using mocks
- Python: fixing random numbers for testing
- Python: PyTest fixtures - temporary directory - tmpdir
- Caching results to speed up process in Python
- Python unittest fails, but return exit code 0 - how to fix
- Testing with PyTest
- Parsing test results from JUnit XML files with Python
- Combine pytest reports
Merge Dictionaries
Insert dictionary into another dictionary.
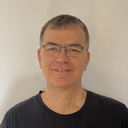
Published on 2015-02-16
Author: Gabor Szabo
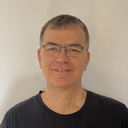
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers, Type Conversion and Mathematics
- Python List
- Python Tuple
- Python Sets
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python Dictionary Comprehension
Python Dictionary setdefault()
Python Dictionary clear()
Python Dictionary popitem()
- Python Dictionary update()
- Python Dictionary keys()
Python Nested Dictionary
In Python, a dictionary is an unordered collection of items. For example:
Here, dictionary has a key:value pair enclosed within curly brackets {} .
To learn more about dictionary, please visit Python Dictionary .
- What is Nested Dictionary in Python?
In Python, a nested dictionary is a dictionary inside a dictionary. It's a collection of dictionaries into one single dictionary.
Here, the nested_dict is a nested dictionary with the dictionary dictA and dictB . They are two dictionary each having own key and value.
- Create a Nested Dictionary
We're going to create dictionary of people within a dictionary.
Example 1: How to create a nested dictionary
When we run above program, it will output:
In the above program, people is a nested dictionary. The internal dictionary 1 and 2 is assigned to people . Here, both the dictionary have key name , age , sex with different values. Now, we print the result of people .
- Access elements of a Nested Dictionary
To access element of a nested dictionary, we use indexing [] syntax in Python.
Example 2: Access the elements using the [] syntax
In the above program, we print the value of key name using i.e. people[1]['name'] from internal dictionary 1 . Similarly, we print the value of age and sex one by one.
- Add element to a Nested Dictionary
Example 3: How to change or add elements in a nested dictionary?
In the above program, we create an empty dictionary 3 inside the dictionary people .
Then, we add the key:value pair i.e people[3]['Name'] = 'Luna' inside the dictionary 3 . Similarly, we do this for key age , sex and married one by one. When we print the people[3] , we get key:value pairs of dictionary 3 .
Example 4: Add another dictionary to the nested dictionary
In the above program, we assign a dictionary literal to people[4] . The literal have keys name , age and sex with respective values. Then we print the people[4] , to see that the dictionary 4 is added in nested dictionary people.
- Delete elements from a Nested Dictionary
In Python, we use “ del “ statement to delete elements from nested dictionary.
Example 5: How to delete elements from a nested dictionary?
In the above program, we delete the key:value pairs of married from internal dictionary 3 and 4 . Then, we print the people[3] and people[4] to confirm changes.
Example 6: How to delete dictionary from a nested dictionary?
In the above program, we delete both the internal dictionary 3 and 4 using del from the nested dictionary people . Then, we print the nested dictionary people to confirm changes.
- Iterating Through a Nested Dictionary
Using the for loops, we can iterate through each elements in a nested dictionary.
Example 7: How to iterate through a Nested dictionary?
In the above program, the first loop returns all the keys in the nested dictionary people . It consist of the IDs p_id of each person. We use these IDs to unpack the information p_info of each person.
The second loop goes through the information of each person. Then, it returns all of the keys name , age , sex of each person's dictionary.
Now, we print the key of the person’s information and the value for that key.
Key Points to Remember:
- Nested dictionary is an unordered collection of dictionary
- Slicing Nested Dictionary is not possible.
- We can shrink or grow nested dictionary as need.
- Like Dictionary, it also has key and value.
- Dictionary are accessed using key.
Table of Contents
- Key Points to Remember
Sorry about that.
Related Tutorials
Python Tutorial
Python Library
C# Add Dictionary to Dictionary
Introduction.
Merging dictionaries is a common operation in C# when working with key-value pairs. In this blog post, we'll explore various ways to add one dictionary to another, providing you with multiple techniques and examples for different scenarios.
Using Union Method
The Union method, available in LINQ, is a straightforward approach to merge two dictionaries. It creates a new sequence that contains distinct elements from both dictionaries.
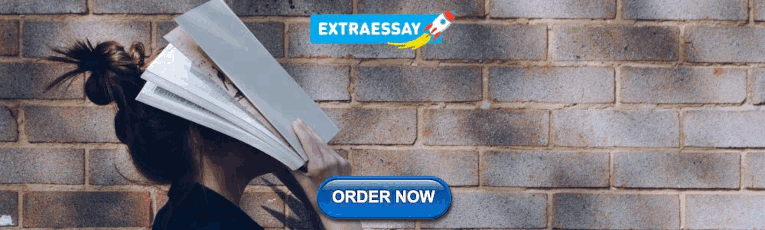
Example 1: Basic Dictionary Union
In this example, the Union method is used to combine the contents of dictionary1 and dictionary2 into a new dictionary.
Using Concat and ToDictionary Methods
The Concat method, along with ToDictionary , is another way to merge dictionaries. It concatenates two sequences and creates a dictionary from the resulting key-value pairs.
Example 2: Dictionary Concatenation
Here, Concat is used to concatenate the key-value pairs, and ToDictionary creates a new dictionary from the combined sequence.
Using Add Method
If you want to modify the original dictionary in place, you can use the Add method to add key-value pairs from one dictionary to another.
Example 3: In-Place Dictionary Addition
In this example, the Add method is used to add key-value pairs from additionalDict to originalDict in place.
By understanding these techniques, you can choose the method that best suits your specific use case when adding one dictionary to another in C#. Experiment with these examples in your Visual Studio Code (VS Code) IDE to enhance your proficiency in handling dictionaries efficiently.
🎓 Programming Mastery
Continue on your path to Programming mastery by selecting an article below!
Learn various methods to obtain unique values from an array in C#. Improve your coding skills with practical examples and ensure efficient handling of distinct elements in your arrays.
Explore how AutoMapper streamlines the mapping of enums to strings in C#. Enhance your coding experience with practical examples, showcasing the seamless integration of AutoMapper for efficient enum-to-string transformations. Improve code readability and reduce development time with these essential techniques.
Explore how AutoMapper simplifies mapping enum values in C#. Elevate your coding experience with practical examples, showcasing the seamless integration of enumeration types using AutoMapper.
Synonyms of assign
- as in to task
- as in to allot
- as in to cede
- as in to appoint
- More from M-W
- To save this word, you'll need to log in. Log In
Thesaurus Definition of assign
Synonyms & Similar Words
- share (out)
- parcel (out)
- redistribute
- reapportion
Antonyms & Near Antonyms
- deprive (of)
- appropriate
- pass (down)
- expropriate
- single (out)
Synonym Chooser
How is the word assign different from other verbs like it?
Some common synonyms of assign are ascribe , attribute , credit , and impute . While all these words mean "to lay something to the account of a person or thing," assign implies ascribing with certainty or after deliberation.
In what contexts can ascribe take the place of assign ?
The synonyms ascribe and assign are sometimes interchangeable, but ascribe suggests an inferring or conjecturing of cause, quality, authorship.
How is attribute related to other words for assign ?
Attribute suggests less tentativeness than ascribe , less definiteness than assign .
Where would credit be a reasonable alternative to assign ?
In some situations, the words credit and assign are roughly equivalent. However, credit implies ascribing a thing or especially an action to a person or other thing as its agent, source, or explanation.
When is it sensible to use impute instead of assign ?
While in some cases nearly identical to assign , impute suggests ascribing something that brings discredit by way of accusation or blame.
Thesaurus Entries Near assign
assiduousness
assignation
Cite this Entry
“Assign.” Merriam-Webster.com Thesaurus , Merriam-Webster, https://www.merriam-webster.com/thesaurus/assign. Accessed 11 May. 2024.
More from Merriam-Webster on assign
Nglish: Translation of assign for Spanish Speakers
Britannica English: Translation of assign for Arabic Speakers
Subscribe to America's largest dictionary and get thousands more definitions and advanced search—ad free!
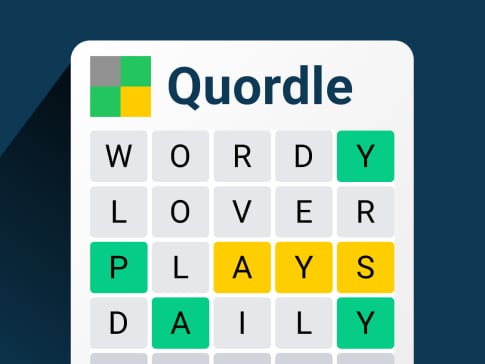
Can you solve 4 words at once?
Word of the day.
See Definitions and Examples »
Get Word of the Day daily email!
Popular in Grammar & Usage
More commonly misspelled words, your vs. you're: how to use them correctly, every letter is silent, sometimes: a-z list of examples, more commonly mispronounced words, how to use em dashes (—), en dashes (–) , and hyphens (-), popular in wordplay, the words of the week - may 10, a great big list of bread words, 10 scrabble words without any vowels, 8 uncommon words related to love, 9 superb owl words, games & quizzes.
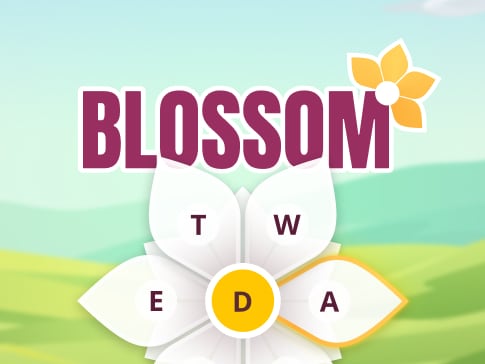

- Cambridge Dictionary +Plus
Synonyms and antonyms of assign in English
Word of the Day
gyroscopically
Your browser doesn't support HTML5 audio
with the use of a gyroscope (= a device containing a wheel that spins freely within a frame, used on aircraft, ships, etc. to help keep them horizontal)

Varied and diverse (Talking about differences, Part 1)
Learn more with +Plus
- Recent and Recommended {{#preferredDictionaries}} {{name}} {{/preferredDictionaries}}
- Definitions Clear explanations of natural written and spoken English English Learner’s Dictionary Essential British English Essential American English
- Grammar and thesaurus Usage explanations of natural written and spoken English Grammar Thesaurus
- Pronunciation British and American pronunciations with audio English Pronunciation
- English–Chinese (Simplified) Chinese (Simplified)–English
- English–Chinese (Traditional) Chinese (Traditional)–English
- English–Dutch Dutch–English
- English–French French–English
- English–German German–English
- English–Indonesian Indonesian–English
- English–Italian Italian–English
- English–Japanese Japanese–English
- English–Norwegian Norwegian–English
- English–Polish Polish–English
- English–Portuguese Portuguese–English
- English–Spanish Spanish–English
- English–Swedish Swedish–English
- Dictionary +Plus Word Lists
To add ${headword} to a word list please sign up or log in.
Add ${headword} to one of your lists below, or create a new one.
{{message}}
Something went wrong.
There was a problem sending your report.
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python - Assign list items to Dictionary
- Python - Accessing Items in Lists Within Dictionary
- Appending a dictionary to a list in Python
- Python | Convert two lists into a dictionary
- Python - Convert Index Dictionary to List
- Python - Common items Dictionary Value List
- Convert a Dictionary to a List in Python
- Python | Sort dictionary keys to list
- Convert a List to Dictionary Python
- Python - Convert Lists of List to Dictionary
- Python | Type conversion of dictionary items
- Python Dictionary items() method
- Adding Items to a Dictionary in a Loop in Python
- Python - Assign values to initialized dictionary keys
- Python - Flatten Dictionary with List
- Python - Pair lists elements to Dictionary
- Python - Create dictionary from the list
- Python - Convert Lists to Nested Dictionary
- Python - Keys associated with value list in dictionary
- Python - Change type of key in Dictionary list
Python – Assign list items to Dictionary
Sometimes, while working with Python dictionaries, we can have a problem in which we need to assign list elements as a new key in dictionary. This task can occur in web development domain. Lets discuss certain ways in which this task can be performed.
Method #1 : Using zip() + loop The combination of above functions can be used to solve this problem. In this, we combine list elements with dictionary using zip() and loop is used to combine iteration logic.
The original list is : [{‘Gfg’: 1, ‘id’: 2}, {‘Gfg’: 4, ‘id’: 4}] The modified dictionary : [{‘best’: 12, ‘Gfg’: 1, ‘id’: 2}, {‘best’: 2, ‘Gfg’: 4, ‘id’: 4}]
Time complexity: O(n), where n is the length of the input list. The loop iterates over each element of the input list once.
Auxiliary Space: O(n), where n is the length of the input list. The space used by the output list ‘res’ is proportional to the length of the input list. Additionally, a constant amount of space is used for initializing variables like ‘new_key’ and ‘add_list’.
Method #2 : Using list comprehension + zip() The combination of above functions can be used to solve this problem. In this, we perform the iteration of elements using list comprehension and hence a shorthand.
The time complexity of the given code is O(n), where n is the length of the input list test_list.
The space complexity of the code is O(n), where n is the length of the input list test_list
Method #3 : Using for loop and update() method
Time Complexity: O(n) where n is the number of elements in the dictionary. The loop and update() method is used to perform the task and it takes O(n) time. Auxiliary Space: O(n) additional space of size n is created where n is the number of elements in the dictionary.
Method #4: Using dictionary comprehension and zip()
This method uses dictionary comprehension and the zip() function to iterate over both test_list and add_list simultaneously. The ** operator is used to unpack each dictionary in test_list, and then the new key-value pair is added using new_key and the corresponding value from add_list. The resulting list of dictionaries is stored in result.
Time Complexity: O(n), where n is the length of the test_list.
Auxiliary Space: O(n), where n is the length of the test_list.
Method #5:Using map() and filter()
1. Initialize test_list, new_key, and add_list. 2. Use filter() to construct a list of dictionaries, with each dictionary having a single key new_key and a value from the add_list. Store the resulting list in res. 3. Use map() to iterate over each dictionary ele in test_list. 4. Use update() to assign the items in the corresponding dictionary from res to the dictionary ele. 5. Print the resulting modified test_list.
Time Complexity: O(N)
Creating the list of dictionaries using filter() takes O(N) time, where N is the length of the add_list. The map() function iterates over each dictionary in test_list, and the update() method takes O(1) time to assign a new item to a dictionary. Thus, the time complexity for updating each dictionary in test_list is O(1). Therefore, the overall time complexity of this algorithm is O(N), where N is the length of the add_list.
Auxiliary Space: O(N)
The space complexity of this algorithm depends on the size of the input lists and the number of dictionaries in test_list. The filter() method creates a new list of dictionaries with a size equal to the length of add_list, so the space complexity is O(N), where N is the length of the add_list. The map() function creates a new iterator object with a size equal to the length of test_list, so the space complexity is also O(N), where N is the length of test_list.
Method 6: Using a list comprehension with enumerate() function:
- Initialize the original list test_list, key new_key and list of values add_list.
- Use a list comprehension to iterate over the list test_list and enumerate function to get the current index and the current sub-dictionary.
- Use dictionary unpacking with **sub to create a new dictionary including the current sub-dictionary and the new key-value pair new_key: add_list[i] (with i being the current index).
- The list comprehension creates a new list of dictionaries with the updated values.
Time complexity: O(n), where n is the number of dictionaries in test_list.
Auxiliary space: O(n), where n is the number of dictionaries in test_list.
Method#7: Using Recursive method.
This function takes three arguments: test_list is the list of dictionaries to modify, new_key is the key to add to each dictionary, and add_list is the list of items to assign to the new_key. The function returns a new list of dictionaries with the new_key assigned to each dictionary.
The function works recursively by processing one sub-list at a time. The base case is when test_list is empty, in which case an empty list is returned. Otherwise, the function modifies the first dictionary in test_list by assigning the first item in add_list to the new_key, and then recursively calls itself with the remainder of test_list and add_list. The function concatenates the modified dictionary with the result of the recursive call, and returns the new list.
The time and space complexities of the recursive method for assigning list items to a dictionary depend on the size of the input list.
In the best case scenario, the input list is empty, and the function simply returns the input dictionary. In this case, the time complexity is O(1), and the space complexity is also O(1).
In the worst case scenario, the input list has n elements. In this case, the function needs to iterate through each element in the list and perform a constant number of operations (updating the dictionary). Therefore, the time complexity is O(n), and the space complexity is O(n) as well, since the recursive calls create n new dictionaries on the call stack.
Overall, the time and space complexities of the recursive method are linear with respect to
Please Login to comment...
Similar reads.
- Python dictionary-programs
- Python list-programs
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Object.assign()
The Object.assign() static method copies all enumerable own properties from one or more source objects to a target object . It returns the modified target object.
The target object — what to apply the sources' properties to, which is returned after it is modified.
The source object(s) — objects containing the properties you want to apply.
Return value
The target object.
Description
Properties in the target object are overwritten by properties in the sources if they have the same key . Later sources' properties overwrite earlier ones.
The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters . Therefore it assigns properties, versus copying or defining new properties. This may make it unsuitable for merging new properties into a prototype if the merge sources contain getters.
For copying property definitions (including their enumerability) into prototypes, use Object.getOwnPropertyDescriptor() and Object.defineProperty() instead.
Both String and Symbol properties are copied.
In case of an error, for example if a property is non-writable, a TypeError is raised, and the target object is changed if any properties are added before the error is raised.
Note: Object.assign() does not throw on null or undefined sources.
Cloning an object
Warning for deep clone.
For deep cloning , we need to use alternatives like structuredClone() , because Object.assign() copies property values.
If the source value is a reference to an object, it only copies the reference value.
Merging objects
Merging objects with same properties.
The properties are overwritten by other objects that have the same properties later in the parameters order.
Copying symbol-typed properties
Properties on the prototype chain and non-enumerable properties cannot be copied, primitives will be wrapped to objects, exceptions will interrupt the ongoing copying task, copying accessors, specifications, browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Polyfill of Object.assign in core-js
- Object.defineProperties()
- Enumerability and ownership of properties
- Spread in object literals
- Daily Crossword
- Word Puzzle
- Word Finder
- Word of the Day
- Synonym of the Day
- Word of the Year
- Language stories
- All featured
- Gender and sexuality
- All pop culture
- Writing hub
- Grammar essentials
- Commonly confused
- All writing tips
- Pop culture
- Writing tips
Advertisement
[ uh - sahyn ]
verb (used with object)
to assign rooms at a hotel.
to assign homework.
to assign one to guard duty.
to assign a day for a meeting.
Synonyms: determine , fix
to assign a cause.
Synonyms: offer , show , advance , allege , adduce
to assign a contract.
- Military. to place permanently on duty with a unit or under a commander.
verb (used without object)
- Law. to transfer property, especially in trust or for the benefit of creditors.
my heirs and assigns.
to assign an expert to the job
to assign advertising to an expert
to assign a day for the meeting
to assign a stone cross to the Vikings
- to transfer (one's right, interest, or title to property) to someone else
- also intr law (formerly) to transfer (property) to trustees so that it may be used for the benefit of creditors
- military to allocate (men or materials) on a permanent basis Compare attach
- computing to place (a value corresponding to a variable) in a memory location
- law a person to whom property is assigned; assignee
Discover More
Derived forms.
- asˈsigner , noun
- asˈsignably , adverb
- asˌsignaˈbility , noun
- asˈsignable , adjective
Other Words From
- as·signer Chiefly Law. as·sign·or [ uh, -sahy-, nawr, as-, uh, -, nawr ] , noun
- misas·sign verb
- nonas·signed adjective
- preas·sign verb (used with object)
- preas·signed adjective
- reas·sign verb (used with object)
- self-as·signed adjective
- unas·signed adjective
- well-as·signed adjective
Word History and Origins
Origin of assign 1
Synonym Study
Example sentences.
It is designed to listen to meetings with multiple participants and will parse discussion patterns to produce informative synopses and assign post-meeting action items.
Such randomized, double-blinded controlled trials randomly assign patients to receive a drug or a placebo, and don’t reveal to participants or doctors who is getting which.
That AI could pore over an astronaut’s symptoms and then recommend medical tests, make diagnoses and assign treatments.
So I rose beyond cleaning, to working as an operational dispatcher for cabin services in the American Airlines traffic control center, assign cleaning crews to each incoming aircraft.
Ideally, the Mars spaceship would be equipped with artificial intelligence that could consider an astronaut’s symptoms, recommend medical tests, make diagnoses and assign treatments.
Now the Kremlin will assign more loyal people to rule the region, mostly military leaders.
When we assign a primitive “not me” status to another individual or social group, it can—and does—take us down a destructive path.
Other folks can debate and assign blame for “who lost Iraq.”
Renee Richardson knows she'll likely never be able to assign blame for her son's death—she's done fighting for that.
Girls are directed through several pages of this until they are asked to assign the guy a series of pre-decided adjectives.
The designs of Russia have long been proverbial; but the exercise of the new art of printing may assign them new features.
With what honest pride did John Smith, the best farmer of them all, step to the fore and assign to each man his place!
If the lessee die, his executor or administrator can assign the remainder of his term.
As the lessee may assign or sublet unless forbidden, so may the lessor part with his interest in the leased premises.
If offered any dish of which you do not wish to partake, decline it, but do not assign any reason.
Related Words
Insert a dictionary into another dictionary
I am working with dictionaries collections I tried put into one dictionary other dictionary as value parameter but It is shown an error that mentions “Expression does nor produce a value”.
where is the error?

This is the string in the value parameter.
SubDicc.Add(“Value”+SubDicc.Count.ToString ,convert.ToDouble( row.Item(4).tostring))
Have you got any solution , how to use dictionary inside dictionary (i have got similar usecase to implement) ?
@RJ_Kadari , Above error is because Cesar Cu is trying to add a value to another dictionary instead of producing a value . i.e Adding a dictionary happens where a value has to be entered. May I know your complete requirement ?

actually i need to add into dictionary in below format , could you please check ?
{ “id”: “123124”, “MetaID”: “235346”, “logID”: “23694”, “ForcedFIelds”: [{ “label”: “FirstName”, “Value”: “abc”, “Mappings”: { “name”: “FirstName”, “datatype”: “String”, “elementType”: “Input” } }, { “label”: “LastName”, “Value”: “efg”, “Mappings”: { “name”: “LastName”, “datatype”: “String”, “elementType”: “Input” } } ], “FIelds”: [{ “label”: “City”, “Value”: “xyz”, “Mappings”: { “name”: “City”, “datatype”: “String”, “elementType”: “Select” } }, { “label”: “StateID”, “Value”: “HYD”, “Mappings”: { “name”: “StateID”, “datatype”: “String”, “elementType”: “Select” } } ]
@RJ_Kadari , Is this entire in a string ? Do you want to add this in a dictionary as a value ?
Can you provide some info on this in detail ??
Hi Dominic, Thanks, Would like to add below format using dictionary? { “label”: “FirstName”, “Value”: “abc”, “Mappings”: { “name”: “FirstName”, “datatype”: “String”, “elementType”: “Input” } }
are these details enough ?
@RJ_Kadari , Not sure about my suggestion. This can be done by JSON not by dictionary I think.
problem solved : we have trieated dictionary inner dictionary as string
Related Topics
Related Words and Phrases
Bottom_desktop desktop:[300x250].
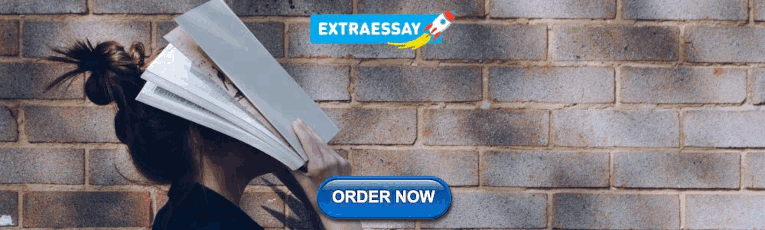
IMAGES
VIDEO
COMMENTS
Since Dictionary<TKey, TValue> explicitly implements the ICollection<KeyValuePair<TKey, TValue>>.Add method, you can also do this: var animalsAsCollection = (ICollection<KeyValuePair<string, string>>) Animals; foreach(var kvp in NewAnimals) animalsAsCollection.Add(kvp); It's a pity the class doesn't have an AddRange method like List<T> does.
In the above code: We initialized a dictionary dict1 and print it, Then we declare an empty dictionary dict2 where we are going to copy dict1, Next, we traverse through the dict1 using a for loop.And using the operation dict2[i]=dict1[i], we copy each and every element from dict1 to dict2.; Note: The = operator creates a reference to iterable objects in the dictionary.
Method #7: Using reduce(): Import the reduce() function from the functools module. Initialize the two dictionaries to be merged. Define a lambda function that takes two arguments: an accumulator dictionary and the next dictionary to merge, and returns a new dictionary that is the result of merging the accumulator dictionary with the next dictionary using the unpacking syntax **.
The solution should add key-value pairs present in the given dictionary into the source dictionary. 1. Using List<T>.ForEach() method. The idea is to convert the second dictionary into a List of KeyValuePair<K,V> Then, insert each entry into the first dictionary using the ForEach() method. The above code throws an ArgumentException when ...
There are two ways to "insert a dictionary in another dictionary". Merge Dictionaries. One of them is to merge the two. All the keys of one of the dictionaries become also the keys of the other dictionary. ... In order to see if these dictionaries are connected or not we can assign a new value to the 'Foo' key of the common dictionary: team ...
In Python, a nested dictionary is a dictionary inside a dictionary. It's a collection of dictionaries into one single dictionary. nested_dict = { 'dictA': {'key_1': 'value_1'}, 'dictB': {'key_2': 'value_2'}} Here, the nested_dict is a nested dictionary with the dictionary dictA and dictB. They are two dictionary each having own key and value.
Here, Concat is used to concatenate the key-value pairs, and ToDictionary creates a new dictionary from the combined sequence. Using Add Method. If you want to modify the original dictionary in place, you can use the Add method to add key-value pairs from one dictionary to another. Example 3: In-Place Dictionary Addition
It makes no sense to write the loop yourself. Part 1 We copy the Dictionary into the second Dictionary "copy" by using the copy constructor. Part 2 The code adds a key to the first Dictionary. And we see that the key added after the copy was made is not in the copy. Part 3 We print the contents of both collections.
Synonyms for ASSIGN: task, entrust, charge, trust, impose, intrust, confer, allocate; Antonyms of ASSIGN: deny, retain, deprive (of), withhold, keep, begrudge, appropriate, stint ... by one's authority for a specific position or duty the mayor assigned the panel with the task of luring a major sports franchise to the city. Synonyms & Similar ...
The auxiliary space complexity of this code is also O(N), as we use a map() object to store the updated values, and then we create a new dictionary using the zip() function. Method 6: Using defaultdict. Use the defaultdict class from the collections module to create a new dictionary with default values set to the values of the original dictionary.
ASSIGN - Synonyms, related words and examples | Cambridge English Thesaurus
The map() function iterates over each dictionary in test_list, and the update() method takes O(1) time to assign a new item to a dictionary. Thus, the time complexity for updating each dictionary in test_list is O(1). Therefore, the overall time complexity of this algorithm is O(N), where N is the length of the add_list. Auxiliary Space: O(N)
Dictionary1.Add(item.Key,item.Value) But It's not returning any value We can't use Assign activity here. dictionary1.Union (dictionary2).ToDictionary (Function (k) k.Key, function (v) v.Value) I am combining both dictionary and put all values into dictionary1 inside an assign activity.
Later sources' properties overwrite earlier ones. The Object.assign() method only copies enumerable and own properties from a source object to a target object. It uses [[Get]] on the source and [[Set]] on the target, so it will invoke getters and setters. Therefore it assigns properties, versus copying or defining new properties.
Assign definition: to give or allocate; allot. See examples of ASSIGN used in a sentence.
Does anyone know a better way to copy the items to another dictionary without using a foreach loop. I'm using the System.Collections.Generic. ... I have two dictionaries that I'm populating in a method and I want to copy the second smaller dictionary into the first one at the end of the method. ... Best way to assign key-value pairs to a ...
Have you got any solution , how to use dictionary inside dictionary (i have got similar usecase to implement) ? Dominic (Dominic) January 19, 2018, 12:56pm 3
To assign a responsibility, duty, or task to. To distribute or apportion by (or as if by) lot. To set aside for a specific use. To give to someone, especially for a specific purpose. To attribute something as belonging to or caused by. To legally give up one's possession or ownership of (something) To come to a judgment or decision on something.
choice1 = c_choice1. choice2 = c_choice2. b) lookup d1 and find the value for c_choice1, c_choice2, etc. c_choice1 = 1.10. c_choice2 = 1.20. c) create a final dictionary with choice1, choice2, etc in d2 replaced with the value in d1. My desired output (using general case as example):