Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
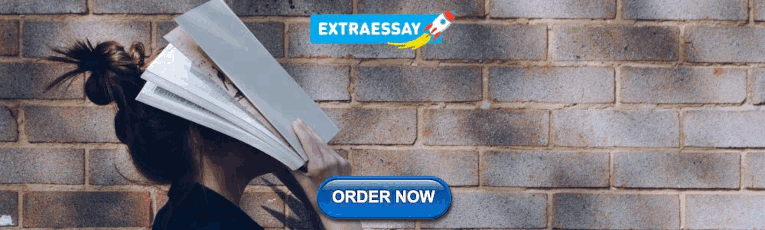
Redirect to another webpage with JavaScript/jQuery
This post will discuss how to redirect to another webpage in JavaScript and jQuery.
1. Redirecting to another webpage with JavaScript
There are several ways to redirect a page in JavaScript, all of which include the use of the window.location property, which returns a Location object, representing the location (URL) of the current document. To redirect to another webpage with JavaScript, we can use any of the following methods:
1. Using location.href
When we assign a URL to the window.location.href property, the associated document navigates to the new page from the same or different origin. Since window is a global object, window.location.href can be shortened to location.href . Also location is a synonym of location.href , we can directly assign a value to the location object.
2. Using location.assign() function
We can also navigate to a new URL using the location.assign() method. The assign() method loads a new web page by changing the location property. It takes a URL as an argument and replaces the current web page with the new one.
Note that the assign() method will save the current page in the session history, i.e., the user can go back using the back button. Also if the specified URL is of a different origin, it may fail due to CORS policy.
3. Using location.replace() function
There is another location object method called replace() , which replaces the current document with the document at the specified URL. Unlike the assign() method, this replaces the session History entry, and the user can’t navigate back to the originating page with the back button.
2. Redirecting to another webpage with jQuery
To redirect to another webpage with jQuery, we can assign a URL to href property of the location object using the .prop() or .attr() method. Here is the complete code for redirecting to another webpage with jQuery:
We can also directly assign a value to the location object.
That’s all about redirecting to another webpage in JavaScript and jQuery.
Refresh/Reload a page with JavaScript/jQuery
Get current URL with JavaScript/jQuery
Setup redirect from HTML page without using JavaScript
Rate this post
Average rating 4.83 /5. Vote count: 41
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
- JS Array Object
- JS Boolean Object
- JS Date Object
- JS Math Object
- JS Number Object
- JS String Object
- JS Reserved Keywords
- More references
JavaScript Window Location
In this tutorial you will learn about the JavaScript window location object.
The Location Object
The location property of a window (i.e. window.location ) is a reference to a Location object; it represents the current URL of the document being displayed in that window.
Since window object is at the top of the scope chain, so properties of the window.location object can be accessed without window. prefix, for example window.location.href can be written as location.href . The following section will show you how to get the URL of page as well as hostname, protocol, etc. using the location object property of the window object.
Getting the Current Page URL
You can use the window.location.href property to get the entire URL of the current page.
The following example will display the complete URL of the page on button click:
Getting Different Part of a URL
Similarly, you can use other properties of the location object such as protocol , hostname , port , pathname , search , etc. to obtain different part of the URL.
Try out the following example to see how to use the location property of a window.
Note: When you visit a website, you're always connecting to a specific port (e.g. http://localhost:3000). However, most browsers will simply not display the default port numbers, for example, 80 for HTTP and 443 for HTTPS.
Loading New Documents
You can use the assign() method of the location object i.e. window.location.assign() to load another resource from a URL provided as parameter, for example:
You can also use the replace() method to load new document which is almost the same as assign() . The difference is that it doesn't create an entry in the browser's history, meaning the user won't be able to use the back button to navigate to it. Here's an example:
Alternatively, you can use the window.location.href property to load new document in the window. It produce the same effect as using assign() method. Here's is an example:
Reloading the Page Dynamically
The reload() method can be used to reload the current page dynamically.
You can optionally specify a Boolean parameter true or false . If the parameter is true , the method will force the browser to reload the page from the server. If it is false or not specified, the browser may reload the page from its cache. Here's an example:
Note: The result of calling reload() method is different from clicking browser's Reload/Refresh button. The reload() method clears form control values that otherwise might be retained after clicking the Reload/Refresh button in some browsers.
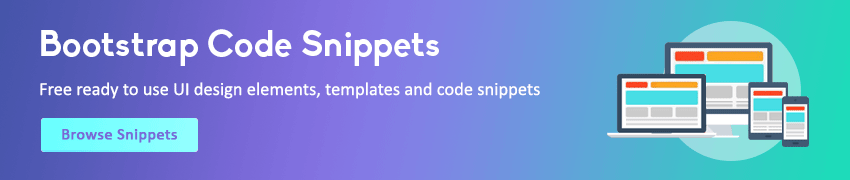
Is this website helpful to you? Please give us a like , or share your feedback to help us improve . Connect with us on Facebook and Twitter for the latest updates.
Interactive Tools
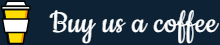
Learn Java and Programming through articles, code examples, and tutorials for developers of all levels.
- online courses
- certification
- free resources
5 ways to redirect a web page using JavaScript and jQuery
How to redirect a web page using javascript, 1. redirection using window.location.href of javascript, 2. redirection using replace() function, 3. redirection using window assign() function, 4. redirection using navigate function, how to redirect web page using jquery.
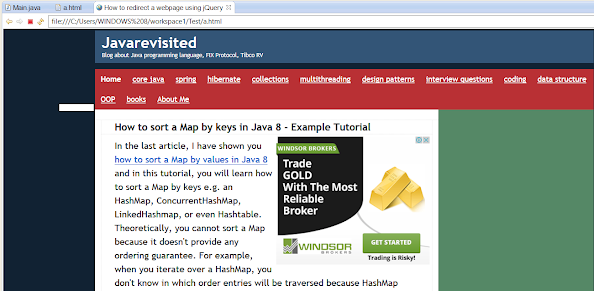
Great !!!!!
Hi Friend, Do you have any coding to redirect the web page from internet explorer to chrome when i click on a hyperlink
Sorry, you redirect to another site, not another browser, is that possible at all or did I misunderstood your question?
you can also do this with the below example setTimeout(function() { window.location.href = "https://www.google.com/"; }, 0);
How to display msg from php to jQuery page
How to use jQuery response
Hi, I have a question, If i want to redirect another page when i type another webpage, Is it possible from javascript?
Feel free to comment, ask questions if you have any doubt.
# window.location Cheatsheet
Looking for a site's URL information, then the window.location object is for you! Use its properties to get information on the current page address or use its methods to do some page redirect or refresh 💫
https://www.samanthaming.com/tidbits/?filter=JS#2
- Difference between host vs hostname
- How to change URL properties
- window.location vs location
- window.location.toString
- assign vs replace
- replace vs assign vs href
- Scratch your own itch 👍
- Community Input
# window.location Properties
# difference between host vs hostname.
In my above example, you will notice that host and hostname returns the value. So why do these properties. Well, it has do with the port number. Let's take a look.
URL without Port
https://www.samanthaming.com
URL with Port
https://www.samanthaming.com:8080
So host will include the port number, whereas hostname will only return the host name.
# How to change URL properties
Not only can you call these location properties to retrieve the URL information. You can use it to set new properties and change the URL. Let's see what I mean.
Here's the complete list of properties that you can change:
The only property you can't set is window.location.origin . This property is read-only.
# Location Object
The window.location returns a Location object. Which gives you information about the current location of the page. But you can also access the Location object in several ways.
The reason we can do this is because these are global variables in our browser.
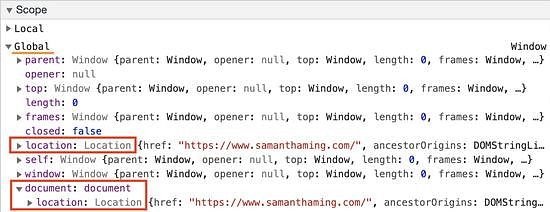
# window.location vs location
All 4 of these properties point at the same Location object. I personally prefer window.location and would actually avoid using location . Mainly because location reads more like a generic term and someone might accidentally name their variable that, which would override the global variable. Take for example:
I think that most developer is aware that window is a global variable. So you're less likely to cause confusion. To be honest, I had no idea location was a global variable until I wrote this post 😅. So my recommendation is to be more explicit and use window.location instead 👍
Here's my personal order of preference:
Of course, this is just my preference. You're the expert of your codebase, there is no best way, the best way is always the one that works best for you and your team 🤓
# window.location Methods
# window.location.tostring.
This method returns the USVString of the URL. It is a read-only version of Location.href
In other words, you can use it to get the href value from the
on this 😊. But I did find a performance test on the difference.
JSPerf: Location toString vs Location href
One thing I want to note about these speed tests is that it is browser specific. Different browser and versions will render different outcome. I'm using Chrome, so the href came out faster then the rest. So that's one I'll use. Also I think it reads more explicit then toString() . It is very obvious that href will provide the URL whereas toString seems like something it being converted to a string 😅
# assign vs replace
Both of these methods will help you redirect or navigate to another URL. The difference is assign will save your current page in history, so your user can use the "back" button to navigate to it. Whereas with replace method, it doesn't save it. Confused? No problem, I was too. Let's walk through an example.
Current Page
I just need to emphasize the "current page" in the definition. It is the page right before you call assign or replace .
# How to Do a Page Redirect
By now, you know we can change the properties of the window.location by assigning a value using = . Similarly, there are methods we can access to do some actions. So in regards to "how to redirect to another page", well there are 3 ways.
# replace vs assign vs href
All three does redirect, the difference has to do with browser history. href and assign are the same here. It will save your current page in history, whereas replace won't. So if you prefer creating an experience where the navigation can't press back to the originating page, then use replace 👍
So the question now is href vs assign . I guess this will come to personal preference. I like the assign better because it's a method so it feels like I'm performing some action. Also there's an added bonus of it being easier to test. I've been writing a lot of Jest tests, so by using a method, it makes it way easier to mock.
But for that that are rooting for href to do a page redirect. I found a performance test and running in my version of Chrome, it was faster. Again performance test ranges with browser and different versions, it may be faster now, but perhaps in future browsers, the places might be swapped.
JSPerf: href vs assign
# Scratch your own itch 👍
Okay, a bit of a tangent and give you a glimpse of how this cheatsheet came to be. I was googling how to redirect to another page and encountered the window.location object. Sometimes I feel a developer is a journalist or detective - there's a lot of digging and combing through multiple sources for you to gather all the information available. Honestly, I was overwhelmed with the materials out there, they all covered different pieces, but I just wanted a single source. I couldn't find much, so I thought, I'll cover this in a tidbit cheatsheet! Scratch your own itch I always say 👍
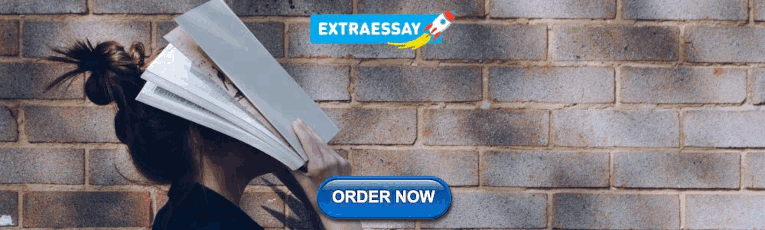
# Community Input
: This is awesome, I’ve used window.location.href in the past, but didn’t realise how simple it is to access sections of the URL!
If you want to see a live-action of what James is talking about, check out the table of content at the top of this article. Click on it and it will scroll down to the specific section of the page.
# Resources
- Share to Twitter Twitter
- Share to Facebook Facebook
- Share to LinkedIn LinkedIn
- Share to Reddit Reddit
- Share to Hacker News Hacker News
- Email Email
Related Tidbits
Console.table to display data, colorful console message, window.location cheatsheet, fresh tidbits.
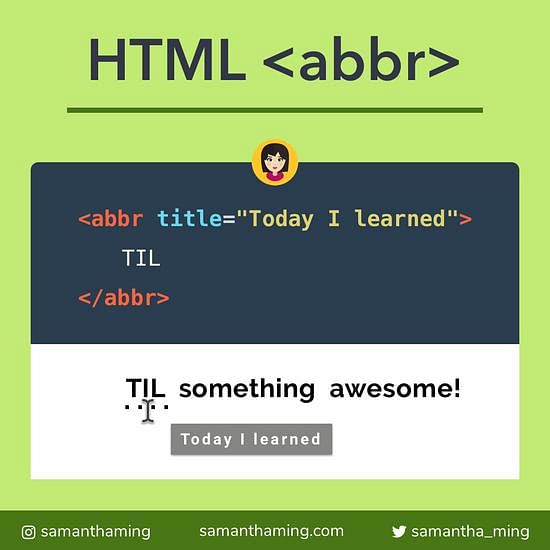
HTML abbr Tag
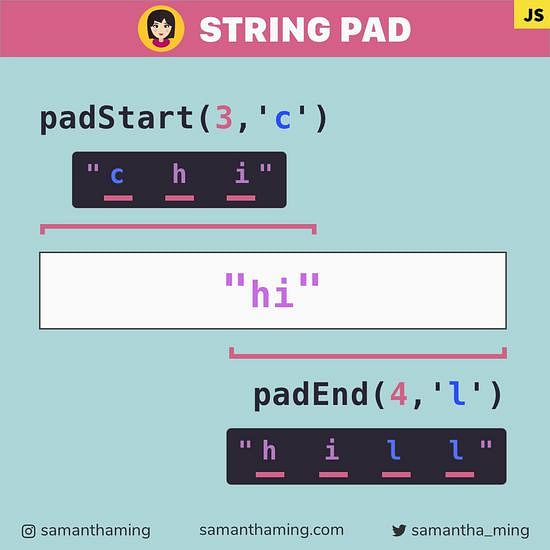
How to Pad a String with padStart and padEnd in JavaScript
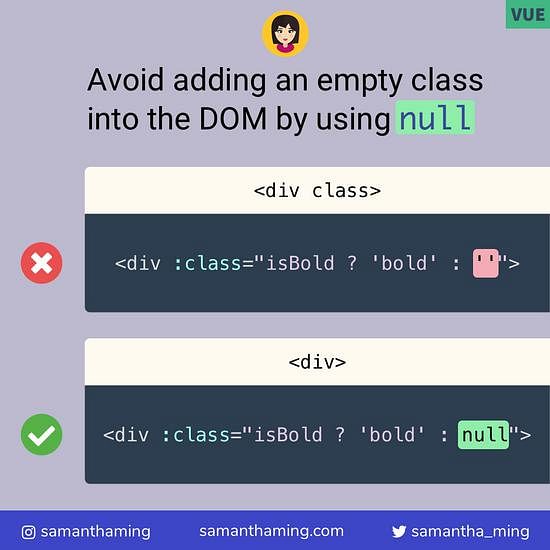
Avoid Empty Class in Vue with Null
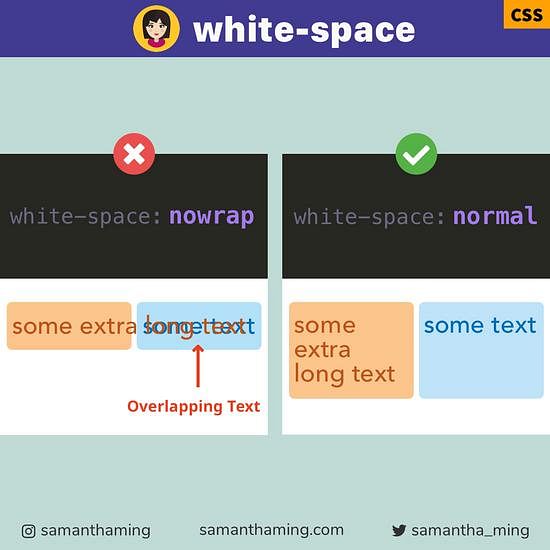
Fix Text Overlap with CSS white-space
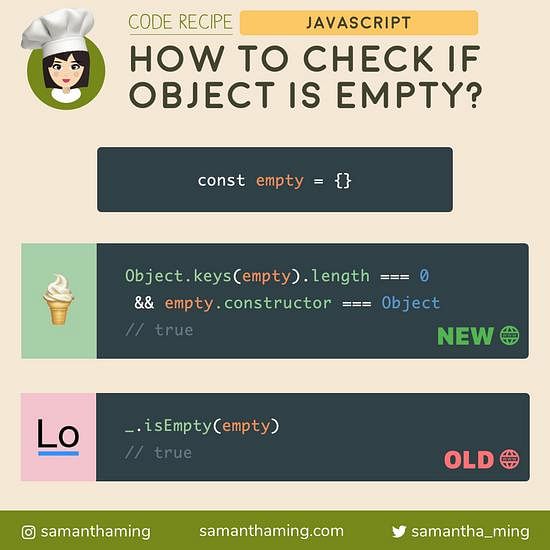
How to Check if Object is Empty in JavaScript
JS Reference
Html events, html objects, other references, window location, the window location object.
The location object contains information about the current URL.
The location object is a property of the window object .
The location object is accessed with:
window.location or just location
Location Object Properties
Location object methods.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript | window.location and document.location Objects
- How to set location and location.href using JavaScript ?
- JavaScript location.host vs location.hostname
- JavaScript BOM Location object
- Window Object in JavaScript
- Difference between window.location.href, window.location.replace and window.location.assign in JavaScript
- What is Window Object in JavaScript ?
- How to print the content of current window using JavaScript ?
- Javascript Window Open() & Window Close() Method
- HTML DOM Window.location Property
- How to open URL in a new window using JavaScript ?
- How to calculate Width and Height of the Window using JavaScript ?
- How to Perform CRUD Operations on JavaScript Object ?
- Global and Local variables in JavaScript
- How to open URL in same window and same tab using JavaScript ?
- Display the number of links present in a document using JavaScript
- How are the JavaScript window and JavaScript document different from one another?
- How to close current tab in a browser window using JavaScript?
- How to check a JavaScript Object is a DOM Object ?
- How to change your location in Chrome in Windows?
window.location and document.location: These objects are used for getting the URL (the current or present page address) and avert browser to a new page or window. The main difference between both is their compatibility with the browsers.
- The window.location is read/write on all compliant browsers.
- The document.location is read-only in Internet Explorer but read/write in Firefox, SeaMonkey that are Gecko-based browsers.
All modern browsers map document.location to the window.location but you can prefer window.location for cross-browser safety.
- window.location.href: It returns the URL of the current working page.
- window.location.hostname: It returns the domain name of web host.
- window.location.pathname: It returns the path and filename of the current working page.
- window.location.protocol: It returns the used protocol (http: or https:).
- window.location.port(): It prints the port number.
- window.location.host(): It prints host name along with port number.
- window.location.assign(): It loads new document.
Example 1: This example using different properties to get different parts of URL.
Note: When you visit a specific website, it is always connected to a port. However, most browsers will not display the default port number.
Example 2: Assign or load new document.
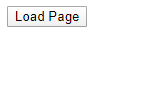
Please Login to comment...
Similar reads.
- javascript-object
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- Remember me Not recommended on shared computers
Forgot your password?
How to: window.location.href target="_blank" ?
By vmars316 November 8, 2020 in JavaScript
Recommended Posts

Trying to redirect from one page to another page
and still leave 1st page open .
This works except that it closes 1st page:
This doesn't work at all:
Link to comment
Share on other sites.

Use window.open() to open a URL in a new tab.

Thanks Ingolme;
Yes , this works perfectly , the 'href' allows me to keep current window open:
Thanks Again
Btw: I miss your foxy Avatar . I would like to have a copy of it possible ?

You can use it like this:
Thanks ishan_shah ;
I had tried this: window.open('https://www.duckduckgo.com' ; 'target= "_blank" ')
But couldn't find the proper format .
Turns out my code above doesn't work , but this works except for one issue:
1st time thru , it opens the clicked Link .
How can I prevent the '1st time thru Link from opening ?
Thanks for your Help...

I think you talking about preventDefault() https://www.w3schools.com/jsref/event_preventdefault.asp
Ah..... Finally;

- 7 months later...
You can redirect a web page via JavaScript using a number of methods. window.location.replace(...) is better than using window.location.href, because replace() does not keep the originating page in the session history, meaning the user won't get stuck in a never-ending back-button fiasco.
If you want to simulate someone clicking on a link, use location.href
If you want to simulate an HTTP redirect, use location.replace
JavaScript redirect example:
// similar behavior as an HTTP redirect
// similar behavior as clicking on a link
- 3 months later...

But you're then technically manipulating their browsing history. Privacy laws in some countries might not permit that.
- 2 years later...

Dhruv Lathia
Thanks @ishan_shah It worked on my code
Create an account or sign in to comment
You need to be a member in order to leave a comment
Create an account
Sign up for a new account in our community. It's easy!
Already have an account? Sign in here.
- Existing user? Sign In
- W3Schools.com
- Online Users
- Leaderboard
- All Activity
- Create New...
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Location: search property
The search property of the Location interface is a search string, also called a query string ; that is, a string containing a '?' followed by the parameters of the URL.
Modern browsers provide URLSearchParams and URL.searchParams to make it easy to parse out the parameters from the querystring.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
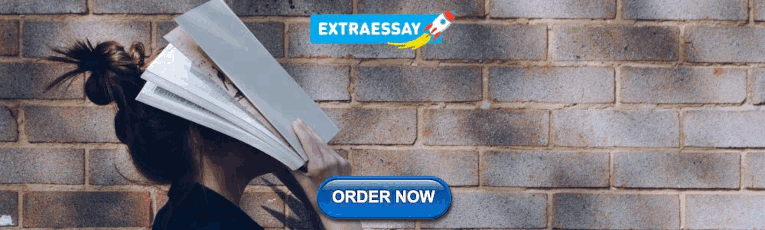
IMAGES
VIDEO
COMMENTS
The assign() method of the Location interface causes the window to load and display the document at the URL specified. After the navigation occurs, the user can navigate back to the page that called Location.assign() by pressing the "back" button.
See Also: The replace() Method. Note. The difference between assign() and replace(): replace() removes the current URL from the document history. With replace() it is not possible to use "back" to navigate back to the original document.
The window.location object can be written without the window prefix. Some examples: window.location.href returns the href (URL) of the current page. window.location.hostname returns the domain name of the web host. window.location.pathname returns the path and filename of the current page. window.location.protocol returns the web protocol used ...
I would avoid this solution because window.open in some cases could return null (but still open a new window as expected) while also triggering location.replace on the current tab resulting in two open tabs with the new url.
1. Using location.href. When we assign a URL to the window.location.href property, the associated document navigates to the new page from the same or different origin. Since window is a global object, window.location.href can be shortened to location.href. Also location is a synonym of location.href, we can directly assign a value to the ...
Location.ancestorOrigins Read only . A static DOMStringList containing, in reverse order, the origins of all ancestor browsing contexts of the document associated with the given Location object.. Location.href. A stringifier that returns a string containing the entire URL. If changed, the associated document navigates to the new page. It can be set from a different origin than the associated ...
The location property of a window (i.e. window.location) is a reference to a Location object; it represents the current URL of the document being displayed in that window. Since window object is at the top of the scope chain, so properties of the window.location object can be accessed without window. prefix, for example window.location.href can ...
2. Redirection using replace () function. Another way to redirect to a web page in jQuery is by using the replace () function of the window.location object. This method removes the current URL from the history and replaces it with a new URL. Here is an example of how to use this function for redirection:
# window.location vs location. All 4 of these properties point at the same Location object. I personally prefer window.location and would actually avoid using location. Mainly because location reads more like a generic term and someone might accidentally name their variable that, which would override the global variable. Take for example:
window.location.assign Property:. The assign function is similar to the href property as it is also used to navigate to a new URL.; The assign method, however, does not show the current location, it is only used to go to a new location. Unlike the replace method, the assign method adds a new record to history (so that when the user clicks the "Back" button, he/she can return to the current ...
So Use the assign () method if you want to load a new document, andwant to give the option to navigate back to the original document. You can change the location object href property using jQuery also like this. $(location).attr('href',url); And hence you can redirect the user to some other url.
If you load the page and click on the button, you will navigate to the webpage you assigned to the property. If you need to check out all of the properties on the location object, here is a link from the MDN docs. Similar methods to assign include:. window.location.reload() - reloads the current URL (like the refresh button). window.location.replace() - redirects to the provided URL.
Learn jQuery Tutorial Reference Learn Vue ... assign() constructor create() defineProperties() ... The Window Location Object. The location object contains information about the current URL. The location object is a property of the window object.
Last Updated : 13 May, 2020. window.location and document.location: These objects are used for getting the URL (the current or present page address) and avert browser to a new page or window. The main difference between both is their compatibility with the browsers. The window.location is read/write on all compliant browsers.
Pull out all their values, dynamically build a form with all of the fields in all three forms, and submit that form. The form can be invisible. Don't use a JQuery AJAX method, use $("#myForm").submit().The form- which will be invisible, and only used to submit values from your client side code, not user input- will never be shown nor otherwise used, and the page will be refreshed.
Location: replace () method. The replace() method of the Location interface replaces the current resource with the one at the provided URL. The difference from the assign() method is that after using replace() the current page will not be saved in session History , meaning the user won't be able to use the back button to navigate to it.
Learn why window.location.assign("link") may not work in some cases and how to fix it with the help of experts on Stack Overflow, the largest online community for programmers.
Create an account or sign in to comment. You need to be a member in order to leave a comment
Location: search property. The search property of the Location interface is a search string, also called a query string; that is, a string containing a '?' followed by the parameters of the URL. Modern browsers provide URLSearchParams and URL.searchParams to make it easy to parse out the parameters from the querystring.
jquery window.location.assign() not working. Ask Question Asked 6 years, 8 months ago. Modified 6 years, 8 months ago. Viewed 1k times -2 I'm sending form data via jquery and cannot get the window.location.assign() to fire off. I am getting a successful submission. I've also tried window.location.href =