- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Comma operator should be used carefully
- Representation of Int Variable in Memory in C++
- Function Overriding in C++
- is_void template in C++
- C++ Installation on MacBook M1 for VS Code
- Trigraphs in C++ with Examples
- Overflow of Values While Typecasting in C ++
- Advantages and Disadvantages of C++
- "Access is Denied error" in C++ Visual Studio and How to resolve it
- History of C++
- Application of OOPs in C++
- Trivial classes in C++
- Scope resolution operator in C++
- Strict Type Checking in C++
- OpenCV C++ Windows Setup using Visual Studio 2019
- Is assignment operator inherited?
- Comma in C++
- Nested Classes in C++
- Introduction to Parallel Programming with OpenMP in C++
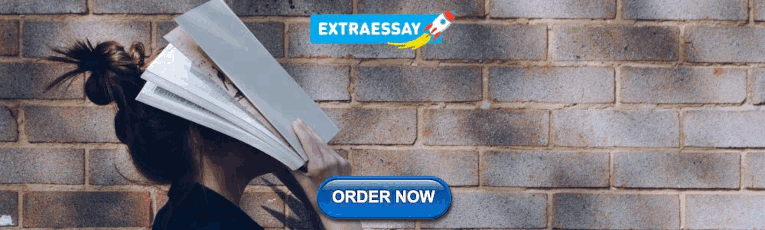
Copy Constructor vs Assignment Operator in C++
Copy constructor and Assignment operator are similar as they are both used to initialize one object using another object. But, there are some basic differences between them:
Consider the following C++ program.
Explanation: Here, t2 = t1; calls the assignment operator , same as t2.operator=(t1); and Test t3 = t1; calls the copy constructor , same as Test t3(t1);
Must Read: When is a Copy Constructor Called in C++?
Please Login to comment...
Similar reads.
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 15:13.
- This page has been accessed 1,333,785 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- Graphics and multimedia
- Language Features
- Unix/Linux programming
- Source Code
- Standard Library
- Tips and Tricks
- Tools and Libraries
- Windows API
- Copy constructors, assignment operators,
Copy constructors, assignment operators, and exception safe assignment


14.14 — Introduction to the copy constructor
Consider the following program:
You might be surprised to find that this program compiles just fine, and produces the result:
Let’s take a closer look at how this program works.
The initialization of variable f is just a standard brace initialization that calls the Fraction(int, int) constructor.
But what about the next line? The initialization of variable fCopy is also clearly an initialization, and you know that constructor functions are used to initialize classes. So what constructor is this line calling?
The answer is: the copy constructor.
The copy constructor
A copy constructor is a constructor that is used to initialize an object with an existing object of the same type. After the copy constructor executes, the newly created object should be a copy of the object passed in as the initializer.
An implicit copy constructor
If you do not provide a copy constructor for your classes, C++ will create a public implicit copy constructor for you. In the above example, the statement Fraction fCopy { f }; is invoking the implicit copy constructor to initialize fCopy with f .
By default, the implicit copy constructor will do memberwise initialization. This means each member will be initialized using the corresponding member of the class passed in as the initializer. In the example above, fCopy.m_numerator is initialized using f.m_numerator (which has value 5 ), and fCopy.m_denominator is initialized using f.m_denominator (which has value 3 ).
After the copy constructor has executed, the members of f and fCopy have the same values, so fCopy is a copy of f . Thus calling print() on either has the same result.
Defining your own copy constructor
We can also explicitly define our own copy constructor. In this lesson, we’ll make our copy constructor print a message, so we can show you that it is indeed executing when copies are made.
The copy constructor looks just like you’d expect it to:
When this program is run, you get:
The copy constructor we defined above is functionally equivalent to the one we’d get by default, except we’ve added an output statement to prove the copy constructor is actually being called. This copy constructor is invoked when fCopy is initialized with f .
Access controls work on a per-class basis (not a per-object basis). This means the member functions of a class can access the private members of any class object of the same type (not just the implicit object).
We use that to our advantage in the Fraction copy constructor above in order to directly access the private members of the fraction parameter. Otherwise, we would have no way to access those members directly (without adding access functions, which we might not want to do).
A copy constructor should not do anything other than copy an object. This is because the compiler may optimize the copy constructor out in certain cases. If you are relying on the copy constructor for some behavior other than just copying, that behavior may or may not occur. We discuss this further in lesson 14.15 -- Class initialization and copy elision .
Best practice
Copy constructors should have no side effects beyond copying.
Prefer the implicit copy constructor
Unlike the implicit default constructor, which does nothing (and thus is rarely what we want), the memberwise initialization performed by the implicit copy constructor is usually exactly what we want. Therefore, in most cases, using the implicit copy constructor is perfectly fine.
Prefer the implicit copy constructor, unless you have a specific reason to create your own.
We’ll see cases where the copy constructor needs to be overwritten when we discuss dynamic memory allocation ( 21.13 -- Shallow vs. deep copying ).
The copy constructor’s parameter must be a reference
It is a requirement that the parameter of a copy constructor be an lvalue reference or const lvalue reference. Because the copy constructor should not be modifying the parameter, using a const lvalue reference is preferred.
If you write your own copy constructor, the parameter should be a const lvalue reference.
Pass by value (and return by value) and the copy constructor
When an object is passed by value, the argument is copied into the parameter. When the argument and parameter are the same class type, the copy is made by implicitly invoking the copy constructor. Similarly, when an object is returned back to the caller by value, the copy constructor is implicitly invoked to make the copy.
We see both happen in the following example:
In the above example, the call to printFraction(f) is passing f by value. The copy constructor is invoked to copy f from main into the f parameter of function printFraction .
When generateFraction returns a Fraction back to main , the copy constructor is implicitly called again. And when f2 is passed to printFraction , the copy constructor is called a third time.
On the author’s machine, this example prints:
If you compile and execute the above example, you may find that only two calls to the copy constructor occur. This is a compiler optimization known as copy elision . We discuss copy elision further in lesson 14.15 -- Class initialization and copy elision .
Using = default to generate a default copy constructor
If a class has no copy constructor, the compiler will implicitly generate one for us. If we prefer, we can explicitly request the compiler create a default copy constructor for us using the = default syntax:
Using = delete to prevent copies
Occasionally we run into cases where we do not want objects of a certain class to be copyable. We can prevent this by marking the copy constructor function as deleted, using the = delete syntax:
In the example, when the compiler goes to find a constructor to initialize fCopy with f , it will see that the copy constructor has been deleted. This will cause it to emit a compile error.
As an aside…
You can also prevent the public from making copies of class object by making the copy constructor private (as private functions can’t be called by the public). However, a private copy constructor can still be called from other members of the class, so this solution is not advised unless that is desired.
For advanced readers
The rule of three is a well known C++ principle that states that if a class requires a user-defined copy constructor, destructor, or copy assignment operator, then it probably requires all three. In C++11, this was expanded to the rule of five , which adds the move constructor and move assignment operator to the list.
Not following the rule of three/rule of five is likely to lead to malfunctioning code. We’ll revisit the rule of three and rule of five when we cover dynamic memory allocation.
We discuss destructors in lesson 15.4 -- Introduction to destructors and 19.3 -- Destructors , and copy assignment in lesson 21.12 -- Overloading the assignment operator .
Question #1
In the lesson above, we noted that the parameter for a copy constructor must be a (const) reference. Why aren’t we allowed to use pass by value?
Show Solution
When we pass a class type argument by value, the copy constructor is implicitly invoked to copy the argument into the parameter.
If the copy constructor used pass by value, the copy constructor would need to call itself to copy the initializer argument into the copy constructor parameter. But that call to the copy constructor would also be pass by value, so the copy constructor would be invoked again to copy the argument into the function parameter. This would lead to an infinite chain of calls to the copy constructor.
Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, c++ introduction.
- C++ Variables and Literals
- C++ Data Types
- C++ Basic I/O
- C++ Type Conversion
- C++ Operators
- C++ Comments
C++ Flow Control
- C++ if...else
- C++ for Loop
- C++ do...while Loop
- C++ continue
- C++ switch Statement
- C++ goto Statement
- C++ Functions
- C++ Function Types
- C++ Function Overloading
- C++ Default Argument
- C++ Storage Class
- C++ Recursion
- C++ Return Reference
C++ Arrays & String
- Multidimensional Arrays
- C++ Function and Array
- C++ Structures
- Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
C++ Object & Class
- C++ Objects and Class
C++ Constructors
- C++ Objects & Function
- C++ Operator Overloading
- C++ Pointers
- C++ Pointers and Arrays
- C++ Pointers and Functions
- C++ Memory Management
- C++ Inheritance
- Inheritance Access Control
- C++ Function Overriding
- Inheritance Types
- C++ Friend Function
- C++ Virtual Function
- C++ Templates
C++ Tutorials
C++ Constructor Overloading
- C++ clock()
C++ Classes and Objects
How to pass and return object from C++ Functions?
C++ Class Templates
- C++ Encapsulation
A constructor is a special type of member function that is called automatically when an object is created.
In C++, a constructor has the same name as that of the class , and it does not have a return type. For example,
Here, the function Wall() is a constructor of the class Wall . Notice that the constructor
- has the same name as the class,
- does not have a return type, and
- C++ Default Constructor
A constructor with no parameters is known as a default constructor . For example,
Here, when the wall1 object is created, the Wall() constructor is called. This sets the length variable of the object to 5.5 .
Note: If we have not defined a constructor in our class, then the C++ compiler will automatically create a default constructor with an empty code and no parameters.
C++ Parameterized Constructor
In C++, a constructor with parameters is known as a parameterized constructor. This is the preferred method to initialize member data. For example,
Here, we have created a parameterized constructor Wall() that has two parameters: double len and double hgt . The values contained in these parameters are used to initialize the member variables length and height .
When we create an object of the Wall class, we pass the values for the member variables as arguments. The code for this is:
With the member variables thus initialized, we can now calculate the area of the wall with the calculateArea() function.
- C++ Copy Constructor
The copy constructor in C++ is used to copy data from one object to another. For example,
In this program, we have used a copy constructor to copy the contents of one object of the Wall class to another. The code of the copy constructor is:
Notice that the parameter of this constructor has the address of an object of the Wall class.
We then assign the values of the variables of the obj object to the corresponding variables of the object, calling the copy constructor. This is how the contents of the object are copied.
In main() , we then create two objects wall1 and wall2 and then copy the contents of wall1 to wall2 :
Here, the wall2 object calls its copy constructor by passing the address of the wall1 object as its argument, i.e., &obj = &wall1 .
Note : A constructor is primarily used to initialize objects. They are also used to run a default code when an object is created.
- C++ Friend Function and Classes
Table of Contents
- Introduction
- Parameterized Constructor C++
Sorry about that.
Related Tutorials
C++ Tutorial
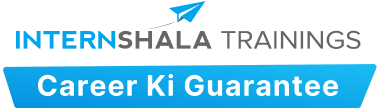
Your favourite senior outside college
Home » Programming » Constructor in C++
Copy Constructor in C++: Syntax, Types and Examples
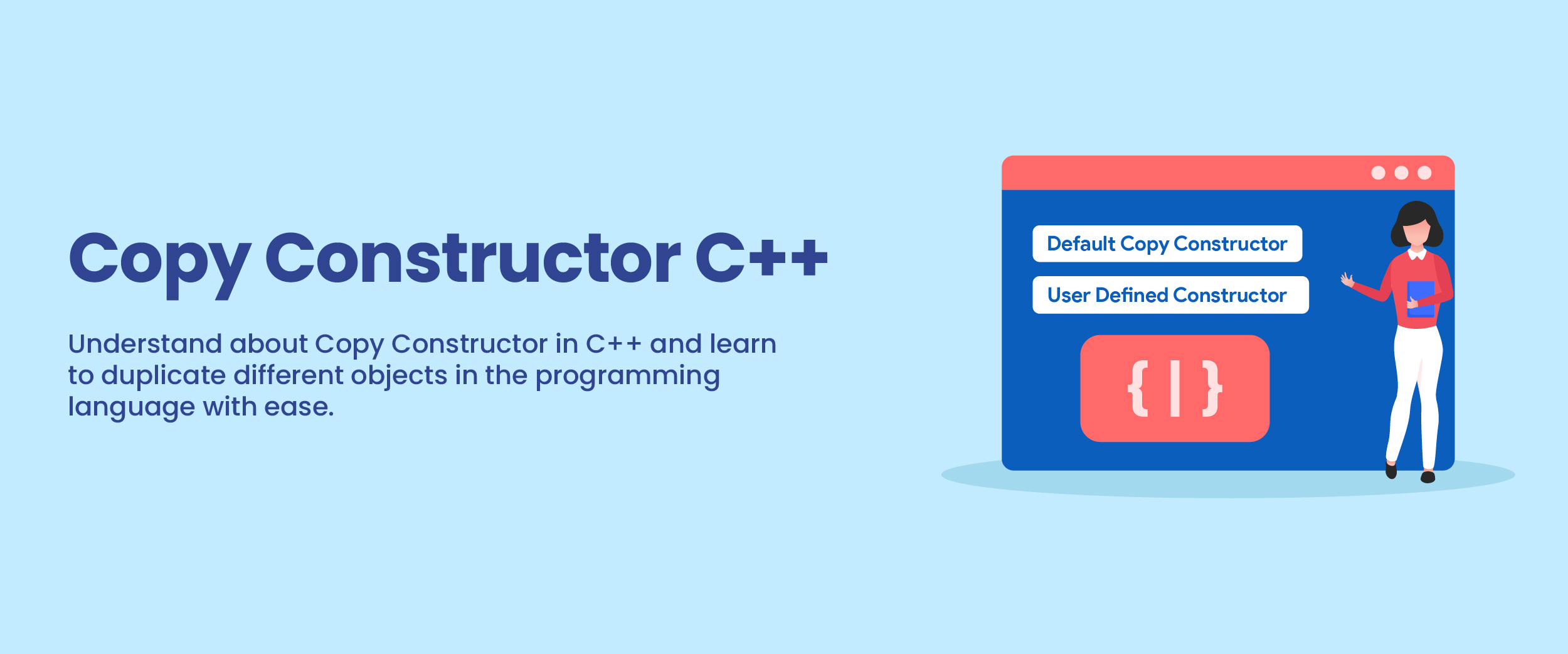
Are you curious to know about copy constructors in C++ programming language? They are a great way to optimize and streamline the duplication of objects. But do you know how they work, what their types are, or why they are so important? In this blog post, we’ll cover all aspects of these powerful features. So, let’s begin understanding what copy constructors do and how to successfully implement them into your codebase.
Table of Contents
Introduction to Constructors
Constructors in C++ are methods invoked automatically at the time of object creation to initialize the data members of the new objects. It has the same name as the class and is called a constructor because it constructs or provides the values for the object. A restriction that applies to a constructor is that it must not have a return type or void.
The following is the syntax for defining a constructor:
You should declare a constructor in the public section of the class, as it is invoked automatically when objects are created. Further, there are three types of constructors in C++: default, parameterized, and copy constructors.
In a default constructor, no argument is passed, and it is known as a constructor with no parameters. Parameterized constructors allow one or more arguments to pass. Copy constructors create an object based on the previously created object of the same class.
Constructors and similar C++ concepts are part of the core proficiencies required in data science, web development, and related domains. If you wish to pursue a career in these fields, check out trending job oriented courses with internship or job placement offers.
Copy Constructor Syntax in C++
The syntax of copy structure in C++ is “ClassName(const ClassName &objectToCopy)”, where “const” indicates no modification to the original object when copying will be made. For example, you have two strings, ‘stringA’ and ‘stringB’. If you wanted ‘stringB’ to take on all properties associated with ‘string A’, without modifying ‘stringA’, then using a copy constructor would do just that.
In this case, “obj2” is created as the new object, which will take on the same values as what was previously seen in “obj1”. To learn more about copy constructor and its syntax, consider opting for an in-depth C++ course .
Example of Copy Constructor in C++
The copy constructor of the Rectangle class is used to create a duplicate object of an existing one by copying its contents. The code for this operation is as follows:
The constructor of this object takes a parameter that is a reference to another Rectangle class instance. This means the values stored in obj’s variables are then assigned to the new object, invoking the copy constructor and effectively copying all data from one object into another. In main(), two rectangle objects, rect1 and rect2 have been created and an example has been given where the contents of rect1 were copied over onto rect2 using this method.
In this instance, the rect2 object invokes its copy constructor by passing a reference to the rect1 object as an argument – namely &obj = &rect1. This is done for constructors to fulfill their purpose of initializing objects and executing default code when they are created.
Also Read: Array in C
Characteristics of Copy Constructor
The following are the key characteristics of a Copy Constructor:
- It is used to initialize a new object’s members by duplicating the members of an existing object. It accepts a reference to an object of the same class as a parameter.
- Copy initialization involves using the copy constructor, which carries out member-wise initialization; copying each individual member respectively between objects of identical classes.
- If not specified explicitly, this process will be handled automatically by compiler-generated code for creating such constructors within programs. These programs can be written in C++ language or similar languages that support Object Oriented Programming (OOP).
- The copy constructor is useful for creating copies of existing objects without having to redefine the object’s entire data structure and attributes again.
- It also allows a programmer to provide custom modifications while copying an object, such as altering members according to certain criteria or making other changes. These changes can be more difficult in regular ‘assignment’ type operations between two identical classes of objects

Types of Copy Constructors in C++
A copy constructor program in C++ program is categorized into the following two types.
1. Default Copy Constructor
A default copy constructor is an implicitly defined one that copies the bases and members of an object like how they would be initialized by a constructor. The order in which these elements are copied during this process follows the same logic of initialization used when creating new objects with constructors.
2. User-Defined Copy Constructor
A user-defined copy constructor is necessary when an object holds pointers or references that cannot be shared, such as a file. In these cases, it’s recommended to also create a destructor and assignment operator in addition to the copy constructor.
A user-defined copy constructor may be necessary when an object contains pointers or any kind of runtime resource allocation, such as file handles or network connections. With a custom copy constructor, it is possible to achieve deep copies instead of the shallow ones created by default constructors. The user can ensure that the pointers in copied objects are directed toward new memory locations with this method.
When is the Copy Constructor in C++ Used?
A copy constructor is called when an object of the same class is:
- Returned by value
- Passed as a parameter to a function
- Created based on another object of the same class
- When a temporary object has been generated by the compiler
Though a copy constructor may be called in some instances, there is no guarantee that it will always be used. The C++ standard allows for the compiler to eliminate redundant copies under specific conditions such as return value optimization (commonly referred to as RVO).
Difference Between Copy Constructor and Assignment Operator
Differences between initialization and assignment in c++.
In the following code segment:
The variable “b” is initialized to “a” since it is created as an exact copy of another variable. This implies that when we create “b”, its value will go from containing unwanted data directly to holding the same value immediately without any intermediate step.
On the other hand, in this revised code example:
The statement “MYClass a, b;” initializes two variables – ‘a’ and ‘b’. The second line of code “b = a” assigns the value contained in variable ‘a’ to another (variable) object ‘b’. This demonstrates the difference between assignment and initialization. Assigning new values or instructions to an existing variable is known as an assignment. On the other hand, when objects receive values at their time of creation, it is referred to as initialization.
Copy constructors in C++ are an essential tool for effective software programming. Not only do they allow for objects to be efficiently duplicated within code, but they also provide better resource and memory management when dealing with pointers or other runtime resources. Programmers must understand the differences between initialization and assignment and master using these copy constructors to create great applications.
Are you a programmer looking for jobs in this domain? Check out this guide on C++ interview questions with proper coding examples to help you crack your next interview with ease.
In C++, the default constructor has an empty body and does not assign default values to data members. The copy constructor is not empty and copies all data members of the passed object to the newly created object.
When the copy constructor is declared private, you cannot copy the class’s objects. It is useful when the class has pointer variables or dynamically allocated resources.
Yes, we can make the copy constructor private. In this case, the constructor can only be called from within the class itself. These constructors are made private when the class is not intended to be used as a standalone object but as a base class for inheritance.
Arguments to a copy constructor must be passed as a reference to avoid unnecessary copying of objects. If an argument is passed by value, the copy constructor is called indefinitely to make a copy of the argument. This results in an infinite loop.
The argument in a copy constructor should be const because const reference enables you to read the value but not modify it within the function. This ensures that the objects are not accidentally modified. Const argument is essential while working with temporary objects.
Copy constructors are required in C++ to initialize the data members of a newly created object in a class by copying the data members of an existing object of the same class.
- ← Previous
- Next →
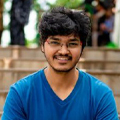
Parinay is an industry expert with over six years of professional experience. He is known for his excellence in Full Stack Development and Web Application Security. The Director of the Tech Team in his college days, Parinay has created websites that have won prestigious awards at national and international events.
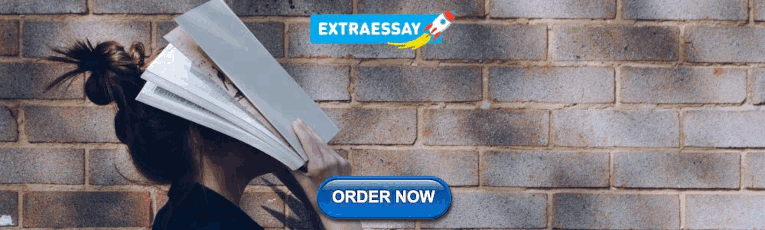
Related Post
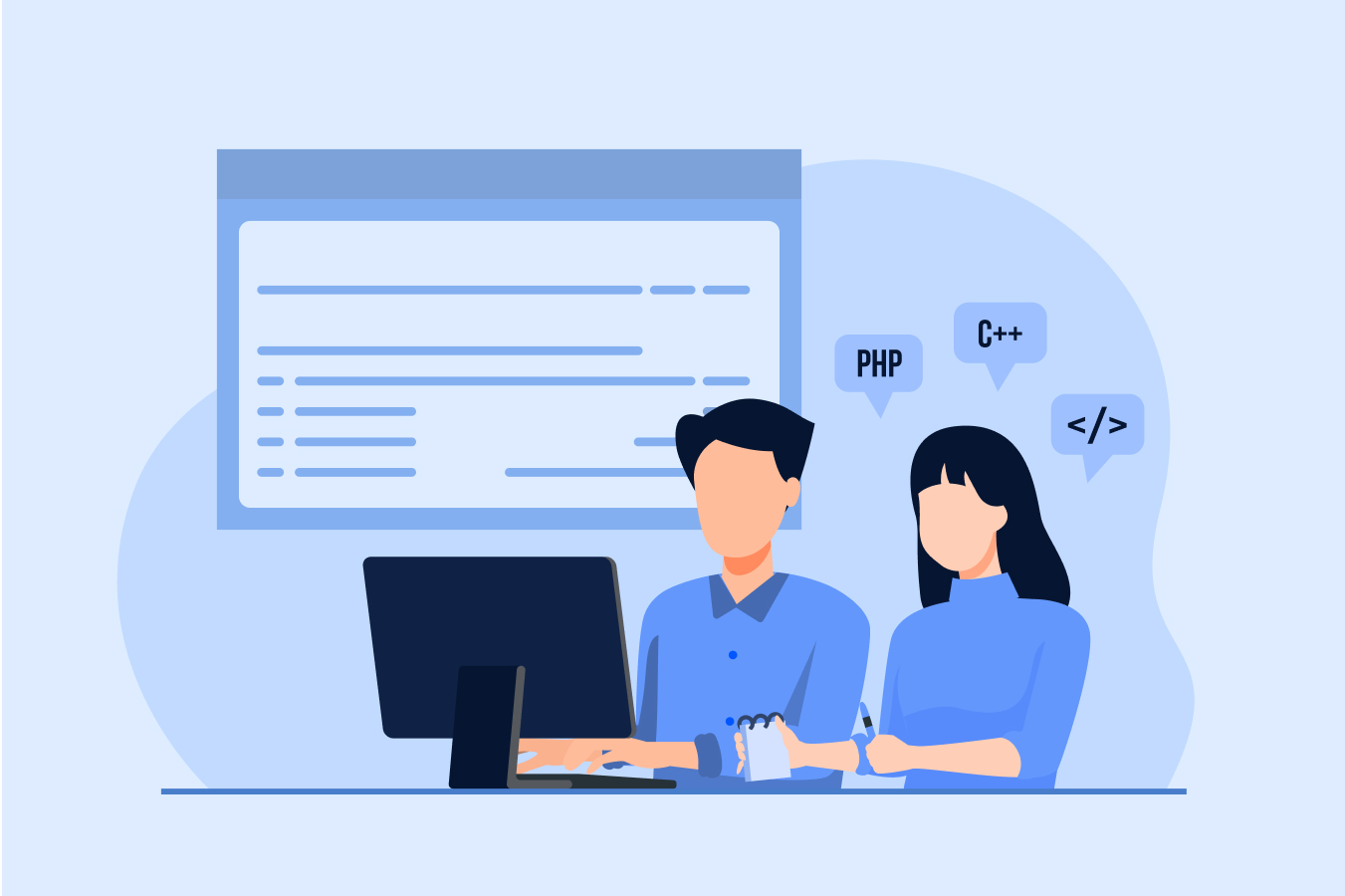
How to Become a Web Developer?: A Step-By-Step Guide
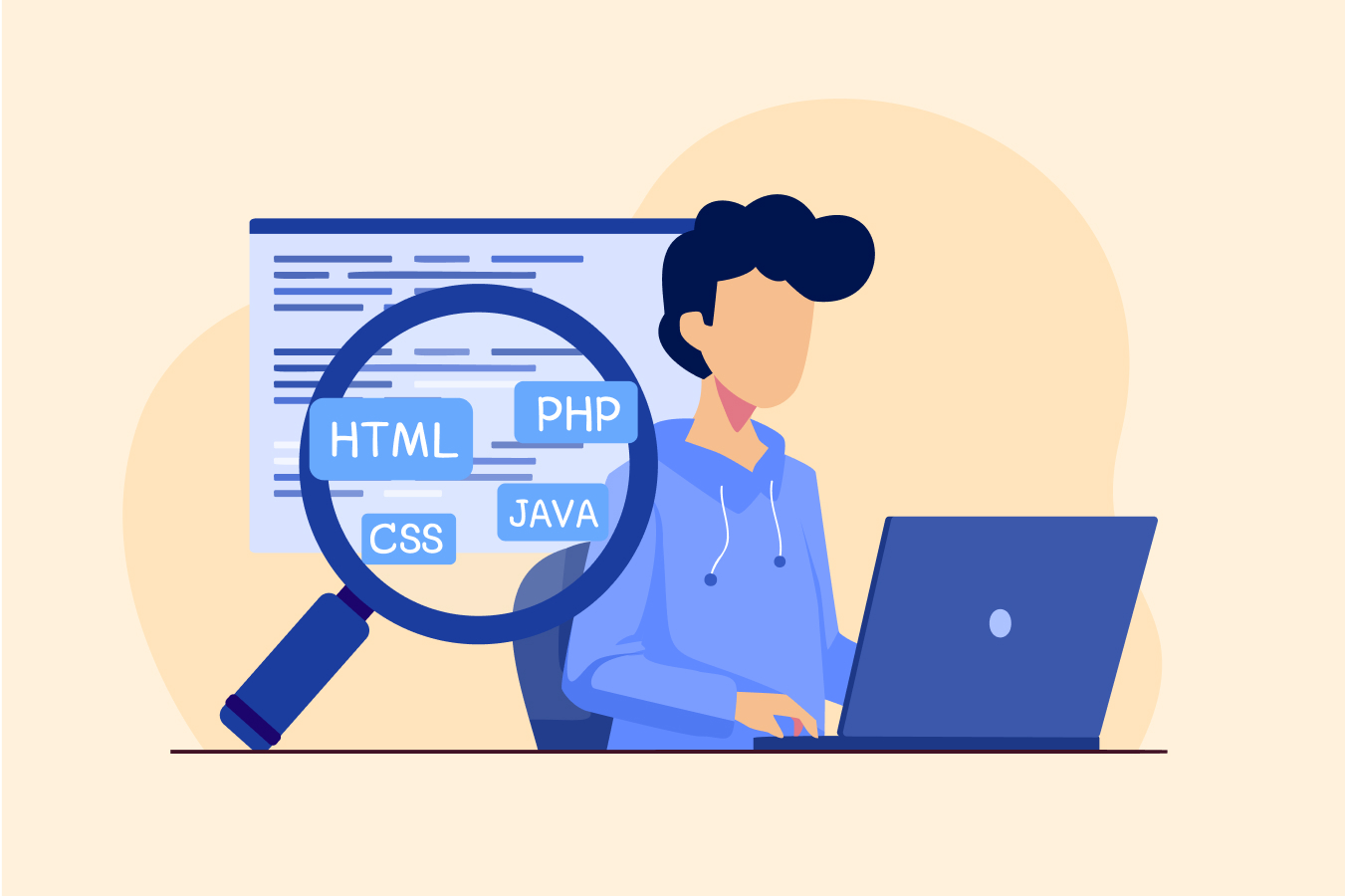
What is Full Stack Web Development?: 2024 Guide
How to become a full stack developer: a complete guide.
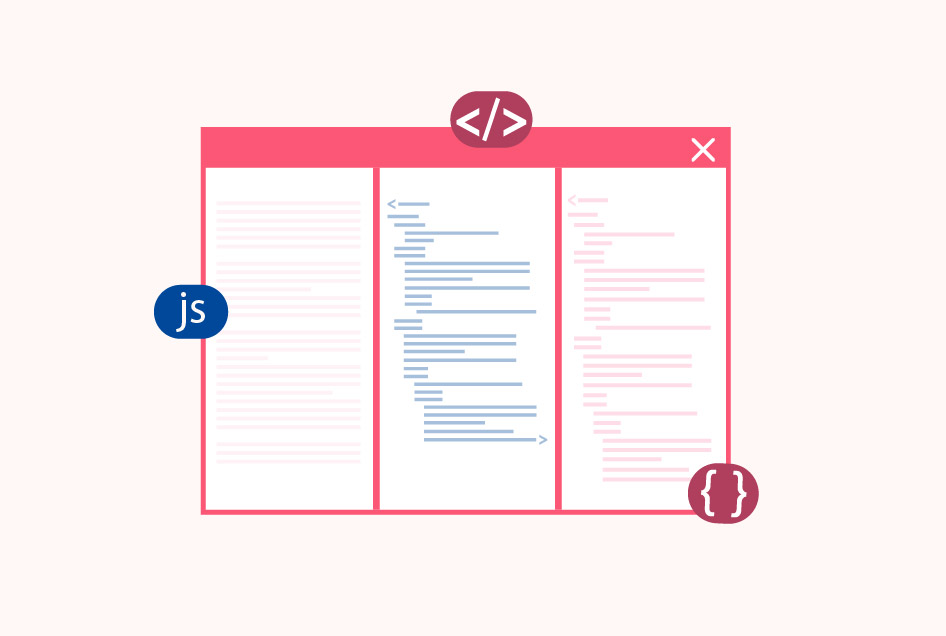
Java Cheat Sheet – A Comprehensive Guide for Developers

Download the Unstop app now!
Check out the latest opportunities just for you!
Copy Constructor In C++ | Types, Uses & More With Examples!
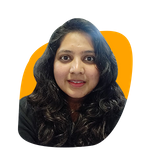
Table of content:
What is a copy constructor in c++, characteristics of copy constructors in c++, types of copy constructors in c++, when is the copy constructor in c++ called, when is a user-defined copy constructor needed, types of constructor copies in c++, can we make the copy constructor in c++ private.
- Copy Constructor Vs Assignment Operator
Example Of Class Where A Copy Constructor Is Essential
A constructor in C++ is a unique member function that is called whenever an object of a class is created. They are in charge of initializing the object's attributes and getting it ready for use. We can use these normal constructors to set default values, allocate separate memory storage, and carry out any other setup procedures. In this article, we will examine one particular constructor, that is, the copy constructor in C++ programming .
We will look at its syntax, concepts about constructor types, and more. The copy constructor is crucial when dealing with the copying of objects because it ensures that copies are made correctly without unintended side effects.
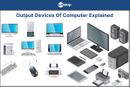
Imagine you have set up a magical workshop to create unique items. Every object has unique qualities, such as a name and a magic power level. Now, imagine that you wish to make an item exactly like an already-existing object. How would you do it? Well, in programming, the copy constructor in C++ provides the perfect way to get the job done. It's like making a magical copy of anything that has the same characteristics and abilities as that of the original one.
A copy constructor in C++ files is a unique type of constructor that makes a copy of an existing object. It resembles casting a spell to copy one object's characteristics into another one. Copy constructors play a crucial role in ensuring that the copy is made correctly and that the new object is an independent instance with its own memory space.
Syntax of Copy Constructor In C++
class ClassName { public: ClassName(const ClassName &obj) { // Copy attributes from obj to the current object } };
- ClassName: This is the name of the class.
- const ClassName &obj: This is a reference to an object of the same class type.
Here is an example of a simple copy constructor in a C++ program .
Example Of Copy Constructor In C++
Person 1: Alice, 25 years Person 2: Alice, 25 years
Explanation:
The code includes the necessary header to use input/output stream functionalities.
We then define a class named Person . Inside this class-
- There are two public member variables, name of type string and age of int type , which store the person's name and age.
- It also has a parameterized constructor, which initializes the variable name and age using the constructor's parameters.
- We also define a copy constructor , which will copy the name and age attributed from one object of the Person class to another.
Then, inside the main() function -
- We create an instance of the Person class , called person1 , where variables name and age are initialized with the values Alice and age 25, using the parameterized constructor .
- Next, we create another object called person2 , which is the copy of person1 using the copy constructor .
Finally, we use the cout commands to print the attributes, name, and age of both person1 and person2.
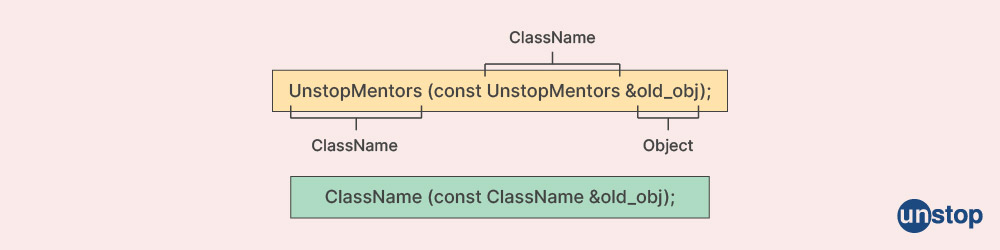
In C++, a copy constructor is a special constructor that is used to create a new object as a copy of an existing object. It's invoked when an object is being initialized with another object of the same class type. Copy constructors play a crucial role in ensuring proper copying of data members and resources between objects. Here are the key characteristics of copy constructors in C++:
Name and Signature: The copy constructor has the same name as the class and takes a single argument of the same class type (by reference or by value). Its primary purpose is to create a new object by copying the contents of another object.
Usage: Copy constructors are invoked in several scenarios, including when an object is being passed by value as a function argument, when an object is being returned by value from a function , and when an object is being explicitly or implicitly copied.
Default Copy Constructor: If you do not provide a copy constructor in your class, the compiler generates a default copy constructor for you. The default copy constructor performs a shallow copy. That is, this compiler-created copy constructor copies the values of the data members without considering any dynamically allocated resources.
Custom Copy Constructor: If your class involves dynamically allocated memory, file handles, or other resources, you should define a custom copy constructor to ensure proper copying and management of these resources. A custom copy constructor should allocate new resources and copy the contents of the source object appropriately.
Reference Parameter: To avoid unnecessary copying and improve performance, the copy constructor usually takes its argument by reference (const reference if the source object is not being modified). This way, the constructor works with the actual object, and no additional copy is made during the invocation.
Deep vs. Shallow Copy: The copy constructor is responsible for deciding whether to perform a shallow copy or a deep copy. A shallow copy simply copies the values of data members, while a deep copy involves creating new copies of dynamically allocated resources.
Initialization Lists: Inside the copy constructor, you should use an initialization list to initialize the data members of the new object. This helps to avoid unnecessary default construction initializations and improve efficiency.
Const-Correctness: If you're copying from a const object, your copy constructor should also be able to accept the const reference type variables as its parameter to ensure const-correctness.
Explicitly Deleted Copy Constructor: You can explicitly delete the copy constructor by using the delete operator. This prevents instances of your class from being copied.
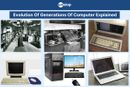
Just as artisans can craft replicas in various styles, copy constructors in C++ also come in different forms to suit specific copying needs. While the classic copy constructor performs a direct replica, some scenarios demand a more intricate approach. This is where the concept of types of copy constructors comes into play. From deep copies that meticulously clone every detail to the default copy constructor that works silently in the background, each type has its purpose and intricacies.
Default Copy Constructors In C++
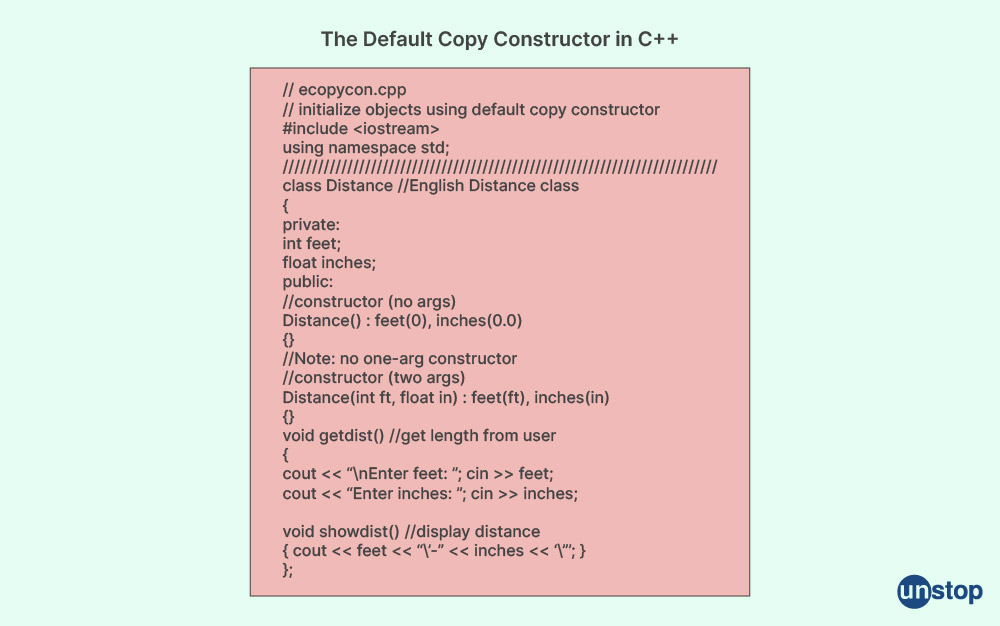
In C++, the default copy constructor is a built-in feature provided by the compiler. It's automatically generated when a class doesn't have a user-defined copy constructor. The default copy constructor performs a member-wise copy of the attributes from the source object to the newly created object. This process involves copying each attribute's value directly, which can lead to shallow copies if the attributes involve pointers or dynamically allocated memory .
Rules and points to note
- When is it Generated: The default copy constructor is generated by the compiler when a class doesn't have any user-defined copy constructor.
- Member-Wise Copy : The default copy constructor copies each attribute from the source object to the new object in a sequential manner.
- Shallow Copy: For attributes involving pointers or dynamically allocated memory, the default copy constructor performs a shallow copy. Meaning it copies the memory addresses, not the actual data. This can sometimes lead to multiple objects pointing to the same memory location, potentially causing unintended side effects.
- Automatic Initialization: You don't need to invoke the default copy constructor explicitly. It is automatically invoked when a new object is created using an existing object.
- Accessibility: The default copy constructor has the same accessibility as the class itself. If the class is defined as public, the copy constructor is accessible from outside the class.
- User-Defined Constructors : If a class has any user-defined constructors, the default copy constructor won't be generated. This emphasizes the need to explicitly define a copy constructor if specific copying behavior is required.
- Overriding: You can provide your own copy constructor by defining it explicitly in the class. This will override the default behavior.
- Default Copy Constructor Limitations: While suitable for simple classes with basic attributes, the default copy constructor may not be sufficient for classes with dynamic memory management or complex relationships between attributes.
Syntax of Default Copy Constructor In C++
The syntax of the default copy constructor is not explicitly written in the code, as it is automatically generated by the compiler when a user-defined copy constructor is not provided. However, here is a conceptual idea of how the default copy constructor works:
ClassName::ClassName(const ClassName &source) { // Perform member-wise copy of attributes from source object to this object }
- ClassName::ClassName : This is the constructor declaration, where ClassName is the name of the class, as well as the constructor.
- (const ClassName &source): This is the parameter list of the constructor, which takes a const reference of an object of the same class. The ampersand (&) is used to pass the object by reference, and the const keyword is used to prevent the object from being modified.
Please note that you don't typically write or see the default copy constructor in code. It is generated implicitly by the compiler when needed. If you want to provide specific copying behavior or handle dynamic memory, it's recommended to define your own copy constructor explicitly.
Code Example:
This example is in continuation of the first example above, which has a Person class with two private data members : name (of type string) and age (of type int).
- The class also has a constructor that initializes the data members, i.e., name with value n, and age with value a (i.e., Person(string n, int a).
Note that since there is no user-defined copy constructor within the class, the compiler generates a default copy constructor.
- We create an object of class Person, called person1 , with values Alice and 25, assigned to variables name and age, respectively.
- Then, we create another object, person2 , which copies person1, using the default copy constructor.
Next, we use the cout commands to print the attributes of both person1 and person2.
Finally, the program returns 0 to indicate successful program completion.
User-Defined Copy Constructors In C++
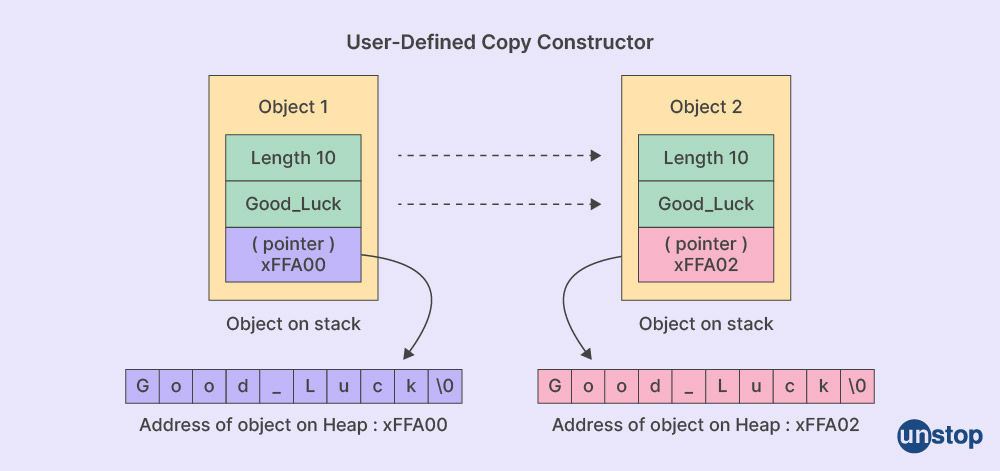
In order to conduct a particular type of copying when creating a new object from an existing one, you must declare a user-defined copy constructor within a class. This will give you greater control over how attributes and resources are replicated in contrast to the default copy constructor, which executes a member-wise shallow copy.
Rules for creating a user-defined copy constructor in C++
- Constructor Declaration: The user-defined copy constructor has the same name as the class, and its parameter is a reference to an object of the same class type, typically marked as const.
- Explicit Definition: You need to define the user-defined copy constructor within the class definition explicitly.
- Customized Copying: You have the freedom to decide how attributes and resources are copied from the source object to the new object. This is especially useful for classes involving dynamic memory or complex copying requirements.
- Deep Copying: User-defined copy constructors are often used to achieve deep copying, where dynamically allocated memory is duplicated to ensure independent instances.
- Shallow vs. Deep Copy: Depending on the scenario, you can implement either a shallow copy (copying memory addresses) or a deep copy (copying actual data) as needed.
class ClassName { public: // Attributes and other member functions // User-defined copy constructor ClassName(const ClassName &source) { // Copy attributes and resources from the source to this object } };
- The class keyword is used to define a class, while ClassName refers to the name of this class and its constructor.
- ClassName(const ClassName &source) is the signature of the user-defined copy constructor. It takes a constant reference to another object of the same class type as its parameter. The parameter name source is just a placeholder; you can use any valid name.
- And the curly brackets {} contain the body of the copy constructor.
Number 1: 42 Number 2: 42
In the example C++ program above-
- We have a constructor that initializes the variable value with n, i.e., Number(int n).
- There is also a user-defined copy constructor that takes a constant Number variable reference as a parameter, i.e., cont Number &other.
- This copies the value variable from the other object to the current object.
- In the main() function ,
- We create an object of the Number class called num1 and initialize it with the value 42 using the constructor.
- Then, we create another object called num2 , which copies object num1 using the user-defined copy constructor.
- Next, we print the values for both num1 and num2 using cout commands .
- Finally, the program returns 0 to indicate successful execution.
Check this out- Boosting Career Opportunities For Engineers Through E-School Competitions
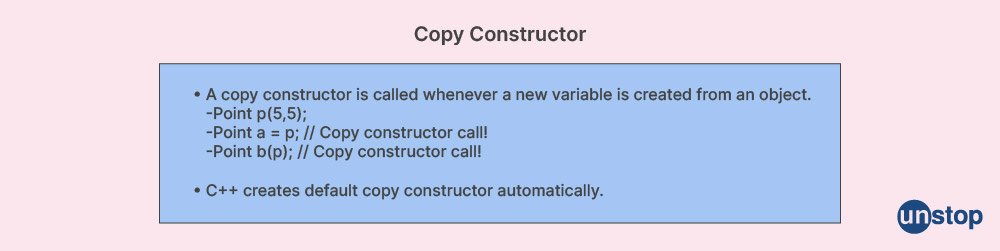
A copy constructor in C++ is typically used when you want to initialize a new object using the values of an existing object of the same class. They are particularly useful when you want to create a deep copy of an object to avoid issues with shared references. Copy constructors are used in the following situations:
When you want a copy of an existing object:
This happens when you initialize a new object using an existing object's data. This can occur during object declaration, passing objects by value, or returning objects by value from functions.
obj2.value: 10
In the code example, we define a class called MyClass with a public data member variable value of type int. The class also contains-
- A parameterized constructor to initialize the value of the given parameter to val.
A copy constructor that takes a const MyClass& other reference as its parameter. This copies the value from the other object to the current object.
In the main() function:
- We create an object of MyClass called obj1 and initialize it with the value 10 using the parameterized constructor .
- Next, we create another object, obj2, and initialize it with the value of obj1 using the copy constructor.
- Then, we print the value of obj2 using the cout command and the dot operator.
- The program returns 0 to indicate successful execution.
When objects are passed by value as function arguments:
When you pass an object by value to a function, a copy of the object is made and sent to the function. This copy is created using the copy constructor.
Inside function: 10
The MyClass class, defined in the code example above, contains a public member variable , value , of type int. The class also contains-
A parameterized constructor , which initializes val to the given parameter value.
A copy constructor that takes the reference of the const variable of MyClass reference as its parameter. It copies the value from the other object to the current object.
- A member function called displayObject() takes an object of MyClass as a parameter. It prints the value of the argument passed to it using the cout command .
Inside the main() function -
- We create an object of the MyClass called obj1 and initialize it with the value 10 using the parameterized constructor .
- Next, we call the displayObject() function using obj1. A copy of obj1 is passed to the function, thus invoking the copy constructor.
- The function prints the value of the object passed to the console.
When objects are returned by value from functions:
When a function returns an object by value, a copy of the object is created using the copy constructor and returned to the caller.
obj2.value: 20
- A parameterized constructor which initializes the given parameter value with val.
- A copy constructor that takes 'const MyClass& other' reference as its parameter. It copies the value from the other object to the current object.
- The createObject() function returns a MyClass object initialized with the value 20. It invokes the copy constructor when returning the object.
In the main() function -
- We create a temporary object of MyClass, obj2 , using the createObject() function.
- This invokes the copy constructor is invoked as the temporary object is returned and assigned to obj2.
- The cout command is then used to print the value of obj2 to the console.
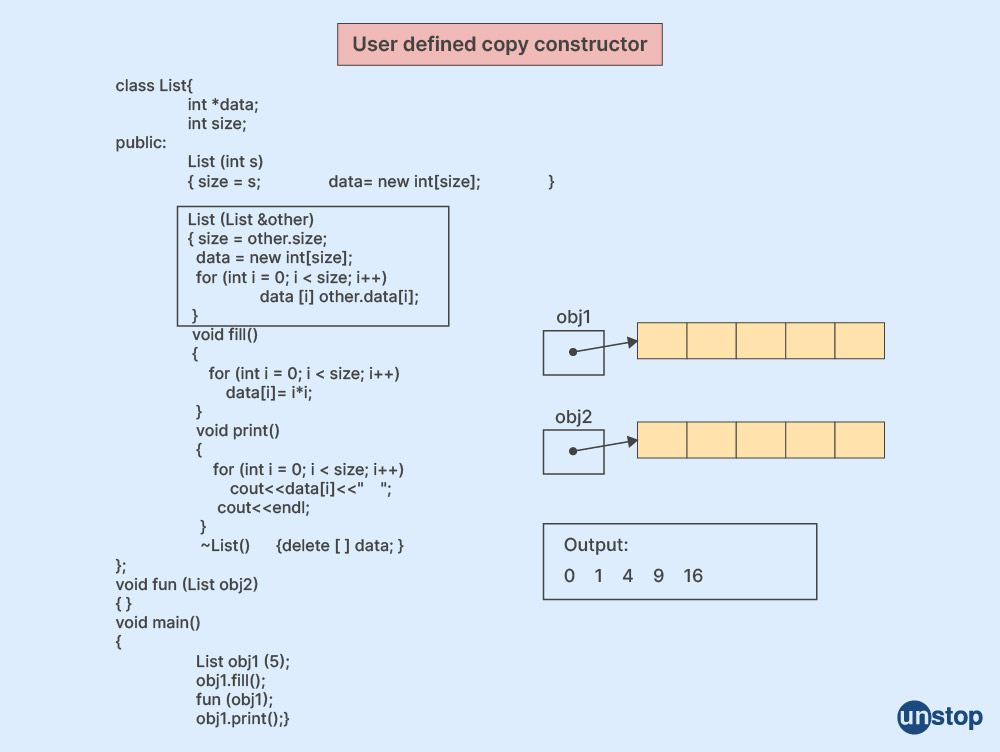
A user-defined copy constructor in C++ is needed in situations where the default copy constructor provided by the compiler does not fulfill the requirements for copying objects properly. The default copy constructor performs a shallow copy, which means it copies the values of member variables directly, including any pointers. This can lead to issues if the class contains dynamically allocated memory, pointers, or other resources that need to be managed properly. In such cases, a user-defined copy constructor can be used to ensure a deep copy or to manage resources during copying properly.
Here are some scenarios when a user-defined copy constructor is needed:
- Dynamic Memory Allocation : If your class contains dynamically allocated memory (e.g., using new to allocate memory during the time of object creation), a shallow copy performed by the default copy constructor will result in multiple objects sharing the same memory, leading to potential memory leaks or double deletion. A user-defined copy constructor can properly manage the memory by creating a new copy of the dynamically allocated memory for the new object.
- Resource Management: If your class manages external resources such as file handles, network connections, or other external entities, the default copy constructor might not handle these resources correctly. A user-defined copy constructor can ensure that each copy of the object maintains its own set of resources and properly handles their lifecycle.
- Non-shareable References: If your class contains pointers to other objects , a shallow copy will result in multiple objects pointing to the same memory locations. If one object modifies the pointed-to data, it could affect other objects unintentionally. A user-defined copy constructor can create a new set of pointers and copy the pointed-to data, resulting in a more independent copy.
- Customized Behavior : In some cases, you might want to implement specific behavior during the copy operation, such as logging, validation, or additional initialization steps. A user-defined copy constructor allows you to implement such custom behavior.
- Inheritance and Polymorphism: If your class is part of an inheritance hierarchy or participates in polymorphism, a proper copy constructor can help maintain the correct behavior of derived classes when copied.
In C++, constructors can be classified into two main types based on how they perform copying, i.e., shallow copy and deep copy.
Shallow Copy
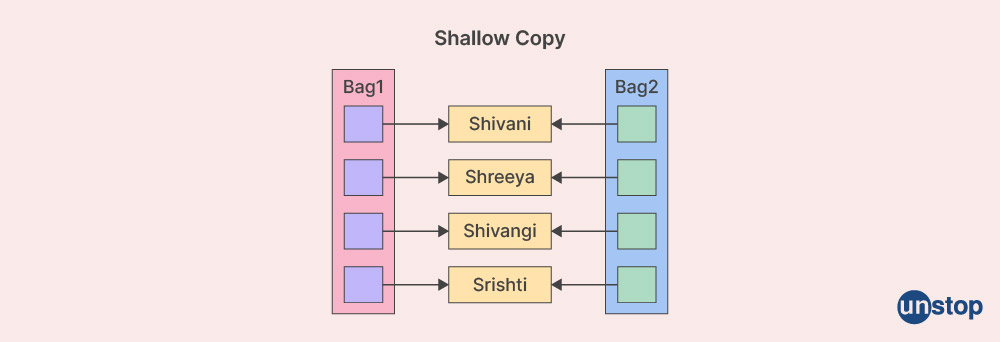
A shallow copy is a special type of copy where the values of the member variables of an object are copied directly to another object. If the class contains pointers or references to external resources, the copy process merely duplicates the memory addresses, leading to multiple objects sharing the same resources. Changes made to the shared resources in one object can affect other objects as well.
obj1.data: 5 obj2.data: 5 After modifying obj1.data... obj1.data: 10 obj2.data: 10
The code defines a class named ShallowCopyExample , which has a public member variable int* data , which points to an integer stored on the heap.
The class includes a constructor which initializes the data pointer with a dynamically allocated integer having the value val.
There is also a shallow copy constructor (i.e., ShallowCopyExample(const ShallowCopyExample& other)) that copies the data pointer from another object, resulting in both objects sharing the same memory address for the integer data.
- We create two objects of the ShallowCopyExample class, called obj1 and obj2, with the value 5 using the constructor.
- Next, we perform the shallow copy function using the assignment operator (i.e., ShallowCopyExample obj2 = obj1). This results in obj2 sharing the same data pointer as obj1.
- We then use the pointer to obj1 and obj2 with cout commands to display the initial data.
- After that, we modify the value pointed to by obj1 to 10, using *ibj1.data=10.
- We once again use the cout command to print the modified data pointer to by obj1.data and obj2.data.
- The output demonstrates that since both objects share the same memory address for their data pointers, modifying obj1.data also affects obj2.data.
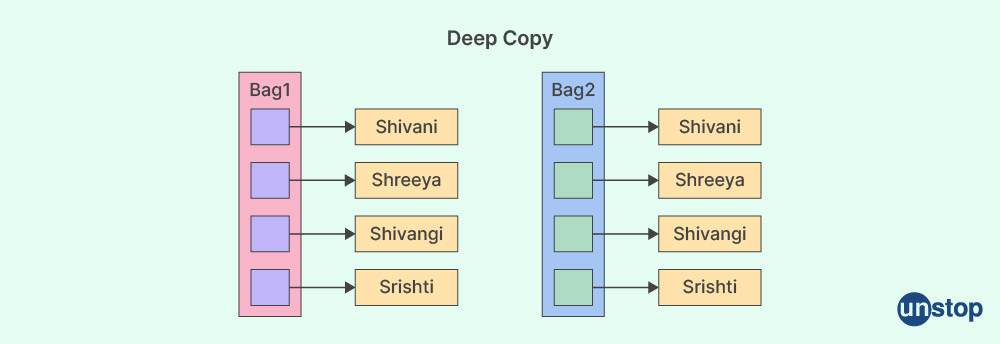
Deep copy is also known as deep clone or deep duplication. It relates to recursively creating new copies of all the objects that an object references. To put it another way, a deep copy makes an entirely separate copy of the original object, replete with all of its nested objects and their data.
Changes made to the copied item or its nested objects will not affect the original object or its nested objects when you conduct a deep copy, and vice versa. The deep copy of each thing completely differs from its original object counterpart in every way.
obj1.data: 5 obj2.data: 5 After modifying obj1.data... obj1.data: 10 obj2.data: 5
In this example, a class named DeepCopyExample is defined to demonstrate deep copy behavior.
- The class has a public integer pointer called data and a constructor that initializes the data pointer with a dynamically allocated integer, using the value passed as an argument.
- It also has a deep copy constructor DeepCopyExample(const DeepCopyExample& other), defined to create an independent copy of an existing DeepCopyExample object (other).
- Inside the deep copy constructor, a new integer is allocated, and its value is copied from the other object's data.
- The destructor ~DeepCopyExample() is also defined to properly deallocate the dynamically allocated memory for data.
- A deep copy of obj1 is made by initializing obj2 with obj1, ensuring that a new integer is allocated and its value is copied.
- The value pointed to by obj1.data is output using std::cout . And the same for obj2.data.
- The value pointed to by obj1.data is changed to 10 , demonstrating that modifications to one object's data do not affect the other.
- After modifying obj1.data, the updated value of obj1.data is output using std::cout.
- The value pointed to by obj2.data is output again using std::cout, showing that it remains unchanged due to the deep copy .
- The return 0; statement marks the end of the main function, indicating successful program execution.
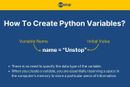
Yes, you can make the copy constructor private to prevent instances of a class from being copied. This is a technique often used to enforce a design pattern called the Singleton pattern , where you want to ensure that only one instance of a class can exist. Let's take a look at an example showcasing its implementation for a better understanding.
obj1.getData(): 10
- We first define a class named Singleton , with a private access specifier used to specify that the following class members will only be accessible from within the class itself.
- It has a private integer member variable data and a private constructor Singleton(int val) , which takes the integer argument val and initializes the private member data with the provided access value.
- Here, the static keyword indicates that the method belongs to the class itself, not an instance of it.
- A static instance of Singleton is declared, initialized with the provided value, and returned.
- This ensures a single instance is created and reused.
- We also define an int getData() const method to allow access to the private member variable data from outside the class.
- We create an instance of Singleton using the copy constructor, as mentioned in the comments . It's prevented due to the private copy constructor.
- Instead, an instance of Singleton is obtained using the getInstance method, creating a single instance of the class.
- We then use the getData method to access and output the value of the data member within the instance.
- The return 0 statement indicates successful program execution, and the program terminates.
Copy Constructor Vs. Assignment Operator
Although both the copy constructor and assignment operator deal with copying data between objects, the copy constructor is typically used when creating new class objects, while the assignment operator is used to update an already existing object. Here's a table outlining the difference between assignment operator and copy constructor in C++:
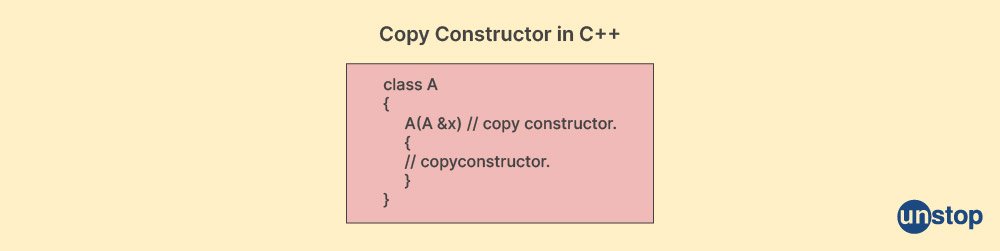
In general, if your class involves any of the following scenarios, you should provide your own copy constructor to ensure correct behavior:
- Dynamic memory allocation: If your class allocates memory dynamically (using new or malloc), the default copy constructor will only copy the memory address, resulting in shallow copies and memory leaks or double deallocations.
- Pointers to external resources: If your class holds pointers to external resources (files, databases, network connections), a proper copy constructor should handle these resources appropriately.
- Complex interactions: If your class has complex internal interactions that need to be duplicated properly when making a copy, the default copy constructor might not suffice.
Original Data: 5 Copied Data: 5
A class named DynamicResource is defined in the example above to manage a dynamic integer resource.
The class contains a private member variable , int* data , to hold the dynamically allocated integer.
It also has a constructor that initializes the data pointer with a dynamically allocated integer set to val .
We also define a custom copy constructor , i.e., DynamicResource(const DynamicResource& other), which creates a deep copy of the resource by allocating new memory and copying the value from other.data .
The class also contains a member function getData(), which takes const and returns the value stored in the dynamically allocated memory.
Next, we have a destructor ~DynamicResource() , which deallocates the dynamically allocated memory pointed to by data.
In the main() function declaration:
- We create an object called originalResource with a data value of 5.
- Another object called copiedResource is created as a copy of the original object using the custom copy constructor.
- The data stored in both objects is displayed using getData() function.
What Happens When We Remove Copy Constructor From The Above Code?
If we remove the custom copy constructor from the provided code, the default copy constructor generated by the compiler will be used instead. However, the default copy constructor performs a shallow copy, which can lead to significant problems due to the presence of dynamically allocated memory in the DynamicResoruce class.
Here's what would happen if you remove the custom copy constructor:
Default Copy Constructor (Shallow Copy): The compiler-generated default copy constructor would copy the value of the data pointer from the source object to the new object. This would result in two objects sharing the same dynamically allocated memory.
Memory Issues: Since both the original object and the copied object would point to the exact memory location, the following problems could arise:
- If one of the objects is destructed or its DynamicResource destructor is called, it would delete the shared memory, leaving the other object with a dangling pointer.
- If one of the objects modifies the shared memory, it will affect the other object, leading to unintended behavior and crashes.
Resource Leaks: When both the original object and copied object are destructed, they would both attempt to delete the same memory location. This would result in a double deletion, leading to undefined behavior.
The copy constructor in C++ is like a special recipe for making copies of objects. Just like when you want to duplicate a drawing, you have to follow a specific set of steps. Similarly, the copy constructor outlines how to create a new object that's an exact copy of another.
By learning about shallow and deep copying, we understand how to copy not only the surface details but also any hidden parts. Copy constructors help prevent messes, like forgetting to clean up after yourself or losing important information when copying. This knowledge helps us build software that's not only reliable but also efficient and well-organized. All in all, just like a recipe, a copy constructor in C++ lets us create duplicate objects with the right ingredients and steps.
Check some important technical interview questions in C++ here- 51 C++ Interview Questions For Freshers & Experienced (With Answers)
Q. Why do we use const in the copy constructor in C++?
In C++, using const (constant) in the copy constructor's parameter serves multiple important purposes. Let's explore why const is used in the copy constructor:
Preventing Modification of Source Object: By using const in the copy constructor's parameter, you are indicating that the source object being copied should not be modified within the copy constructor. This helps ensure that the copy constructor does not unintentionally modify the source object's state, maintaining the object's integrity.
class MyClass { public: // Copy constructor with const parameter MyClass(const MyClass &other) { // 'other' is treated as read-only // ... } };
Allowing Const and Non-Const Source Objects: Using const in the copy constructor's parameter allows you to copy both constant ( const MyClass obj; ) and non-constant source objects ( MyClass obj; ). If the object with parameter were not const , you would only be able to copy non-const source objects.
Supporting Temporary Objects: When using the copy constructor to copy temporary objects (created during expressions), a const reference in the copy constructor allows you to handle these temporary objects effectively.
Consistency with Immutable Objects: If your class or its members are designed to be immutable (cannot be modified after creation), using const in the copy constructor reinforces this immutability concept by indicating that the source object won't be modified.
Q. Can a copy constructor be made private?
Yes, a copy constructor can be made private in C++. Making the copy constructor private has specific use cases, often related to controlling or preventing certain operations involving object copying.
Use Cases for Private Copy Constructor in C++ are:
Singleton Pattern: In the Singleton design pattern, where you want to ensure that only one instance of a class can exist, making the copy constructor private prevents the creation of additional instances through copying.
Immutable Classes: If you're designing an immutable class where instances cannot be modified after creation, a private copy constructor prevents the creation of mutable copies of the object.
Preventing Direct Copying: In cases where you want to enforce certain rules or restrictions when copying objects, making the copy constructor private gives you control over how objects are copied.
Here's an example illustrating how to make the copy constructor in C++ private:
class Singleton { private: Singleton() { /* Constructor implementation */ } Singleton(const Singleton &other); // Private copy constructor public: static Singleton& getInstance() { static Singleton instance; return instance; } }; int main() { Singleton obj1 = Singleton::getInstance(); // Singleton obj2 = obj1; // This line will cause a compiler error due to private copy constructor return 0; }
In this example, the copy constructor of the Singleton class is made private, preventing direct copying of instances. This enforces the Singleton pattern's rule of having only one instance.
Q. Is the copy constructor in C++ automatically generated?
Yes, if you don't explicitly define a copy constructor in your C++ class, the compiler will automatically generate a default copy constructor for you.
- This default copy constructor performs a member-wise copy of the class's data members.
- It essentially creates a shallow copy, which might not be suitable for classes that manage dynamic resources or require special copying logic.
- The automatically generated copy constructor has the following signature: ClassName(const ClassName &other); Here, ClassName represents the name of your class.
It's important to note that while the automatically generated copy constructor is convenient, it might not work correctly for classes that involve deep copying or resource management. If your class contains pointers or dynamically allocated memory, you might need to define a custom copy constructor to ensure proper copying and resource handling.
Q. What are the two features of a copy constructor in C++?
The two primary features of a copy constructor in C++ are:
- Creation of a New Object: The primary purpose of a copy constructor is to create a copy of an existing object. It initializes the new object's member variables with the values of the corresponding member variables of the source object.
- Passive Invocation: The copy constructor is invoked automatically by the C++ compiler when a new object is being created as a copy of an existing object. It is called implicitly and is not directly invoked in the code sample.
Q. Does the copy constructor in C++ return a value?
No, a copy constructor in C++ does not return a value because the purpose of a copy constructor is to create a new object by copying the content of an existing object. It is responsible for initializing the new object's member variables with the values of the corresponding member variables of the source object. The copy constructor is invoked when an object is being created as a copy of another object, typically during initialization or when objects are passed by value.
Q. What is the difference between Copy Constructor and Cloning?
Q. what happens if there is no copy constructor in c++.
If you don't provide a copy constructor for a class, C++ will automatically generate a default copy constructor for you. This default constructor performs a member-wise copy of the class's data members. However, this default copy constructor might not work correctly for classes that contain dynamically allocated resources (like pointers), leading to unexpected behavior and sharing of memory issues.
Here's what happens if there is no copy constructor or if the default copy constructor is used:
Shallow Copy: The default copy constructor performs a shallow copy of the object's data members. This means that it simply copies the values of the member variables. If your class contains pointers or dynamically allocated memory, the copy will copy the pointers, not the actual data they point to.
Shared Resources: If the original object (let's call it objectA ) and the copied object ( objectB ) both contain pointers pointing to the same dynamically allocated memory (like an array or another object). Modifying the data through one object will affect the data accessed through the other object. This can lead to unintended side effects and bugs.
Memory Leaks and Double Deletion: If your class involves dynamically allocated memory and the default copy constructor is used, there can be memory leaks or double deletion issues. When the copied object goes out of scope, it might delete the same memory that the original object is still using.
Inadequate Resource Management: The default copy constructor doesn't account for proper resource management. If your class manages resources that need cleanup (e.g., file handles, network connections), the copied object might not handle these resources correctly.
To address these issues, when you have classes with separate memory allocations or other resources, it's important to define a custom copy constructor. This custom copy constructor in C++ should create new copies of dynamically allocated data and handle resource management properly to ensure that each object operates independently without causing memory leaks or unintended sharing of resources.
You might also be interested in reading the following:
- Array Of Objects In C++ | A Complete Guide To Creation (Using Examples)
- Virtual Function In C++ | A Comprehensive Guide For All (+Examples)
- Friend Function In C++ | Class, Global Use, & More! (+Examples)
- C++ Templates | Class, Function, & Specialization (With Examples)
- C++ Exception Handling | Use Try, Catch, & Throw (+Examples)
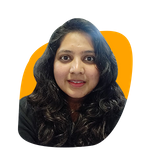
I am an economics graduate using my qualifications and life skills to observe & absorb what life has to offer. A strong believer in 'Don't die before you are dead' philosophy, at Unstop I am producing content that resonates and enables you to be #Unstoppable. When I don't have to be presentable for the job, I'd be elbow deep in paint/ pencil residue, immersed in a good read or socializing in the flesh.
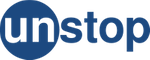
to our newsletter
Blogs you need to hog!
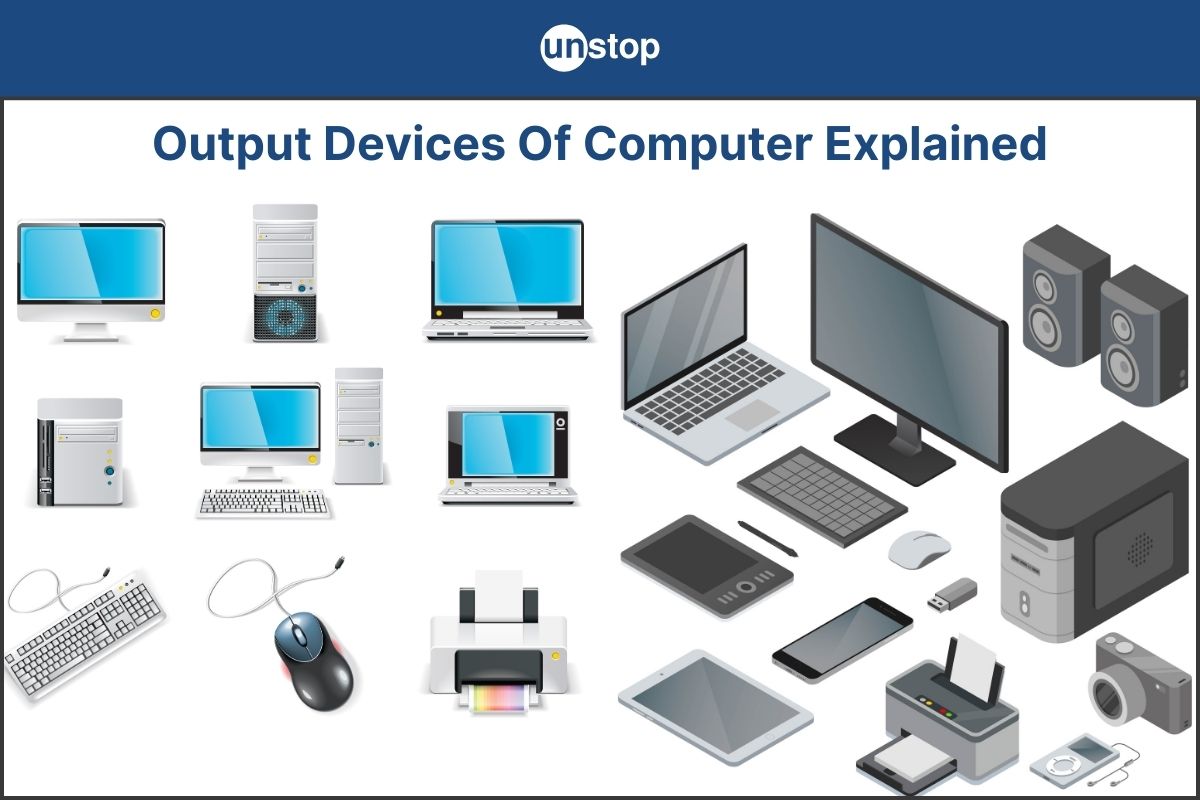
Output Devices Of Computer | Types, Functions & Applications
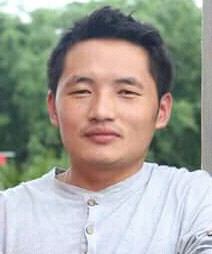
Generations Of Computer | 1st, 2nd, 3rd, 4th & 5th Generations
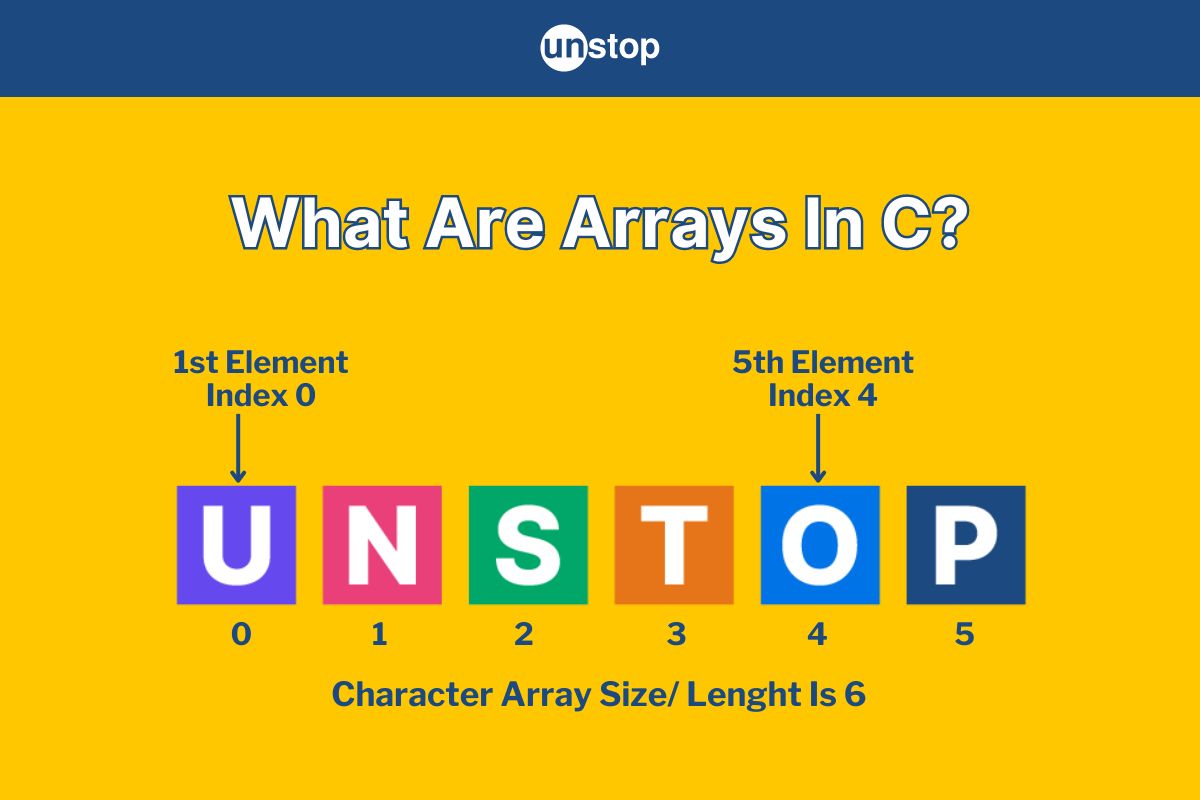
Arrays In C | Declare, Initialize, Manipulate & More (+Code Examples)
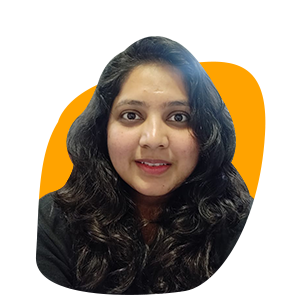
HR Compliance | Definition, Importance & Best Practices
Difference Between Copy Constructor and Assignment Operator in C++
In C++, constructors and assignment operators play critical roles in object initialization and memory management. It is crucial to understand the difference between the copy constructor and assignment operator for efficient programming.
The C++ language provides special member functions for creating and manipulating objects. Copy constructor and assignment operator are two such functions used for object copying and assignment.
The copy constructor is used to create a new object as a copy of an existing object, while the assignment operator is used to assign the value of one object to another object of the same class.
Table of Contents
Key Takeaways
What is a copy constructor in c++, what is an assignment operator in c++, key differences between copy constructor and assignment operator, object copying: deep copy vs shallow copy, syntax and usage: copy constructor, syntax and usage: assignment operator, when to use copy constructor, when to use assignment operator, example: copy constructor in c++, example: assignment operator in c++, understanding copy constructor and assignment operator in c++, c++ copy constructor and assignment operator distinction, difference between copy constructor and assignment operator in c++ with examples, copy constructor example, assignment operator example, c++ memory management: deep copy vs shallow copy, q: what is the difference between a copy constructor and an assignment operator in c++, q: what is a copy constructor in c++, q: what is an assignment operator in c++, q: what are the key differences between a copy constructor and an assignment operator, q: what is the difference between deep copy and shallow copy in object copying, q: what is the syntax and usage of a copy constructor in c++, q: what is the syntax and usage of an assignment operator in c++, q: when should a copy constructor be used, q: when should an assignment operator be used, q: can you provide an example of a copy constructor in c++, q: can you provide an example of an assignment operator in c++, q: how can i understand the copy constructor and assignment operator in c++, q: what is the distinction between the copy constructor and assignment operator in c++, q: can you explain the difference between the copy constructor and assignment operator in c++ with examples, q: how does memory management differ between deep copy and shallow copy in c++.
- The copy constructor and assignment operator are essential for object copying and assignment in C++.
- Understanding the difference between the two functions is crucial for efficient programming.
- C++ provides specific rules and conventions for syntax and usage of these functions.
In C++, object initialization is a critical aspect of memory management. A copy constructor is a special member function that is used to create a new object as a copy of an existing object. When a new object is being initialized with an existing object, the copy constructor is invoked.
The copy constructor is used to create a deep copy of an object. A deep copy means that a new copy of an object is created along with any dynamically allocated memory that the original object may have. This is different from a shallow copy, which only creates a new object that refers to the same memory as the original object.
Creating a copy constructor for a class is straightforward. The syntax for a copy constructor is as follows:
ClassName ( const ClassName & obj);
The copy constructor takes a reference to a constant object of the same class as an argument. The reference must be constant to avoid accidental modification of the original object.
Here’s an example of a copy constructor:
In this example, we have a class called Car with a private string member called make , another private string member called model , and a private integer member called year . We have defined a copy constructor for this class that takes a reference to a constant Car object as an argument.
Inside the copy constructor, we use the this keyword to refer to the current object and the dot operator to access its member variables. We then assign the values of the corresponding member variables of the passed object to the current object.
This is a simple example, but it demonstrates the basic syntax and usage of a copy constructor in C++. In the next section, we’ll explore the assignment operator and how it differs from the copy constructor.
In C++, the assignment operator is a special member function that assigns the value of one object to another object of the same class. This operator is denoted by the equal sign (=) and is invoked when this symbol is used to assign one object to another.
The assignment operator is used primarily for object assignment, which involves modifying the state of an existing object rather than creating a new one. This process can involve complex memory management capabilities, as the assignment operator must manage the allocation and deallocation of memory within the object.
Proper usage of the assignment operator is crucial for effective object manipulation, as it enables the copying of objects and their states. When used correctly, the assignment operator can facilitate memory management and efficient coding practices.
Having a solid understanding of the assignment operator in C++ is critical for proper object manipulation and memory management. Our next section will explore the key differences between the copy constructor and assignment operator, which are both essential for efficient programming.
While the copy constructor and assignment operator in C++ are both used for object copying, there are key differences between them. Understanding these differences is crucial for proper usage.
The first major difference is in the invocation of each function. The copy constructor is invoked when a new object is being initialized with an existing object, while the assignment operator is invoked when an object is already initialized and being assigned a new value.
Another significant distinction is in the return type of the functions. The copy constructor returns a new object of the same type as the original object being copied, while the assignment operator returns a reference to the object being assigned.
A third difference lies in the nature of the copying process itself. The copy constructor creates a new object as an exact duplicate of the original object, while the assignment operator modifies an existing object with the values of another object.
Finally, when it comes to memory management, the copy constructor and assignment operator also function differently. The copy constructor performs a deep copy, creating a new object with its own copy of dynamically allocated memory, while the assignment operator performs a shallow copy, where both objects share the same memory.
In summary, understanding the differences between the copy constructor and assignment operator is essential for proper object manipulation in C++. By keeping these distinctions in mind, we can efficiently and safely use these functions in our code.
When an object is copied in C++, there are two methods for handling the copying process: deep copy and shallow copy. These approaches have distinct implications for object initialization and memory management.
Deep Copy : When an object is deep copied, a new memory location is allocated for the copied object, and all of the data members and sub-objects are also copied. This means that changes made to the original object will not affect the copied object, and vice versa. In other words, the two objects are independent.
Shallow Copy : When an object is shallow copied, a new memory location is allocated for the copied object, but the data members and sub-objects are not copied. Instead, the copied object simply points to the same memory location as the original object. As a result, changes made to the original object will also affect the copied object, and vice versa. In other words, the two objects share the same data.
The copy constructor and assignment operator have different implications for deep copy and shallow copy:
Understanding the difference between deep copy and shallow copy is crucial for proper object initialization and memory management. The decision to use deep copy or shallow copy will depend on the specific needs of the program and the objects involved.
In C++, the copy constructor is a special member function that creates a new object as a copy of an existing object. It is invoked when an object is being initialized with an existing object.
The syntax for the copy constructor is as follows:
ClassName ( const ClassName& obj )
Here, ClassName is the name of the class, const ClassName& obj is a reference to the object being copied, and the constructor body initializes the new object based on the existing object.
Let’s look at an example to see how the copy constructor works in practice:
When using the copy constructor, it’s important to note that a shallow copy of the object is created if no special instructions are given. In other words, if the object contains pointers or other reference types, the copy will point to the same memory locations as the original object. To create a deep copy that copies all the data as well as any dynamic memory, it’s necessary to write a custom copy constructor or overload the assignment operator.
Now that we have discussed the copy constructor, let’s move on to the assignment operator. The syntax for the assignment operator is as follows:
class_name& operator=(const class_name& other_object)
The assignment operator is a member function of a class, just like the copy constructor. It is used to assign one object to another object of the same class. Let’s take a look at an example:
In the example above, we define the assignment operator function for the MyClass class. The function takes a constant reference to another object of the same class as a parameter. We first check if the object being assigned is not the same as the current object, to avoid self-assignment issues. We then copy the data from the other object to the current object, and finally, we return a reference to the current object.
Using the assignment operator is easy and intuitive. Suppose we have two objects of the MyClass class, obj1 and obj2. We can use the assignment operator to assign the value of obj2 to obj1 as follows:
obj1 = obj2;
This will copy the data from obj2 to obj1.
Knowing when to use the copy constructor in C++ is essential for efficient object copying. The copy constructor is typically used when we need to create a new object that is a copy of an existing object. This can occur in several scenarios, such as:
- Passing an object by value to a function
- Returning an object from a function
- Initializing an object with another object of the same class
It’s important to note that when using the copy constructor, a new object is created with its own memory and resources, which is known as a deep copy. This ensures that any changes made to the copied object do not affect the original object, avoiding any memory-related issues.
In summary, when we need to create a new object as a copy of an existing object, we should use the copy constructor in C++. This provides us with a deep copy of the object, which is vital for proper memory management and avoiding issues related to object copying.
Now that we have covered the basics of the assignment operator in C++, let’s discuss when it should be used. The assignment operator is primarily used when we want to assign the value of one object to another object of the same class. This is known as object assignment.
For example, let’s say we have two objects, object1 and object2, of the same class. We can use the assignment operator to transfer the value of object1 to object2, like this:
object2 = object1;
This will make the value of object2 identical to that of object1, effectively copying object1’s data into object2. Keep in mind that the objects must be of the same class for this to work properly.
The assignment operator is a useful tool for manipulating objects in C++. However, it should be used with caution. Improper use of the assignment operator can lead to memory leaks and other issues, so it’s important to understand its syntax and proper usage.
Now that we understand the basics of copy constructors and their differences with assignment operators, let’s take a closer look at an example that showcases how a copy constructor works in C++.
“A copy constructor is used to initialize an object from another object of the same type. It is called when an object is constructed based on another object of the same class.”
Consider the following code:
In the code above, we have defined a Person class with a default constructor, a custom constructor, and a copy constructor. We then create three instances of the Person class – john , mary , and emily , using different constructors.
The line Person emily = mary; initializes the emily object as a copy of the mary object, using the copy constructor. This means that emily now has the same values for name and age as mary .
The output of the code will be:
We can see that emily has been correctly initialized as a copy of mary , using the copy constructor.
Overall, the copy constructor is an essential part of C++ object initialization and is particularly useful when we need to make a copy of an existing object, such as when passing objects as function arguments or returning objects from functions.
In the next section, we will explore an example that showcases the usage and differences of the assignment operator in C++.
Now that we’ve seen an example of the copy constructor in action, let’s take a closer look at the assignment operator. As we mentioned earlier, the assignment operator is used to assign the value of one object to another object of the same class. The syntax for the assignment operator is similar to that of the copy constructor, but with a few key differences.
To illustrate the usage of the assignment operator, let’s consider a simple example class called Person:
// Person.h class Person { public: Person(); Person(const std::string& name, int age); ~Person(); std::string getName() const; int getAge() const; void setName(const std::string& name); void setAge(int age); void print(); Person operator=(const Person& other); private: std::string m_name; int m_age; };
In this class, we have a constructor, destructor, getter and setter functions for the name and age attributes, and a print function to display the name and age of a person. We also have defined an assignment operator, which takes an object of the same class as input and assigns its values to the current object:
// Person.cpp Person Person::operator=(const Person& other) { m_name = other.m_name; m_age = other.m_age; return *this; }
In this example, we’ve implemented a simple assignment operator that copies the name and age attributes of the passed object to the current object. Note that we’re returning a reference to the current object.
Let’s see how we can use the assignment operator:
// main.cpp #include “Person.h” #include <iostream> int main() { Person p1(“John”, 25); Person p2; p2 = p1; p1.print(); p2.print(); return 0; }
In this example, we create two Person objects, p1 and p2. We then assign the value of p1 to p2 using the assignment operator. Finally, we print the values of both objects to confirm that the assignment was successful.
This example demonstrates how to use the assignment operator to assign the values of one object to another object of the same class. Note that the syntax and usage of the assignment operator are different from those of the copy constructor.
By now, we’ve explored the differences between the copy constructor and assignment operator in C++, with specific examples illustrating their usage. Both of these functions are essential for object copying and assignment, and understanding their differences is crucial for effective programming. In the next section, we’ll discuss the distinction between shallow copy and deep copy, and how it related to object copying in C++.
As professional copywriting journalists, we understand the importance of constructors and assignment operators in C++. The copy constructor and assignment operator are used to copy and assign objects, respectively. Although they may seem similar, they serve different purposes, and understanding their differences is crucial for efficient programming.
In summary, the copy constructor creates a new object as a copy of an existing object, while the assignment operator assigns the value of one object to another object of the same class. The copy constructor is typically used when an object needs to be initialized as a copy of another object, while the assignment operator is commonly used when an object needs to be assigned the value of another object.
It is essential to understand the syntax and usage of the copy constructor and assignment operator to implement them correctly. When implementing the copy constructor, it is important to consider whether a deep or shallow copy is required. A deep copy creates a new object and copies all the data members of the existing object while a shallow copy copies only the address of the data members, resulting in two objects sharing the same memory.
Similarly, when implementing the assignment operator, it is important to consider memory management. A deep copy is generally required to ensure that the objects that are being assigned do not share the same memory.
In summary, understanding the differences between the copy constructor and assignment operator and their appropriate usage is essential for efficient object manipulation in C++. Always remember to consider memory management when implementing these member functions.
It is important to understand the distinction between the copy constructor and assignment operator in C++. While both are used for object copying, they serve different purposes. The copy constructor is used to initialize an object as a copy of an existing object. On the other hand, the assignment operator is used to assign the value of one object to another object of the same class.
One key difference between the two is in their invocation. The copy constructor is automatically invoked when a new object is being initialized with an existing object. The assignment operator, on the other hand, is manually invoked using the “=” operator to assign one object to another.
Another significant difference lies in their implementation. The copy constructor creates a new object and initializes it as an exact copy of an existing object. The assignment operator, on the other hand, does not create a new object but instead assigns the value of an existing object to another object of the same class.
Understanding the distinction between the copy constructor and assignment operator is necessary for proper object manipulation in C++. Knowing when to use each one is essential for effective programming and memory management.
In C++, constructors and assignment operators play integral roles in object initialization and memory management. It is essential to understand the difference between the copy constructor and assignment operator for efficient programming.
Although both the copy constructor and assignment operator are used in object copying, they differ in their usage and purpose:
The copy constructor is utilized when a new object is being initialized with an existing object, creating a new object as a copy of an existing object. On the other hand, the assignment operator is utilized when the value of one object is assigned to another object of the same class.
Let’s explore examples that highlight the differences between the copy constructor and assignment operator in C++ in more detail:
Consider a class named Car with private properties such as model, make, and year. Here’s an example of a copy constructor in C++, which initializes a new Car object as a copy of an existing Car object:
When the copy constructor is invoked in the above example, it creates a new Car object named myNewCar, which is a copy of the existing object myCar. The output shows that both cars have the same make, model, and year, confirming that the copy constructor has worked correctly.
Let’s now look at an example that demonstrates the usage of the assignment operator in C++. Consider the same Car class with private properties such as model, make, and year:
In the above example, the assignment operator is used to assign the value of myCar to myNewCar, replacing the original value of myNewCar. The output shows that myNewCar has been assigned the same make, model, and year as myCar.
By understanding the differences between the copy constructor and assignment operator, you can ensure efficient and safe programming in C++. Implementing these methods correctly can prevent errors and enable better memory management, leading to more effective object manipulation in your programs.
When dealing with object copying in C++, proper memory management is crucial. The way an object is copied can have a significant impact on the program’s memory usage and overall efficiency. There are two ways to handle object copying in C++: deep copy and shallow copy.
Deep copy involves copying all the members of an object, including dynamic memory allocations. This means that a completely new object is created, with its own separate memory space. Deep copying is useful for complex objects with dynamically allocated memory, where each object needs to have its own copy of the data.
Shallow copy , on the other hand, only copies the memory address of each object’s members. This means that two objects may share the same memory locations, making changes to one object affect the other. Shallow copying is useful for simple objects, where there is no dynamically allocated memory.
To ensure proper memory management and prevent memory leaks, it is essential to understand the implications of each approach and use them appropriately.
As we have learned, the copy constructor and assignment operator serve distinct purposes in C++ object initialization and assignment.
Understanding their differences is crucial for proper object copying and assignment. The copy constructor is used when an object needs to be initialized as a copy of another object, while the assignment operator is commonly used when an object needs to be assigned the value of another object.
Furthermore, knowing the difference between deep copy and shallow copy is essential for efficient memory management when dealing with object copying. Deep copy creates a new object with its own memory, while shallow copy shares memory with the original object, potentially leading to memory-related issues.
By implementing the copy constructor and assignment operator correctly and understanding their appropriate usage, we can ensure efficient and error-free programming in C++. Remember to always pay attention to memory management and choose the right method of object copying for your specific needs.
A: The copy constructor is used to create a new object as a copy of an existing object, while the assignment operator is used to assign the value of one object to another object of the same class.
A: A copy constructor is a special member function that is used to create a new object as a copy of an existing object. It is invoked when a new object is being initialized with an existing object.
A: An assignment operator is a special member function that is used to assign the value of one object to another object of the same class. It is invoked when the “=” operator is used to assign one object to another.
A: Although both the copy constructor and assignment operator are used for object copying, there are some key differences between them. Understanding these differences is essential for proper usage.
A: When an object is copied, there are two ways to handle the copying process: deep copy and shallow copy. Understanding the implications of each approach is vital for correct object initialization.
A: The syntax and usage of a copy constructor in C++ involve specific rules and conventions. Knowing how to implement and use the copy constructor correctly is essential for proper object copying.
A: The syntax and usage of an assignment operator in C++ also follow specific rules and conventions. Understanding how to implement and use the assignment operator properly is crucial for object assignment.
A: The copy constructor is typically used when an object needs to be initialized as a copy of another object. Knowing when to use the copy constructor is important for efficient and safe programming.
A: The assignment operator is commonly used when an object needs to be assigned the value of another object. Understanding when to use the assignment operator is crucial for proper object manipulation.
A: To illustrate the usage and differences between the copy constructor and assignment operator, let’s look at an example that showcases the copy constructor in C++.
A: Continuing from the previous example, let’s explore an example that demonstrates the usage and differences of the assignment operator in C++.
A: By now, you should have a solid understanding of the copy constructor and assignment operator in C++. Knowing their purpose and differences is essential for effective object manipulation.
A: The distinction between the copy constructor and assignment operator lies in their usage and purpose. Understanding this distinction is crucial for correctly managing object copying and assignment in C++.
A: To further clarify the differences between the copy constructor and assignment operator, let’s explore some specific examples that highlight these distinctions.
A: Proper memory management is crucial when dealing with object copying in C++. Understanding the difference between deep copy and shallow copy is essential for avoiding memory-related issues.
Deepak Vishwakarma
Difference Between Error and Exception in Java
Difference Between Inline and Macro in C++
RELATED Articles
Software Engineer Home Depot Salary
Senior principal software engineer salary, mastercard software engineer salary, optiver software engineer salary, capital one senior software engineer salary, ford software engineer salary, leave a comment cancel reply.
Save my name, email, and website in this browser for the next time I comment.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
State Farm Software Engineer Salary
Google Sheet Tutorials
Interview Questions and Answers
© 2024 Saintcoders • Designed by Coding Interview Pro
Privacy policy
Terms and Conditions
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Copy constructors and copy assignment operators (C++)
- 8 contributors
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment . In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++) .
Both the assignment operation and the initialization operation cause objects to be copied.
Assignment : When one object's value is assigned to another object, the first object is copied to the second object. So, this code copies the value of b into a :
Initialization : Initialization occurs when you declare a new object, when you pass function arguments by value, or when you return by value from a function.
You can define the semantics of "copy" for objects of class type. For example, consider this code:
The preceding code could mean "copy the contents of FILE1.DAT to FILE2.DAT" or it could mean "ignore FILE2.DAT and make b a second handle to FILE1.DAT." You must attach appropriate copying semantics to each class, as follows:
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x); .
Use the copy constructor.
If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you. Similarly, if you don't declare a copy assignment operator, the compiler generates a member-wise copy assignment operator for you. Declaring a copy constructor doesn't suppress the compiler-generated copy assignment operator, and vice-versa. If you implement either one, we recommend that you implement the other one, too. When you implement both, the meaning of the code is clear.
The copy constructor takes an argument of type ClassName& , where ClassName is the name of the class. For example:
Make the type of the copy constructor's argument const ClassName& whenever possible. This prevents the copy constructor from accidentally changing the copied object. It also lets you copy from const objects.
Compiler generated copy constructors
Compiler-generated copy constructors, like user-defined copy constructors, have a single argument of type "reference to class-name ." An exception is when all base classes and member classes have copy constructors declared as taking a single argument of type const class-name & . In such a case, the compiler-generated copy constructor's argument is also const .
When the argument type to the copy constructor isn't const , initialization by copying a const object generates an error. The reverse isn't true: If the argument is const , you can initialize by copying an object that's not const .
Compiler-generated assignment operators follow the same pattern for const . They take a single argument of type ClassName& unless the assignment operators in all base and member classes take arguments of type const ClassName& . In this case, the generated assignment operator for the class takes a const argument.
When virtual base classes are initialized by copy constructors, whether compiler-generated or user-defined, they're initialized only once: at the point when they are constructed.
The implications are similar to the copy constructor. When the argument type isn't const , assignment from a const object generates an error. The reverse isn't true: If a const value is assigned to a value that's not const , the assignment succeeds.
For more information about overloaded assignment operators, see Assignment .
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
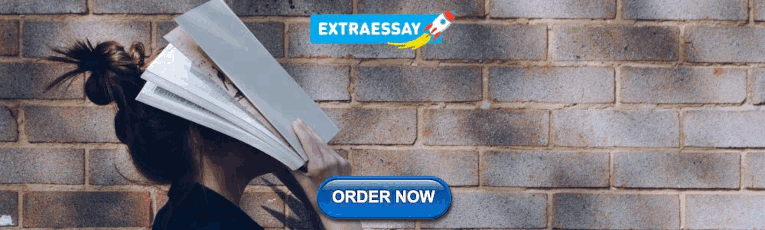
IMAGES
VIDEO
COMMENTS
C++ compiler implicitly provides a copy constructor, if no copy constructor is defined in the class. A bitwise copy gets created, if the Assignment operator is not overloaded. Consider the following C++ program. Explanation: Here, t2 = t1; calls the assignment operator, same as t2.operator= (t1); and Test t3 = t1; calls the copy constructor ...
The copy constructor is for creating a new object. It copies an existing object to a newly constructed object.The copy constructor is used to initialize a new instance from an old instance. It is not necessarily called when passing variables by value into functions or as return values out of functions. The assignment operator is to deal with an ...
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
The first line runs the copy constructor of T, which can throw; the remaining lines are assignment operators which can also throw. HOWEVER, if you have a type T for which the default std::swap() may result in either T's copy constructor or assignment operator throwing, you are
using the copy constructor. What C++ Does For You Unless you specify otherwise, C++ will automatically provide objects a basic copy constructor and assignment operator that simply invoke the copy constructors and assignment operators of all the class's data members. In many cases, this is exactly what you want. For example, consider the ...
The purpose of the copy constructor and the copy assignment operator are almost equivalent -- both copy one object to another. However, the copy constructor initializes new objects, whereas the assignment operator replaces the contents of existing objects. ... In this particular example, the self-assignment causes each member to be assigned to ...
The rule of three is a well known C++ principle that states that if a class requires a user-defined copy constructor, destructor, or copy assignment operator, then it probably requires all three. In C++11, this was expanded to the rule of five, which adds the move constructor and move assignment operator to the list.
In C++, assignment and copy construction are different because the copy constructor initializes uninitialized memory, whereas assignment starts with an existing initialized object. If your class contains instances of other classes as data members, the copy constructor must first construct these data members before it calls operator=.
The copy constructor in C++ is used to copy data from one object to another. For example, #include <iostream> using namespace std; // declare a class class Wall { private: double length; double height; public: // initialize variables with parameterized constructor. Wall(double len, double hgt) {.
Your copy assignment operator is implemented incorrectly. The object being assigned to leaks the object its base points to.. Your default constructor is also incorrect: it leaves both base and var uninitialized, so there is no way to know whether either is valid and in the destructor, when you call delete base;, Bad Things Happen.. The easiest way to implement the copy constructor and copy ...
The Copy Assignment Operator in a class is a non-template non-static member function that is declared with the operator=. When you create a class or a type that is copy assignable (that you can copy with the = operator symbol), it must have a public copy assignment operator. Here is a simple syntax for the typical declaration of a copy ...
Copy Constructor Syntax in C++. // body of the constructor. The syntax of copy structure in C++ is "ClassName (const ClassName &objectToCopy)", where "const" indicates no modification to the original object when copying will be made. For example, you have two strings, 'stringA' and 'stringB'.
A copy constructor in C++ files is a unique type of constructor that makes a copy of an existing object. It resembles casting a spell to copy one object's characteristics into another one. Copy constructors play a crucial role in ensuring that the copy is made correctly and that the new object is an independent instance with its own memory space.
It actually declares a variable other of type FeatureValue. This is because constructors to not have names and cannot be called directly. You can safely invoke the copy assignment operator from the constructor as long as the operator is not declared virtual. FeatureValue::FeatureValue(const FeatureValue& other) : m_value(nullptr), m_size(0)
In C++, constructors and assignment operators play critical roles in object initialization and memory management. It is crucial to understand the difference between the copy constructor and assignment operator for efficient programming.. The C++ language provides special member functions for creating and manipulating objects.
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment. In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see
No assignment operator is used in the first test-case. It just uses the initialization form called "copy initialization". Copy initialization does not consider explicit constructors when initializing the object. struct A {. A(); // explicit copy constructor. explicit A(A const&); // explicit constructor. explicit A(int);
5. I am writing unit tests for a few classes (C++), and came across an issue attempting to write a unit test for the copy constructor and assignment operator. A basic thing that could be wrong with either is that a programmer adds a member to the class and then forgets to update the c'ctor and/or operator=. I could of course write a unit test ...