
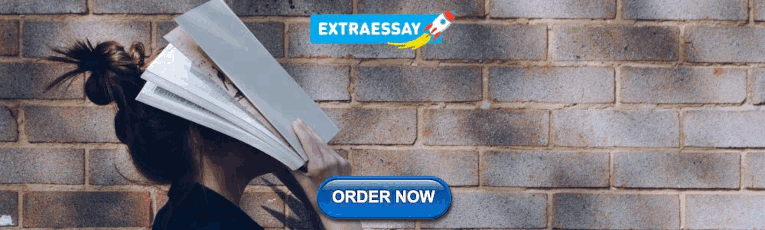
7th Gear (v3.57.1) edition
- Install now
Rule return_assignment ¶
Local, dynamic and directly referenced variables should not be assigned and directly returned by a function or method.
Example #1 ¶
Rule sets ¶.
The rule is part of the following rule set:
@PhpCsFixer
References ¶
Fixer class: PhpCsFixer\Fixer\ReturnNotation\ReturnAssignmentFixer
Test class: PhpCsFixer\Tests\Fixer\ReturnNotation\ReturnAssignmentFixerTest
The test class defines officially supported behaviour. Each test case is a part of our backward compatibility promise.
Return Statement in PHP : Tutorial
Home » PHP Tutorial » PHP Operators
PHP Operators
Summary : in this tutorial, you will learn about PHP operators and how to use them effectively in your script.
An operator takes one or more values, known as operands, and performs a specific operation on them.
For example, the + operator adds two numbers and returns the sum of them.
PHP supports many kinds of operators:
Arithmetic Operators
Assignment operators, bitwise operators, comparison operators.
- Increment/Decrement Operators
Logical Operators
- Concatenating Operators
The arithmetic operators require numeric values. If you apply them to non-numeric values, they’ll convert them to numeric values before carrying the arithmetic operation.
The following are the list of arithmetic operators:
The following example uses the arithmetic operators:
Comparison operators allow you to compare two operands.
A comparison operator returns a Boolean value, either true or false . If the comparison is truthful, the comparison operator returns true , otherwise, it returns false .
The following are the list of comparison operators in PHP:
Logical operators allow you to construct logical expressions. A logical operator returns a Boolean value.
PHP provides the following logical operators:
Bitwise operators perform operations on the binary representation of the operands. The following illustrates bitwise operators in PHP:
Incrementing/ Decrementing Operators
Increment (++) and decrement (–) operators give you a quick way to increase and decrease the value of a variable by 1.
The following table illustrates the increment and decrement operators:
Concatenating Operator
Concatenating operator (.) allows you to combine two strings into one. It appends the second string to the first one and returns the combined string. For example:
Assignment operator ( = ) assigns a value to a variable and returns a value. The operand on the left is always a variable, while the operand on the right can be a literal value, variable, expression, or a function call that returns a value. For example:
In the first expression, we assigned $x variable value 10 . In the second one, we assigned the value of $x to $y variable. The third one is a little bit complicated. First, we assigned 20 to $x . The assignment operator ( = ) returns 20 and then 20 is assigned to $z variable.
Besides the basic assignment operator( = ), PHP provides you with some assignment operators:
- plus-equal +=
- minus-equal -=
- divide-equal /=
- multiplication-equal *=
- modulus-equal %=
- XOR-equal ^=
- AND-equal &=
- OR-equal |=
- concatenate-equal .=
PHP operators precedence
The precedence of an operator decides which order the operator is evaluated in an expression.
PHP assigned each operator precedence. Some operators have the same precedence, e.g., precedences of the addition ( + ) and subtraction( - ) are equal.
However, some operators have higher precedence than others.
For example, the precedence of the multiplication operator ( * ) is higher than the precedence of the add( + ) and the subtract ( - ) operators:
Because the precedence of the multiplication operator ( * ) is higher than the precedence of the add( + ) operator, PHP evaluates the multiplication operator ( * ) first and then add operator ( * ) second.
To force the evaluation in a particular order, you put the expression inside parentheses () , for example:
In this tutorial, you have briefly learned about the most commonly used PHP operators.

- PHP All Exercises & Assignments
Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database.
Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.
Write a program to count 5 to 15 using PHP loop
Description: Write a Program to display count, from 5 to 15 using PHP loop as given below.
Rules & Hint
- You can use “for” or “while” loop
- You can use variable to initialize count
- You can use html tag for line break
View Solution/Program
5 6 7 8 9 10 11 12 13 14 15
Write a program to print “Hello World” using echo
Description: Write a program to print “Hello World” using echo only?
Conditions:
- You can not use any variable.
View Solution /Program
Hello World
Write a program to print “Hello PHP” using variable
Description: Write a program to print “Hello PHP” using php variable?
- You can not use text directly in echo but can use variable.
Write a program to print a string using echo+variable.
Description: Write a program to print “Welcome to the PHP World” using some part of the text in variable & some part directly in echo.
- You have to use a variable that contains string “PHP World”.
Welcome to the PHP World
Write a program to print two variables in single echo
Description: Write a program to print 2 php variables using single echo statement.
- First variable have text “Good Morning.”
- Second variable have text “Have a nice day!”
- Your output should be “Good morning. Have a nice day!”
- You are allowed to use only one echo statement in this program.
Good Morning. Have a nice day!
Write a program to check student grade based on marks
Description:.
Write a program to check student grade based on the marks using if-else statement.
- If marks are 60% or more, grade will be First Division.
- If marks between 45% to 59%, grade will be Second Division.
- If marks between 33% to 44%, grade will be Third Division.
- If marks are less than 33%, student will be Fail.
Click to View Solution/Program
Third Division
Write a program to show day of the week using switch
Write a program to show day of the week (for example: Monday) based on numbers using switch/case statements.
- You can pass 1 to 7 number in switch
- Day 1 will be considered as Monday
- If number is not between 1 to 7, show invalid number in default
It is Friday!
Write a factorial program using for loop in php
Write a program to calculate factorial of a number using for loop in php.
The factorial of 3 is 6
Factorial program in PHP using recursive function
Exercise Description: Write a PHP program to find factorial of a number using recursive function .
What is Recursive Function?
- A recursive function is a function that calls itself.
Write a program to create Chess board in PHP using for loop
Write a PHP program using nested for loop that creates a chess board.
- You can use html table having width=”400px” and take “30px” as cell height and width for check boxes.

Write a Program to create given pattern with * using for loop
Description: Write a Program to create following pattern using for loops:
- You can use for or while loop
- You can use multiple (nested) loop to draw above pattern
View Solution/Program using two for loops
* ** *** **** ***** ****** ******* ********
Simple Tips for PHP Beginners
When a beginner start PHP programming, he often gets some syntax errors. Sometimes these are small errors but takes a lot of time to fix. This happens when we are not familiar with the basic syntax and do small mistakes in programs. These mistakes can be avoided if you practice more and taking care of small things.
I would like to say that it is never a good idea to become smart and start copying. This will save your time but you would not be able to understand PHP syntax. Rather, Type your program and get friendly with PHP code.
Follow Simple Tips for PHP Beginners to avoid errors in Programming
- Start with simple & small programs.
- Type your PHP program code manually. Do not just Copy Paste.
- Always create a new file for new code and keep backup of old files. This will make it easy to find old programs when needed.
- Keep your PHP files in organized folders rather than keeping all files in same folder.
- Use meaningful names for PHP files or folders. Some examples are: “ variable-test.php “, “ loops.php ” etc. Do not just use “ abc.php “, “ 123.php ” or “ sample.php “
- Avoid space between file or folder names. Use hyphens (-) instead.
- Use lower case letters for file or folder names. This will help you make a consistent code
These points are not mandatory but they help you to make consistent and understandable code. Once you practice this for 20 to 30 PHP programs, you can go further with more standards.
The PHP Standard Recommendation (PSR) is a PHP specification published by the PHP Framework Interop Group.
Experiment with Basic PHP Syntax Errors
When you start PHP Programming, you may face some programming errors. These errors stops your program execution. Sometimes you quickly find your solutions while sometimes it may take long time even if there is small mistake. It is important to get familiar with Basic PHP Syntax Errors
Basic Syntax errors occurs when we do not write PHP Code correctly. We cannot avoid all those errors but we can learn from them.
Here is a working PHP Code example to output a simple line.
Output: Hello World!
It is better to experiment with PHP Basic code and see what errors happens.
- Remove semicolon from the end of second line and see what error occurs
- Remove double quote from “Hello World!” what error occurs
- Remove PHP ending statement “?>” error occurs
- Use “
- Try some space between “
Try above changes one at a time and see error. Observe What you did and what error happens.
Take care of the line number mentioned in error message. It will give you hint about the place where there is some mistake in the code.
Read Carefully Error message. Once you will understand the meaning of these basic error messages, you will be able to fix them later on easily.
Note: Most of the time error can be found in previous line instead of actual mentioned line. For example: If your program miss semicolon in line number 6, it will show error in line number 7.
Using phpinfo() – Display PHP Configuration & Modules
phpinfo() is a PHP built-in function used to display information about PHP’s configuration settings and modules.
When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality. For example, the date-time extension provides some ready-made function related to date and time formatting. MySQL modules are integrated to deal with PHP Connections.
It is good to take a look on those extensions. Simply use
phpinfo() function as given below.
Example Using phpinfo() function

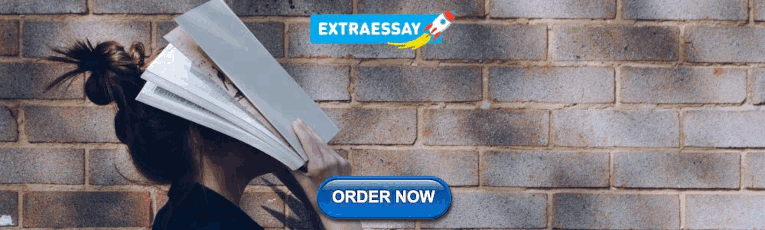
Write a PHP program to add two numbers
Write a program to perform sum or addition of two numbers in PHP programming. You can use PHP Variables and Operators
PHP Program to add two numbers:
Write a program to calculate electricity bill in php.
You need to write a PHP program to calculate electricity bill using if-else conditions.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements .
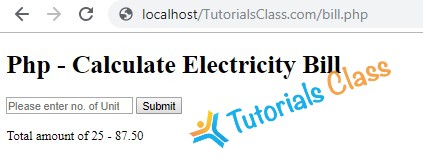
Write a simple calculator program in PHP using switch case
You need to write a simple calculator program in PHP using switch case.
Operations:
- Subtraction
- Multiplication

Remove specific element by value from an array in PHP?
You need to write a program in PHP to remove specific element by value from an array using PHP program.
Instructions:
- Take an array with list of month names.
- Take a variable with the name of value to be deleted.
- You can use PHP array functions or foreach loop.
Solution 1: Using array_search()
With the help of array_search() function, we can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 2: Using foreach()
By using foreach() loop, we can also remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 3: Using array_diff()
With the help of array_diff() function, we also can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [2]=> string(5) “march” [4]=> string(3) “may” }
Write a PHP program to check if a person is eligible to vote
Write a PHP program to check if a person is eligible to vote or not.
- Minimum age required for vote is 18.
- You can use PHP Functions .
- You can use Decision Making Statements .
Click to View Solution/Program.
You Are Eligible For Vote
Write a PHP program to calculate area of rectangle
Write a PHP program to calculate area of rectangle by using PHP Function.
- You must use a PHP Function .
- There should be two arguments i.e. length & width.
View Solution/Program.
Area Of Rectangle with length 2 & width 4 is 8 .
- Next »
- PHP Exercises Categories
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php return keyword.
❮ PHP Keywords
Return a value from a function:
Definition and Usage
The return keyword ends a function and, optionally, uses the result of an expression as the return value of the function.
If return is used outside of a function, it stops PHP code in the file from running. If the file was included using include , include_once , require or require_once , the result of the expression is used as the return value of the include statements.
Related Pages
Learn more functions in our PHP Functions Tutorial .

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

- SUGGESTED TOPICS
- The Magazine
- Newsletters
- Managing Yourself
- Managing Teams
- Work-life Balance
- The Big Idea
- Data & Visuals
- Reading Lists
- Case Selections
- HBR Learning
- Topic Feeds
- Account Settings
- Email Preferences
When Someone You Manage Isn’t Following the Return-to-Office Policy
- Rebecca Knight

How to strike a balance between enforcing company mandates and supporting the needs of your team.
What should you do if some of your team members still don’t want to come into the office after your company has imposed a mandate? How do you strike the right balance between following company policies and understanding the needs of your employees ? In this article, the author shares advice from two experts on how to navigate this complicated situation.
Ever since the first return-to-office mandates emerged in 2021 , managers have grappled with the delicate task of balancing organizational policies with the preferences of their employees. The pressure from higher-ups to bring people back to their cubicles has been building over the years, and as a manager, you need to tow the company line.

- RK Rebecca Knight is a journalist who writes about all things related to the changing nature of careers and the workplace. Her essays and reported stories have been featured in The Boston Globe, Business Insider, The New York Times, BBC, and The Christian Science Monitor. She was shortlisted as a Reuters Institute Fellow at Oxford University in 2023. Earlier in her career, she spent a decade as an editor and reporter at the Financial Times in New York, London, and Boston.
Partner Center
Cruise ships to return to Baltimore two months after bridge collapse
Royal Caribbean and Carnival temporarily relocated to Norfolk after the Key Bridge collapse in March.

Cruises will soon set sail from Baltimore again, resuming operations two months after a cargo ship took down the Francis Scott Key Bridge.
In an X post on Wednesday, the Port of Baltimore revealed that Royal Caribbean International’s Vision of the Seas would leave on May 25 for a five-night trip to Bermuda. It will be the first cruise from a major carrier that leaves from Baltimore since the deadly bridge collapse on March 26.
The cruise line confirmed the news, saying in a statement it “looks forward to returning to the Port of Baltimore once again.”
Three major cruise lines sail from Baltimore, though only two had ships based at the port during the spring. No cruise ships were in port when the accident happened. After some scrambling , Royal Caribbean and Carnival Cruise Line both moved their Baltimore-based operations to Norfolk.
Carnival said in a statement that it plans to sail the Pride from Norfolk on May 19. That ship is expected to finish the cruise in Baltimore a week later. Because of those plans, the cruise line is encouraging guests to make their way to Baltimore ahead of the trip, and take a free, Carnival-provided bus more than 200 miles to Norfolk.
The ship is scheduled to leave Baltimore for a 14-day Greenland and Canada itinerary on May 26 before resuming seven-day Bahamas or Caribbean cruises.
“We remain in close contact with local, state and federal officials regarding the return of our operations at the Port of Baltimore,” Carnival said. The port said Wednesday it could only confirm Royal Caribbean’s schedule.
Norwegian Cruise Line does not have any Baltimore sailings scheduled until September. American Cruise Lines, a smaller company that operates river and coastal cruises, was able to start operating Chesapeake Bay trips from Baltimore earlier this month.
More than 444,000 people cruised out of Baltimore’s port last year, according to a news release issued by Maryland Gov. Wes Moore’s office.
Baltimore bridge collapse
How it happened: Baltimore’s Francis Scott Key Bridge collapsed after being hit by a cargo ship . The container ship lost power shortly before hitting the bridge, Maryland Gov. Wes Moore (D) said. Video shows the bridge collapse in under 40 seconds.
Victims: Divers have recovered the bodies of two construction workers , officials said. They were fathers, husbands and hard workers . A mayday call from the ship prompted first responders to shut down traffic on the four-lane bridge, saving lives.
Economic impact: The collapse of the bridge severed ocean links to the Port of Baltimore, which provides about 20,000 jobs to the area . See how the collapse will disrupt the supply of cars, coal and other goods .
Rebuilding: The bridge, built in the 1970s , will probably take years and cost hundreds of millions of dollars to rebuild , experts said.

- SI SWIMSUIT
- SI SPORTSBOOK
Padres' All-Star Joe Musgrove Provides Injury Update as Return Nears
Ricardo sandoval | may 15, 2024.
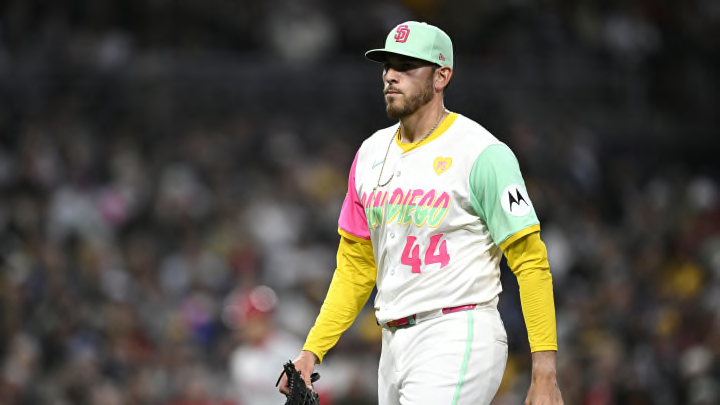
- San Diego Padres
San Diego Padres All-Star starting pitcher Joe Musgrove feels much better as he's recovering from inflammation in his right elbow. In a recent interview with the media, including the San Diego Union-Tribune's Jeff Sanders , Musgrove said he felt good in his recent Monday bullpen session.
"I feel really good today," Musgrove said. "The stuff was coming out really good yesterday. Didn't spin very much, but we're going to do that tomorrow. I was spinning balls in catch, but we didn't throw any off the mound. Yeah, it went really well."
Musgrove threw a "light" bullpen session on Monday and completed his first since joining the 15-day injured list May 5. Musgrove is eligible to come off the IL on Friday. However, returning on Sunday — when Randy Vásquez would start next — keeps the rest of the rotation on turn. If Musgrove looks excellent in his Wednesday session, he could return in the upcoming series against the National League powerhouse Atlanta Braves.
Musgrove has had a poor start to the season. In eight starts thus far, he's 3-3 with a 6.37 ERA, a 61 ERA+, and a 1.51 WHIP in 41 innings. The 31-year-old suffered from a shoulder injury last year that ended his season prematurely in July. Combined with a weight room accident in spring training that left him with a broken toe , Musgrove was limited to just 17 starts and 101.1 innings in 2023.
So far, it's been more of the same on the injury front for Musgrove. Nonetheless, the one-time All-Star is trending in the right direction. This is the right-handed pitcher's fourth season as a Friar.
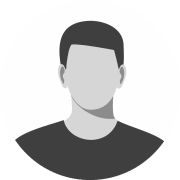
RICARDO SANDOVAL
Baseball Enthusiast
- Share full article
Advertisement
Supported by
In Britain, Chasing a Glimpse of the Northern Lights
Sightings of the aurora borealis are unlikely in the U.K. this weekend, but the northern lights could return on Monday, forecasters said.

By Isabella Kwai
Reporting from London, where she failed to see the Northern Lights
They were peering over their balconies, huddled at the top of lookouts or drinking coffee, eyes turned to the sky for a hint of color — any color.
As night fell, chasers of the aurora borealis in the northern hemisphere on Friday were out again. The vivid hues, which are most often seen closer to the North Pole, lit up skies in an unusual showing in the lower latitudes of Europe and North America last week .
Those who missed the lights, or who were eager to see another showing, set their alarms and monitored aurora watch apps, hopeful for another opportunity. Some people in Canada and Britain said they were rewarded, while others in the northern United States kept a watchful eye on forecasts .
But predicting when an aurora will show up can be tricky, forecasters said, given that sun activity during its cycle is constantly evolving.
“Broadly speaking, though, we do know that activity and sunspot numbers should increase in this part of the cycle,” Tom Morgan, a meteorologist for the Met Office, said.
At least for this weekend, aurora sightings in the United Kingdom are unlikely, according to the Met Office, though there is a “slight chance” that the lights may appear in northern Scotland before sunrise on Sunday.
The northern lights could return on Monday over Scotland and Northern Ireland, and there is a chance they could be visible to the naked eye in northern England and Wales. Monday is expected to be clear, with some showers.
Seeing the northern lights, seasoned chasers say, takes planning, patience and a group effort.
“We set up a small aurora group in my little village,” said Steve Emery, 50, who lives in the village of Hesket Newmarket in northwest England. He said that a group of about 20 people had been chatting about the forecast.
“It’s kind of become a local hobby, which is quite fun,” he said.
Mr. Emery was sitting in bed when alerts pinged at 1 a.m. that the northern lights might be visible. He and others in a chat group rushed in their cars to the top of a nearby hill.
“They were faint but you could definitely see the greens, particularly, and the purples,” he said. “They were shimmering as well.”
Mr. Emery, 50, said that the movement of the lights reminded him of closed curtains swaying at the end of a theater show. Within five minutes, he said, they had come and gone.
“It happens so quick, you need to be ready for it,” he said, adding that the colors are different each time. “You never know quite what you’re going to see. That’s the addictive nature of it .”
Others, even with preparation, were foiled by light pollution, a bright moon or clouds.
Had they glimpsed the aurora, or was it simply nearby light pollution? (That unique disappointment was captured in a TikTok video when two friends in Norwich shared that the purple glow of what they had thought was the aurora borealis was, in fact, the light coming from a Premier Inn.)
Lt. Bryan R. Brasher, project manager at the Space Weather Prediction Center, said that by Sunday and Monday, “conditions are expected to increase slightly back to unsettled or active levels,” with a chance for minor solar storms.
“At that level of storming, aurora would likely be viewable across parts of Alaska and Northern Canada, with a slight chance for aurora viewing along the northern border of the contiguous U.S.,” he said.
The display of northern lights last week came after the National Oceanic and Atmospheric Administration issued a rare warning on May 10 that a Level 5 solar storm had reached Earth — an extreme event not recorded since October 2003.
Seasoned chasers and experts have a few tips: Get away from the city lights. Go to a vantage point with clear views, like the top of a hill. Look north. And use your cellphone to take photos since it can pick up more wavelengths than the naked eye.
In Norway, Cathe Sletaker was getting ready for bed in her home in Hole, about an hour northwest of Oslo, when she got an alert. She went onto her balcony.
The sky was light, but she caught a pale showing of purple, lilac and green lights.
“I stayed there until 3 o’clock in the morning,” she said. “My cat visited me, too, and I took a nice picture of her.”
The lights, she said, were not as strong as the spectacle last week, but Ms. Sletaker still felt a tingle.
“I get the feeling — perhaps it’s a bit big to say — of the universe; everything comes a bit close from outer space,” she said, adding, “It’s a kind of magic.”
Isabella Kwai is a Times reporter based in London, covering breaking news and other trends. More about Isabella Kwai
What’s Up in Space and Astronomy
Keep track of things going on in our solar system and all around the universe..
Never miss an eclipse, a meteor shower, a rocket launch or any other 2024 event that’s out of this world with our space and astronomy calendar .
A dramatic blast from the sun set off the highest-level geomagnetic storm in Earth’s atmosphere, making the northern lights visible around the world .
With the help of Google Cloud, scientists who hunt killer asteroids churned through hundreds of thousands of images of the night sky to reveal 27,500 overlooked space rocks in the solar system .
A celestial image, an Impressionistic swirl of color in the center of the Milky Way, represents a first step toward understanding the role of magnetic fields in the cycle of stellar death and rebirth.
Scientists may have discovered a major flaw in their understanding of dark energy, a mysterious cosmic force . That could be good news for the fate of the universe.
Is Pluto a planet? And what is a planet, anyway? Test your knowledge here .
- Function Reference
- Variable and Type Related Extensions
- Array Functions
(PHP 4, PHP 5, PHP 7, PHP 8)
list — Assign variables as if they were an array
Description
Like array() , this is not really a function, but a language construct. list() is used to assign a list of variables in one operation. Strings can not be unpacked and list() expressions can not be completely empty.
Note : Before PHP 7.1.0, list() only worked on numerical arrays and assumes the numerical indices start at 0.
A variable.
Further variables.
Return Values
Returns the assigned array.
Example #1 list() examples
Example #2 An example use of list()
Example #3 Using nested list()
Example #4 list() and order of index definitions
The order in which the indices of the array to be consumed by list() are defined is irrelevant.
Gives the following output (note the order of the elements compared in which order they were written in the list() syntax):
Example #5 list() with keys
As of PHP 7.1.0 list() can now also contain explicit keys, which can be given as arbitrary expressions. Mixing of integer and string keys is allowed; however, elements with and without keys cannot be mixed.
The above example will output:
- each() - Return the current key and value pair from an array and advance the array cursor
- array() - Create an array
- extract() - Import variables into the current symbol table from an array
Improve This Page
User contributed notes 25 notes.

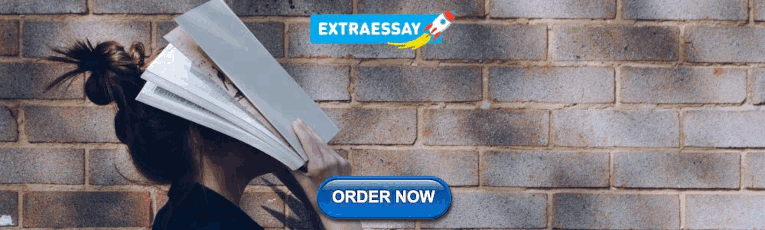
IMAGES
VIDEO
COMMENTS
Now, I'm aware that I can simply perform the assignment before the return statement, but this is just a contrived example, and I'm curious as to the feasibility. Edit : Providing a little more clarification.
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic, array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression.For example:
Use PHP assignment operator ( =) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
Values are returned by using the optional return statement. Any type may be returned, including arrays and objects. This causes the function to end its execution immediately and pass control back to the line from which it was called. See return for more information. If the return is omitted the value null will be returned.
I haven't seen anyone note method chaining in PHP5. When an object is returned by a method in PHP5 it is returned by default as a reference, and the new Zend Engine 2 allows you to chain method calls from those returned objects.
PHP User Defined Functions. Besides the built-in PHP functions, it is possible to create your own functions. A function is a block of statements that can be used repeatedly in a program. A function will not execute automatically when a page loads. A function will be executed by a call to the function.
This tutorial will teach you how to use the PHP Ternary Operator to make the code shorter and more readable. ... If it's true, the right-hand expression returns the value1; otherwise, it returns the value2. Second, PHP assigns the result of the right-hand expression to ... Assignment Operators; Comparison Operators; AND (&&) Operator; OR ...
PHP Coding Standards Fixer. Fork me on GitHub. PHP Coding Standards Fixer. 15 Keys Accelerate (v3.56.1) edition Install now Rule return_assignment ¶ Local, dynamic and directly referenced variables should not be assigned and directly returned by a function or method. ...
In PHP, the reference assignment operator (=&) is used to create a new variable as an alias to an existing spot in memory. In other words, the reference assignment operator (=&) creates two variable names which point to the same value. So, changes to one variable will affect the other, without having to copy the existing data.
PHP Strings and Variables. Variable Reassignment. The word variable comes from the latin variāre which means "to make changeable." This is an apt name because the value assigned to a variable can change. ... During variable assignment or reassignment, the variable on the left of the assignment operator is treated as a variable (named ...
Return statement are used to terminate the execution of a function and return control to the calling function. Return statement can also return a value to the calling function. Return is a language construct, it is not a function, hence parentheses are not required to surround the arguments. If no arguments are provided NULL is returned.
An operator takes one or more values, known as operands, and performs a specific operation on them. For example, the + operator adds two numbers and returns the sum of them. PHP supports many kinds of operators: Arithmetic Operators. Assignment Operators. Bitwise Operators. Comparison Operators.
return returns program control to the calling module. Execution resumes at the expression following the called module's invocation. If called from within a function, the return statement immediately ends execution of the current function, and returns its argument as the value of the function call. return also ends the execution of an eval ...
PHP All Exercises & Assignments. Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database. Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
The return keyword ends a function and, optionally, uses the result of an expression as the return value of the function. If return is used outside of a function, it stops PHP code in the file from running. If the file was included using include, include_once, require or require_once, the result of the expression is used as the return value of ...
In the months following return-to-office mandates, an increased number of senior employees departed Apple, Microsoft and SpaceX, often to work for competitors.
Zoo Atlanta will return Lun Lun, Yang Yang and their 7-year-old twins Ya Lun and Xi Lun to China late this year, another chapter of "panda diplomacy."
Ever since the first return-to-office mandates emerged in 2021, managers have grappled with the delicate task of balancing organizational policies with the preferences of their employees. The ...
Mr. Gantz, who leads the National Unity party, said he would give Mr. Netanyahu until Jun. 8 — about three weeks — to reach an agreement in Israel's war cabinet on a six-point plan to bring ...
Baltimore bridge collapse. How it happened: Baltimore's Francis Scott Key Bridge collapsed after being hit by a cargo ship.The container ship lost power shortly before hitting the bridge ...
why it may returns 1? It is not returning 1 but the actual value that is 'test' but since this value is assigned properly because this is not NULL, false or empty. the if statement evaluates to true.
Houston area could face more power issues as heat returns this week. High temperatures are likely to soar into the low 90s. By Timothy Malcolm May 18, 2024.
Doing a normal (not by reference) assignment with a reference on the right side does not turn the left side into a reference, but references inside arrays are preserved in these normal assignments. This also applies to function calls where the array is passed by value.
New York Yankees third baseman DJ LeMahieu appears ready to resume a rehab assignment this week after getting live at-bats at the team's complex in Tampa, Fla., on Tuesday. Per the New York Post ...
San Diego Padres All-Star starting pitcher Joe Musgrove feels much better as he's recovering from his elbow injury. In a recent interview with the media, includ
So it can be assumed PHP (in any of the expression) will first assign the return value of the function to the variable and then compare. Share Improve this answer
class. Basic class definitions begin with the keyword class, followed by a class name, followed by a pair of curly braces which enclose the definitions of the properties and methods belonging to the class.. The class name can be any valid label, provided it is not a PHP reserved word.A valid class name starts with a letter or underscore, followed by any number of letters, numbers, or underscores.
A U.S. district court judge on Thursday scheduled U.S. Rep. Henry Cuellar's trial for a spring start date, sooner than his defense team asked for.
Sightings of the aurora borealis are unlikely in the U.K. this weekend, but the northern lights could return on Monday, forecasters said. By Isabella Kwai Reporting from London, where she failed ...
Like array(), this is not really a function, but a language construct. list() is used to assign a list of variables in one operation. Strings can not be unpacked and list() expressions can not be completely empty.. Note: . Before PHP 7.1.0, list() only worked on numerical arrays and assumes the numerical indices start at 0.