C Programming/String manipulation
A string in C is merely an array of characters. The length of a string is determined by a terminating null character: '\0' . So, a string with the contents, say, "abc" has four characters: 'a' , 'b' , 'c' , and the terminating null ( '\0' ) character.
The terminating null character has the value zero.
- 1.1 backslash escapes
- 1.2 Wide character strings
- 1.3 Character encodings
- 2.1.1 The strcat function
- 2.1.2 The strchr function
- 2.1.3 The strcmp function
- 2.1.4 The strcpy function
- 2.1.5 The strlen function
- 2.1.6 The strncat function
- 2.1.7 The strncmp function
- 2.1.8 The strncpy function
- 2.1.9 The strrchr function
- 2.2.1.1 The memcpy function
- 2.2.1.2 The memmove function
- 2.2.2.1 The memcmp function
- 2.2.2.2 The strcoll and strxfrm functions
- 2.2.3.1 The memchr function
- 2.2.3.2 The strcspn, strpbrk, and strspn functions
- 2.2.3.3 The strstr function
- 2.2.3.4 The strtok function
- 2.2.4.1 The memset function
- 2.2.4.2 The strerror function
- 3.1 Exercises
- 4 References
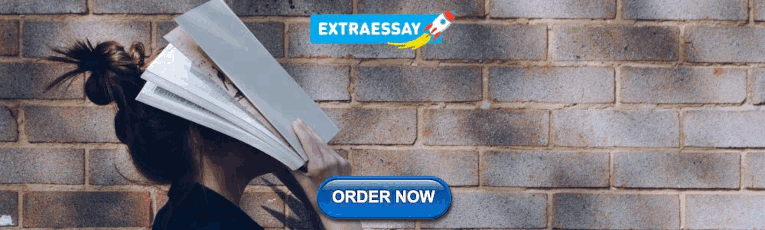
Syntax [ edit | edit source ]
In C, string constants (literals) are surrounded by double quotes ( " ), e.g. "Hello world!" and are compiled to an array of the specified char values with an additional null terminating character (0-valued) code to mark the end of the string. The type of a string constant is char [] .
backslash escapes [ edit | edit source ]
String literals may not directly in the source code contain embedded newlines or other control characters, or some other characters of special meaning in string.
To include such characters in a string, the backslash escapes may be used, like this:
Wide character strings [ edit | edit source ]
C supports wide character strings, defined as arrays of the type wchar_t , 16-bit (at least) values. They are written with an L before the string like this
This feature allows strings where more than 256 different possible characters are needed (although also variable length char strings can be used). They end with a zero-valued wchar_t . These strings are not supported by the <string.h> functions. Instead they have their own functions, declared in <wchar.h> .
Character encodings [ edit | edit source ]
What character encoding the char and wchar_t represent is not specified by the C standard, except that the value 0x00 and 0x0000 specify the end of the string and not a character. It is the input and output code which are directly affected by the character encoding. Other code should not be too affected. The editor should also be able to handle the encoding if strings shall be able to written in the source code.
There are three major types of encodings:
- One byte per character. Normally based on ASCII. There is a limit of 255 different characters plus the zero termination character.
- Variable length char strings, which allows many more than 255 different characters. Such strings are written as normal char -based arrays. These encodings are normally ASCII-based and examples are UTF-8 or Shift JIS .
- Wide character strings. They are arrays of wchar_t values. UTF-16 is the most common such encoding, and it is also variable-length, meaning that a character can be two wchar_t .
The <string.h> Standard Header [ edit | edit source ]
Because programmers find raw strings cumbersome to deal with, they wrote the code in the <string.h> library. It represents not a concerted design effort but rather the accretion of contributions made by various authors over a span of years.
First, three types of functions exist in the string library:
- the mem functions manipulate sequences of arbitrary characters without regard to the null character;
- the str functions manipulate null-terminated sequences of characters;
- the strn functions manipulate sequences of non-null characters.
The more commonly-used string functions [ edit | edit source ]
The nine most commonly used functions in the string library are:
- strcat - concatenate two strings
- strchr - string scanning operation
- strcmp - compare two strings
- strcpy - copy a string
- strlen - get string length
- strncat - concatenate one string with part of another
- strncmp - compare parts of two strings
- strncpy - copy part of a string
- strrchr - string scanning operation
Other functions, such as strlwr (convert to lower case), strrev (return the string reversed), and strupr (convert to upper case) may be popular; however, they are neither specified by the C Standard nor the Single Unix Standard. It is also unspecified whether these functions return copies of the original strings or convert the strings in place.
The strcat function [ edit | edit source ]
Some people recommend using strncat() or strlcat() instead of strcat, in order to avoid buffer overflow.
The strcat() function shall append a copy of the string pointed to by s2 (including the terminating null byte) to the end of the string pointed to by s1 . The initial byte of s2 overwrites the null byte at the end of s1 . If copying takes place between objects that overlap, the behavior is undefined. The function returns s1 .
This function is used to attach one string to the end of another string. It is imperative that the first string ( s1 ) have the space needed to store both strings.
Before calling strcat() , the destination must currently contain a null terminated string or the first character must have been initialized with the null character (e.g. penText[0] = '\0'; ).
The following is a public-domain implementation of strcat :
The strchr function [ edit | edit source ]
The strchr() function shall locate the first occurrence of c (converted to a char ) in the string pointed to by s . The terminating null byte is considered to be part of the string. The function returns the location of the found character, or a null pointer if the character was not found.
This function is used to find certain characters in strings.
At one point in history, this function was named index . The strchr name, however cryptic, fits the general pattern for naming.
The following is a public-domain implementation of strchr :
The strcmp function [ edit | edit source ]
A rudimentary form of string comparison is done with the strcmp() function. It takes two strings as arguments and returns a value less than zero if the first is lexographically less than the second, a value greater than zero if the first is lexographically greater than the second, or zero if the two strings are equal. The comparison is done by comparing the coded (ascii) value of the characters, character by character.
This simple type of string comparison is nowadays generally considered unacceptable when sorting lists of strings. More advanced algorithms exist that are capable of producing lists in dictionary sorted order. They can also fix problems such as strcmp() considering the string "Alpha2" greater than "Alpha12". (In the previous example, "Alpha2" compares greater than "Alpha12" because '2' comes after '1' in the character set.) What we're saying is, don't use this strcmp() alone for general string sorting in any commercial or professional code.
The strcmp() function shall compare the string pointed to by s1 to the string pointed to by s2 . The sign of a non-zero return value shall be determined by the sign of the difference between the values of the first pair of bytes (both interpreted as type unsigned char ) that differ in the strings being compared. Upon completion, strcmp() shall return an integer greater than, equal to, or less than 0, if the string pointed to by s1 is greater than, equal to, or less than the string pointed to by s2 , respectively.
Since comparing pointers by themselves is not practically useful unless one is comparing pointers within the same array, this function lexically compares the strings that two pointers point to.
This function is useful in comparisons, e.g.
The collating sequence used by strcmp() is equivalent to the machine's native character set. The only guarantee about the order is that the digits from '0' to '9' are in consecutive order.
The following is a public-domain implementation of strcmp :
The strcpy function [ edit | edit source ]
Some people recommend always using strncpy() instead of strcpy, to avoid buffer overflow.
The strcpy() function shall copy the C string pointed to by s2 (including the terminating null byte) into the array pointed to by s1 . If copying takes place between objects that overlap, the behavior is undefined. The function returns s1 . There is no value used to indicate an error: if the arguments to strcpy() are correct, and the destination buffer is large enough, the function will never fail.
Important: You must ensure that the destination buffer ( s1 ) is able to contain all the characters in the source array, including the terminating null byte. Otherwise, strcpy() will overwrite memory past the end of the buffer, causing a buffer overflow, which can cause the program to crash, or can be exploited by hackers to compromise the security of the computer.
The following is a public-domain implementation of strcpy :
The strlen function [ edit | edit source ]
The strlen() function shall compute the number of bytes in the string to which s points, not including the terminating null byte. It returns the number of bytes in the string. No value is used to indicate an error.
The following is a public-domain implementation of strlen :
Note how the line
declares and initializes a pointer p to an integer constant, i.e. p cannot change the value it points to.
The strncat function [ edit | edit source ]
The strncat() function shall append not more than n bytes (a null byte and bytes that follow it are not appended) from the array pointed to by s2 to the end of the string pointed to by s1 . The initial byte of s2 overwrites the null byte at the end of s1 . A terminating null byte is always appended to the result. If copying takes place between objects that overlap, the behavior is undefined. The function returns s1 .
The following is a public-domain implementation of strncat :
The strncmp function [ edit | edit source ]
The strncmp() function shall compare not more than n bytes (bytes that follow a null byte are not compared) from the array pointed to by s1 to the array pointed to by s2 . The sign of a non-zero return value is determined by the sign of the difference between the values of the first pair of bytes (both interpreted as type unsigned char ) that differ in the strings being compared. See strcmp for an explanation of the return value.
This function is useful in comparisons, as the strcmp function is.
The following is a public-domain implementation of strncmp :
The strncpy function [ edit | edit source ]
The strncpy() function shall copy not more than n bytes (bytes that follow a null byte are not copied) from the array pointed to by s2 to the array pointed to by s1 . If copying takes place between objects that overlap, the behavior is undefined. If the array pointed to by s2 is a string that is shorter than n bytes, null bytes shall be appended to the copy in the array pointed to by s1 , until n bytes in all are written. The function shall return s1; no return value is reserved to indicate an error.
It is possible that the function will not return a null-terminated string, which happens if the s2 string is longer than n bytes.
The following is a public-domain version of strncpy :
The strrchr function [ edit | edit source ]
The strrchr function is similar to the strchr function, except that strrchr returns a pointer to the last occurrence of c within s instead of the first.
The strrchr() function shall locate the last occurrence of c (converted to a char ) in the string pointed to by s . The terminating null byte is considered to be part of the string. Its return value is similar to strchr 's return value.
At one point in history, this function was named rindex . The strrchr name, however cryptic, fits the general pattern for naming.
The following is a public-domain implementation of strrchr :
The less commonly-used string functions [ edit | edit source ]
The less-used functions are:
- memchr - Find a byte in memory
- memcmp - Compare bytes in memory
- memcpy - Copy bytes in memory
- memmove - Copy bytes in memory with overlapping areas
- memset - Set bytes in memory
- strcoll - Compare bytes according to a locale-specific collating sequence
- strcspn - Get the length of a complementary substring
- strerror - Get error message
- strpbrk - Scan a string for a byte
- strspn - Get the length of a substring
- strstr - Find a substring
- strtok - Split a string into tokens
- strxfrm - Transform string
Copying functions [ edit | edit source ]
The memcpy function [ edit | edit source ].
The memcpy() function shall copy n bytes from the object pointed to by s2 into the object pointed to by s1 . If copying takes place between objects that overlap, the behavior is undefined. The function returns s1 .
Because the function does not have to worry about overlap, it can do the simplest copy it can.
The following is a public-domain implementation of memcpy :
The memmove function [ edit | edit source ]
The memmove() function shall copy n bytes from the object pointed to by s2 into the object pointed to by s1 . Copying takes place as if the n bytes from the object pointed to by s2 are first copied into a temporary array of n bytes that does not overlap the objects pointed to by s1 and s2 , and then the n bytes from the temporary array are copied into the object pointed to by s1 . The function returns the value of s1 .
The easy way to implement this without using a temporary array is to check for a condition that would prevent an ascending copy, and if found, do a descending copy.
The following is a public-domain, though not completely portable, implementation of memmove :
Comparison functions [ edit | edit source ]
The memcmp function [ edit | edit source ].
The memcmp() function shall compare the first n bytes (each interpreted as unsigned char ) of the object pointed to by s1 to the first n bytes of the object pointed to by s2 . The sign of a non-zero return value shall be determined by the sign of the difference between the values of the first pair of bytes (both interpreted as type unsigned char ) that differ in the objects being compared.
The following is a public-domain implementation of memcmp :
The strcoll and strxfrm functions [ edit | edit source ]
size_t strxfrm(char *s1, const char *s2, size_t n);
The ANSI C Standard specifies two locale-specific comparison functions.
The strcoll function compares the string pointed to by s1 to the string pointed to by s2 , both interpreted as appropriate to the LC_COLLATE category of the current locale. The return value is similar to strcmp .
The strxfrm function transforms the string pointed to by s2 and places the resulting string into the array pointed to by s1 . The transformation is such that if the strcmp function is applied to the two transformed strings, it returns a value greater than, equal to, or less than zero, corresponding to the result of the strcoll function applied to the same two original strings. No more than n characters are placed into the resulting array pointed to by s1 , including the terminating null character. If n is zero, s1 is permitted to be a null pointer. If copying takes place between objects that overlap, the behavior is undefined. The function returns the length of the transformed string.
These functions are rarely used and nontrivial to code, so there is no code for this section.
Search functions [ edit | edit source ]
The memchr function [ edit | edit source ].
The memchr() function shall locate the first occurrence of c (converted to an unsigned char ) in the initial n bytes (each interpreted as unsigned char ) of the object pointed to by s . If c is not found, memchr returns a null pointer.
The following is a public-domain implementation of memchr :
The strcspn , strpbrk , and strspn functions [ edit | edit source ]
The strcspn function computes the length of the maximum initial segment of the string pointed to by s1 which consists entirely of characters not from the string pointed to by s2 .
The strpbrk function locates the first occurrence in the string pointed to by s1 of any character from the string pointed to by s2 , returning a pointer to that character or a null pointer if not found.
The strspn function computes the length of the maximum initial segment of the string pointed to by s1 which consists entirely of characters from the string pointed to by s2 .
All of these functions are similar except in the test and the return value.
The following are public-domain implementations of strcspn , strpbrk , and strspn :
The strstr function [ edit | edit source ]
The strstr() function shall locate the first occurrence in the string pointed to by haystack of the sequence of bytes (excluding the terminating null byte) in the string pointed to by needle . The function returns the pointer to the matching string in haystack or a null pointer if a match is not found. If needle is an empty string, the function returns haystack .
The following is a public-domain implementation of strstr :
The strtok function [ edit | edit source ]
A sequence of calls to strtok() breaks the string pointed to by s1 into a sequence of tokens, each of which is delimited by a byte from the string pointed to by delimiters . The first call in the sequence has s1 as its first argument, and is followed by calls with a null pointer as their first argument. The separator string pointed to by delimiters may be different from call to call.
The first call in the sequence searches the string pointed to by s1 for the first byte that is not contained in the current separator string pointed to by delimiters . If no such byte is found, then there are no tokens in the string pointed to by s1 and strtok() shall return a null pointer. If such a byte is found, it is the start of the first token.
The strtok() function then searches from there for a byte (or multiple, consecutive bytes) that is contained in the current separator string. If no such byte is found, the current token extends to the end of the string pointed to by s1 , and subsequent searches for a token shall return a null pointer. If such a byte is found, it is overwritten by a null byte, which terminates the current token. The strtok() function saves a pointer to the following byte, from which the next search for a token shall start.
Each subsequent call, with a null pointer as the value of the first argument, starts searching from the saved pointer and behaves as described above.
The strtok() function need not be reentrant. A function that is not required to be reentrant is not required to be thread-safe.
Because the strtok() function must save state between calls, and you could not have two tokenizers going at the same time, the Single Unix Standard defined a similar function, strtok_r() , that does not need to save state. Its prototype is this:
char *strtok_r(char *s, const char *delimiters, char **lasts);
The strtok_r() function considers the null-terminated string s as a sequence of zero or more text tokens separated by spans of one or more characters from the separator string delimiters . The argument lasts points to a user-provided pointer which points to stored information necessary for strtok_r() to continue scanning the same string.
In the first call to strtok_r() , s points to a null-terminated string, delimiters to a null-terminated string of separator characters, and the value pointed to by lasts is ignored. The strtok_r() function shall return a pointer to the first character of the first token, write a null character into s immediately following the returned token, and update the pointer to which lasts points.
In subsequent calls, s is a null pointer and lasts shall be unchanged from the previous call so that subsequent calls shall move through the string s , returning successive tokens until no tokens remain. The separator string delimiters may be different from call to call. When no token remains in s , a NULL pointer shall be returned.
The following public-domain code for strtok and strtok_r codes the former as a special case of the latter:
Miscellaneous functions [ edit | edit source ]
These functions do not fit into one of the above categories.
The memset function [ edit | edit source ]
The memset() function converts c into unsigned char , then stores the character into the first n bytes of memory pointed to by s .
The following is a public-domain implementation of memset :
The strerror function [ edit | edit source ]
This function returns a locale-specific error message corresponding to the parameter. Depending on the circumstances, this function could be trivial to implement, but this author will not do that as it varies.
The Single Unix System Version 3 has a variant, strerror_r , with this prototype:
int strerror_r(int errcode, char *buf, size_t buflen);
This function stores the message in buf , which has a length of size buflen .
Examples [ edit | edit source ]
To determine the number of characters in a string, the strlen() function is used:
Note that the amount of memory allocated for 'turkey' is one plus the sum of the lengths of the strings to be concatenated. This is for the terminating null character, which is not counted in the lengths of the strings.
Exercises [ edit | edit source ]
- The string functions use a lot of looping constructs. Is there some way to portably unravel the loops?
- What functions are possibly missing from the library as it stands now?
References [ edit | edit source ]
- A Little C Primer/C String Function Library
- C++ Programming/Code/IO/Streams/string
- Because so many functions in the standard string.h library are vulnerable to buffer overflow errors, some people recommend avoiding the string.h library and "C style strings" and instead using a dynamic string API, such as the ones listed in the String library comparison .
- There's a tiny public domain concat() function, which will allocate memory and safely concatenate any number of strings in portable C/C++ code
- Book:C Programming
Navigation menu
Length of C String – How to Find the Size of a String in C
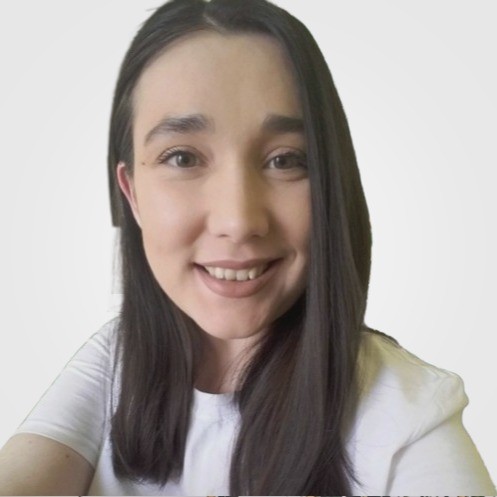
When working with strings in C, you need to know how to find their length.
Finding the length of a string in C is essential for many reasons.
You may need to perform string manipulation, and many string manipulation functions require the length of the string as an argument. You may also need to validate user input, compare two strings, or manage and allocate memory dynamically.
In this this article, you will learn different ways to find the length of a string in C.
What are Strings in C?
Unlike other programming languages, C doesn’t have a built-in string data type.
Instead, strings in C are arrays of characters that have a character called the null terminator \0 at the end.
There are many ways to create a string in C. Here is an example of one way:
In the code above, I created a character array named greeting using the char data type followed by square brackets, [] .
I then assigned the the string Hello which is surrounded by double quotes to greeting .
In this example, the size of the array is not specified explicitly – the size is determined by the size of the string assigned to it. Also, the null terminator, \0, is automatically added to the end of the string.
What is the string.h header file in C?
The string.h header file provides functions for manipulating and working with strings.
It contains functions that complete tasks such as copying and concatenating. It also provides functions for finding the length of a string, such as strlen() which you will learn how to use in the following section.
To use functions from string.h , you need to include it at the beginning of your file like this:
How to Find the Length of a String in C Using the strlen() Function
The strlen() function is defined in the string.h header file, and is used to find the length of a string.
Let’s take the following example:
In the example above, I first included the stdio.h header file to be able to use input/output functions such as printf() . I also included the string.h header file so I could use the strlen() function.
Inside the main() function, I created a greeting array and stored the string Hello .
Then, I called the strlen() function and passed greeting as the argument – this is the string I want to find the length of.
Lastly, I used the value returned in length and printed it using the printf() function.
Note that the strlen() function returns the number of characters in the string excluding the null terminator ( \0 ).
How to Find the Length of a String in C Using the sizeof() Operator
Another way to find the length of a string in C is using the sizeof() operator.
The sizeof() operator returns the total size in bytes of a string.
Let's take the following example:
In the following example, sizeof(greeting) returns the entire size of the greeting array in bytes – including the null operator, \0 .
This is not always very helpful.
To exclude this character, you need to subtract one from the total size :
Although the sizeof() operator doesn’t require you to include the string.h header file like strlen() does, it returns the total size of the array and not the length of the string.
The total size of the array includes the null terminator, \0 , whereas the length of the string is the number of characters before the null terminator.
How to Find the Length of a String in C Using a while Loop
Another way to find the length of a string is C is using a while loop.
The way this works is you keep iterating over the characters in a string until you reach the end of it and encounter the null terminator \0 .
Let's look at the following example:
Let’s break down how the loop works.
I initialized a counter variable, length , to 0 . This variable will store the length of the string.
The condition of the while loop, greeting[length] != '\0' , checks if the character at index length of the string is not equal to the null terminator \0 .
If it is not, the length variable is incremented and the loop continues and moves on to the next character in greeting .
The while loop iterates over greeting until it encounters \0 and then the iteration stops.
In this article, you learned how to find the length of a string in C.
You learned how to use the strlen() function, which returns the number of characters in the string excluding the null terminator.
You also learned how to use the sizeof() operator which does not always return the desired result as it includes the null terminator in the length.
Lastly, you learned how to use a while loop to find the length of a string. A loop counts the characters in the string until it reaches the null terminator.
Thank you for reading, and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
C Functions
C structures.
Strings are used for storing text/characters.
For example, "Hello World" is a string of characters.
Unlike many other programming languages, C does not have a String type to easily create string variables. Instead, you must use the char type and create an array of characters to make a string in C:
Note that you have to use double quotes ( "" ).
To output the string, you can use the printf() function together with the format specifier %s to tell C that we are now working with strings:
Access Strings
Since strings are actually arrays in C, you can access a string by referring to its index number inside square brackets [] .
This example prints the first character (0) in greetings :
Note that we have to use the %c format specifier to print a single character .
Modify Strings
To change the value of a specific character in a string, refer to the index number, and use single quotes :
Loop Through a String
You can also loop through the characters of a string, using a for loop:
And like we specified in the arrays chapter, you can also use the sizeof formula (instead of manually write the size of the array in the loop condition (i < 5) ) to make the loop more sustainable:
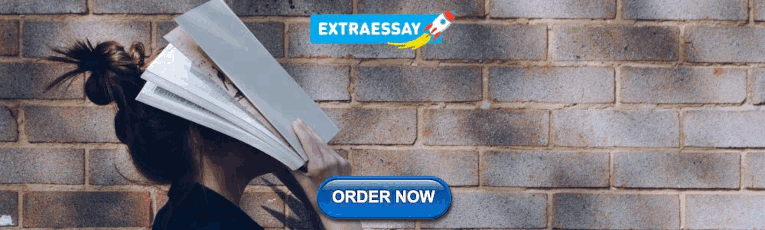
Another Way Of Creating Strings
In the examples above, we used a "string literal" to create a string variable. This is the easiest way to create a string in C.
You should also note that you can create a string with a set of characters. This example will produce the same result as the example in the beginning of this page:
Why do we include the \0 character at the end? This is known as the "null terminating character", and must be included when creating strings using this method. It tells C that this is the end of the string.
Differences
The difference between the two ways of creating strings, is that the first method is easier to write, and you do not have to include the \0 character, as C will do it for you.
You should note that the size of both arrays is the same: They both have 13 characters (space also counts as a character by the way), including the \0 character:
Real-Life Example
Use strings to create a simple welcome message:
C Exercises
Test yourself with exercises.
Fill in the missing part to create a "string" named greetings , and assign it the value "Hello".
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
cppreference.com
Array declaration.
Array is a type consisting of a contiguously allocated nonempty sequence of objects with a particular element type . The number of those objects (the array size) never changes during the array lifetime.
[ edit ] Syntax
In the declaration grammar of an array declaration, the type-specifier sequence designates the element type (which must be a complete object type), and the declarator has the form:
[ edit ] Explanation
There are several variations of array types: arrays of known constant size, variable-length arrays, and arrays of unknown size.
[ edit ] Arrays of constant known size
If expression in an array declarator is an integer constant expression with a value greater than zero and the element type is a type with a known constant size (that is, elements are not VLA) (since C99) , then the declarator declares an array of constant known size:
Arrays of constant known size can use array initializers to provide their initial values:
[ edit ] Arrays of unknown size
If expression in an array declarator is omitted, it declares an array of unknown size. Except in function parameter lists (where such arrays are transformed to pointers) and when an initializer is available, such type is an incomplete type (note that VLA of unspecified size, declared with * as the size, is a complete type) (since C99) :
[ edit ] Qualifiers
[ edit ] assignment.
Objects of array type are not modifiable lvalues , and although their address may be taken, they cannot appear on the left hand side of an assignment operator. However, structs with array members are modifiable lvalues and can be assigned:
[ edit ] Array to pointer conversion
Any lvalue expression of array type, when used in any context other than
- as the operand of the address-of operator
- as the operand of sizeof
- as the operand of typeof and typeof_unqual (since C23)
- as the string literal used for array initialization
undergoes an implicit conversion to the pointer to its first element. The result is not an lvalue.
If the array was declared register , the behavior of the program that attempts such conversion is undefined.
When an array type is used in a function parameter list, it is transformed to the corresponding pointer type: int f ( int a [ 2 ] ) and int f ( int * a ) declare the same function. Since the function's actual parameter type is pointer type, a function call with an array argument performs array-to-pointer conversion; the size of the argument array is not available to the called function and must be passed explicitly:
[ edit ] Multidimensional arrays
When the element type of an array is another array, it is said that the array is multidimensional:
Note that when array-to-pointer conversion is applied, a multidimensional array is converted to a pointer to its first element, e.g., pointer to the first row:
[ edit ] Notes
Zero-length array declarations are not allowed, even though some compilers offer them as extensions (typically as a pre-C99 implementation of flexible array members ).
If the size expression of a VLA has side effects, they are guaranteed to be produced except when it is a part of a sizeof expression whose result doesn't depend on it:
[ edit ] References
- C23 standard (ISO/IEC 9899:2023):
- 6.7.6.2 Array declarators (p: TBD)
- C17 standard (ISO/IEC 9899:2018):
- 6.7.6.2 Array declarators (p: 94-96)
- C11 standard (ISO/IEC 9899:2011):
- 6.7.6.2 Array declarators (p: 130-132)
- C99 standard (ISO/IEC 9899:1999):
- 6.7.5.2 Array declarators (p: 116-118)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.5.4.2 Array declarators
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 16 January 2024, at 16:08.
- This page has been accessed 250,901 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Previous: Constructing Array Values , Up: Arrays [ Contents ][ Index ]
16.9 Arrays of Variable Length
In GNU C, you can declare variable-length arrays like any other arrays, but with a length that is not a constant expression. The storage is allocated at the point of declaration and deallocated when the block scope containing the declaration exits. For example:
(This uses some standard library functions; see String and Array Utilities in The GNU C Library Reference Manual .)
The length of an array is computed once when the storage is allocated and is remembered for the scope of the array in case it is used in sizeof .
Warning: don’t allocate a variable-length array if the size might be very large (more than 100,000), or in a recursive function, because that is likely to cause stack overflow. Allocate the array dynamically instead (see Dynamic Memory Allocation ).
Jumping or breaking out of the scope of the array name deallocates the storage. Jumping into the scope is not allowed; that gives an error message.
You can also use variable-length arrays as arguments to functions:
As usual, a function argument declared with an array type is really a pointer to an array that already exists. Calling the function does not allocate the array, so there’s no particular danger of stack overflow in using this construct.
To pass the array first and the length afterward, use a forward declaration in the function’s parameter list (another GNU extension). For example,
The int len before the semicolon is a parameter forward declaration , and it serves the purpose of making the name len known when the declaration of data is parsed.
You can write any number of such parameter forward declarations in the parameter list. They can be separated by commas or semicolons, but the last one must end with a semicolon, which is followed by the “real” parameter declarations. Each forward declaration must match a “real” declaration in parameter name and data type. ISO C11 does not support parameter forward declarations.
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively.
- Sort Elements in Lexicographical Order (Dictionary Order)
- Copy String Without Using strcpy()
Concatenate Two Strings
- Find the Length of a String
Remove all Characters in a String Except Alphabets
- Count the Number of Vowels, Consonants and so on
Find the Frequency of Characters in a String
C Tutorials
- String Examples in C Programming
- String Manipulations In C Programming Using Library Functions
- C Programming Strings
C Program to Find the Length of a String
To understand this example, you should have the knowledge of the following C programming topics:
As you know, the best way to find the length of a string is by using the strlen() function. However, in this example, we will find the length of a string manually.
Calculate Length of String without Using strlen() Function
Here, using a for loop, we have iterated over characters of the string from i = 0 to until '\0' (null character) is encountered. In each iteration, the value of i is increased by 1.
When the loop ends, the length of the string will be stored in the i variable.
Note: Here, the array s[] has 19 elements. The last element s[18] is the null character '\0' . But our loop does not count this character as it terminates upon encountering it.
Sorry about that.
Related Examples
Find ASCII Value of a Character
How to find the length of a string in C
New Courses Coming Soon
Join the waiting lists
A practical tutorial on how to find the length of a string in C
Use the strlen() function provided by the C standard library string.h header file.
This function will return the length of a string as an integer value.
Working example:
Here is how can I help you:
- COURSES where I teach everything I know
- CODING BOOTCAMP cohort course - next edition in 2025
- BOOKS 16 coding ebooks you can download for free on JS Python C PHP and lots more
- Follow me on X
- <cassert> (assert.h)
- <cctype> (ctype.h)
- <cerrno> (errno.h)
- C++11 <cfenv> (fenv.h)
- <cfloat> (float.h)
- C++11 <cinttypes> (inttypes.h)
- <ciso646> (iso646.h)
- <climits> (limits.h)
- <clocale> (locale.h)
- <cmath> (math.h)
- <csetjmp> (setjmp.h)
- <csignal> (signal.h)
- <cstdarg> (stdarg.h)
- C++11 <cstdbool> (stdbool.h)
- <cstddef> (stddef.h)
- C++11 <cstdint> (stdint.h)
- <cstdio> (stdio.h)
- <cstdlib> (stdlib.h)
- <cstring> (string.h)
- C++11 <ctgmath> (tgmath.h)
- <ctime> (time.h)
- C++11 <cuchar> (uchar.h)
- <cwchar> (wchar.h)
- <cwctype> (wctype.h)
Containers:
- C++11 <array>
- <deque>
- C++11 <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- C++11 <unordered_map>
- C++11 <unordered_set>
- <vector>
Input/Output:
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
Multi-threading:
- C++11 <atomic>
- C++11 <condition_variable>
- C++11 <future>
- C++11 <mutex>
- C++11 <thread>
- <algorithm>
- <bitset>
- C++11 <chrono>
- C++11 <codecvt>
- <complex>
- <exception>
- <functional>
- C++11 <initializer_list>
- <iterator>
- <limits>
- <locale>
- <memory>
- <new>
- <numeric>
- C++11 <random>
- C++11 <ratio>
- C++11 <regex>
- <stdexcept>
- <string>
- C++11 <system_error>
- C++11 <tuple>
- C++11 <type_traits>
- C++11 <typeindex>
- <typeinfo>
- <utility>
- <valarray>
class templates
- basic_string
- char_traits
- C++11 u16string
- C++11 u32string
- C++11 stold
- C++11 stoll
- C++11 stoul
- C++11 stoull
- C++11 to_string
- C++11 to_wstring
- string::~string
- string::string
member functions
- string::append
- string::assign
- C++11 string::back
- string::begin
- string::c_str
- string::capacity
- C++11 string::cbegin
- C++11 string::cend
- string::clear
- string::compare
- string::copy
- C++11 string::crbegin
- C++11 string::crend
- string::data
- string::empty
- string::end
- string::erase
- string::find
- string::find_first_not_of
- string::find_first_of
- string::find_last_not_of
- string::find_last_of
- C++11 string::front
- string::get_allocator
- string::insert
- string::length
- string::max_size
- string::operator[]
- string::operator+=
- string::operator=
- C++11 string::pop_back
- string::push_back
- string::rbegin
- string::rend
- string::replace
- string::reserve
- string::resize
- string::rfind
- C++11 string::shrink_to_fit
- string::size
- string::substr
- string::swap
member constants
- string::npos
non-member overloads
- getline (string)
- operator+ (string)
- operator<< (string)
- >/" title="operator>> (string)"> operator>> (string)
- relational operators (string)
- swap (string)
std:: string ::length
Return value, iterator validity, exception safety.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Properties of Array in C
- Length of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Jagged Array or Array of Arrays in C with Examples
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- How to pass an array by value in C ?
Variable Length Arrays (VLAs) in C
- What are the data types for which it is not possible to create an array?
- Strings in C
- Array of Strings in C
- C Library - <string.h>
- C String Functions
- What is the difference between single quoted and double quoted declaration of char array?
- Array C/C++ Programs
- String C/C++ Programs
In C, variable length arrays (VLAs) are also known as runtime-sized or variable-sized arrays. The size of such arrays is defined at run-time.
Variably modified types include variable-length arrays and pointers to variable-length arrays. Variably changed types must be declared at either block scope or function prototype scope.
Variable length arrays are a feature where we can allocate an auto array (on stack) of variable size. It can be used in a typedef statement. C supports variable-sized arrays from the C99 standard. For example, the below program compiles and runs fine in C.
Syntax of VLAs in C
Note: In C99 or C11 standards, there is a feature called flexible array members, which works the same as the above.
Example of Variable Length Arrays
The below example shows the implementation of variable length arrays in C program
Explanation: The above program illustrate how to create a variable size array in a function in C program. This size is passed as parameter and the variable array is created on the stack memory.
Please Login to comment...
Similar reads.
- C Array and String
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

17.10 — C-style strings
In lesson 17.7 -- Introduction to C-style arrays , we introduced C-style arrays, which allow us to define a sequential collection of elements:
In lesson 5.2 -- Literals , we defined a string as a collection of sequential characters (such as “Hello, world!”), and introduced C-style string literals. We also noted that the C-style string literal “Hello, world!” has type const char[14] (13 explicit characters plus 1 hidden null-terminator character).
If you hadn’t connected the dots before, it should be obvious now that C-style strings are just C-style arrays whose element type is char or const char !
Although C-style string literals are fine to use in our code, C-style string objects have fallen out of favor in modern C++ because they are hard to use and dangerous (with std::string and std::string_view being the modern replacements). Regardless, you may still run across uses of C-style string objects in older code, and we would be remiss not to cover them at all.
Therefore, in this lesson, we’ll take a look at the most important points regarding C-style string objects in modern C++.
Defining C-style strings
To define a C-style string variable, simply declare a C-style array variable of char (or const char / constexpr char ):
Remember that we need an extra character for the implicit null terminator.
When defining C-style strings with an initializer, we highly recommend omitting the array length and letting the compiler calculate the length. That way if the initializer changes in the future, you won’t have to remember to update the length, and there is no risk in forgetting to include an extra element to hold the null terminator.
C-style strings will decay
In lesson 17.8 -- C-style array decay , we discussed how C-style arrays will decay into a pointer in most circumstances. Because C-style strings are C-style arrays, they will decay -- C-style string literals decay into a const char* , and C-style string arrays decay into either a const char* or char* depending on whether the array is const. And when a C-style string decays into a pointer, the length of the string (encoded in the type information) is lost.
This loss of length information is the reason C-style strings have a null-terminator. The length of the string can be (inefficiently) regenerated by counting the number of elements between the start of the string and the null terminator. Alternatively, the string can be traversed by iterating from the start until we hit the null terminator.
Outputting a C-style string
When outputting a C-style string, std::cout outputs characters until it encounters the null terminator. This null terminator marks the end of the string, so that decayed strings (which have lost their length information) can still be printed.
If you try to print a string that does not have a null terminator (e.g. because the null-terminator was overwritten somehow), the result will be undefined behavior. The most likely outcome in this case will be that all the characters in the string are printed, and then it will just keep printing everything in adjacent memory slots (interpreted as a character) until it happens to hit a byte of memory containing a 0 (which will be interpreted as a null terminator)!
Inputting C-style strings
Consider the case where we are asking the user to roll a die as many times as they wish and enter the numbers rolled without spaces (e.g. 524412616 ). How many characters will the user enter? We have no idea.
Because C-style strings are fixed-size arrays, the solution is to declare an array larger than we are ever likely to need:
Prior to C++20, std::cin >> rolls would extract as many characters as possible to rolls (stopping at the first non-leading whitespace). Nothing is stopping the user from entering more than 254 characters (either unintentionally, or maliciously). And if that happens, the user’s input will overflow the rolls array and undefined behavior will result.
Key insight
Array overflow or buffer overflow is a computer security issue that occurs when more data is copied into storage than the storage can hold. In such cases, the memory just beyond the storage will be overwritten, leading to undefined behavior. Malicious actors can potentially exploit such flaws to overwrite the contents of memory, hoping to change the program’s behavior in some advantageous way.
In C++20, operator>> was changed so that it only works for inputting non-decayed C-style strings. This allows operator>> to extract only as many characters as the C-style string’s length will allow, preventing overflow. But this also means you can no longer use operator>> to input to decayed C-style strings.
The recommended way of reading C-style strings using std::cin is as follows:
This call to cin.getline() will read up to 254 characters (including whitespace) into rolls . Any excess characters will be discarded. Because getline() takes a length, we can provide the maximum number of characters to accept. With a non-decayed array, this is easy -- we can use std::size() to get the array length. With a decayed array, we have to determine the length in some other way. And if we provide the wrong length, our program may malfunction or have security issues.
In modern C++, when storing inputted text from the user, it’s safer to use std::string , as std::string will adjust automatically to hold as many characters as needed.
Modifying C-style strings
One important point to note is that C-style strings follow the same rules as C-style arrays. This means you can initialize the string upon creation, but you can not assign values to it using the assignment operator after that!
This makes using C-style strings a bit awkward.
Since C-style strings are arrays, you can use the [] operator to change individual characters in the string:
This program prints:
Getting the length of an C-style string
Because C-style strings are C-style arrays, you can use std::size() (or in C++20, std::ssize() ) to get the length of the string as an array. There are two caveats here:
- This doesn’t work on decayed strings.
- Returns the actual length of the C-style array, not the length of the string.
An alternate solution is to use the strlen() function, which lives in the <cstring> header. strlen() will work on decayed arrays, and returns the length of the string being held, excluding the null terminator:
However, std::strlen() is slow, as it has to traverse through the whole array, counting characters until it hits the null terminator.
Other C-style string manipulating functions
Because C-style strings are the primary string type in C, the C language provides many functions for manipulating C-style strings. These functions have been inherited by C++ as part of the <cstring> header.
Here are a few of the most useful that you may see in older code:
- strlen() -- returns the length of a C-style string
- strcpy(), strncpy(), strcpy_s() -- overwrites one C-style string with another
- strcat(), strncat() -- Appends one C-style string to the end of another
- strcmp(), strncmp() -- Compares two C-style strings (returns 0 if equal)
Except for strlen() , we generally recommend avoiding these.
Avoid non-const C-style string objects
Unless you have a specific, compelling reason to use non-const C-style strings, they are best avoided, as they are awkward to work with and are prone to overruns, which will cause undefined behavior (and are potential security issues).
In the rare case that you do need to work with C-style strings or fixed buffer sizes (e.g. for memory-limited devices), we’d recommend using a well-tested 3rd party fixed-length string library designed for the purpose.
Best practice
Avoid non-const C-style string objects in favor of std::string .
Question #1
Write a function to print a C-style string character by character. Use a pointer and pointer arithmetic to step through each character of the string and print that character. Write a main function that tests the function with the string literal “Hello, world!”.
Show Solution
Question #2
Repeat quiz #1, but this time the function should print the string backwards.
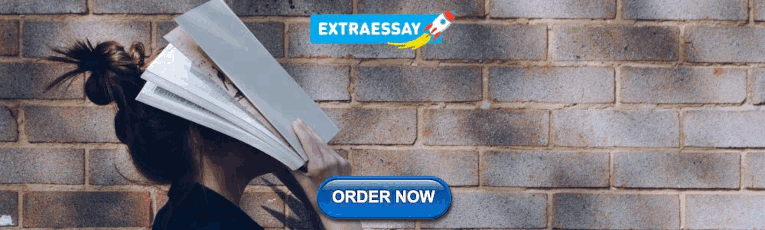
IMAGES
VIDEO
COMMENTS
In C you can have the storage decoupled from these special variables, called pointers. The example of wanting a variable length string is actually a good example of why would you want that: I want an entity that holds knowledge of where the storage is at; I want methods to allow me to change the storage size.
What is a string in C. String is a part of char array, terminated by \0. String functions stop reading the array when they encounter the first \0 character. Remember that strlen returns the number of characters before the first \0, so even if the array ends immediately after the first \0, string length is strictly less than the underlying array ...
The length of strings in C. The length of a string in C is just the number of characters in a word, without including the string terminator (despite it always being used to terminate strings). The string terminator is not accounted for when you want to find the length of a string. For example, the string freeCodeCamp has a length of 12 characters.
C strlen () The strlen() function takes a string as an argument and returns its length. The returned value is of type size_t (an unsigned integer type). It is defined in the <string.h> header file.
C Programming Strings. In C programming, a string is a sequence of characters terminated with a null character \0. For example: char c[] = "c string"; When the compiler encounters a sequence of characters enclosed in the double quotation marks, it appends a null character \0 at the end by default. Memory Diagram.
A string in C is merely an array of characters. The length of a string is determined by a terminating null character: '\0'. So, a string with the contents, ... (although also variable length char strings can be used). They end with a zero-valued wchar_t. These strings are not supported by the <string.h> functions.
C String Declaration Syntax. Declaring a string in C is as simple as declaring a one-dimensional array. Below is the basic syntax for declaring a string. char string_name [size]; In the above syntax string_name is any name given to the string variable and size is used to define the length of the string, i.e the number of characters strings will ...
In this this article, you will learn different ways to find the length of a string in C. What are Strings in C? Unlike other programming languages, C doesn't have a built-in string data type. Instead, strings in C are arrays of characters that have a character called the null terminator \0 at the end. There are many ways to create a string in C.
Hence, to display a String in C, you need to make use of a character array. The general syntax for declaring a variable as a String in C is as follows, char string_variable_name [array_size]; The classic Declaration of strings can be done as follow: char string_name[string_length] = "string"; The size of an array must be defined while declaring ...
Strings are used for storing text/characters. For example, "Hello World" is a string of characters. Unlike many other programming languages, C does not have a String type to easily create string variables. Instead, you must use the char type and create an array of characters to make a string in C: char greetings [] = "Hello World!";
Variable-length arrays. If expression is not an integer constant expression, the declarator is for an array of variable size.. Each time the flow of control passes over the declaration, expression is evaluated (and it must always evaluate to a value greater than zero), and the array is allocated (correspondingly, lifetime of a VLA ends when the declaration goes out of scope).
In GNU C, you can declare variable-length arrays like any other arrays, but with a length that is not a constant expression. The storage is allocated at the point of declaration and deallocated when the block scope containing the declaration exits. For example: (This uses some standard library functions; see String and Array Utilities in The ...
5. strchr() The strchr() function in C is a predefined function used for string handling. This function is used to find the first occurrence of a character in a string. Syntax. char *strchr(const char *str, int c); Parameters. str: specifies the pointer to the null-terminated string to be searched in. ch: specifies the character to be searched for. Here, str is the string and ch is the ...
printf("Length of the string: %d", i); return 0; Output. Here, using a for loop, we have iterated over characters of the string from i = 0 to until '\0' (null character) is encountered. In each iteration, the value of i is increased by 1. When the loop ends, the length of the string will be stored in the i variable.
char[25] lets you store C strings of length between zero and 24, inclusive (one character is needed for '\0' terminator). You can use one of two solutions: Use a flexible array member, or; Use a pointer. The first solution lets you keep the name together with the rest of the struct, but you would not be able to make arrays of these structs:. struct person{ int c; char name[]; };
A practical tutorial on how to find the length of a string in C. Use the strlen() function provided by the C standard library string.h header file. char name [7] = "Flavio"; strlen (name); This function will return the length of a string as an integer value. Working example:
Returns the length of the string, in terms of bytes. This is the number of actual bytes that conform the contents of the string, which is not necessarily equal to its storage capacity. Note that string objects handle bytes without knowledge of the encoding that may eventually be used to encode the characters it contains. Therefore, the value returned may not correspond to the actual number of ...
In C, variable length arrays (VLAs) are also known as runtime-sized or variable-sized arrays. The size of such arrays is defined at run-time. Variably modified types include variable-length arrays and pointers to variable-length arrays. Variably changed types must be declared at either block scope or function prototype scope.
Many C++ std::string implementations now use a "Small String Optimization". In pseudo-code: struct string { Int32 length union { char[12] shortString struct { char* longerString Int32 heapReservedSpace } } } The idea is that string up to 12 characters are stored in the shortString array. The entire string will be contiguous and use only a ...
In C++20, operator>> was changed so that it only works for inputting non-decayed C-style strings. This allows operator>> to extract only as many characters as the C-style string's length will allow, preventing overflow. But this also means you can no longer use operator>> to input to decayed C-style strings.. The recommended way of reading C-style strings using std::cin is as follows:
Please don't ever use unsafe things like scanf("%s") or my personal non-favourite, gets() - there's no way to prevent buffer overflows for things like that.. You can use a safer input method such as: #include <stdio.h> #include <string.h> #define OK 0 #define NO_INPUT 1 #define TOO_LONG 2 static int getLine (char *prmpt, char *buff, size_t sz) { int ch, extra; // Get line with buffer overrun ...