Understanding the Event Loop, the Task Queue, and the Microtask Queue in JavaScript
Luis Paredes
Jun 4, 2023
When working with JavaScript one of the concepts that confuses the most is the event loop mechanism and how it interacts with Javascript's internal data structures involved in the execution process.
In this article, we'll examine all of the concepts necessary to fully grok the mechanism and see how knowing this can help us avoid common pitfalls and get to the root cause of some bugs more efficiently.
Let's get started!
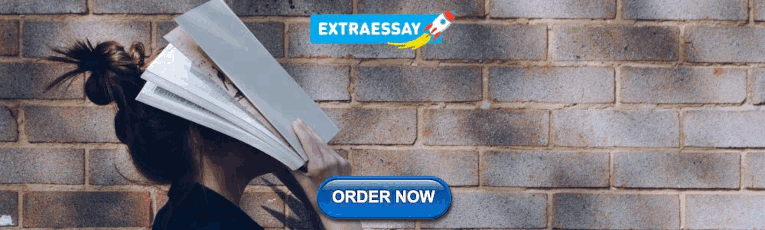
Concurrency in JavaScript
Because of the single threaded nature of Javascript, the language runtime cannot run several process in parallel 1 but rather relies on a clever concurrency model to be able to handle several operations in a non-blocking manner.
This model relies on the use of several internal queues that hold deferred items for execution after all the synchronous code has been handled.
Let's take a look at the most important queues, how they behave, as well as other important internal data structures to understand how it all fits together.
JavaScript internal data structures
The call stack.
The call stack is an essential concept in JavaScript's execution model. It serves as a memory structure that keeps track of function calls during code execution. Whenever a function is invoked, a new frame is created and added to the top of the call stack. This frame contains information about the function, including its arguments and local variables.
As the function executes, it may call other functions, which in turn create new frames and are stacked on top of the previous ones. This stacking behavior forms a hierarchical structure, resembling a stack of plates. When a function finishes executing, its frame is popped off the call stack, and the control returns to the function that invoked it.
The call stack operates on a Last-In-First-Out (LIFO) principle. The most recently added function is always at the top of the stack and is the one currently executing. This sequential behavior ensures that functions are executed in the order they are called, preventing concurrent execution and guaranteeing the integrity of the program's state.
Understanding the call stack is crucial for debugging JavaScript code. When an error occurs, the call stack provides valuable information about the chain of function calls that led to the error, enabling developers to identify the source of the problem. By examining the call stack, developers can trace the execution flow and understand how functions interact with each other, aiding in the resolution of errors and the overall comprehension of program behavior.
The task queue, also known as the macrotask queue (to diferentiate it from the microtask queue) or callback queue, is another crucial component in JavaScript's event-driven architecture. It is responsible for storing some of the tasks that are executed asynchronously, ensuring non-blocking behavior and efficient handling of operations that may take longer to complete.
When asynchronous operations, such as a DOM event, a setTimeout callback, or an AJAX request are encountered, the corresponding task is added to the task queue.
The task queue follows a First-In-First-Out (FIFO) order, meaning that tasks are executed in the order they were added to the queue. This ensures that tasks are processed sequentially, maintaining the integrity of the program's state and avoiding race conditions.
The task queue plays a vital role in managing asynchronous operations in JavaScript. By offloading time-consuming tasks to the task queue, JavaScript can continue executing other operations without waiting for the completion of each individual task. This allows for smoother user experiences, as the browser remains responsive even during heavy processing or long-running tasks.
It's worth noting that while the task queue is commonly referred to as the macrotask queue, it is also sometimes called the callback queue. This is because many asynchronous operations in JavaScript involve providing a callback function that will be executed when the task is ready to be processed. Hence, the task queue can be seen as a queue of callbacks waiting to be executed.
Microtask queue
The microtask queue is a critical part of JavaScript's asynchronous mechanism, working in tandem with the task queue. It is responsible for handling microtasks, which typically include promises and Mutation Observers.
Microtasks have a higher precedence than tasks in the execution. This means that when the call stack is empty and JavaScript is ready to process new tasks, it first checks the microtask queue. If there are any microtasks waiting to be executed, they take precedence and are processed before moving on to the next task in the task queue.
One important aspect to note is that even if new microtasks are added to the microtask queue while it is being processed, these newly added microtasks will still be executed before moving on to the next queue. This ensures that any subsequent microtasks generated during the execution of existing microtasks are given a chance to be processed without delay.
The microtask queue is especially useful for handling asynchronous operations that need to be executed immediately after the current task completes. For example, when a promise is resolved or rejected, the associated callbacks are scheduled as microtasks. This allows for precise control and sequencing of operations, ensuring that dependent actions occur promptly after a promise settles.
Other internal data structures
Depending on the context of execution (Node or the browser), there may be other queues involved in the concurrency mechanism of JavaScript, however, the 3 data structures we mentioned in this section are present regardless of the execution context.
In the next section, we'll see how the event loop makes use of these data structures.
The event loop
In JavaScript, the runtime model is managed through an event loop, which oversees the execution of code, handles events, and deal with queued sub-tasks.
The mechanism got its name because its implementation usually resembles the following pseudo-code:
When taking into account the internal data structures, explained in the previous section, the whole mechanism behaves like this:
- The event loop continuously checks the state of the call stack, microtask queue, and task queue.
- Take the next microtask from the microtask queue.
- Execute the associated callback.
- Repeat until all microtasks are processed.
- Take the next task from the task queue.
- Execute the task's associated code.
- Repeat the above steps until the task queue is empty.
- Once all microtasks and tasks are processed, return to the start of the event loop and repeat the process.
Let's take a look at the mechanism using an example:
Given the previous snippet, what will be printed to the console and why? —Take a moment to analyze the execution order based on the event loop mechanism before looking at the explanation below.
Explanation:
- The first statement to be processed will be console.log(1) . As it is a synchronous statement, it will be added directly to the call stack and the frame will be processed right away, hence 1 will be printed to the console.
- Next, we have setTimeout(() => console.log(2), 1000) , the setTimeout itself gets processed right away, but it's callback ( () => console.log(2) ) gets deferred to be executed at least 1000 ms (1s) after the setTimeout itself was executed (after all the synchronous code gets executed and after the microtasks have been handled since the setTimeout callback will get enqueued in the task queue).
- Then, we get to Promise.resolve().then(() => console.log(3)) , which similarly to the previous line, gets executed right away, but the then() callback gets deferred (this is a microtask that will be picked up as soon as as all the synchronous code gets processed)
- setTimeout(() => console.log(4), 0) gets processed just like the first setTimeout , notice that the deferral time is significantly smaller than the previous timeout ( 1000 ms vs 0 ms)
- Now we execute the final statement console.log(5) , which happens to be synchronous and as a result, we get 5 logged to the console.
- After all of the synchronous code has been handled, it's time to take a look at the queues. The first one to be processed would be the microtask queue, and as there's a then() callback there, this callback ( () => console.log(3) ) will be executed and as result, 3 will be logged to the console.
- Lastly, the event loop moves to the task queue because all of the messages in the microtask queues have been processed. If both of the timeouts had the same deferral time (or a sufficiently small deferral time difference 2 ), the callback corresponding to the first setTimout callback ( () => console.log(2) ) would get enqueued first and hence be processed first, however, since the deferral time difference is big enough, the second callback ( () => console.log(4) ) will get enqueued first and hence processed first and as a result, 4 will get printed before 2 .
As a programmer, I'm sure you're a familiar with the need to prevent infinite loops when working with loop operators such as for and while as well as when working with recursive functions.
Now that you understand the basics of the event loop in JavaScript, there's another patter you need to watch out for: infinite microtasks loops.
If we were to create and invoke a function like this:
JavaScript wouldn't have any issues processing the infinite execution because:
- any synchronous code would be processed before processing the callbacks in the task queue
- any microtasks would be processed before processing the messages in the task queue
- messages in other internal higher priority queues (rendering related queues in the browser for example) would be processed first
- even though each invocation enqueues a new callback, the callback will be processed in the next cycle of the event loop
However, if we were to change the asynchronous behavior for something like this:
we would be blocking the execution of the program because this time we're dealing with microtasks, and the event loop won't let go of the microtask queue until there are no messages left to process (which will never happen because each message adds a new one).
Of course, we won't be implementing something literally like the function above, however, we can fall into the pattern without realizing it, so keep this in mind when dealing with asynchronous code in JavaScript.
Other resources
If you'd like to dig deeper into the topic, here are a couple of talks I recommend to gain a deeper understanding of the event loop in the browser context :
- What the heck is the event loop anyway? | Philip Roberts | JSConf EU
- Jake Archibald on the web browser event loop, setTimeout, micro tasks, requestAnimationFrame, ...
By now, hopefully you have a solid grasp of the essentials of the event loop mechanism and its associated data structures.
By comprehending how the event loop interacts with these data structures, developers can avoid common pitfalls, debug errors more efficiently, and ensure the proper execution order of tasks and microtasks.
[1] Unless configuring a Node cluster or using Web workers.
[2] If we were to change 1000 by 1 in the example, Node will change the execution order becauese the deferral time difference is not big enough to prevent the first setTimout callback to get into the task queue before the other one. However, when executed in the browser, the 1ms difference is enough to make the first timeout callback end up being executed after the second, the reason being, that the browser handles more queues (related to DOM events and rendering) that modifify the actual deferral time (remember that the timeout time only guarantees the minimum deferral time, hence the actual deferral time can be much higher).
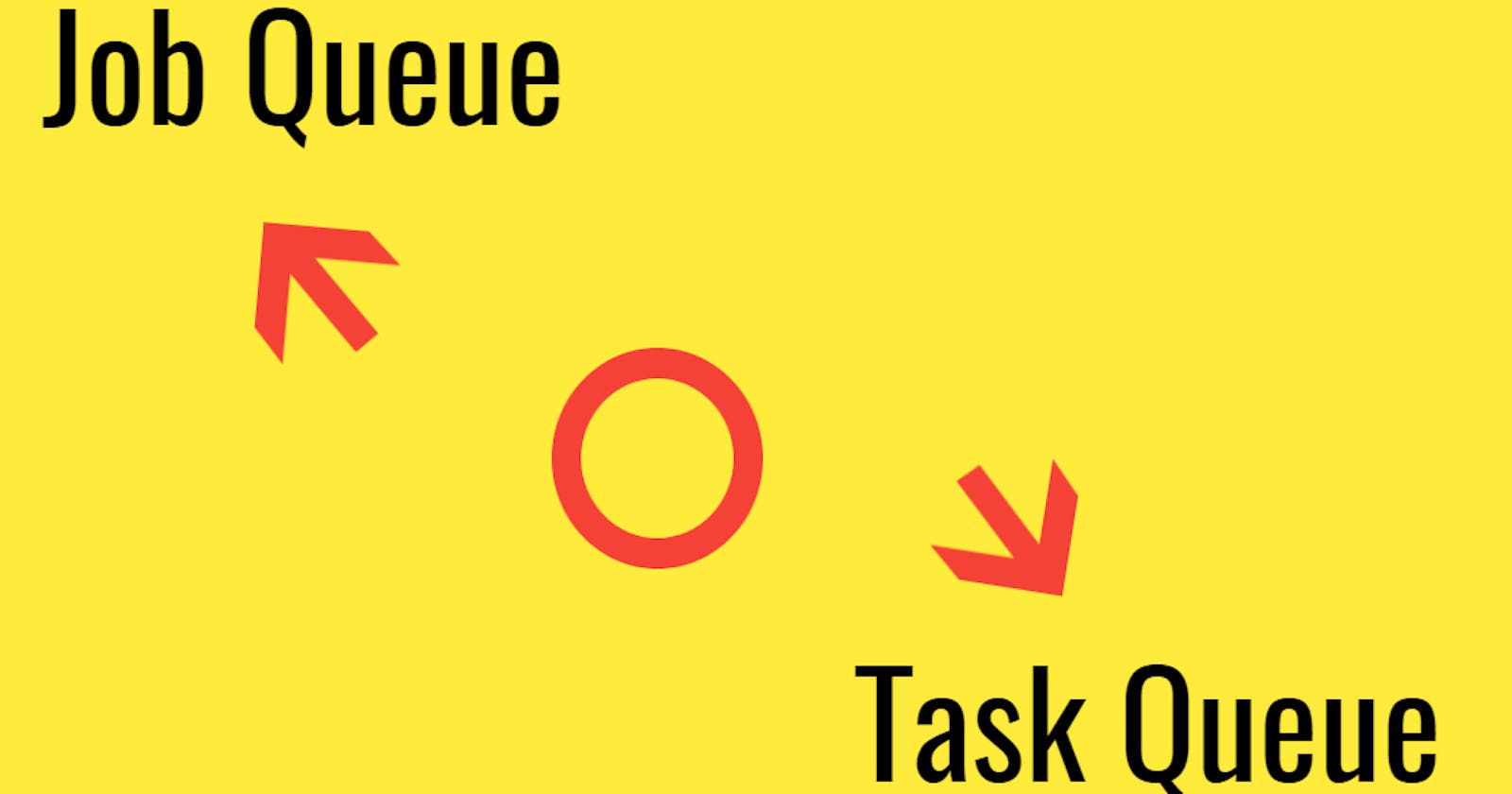
Task Queue and Job Queue - Deep dive into Javascript Event Loop Model
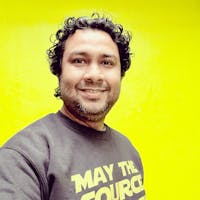
There is a famous saying,
Keep every promise you make and only make promises you can keep.
In my last post , I also made a promise that I shall write about the concept of Task and Job Queues. Here is the post on it. Interestingly, this post is mostly about How to keep a Promise and execute it .
Before we move further, I would like to clarify that I will not explain Promises as a concept here. There are plenty of good reads on it. Here is my personal favorite . However, this particular post is about understanding what goes under the hood when a promise gets executed? Along with it, we will also learn the difference between Task Queue and Job Queue.
To recall, some of it already explained here that there is Task Queue in the Event Loop Model. There is a Job Queue as well. Here are a few essential points to note:
- Not all the tasks are created of the same priority. There are macrotasks and microtasks.
- MacroTasks are called Tasks , and MicroTasks are called Jobs .
- Examples of macrotasks are setTimeout, setInterval, setImmediate, I/O tasks, etc.
- Examples of Microtasks are, Promises, processes.nextTick, etc.
- The Queue in Event Loop Model holds the Tasks(or the MacroTasks) called, TaskQueue.
- The Queue in Event Loop Model holds the Jobs(or the MicroTasks) called, JobQueue.
For example, Promises are in Job Queue, and the functions for setTimeOut are in TaskQueue.
With the explanation above, let us re-visit the Event Loop Model once more than last time .
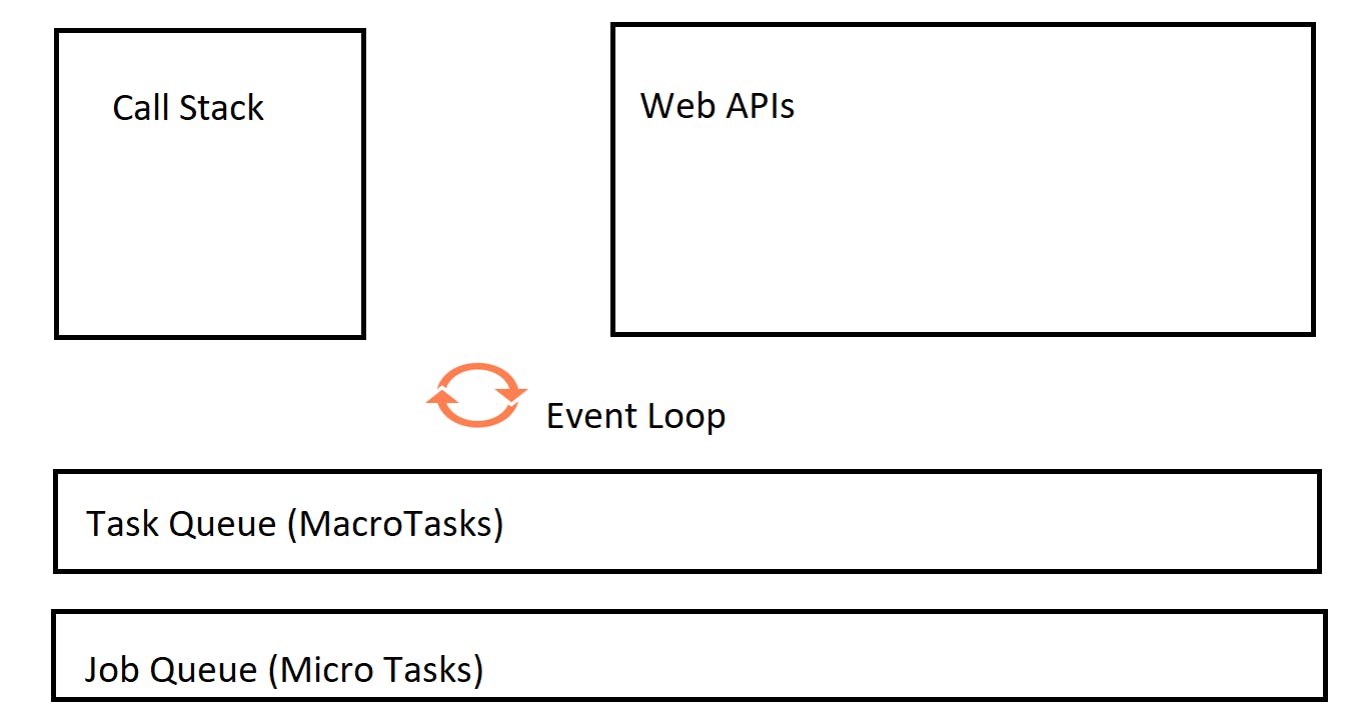
The obvious question would be, how does the event loop decide which queue to de-queue from and push to Call Stack when the Stack is empty? The answer depends on this logic(or, set of rules):
- For each loop of the 'event loop', one macrotask(Task) is completed out of the macrotask(Task) queue.
- Once that task is complete, the event loop visits the microtask(Job) queue. The entire microtask(Job) queue is completed before the 'event loop' looks into the next thing.
- At any point in time, if both the queues got entries, JobQueue gets higher precedence than TaskQueue.
Overall, the Event Loop got one more item to consider in its orchestration of Code Execution. Let us understand the above logic with a Code execution flow.
So the expected output is,
Let me explain Why?
- The function cartoon gets into the Call Stack.
- It executes the console log of the text Cartoon. The setTimeOut web API goes outside of the Call Stack in the following execution line, and the associated function tom gets placed into TaskQueue.
- In the following line of execution, we encounter a Promise. A callback of a promise gets a place in JobQueue. Hence the console log function execution on the promise goes to JobQueue.
- In the following execution line, the function jerry is pushed into the Stack and executed.
- Now the fun begins. We have one entry in TaskQueue and one in JobQueue. The Event Loop Model prioritizes all the Jobs in JobQueue over anything in TaskQueue. Hence the callback of the promise get to the Call Stack first, gets executed, and then the function tom gets to the Call Stack from TaskQueue and gets executed.
That's all about it. I hope you got the core concept. Now, Here is a puzzle for you. Let me know what the expected output of this code execution is? Feel free to post a comment with your answer.
Tips: Don't be upset if you hear any of your friends talking about another queue called Message Queue . They are just referring to Task Queue just by another name.
I hope you liked the post. Cheers!
Did you find this article valuable?
Support Tapas Adhikary by becoming a sponsor. Any amount is appreciated!
Tasks, microtasks, queues and schedules
When I told my colleague Matt Gaunt I was thinking of writing a piece on microtask queueing and execution within the browser's event loop, he said "I'll be honest with you Jake, I'm not going to read that". Well, I've written it anyway, so we're all going to sit here and enjoy it, ok?
Actually, if video's more your thing, Philip Roberts gave a great talk at JSConf on the event loop - microtasks aren't covered, but it's a great introduction to the rest. Anyway, on with the show…
Take this little bit of JavaScript:
In what order should the logs appear?
Clear log Run test
The correct answer: script start , script end , promise1 , promise2 , setTimeout , but it's pretty wild out there in terms of browser support.
Microsoft Edge, Firefox 40, iOS Safari and desktop Safari 8.0.8 log setTimeout before promise1 and promise2 - although it appears to be a race condition. This is really weird, as Firefox 39 and Safari 8.0.7 get it consistently right.
Why this happens
To understand this you need to know how the event loop handles tasks and microtasks. This can be a lot to get your head around the first time you encounter it. Deep breath…
Each 'thread' gets its own event loop , so each web worker gets its own, so it can execute independently, whereas all windows on the same origin share an event loop as they can synchronously communicate. The event loop runs continually, executing any tasks queued. An event loop has multiple task sources which guarantees execution order within that source (specs such as IndexedDB define their own), but the browser gets to pick which source to take a task from on each turn of the loop. This allows the browser to give preference to performance sensitive tasks such as user-input. Ok ok, stay with me…
Tasks are scheduled so the browser can get from its internals into JavaScript/DOM land and ensures these actions happen sequentially. Between tasks, the browser may render updates. Getting from a mouse click to an event callback requires scheduling a task, as does parsing HTML, and in the above example, setTimeout .
setTimeout waits for a given delay then schedules a new task for its callback. This is why setTimeout is logged after script end , as logging script end is part of the first task, and setTimeout is logged in a separate task. Right, we're almost through this, but I need you to stay strong for this next bit…
Microtasks are usually scheduled for things that should happen straight after the currently executing script, such as reacting to a batch of actions, or to make something async without taking the penalty of a whole new task. The microtask queue is processed after callbacks as long as no other JavaScript is mid-execution, and at the end of each task. Any additional microtasks queued during microtasks are added to the end of the queue and also processed. Microtasks include mutation observer callbacks, and as in the above example, promise callbacks.
Once a promise settles, or if it has already settled, it queues a microtask for its reactionary callbacks. This ensures promise callbacks are async even if the promise has already settled. So calling .then(yey, nay) against a settled promise immediately queues a microtask. This is why promise1 and promise2 are logged after script end , as the currently running script must finish before microtasks are handled. promise1 and promise2 are logged before setTimeout , as microtasks always happen before the next task.
So, step by step:
Yes that's right, I created an animated step-by-step diagram. How did you spend your Saturday? Went out in the sun with your friends ? Well I didn't . Um, in case it isn't clear from my amazing UI design, click the arrows above to advance.
What are some browsers doing differently?
Some browsers log script start , script end , setTimeout , promise1 , promise2 . They're running promise callbacks after setTimeout . It's likely that they're calling promise callbacks as part of a new task rather than as a microtask.
This is sort-of excusable, as promises come from ECMAScript rather than HTML. ECMAScript has the concept of "jobs" which are similar to microtasks, but the relationship isn't explicit aside from vague mailing list discussions . However, the general consensus is that promises should be part of the microtask queue, and for good reason.
Treating promises as tasks leads to performance problems, as callbacks may be unnecessarily delayed by task-related things such as rendering. It also causes non-determinism due to interaction with other task sources, and can break interactions with other APIs, but more on that later.
Here's an Edge ticket for making promises use microtasks. WebKit nightly is doing the right thing, so I assume Safari will pick up the fix eventually, and it appears to be fixed in Firefox 43.
Really interesting that both Safari and Firefox suffered a regression here that's since been fixed. I wonder if it's just a coincidence.
How to tell if something uses tasks or microtasks
Testing is one way. See when logs appear relative to promises & setTimeout , although you're relying on the implementation to be correct.
The certain way, is to look up the spec. For instance, step 14 of setTimeout queues a task, whereas step 5 of queuing a mutation record queues a microtask.
As mentioned, in ECMAScript land, they call microtasks "jobs". In step 8.a of PerformPromiseThen , EnqueueJob is called to queue a microtask.
Now, let's look at a more complicated example. Cut to a concerned apprentice "No, they're not ready!". Ignore him, you're ready. Let's do this…
Level 1 bossfight
Before writing this post I'd have gotten this wrong. Here's a bit of html:
Given the following JS, what will be logged if I click div.inner ?
Go on, give it a go before peeking at the answer. Clue: Logs can happen more than once.
Click the inner square to trigger a click event:
Was your guess different? If so, you may still be right. Unfortunately the browsers don't really agree here:
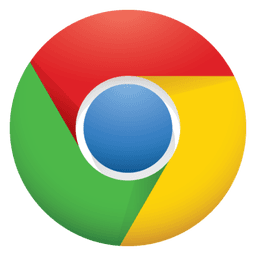
Who's right?
Dispatching the 'click' event is a task. Mutation observer and promise callbacks are queued as microtasks. The setTimeout callback is queued as a task. So here's how it goes:
So it's Chrome that gets it right. The bit that was 'news to me' is that microtasks are processed after callbacks (as long as no other JavaScript is mid-execution), I thought it was limited to end-of-task. This rule comes from the HTML spec for calling a callback:
If the stack of script settings objects is now empty, perform a microtask checkpoint — HTML: Cleaning up after a callback step 3
…and a microtask checkpoint involves going through the microtask queue, unless we're already processing the microtask queue. Similarly, ECMAScript says this of jobs:
Execution of a Job can be initiated only when there is no running execution context and the execution context stack is empty… — ECMAScript: Jobs and Job Queues
…although the "can be" becomes "must be" when in an HTML context.
What did browsers get wrong?
Firefox and Safari are correctly exhausting the microtask queue between click listeners, as shown by the mutation callbacks, but promises appear to be queued differently. This is sort-of excusable given that the link between jobs & microtasks is vague, but I'd still expect them to execute between listener callbacks. Firefox ticket . Safari ticket .
With Edge we've already seen it queue promises incorrectly, but it also fails to exhaust the microtask queue between click listeners, instead it does so after calling all listeners, which accounts for the single mutate log after both click logs. Bug ticket .
Level 1 boss's angry older brother
Ohh boy. Using the same example from above, what happens if we execute:
This will start the event dispatching as before, but using script rather than a real interaction.
And here's what the browsers say:
And I swear I keep getting different results from Chrome, I've updated this chart a ton of times thinking I was testing Canary by mistake. If you get different results in Chrome, tell me which version in the comments.
Why is it different?
Here's how it should happen:
So the correct order is: click , click , promise , mutate , promise , timeout , timeout , which Chrome seems to get right.
After each listener callback is called…
Previously, this meant that microtasks ran between listener callbacks, but .click() causes the event to dispatch synchronously, so the script that calls .click() is still in the stack between callbacks. The above rule ensures microtasks don't interrupt JavaScript that's mid-execution. This means we don't process the microtask queue between listener callbacks, they're processed after both listeners.
Does any of this matter?
Yeah, it'll bite you in obscure places (ouch). I encountered this while trying to create a simple wrapper library for IndexedDB that uses promises rather than weird IDBRequest objects. It almost makes IDB fun to use .
When IDB fires a success event, the related transaction object becomes inactive after dispatching (step 4). If I create a promise that resolves when this event fires, the callbacks should run before step 4 while the transaction is still active, but that doesn't happen in browsers other than Chrome, rendering the library kinda useless.
You can actually work around this problem in Firefox, because promise polyfills such as es6-promise use mutation observers for callbacks, which correctly use microtasks. Safari seems to suffer from race conditions with that fix, but that could just be their broken implementation of IDB . Unfortunately, things consistently fail in IE/Edge, as mutation events aren't handled after callbacks.
Hopefully we'll start to see some interoperability here soon.
You made it!
In summary:
- Tasks execute in order, and the browser may render between them
- after every callback, as long as no other JavaScript is mid-execution
- at the end of each task
Hopefully you now know your way around the event loop, or at least have an excuse to go and have a lie down.
Actually, is anyone still reading? Hello? Hello?
Thanks to Anne van Kesteren, Domenic Denicola, Brian Kardell, and Matt Gaunt for proofreading & corrections. Yeah, Matt actually read it in the end, I didn't even need to go full "Clockwork Orange" on him.
View this page on GitHub
Hello, I’m Jake and that is my tired face. I’m a developer of sorts.
Feel free to throw me an email , unless you're a recruiter, or someone trying to offer me 'sponsored content' for this site, in which case write your request on a piece of paper, and fling it out the window.
An Introduction to JavaScript's queueMicrotask
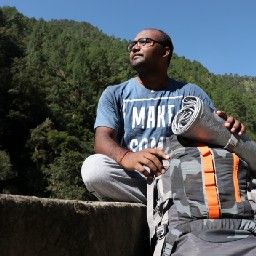
Introduction
queueMicrotask is a new browser API which can be used to convert your synchronous code into async:
It's similar to what we were doing using setTimeout:
So what's the use of queueMicrotask when we already have setTimeout ?
queueMicrotask adds the function (task) into a queue and each function is executed one by one (FIFO) after the current task has completed its work and when there is no other code waiting to be run before control of the execution context is returned to the browser's event loop.
Basically the tasks of queueMicrotask are executed just after current callstack is empty before passing the execution to the event loop.
In the case of setTimeout , each task is executed from the event queue, after control is given to the event loop.
So if we execute setTimeout first and then queueMicrotask , which will be called first? Execute the below code and check out yourself:
Node.js does the same thing with "process.nextTick".
When to Use It
There is no rule for when to use queueMicrotask, but it can be used cleverly to run a piece of code without stopping the current execution.
For example, let's say we have an array where we are maintaining list of values. After every value is inserted, we sort the array so that searching for values is faster.
However, searching for an element is done whenever someone uses a search input box. This means the event handler will be called after control is transferred to the event loop, so sorting the data blocks the execution of other important synchronous code.
Here's how we can use queueMicrotask to improve our code:
- https://developer.mozilla.org/en-US/docs/Web/API/WindowOrWorkerGlobalScope/queueMicrotask
Creator of open source javascript library jsstore | Full stack web developer | Polyglot | Tech enthusiast | Javascript & Typescript Lover | Sleeper
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
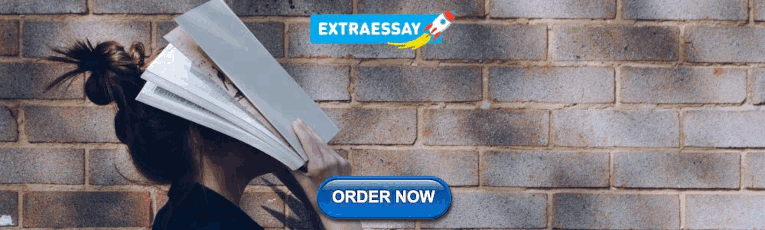
queueMicrotask() global function
Note: This feature is available in Web Workers .
The queueMicrotask() method, which is exposed on the Window or Worker interface, queues a microtask to be executed at a safe time prior to control returning to the browser's event loop.
The microtask is a short function which will run after the current task has completed its work and when there is no other code waiting to be run before control of the execution context is returned to the browser's event loop.
This lets your code run without interfering with any other, potentially higher priority, code that is pending, but before the browser regains control over the execution context, potentially depending on work you need to complete. You can learn more about how to use microtasks and why you might choose to do so in our microtask guide .
The importance of microtasks comes in its ability to perform tasks asynchronously but in a specific order. See Using microtasks in JavaScript with queueMicrotask() for more details.
Microtasks are especially useful for libraries and frameworks that need to perform final cleanup or other just-before-rendering tasks.
A function to be executed when the browser engine determines it is safe to call your code. Enqueued microtasks are executed after all pending tasks have completed but before yielding control to the browser's event loop.
Return value
None ( undefined ).
Taken from the queueMicrotask spec :
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Polyfill of queueMicrotask() in core-js
- Using microtasks in JavaScript with queueMicrotask()
- Asynchronous JavaScript
- queueMicrotask explainer
- Tasks, microtasks, queues and schedules by Jake Archibald

Demystifying JavaScript Runtime Environment: Web API, Task Queue, and Event Loop self.__wrap_n=self.__wrap_n||(self.CSS&&CSS.supports("text-wrap","balance")?1:2);self.__wrap_b=(t,n,e)=>{e=e||document.querySelector(`[data-br="${t}"]`);let s=e.parentElement,r=O=>e.style.maxWidth=O+"px";e.style.maxWidth="";let o=s.clientWidth,u=s.clientHeight,a=o/2-.25,l=o+.5,d;if(o){for(r(a),a=Math.max(e.scrollWidth,a);a+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(s):process.env.NODE_ENV==="development"&&console.warn("The browser you are using does not support the ResizeObserver API. Please consider add polyfill for this API to avoid potential layout shifts or upgrade your browser. Read more: https://github.com/shuding/react-wrap-balancer#browser-support-information"))};self.__wrap_n!=1&&self.__wrap_b(":R14nln6:",1)
JavaScript's inner workings can be complex and fascinating. In this article, we'll delve deeper into the JavaScript runtime environment, exploring asynchronous code execution, and how the browser provides essential features for web development.
In one of the previous articles, we delved into the fundamentals of the JavaScript engine, covering essential language features such as calculations and loops. However, the world of JavaScript is far more intricate, especially when it comes to handling asynchronous code and utilizing browser-provided objects. To navigate this complexity, we'll dive into the JavaScript runtime environment, which comprises Web API, Task Queue, and the Event Loop.
Browser API
Browser Provides Features Beyond the JavaScript Engine
The Web API, also known as the Browser API, is an essential part of the runtime environment provided by the browser. It's separate from the JavaScript engine but allows you to interact with browser features using JavaScript. Some aspects of the Web API include its facilitation of concurrent operations external to the JavaScript interpreter, despite JavaScript's inherent single-threaded nature.
When a function call involves asynchronous operations, like making an HTTP request, setTimeout, or handling events, the JavaScript engine delegates the handling of these operations to the browser's corresponding API (e.g., XMLHttpRequest or Fetch API) instead of blocking the call stack and waiting for the operation to complete (which would freeze the browser interface). Along with delegating the task, the engine also registers a callback function to be executed once the operation completes or an event occurs. Upon completion, when a timer reaches its designated time, or upon a user's click event, the corresponding callback is typically dispatched to the Task Queue.
Examples of Web APIs
Document Manipulation: Utilizing the DOM (Document Object Model) for interactive web content manipulation and organization.
- Server Data Retrieval: Employing the Fetch API to seamlessly retrieve data from servers.
- Timer Functions: Utilizing APIs to manage and trigger time-based operations within web applications.
- Graphics Drawing and Manipulation: Leveraging the Canvas API and WebGL API for graphical content creation and manipulation on web platforms.
- Audio and Video Handling: Utilizing APIs like the Web Audio API and WebRTC API for audio/video streaming and manipulation.
- Device-Related Functions: Utilizing the Geolocation API to access location-based services on web applications.
- Client-Side Storage: Leveraging Web Storage API and IndexedDB API for efficient client-side data storage and retrieval within browsers.
In Node.js, there are no browser-provided Web APIs. Instead, Node.js presents a distinct set of APIs unrelated to browsers. These encompass functionalities for file system operations, network communication, and more. As Node.js is primarily utilized for server-side JavaScript applications, its APIs are specifically designed for that environment.
The Task Queue functions as a message queue where each message corresponds to an associated function for execution. Here are its key characteristics:
- Messages follow a First-In, First-Out (FIFO) processing order.
- It enables the execution of code after the completion of API calls.
- Within the Event Loop, once the call stack is clear, the runtime proceeds to process the oldest message in the queue by invoking its functions and adding them to the Call Stack.
- Function execution continues until the Call Stack is empty, after which the next message in the queue is processed.
The Task Queue is sometimes referred to as the "Event Queue" or "Callback Queue." Tasks are added to this queue when an event triggers a callback or when a specified timeout/interval expires.
Microtask queue
The Microtask Queue is similar to the task queue but has higher priority. It handles Microtask callbacks, including promise handlers and mutation observers.
Microtasks consist of concise functions executed after the parent function or program finishes, but only when the Call Stack is clear. Some essential aspects of microtasks include:
- Microtasks have the capability to enqueue additional microtasks, and these subsequently queued microtasks execute before the next task initiates, all within the same event loop iteration.
- They are designed to handle actions that require immediate execution following the current script, such as responding to a series of actions or implementing asynchronous functionality without incurring the overhead of a completely new task.
The Event Loop stands as a crucial element within the JavaScript runtime environment, functioning in the following manner:
- Continuously monitoring the status of the call stack to determine if it's empty.
- Upon encountering an empty call stack, all pending Microtasks from the Microtask Queue are introduced into the call stack.
- When both the call stack and Microtask Queue are devoid of tasks, the event loop proceeds to dequeue tasks from the Task Queue and execute them.
- Ensuring the prevention of a "starved event loop" remains pivotal for maintaining seamless JavaScript execution.
In Node.js, the Event Loop shares similarities with the browser environment, yet it diverges in its handling of I/O operations and event-driven code. Node.js leverages a unique array of modules like fs for file system operations and http for constructing HTTP servers, pivotal to its event-driven architecture while maintaining the core concepts of the Event Loop and Task Queue.
Exploring how JavaScript works behind the scenes revealed a bunch of moving parts. There's the Web API that helps JavaScript do more than it could on its own, handling tasks like fetching data or dealing with graphics. Then there's the Task Queue and Microtask Queue, kind of like waiting lines for different jobs. The Event Loop is like a supervisor, making sure tasks get done in the right order without things getting stuck. In a different world called Node.js, it's similar but has its own special tools for handling stuff. Understanding how all this works helps folks build cooler and faster websites and apps that keep up with the ever-changing web.
- https://medium.com/@antwan29/browser-and-web-apis-d48c3fd8739
- https://frontendmasters.com/books/front-end-handbook/2018/learning/web-api.html
- https://developer.mozilla.org/en-US/docs/Web/API
- https://developer.mozilla.org/en-US/docs/Web/API/HTML_DOM_API/Microtask_guide
- https://developer.mozilla.org/en-US/docs/Web/API/HTML_DOM_API/Microtask_guide/In_depth
- https://dev.to/lydiahallie/javascript-visualized-event-loop-3dif
- https://javascript.info/event-loop
What will be the output of this code?
The console output is: 1 7 3 5 2 6 4.
The task is quite simple, we just need to know how microtask and macrotask queues work.
Let’s see what’s going on, step by step.
To summarize,
- Numbers 1 and 7 show up immediately, because simple console.log calls don’t use any queues.
- It has commands: console.log(3); setTimeout(...4); console.log(5) .
- Numbers 3 and 5 show up, while setTimeout(() => console.log(4)) adds the console.log(4) call to the end of the macrotask queue.
- The macrotask queue is now: console.log(2); console.log(6); console.log(4) .
- After the microtask queue becomes empty, the macrotask queue executes. It outputs 2 , 6 , 4 .
Finally, we have the output: 1 7 3 5 2 6 4 .
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
What is Task Queue in JavaScript Event Loop? self.__wrap_b=(t,n,e)=>{e=e||document.querySelector(`[data-br="${t}"]`);let a=e.parentElement,r=R=>e.style.maxWidth=R+"px";e.style.maxWidth="";let o=a.clientWidth,c=a.clientHeight,i=o/2-.25,l=o+.5,u;if(o){for(;i+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(a):process.env.NODE_ENV==="development"&&console.warn("The browser you are using does not support the ResizeObserver API. Please consider add polyfill for this API to avoid potential layout shifts or upgrade your browser. Read more: https://github.com/shuding/react-wrap-balancer#browser-support-information"))};self.__wrap_b(":R4mr36:",1)
Understanding JavaScript
The event loop, microtask queue, event loop, task queue, and microtask queue in action.

Greetings, codedamn community! This blog post is aimed to delve deeper into the advanced concept of Task Queues in JavaScript Event Loop. As experienced developers, we all might have encountered scenarios where understanding the intricate workings of JavaScript becomes crucial. One such situation is when dealing with asynchronous programming, and here is where the concept of Task Queue and Event Loop becomes indispensable. So, let's unravel the mystery behind it!
Before diving into the main topic, let's briefly touch upon JavaScript's nature. JavaScript is a single-threaded, non-blocking, asynchronous, concurrent language, which means it can handle only one task at a time. However, JavaScript has features that allow it to handle tasks asynchronously, and this is where things like asynchronous callbacks, Promises, Async/Await, and our main topic, the Task Queue in the Event Loop, come into play.
The Event Loop in JavaScript is a mechanism that keeps checking whether the call stack is empty. If it is, it takes the first task from the Task Queue and pushes it to the Call Stack. It's an infinite loop responsible for executing queued code snippets at appropriate times, hence the name 'Event Loop.'
The Event Loop has one simple job — to monitor the Call Stack and the Task Queue. If the Call Stack is empty, it will take the first event from the queue and will push it to the Call Stack, which effectively runs it.
Also known as the 'Message Queue,' the Task Queue in JavaScript is a list of messages to be processed. Each message is associated with a function, which gets called to handle that message. As the stack unwinds, it keeps looking at the Task Queue to check if there's something to be executed.
Let’s take an example to understand this better.
In the above scenario, 'Hello' and '!' are logged immediately, while 'World' waits until the Call Stack is empty, even though the timeout is set to 0 milliseconds. This is because setTimeout is a Web API that puts a message (in this case, the function to log 'World') into the Task Queue to be executed later.
Apart from the Task Queue, there's another queue called the 'Microtask Queue.' This queue has higher priority and includes microtasks such as Promises. The Event Loop will process all tasks in the Microtask Queue before it gets to the Task Queue.
This is important to note because it can affect the order of execution in your JavaScript code. For instance, Promise callbacks will always run before setTimeout and setInterval callbacks, due to the higher priority of the Microtask Queue.
Let’s see all these components in action with a piece of code:
In this case, the output will be 'Hello', '!', and then 'World'. This order is due to the priority of Microtask Queue (Promise) over Task Queue (setTimeout).
Q: What is the difference between Task Queue and Microtask Queue?
A: The key difference is their priority in the Event Loop. The Event Loop will process all tasks in the Microtask Queue before it gets to the Task Queue.
Q: Can JavaScript handle multiple tasks simultaneously?
A: JavaScript is single-threaded, meaning it can handle only one task at a time. However, it can manage tasks asynchronously using mechanisms like the Event Loop and Task Queue.
Q: How does the Event Loop affect my JavaScript code execution?
A: The Event Loop ensures your JavaScript code doesn't block the single thread by setting aside asynchronous callbacks and pushing them to the Call Stack only when it's empty. This enables a smooth UI and better user experience.
For more in-depth understanding, you can refer to the official MDN Web docs . Understanding these mechanisms helps make your JavaScript code more efficient and manageable. Happy coding!
Remember, the beauty of JavaScript lies in its asynchronicity, and understanding the Event Loop, Task Queue, and Microtask Queue is a significant step towards mastering it. So keep exploring, keep learning!
Sharing is caring
Did you like what Pranav wrote? Thank them for their work by sharing it on social media.
No comment s so far
Curious about this topic? Continue your journey with these coding courses:
3.69k students learning
Mehul Mohan
Advanced Theoretical JavaScript
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
A lightweight JavaScript task queue
good-ware/js-task-queue
Folders and files, repository files navigation, @goodware/task-queue: a lightweight task queue.
- Release History
- RunKit Example
Requirements
Installation.
npm i --save @goodware/task-queue
This lightweight, battle-tested, single-dependency (joi) task queue limits the number of tasks (synchronous or asynchronous) that execute concurrently. The purpose of limiting task execution is to control resource usage such as memory and database connections.
Although several packages address this use case, this is apparently the only library that can queue tasks post-instantiation without using generators. The API is, most of all, easy to learn and use.
A task-queue object is instantiated by providing a configuration object to the constructor. The configuration object currently has one required and one optional property:
size can be provided without workers. workers can provided without size.
Functions are queued via the asynchronous method push(task) . This method accepts a function named task and returns a Promise that resolves to an object when the task function is called ( not when task returns). task is called only when a worker is available. task does not need to return a Promise, but if it does, it can be acquired via the promise property of the object returned by push().
Brief Example
- Create a queue that runs at most 10 running tasks
- Wait for the provided function to be invoked:
- Wait for the provided function to finish:
Code Sample
This example runs at most two tasks at a time. It outputs: 2, 1, 4, 3.
Minimizing Memory Usage
push() returns a new Promise each time it is called, thus consuming memory. Depending on your application, it may be necessary to limit calls to push() when the queue is full if you are unable to control the number of calls to push().
For example, consider the following constraints:
- Up to 10 workers can execute at the same time
- When 10 workers are running, up to 50 tasks can call push() and immediately continue their work. Subsequent callers will wait until a worker has finished.
Although it appears that resources are properly constrained in this scenario, if push() is called, say, 1,000 times a second, and the workers take longer than 1 second each, the process will likely run out of memory. One solution to this scenario is backpressure.
No form of backpressure is a silver bullet. External systems must handle errors and retry.
- JavaScript 100.0%
Queue Data Structures: How to Build a Node Task Queue
Share this article
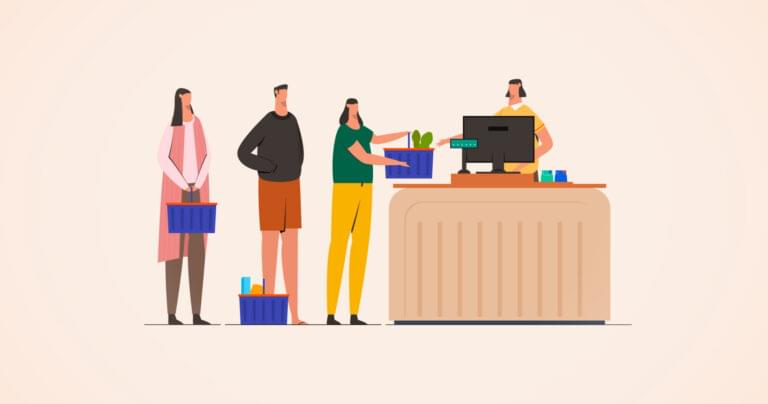
What Are Queue Data Structures?
A basic javascript task queue data structure, queuing platforms.
- Use MongoDB as Our Node Task Queue’s Message Broker
Node Task Queue Project: Getting Started
How the queue-mongodb module works, queue-mongodb module: initialization, queue-mongodb module: queue constructor, queue-mongodb module: queue.send() method, queue-mongodb module: queue.receive() method, queue-mongodb module: queue.remove() method, queue-mongodb module: queue.purge() method, queue-mongodb module: queue.count() method, queue-mongodb module: queue.close() method, a new queue, faqs about using the queue data structure in node.
This tutorial explains queue data structures and demonstrates queuing systems. Queues are often used to process long-running tasks such as email newsletter delivery. Below, you’ll build a simple Node task queue.
It’s not always practical to execute a task the moment it’s requested.
Consider an email newsletter administration system. After writing, an administrator must hit a big red “SEND NOW” button. The application could send every email immediately and show a “completed” response. That would work for a dozen messages, but how long would it take for 1,000 subscribers or more? The browser request would time out before the process completed.
Another example: a user can upload any number of photographs to a gallery application. The system resizes and sharpens each image for alternative dimensions. This process could run on upload, but it would to incur a delay for every image.
It’s more effective to decouple tasks in these situations. The user receives an instant response but task processing occurs in the background. Other applications or servers handle tasks and schedule re-attempts on failure. The user can receive alerts or examine logs to determine progress.
A queue is a data structure which holds a collection of items:
- Any process can send (or enqueue ) an item at any time — such as send newsletter X to recipient Y.
- Any process can receive (or dequeue ) the item at the front of the queue — for example, the item that’s been in the queue for longest.
Queue data structures are a first-in-first-out (FIFO) structure. The first item added to the queue will be the first out.
You can create a task queue using a JavaScript array. The push() method adds an item to the end of an Array while the shift() method removes and returns an item from the start:
Your queue data structures can hold any data in individual array elements. You can push strings, numbers, Booleans, other arrays, or objects.
You can use an ES6 class to define any number of separate queues:
These simple queue data structures may be useful for less critical client-side code such as queuing UI updates so processing occurs in a single DOM update. localStorage or IndexedDB can offer a level of data persistence if necessary.
In-memory queues are less practical for complex server applications:
- Two or more separate applications can’t (easily) access the same queue.
- Queue data disappears when the application terminates.
Purpose built message-broker software provides more robust queuing. Platforms vary, but offer features such as:
- data persistence in a choice of databases with replication, sharding, and clustering options
- a range of access protocols, often including HTTP and Web Sockets
- any number of separate queues
- delayed messaging, where message processing can occur at a later time
- transaction-like support, where a message is re-queued when processing isn’t confirmed
- publish-subscribe patterns, where applications receive an event when a new item appears on a queue
Message-broker software includes Redis , RabbitMQ , Apache ActiveMQ , and Gearman . Cloud messaging services include Amazon SQS , Azure Service Bus , and Google Pub/Sub .
These may be viable options for enterprise-level applications. Yet they could be overkill if you have simpler requirements and already use a database.
Use MongoDB as Our Node Task Queue’s Message Broker
It’s possible to develop a sophisticated Node task queue system to manage queue data structures in a couple of hundred lines of code.
The queue-mongodb module described here uses MongoDB for data storage, but the same concepts could be adopted by any SQL or NoSQL database. The code is available on GitHub and npm .
Make sure you have Node.js 14 or above installed, then create a new project folder such as queue-test . Add a new package.json file:
Note: "type": "module" configures the project to use ES6 modules. The "scripts" will send and receive queued items.
Install the queue-mongodb module:
Then create a .env file with your MongoDB database connection credentials. For example:
Note: this creates a queue collection ( QUEUE_DB_COLL ) in the qdb database ( QUEUE_DB_NAME ). You can use an existing database, but make sure the collection doesn’t clash with another.
Database read/write access must be granted to the user root ( QUEUE_DB_USER ) with password mysecret ( QUEUE_DB_PASS ). Set both values blank if no authentication is required.
Start a MongoDB database if it’s not already running. Those with Docker and Docker Compose can create a new docker-compose.yml file:
Then run docker-compose up to download and start MongoDB with a persistent data volume.
Docker is available Linux, macOS, and Windows 10. See the Docker installation instructions .
Create a new send.js file to add a randomly generated email messages to a queue named news :
Execute it with npm run send and you’ll see output such as this:
The .send() method returns an qItem object containing:
- the MongoDB document _id
- the date/time the item was originally queued, and
- a copy of the message data
Run the script any number of times to add further items to the queue. The items queued will increment on every run.
Now create a new receive.js file to retrieve messages from the same Node task queue:
Run npm run receive to fetch and process queued items:
No email is sent in this example, but that could be implemented using Nodemailer or another suitable module.
If processing fails — perhaps because the mail server is down — an item can be re-queued with this:
The second 600 argument is an optional number of seconds or future date. This command re-queues the item after 600 seconds (ten minutes) have elapsed.
This is a simple example, but any application can send data to any number of queues. Another process, perhaps started as a cron job, can receive and process items when necessary.
The type string passed to the class constructor defines a queue name. The .send() method creates a new MongoDB document when passed data to add to the queue. The MongoDB document contains:
- A MongoDB _id (the creation date/time is encoded within the value).
- The queue type .
- A processing date/time value named proc . It’s possible to set a future time but the current time is the default.
- The item data . This can be anything: a Boolean, number, string, array, object, and so on.
The .receive() method locates the oldest document that has a matching type and a proc date/time in the past. The document is formatted, returned to the calling code, and deleted from the database.
The following sections describe the module in further detail.
The dotenv module reads the .env environment variables if necessary. A database connection object is created using the official mongodb driver module :
The qCollection variable holds a reference to the database’s queue collection (defined by QUEUE_DB_COLL ). It’s created and returned by the dbConnect() function, which also defines the collection schema and indexes when necessary. All Queue methods run const q = await dbConnect(); to get the collection reference:
The dbClose() function closes the database connection:
The Queue constructor sets the queue type or name:
The .send() method adds data to the queue with the appropriate type . It has an optional delayUntil parameter, which adds an item to the queue at a future time by specifying a number of seconds or a Date() .
The method inserts a new document into the database and returns a qItem object ( { _id , sent , data } ) or null if unsuccessful:
The .receive() method retrieves and deletes the oldest queued item in the database with a specific type and a proc date/time in the past. It returns a qItem object ( { _id , sent , data } ) or null if nothing is available or an error occurs:
The .remove() method deletes the queued item identified by a qItem object ( { _id , sent , data } ) returned by the .send() method. It can be used to remove a queued item regardless of its position in the queue.
The method returns the number of deleted documents (normally 1) or null when an error occurs:
The .purge() method deletes all queued items of the same type and returns the number of deletions:
The .count() method returns the number of queued items of the same type :
The .close() method runs the dbClose() function to terminate the database connection so the Node.js event loop can end:
Queues are a consideration for any web application with computationally expensive functions that could cause a bottleneck. They can improve performance and maintenance by decoupling applications into smaller, faster, more robust processes. Dedicated message broker software is an option, but simple queuing systems like the Node task queue we built today are possible in a few dozen lines of code.
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, where the first element added is the first to be removed. In a queue, elements are added to the rear and removed from the front.
Queues are useful for managing tasks or data in a sequential manner. They are commonly used for background processing, task scheduling, event handling, and managing resources like connections and requests.
You can implement a queue using arrays or linked lists. In Node.js, you can use the built-in array data structure or implement a custom queue using JavaScript objects and methods.
The key difference is the order of removal. In a queue, the first element added is the first to be removed (FIFO), while in a stack, the last element added is the first to be removed (LIFO).
Queues are used for task scheduling, managing concurrent requests, processing messages in a message queue, handling event listeners, and more. They are vital for scenarios where order and sequence matter.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler .

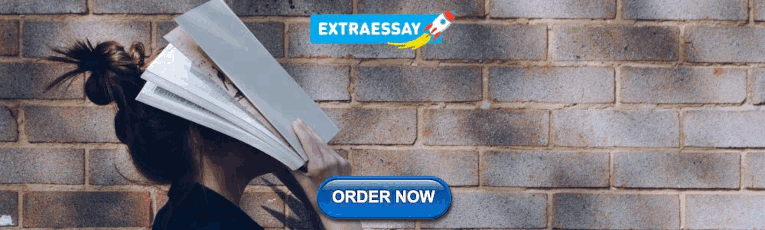
IMAGES
VIDEO
COMMENTS
Tasks get added to the task queue when: A new JavaScript program or subprogram is executed (such as from a console, or by running the code in a <script> element) directly. An event fires, adding the event's callback function to the task queue. A timeout or interval created with setTimeout() or setInterval() is reached, causing the corresponding ...
Summary. A more detailed event loop algorithm (though still simplified compared to the specification ): Dequeue and run the oldest task from the macrotask queue (e.g. "script"). Execute all microtasks : While the microtask queue is not empty: Dequeue and run the oldest microtask. Render changes if any.
This Promise can be used to handle the response of your async task Fn. enqueue() actually push() an Object into the queue, that holds the task Fn and the control methods for the returned Promise. Since the unwrapped returned Promise insta. starts to run, this.dequeue() is invoked each time we enqueue a new task.
The queue is first-in-first-out: tasks enqueued first are run first. Execution of a task is initiated only when nothing else is running. Or, to put it more simply, when a promise is ready, its .then/catch/finally handlers are put into the queue; they are not executed yet. When the JavaScript engine becomes free from the current code, it takes a ...
Task Queue (Callback Queue) As we traverse the landscape of JavaScript's event loop, our attention now turns to the task queue, also known as the callback queue. This queue is where tasks, often ...
The microtask queue is a critical part of JavaScript's asynchronous mechanism, working in tandem with the task queue. It is responsible for handling microtasks, which typically include promises and Mutation Observers. Microtasks have a higher precedence than tasks in the execution. This means that when the call stack is empty and JavaScript is ...
The Queue in Event Loop Model holds the Tasks (or the MacroTasks) called, TaskQueue. The Queue in Event Loop Model holds the Jobs (or the MicroTasks) called, JobQueue. For example, Promises are in Job Queue, and the functions for setTimeOut are in TaskQueue. With the explanation above, let us re-visit the Event Loop Model once more than last time.
JavaScript promises use the microtask queue for running callbacks. This chapter is dedicated to the exploration of microtasks in JavaScript. Before getting to microtasks it is useful to define JavaScript tasks. A task is considered a JavaScript code that is scheduled to be executed by standard mechanisms.
The microtask queue is processed after callbacks as long as no other JavaScript is mid-execution, and at the end of each task. Any additional microtasks queued during microtasks are added to the end of the queue and also processed. ... The certain way, is to look up the spec. For instance, step 14 of setTimeout queues a task, whereas step 5 of ...
Basically the tasks of queueMicrotask are executed just after current callstack is empty before passing the execution to the event loop. In the case of setTimeout, each task is executed from the event queue, after control is given to the event loop. So if we execute setTimeout first and then queueMicrotask, which will be called first? Execute ...
In this video, we will look at how JavaScript does Asynchronous operations and how the task queue and event loop works.Event Loop & Microtask Queue Article:h...
Javascript visualizer. JavaScript is an incredibly versatile language, known for its single-threaded, non-blocking nature. While this might sound counterintuitive for handling multiple tasks simultaneously, JavaScript employs a clever system involving the event loop, task queue, and microtask queue to manage asynchronous operations efficiently.
queueMicrotask () global function. Note: This feature is available in Web Workers. The queueMicrotask() method, which is exposed on the Window or Worker interface, queues a microtask to be executed at a safe time prior to control returning to the browser's event loop. The microtask is a short function which will run after the current task has ...
The Event Loop stands as a crucial element within the JavaScript runtime environment, functioning in the following manner: Continuously monitoring the status of the call stack to determine if it's empty. Upon encountering an empty call stack, all pending Microtasks from the Microtask Queue are introduced into the call stack. When both the call ...
The task is quite simple, we just need to know how microtask and macrotask queues work. Let's see what's going on, step by step. ... The macrotask queue is now: console.log(2); console.log(6); console.log(4). After the microtask queue becomes empty, the macrotask queue executes.
The Event Loop in JavaScript is a mechanism that keeps checking whether the call stack is empty. If it is, it takes the first task from the Task Queue and pushes it to the Call Stack. It's an infinite loop responsible for executing queued code snippets at appropriate times, hence the name 'Event Loop.'. The Event Loop has one simple job — to ...
Overview. This lightweight, battle-tested, single-dependency (joi) task queue limits the number of tasks (synchronous or asynchronous) that execute concurrently. The purpose of limiting task execution is to control resource usage such as memory and database connections. Although several packages address this use case, this is apparently the ...
A Basic JavaScript Task Queue Data Structure; Queuing Platforms; Use MongoDB as Our Node Task Queue's Message Broker; Node Task Queue Project: Getting Started; How the queue-mongodb Module Works;
1. In JavaScript Runtime, the Job Queue is created with Task Queue during the JavaScript Runtime, which is much similar to the task queue, but it has priority over the task queue. This means the JavaScript event loop first looks into the job queue. If there is any task in the job queue, the event loop will check the stack.
Ask questions, find answers and collaborate at work with Stack Overflow for Teams. Explore Teams Create a free Team