Home » PHP OOP » PHP Objects
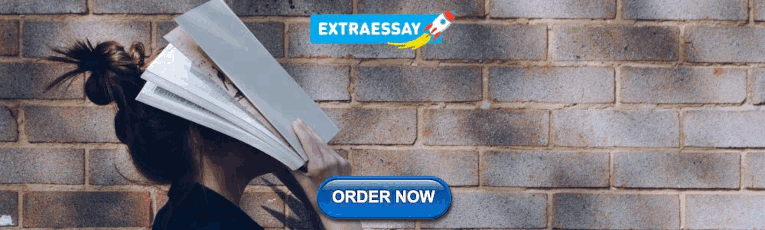
PHP Objects
Summary : in this tutorial, you will learn about PHP objects, how to define a class, and how to create an object from a class.
What is an Object
If you look at the world around you, you’ll find many examples of tangible objects: lamps, phones, computers, and cars. Also, you can find intangible objects such as bank accounts and transactions.
All of these objects share the two common key characteristics:
For example, a bank account has the state that consists of:
- Account number
A bank account also has the following behaviors:
PHP objects are conceptually similar to real-world objects because they consist of state and behavior.
An object holds its state in variables that are often referred to as properties . An object also exposes its behavior via functions which are known as methods .
What is a class?
In the real world, you can find many same kinds of objects. For example, a bank has many bank accounts. All of them have account numbers and balances.
These bank accounts are created from the same blueprint. In object-oriented terms, we say that an individual bank account is an instance of a Bank Account class.
By definition, a class is the blueprint of objects. For example, from the Bank Account class, you can create many bank account objects.
The following illustrates the relationship between the BankAccount class and its objects. From the BankAccount class you can create many BankAccount objects. And each object has its own account number and balance.
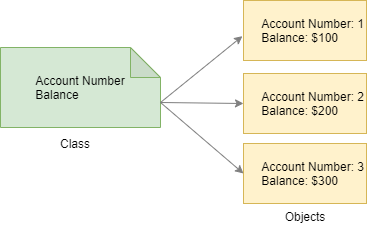
Define a class
To define a class, you specify the class keyword followed by a name like this:
For example, the following defines a new class called BankAccount :
By convention, you should follow these rules when defining a class:
- A class name should be in the upper camel case where each word is capitalized. For example, BankAccount , Customer , Transaction , and DebitNote .
- If a class name is a noun, it should be in the singular noun.
- Define each class in a separate PHP file.
From the BankAccount class, you can create a new bank account object by using the new keyword like this:
In this syntax, the $account is a variable that references the object created by the BankAccount class. The parentheses that follow the BankAccount class name are optional. Therefore, you can create a new BankAccount object like this:
The process of creating a new object is also called instantiation. In other words, you instantiate an object from a class. Or you create a new object from a class.
The BankAccount class is empty because it doesn’t have any state and behavior.
Add properties to a class
To add properties to the BankAccount class, you place variables inside it. For example:
The BankAccount class has two properties $accountNumber and $balance . In front of each property, you see the public keyword.
The public keyword determines the visibility of a property . In this case, you can access the property from the outside of the class.
To access a property, you use the object operator ( -> ) like this:
The following example shows how to set the values of the accountNumber and balance properties:
Besides the public keyword, PHP also has private and protected keywords which you’ll learn in the access modifiers tutorial .
Add methods to a class
The following shows the syntax for defining a method in a class:
Like a property, a method also has one of the three visibility modifiers: public , private , and protected . If you define a method without any visibility modifier, it defaults to public .
The following example defines the deposit() method for the BankAccount class:
The deposit() method accepts an argument $amount . It checks if the $amount is greater than zero before adding it to the balance.
To call a method, you also use the object operator ( -> ) as follows:
The new syntax in the deposit() method is the $this variable. The $this variable is the current object of the BankAccount class.
For example, when you create a new object $account and call the deposit() method, the $this inside the method is the $account object:
Similarly, you can add the withdraw() method to the BankAccount class as follows:
The withdraw() method checks the current balance.
If the balance is less than the withdrawal amount, the withdraw() method returns false .
Later, you’ll learn how to throw an exception instead. Otherwise, it deducts the withdrawal amount from the balance and returns true .
- Objects have states and behaviors.
- A class is a blueprint for creating objects.
- Properties represent the object’s state, and methods represent the object’s behavior. Properties and methods have visibility.
- Use the new keyword to create an object from a class.
- The $this variable references the current object of the class.
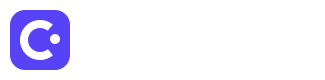
PHP Objects: A Comprehensive Guide to Object-Oriented Programming in PHP

PHP is one of the most popular server-side programming languages used today. It’s widely used for web development and has become an object-oriented language. Object-oriented programming (OOP) is a programming paradigm that uses objects to represent and manipulate data. It’s a powerful way to organize code and make it more reusable, maintainable, and scalable. In this article, we’ll explore PHP objects, how they work, and how to use them in your code.
What are Objects in PHP?
Objects in PHP are instances of classes . A class is a blueprint for creating objects that defines their properties and methods. Properties are variables that hold data, while methods are functions that operate on that data. When you create an object, you’re creating an instance of a class with its own properties and methods.
Let’s take a look at a simple example. Suppose you have a class called Person that defines a person’s name and age:
To create an object of this class, you use the new keyword followed by the class name:
Now you can set the properties of the object using the arrow operator ( -> ):
You can also access the properties of the object using the arrow operator:
Encapsulation, Inheritance, and Polymorphism
Three key concepts in OOP are encapsulation, inheritance, and polymorphism. Let’s take a closer look at each of these concepts and see how they apply to PHP objects.
Encapsulation
Encapsulation is the practice of hiding the implementation details of a class from the outside world. This is done by making the class properties and methods private or protected. Private properties and methods can only be accessed from within the class, while protected properties and methods can be accessed from within the class and its subclasses.
Encapsulation is important because it prevents external code from directly modifying the internal state of an object. This makes the code more robust and less error-prone. Let’s modify our Person class to make the properties private:
Now we can only set and get the properties of the object using public methods. This ensures that the internal state of the object remains consistent.
Inheritance
Inheritance is the process by which one class acquires the properties and methods of another class. The class that is being inherited from is called the parent or superclass, while the class that inherits from it is called the child or subclass.
Inheritance is useful when you want to reuse code across multiple classes. You can define common properties and methods in a parent class and have the child classes inherit from it. This reduces code duplication and makes the code more maintainable.
Let’s create a new class called Employee that inherits from the Person class:
The `Employee` class now has access to the properties and methods of the `Person` class, as well as its own `salary` property and `getSalary()` method.
Polymorphism
Polymorphism is the ability of objects of different classes to be used interchangeably. This means that you can use a child object wherever a parent object is expected. Polymorphism is achieved through inheritance and interfaces.
Let’s say we have a function that takes a `Person` object as a parameter and returns their name and age:
We can pass in an object of the Person class or any of its subclasses and the function will work correctly. For example:
Magic Methods
PHP has a number of special methods that are known as magic methods. These methods are called automatically by PHP when certain actions are performed on an object. Magic methods can be used to implement functionality such as object serialization, cloning, and method overloading.
Here are some of the most commonly used magic methods:
__construct()
The __construct() method is called when an object is created and can be used to initialize the object’s properties.
__toString()
The __toString() method is called when an object is used as a string. It should return a string representation of the object.
__get() and __set()
The __get() and __set() methods are called when an attempt is made to read or write to a non-existent property of an object.
The __clone() method is called when an object is cloned using the clone keyword. It can be used to perform custom cloning operations.
Interfaces are similar to classes in that they define methods, but they don’t provide implementations. Instead, they specify a contract that a class must follow. Any class that implements an interface must define all of its methods.
Interfaces are useful when you want to define a common set of methods that multiple classes can implement. This allows you to write code that works with any object that implements the interface.
Here’s an example of an interface called Movable that defines a move() method:
Now we can create classes that implement this interface, such as a Car class and a Person class:
We can then write code that works with any object that implements the Movable interface:
In this article, we’ve explored PHP objects, how they work, and how to use them in your code. We’ve covered key concepts such as encapsulation, inheritance, and polymorphism, as well as magic methods and interfaces. By using object-oriented programming in PHP, you can write code that’s more reusable, maintainable, and scalable. Hopefully, this article has given you a solid foundation for using objects in your PHP code.
📕 Related articles about PHP
- PHP Loops: An In-Depth Guide for Developers
Understanding PHP Syntax: A Comprehensive Guide
Mastering php constants: a comprehensive guide.
- PHP Classes: A Comprehensive Guide for Software Developers
- How to install PHP on Windows, Linux, and Mac: A Comprehensive Guide [5 easy steps]
- The Ultimate Guide to PHP Namespaces
Matthew is a highly skilled software developer with more than five years of experience in the industry. He has an extensive background in working with various projects, including both frontend and backend frameworks. Matthew is an expert in building robust and scalable software solutions that meet the needs of businesses and end-users.
Similar Posts
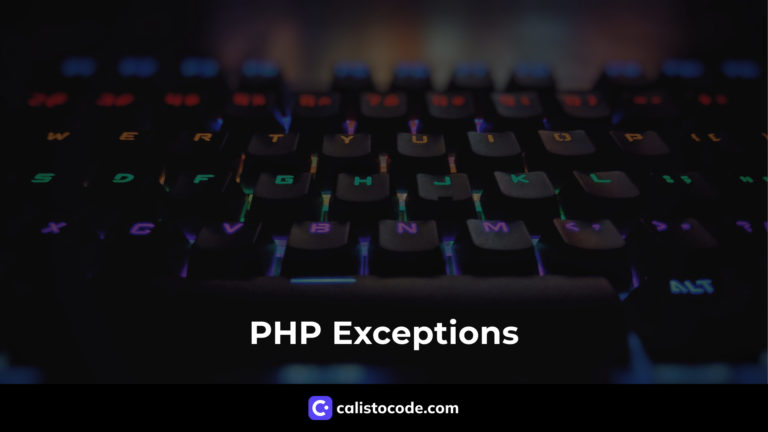
PHP Exceptions: Understanding How to Use Them Effectively
Exceptions are an essential part of any programming language, and PHP is no exception. As a software developer, it’s essential to understand what exceptions are, how they work, and when to use them. This article will dive deeply into PHP exceptions, covering everything from the basics to best practices. What are Exceptions in PHP? In…

The Power of AJAX XML in Modern Software Development
Web developers have been utilizing AJAX and XML, two powerful technologies, for years to create dynamic and responsive web applications. They can make user interfaces highly interactive and decrease page loading times by making asynchronous requests to servers. In this article, we’ll dive deeper into what AJAX XML is and how it’s used in modern…

PHP Data Types
PHP is a popular programming language that is widely used for developing dynamic websites and web applications. One of the fundamental concepts in PHP is data types. Data types in PHP refer to the different kinds of values that can be stored and manipulated by PHP programs. In this article, we will explore the various…

If you’re an experienced software developer, you know that constants are a fundamental element of programming –– and PHP is no exception. Constants are values that cannot be modified during runtime and can be used to store any data type that can be assigned to a variable. However, unlike a variable, a constant’s value cannot…

How to Use PHP Mail Function to Improve Your Email Delivery
Introduction Sending emails in PHP is necessary for web developers who are working on applications that need to send emails. Email is mainly used for communication between applications and users. PHP mail function is an inbuilt PHP function that allows developers to send emails from PHP scripts quickly. In this article, we will discuss PHP…

If you are a web developer, you may have heard of PHP. It is a popular server-side scripting language that is widely used to build dynamic web pages. Understanding PHP syntax is crucial for building robust web applications that are both efficient and secure. In this article, we will explore the basics of PHP syntax,…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
PHP Tutorial
Php advanced, mysql database, php examples, php reference, php oop - classes and objects.
A class is a template for objects, and an object is an instance of class.
Let's assume we have a class named Fruit. A Fruit can have properties like name, color, weight, etc. We can define variables like $name, $color, and $weight to hold the values of these properties.
When the individual objects (apple, banana, etc.) are created, they inherit all the properties and behaviors from the class, but each object will have different values for the properties.
Define a Class
A class is defined by using the class keyword, followed by the name of the class and a pair of curly braces ({}). All its properties and methods go inside the braces:
Below we declare a class named Fruit consisting of two properties ($name and $color) and two methods set_name() and get_name() for setting and getting the $name property:
Note: In a class, variables are called properties and functions are called methods!
Define Objects
Classes are nothing without objects! We can create multiple objects from a class. Each object has all the properties and methods defined in the class, but they will have different property values.
Objects of a class are created using the new keyword.
In the example below, $apple and $banana are instances of the class Fruit:
In the example below, we add two more methods to class Fruit, for setting and getting the $color property:
Advertisement
PHP - The $this Keyword
The $this keyword refers to the current object, and is only available inside methods.
Look at the following example:
So, where can we change the value of the $name property? There are two ways:
1. Inside the class (by adding a set_name() method and use $this):
2. Outside the class (by directly changing the property value):
PHP - instanceof
You can use the instanceof keyword to check if an object belongs to a specific class:

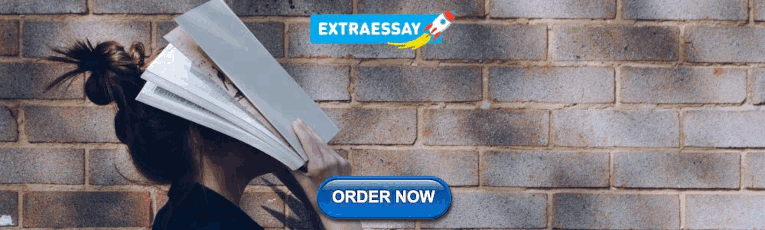
COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Modern PHP relies heavily on an object oriented style for structuring code. You'll find this style in many libraries, frameworks and projects.
What is an object?
In PHP object is one of the primitive types. It describes a value that is a bundle of data and often also logic to work on that data.
An object lets you handle complex data structures as single values. Let's take an address for example. It consists of several pieces of data:
- house number
- postal code
With the simpler primitive types, we can't store an address in a meaningful way so we need a data structure to store an address in one single value to make sure, we keep track of every piece of data that belongs to it.
We also need to make sure that all addresses have the same structure, so that the parts of our application that handle addresses can rely on. For example, the postal_code property of an address should always have the same name. If it's called zip_code sometimes, it leads to inconsistency and potentially dangerous bugs.
To ensure a certain object structure, we use "class", a kind of blueprint for an object.
This is a class definition. It introduces a class called Address and defines that all objects of this class have the same five properties that we need to store an address.
Class names are written in a style called StudlyCaps : like MyAwesomeClass .
To make an address object from this class, we use the new operator:
The output tells us, it is an object of the class Address and it has 5 properties. The #1 is an internal ID that PHP assigns to every object to keep track of them.
Now, every property of our object is NULL which is PHP's special value for "there's nothing here". To fill this address value with actual data, we could set every single property individually:
To access a property of an object, PHP uses the -> operator, so $my_address->street means "the street property of the object in the variable $my_address ".
But setting every single property is tedious and not very easy to read. What if we could set it all at once? We'll need to add something to the class to do that.
What we added, is a method. A function that is added to every object of our class. Inside a method, there's a special variable called $this , it refers to the object that the method was called on, in this case, the address object.
The set method just copies the values that it got via its arguments to the corresponding object properties.
Constructors
Making an address object without any data in the first place doesn't really make sense. It would be useful, and simpler to use, if we could set the data right when we create the object. There's a special method that does that, it's called a "constructor".
The output will remain the same again. But this time, we didn't need to call an extra method to initialize our object. If a class has a method with the exact name __construct , it will be called when the object is created with the new operator. All arguments from the new call will also be passed to the constructor.
Right now everything in our Address objects is accessible from the outside. A piece of code that doesn't behave well could do this:
That function would modify an address after it has been created and filled with data. That doesn't make sense, a place doesn't change its address, streets don't magically move to a different city. People can move to a new address but the address itself can't change. We need to prevent the address from being changed after it's created. That's where the concept of "visibility" comes in. You might have wondered, what all the public keywords were for until now:
Now we changed the visibility of our address' properties to protected . The do_bad_things function from before will now fail:
There are three levels of visibility in PHP: public , protected and private . For now, public means "is accessible from outside the object and protected means "is not accessible from the outside". We'll go into more detail on this and what private means when we talk about inheritance later.
Making Addresses useful again
Now we have a nice immutable (not changeable) Address object but it can't do anything. We can't even read the data from it. Let's say we need these addresses in plain text form, maybe to send them in an email or to send them to the shipping company for our shop website.
We'll add a method that gives us the address data in a nicely formatted piece of text:
Now we can create an address and get the formatted text representation of it:
And the output will be:
We can make this more convenient by using another "magic method". PHP classes have a bunch of special methods that all start with __ and are executed automatically at certain points, __construct() is also one of them.
This time we will make a __toString() method from our getAsText() . It is called every time when our object is used as if it were a string, for example when we try echo $my_address; .
Now we can just use the address as if it were already a string:
This will produce the same output as before.
We now have a class for address objects that bundles data (the properties) and some logic (the constructor and toString method) and is protected from outside modification.
While properties and methods usually belong to instances of classes, there are cases when a class itself needs to hold values in properties or offer methods. Those are called static properties and methods:
We can call the do_something() method without making an instance of Foo at all. Instead of -> we use :: to access a class' static properties and methods.
The visibility modifiers ( public , protected and private ) apply in the same way as for instances.
Static access is less common in PHP as it often introduces similar problems as global variables: a class with a static property is basically the same as a global variable and can make it very hard to keep track of where it is changed and where it is read from.
A blog about all things code
Accessing private properties in PHP
June 09, 2018 - Last modified at May 19, 2023
Private properties can only be accessed by the class that defines the property… right? Actually, PHP has a few ways to circumvent this: reflection, closures and array casting.
Reflection: slow, but clean
PHP provides a reflection API to retrieve metadata of classes, methods, interfaces, and so on. Of special interest to us is the ReflectionProperty class. Among other things, it has the wonderful method setAccessible .
In our code samples, we’re going to try to retrieve and change the property $property of the following class:
Using reflection, we can access the property using a clean API. Both reading the property and changing it are possible after a call to setAccessible .
Closures: an interesting alternative
PHP uses a class to represent functions: Closure . While originally closures were just an implementation detail, they were officially added to the spec in PHP 5.4. As it turns out, closures can be abused to both read and write private properties. Marco Pivetta explains this in detail in his blog post .
The idea is to create a getter using a closure, and then bind it to the class you want to access.
While this is pretty cool, it gets even better. You can retrieve the private property by reference . So not only will you be able to retrieve its value, you’ll be able to change it as well. Neat!
Casting to array
As a last option, we’ll abuse PHP’s implementation of casting an object to an array. When you cast an object to an array, all properties get exposed. Let’s see it in action:
As you can see, PHP uses a null character to separate the visibility scope from the property name.
- For public properties, only the property name is used as the key.
- Protected properties get * as their visibility scope.
- Private properties are prefixed by a fully qualified class name.
While this is technically undefined behaviour, a test case has been created to ensure it doesn’t get changed. Knowing this, we can create a way to access any property we want.
So, we can read properties using this method. But what about writing? One option is to use unserialize . When you serialize an object, the string representation is basically that of a serialized array, prefixed with the class name. A comparison:
Knowing this, we can fabricate a string to pass to unserialize . The result will be an new object with our new value for its property.
This is far from ideal however. unserialize is slow , and the resulting object is a new instance. This makes it pretty useless. On top of that, the code needed to achieve this looks really ugly.
We can do better than this. You probably know about array_walk . It walks over each element of an array, and applies a callback to it. This array is passed by reference. That’s cool and all, but how is this going to solve our problem? Well, apparently you can pass an object to array_walk without PHP complaining. It will implicitly get cast to an array, but , the values can be retrieved by reference. Interesting! We now know enough to set properties as well.
One note here: we’re abusing undefined behaviour. There is no guarantee this will continue to work in future PHP versions.
Performance
So, we’ve got a couple of ways to access and even mutate private properties. But what about performance? I’ve put together some benchmarking code to test this. While not 100% accurate, it gives us an indication about the relative performance. The results for 1 million iterations:
This shows us a couple of things:
- Array casting is the fastest way to read private properties, and array_walk comes out as the fastest way to write to them.
- Reflection is ~4.5 times slower than using a simple getter or setter.
- unserialize is slow , about 10 times slower than using a setter.
Conclusion
If you’re concerned about speed , cast your object to an array to read it, and use array_walk to write to it. It’s really fast! However, if you’re writing production code , consider if you really want to do this. Properties are private for a reason! If you have a valid use case, go for reflection. Your code will be more readable and thus easier to maintain. Plus, its behaviour is well-defined and documented. The performance gain isn’t worth sacrificing this for.
PHP: How to access properties and methods of an object
Understanding how to interact with objects is fundamental in object-oriented programming. In PHP, accessing properties and methods of an object is straightforward and allows for encapsulated, organized code. This tutorial will guide you through the principles and practices with in-depth examples.
Introduction to PHP Objects
Objects in PHP are instances of classes, which can contain properties (attributes) and methods (functions). To create an object, you need to define a class and use the new keyword. Here’s a basic example:
To access the properties and methods of this Car object, you use the ‘->’ (arrow) operator:
Accessing Public Properties and Methods
Public properties and methods are the most accessible members of an object, as they can be accessed anywhere:
Handling Private and Protected Members
Not all properties and methods are publicly accessible. private and protected members have restricted access, and PHP provides ways to work with these encapsulated elements:
Such methods are commonly referred to as getters and setters .
Navigating Inheritance and Overriding Methods
Inheritance is a core concept in object-oriented programming. Let’s see how a derived class can access and override methods from a base class:
Understanding Magic Methods
Magic methods, such as __get() and __set() , can be used for property overloading where properties don’t actually exist. Let’s see how they are implemented:
Accessing Static Properties and Methods
Static members belong to the class rather than an instance of the class. Here’s how you access static properties and methods:
Working with Interfaces and Abstract Classes
PHP also supports interfaces and abstract classes for defining contracts for other classes. Members of interfaces and abstract classes can be accessed like any other class, provided the concrete class implements all abstract methods:
Using Objects as Types
Type hinting allows you to specify that a function expects an object of a certain type as an argument or return:
Object Iteration with Iterators
PHP provides the Iterator interface for stepping through custom object properties during a loop. Here’s a glimpse into how you might implement iteration in an object:
This tutorial has explored different ways to access and interact with objects in PHP. From essential object manipulation, managing encapsulation through visibility, to advanced topics like inheritance, magic methods, and interfaces, you’re now more equipped to write flexible and robust PHP code.
Next Article: How to Insert an Element into an Array in PHP
Previous Article: How to fix PHP error: Calling a member function on a non-object
Series: PHP Data Structure Tutorials
Related Articles
- Using cursor-based pagination in Laravel + Eloquent
- Pandas DataFrame.value_counts() method: Explained with examples
- Constructor Property Promotion in PHP: Tutorial & Examples
- Understanding mixed types in PHP (5 examples)
- Union Types in PHP: A practical guide (5 examples)
- PHP: How to implement type checking in a function (PHP 8+)
- Symfony + Doctrine: Implementing cursor-based pagination
- Laravel + Eloquent: How to Group Data by Multiple Columns
- PHP: How to convert CSV data to HTML tables
- Using ‘never’ return type in PHP (PHP 8.1+)
- Nullable (Optional) Types in PHP: A practical guide (5 examples)
- Explore Attributes (Annotations) in Modern PHP (5 examples)
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
- Function Reference
- Variable and Type Related Extensions
The ReflectionProperty class
(PHP 5, PHP 7, PHP 8)
Introduction
The ReflectionProperty class reports information about class properties.
Class synopsis
Name of the property. Read-only, throws ReflectionException in attempt to write.
Name of the class where the property is defined. Read-only, throws ReflectionException in attempt to write.
Predefined Constants
Reflectionproperty modifiers.
Indicates static properties. Prior to PHP 7.4.0, the value was 1 .
Indicates readonly properties. Available as of PHP 8.1.0.
Indicates public properties. Prior to PHP 7.4.0, the value was 256 .
Indicates protected properties. Prior to PHP 7.4.0, the value was 512 .
Indicates private properties. Prior to PHP 7.4.0, the value was 1024 .
Note : The values of these constants may change between PHP versions. It is recommended to always use the constants and not rely on the values directly.
Table of Contents
- ReflectionProperty::__clone — Clone
- ReflectionProperty::__construct — Construct a ReflectionProperty object
- ReflectionProperty::export — Export
- ReflectionProperty::getAttributes — Gets Attributes
- ReflectionProperty::getDeclaringClass — Gets declaring class
- ReflectionProperty::getDefaultValue — Returns the default value declared for a property
- ReflectionProperty::getDocComment — Gets the property doc comment
- ReflectionProperty::getModifiers — Gets the property modifiers
- ReflectionProperty::getName — Gets property name
- ReflectionProperty::getType — Gets a property's type
- ReflectionProperty::getValue — Gets value
- ReflectionProperty::hasDefaultValue — Checks if property has a default value declared
- ReflectionProperty::hasType — Checks if property has a type
- ReflectionProperty::isDefault — Checks if property is a default property
- ReflectionProperty::isInitialized — Checks whether a property is initialized
- ReflectionProperty::isPrivate — Checks if property is private
- ReflectionProperty::isPromoted — Checks if property is promoted
- ReflectionProperty::isProtected — Checks if property is protected
- ReflectionProperty::isPublic — Checks if property is public
- ReflectionProperty::isReadOnly — Checks if property is readonly
- ReflectionProperty::isStatic — Checks if property is static
- ReflectionProperty::setAccessible — Set property accessibility
- ReflectionProperty::setValue — Set property value
- ReflectionProperty::__toString — To string
Improve This Page
User contributed notes 2 notes.

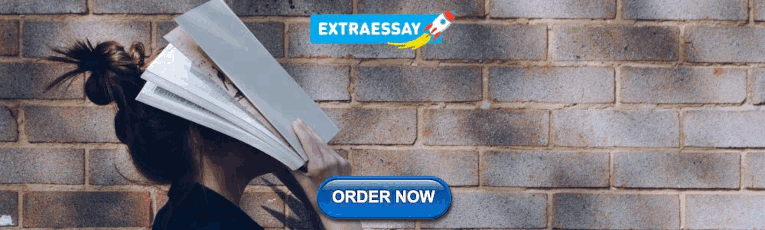
IMAGES
VIDEO
COMMENTS
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
Properties. Class member variables are called properties.They may be referred to using other terms such as fields, but for the purposes of this reference properties will be used. They are defined by using at least one modifier (such as Visibility, Static Keyword, or, as of PHP 8.1.0, readonly), optionally (except for readonly properties), as of PHP 7.4, followed by a type declaration, followed ...
Converting to object ¶. If an object is converted to an object, it is not modified. If a value of any other type is converted to an object, a new instance of the stdClass built-in class is created. If the value was null, the new instance will be empty. An array converts to an object with properties named by keys and corresponding values.
The First Steps Setup PHP on Windows Setup PHP on MacOS Set Up PHP in Ubuntu Upgrade PHP Windows Upgrade PHP in MacOS ... This tutorial will teach you several ways to add properties to PHP objects. These methods range from directly setting a property on an instance of a stdClass to leveraging the magic __set method within a class definition ...
In PHP, you can create a new property for an object dynamically by using the magic method __set. Books. Learn HTML Learn CSS Learn Git Learn Javascript Learn PHP Learn python Learn Java. ... In PHP, you can create a new property for an object dynamically by using the magic method __set. Here's an example: <?php class MyClass ...
Summary. Objects have states and behaviors. A class is a blueprint for creating objects. Properties represent the object's state, and methods represent the object's behavior. Properties and methods have visibility. Use the new keyword to create an object from a class.
What are Objects in PHP? Objects in PHP are instances of classes. A class is a blueprint for creating objects that defines their properties and methods. Properties are variables that hold data, while methods are functions that operate on that data. When you create an object, you're creating an instance of a class with its own properties and ...
One of the key-points of PHP OOP that is often mentioned is that "objects are passed by references by default". This is not completely true. This section rectifies that general thought using some examples. A PHP reference is an alias, which allows two different variables to write to the same value. In PHP, an object variable doesn't contain the ...
Define Objects. Classes are nothing without objects! We can create multiple objects from a class. Each object has all the properties and methods defined in the class, but they will have different property values.
In PHP object is one of the primitive types. It describes a value that is a bundle of data and often also logic to work on that data. An object lets you handle complex data structures as single values. Let's take an address for example. It consists of several pieces of data: street. house number. city. postal code.
As you can see, PHP uses a null character to separate the visibility scope from the property name. For public properties, only the property name is used as the key. Protected properties get * as their visibility scope. Private properties are prefixed by a fully qualified class name.
Object Iteration. PHP provides a way for objects to be defined so it is possible to iterate through a list of items, with, for example a foreach statement. By default, all visible properties will be used for the iteration. Example #1 Simple Object Iteration.
Introduction to PHP Objects. Objects in PHP are instances of classes, which can contain properties (attributes) and methods (functions). To create an object, you need to define a class and use the new keyword. Here's a basic example:
PHP __get and __set magic methods. The __get and __set magic methods in PHP are used to intercept calls to get or set the value of inaccessible properties of an object. They allow you to define custom behaviors when getting or setting the value of a property that is not directly accessible. Here is an example of how you can use the __get and ...
Notes. Note: . Using this function will use any registered autoloaders if the class is not already known. Note: . The property_exists() function cannot detect properties that are magically accessible using the __get magic method.
Version Description; 8.3.0: Calling this method with a single argument is deprecated, ReflectionClass::setStaticPropertyValue() should be used instead to modify static properties. 8.1.0: Private and protected properties can be accessed by ReflectionProperty::setValue() right away. Previously, they needed to be made accessible by calling ReflectionProperty::setAccessible(); otherwise a ...
PHP - set object property within another object's array. 1. How to dynamically set an object property value? Hot Network Questions What's the name of the room where you watch a movie inside the movie theater? What size wire in conduit before feeding 20A nm circuit? ...
As noted by others, you will want to set the property to emptyish values, certainly 0 and "" and []. Null seems the best, although it still precludes actually setting the property to null. I can't see an easy way around that ATM, other than another method, or not doing the getset single function.
Prior to PHP 7.4.0, the value was 1. ReflectionProperty::IS_READONLY. ... ReflectionProperty::setValue — Set property value; ReflectionProperty::__toString — To string; ... The fact that it accepts an object as argument is just a convenience feature - you are actually inspecting the class of that object, not the object itself, so it's ...
I am running into a REALLY odd issue, and my best guess is that it's a bug within PHP itself. When comparing (===) the value of a property on one object with the value of a property on another object, all the values of the properties on one of the objects are deleted. PHP 8.3.2-1+0~20240120.16+debian11~1.gbpb43448; Laravel Framework 11.4.0