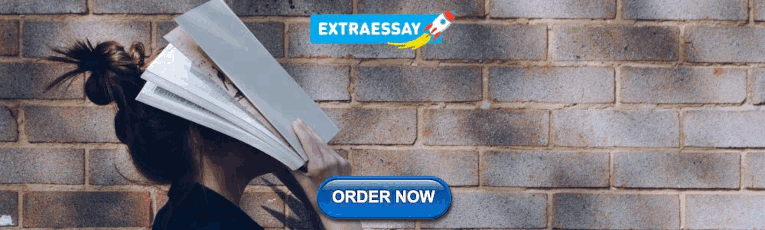
Crafting a Task.TimeoutAfter Method
November 10th, 2011 0 0
Imagine that you have a Task handed to you by a third party, and that you would like to force this Task to complete within a specified time period. However, you cannot alter the “natural” completion path and completion state of the Task, as that may cause problems with other consumers of the Task. So you need a way to obtain a copy or “proxy” of the Task that will either (A) complete within the specified time period, or (B) will complete with an indication that it had timed out.
In this blog post, I will show how one might go about implementing a Task.TimeoutAfter method to support this scenario. The signature of TimeoutAfter would look like this:
The returned proxy Task can complete in one of two ways:
- If task completes before the specified timeout period has elapsed, then the proxy Task finishes when task finishes, with task’s completion status being copied to the proxy.
- If task fails to complete before the specified timeout period has elapsed, then the proxy Task finishes when the timeout period expires, in Faulted state with a TimeoutException.
In addition to showing how to implement Task.TimeoutAfter, this post will also shed some light on the general thought process that should go into implementing such a feature.
A First Try
Here’s some code that will do the trick:
Simple enough, right? You start a Timer job that faults the proxy Task, and also add a continuation off of the source Task that transfers the completion state of the source to the proxy. The final state of the proxy will therefore depend on which completes first, the Timer job or the source Task.
And by the way, MarshalTaskResults is implemented like this:
he “RanToCompletion” handling might seem a little more complicated than it needs to be, but it will allow us to handle Task<TResult> objects correctly (discussed briefly below).
Can We Do Better?
While our first stab at a TimeoutAfter method is functionally correct, we could streamline it and improve its performance. Specifically, notice that our Timer and continuation delegates “capture” variables; this will cause the compiler to allocate special “closure” classes for these delegates behind the scenes, which will slow down our method. To eliminate the need for the closure class allocations, we can pass all “captured” variables in through state variables for those respective calls, like this:
Some ad-hoc performance tests show that this little optimization shaves about 12% off of the overhead from the TimeoutAfter method.
What about Edge Cases?
What do we do when the caller specifies a zero timeout, or an infinite timeout? What if the source Task has already completed by the time that we enter the TimeoutAfter method? We can address these edge cases in the TimeoutAfter implementation as follows:
Such changes ensure efficient handling of the edge cases associated with TimeoutAfter.
A Different Approach
My colleague Stephen Toub informed me of another potential implementation of Task.TimeoutAfter:
The implementation above takes advantage of the new async/await support in .NET 4.5, and is pleasingly concise. However, it does lack some optimizations:
- The edge cases described previously are not handled well. (But that could probably be fixed.)
- A Task is created via Task.Delay, instead of just a simple timer job.
- In the cases where the source Task (task) completes before the timeout expires, no effort is made to cancel the internal timer job that was launched in the Task.Delay call. If the number of “zombie” timer jobs starts becoming significant, performance could suffer.
Nevertheless, it is good to consider the use of async/await support in implementing features like this. Often await will be optimized in ways that simple continuations are not.
What about TimeoutAfter<TResult>?
Suppose that we want to implement the generic version of TimeoutAfter?
It turns out that the implementation of the above would be nearly identical to the non-generic version, except that a TaskCompletionSource<TResult> would be used instead of a TaskCompletionSource<VoidTypeStruct>. The MarshalTaskResults method was already written to correctly handle the marshaling of the results of generic Tasks.
[1] There is no non-generic version of TaskCompletionSource<TResult>. So, if you want a completion source for a Task (as opposed to a Task<TResult>), you still need to provide some throwaway TResult type to TaskCompletionSource. For this example, we’ve created a dummy type (VoidTypeStruct), and we create a TaskCompletionSource<VoidTypeStruct>.
[2] So does it really buy you anything to replace a closure allocation with a tuple allocation? The answer is “yes”. If you were to examine the IL produced from the original code, you would see that both a closure object and a delegate need to be allocated for this call. Eliminating variable capture in the delegate typically allows the compiler to cache the delegate, so in effect two allocations are saved by eliminating variable capture. Thus in this code we’ve traded closure and delegate allocations for a Tuple allocation, so we still come out ahead.
Discussion is closed.

Siderite's Blog
Some features of this website do not work as expected when JavaScript is disabled
How to timeout a task AND make sure it ends
- programming
- you control what the task does
- you can split the operation into small chunks that are either executed sequentially or in a loop so you interrupt their flow
Be the first to post a comment
Post a comment
Enable JavaScript to post comments on this blog.
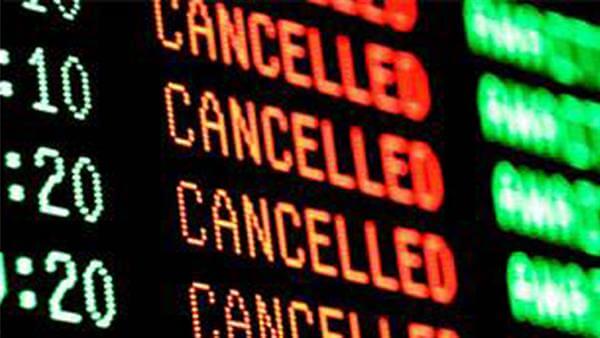
Andrew Lock | .NET Escapades Andrew Lock
- .NET Core 6
- Source Generators
Cancelling await calls in .NET 6 with Task.WaitAsync()
In this post I discuss the new Task.WaitAsync() APIs introduced in .NET 6, how you can use them to "cancel" an await call, and how they can replace other approaches you may be using currently.
The new Task.WaitAsync API in .NET 6
In a recent post , I described how to use a TaskCompletionSource with IHostApplicationLifetime as a way of "pausing" a background service until the application starts up. In that code I used the following function that waits for a TaskCompletionSource.Task to complete, but also supports cancellation via a CancellationToken :
This code works on many versions of .NET, but in the post I specifically mentioned that this was talking about .NET 6, so Andreas Gehrke pointed out that I could have used a simpler approach:
Great post! Since this is .NET 6 couldn’t you just call WaitAsync() on your tcs and pass the stoppingToken? — Andreas Gehrke (@agehrke) February 15, 2022
Andreas is referring to a new API introduced to the Task (and Task<T> ) API, which allows you to await a Task while also making that await cancellable:
As you can see, there are three new methods added to Task , all are overloads of WaitAsync() . This is useful for the exact scenario I described earlier—you want to await the completion of a Task , but want that await to be cancellable by a CancellationToken .
Based on this new API, we could rewrite the WaitForAppStartup function as the following:
I think this is much easier to read, so thanks Andreas for pointing it out!
Awaiting a Task with a timeout
The Task.WaitAsync(CancellationToken cancellationToken) method (and its counterpart on Task<T> ) is very useful when you want to make an await cancellable by a CancellationToken . The other overloads are useful if you want to make it cancellable based on a timeout.
For example, consider the following pseudo code:
This code shows a single public method, with two local functions:
- GetResult() returns the result of an expensive operation, the result of which may be cached
- LoadFromCache() returns the result from a cache, with a short delay
- LoadDirectly() returns the result from the original source, which takes a lot longer
This code is pretty typical for when you need to cache the result of an expensive operation. But note that the "caching API" in this example is async . This could be because you're using the IDistributedCache in ASP.NET Core for example.
If all goes well then calling GetResult() multiple times should work like the following:
In this case, the cache is doing a great job speeding up subsequent requests for the result.
But what if something goes wrong with the distributed cache?
For example, maybe you're using Redis as a distributed cache , which most of the time is lighting-fast. But for some reason, your Redis server suddenly becomes unavailable: maybe the server crashes, there's network problems, or the network just becomes very slow.
Suddenly, your LoadFromCache() method is actually making the call to GetResult() slower , not faster!😱
Ideally, you want to be able to say "Try and load this from the cache, but if it takes longer than x milliseconds, then stop trying". i.e. you want to set a timeout.
Now, you may well be able to add a sensible timeout within the Redis connection library itself, but assume for a moment that you can't, or that your caching API doesn't provide any such APIs. In that case, you can use .NET 6's Task<T>.WaitAsync(TimeSpan) :
With this change, GetResult() won't wait longer than 100ms for the cache to return. If LoadFromCache() exceeds that timeout, Task.WaitAsync() throws a TimeoutException and the function immediately loads from LoadDirectly() instead.
Note that if you're using the CancellationToken overload of WaitAsync() , you'll get a TaskCanceledException when the task is cancelled. If you use a timeout, you'll get TimeoutException .
If you wanted this behaviour before .NET 6, you could replicate it using an extension something like the following:
Having this code be part of the .NET base class library is obviously very handy, but it also helps avoid subtle bugs from writing this code yourself. In the extension above, for example, it would be easy to forget to cancel the Task.Delay() call. This would leak a Timer instance until the delay trigger fires in the background. In high-throughput code, that could easily become an issue!
On top of that, .NET 6 adds a further overload that supports both a timeout, and a CancellationToken , saving you one more extension method to write 🙂 In the next post I'll dive into how this is actually implemented under the hood, as there's a lot more to it than the extension method above!
In this post I discussed the new Task.WaitAsync() method overloads introduced in .NET 6, and how you can use them to simplify any code where you wanted to wait for a Task , but wanted the await to be cancellable either via a CancellationToken or after a specified timeout.
Popular Tags
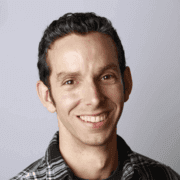
Stay up to the date with the latest posts!
This senator wants Canadian banks to fight climate change
Rosa galvez says financial sector needs to be more strongly regulated.
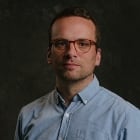
Social Sharing
Rosa Galvez has taken on a Herculean task: force Canadian financial institutions to prioritize the fight against climate change.
More than two years ago, the independent senator from Quebec proposed legislation that would force banks and pension funds to steer away from emissions-intensive investments, such as the oil and gas sector.
It would also increase regulatory oversight to determine whether the climate plans laid out by institutions are credible, and limit the presence of fossil fuel executives on the their boards.
The bill, known as the Climate-Aligned Finance Act , has moved slowly. The Senate banking committee only began hearing from witnesses late last year, and had another round of testimony earlier this month.
In a recent interview, Galvez was clear she's under no illusion the bill is likely to become law any time soon. But at the very least, she wants it to generate a debate about the role of the finance sector in climate change.
"I'm not asking for the moon," said Galvez. "I don't want the bill to be passed just like that, or to be rejected just like that."
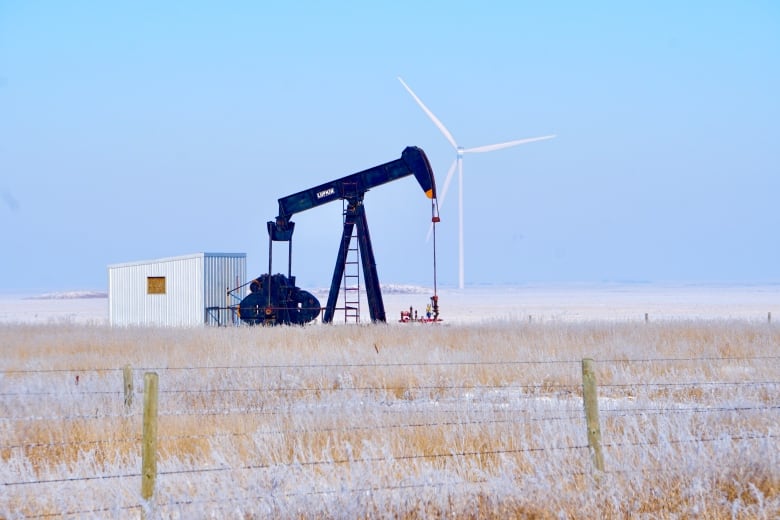
The missing link
Before being appointed to the Senate in 2016, Galvez, who is originally from Peru, was an engineer and professor at Université Laval à Québec for over 25 years.
She researched the impact of the oil spill in the Lac Megantic rail disaster and worked as a consultant on how to protect the Great Lakes and the St. Lawrence River.
As a senator, Galvez said her focus turned to banks and pension funds, which she views as a driving force of the transition toward renewable energy.
"You come to the conclusion that the part that is missing is the finance sector, which actually should be the first sector in order to start obtaining results," said Galvez.
The legislation would give the Office of the Superintendent of Financial Institutions, which regulates the financial sector, greater power to oversee the climate plans of financial institutions.
- Big Canadian banks may be making misleading claims on sustainability, says securities complaint
It would also require fossil fuel projects and other high-emissions sectors to be considered high risk, effectively requiring more capital to obtain a loan — and potentially a higher interest rate once one is secured.
There is logic in a high-risk designation because companies whose value relies on pulling oil, gas or coal from the ground may find their assets "stranded" in a low-carbon economy, said Olaf Weber, a sustainable finance professor at York University's Schulich School of Business.
"As a bank you have a five year loan to fossil fuel company, are the risks higher than in other industries? Probably not," he said.
"If you have a longer term connection with them, are the risks higher? Probably yes, because sooner or later we will see that income goes down or costs go up," he added, pointing to an eventual decline in demand and higher carbon price as factors.
The proposal enjoys wide support from environmental groups and has received backing from some Liberal, NDP and Bloc Quebecois MPs . But it has been met with pushback from financial institutions.
Canadian banks remain among the largest financiers of oil, gas and coal globally. A report released this month calculated that Canada's top banks pumped a combined $103.85 billion US into fossil fuel projects globally in 2023.
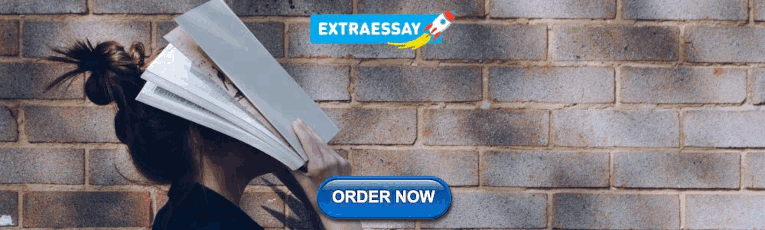
Banking industry voices concerns
Darren Hannah, senior-vice president of the Canadian Bankers Association (CBA), which represents the country's largest banks, was highly critical in his testimony before the Senate banking committee last November.
The bill, if put into law, would amount to a "de facto ban" on lending to fossil fuel companies because of rules that would require lending to be viewed as a much higher investment risk, Hannah said.
Such a change would be "fundamentally unfair to the thousands of Canadians who work in the energy sector and the energy companies that are building transition paths to a low-carbon future," he said.
He argued, as well, that this would "increase costs for most Canadians that rely on their vehicle for day-to-day needs or many who rely on hydrocarbon fuels to heat their homes."
Yrjo Koskinen, a sustainable finance professor at University of Calgary, questions whether the law would actually lead to a "de facto ban." Rather, he said it would would likely lead banks to charge higher interest rates to oil and gas companies.
Maggie Cheung, a CBA spokesperson, added in a statement that "Canada's banks understand the important role that the financial sector can play in facilitating an orderly transition to a low-carbon future."
"This includes working with clients across industries to help them decarbonize and pursue energy transition opportunities, and financing new and existing green projects that will help Canada meet its net-zero ambitions," Cheung said.
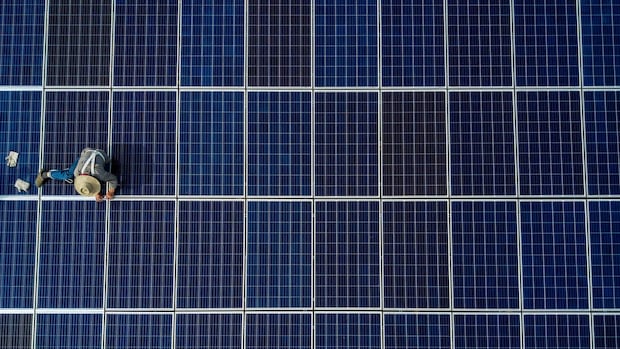
3 ways the world is making progress on green energy
Financial sector 'off track'.
Exactly how the financial sector will help drive this transition remains unclear. Julie Segal, who specializes in climate finance with the advocacy group Environmental Defence, said most Canadian financial institutions have not voluntarily shifted their portfolios away from polluting investments.
In 2021, Canada's five largest banks committed to reduce the climate-related emissions from their investment and loan portfolios to net-zero by 2050, but there has been backsliding in the years since, she said.
Between 2020 and 2022, those same five banks increased their fossil fuel financing exposure from an average of 16 per cent in 2020 to 18 per cent in 2022, facilitating a total of $275 billion US in financing to fossil fuel value chain companies over those three years, according to a recent report from InfluenceMap , a climate change think tank.
Segal pointed to the European Union, which announced a sustainable finance policy in its 2020 Green Deal aimed at reducing emissions, and the United Kingdom, which also has a green finance plan , as examples of jurisdictions further along.
"Right now, our financial sector at large remains off track," she said. "Almost all of our financial institutions have climate commitments, but none of them are on track to align with investing in a way that keeps a safe planet and builds climate resilience."
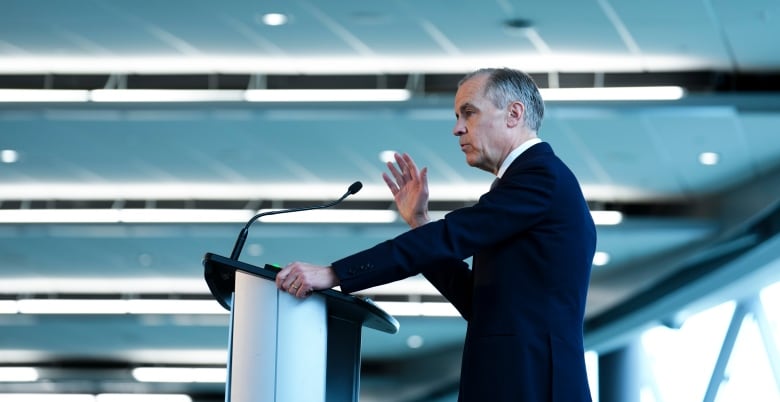
Similarly, Mark Carney, the former Bank of Canada governor and sometimes touted as a possible Liberal leader, testified before the committee earlier this month that "Canada is lagging its international peers" when it comes to sustainable finance.
"Canadian climate disclosure efforts have been patchwork, delivered late and falling short of international standards," said Carney, the UN Special Envoy on Climate Action and Finance.
- What on Earth? One worker's push to get her pension to divest from fossil fuels
For now, the Climate-Aligned Finance Act is still at the committee stage and faces several more hurdles before it would be put to a vote in the Senate, and then make its way to the House of Commons.
That all could take time, and with an election expected in 2025, it could become a campaign issue. For now, Galvez said "the first goal was just to educate everybody on these connections."
ABOUT THE AUTHOR
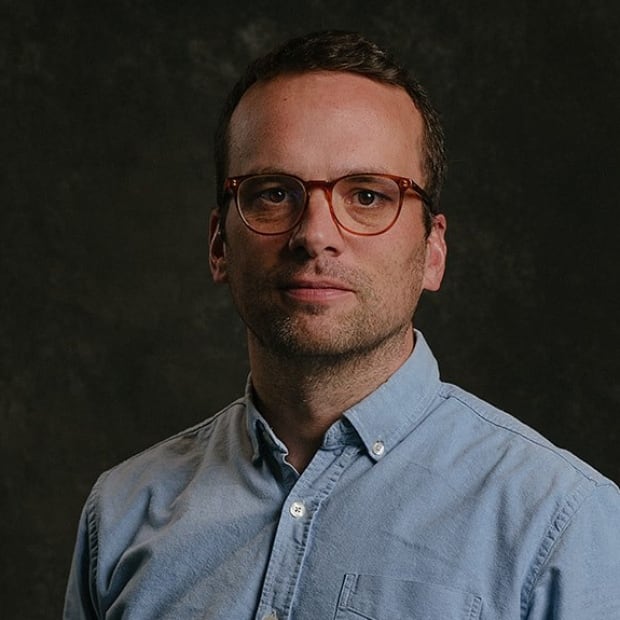
Benjamin Shingler is a senior writer based in Montreal, covering climate policy, health and social issues. He previously worked at The Canadian Press and the New Brunswick Telegraph-Journal.
Related Stories
- U.S. investors successfully demand RBC change how it reports on green, fossil fuel investments
- Canada's top pensions have trillions in assets. Should they do more to fuel the energy transition?
Add some “good” to your morning and evening.
The environment is changing. This newsletter is your weekly guide to what we’re doing about it.
This site is protected by reCAPTCHA and the Google Privacy Policy and Google Terms of Service apply.
Rescuing people buried in Papua New Guinea's landslide is a massive task — here's why
The landslide that struck Yambali village in Papua New Guinea's Enga province early on Friday morning came without apparent warning and while people slept.
As estimates for the number of villagers buried under the earth range from 670 to 2,000, people are being forced to use shovels, sticks and their bare hands to locate bodies and survivors.
While landslides have long affected countries with large mountainous areas and high rainfall such as Papua New Guinea, engineers and scientists say they are learning more about them.
It may be possible to use this knowledge to save those buried and prevent future disasters.
But there remains a lot of uncertainty about where and when landslides will strike, and rescue efforts come with great risks.
How hard is it to rescue people from landslides?
Rescuers need to consider the potential for more landslides and the instability of the debris.
To reach survivors in time, machinery is needed to remove the vast amounts of debris.
But this comes with risks, and machines need to be used carefully to avoid hitting any people buried underground or creating another landslide, according to Pierre Rognon, an expert in landslides and engineering at the University of Sydney.
He says it can also be harder to locate survivors of landslides than earthquakes.
"The landslide … carries with it everything and everyone — where exactly to, we don't know for sure," he said.
"So before even addressing the question of how do we get equipment to the [site], is it safe to go there, we don't even know where to put it.
"So we need to take a bet. We need the best guess of where the landslide might have gone and where the people got carried to."
Another difference from earthquakes affecting rescue efforts is there may be fewer air pockets letting survivors breathe under the debris.
One challenge in Enga province is the lack of infrastructure, but it's not insurmountable.
Engineering geologist Mark Eggers says it will take a couple of days for heavy vehicles to travel from Lae, the second-largest city in PNG, to Yambali in Enga.
There's also an airport at Mount Hagen that receives charter flights from Australia, he said.
The Australian government says it flew the first representatives of PNG's national disaster committee to the landslide site on a RAAF Spartan and helicopter.
"In addition to the disaster response experts who are on their way to PNG now, the emphasis is on helping those who are displaced, and so we are working on transporting 750 family-sized shelters to the site," Australian Defence Minister Richard Marles said on Tuesday.
Why do landslides happen?
There are two major natural triggers for landslides: earthquakes and heavy rain.
Earthquakes weaken the ground and send waves of stress through it, causing it to break up and slide.
Dr Rognon says rain can also double the weight of the ground and cause a landslide.
It's not clear what triggered the landslide at Yambali village. However, there was no apparent earthquake.
Another factor common to landslides is steep terrain, and PNG's makes it vulnerable.
University of Adelaide geologist Alan Collins says while an earthquake doesn't appear to have caused this landslide directly, tectonic activity has had an influence in the long term.
"The reason we have such steep topography and steep slopes and high mountains … is because of the active tectonics, because of these earthquakes that happen very, very commonly," he said.
Are landslides all the same?
Landslides can happen in many ways depending on their cause and the materials falling down.
The land can slide, topple, flow, or break off from a cliff face.
Sometimes, Dr Rognon says, the land "creeps" down a slope as slowly as one centimetre a year.
"The speed at which the landslide propagates, it can be as fast as a freefall, in which case we've got no chance to escape," he said.
Dr Rognon says the risk the landslide poses depends on the material falling down, which can include clay, sand, fine particles or dirt.
Earth scientist Dave Petley has previously written this is all critical knowledge in rescue efforts — determining how, where and why people might survive.
Mr Eggers says the disaster in Enga province can be described as a "rock slide".
Can they be prevented?
Landslides could be prevented if they were "fairly small", according to Dr Rognon.
To stop harm from small landslides that involve a cubic metre of material — enough to crush a car or block a road — there are engineering techniques that can help.
"We can build retaining walls, we can batter the slopes to make them less steep," he said.
"And that could work fairly well to stabilise a range of slopes."
Once a landslide gets going, it is harder to catch with barriers.
Dr Rognon says a landslide a few cubic metres in size — weighing a few tonnes and travelling at the speed of a car — needs a big structure to catch it.
And he says there's no stopping large landslides, which may have a million cubic metres of material.
"No-one will be able to prevent it from falling, no-one will be able to prevent it from going where it needs to go," he said.
Can they be predicted?
Geologists and geotechnical engineers have tools that help predict whether a landslide in an area may be large or small.
If they know the materials in the slope, and their strength, they can use software to calculate the size of a potential landslide.
But it's not that easy.
That's because it's hard to tell what kind of material is deeper underground.
"We are talking about geological strata of hundreds of metres that can be anything from a very solid intact rock to a very soft clay," he said.
"It can be sand, it can be weathered rocks, or rocks with lots of fractures that had been broken due to stresses or simply water ingress.
"All those materials, they will have different strengths."
This is critical information to have — without it, geologists and engineers will miss the weak link in the land that will cause a landslide.
"We've got to come up with some scenarios, what if it's weak, what if it's strong, and then run our models, and then take our best bet," Dr Rognon said.
But he says despite the uncertainty, experts can still gather enough information to warn communities about potentially large landslides.
- X (formerly Twitter)
Related Stories
Why does papua new guinea experience so many fatal landslides — and what can be done to help.
Woman loses 18 members of her family in PNG landslide as hopes of finding survivors fade
People using bare hands to recover villagers in PNG landslide, with fears of up to 2,000 people buried
- Disaster Preparedness
- Disaster Relief
- Disaster and Emergency Response
- Earthquakes
- Engineering
- Papua New Guinea
Mobile Menu Overlay
The White House 1600 Pennsylvania Ave NW Washington, DC 20500
FACT SHEET: Biden- Harris Administration Announces New Principles for High-Integrity Voluntary Carbon Markets
Since Day One, President Biden has led and delivered on the most ambitious climate agenda in history, including by securing the Inflation Reduction Act, the largest-ever climate investment, and taking executive action to cut greenhouse gas emissions across every sector of the economy. The President’s Investing in America agenda has already catalyzed more than $860 billion in business investments through smart, public incentives in industries of the future like electric vehicles (EVs), clean energy, and semiconductors. With support from the Bipartisan Infrastructure Law, CHIPS and Science Act, and Inflation Reduction Act, these investments are creating new American jobs in manufacturing and clean energy and helping communities that have been left behind make a comeback.
The Biden-Harris Administration is committed to taking ambitious action to drive the investments needed to achieve our nation’s historic climate goals – cutting greenhouse gas emissions in half by 2030 and reaching net zero by 2050. President Biden firmly believes that these investments must create economic opportunities across America’s diverse businesses – ranging from farms in rural communities, to innovative technology companies, to historically- underserved entrepreneurs.
As part of this commitment, the Biden-Harris Administration is today releasing a Joint Statement of Policy and new Principles for Responsible Participation in Voluntary Carbon Markets (VCMs) that codify the U.S. government’s approach to advance high-integrity VCMs. The principles and statement, co-signed by Treasury Secretary Janet Yellen, Agriculture Secretary Tom Vilsack, Energy Secretary Jennifer Granholm, Senior Advisor for International Climate Policy John Podesta, National Economic Advisor Lael Brainard, and National Climate Advisor Ali Zaidi, represent the U.S. government’s commitment to advancing the responsible development of VCMs, with clear incentives and guardrails in place to ensure that this market drives ambitious and credible climate action and generates economic opportunity.
The President’s Investing in America agenda has crowded in a historic surge of private capital to take advantage of the generational investments in the Inflation Reduction Act and Bipartisan Infrastructure Law. High-integrity VCMs have the power to further crowd in private capital and reliably fund diverse organizations at home and abroad –whether climate technology companies, small businesses, farmers, or entrepreneurs –that are developing and deploying projects to reduce carbon emissions and remove carbon from the atmosphere. However, further steps are needed to strengthen this market and enable VCMs to deliver on their potential. Observers have found evidence that several popular crediting methodologies do not reliably produce the decarbonization outcomes they claim. In too many instances, credits do not live up to the high standards necessary for market participants to transact transparently and with certainty that credit purchases will deliver verifiable decarbonization. As a result, additional action is needed to rectify challenges that have emerged, restore confidence to the market, and ensure that VCMs live up to their potential to drive climate ambition and deliver on their decarbonization promise. This includes: establishing robust standards for carbon credit supply and demand; improving market functioning; ensuring fair and equitable treatment of all participants and advancing environmental justice, including fair distribution of revenue; and instilling market confidence.
The Administration’s Principles for Responsible Participation announced today deliver on this need for action to help VCMs achieve their potential. These principles include:
- Carbon credits and the activities that generate them should meet credible atmospheric integrity standards and represent real decarbonization.
- Credit-generating activities should avoid environmental and social harm and should, where applicable, support co-benefits and transparent and inclusive benefits-sharing.
- Corporate buyers that use credits should prioritize measurable emissions reductions within their own value chains.
- Credit users should publicly disclose the nature of purchased and retired credits.
- Public claims by credit users should accurately reflect the climate impact of retired credits and should only rely on credits that meet high integrity standards.
- Market participants should contribute to efforts that improve market integrity.
- Policymakers and market participants should facilitate efficient market participation and seek to lower transaction costs.
The Role of High-Quality Voluntary Carbon Markets in Addressing Climate Change President Biden, through his executive actions and his legislative agenda, has led and delivered on the most ambitious climate agenda in history. Today’s release of the Principles for Responsible Participation in Voluntary Carbon Markets furthers the President’s commitment to restoring America’s climate leadership at home and abroad by recognizing the role that high- quality VCMs can play in amplifying climate action alongside, not in place of, other ambitious actions underway.
High-integrity, well-functioning VCMs can accelerate decarbonization in several ways. VCMs can deliver steady, reliable revenue streams to a range of decarbonization projects, programs, and practices, including nature-based solutions and innovative climate technologies that scale up carbon removal. VCMs can also deliver important co-benefits both here at home and abroad, including supporting economic development, sustaining livelihoods of Tribal Nations, Indigenous Peoples, and local communities, and conserving land and water resources and biodiversity. Credit-generating activities should also put in place safeguards to identify and avoid potential adverse impacts and advance environmental justice.
To deliver on these benefits, VCMs must consistently deliver high-integrity carbon credits that represent real, additional, lasting, unique, and independently verified emissions reductions or removals. Put simply, stakeholders must be certain that one credit truly represents one tonne of carbon dioxide (or its equivalent) reduced or removed from the atmosphere, beyond what would have otherwise occurred. In addition, there must be a high level of “demand integrity” in these markets. Credit buyers should support their purchases with credible, scientifically sound claims regarding their use of credits. Purchasers and users should prioritize measurable and feasible emissions reductions within their own value chains and should not prioritize credit price and quantity at the expense of quality or engage in “greenwashing” that undercuts the decarbonization impact of VCMs. The use of credits should complement, not replace, measurable within-value-chain emissions reductions.
VCMs have reached an inflection point. The Biden-Harris Administration believes that VCMs can drive significant progress toward our climate goals if action is taken to support robust markets undergirded by high-integrity supply and demand. With these high standards in place, corporate buyers and others will be able to channel significant, necessary financial resources to combat climate change through VCMs. A need has emerged for leadership to guide the development of VCMs toward high-quality and high-efficacy decarbonization actions. The Biden-Harris Administration is stepping up to meet that need.
Biden-Harris Administration Actions to Develop Voluntary Carbon Markets
These newly released principles build on existing and ongoing efforts across the Biden-Harris Administration to encourage the development of high-integrity voluntary carbon markets and to put in place the necessary incentives and guardrails for this market to reach its potential. These include:
- Creating New Climate Opportunities for America’s Farmers and Forest Landowners. Today, The Department of Agriculture’s (USDA) Agricultural Marketing Service (AMS) published a Request for Information (RFI) in the Federal Register asking for public input relating to the protocols used in VCMs. This RFI is USDA’s next step in implementing the Greenhouse Gas Technical Assistance Provider and Third-Party Verifier Program as part of the Growing Climate Solutions Act. In February 2024, USDA announced its intent to establish the program, which will help lower barriers to market participation and enable farmers, ranchers, and private forest landowners to participate in voluntary carbon markets by helping to identify high-integrity protocols for carbon credit generation that are designed to ensure consistency, effectiveness, efficiency, and transparency. The program will connect farmers, ranchers and private landowners with resources on trusted third-party verifiers and technical assistance providers. This announcement followed a previous report by the USDA, The General Assessment of the Role of Agriculture and Forestry in the U.S. Carbon Markets , which described how voluntary carbon markets can serve as an opportunity for farmers and forest landowners to reduce emissions. In addition to USDA AMS’s work to implement the Growing Climate Solutions Act, USDA’s Forest Service recently announced $145 million inawards under President Biden’s Inflation Reduction Act to underserved and small- acreage forest landowners to address climate change, while also supporting rural economies and maintaining land ownership for future generations through participation in VCMs.
- Conducting First-of-its-kind Credit Purchases. Today, the Department of Energy (DOE) announced the semifinalists for its $35 million Carbon Dioxide Removal Purchase Pilot Prize whereby DOE will purchase carbon removal credits directly from sellers on a competitive basis. The Prize will support technologies that remove carbon emissions directly from the atmosphere, including direct air capture with storage, biomass with carbon removal and storage, enhanced weathering and mineralization, and planned or managed carbon sinks. These prizes support technology advancement for decarbonization with a focus on incorporating environmental justice, community benefits planning and engagement, equity, and workforce development. To complement this effort, the Department of Energy also issued a notice of intent for a Voluntary Carbon Dioxide Removal Purchase Challenge, which proposes to create a public leaderboard for voluntary carbon removal purchases while helping to connect buyers and sellers.
- Advancing Innovation in Carbon Dioxide Removal (CDR) Technology. Aside from direct support for voluntary carbon markets, the Biden-Harris Administration is investing in programs that will accelerate the development and deployment of critical carbon removal technologies that will help us reach net zero. For example, DOE’s Carbon Negative Shot pilot program provides $100 million in grants for small projects that demonstrate and scale solutions like biomass carbon removal and storage and small mineralization pilots, complementing other funding programs for small marine CDR and direct air capture pilots. DOE’s Regional Direct Air Capture Hubs program invests up to $3.5 billion from the Bipartisan Infrastructure Law in demonstration projects that aim to help direct air capture technology achieve commercial viability at scale while delivering community benefits. Coupled with DOE funding to advance monitoring, measurement, reporting, and verification technology and protocols and Department of the Treasury implementation of the expanded 45Q tax credit under the Inflation Reduction Act, the U.S. is making comprehensive investments in CDR that will enable more supply of high- quality carbon credits in the future.
- Leading International Standards Setting. Several U.S. departments and agencies help lead the United States’ participation in international standard-setting efforts that help shape the quality of activities and credits that often find a home in VCMs. The Department of Transportation and Department of State co-lead the United States’ participation in the Carbon Offsetting and Reduction Scheme for International Aviation (CORSIA), a global effort to reduce aviation-related emissions. The Department of State works bilaterally and with international partners and stakeholders to recognize and promote best practice in carbon credit market standard-setting—for example, developing the G7’s Principles for High-Integrity Carbon Markets and leading the United States’ engagement on designing the Paris Agreement’s Article 6.4 Crediting Mechanism . The U.S. government has also supported a number of initiatives housed at the World Bank that support the development of standards for jurisdictional crediting programs, including the Forest Carbon Partnership Facility and the Initiative for Sustainable ForestLandscapes, and the United States is the first contributor to the new SCALE trust fund.
- Supporting International Market Development. The U.S. government is engaged in a number of efforts to support the development of high-integrity VCMs in international markets, including in developing countries, and to provide technical and financial assistance to credit-generating projects and programs in those countries. The State Department helped found and continues to coordinate the U.S. government’s participation in the LEAF Coalition , the largest public-private VCM effort, which uses jurisdictional-scale approaches to help end tropical deforestation. The State Department is also a founding partner and coordinates U.S. government participation in the Energy Transition Accelerator, which is focused on sector-wide approaches to accelerate just energy transitions in developing markets. USAID also has a number of programs that offer financial aid and technical assistance to projects and programs seeking to generate carbon credits in developing markets, ensuring projects are held to the highest standards of transparency, integrity, reliability, safety, and results and that they fairly benefit Indigenous Peoples and local communities. This work includes the Acorn Carbon Fund, which mobilizes $100 million to unlock access to carbon markets and build the climate resilience of smallholder farmers, and supporting high-integrity carbon market development in a number of developing countries. In addition, the Department of the Treasury is working with international partners, bilaterally and in multilateral forums like the G20 Finance Track, to promote high-integrity VCMs globally. This includes initiating the first multilateral finance ministry discussion about the role of VCMs as part of last year’s Asia Pacific Economic Cooperation (APEC) forum.
- Providing Clear Guidance to Financial Institutions Supporting the Transition to Net Zero. In September 2023, the Department of the Treasury released its Principles for Net- Zero Financing and Investment to support the development and execution of robust net- zero commitments and transition plans. Later this year, Treasury will host a dialogue on accelerating the deployment of transition finance and a forum on further improving market integrity in VCMs.
- Enhancing Measuring, Monitoring, Reporting, and Verification (MMRV) The Biden-Harris Administration is also undertaking a whole-of-government effort to enhance our ability to measure and monitor greenhouse gas (GHG) emissions, a critical function underpinning the scientific integrity and atmospheric impact of credited activities. In November 2023, the Biden-Harris Administration released the first-ever National Strategy to Advance an Integrated U.S. Greenhouse Gas Measurement, Monitoring, and Information System , which seeks to enhance coordination and integration of GHG measurement, modeling, and data efforts to provide actionable GHG information. As part of implementation of the National Strategy, federal departments and agencies such as DOE, USDA, the Department of the Interior, the Department of Commerce, and the National Aeronautics and Space Administration are engaging in collaborative efforts to develop, test, and deploy technologies and other capabilities to measure, monitor, and better understand GHG emissions.
- Advancing Market Integrity and Protecting Against Fraud and Abuse. U.S. regulatory agencies are helping to build high-integrity VCMs by promoting the integrity of these markets. For example, the Commodity Futures Trading Commission (CFTC) proposed new guidance at COP28 to outline factors that derivatives exchanges may consider when listing voluntary carbon credit derivative contracts to promote the integrity, transparency, and liquidity of these developing markets. Earlier in 2023, the CFTC issued a whistleblower alert to inform the American public of how to identify and report potential Commodity Exchange Act violations connected to fraud and manipulation in voluntary carbon credit spot markets and the related derivative markets. The CFTC also stood up a new Environmental Fraud Task Force to address fraudulent activity and bad actors in these carbon markets. Internationally, the CFTC has also promoted the integrity of the VCMs by Co-Chairing the Carbon Markets Workstream of the International Organization of Securities Commission’s Sustainable Finance Task Force, which recently published a consultation on 21 good practices for regulatory authorities to consider in structuring sound, well-functioning VCMs.
- Taking a Whole-of-Government Approach to Coordinate Action. To coordinate the above actions and others across the Administration, the White House has stood up an interagency Task Force on Voluntary Carbon Markets. This group, comprising officials from across federal agencies and offices, will ensure there is a coordinated, government- wide approach to address the challenges and opportunities in this market and support the development of high-integrity VCMs.
The Biden-Harris Administration recognizes that the future of VCMs and their ability to effectively address climate change depends on a well-functioning market that links a supply of high-integrity credits to high-integrity demand from credible buyers. Today’s new statement and principles underscore a commitment to ensuring that VCMs fulfill their intended purpose to drive private capital toward innovative technological and nature-based solutions, preserve and protect natural ecosystems and lands, and support the United States and our international partners in our collective efforts to meet our ambitious climate goals.
Stay Connected
We'll be in touch with the latest information on how President Biden and his administration are working for the American people, as well as ways you can get involved and help our country build back better.
Opt in to send and receive text messages from President Biden.
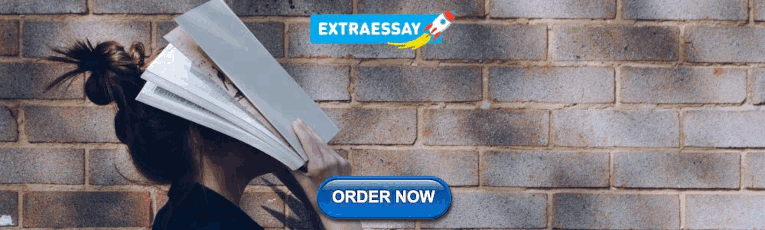
IMAGES
VIDEO
COMMENTS
I want to wait for a Task<T> to complete with some special rules: If it hasn't completed after X milliseconds, I want to display a message to the user. And if it hasn't completed after Y milliseconds, I want to automatically request cancellation.. I can use Task.ContinueWith to asynchronously wait for the task to complete (i.e. schedule an action to be executed when the task is complete), but ...
TimeSpan timeout, T fallback = default(T)) {. return (await Task.WhenAny(. task, DelayedResultTask<T>(timeout, fallback))).Result; } This time, our delayed dummy result is no longer a dummy result. If the task times out, then the result of Task.WhenAny is the timeout task, and its result is what becomes the result of the TaskWithTimeout ...
Examples. The following example calls the Wait(Int32, CancellationToken) method to provide both a timeout value and a cancellation token that can end the wait for a task's completion. A new thread is started and executes the CancelToken method, which pauses and then calls the CancellationTokenSource.Cancel method to cancel the cancellation tokens. A task is then launched and delays for 5 seconds.
In this post I look at how the new Task.WaitAsync() API is implemented in .NET 6, looking at the internal types used to implement it.. Adding a timeout or cancellation support to await Task. In my previous post, I showed how you could "cancel" an await Task call for a Task that didn't directly support cancellation by using the new WaitAsync() API in .NET 6.
Show 3 more. When you use the Task-based Asynchronous Pattern (TAP) to work with asynchronous operations, you can use callbacks to achieve waiting without blocking. For tasks, this is achieved through methods such as Task.ContinueWith. Language-based asynchronous support hides callbacks by allowing asynchronous operations to be awaited within ...
Continue With<TResult> (Func<Task,TResult>, Task Scheduler) Creates a continuation that executes asynchronously when the target Task completes and returns a value. The continuation uses a specified scheduler. Delay (Int32) Creates a task that completes after a specified number of milliseconds.
At the end of my previous post, in which I took a deep-dive into the new .NET 6 API Task.WaitAsync(), I included a brief side-note about what happens to your Task when you use Task.WaitAsync().Namely, that even if the WaitAsync() call is cancelled or times-out, the original Task continues running in the background.. Depending on your familiarity with the Task Parallel Library (TPL) or .NET in ...
This will wait timeout milliseconds for the task completion however, the task may still continue, like @Jesse pointed out. ... you can here use for NET 4.5 the overloaded Task.Run(Action, CancellationToken) method, which can be seen as a simplified call to Task.Factory.StartNew().
The sync and async codes are handled differently so you have to be aware of this when you define a policy. The Timeout policy can work in two modes: optimistic and pessimistic. The former one allows you to cancel the decorated method either by the user provided CancellationToken or by the timeout policy itself.
While the core concept (using Task.WhenAny to await on two tasks) is spot-on, the API around it feels dirty.Action for success is messy if you need to wire up another Task from it - you'd end up with ugly captures. I'd simply throw a TimeoutException in the timeout scenario and treat successful completion as, well, successful completion. In performance-critical cases a Task<bool> return type ...
A First Try. Here's some code that will do the trick: Copy. internal struct VoidTypeStruct { } // See Footnote #1 static class TaskExtensions { public static Task TimeoutAfter(this Task task, int millisecondsTimeout) { // tcs.Task will be returned as a proxy to the caller TaskCompletionSource<VoidTypeStruct> tcs = new TaskCompletionSource ...
You go to StackOverflow, of course, and find this answer: Asynchronously wait for Task<T> to complete with timeout. It's an elegant solution, mainly to also start a Task.Delayand continue when either task completes. However, in order to cancel the initial operation, one needs to pass the cancellation token to the original task and manually ...
// Task timed out, populate result with timeout message Result.timedOut(rqst.RequestNumber); Comments should tell the reader why something is done in the way it is done. What is done should be told by the code itself using meaningful names for classes, methods, properties and variables. Furthermore comments shouldn't lie like this comment is doing.
WithTimeout (Task, TimeSpan) Returns a task that completes as the original task completes or when a timeout expires, whichever happens first. WithTimeout<T> (Task<T>, TimeSpan) Returns a task that completes as the original task completes or when a timeout expires, whichever happens first.
Awaiting a Task with a timeout. The Task.WaitAsync(CancellationToken cancellationToken) method (and its counterpart on Task<T>) is very useful when you want to make an await cancellable by a CancellationToken. The other overloads are useful if you want to make it cancellable based on a timeout. For example, consider the following pseudo code ...
A simpler and clearer solution for an async operation timeout would be to await both the actual operation and a timeout task using Task.WhenAny. If the timeout task completes first, you got yourself a timeout. Otherwise, the operation completed successfully: public static async Task<TResult> WithTimeout<TResult>(this Task<TResult> task ...
Implementing auto logout for multi-tab/multi-browser sessions in .NET Core enhances user session security by ensuring that when a user logs out from one tab or browser, all active sessions across different tabs and browsers are simultaneously terminated, preventing unauthorized access.
Rosa Galvez has taken on a Herculean task: force Canadian financial institutions to prioritize the fight against climate change. More than two years ago, the independent senator from Quebec ...
Rescuing people buried in Papua New Guinea's landslide is a massive task — here's why By Doug Dingwall Posted Tue 28 May 2024 at 6:46pm Tuesday 28 May 2024 at 6:46pm Tue 28 May 2024 at 6:46pm
The following example launches a task that includes a call to the Delay (TimeSpan, CancellationToken) method with a 1.5 second delay. Before the delay interval elapses, the token is cancelled. The output from the example shows that, as a result, a TaskCanceledException is thrown, and the tasks' Status property is set to Canceled.
Providing Clear Guidance to Financial Institutions Supporting the Transition to Net Zero. ... of the International Organization of Securities Commission's Sustainable Finance Task Force, ...
Tasks support cooperative cancellation, that means if you want to cancel a Task that's already running, the code inside the Task has to support cancellation and it decides when will it be canceled. The way to achieve this is to pass the cancellation token to the method and call ThrowIfCancellationRequested() at suitable places in the code of that method.
Since interrupt handler is an RT task, it takes over the CPU and the flag-clearing task never gets scheduled, thus we have a lock-up. Delete this unnecessary flag. 2024-05-20: 5.5: CVE-2024-35997 416baaa9-dc9f-4396-8d5f-8c081fb06d67 416baaa9-dc9f-4396-8d5f-8c081fb06d67 416baaa9-dc9f-4396-8d5f-8c081fb06d67 416baaa9-dc9f-4396-8d5f-8c081fb06d67
The timeout middleware can be used in all types of ASP.NET Core apps: Minimal API, Web API with controllers, MVC, and Razor Pages. The sample app is a Minimal API, but every timeout feature it illustrates is also supported in the other app types. Request timeouts are in the Microsoft.AspNetCore.Http.Timeouts namespace.
Task.Wait() waits up to specified period for task completion and returns whether the task completed in the specified amount of time (or earlier) or not. The task itself is not modified and does not rely on waiting. Read nice series: Parallelism in .NET, Parallelism in .NET - Part 10, Cancellation in PLINQ and the Parallel class by Reed Copsey And: .NET 4 Cancellation Framework / Parallel ...
Examples. The following example calls the Run(Action, CancellationToken) method to create a task that iterates the files in the C:\Windows\System32 directory. The lambda expression calls the Parallel.ForEach method to add information about each file to a List<T> object. Each detached nested task invoked by the Parallel.ForEach loop checks the state of the cancellation token and, if ...