Learn C practically and Get Certified .
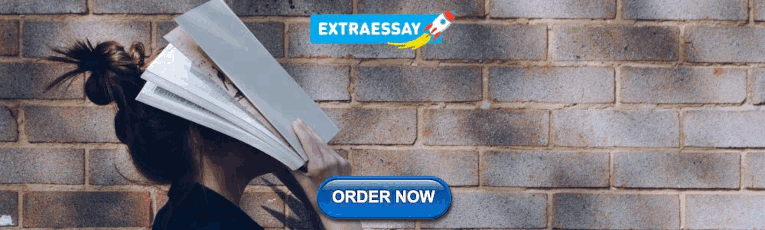
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Getting Started with C
- Your First C Program
C Fundamentals
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
C Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
C Programming Arrays
C Multidimensional Arrays
Pass arrays to a function in C
C Programming Pointers
Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
C Structure and Union
- C structs and Pointers
- C Structure and Function
C Programming Files
- C File Handling
C Files Examples
C Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Tutorials
- Find Largest Element in an Array
- Calculate Average Using Arrays
- Access Array Elements Using Pointer
- Add Two Matrices Using Multi-dimensional Arrays
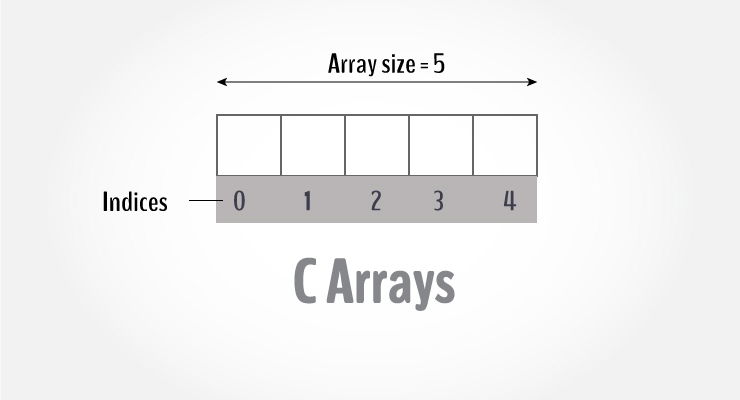
An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it.
How to declare an array?
For example,
Here, we declared an array, mark , of floating-point type. And its size is 5. Meaning, it can hold 5 floating-point values.
It's important to note that the size and type of an array cannot be changed once it is declared.
Access Array Elements
You can access elements of an array by indices.
Suppose you declared an array mark as above. The first element is mark[0] , the second element is mark[1] and so on.
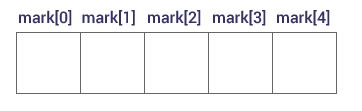
Few keynotes :
- Arrays have 0 as the first index, not 1. In this example, mark[0] is the first element.
- If the size of an array is n , to access the last element, the n-1 index is used. In this example, mark[4]
- Suppose the starting address of mark[0] is 2120d . Then, the address of the mark[1] will be 2124d . Similarly, the address of mark[2] will be 2128d and so on. This is because the size of a float is 4 bytes.
How to initialize an array?
It is possible to initialize an array during declaration. For example,
You can also initialize an array like this.
Here, we haven't specified the size. However, the compiler knows its size is 5 as we are initializing it with 5 elements.
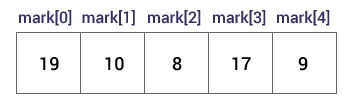
Change Value of Array elements
Input and output array elements.
Here's how you can take input from the user and store it in an array element.
Here's how you can print an individual element of an array.
Example 1: Array Input/Output
Here, we have used a for loop to take 5 inputs from the user and store them in an array. Then, using another for loop, these elements are displayed on the screen.
Example 2: Calculate Average
Here, we have computed the average of n numbers entered by the user.
Access elements out of its bound!
Suppose you declared an array of 10 elements. Let's say,
You can access the array elements from testArray[0] to testArray[9] .
Now let's say if you try to access testArray[12] . The element is not available. This may cause unexpected output (undefined behavior). Sometimes you might get an error and some other time your program may run correctly.
Hence, you should never access elements of an array outside of its bound.
Multidimensional arrays
In this tutorial, you learned about arrays. These arrays are called one-dimensional arrays.
In the next tutorial, you will learn about multidimensional arrays (array of an array) .
Table of Contents
- C Arrays (Introduction)
- Declaring an Array
- Access array elements
- Initializing an array
- Change Value of Array Elements
- Array Input/Output
- Example: Calculate Average
- Array Elements Out of its Bound
Video: C Arrays
Sorry about that.
Related Tutorials

Welcome.please sign up.
By signing up or logging in, you agree to our Terms of service and confirm that you have read our Privacy Policy .
Already a member? Go to Log In
Welcome.please login.
Forgot your password
Not registered yet? Go to Sign Up
- Introduction
- Let's start
- Decide if/else
- Loop and loop
- Decide and loop
- My functions
- Pre-processor
- Enjoy with files
- Dynamic memory
- Storage classes
Arrays in C
In simple English, an array is a collection.
In C also, it is a collection of similar type of data which can be either of int, float, double, char (String), etc. All the data types must be same. For example, we can't have an array in which some of the data are integer and some are float.

Suppose we need to store marks of 50 students in a class and calculate the average marks. So, declaring 50 separate variables will do the job but no programmer would like to do so. And there comes array in action.
How to declare an array
datatype array_name [ array_size ] ;
For example, take an array of integers 'n'.
n[ ] is used to denote an array 'n'. It means that 'n' is an array.
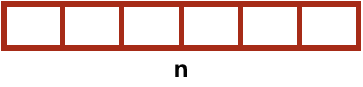
We need to give the size of the array because the complier needs to allocate space in the memory which is not possible without knowing the size. Compiler determines the size required for an array with the help of the number of elements of an array and the size of the data type present in the array.
Here 'int n[6]' will allocate space to 6 integers.
We can also declare an array by another method.
int n[ ] = {2, 3, 15, 8, 48, 13};
In this case, we are declaring and assigning values to the array at the same time. Here, there is no need to specify the array size because compiler gets it from { 2,3,15,8,48,13 } .
Index of an Array
Every element of an array has its index. We access any element of an array using its index.
Pictorial view of the above mentioned array is:
0, 1, 2, 3, 4 and 5 are indices. It is like they are identity of 6 different elements of an array. Index always starts from 0. So, the first element of an array has a index of 0.
We access any element of an array using its index and the syntax to do so is:
array_name[index]
For example, if the name of an array is 'n', then to access the first element (which is at 0 index), we write n[0] .
Here, n[0] is 2 n[1] is 3 n[2] is 15 n[3] is 8 n[4] is 48 n[5] is 13

n[0] , n[1] , etc. are like any other variables we were using till now i.e., we can set there value as n[0] = 5; like we do with any other variables ( x = 5; , y = 6; , etc.).
Assigning Values to Array
By writing int n[ ]={ 2,4,8 }; , we are declaring and assigning values to the array at the same time, thus initializing it.
But when we declare an array like int n[3]; , we need to assign values to it separately. Because 'int n[3];' will definitely allocate space of 3 integers in memory but there are no integers in that space.
To initialize it, assign a value to each of the elements of the array.
n[0] = 2; n[1] = 4; n[2] = 8;
It is just like we are declaring some variables and then assigning values to them.
int x,y,z; x=2; y=4; z=8;
Thus, the first way of assigning values to the elements of an array is by doing so at the time of its declaration.
int n[ ]={ 2,4,8 };
And the second method is declaring the array first and then assigning values to its elements.
int n[3]; n[0] = 2; n[1] = 4; n[2] = 8;
You can understand this by treating n[0] , n[1] and n[2] as similar to different variables you used before.
Just like variable, array can be of any other data type also.
float f[ ]= { 1.1, 1.4, 1.5};
Here, 'f' is an array of floats.
First, let's see the example to calculate the average of the marks of 3 students. Here, marks[0] represents the marks of the first student, marks[1] represents marks of the second and marks[2] represents marks of the third student.
Here you just saw a working example of array, we treated elements of the array in an exactly similar way as we had treated normal variables. &marks[0], &marks[1] and &marks[2] represent the addresses of marks[0], marks[1] and marks[2] respectively.
We can also use for loop as done in the next example.
The above code was just to make you familiar with using loops with an array because you will be doing this many times later.
The code is simple, 'i' and 'j' start from 0 because the index of an array starts from 0 and goes up to 9 (for 10 elements). So, 'i' and 'j' goes up to 9 and not 10 ( i<10 and j<10 ). So, in the code n[i] will be n[1], n[2], ...., n[9] and things will go accordingly.
Suppose we declare and initialize an array as
int n[5] = { 12, 13, 5 };
This means that n[0]=12, n[1]=13 and n[2]=5 and rest all elements are zero i.e. n[3]=0 and n[4]=0.
int n[5]; n[0] = 12; n[1] = 13; n[2] = 5;
In the above code, n[0], n[1] and n[2] are initialized to 12, 13 and 5 respectively. Therefore, n[4] and n[5] are both 0.
Pointer to Arrays
Till now, we have seen how to declare and initialize an array. Now, we will see how we can have pointers to arrays too. But before starting, we are assuming that you have gone through Pointers from the topic Point Me . If not, then first read the topic Pointers and practice some problems from the Practice section .
As we all know that pointer is a variable whose value is the address of some other variable i.e., if a variable 'y' points to another variable 'x', it means that the value of the variable 'y' is the address of 'x'.
Similarly, if we say that a variable 'y' points to an array 'n', it would mean that the value of 'y' is the address of the first element of the array i.e. n[0]. It means that the pointer of an array is the pointer of its first element.
If 'p' is a pointer to array 'age', means that p (or age) points to age[0].
int age[50]; int *p; p = age;
The above code assigns 'p' the address of the first element of the array 'age'.
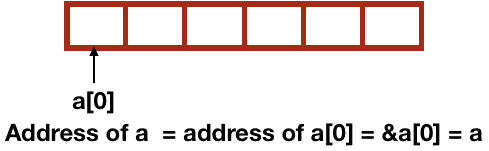
Now, since 'p' points to the first element of array 'age', '*p' is the value of the first element of the array.
So, *p is age[0], *(p+1) is age[1], *(p+2) is age[2].
Similarly, *age is age[0] (value at age), *(age+1) is age[1] (value at age+1), *(age+2) is age[2] (value at age+2) and so on.
That's all in pointer to arrays.
Now let's see some examples.
As 'p' is pointing to the first element of array, so, *p or *(p+0) represents the value at p[0] or the value at the first element of 'p'. Similarly, *(p+1) represents value at p[1]. And *(p+3) and *(p+4) represents p[3] and p[4] respectively. So accordingly, things were printed.
The above example sums up the above concepts. Now, let's print the address of the array and also individual elements of the array.
As you have noticed that the address of the first element of n and p are same and this means I was not lying! You can print other elements' addresses by using (p+1), (p+2) and (p+3) also.
Let's pass whole Array in Function
In C, we can pass an element of an array or the full array as an argument to a function.
Let's first pass a single array element to function.
Passing an entire Array in a Function
We can also pass the entire array to a function by passing array name as the argument. Yes, the trick is that we will pass the address of an array, that is the address of the first element of the array. Thus by having the pointer of the first element, we can get the entire array as we have done in examples above.

Let's see an example to understand it.
average(float a[]) → It is the function that is taking an array of float. And rest of the body of the function is performing accordingly.
b = average(n) → One thing you should note here is that we passed 'n'. And as discussed earlier, 'n' is the pointer to the first element or pointer to the array n[] . So, we have actually passed the pointer.
In the above example in which we calculated the average of the values of the elements of an array, we already knew the size of the array i.e. 8.
Suppose we are taking the size of the array from the user. In that case, the size of the array is not fixed. Here, we need to pass the size of array as the second argument to the function.
The code is similar to the previous one except that we passed the size of array explicitly - float average(float a[], int size ) .
We can also pass an array to a function using pointers. Let's see how.
In the above example, the address of the array i.e. address of n[0] is passed to the formal parameters of the function.
display(int *p) → This means that function 'display' is taking a pointer to an integer.
Now we passed the pointer of an integer i.e. pointer of the array n[] - 'n' as per the demand of our function 'display'.
Since 'p' is the address of the array n[] in the function 'display' i.e., address of the first element of the array (n[0]), therefore *p represents the value of n[0]. In the for loop in function, p++ increases the value of p by 1. So, when i=0, the value of *p gets printed. Then p++ increases *p to *(p+1) and in the second loop, the value of *(p+1) i.e. n[1] also gets printed. This loop continues till i=7 when the value of *(p+7) i.e. n[7] gets printed.

What if arrays are 2 dimensional?
Yes, 2-dimensional arrays also exist and are generally known as matrix . These consist of rows and columns.
Before going into its application, let's first see how to declare and initialize a 2D array.
Declaration of 2D Array
Similar to one-dimensional array, we define a 2-dimensional array as below.
int a[2][4];
Here, 'a' is a 2D array of integers which consists of 2 rows and 4 columns .
Now let's see how to initialize a 2-dimensional array.
Assigning Values to a 2 D Array
Same as in one-dimensional array, we can assign values to the elements of a 2-dimensional array in 2 ways as well.
In the first method, just assign a value to the elements of the array. If no value is assigned to any element, then its value is assumed to be zero.
Suppose we declared a 2-dimensional array a[2][2] . Now, we need to assign values to its elements.
int a[2][2]; a[0][0]=1; a[0][1]=2; a[1][0]=3; a[1][1]=4;
The second way is to declare and assign values at the same time as we did in one-dimensional array.
int a[2][3] = { 1, 2, 3, 4, 5, 6 };
Here, value of a[0][0] is 1, a[0][1] is 2, a[0][2] is 3, a[1][0] is 4, a[1][1] is 5 and a[1][2] is 6.
We can also write the above code as:
int a[2][3] = { {1, 2, 3}, {4, 5, 6 } };
Let's consider different cases of assigning values to an array at the time of declaration.
int a[2][2] = { 1, 2, 3, 4 }; /* valid */ int a[ ][2] = { 1, 2, 3, 4 }; /* valid */ int a[2][ ] = { 1, 2, 3, 4 }; /* invalid */ int a[ ][ ] = { 1, 2, 3, 4 }; /* invalid */
Why use of 2 D Array
Suppose we have 3 students each studying 2 subjects (subject 1 and subject 2) and we have to display the marks in both the subjects of the 3 students. Let's input the marks from the user.
This is something like
- Array vs Linked list in C
- Prime numbers using Sieve Algorithm in C
- Sorting an array using bubble sort in C
- Sorting an array using selection sort in C
- Sorting an array using insertion sort in C
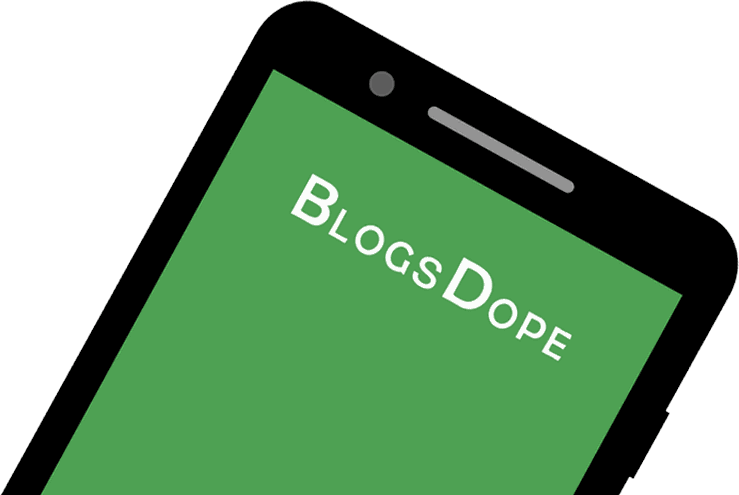
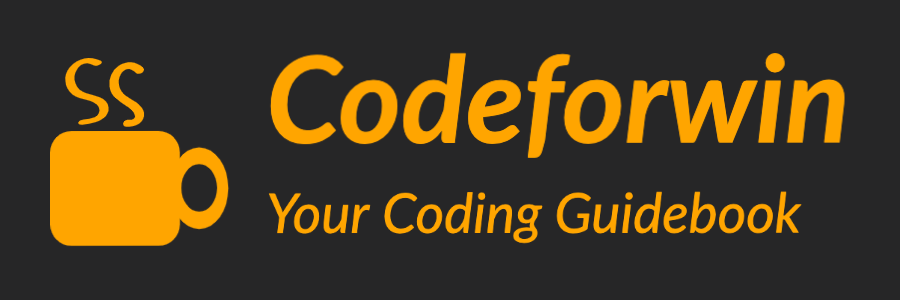
Arrays in C – Declare, initialize and access
Array is a data structure that hold finite sequential collection of homogeneous data .
To make it simple let’s break the words.
- Array is a collection – Array is a container that can hold a collection of data.
- Array is finite – The collection of data in array is always finite, which is determined prior to its use.
- Array is sequential – Array stores collection of data sequentially in memory.
- Array contains homogeneous data – The collection of data in array must share a same data type .
We can divide arrays in two categories.
- One-dimensional array (Or single-dimensional array)
- Multi-dimensional array
Why we need arrays?
Let us understand the significance of arrays through an example.
Suppose, I asked you to write a program to input 1000 students marks from user. Finally print average of their marks .
To solve the problem you will declare 1000 integer variable to input marks. Call I/O functions to input marks in 1000 variables and finally find the average.
Think for a while how tedious will be to code if solved using above approach. Declare 1000 variables, take input in all variables, then find average and finally print its average. The above increases length of code, complexity and degrades performance. If you don’t believe, try to code solution using above approach.
To solve above problem efficiently we use arrays. Arrays are good at handling collection of data (collection of 1000 student marks). Mind that in programming, we will always use some data structure (array in our case) to handle a collection of data efficiently.
How to use arrays?

Array in memory is stored as a continuous sequence of bytes. Like variables we give name to an array. However unlike variables, arrays are multi-valued they contain multiple values. Hence you cannot access specific array element directly.
For example, you can write sum = 432; to access sum . But, you cannot access specific array element directly by using array variable name. You cannot write marks to access 4 th student marks.
In array, we use an integer value called index to refer at any element of array. Array index starts from 0 and goes till N - 1 (where N is size of the array). In above case array index ranges from 0 to 4 .
To access individual array element we use array variable name with index enclosed within square brackets [ and ] . To access first element of marks array, we use marks[0] . Similarly to access third element we use marks[2] .
How to declare an array?
Syntax to declare an array.
- data_type is a valid C data type that must be common to all array elements.
- array_name is name given to array and must be a valid C identifier .
- SIZE is a constant value that defines array maximum capacity.
Example to declare an array
How to initialize an array.
There are two ways to initialize an array.
- Static array initialization – Initializes all elements of array during its declaration.
- Dynamic array initialization – The declared array is initialized some time later during execution of program.
Static initialization of array
We define value of all array elements within a pair of curly braces { and } during its declaration. Values are separated using comma , and must be of same type.
Example of static array initialization
Note: Size of array is optional when declaring and initializing array at once. The C compiler automatically determines array size using number of array elements. Hence, you can write above array initialization as.
Dynamic initialization of array
You can assign values to an array element dynamically during execution of program. First declare array with a fixed size. Then use the following syntax to assign values to an element dynamically.
Example to initialize an array dynamically
Instead of hard-coding marks values, you can ask user to input values to array using scanf() function.
The array index is an integer value, so instead of hard-coding you can wrap array input code inside a loop .
The above code will run 5 times from 0 to 4 . In each iteration it ask user to input an integer and stores it in successive elements of marks array.
Example program to implement one-dimensional array
Let us write a C program to declare an array capable of storing 10 student marks. Input marks of all 10 students and find their average.
Output –
Array best practices
- Arrays are fixed size, hence always be cautious while accessing arrays. Accessing an element that does not exists is undefined. You may get a valid value or the program may crash.For example, consider the below program. int array[5]; // Declare an integer array of size 5 /* Accessing sixth element of array which is undefined. */ array[5] = 50; /* Accessing -1th element of array is undefined */ array[-1] = 10;
- Always keep in mind that all array element store a value of similar type.
Recommended array example programs
- Program to read and print array element.
- Program to find maximum and minimum element in array.
- Program to insert a new element in array.
- Program to search an element in array.
- Program to sort array elements.
Practice more array programming exercises to learn more.
C Programming/Pointers and arrays
A pointer is a value that designates the address (i.e., the location in memory), of some value. Pointers are variables that hold a memory location.
There are four fundamental things you need to know about pointers:
- How to declare them (with the address operator ' & ': int *pointer = &variable; )
- How to assign to them ( pointer = NULL; )
- How to reference the value to which the pointer points (known as dereferencing , by using the dereferencing operator ' * ': value = *pointer; )
- How they relate to arrays (the vast majority of arrays in C are simple lists, also called "1 dimensional arrays", but we will briefly cover multi-dimensional arrays with some pointers in a later chapter ).
Pointers can reference any data type, even functions. We'll also discuss the relationship of pointers with text strings and the more advanced concept of function pointers.
- 1 Declaring pointers
- 2 Assigning values to pointers
- 3 Pointer dereferencing
- 4 Pointers and Arrays
- 5 Pointers in Function Arguments
- 6 Pointers and Text Strings
- 7.1 Practical use of function pointers in C
- 8 Examples of pointer constructs
- 10 External Links
Declaring pointers [ edit | edit source ]
Consider the following snippet of code which declares two pointers:
Lines 1-4 define a structure . Line 8 declares a variable that points to an int , and line 9 declares a variable that points to something with structure MyStruct. So to declare a variable as something that points to some type, rather than contains some type, the asterisk ( * ) is placed before the variable name.
In the following, line 1 declares var1 as a pointer to a long and var2 as a long and not a pointer to a long. In line 2, p3 is declared as a pointer to a pointer to an int.
Pointer types are often used as parameters to function calls. The following shows how to declare a function which uses a pointer as an argument. Since C passes function arguments by value, in order to allow a function to modify a value from the calling routine, a pointer to the value must be passed. Pointers to structures are also used as function arguments even when nothing in the struct will be modified in the function. This is done to avoid copying the complete contents of the structure onto the stack. More about pointers as function arguments later.
Assigning values to pointers [ edit | edit source ]
So far we've discussed how to declare pointers. The process of assigning values to pointers is next. To assign the address of a variable to a pointer, the & or 'address of' operator is used.
Here, pPointer will now reference myInt and pKeyboard will reference dvorak.
Pointers can also be assigned to reference dynamically allocated memory. The malloc() and calloc() functions are often used to do this.
The malloc function returns a pointer to dynamically allocated memory (or NULL if unsuccessful). The size of this memory will be appropriately sized to contain the MyStruct structure.
The following is an example showing one pointer being assigned to another and of a pointer being assigned a return value from a function.
When returning a pointer from a function, do not return a pointer that points to a value that is local to the function or that is a pointer to a function argument. Pointers to local variables become invalid when the function exits. In the above function, the value returned points to a static variable. Returning a pointer to dynamically allocated memory is also valid.
Pointer dereferencing [ edit | edit source ]
To access a value to which a pointer points, the * operator is used. Another operator, the -> operator is used in conjunction with pointers to structures. Here's a short example.
The expression bb->m_aNumber is entirely equivalent to (*bb).m_aNumber . They both access the m_aNumber element of the structure pointed to by bb . There is one more way of dereferencing a pointer, which will be discussed in the following section.
When dereferencing a pointer that points to an invalid memory location, an error often occurs which results in the program terminating. The error is often reported as a segmentation error. A common cause of this is failure to initialize a pointer before trying to dereference it.
C is known for giving you just enough rope to hang yourself, and pointer dereferencing is a prime example. You are quite free to write code that accesses memory outside that which you have explicitly requested from the system. And many times, that memory may appear as available to your program due to the vagaries of system memory allocation. However, even if 99 executions allow your program to run without fault, that 100th execution may be the time when your "memory pilfering" is caught by the system and the program fails. Be careful to ensure that your pointer offsets are within the bounds of allocated memory!
The declaration void *somePointer; is used to declare a pointer of some nonspecified type. You can assign a value to a void pointer, but you must cast the variable to point to some specified type before you can dereference it. Pointer arithmetic is also not valid with void * pointers.
Pointers and Arrays [ edit | edit source ]
Up to now, we've carefully been avoiding discussing arrays in the context of pointers. The interaction of pointers and arrays can be confusing but here are two fundamental statements about it:
- A variable declared as an array of some type acts as a pointer to that type. When used by itself, it points to the first element of the array.
- A pointer can be indexed like an array name.
The first case often is seen to occur when an array is passed as an argument to a function. The function declares the parameter as a pointer, but the actual argument may be the name of an array. The second case often occurs when accessing dynamically allocated memory.
Let's look at examples of each. In the following code, the call to calloc() effectively allocates an array of struct MyStruct items.
Pointers and array names can pretty much be used interchangeably; however, there are exceptions. You cannot assign a new pointer value to an array name. The array name will always point to the first element of the array. In the function returnSameIfAnyEquals , you could however assign a new value to workingArray, as it is just a pointer to the first element of workingArray. It is also valid for a function to return a pointer to one of the array elements from an array passed as an argument to a function. A function should never return a pointer to a local variable, even though the compiler will probably not complain.
When declaring parameters to functions, declaring an array variable without a size is equivalent to declaring a pointer. Often this is done to emphasize the fact that the pointer variable will be used in a manner equivalent to an array.
Now we're ready to discuss pointer arithmetic. You can add and subtract integer values to/from pointers. If myArray is declared to be some type of array, the expression *(myArray+j) , where j is an integer, is equivalent to myArray[j] . For instance, in the above example where we had the expression secondArray[i].otherNumber , we could have written that as (*(secondArray+i)).otherNumber or more simply (secondArray+i)->otherNumber .
Note that for addition and subtraction of integers and pointers, the value of the pointer is not adjusted by the integer amount, but is adjusted by the amount multiplied by the size of the type to which the pointer refers in bytes. (For example, pointer + x can be thought of as pointer + (x * sizeof(*type)) .)
One pointer may also be subtracted from another, provided they point to elements of the same array (or the position just beyond the end of the array). If you have a pointer that points to an element of an array, the index of the element is the result when the array name is subtracted from the pointer. Here's an example.
You may be wondering how pointers and multidimensional arrays interact. Let's look at this a bit in detail. Suppose A is declared as a two dimensional array of floats ( float A[D1][D2]; ) and that pf is declared a pointer to a float. If pf is initialized to point to A[0][0], then *(pf+1) is equivalent to A[0][1] and *(pf+D2) is equivalent to A[1][0]. The elements of the array are stored in row-major order.
Let's look at a slightly different problem. We want to have a two dimensional array, but we don't need to have all the rows the same length. What we do is declare an array of pointers. The second line below declares A as an array of pointers. Each pointer points to a float. Here's some applicable code:
We also note here something curious about array indexing. Suppose myArray is an array and i is an integer value. The expression myArray[i] is equivalent to i[myArray] . The first is equivalent to *(myArray+i) , and the second is equivalent to *(i+myArray) . These turn out to be the same, since the addition is commutative.
Pointers can be used with pre-increment or post-decrement, which is sometimes done within a loop, as in the following example. The increment and decrement applies to the pointer, not to the object to which the pointer refers. In other words, *pArray++ is equivalent to *(pArray++) .
Pointers in Function Arguments [ edit | edit source ]
Often we need to invoke a function with an argument that is itself a pointer. In many instances, the variable is itself a parameter for the current function and may be a pointer to some type of structure. The ampersand ( & ) character is not needed in this circumstance to obtain a pointer value, as the variable is itself a pointer. In the example below, the variable pStruct , a pointer, is a parameter to function FunctTwo , and is passed as an argument to FunctOne .
The second parameter to FunctOne is an int. Since in function FunctTwo , mValue is a pointer to an int, the pointer must first be dereferenced using the * operator, hence the second argument in the call is *mValue . The third parameter to function FunctOne is a pointer to a long. Since pAA is itself a pointer to a long, no ampersand is needed when it is used as the third argument to the function.
Pointers and Text Strings [ edit | edit source ]
Historically, text strings in C have been implemented as arrays of characters, with the last byte in the string being a zero, or the null character '\0'. Most C implementations come with a standard library of functions for manipulating strings. Many of the more commonly used functions expect the strings to be null terminated strings of characters. To use these functions requires the inclusion of the standard C header file "string.h".
A statically declared, initialized string would look similar to the following:
The variable myFormat can be viewed as an array of 21 characters. There is an implied null character ('\0') tacked on to the end of the string after the 'd' as the 21st item in the array. You can also initialize the individual characters of the array as follows:
An initialized array of strings would typically be done as follows:
The initialization of an especially long string can be split across lines of source code as follows.
The library functions that are used with strings are discussed in a later chapter.
Pointers to Functions [ edit | edit source ]
C also allows you to create pointers to functions. Pointers to functions syntax can get rather messy. As an example of this, consider the following functions:
Declaring a typedef to a function pointer generally clarifies the code. Here's an example that uses a function pointer, and a void * pointer to implement what's known as a callback. The DoSomethingNice function invokes a caller supplied function TalkJive with caller data. Note that DoSomethingNice really doesn't know anything about what dataPointer refers to.
Some versions of C may not require an ampersand preceding the TalkJive argument in the DoSomethingNice call. Some implementations may require specifically casting the argument to the MyFunctionType type, even though the function signature exacly matches that of the typedef.
Function pointers can be useful for implementing a form of polymorphism in C. First one declares a structure having as elements function pointers for the various operations to that can be specified polymorphically. A second base object structure containing a pointer to the previous structure is also declared. A class is defined by extending the second structure with the data specific for the class, and static variable of the type of the first structure, containing the addresses of the functions that are associated with the class. This type of polymorphism is used in the standard library when file I/O functions are called.
A similar mechanism can also be used for implementing a state machine in C. A structure is defined which contains function pointers for handling events that may occur within state, and for functions to be invoked upon entry to and exit from the state. An instance of this structure corresponds to a state. Each state is initialized with pointers to functions appropriate for the state. The current state of the state machine is in effect a pointer to one of these states. Changing the value of the current state pointer effectively changes the current state. When some event occurs, the appropriate function is called through a function pointer in the current state.
Practical use of function pointers in C [ edit | edit source ]
Function pointers are mainly used to reduce the complexity of switch statement. Example with switch statement:
Without using a switch statement:
Function pointers may be used to create a struct member function:
Use to implement this pointer (following code must be placed in library).
Examples of pointer constructs [ edit | edit source ]
Below are some example constructs which may aid in creating your pointer.
sizeof [ edit | edit source ]
The sizeof operator is often used to refer to the size of a static array declared earlier in the same function.
To find the end of an array (example from wikipedia:Buffer overflow ):
To iterate over every element of an array, use
Note that the sizeof operator only works on things defined earlier in the same function. The compiler replaces it with some fixed constant number. In this case, the buffer was declared as an array of 10 char's earlier in the same function, and the compiler replaces sizeof(buffer) with the number 10 at compile time (equivalent to us hard-coding 10 into the code in place of sizeof(buffer) ). The information about the length of buffer is not actually stored anywhere in memory (unless we keep track of it separately) and cannot be programmatically obtained at run time from the array/pointer itself.
Often a function needs to know the size of an array it was given -- an array defined in some other function. For example,
Unfortunately, (in C and C++) the length of the array cannot be obtained from an array passed in at run time, because (as mentioned above) the size of an array is not stored anywhere. The compiler always replaces sizeof with a constant. This sum() routine needs to handle more than just one constant length of an array.
There are some common ways to work around this fact:
- Write the function to require, for each array parameter, a "length" parameter (which has type "size_t"). (Typically we use sizeof at the point where this function is called).
- Use of a convention, such as a null-terminated string to mark the end of the array.
- Instead of passing raw arrays, pass a structure that includes the length of the array (such as ".length") as well as the array (or a pointer to the first element); similar to the string or vector classes in C++.
It's worth mentioning that sizeof operator has two variations: sizeof ( type ) (for instance: sizeof (int) or sizeof (struct some_structure) ) and sizeof expression (for instance: sizeof some_variable.some_field or sizeof 1 ).
External Links [ edit | edit source ]
- "Common Pointer Pitfalls" by Dave Marshall
- Book:C Programming
Navigation menu
Integer Array in C – How to Declare Int Arrays with C Programming
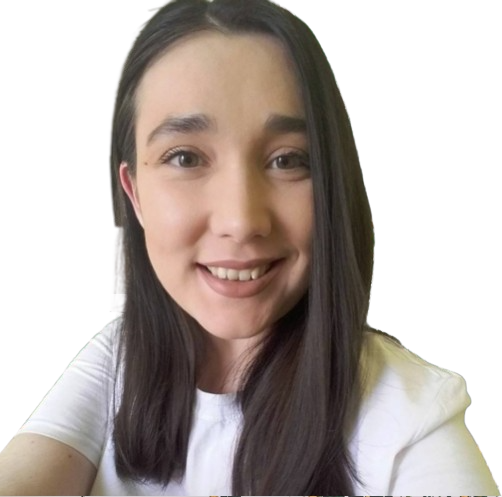
In this article, you will learn how to work with arrays in C.
I will first explain how to declare and initialize arrays.
Then, I will also go over how to access and change items in an array in C with the help of code examples along the way.
Let's get into it!
What Is An Array in C Programming?
An array is a data structure that stores multiple values in a single variable and in a sequential order that is easily accessible.
Arrays in C are a collection of values that store items of the same data type – an integer array holds only elements of the type int , a float array holds only elements of the type float , and so on.
How to Declare an Integer Array in C Programming
The general syntax for declaring an array in C looks as you can see it in the code snippet below:
Let's take the following example:
Let's break it down:
- I first defined the data type of the array, int .
- I then specified the name, my_numbers , followed by a pair of opening and closing square brackets, [] .
- Inside the square brackets, I defined the size ( 5 ), meaning the array can hold 5 integer values.
- Finally, I ended the statement with a semicolon ( ; ).
Once you have set the array type and size during declaration, the array can't hold items of another type.
The array is also fixed in size, meaning you cannot add or remove items.
How to Initialize an Integer Array in C Programming
There are a couple of ways you can initialize an integer array in C.
The first way is to initialize the array during declaration and insert the values inside a pair of opening and closing curly braces, {} .
The general syntax to do that looks like this:
Let's take the array I declared in the previous section that can hold five integers and initialize it with some values:
In the example above, I placed five comma-separated values inside curly braces, and assigned those values to my_numbers through the assignment operator ( = ).
Something to note here is that when you specify the size of the array, you can assign less number of elements, like so:
Although the size of the array is 5 , I only placed three values inside it.
The array can hold two more items, and those remaining two positions have a default value of 0 .
Another way to initialize an array is to not specify the size, like so:
Even though I did not set the size of the array, the compiler knows its size because it knows the number of items stored inside it.
How to Access Items in an Integer Array in C Programming
To access an element in an array, you have to specify the index of the element in square brackets after the array name.
The syntax to access an element looks like this:
In C and programming in general, an array index always starts at 0 , becuase in computer science, counting starts from 0 .
So, the first item in an array has an index of 0 , the second item has an index of 1 , the third item has an index of 2 , and so on.
Taking the same array from the previous section, here is how you would access the first element, that is, 10 :
Keep in mind that the last element in an array has an index of array_size -1 – it is always one less than the size of the array. So, in an array that holds five elements, the index of the last element is 4 .
If in an array of five items, you try to access the last element by using an index of 5 , the program will run, but the element is not available, and you will get undefined behavior:
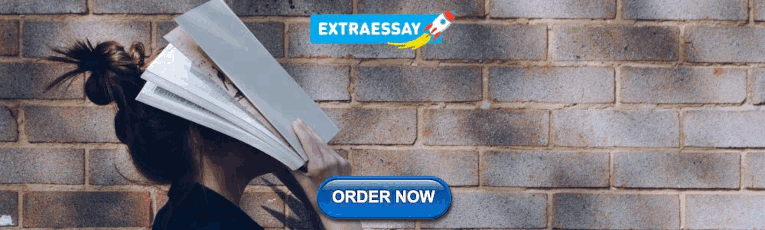
How to Change Items in an Integer Array in C Programming
To change the value of a specific element, specify its index number and, with the assignment operator, = , assign a new value:
In the example above, I changed the first item in the array from 10 to 11 .
And there you have it! You now know the basics of working with arrays in C.
To learn more about C, give this C beginner's handbook a read to become familiar with the basics of the language.
Thanks for reading, and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
C Functions
C structures.
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To create an array, define the data type (like int ) and specify the name of the array followed by square brackets [] .
To insert values to it, use a comma-separated list, inside curly braces:
We have now created a variable that holds an array of four integers.
Access the Elements of an Array
To access an array element, refer to its index number .
Array indexes start with 0 : [0] is the first element. [1] is the second element, etc.
This statement accesses the value of the first element [0] in myNumbers :
Change an Array Element
To change the value of a specific element, refer to the index number:
Loop Through an Array
You can loop through the array elements with the for loop.
The following example outputs all elements in the myNumbers array:
Set Array Size
Another common way to create arrays, is to specify the size of the array, and add elements later:
Using this method, you should know the number of array elements in advance, in order for the program to store enough memory.
You are not able to change the size of the array after creation.
C Exercises
Test yourself with exercises.
Create an array of type int called myNumbers .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
- Properties of Array in C
- Length of Array in C
- Multidimensional Arrays in C
- Initialization of Multidimensional Array in C
- Jagged Array or Array of Arrays in C with Examples
- Pass Array to Functions in C
- How to pass a 2D array as a parameter in C?
- How to pass an array by value in C ?
- Variable Length Arrays (VLAs) in C
- What are the data types for which it is not possible to create an array?
- Strings in C
Array of Strings in C
- C Library - <string.h>
- C String Functions
- What is the difference between single quoted and double quoted declaration of char array?
- Array C/C++ Programs
- String C/C++ Programs
In C programming String is a 1-D array of characters and is defined as an array of characters. But an array of strings in C is a two-dimensional array of character types. Each String is terminated with a null character (\0). It is an application of a 2d array.
- var_name is the name of the variable in C.
- r is the maximum number of string values that can be stored in a string array.
- c is the maximum number of character values that can be stored in each string array.
Example:
Below is the Representation of the above program
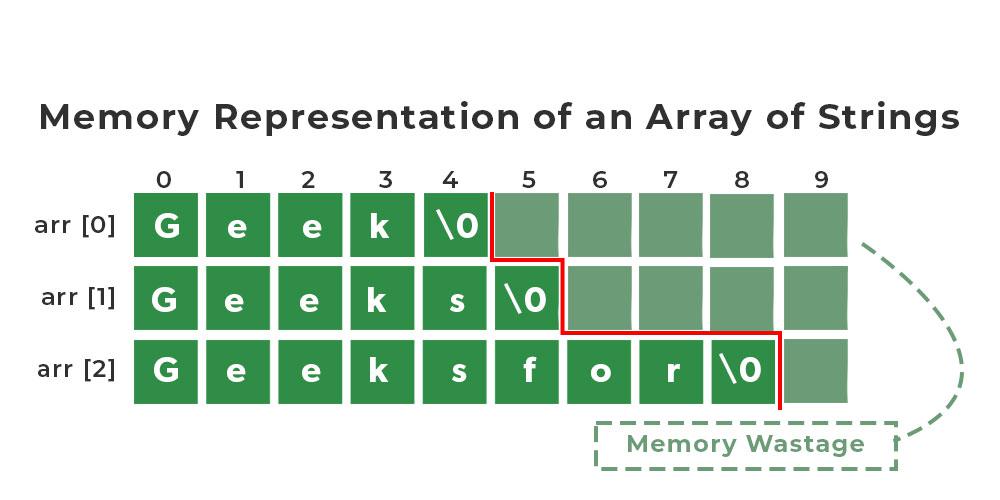
We have 3 rows and 10 columns specified in our Array of String but because of prespecifying, the size of the array of strings the space consumption is high. So, to avoid high space consumption in our program we can use an Array of Pointers in C.
Invalid Operations in Arrays of Strings
We can’t directly change or assign the values to an array of strings in C.
Here, arr[0] = “GFG”; // This will give an Error that says assignment to expression with an array type.
To change values we can use strcpy() function in C
Array of Pointers of Strings
In C we can use an Array of pointers. Instead of having a 2-Dimensional character array, we can have a single-dimensional array of Pointers. Here pointer to the first character of the string literal is stored.
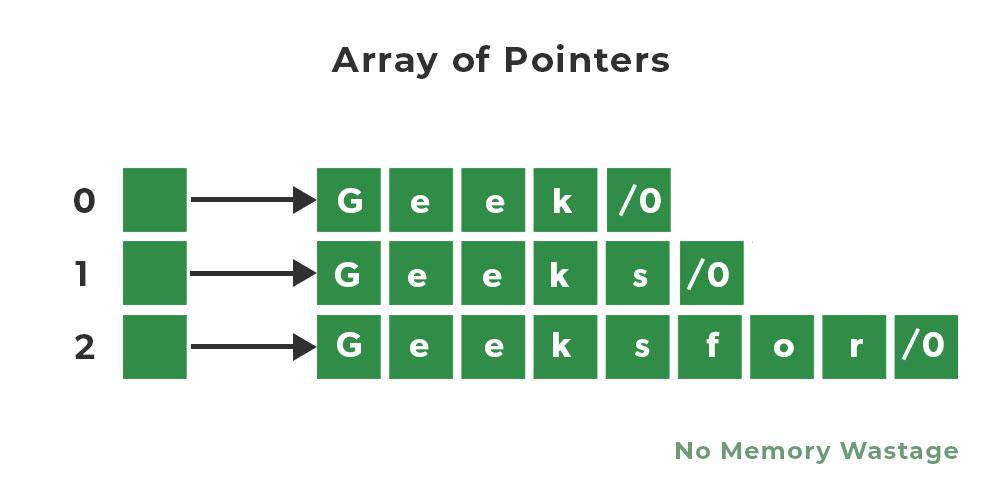
Below is the C program to print an array of pointers:
Please Login to comment...
Similar reads.
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Pointers and Arrays in C
In C programming, the concepts of arrays and pointers have a very important role. There is also a close association between the two. In this chapter, we will explain in detail the relationship between arrays and pointers in C programming.
Arrays in C
An array in C is a homogenous collection of elements of a single data type stored in a continuous block of memory. The size of an array is an integer inside square brackets, put in front of the name of the array.
Declaring an Array
To declare an array, the following syntax is used −
Each element in the array is identified by a unique incrementing index, starting from "0". An array can be declared and initialized in different ways.
You can declare an array and then initialize it later in the code, as and when required. For example −
You can also declare and initialize an array at the same time. The values to be stored are put as a comma separated list inside curly brackets.
Note: When an array is initialized at the time of declaration, mentioning its size is optional, as the compiler automatically computes the size. Hence, the following statement is also valid −
Example: Lower Bound and Upper Bound of an Array
All the elements in an array have a positional index, starting from "0". The lower bound of the array is always "0", whereas the upper bound is "size − 1" . We can use this property to traverse, assign, or read inputs into the array subscripts with a loop.
Take a look at this following example −
Run the code and check its output −
Pointers in C
A pointer is a variable that stores the address of another variable. In C, the symbol (&) is used as the address-of operator . The value returned by this operator is assigned to a pointer.
To declare a variable as a pointer, you need to put an asterisk (*) before the name. Also, the type of pointer variable must be the same as the type of the variable whose address it stores.
In this code snippet, "b" is an integer pointer that stores the address of an integer variable "a" −
In case of an array, you can assign the address of its 0 th element to the pointer.
In C, the name of the array itself resolves to the address of its 0 th element. It means, in the above case, we can use "arr" as equivalent to "&[0]":
Example: Increment Operator with Pointer
Unlike a normal numeric variable (where the increment operator "++" increments its value by 1), the increment operator used with a pointer increases its value by the sizeof its data type.
Hence, an int pointer, when incremented, increases by 4.
The Dereference Operator in C
In C, the "*" symbol is used as the dereference operator. It returns the value stored at the address to which the pointer points.
Hence, the following statement returns "5", which is the value stored in the variable "a", the variable that "b" points to.
Note: In case of a char pointer, it will increment by 1; in case of a double pointer, it will increment by 8; and in case of a struct type, it increments by the sizeof value of that struct type.
Example: Traversing an Array Using a Pointer
We can use this property of the pointer to traverse the array element with the help of a pointer.
Points to Note
It may be noted that −
- "&arr[0]" is equivalent to "b" and "arr[0]" to "*b".
- Similarly, "&arr[1]" is equivalent to "b + 1" and "arr[1]" is equivalent to "*(b + 1)".
- Also, "&arr[2]" is equivalent to "b + 2" and "arr[2]" is equivalent to "*(b+2)".
- In general, "&arr[i]" is equivalent to "b + I" and "arr[i]" is equivalent to "*(b+i)".
Example: Traversing an Array using the Dereference Operator
We can use this property and use a loop to traverse the array with the dereference operator.
You can also increment the pointer in every iteration and obtain the same result −
The concept of arrays and pointers in C has a close relationship. You can use pointers to enhance the efficiency of a program, as pointers deal directly with the memory addresses. Pointers can also be used to handle multi−dimensional arrays.
To Continue Learning Please Login
cppreference.com
Array declaration.
Declares an object of array type.
[ edit ] Syntax
An array declaration is any simple declaration whose declarator has the form
A declaration of the form T a [ N ] ; , declares a as an array object that consists of N contiguously allocated objects of type T . The elements of an array are numbered 0 , …, N - 1 , and may be accessed with the subscript operator [] , as in a [ 0 ] , …, a [ N - 1 ] .
Arrays can be constructed from any fundamental type (except void ), pointers , pointers to members , classes , enumerations , or from other arrays of known bound (in which case the array is said to be multi-dimensional). In other words, only object types except for array types of unknown bound can be element types of array types. Array types of incomplete element type are also incomplete types.
There are no arrays of references or arrays of functions.
Applying cv-qualifiers to an array type (through typedef or template type manipulation) applies the qualifiers to the element type, but any array type whose elements are of cv-qualified type is considered to have the same cv-qualification.
When used with new[]-expression , the size of an array may be zero; such an array has no elements:
[ edit ] Assignment
Objects of array type cannot be modified as a whole: even though they are lvalues (e.g. an address of array can be taken), they cannot appear on the left hand side of an assignment operator:
[ edit ] Array-to-pointer decay
There is an implicit conversion from lvalues and rvalues of array type to rvalues of pointer type: it constructs a pointer to the first element of an array. This conversion is used whenever arrays appear in context where arrays are not expected, but pointers are:
[ edit ] Multidimensional arrays
When the element type of an array is another array, it is said that the array is multidimensional:
Note that when array-to-pointer decay is applied, a multidimensional array is converted to a pointer to its first element (e.g., a pointer to its first row or to its first plane): array-to-pointer decay is applied only once.
[ edit ] Arrays of unknown bound
If expr is omitted in the declaration of an array, the type declared is "array of unknown bound of T", which is a kind of incomplete type , except when used in a declaration with an aggregate initializer :
Because array elements cannot be arrays of unknown bound, multidimensional arrays cannot have unknown bound in a dimension other than the first:
If there is a preceding declaration of the entity in the same scope in which the bound was specified, an omitted array bound is taken to be the same as in that earlier declaration, and similarly for the definition of a static data member of a class:
References and pointers to arrays of unknown bound can be formed, but cannot (until C++20) and can (since C++20) be initialized or assigned from arrays and pointers to arrays of known bound. Note that in the C programming language, pointers to arrays of unknown bound are compatible with pointers to arrays of known bound and are thus convertible and assignable in both directions.
Pointers to arrays of unknown bound cannot participate in pointer arithmetic and cannot be used on the left of the subscript operator , but can be dereferenced.
[ edit ] Array rvalues
Although arrays cannot be returned from functions by value and cannot be targets of most cast expressions, array prvalues may be formed by using a type alias to construct an array temporary using brace-initialized functional cast .
Array xvalues may be formed directly by accessing an array member of a class rvalue or by using std::move or another cast or function call that returns an rvalue reference.
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 4 April 2024, at 07:41.
- This page has been accessed 364,165 times.
- Privacy policy
- About cppreference.com
- Disclaimers

TechRepublic
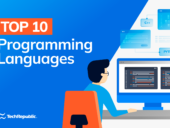
TIOBE Index for May 2024: Top 10 Most Popular Programming Languages
Fortran is in the spotlight again in part due to the increased interest in artificial intelligence.
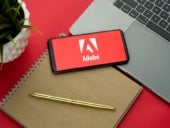
Adobe Adds Firefly and Content Credentials to Bug Bounty Program
Security researchers can earn up to $10,000 for critical vulnerabilities in the generative AI products.
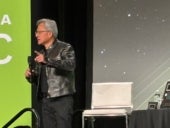
NVIDIA GTC 2024: CEO Jensen Huang’s Predictions About Prompt Engineering
"The job of the computer is to not require C++ to be useful," said Huang at NVIDIA GTC 2024.
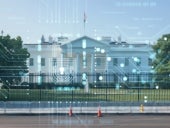
White House Recommends Memory-Safe Programming Languages and Security-by-Design
A new report promotes preventing cyberattacks by using memory-safe languages and the development of software safety standards.
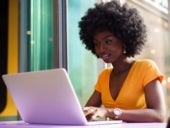
How to Hire a Python Developer
Spend less time researching and more time recruiting the ideal Python developer. Find out how in this article.
Latest Articles
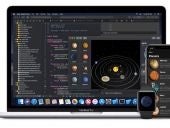
The Apple Developer Program: What Professionals Need to Know
If you want to develop software for macOS, iOS, tvOS, watchOS or visionOS, read this overview of Apple's Developer Program.
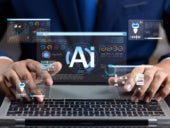
U.K.’s AI Safety Institute Launches Open-Source Testing Platform
Inspect is the first AI safety testing platform created by a state-backed body to be made freely available to the global AI community.
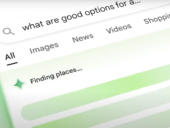
Google I/O 2024: Google Search’s AI Overviews Are Generally Available This Week
Plus, Google reveals plans to unleash Gemini across Workspace to make interpreting long email threads or creating spreadsheets easier.
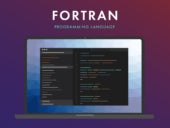
TIOBE Index News (May 2024): Why is Fortran Popular Again?
The AI boom is starting to show up on the TIOBE Index by bringing back a formative programming language.
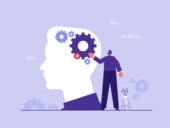
Udemy Report: Which IT Skills Are Most in Demand in Q1 2024?
Informatica PowerCenter, Microsoft Playwright and Oracle Database SQL top Udemy’s list of most popular tech courses.
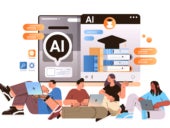
The 10 Best AI Courses in 2024
Today’s options for best AI courses offer a wide variety of hands-on experience with generative AI, machine learning and AI algorithms.
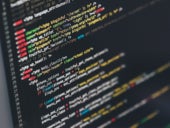
Learn Windows PowerShell for just $17
Streamline your workflow, automate tasks and more with The 2024 Windows PowerShell Certification Bundle.
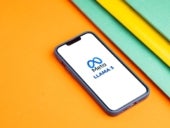
Llama 3 Cheat Sheet: A Complete Guide for 2024
Learn how to access Meta’s new AI model Llama 3, which sets itself apart by being open to use under a license agreement.
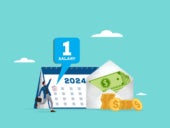
How Are APAC Tech Salaries Faring in 2024?
The year 2024 is bringing a return to stable tech salary growth in APAC, with AI and data jobs leading the way. This follows downward salary pressure in 2023, after steep increases in previous years.
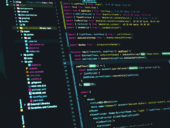
Learn Python for Just $16 Through 5/5
One of our best-selling Python bundles is discounted even further to just $15.97 through May 5. Now's the time to learn the popular programming language.
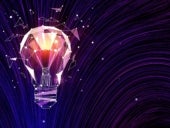
TechRepublic Premium Editorial Calendar: Policies, Checklists, Hiring Kits and Glossaries for Download
TechRepublic Premium content helps you solve your toughest IT issues and jump-start your career or next project.
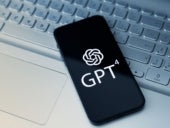
OpenAI’s GPT-4 Can Autonomously Exploit 87% of One-Day Vulnerabilities, Study Finds
Researchers from the University of Illinois Urbana-Champaign found that OpenAI’s GPT-4 is able to exploit 87% of a list of vulnerabilities when provided with their NIST descriptions.
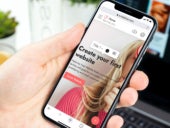
Create Easy, Professional Websites with Mobirise – Now Just $80 Through 4/21
Most user-friendly website builders create basic, non-professional or ineffective websites. Not anymore! With Mobirise, new users can get a year of easily built beautiful sites. Get a year’s access for only $79.97 through April 21.
Create a TechRepublic Account
Get the web's best business technology news, tutorials, reviews, trends, and analysis—in your inbox. Let's start with the basics.
* - indicates required fields
Sign in to TechRepublic
Lost your password? Request a new password
Reset Password
Please enter your email adress. You will receive an email message with instructions on how to reset your password.
Check your email for a password reset link. If you didn't receive an email don't forgot to check your spam folder, otherwise contact support .
Welcome. Tell us a little bit about you.
This will help us provide you with customized content.
Want to receive more TechRepublic news?
You're all set.
Thanks for signing up! Keep an eye out for a confirmation email from our team. To ensure any newsletters you subscribed to hit your inbox, make sure to add [email protected] to your contacts list.
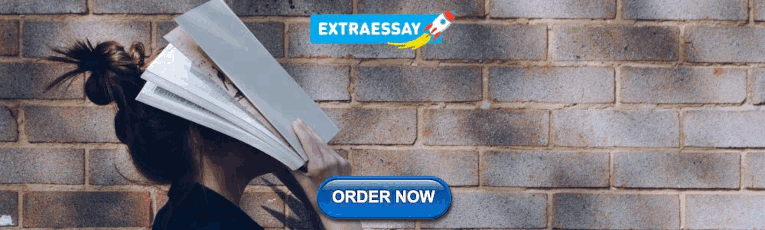
IMAGES
VIDEO
COMMENTS
You call GEN_ARRAY_ASSIGN_FUNC for every type and you add the type to the _Generic arguments. And use it like this: ARRAY_ASSIGN(coordinates, 1.f, 2.f, 3.f, 4.f); Or if you prefer providing count rather than NULL termination:
Array is not a pointer. Arrays only decay to pointers causing a lots of confusion when people learn C language. You cant assign arrays in C. You only assign scalar types (integers, pointers, structs and unions) but not the arrays. Structs can be used as a workaround if you want to copy the array
Arrays in C. An array is a variable that can store multiple values. For example, if you want to store 100 integers, you can create an array for it. int data[100]; How to declare an array? dataType arrayName[arraySize]; For example, float mark[5]; Here, we declared an array, mark, of floating-point type. And its size is 5.
Array in C is one of the most used data structures in C programming. It is a simple and fast way of storing multiple values under a single name. In this article, we will study the different aspects of array in C language such as array declaration, definition, initialization, types of arrays, array syntax, advantages and disadvantages, and many more.
Same as in one-dimensional array, we can assign values to the elements of a 2-dimensional array in 2 ways as well. In the first method, just assign a value to the elements of the array. If no value is assigned to any element, then its value is assumed to be zero. Suppose we declared a 2-dimensional array a[2][2]. Now, we need to assign values ...
Array is a type consisting of a contiguously allocated nonempty sequence of objects with a particular element type. The number of those objects (the array size) never changes during the array lifetime. ... Assignment. Objects of array type are not modifiable lvalues, and although their address may be taken, they cannot appear on the left hand ...
The C compiler automatically determines array size using number of array elements. Hence, you can write above array initialization as. int marks[] = {90, 86, 89, 76, 91}; Dynamic initialization of array. You can assign values to an array element dynamically during execution of program. First declare array with a fixed size.
C Programming/Pointers and arrays. Pointer a pointing to variable b. Note that b stores a number, whereas a stores the address of b in memory (1462) A pointer is a value that designates the address (i.e., the location in memory), of some value. Pointers are variables that hold a memory location. There are four fundamental things you need to ...
16 Arrays. An array is a data object that holds a series of elements, all of the same data type.Each element is identified by its numeric index within the array. We presented arrays of numbers in the sample programs early in this manual (see Array Example).However, arrays can have elements of any data type, including pointers, structures, unions, and other arrays.
The general syntax for declaring an array in C looks as you can see it in the code snippet below: data_type array_name[array_size]; Let's take the following example: int my_numbers[5]; Let's break it down: I first defined the data type of the array, int. I then specified the name, my_numbers, followed by a pair of opening and closing square ...
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To create an array, define the data type (like int) and specify the name of the array followed by square brackets [] . To insert values to it, use a comma-separated list, inside curly braces: We have now created a variable that ...
Initialization from strings. String literal (optionally enclosed in braces) may be used as the initializer for an array of matching type: . ordinary string literals and UTF-8 string literals (since C11) can initialize arrays of any character type (char, signed char, unsigned char) ; L-prefixed wide string literals can be used to initialize arrays of any type compatible with (ignoring cv ...
An array in C is a collection of data items of similar data type. One or more values same data type, which may be primary data types (int, float, char), or user-defined types such as struct or pointers can be stored in an array. In C, the type of elements in the array should match with the data type of the array itself.
5. In C you cannot assign arrays directly. At first I thought this might because the C facilities were supposed to be implementable with a single or a few instructions and more complicated functionality was offloaded to standard library functions. After all using memcpy() is not that hard.
structure assigment such as r1 = r2 copies array fields' contents just as it copies all the other fields. This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct. You can't copy the contents of the data field as an array, because.
Assuming you have some understanding of pointers in C, let us start: An array name is a constant pointer to the first element of the array. Therefore, in the declaration −. balance is a pointer to &balance [0], which is the address of the first element of the array balance. Thus, the following program fragment assigns p as the address of the ...
But an array of strings in C is a two-dimensional array of character types. Each String is terminated with a null character (\0). It is an application of a 2d array. Syntax: char variable_name[r] = {list of string}; Here, var_name is the name of the variable in C. r is the maximum number of string values that can be stored in a string array.
Arrays in C. An array in C is a homogenous collection of elements of a single data type stored in a continuous block of memory. The size of an array is an integer inside square brackets, put in front of the name of the array. Declaring an Array. To declare an array, the following syntax is used −. data_type arr_name[size]; Each element in the ...
Array Element Assignment [closed] Ask Question Asked yesterday. Modified today. Viewed 83 times -2 Closed. This question needs ... Are your array dimensions B[k] compile-time constants or the code should work with them changing in runtime? That's important information, optimization-wise. ...
References and pointers to arrays of unknown bound can be formed, but cannot (until C++20) and can (since C++20) be initialized or assigned from arrays and pointers to arrays of known bound. Note that in the C programming language, pointers to arrays of unknown bound are compatible with pointers to arrays of known bound and are thus convertible and assignable in both directions.
Others already explained what you got wrong. I'm writing that answer to explain that actually the compiler could assign an array to another, and you can achieve the same effect with minimal change to your sample code. Just wrap your array in a structure. struct Array3 {. int t[3]; }; struct Array3 a = {{1,2,3}};
Developer TR Academy Create Easy, Professional Websites with Mobirise - Now Just $80 Through 4/21 . Most user-friendly website builders create basic, non-professional or ineffective websites.
First part, you try to copy two array of character (string is not a pointer, it is array of character that is terminated by null character \0 ). If you want to copy value of an array to another, you can use memcpy, but for string, you can also use strcpy. E[0].nom = "reda"; change to: strcpy(E[0].nom,"reda"); Second part, you make the pointer ...
There is a difference between initialization and assignment. What you want to do is not initialization, but assignment. But such assignment to array is not possible in C++. Here is what you can do: #include <algorithm>. int array [] = {1,3,34,5,6}; int newarr [] = {34,2,4,5,6}; std::ranges::copy(newarr, array); // C++20.