JavaScript: Update/Replace a Specific Element in an Array
This quick and straightforward article shows you a couple of different ways to update or replace a specific element in an array in modern JavaScript.
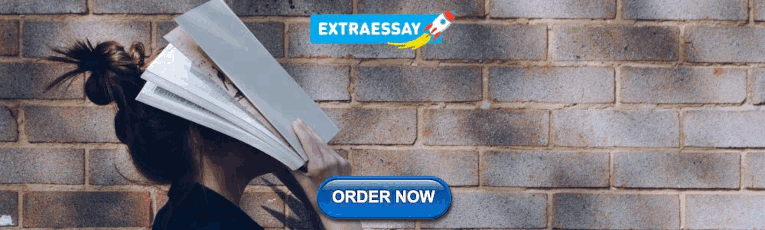
Using array[index] syntax
You can directly access an element in an array using its index and update its value:
If you don’t know the index of an element but already know its value, you can its index by using the indexOf() method. The example below will update the element whose value is Dog :
Using the splice() method
The splice() method allows you to add or remove elements from an array. You can use it to replace an element by specifying the index, the number of elements to remove (which is 1 in this case), and the new element to add.
That’s it. Happy coding!
Next Article: How to Remove Elements from an Array in JavaScript
Previous Article: JavaScript: How to Identify Mode(s) of an Array (3 Approaches)
Series: Working with Arrays in JavaScript
Related Articles
- JavaScript: Press ESC to exit fullscreen mode (2 examples)
- Can JavaScript programmatically bookmark a page? (If not, what are alternatives)
- Dynamic Import in JavaScript: Tutorial & Examples (ES2020+)
- JavaScript: How to implement auto-save form
- JavaScript: Disable a Button After a Click for N Seconds
- JavaScript: Detect when a Div goes into viewport
- JavaScript Promise.any() method (basic and advanced examples)
- Using logical nullish assignment in JavaScript (with examples)
- Understanding WeakRef in JavaScript (with examples)
- JavaScript Numeric Separators: Basic and Advanced Examples
- JavaScript: Using AggregateError to Handle Exceptions
- JavaScript FinalizationRegistry: Explained with Examples
Search tutorials, examples, and resources
- PHP programming
- Symfony & Doctrine
- Laravel & Eloquent
- Tailwind CSS
- Sequelize.js
- Mongoose.js
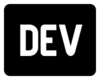
DEV Community

Posted on Jul 6, 2020 • Updated on May 10, 2021 • Originally published at inspiredwebdev.com
Find and Replace elements in Array with JavaScript
This article was originally posted on my blog . Head over to inspiredwebdev.com for more articles and tutorials. Check out my JavaScript course on Educative to learn everything from ES6 to ES2020.
Arrays are a very common data structure and it's important to know how to manipulate them by retrieving, adding, and replacing data inside of them.
In this article, we are going to learn what are the different ways to find and replace items inside of arrays.
Check that an Array contains a value
First, let's look at different ways of checking if our Array includes a certain value provided.
We can do that in different ways such as:
Array.includes is probably the easiest method to remember and it will return us true or false if our Array includes or not the value we passed.
This method can take an additional argument which defines the index from where we want to start looking, leave empty if you want to check the whole Array.
Let's continue with more methods:
Array.find is also another method we can use to check if our Array contains a certain value.
This method will return the value itself or undefined if no value is found so we can use the !! operator to convert the result to boolean and quickly see if there's a match or not.
It's a more powerful method compared to Array.includes as we can pass a callback to it, not just a value to check, meaning that we can do more complex checks such as:
Being able to pass a callback to it it means that unless your check is a very straightforward one, you are most likely going to use find over includes .
You can pass a second argument to the callback function defining the starting point where to start checking, leave empty to check the whole Array.
Next up we have Array.indexOf and Array.findIndex :
Array.indexOf and Array.findIndex are similar because they both return the index of the first matching element found in our Array, returning us -1 if it's not found.
To check if an element exists, we simply need to check if the returned value is -1 or not.
These methods are useful because they can be used to both checks if an element exists in the Array while at the same time getting a reference as to where that element is positioned, which we can use to then replace that said element.
The difference between the two methods is the same as the one we saw between Array.includes and Array.find , where the first one ( Array.indexOf ) will accept a value to check whereas the second one ( Array.findIndex ) will accept a callback to perform more advanced checks.
Similarly to all the methods we previously saw, you can also define a starting index where to start check the Array.
Next up are two new metho introduced in ES6 (ES2015):
Array.some will check if at least one value in the array matches the condition in our callback function and Array.every will check that ALL of the elements in the Array match that condition.
Replacing an element of an Array at a specific index
Now that we know how to check if the Array includes a specific element, let's say we want to replace that element with something else.
Knowing the methods above, it couldn't be easier!
In order to replace an element we need to know its index, so let's see some examples using the methods we just learned:
As you can see, first, we got the index of the element we wanted to change, in this case, the number 2 and then we replaced it using the brackets notation arr[index] .
We can do the same using findIndex :
Pretty easy right? Using findIndex we can also check scenarios like the following where we have an Array of Objects:
As you can see, using findIndex we can easily find and then replace Objects in an Array of Objects.
Let's say we are not interested in replacing a value but we just want to remove it, we will now look at different ways of doing so.
&bnbsp;
Removing a value from an Array
First, let's look at the more basic methods to remove values from an Array: Array.pop and Array.shift
Array.pop will remove the last element of the Array while Array.shift will remove the first one. No additional arguments are allowed, so you can see that these methods are fairly basic.
Both methods will modify your origianl array and both return the removed element so you can do the following:
Now we will look at a couple of ways to remove a specific element from an array.
First, let's look at Array.splice used in combination with Array.indexOf .
Array.splice allows us to remove elements from an Array starting from a specific index. We can provide a second argument to specify how many elements to delete.
As you can see, in the first example we specified 1 as the number of elements to remove, whereas in the second example we didn't pass any argument thus removing all items in the array from our starting index.
Array.splice will modify your original array and return the removed elements so you can do the following:
Next up, we can also remove elements from an array based on a condition and not just on an index with the use of Array.filter :
Differently from Array.pop , Array.shift and Array.splice , Array.filter creates a new array with all the elements that pass the condition in the callback function so your original array won't get modified as you can see from the code above.
In this case, our new Array consisted of all the elements of the original that are greater than 2.
Thank you very much for reading. Follow me on DevTo or on my blog at inspiredwebdev or on twitter . Check out Educative.io for interactive programming courses.
Disclaimer: Links to Amazon and Educative are affiliate links, purchases you make will generate extra commissions for me. Thank you

Top comments (12)
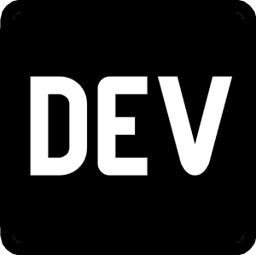
Templates let you quickly answer FAQs or store snippets for re-use.

- Location san francisco, ca
- Education university of california, berkeley
- Work señor software engineer at 📦 💨
- Joined Jun 22, 2020
Hey man -- the first section with regards to removing items from an array appears to be incorrect as Array.prototype.pop() and Array.prototype.shift() do opposite of what you say. The comment showing the results also appear to be incorrect. I believe the they should be:
Your current code:
Corrected code:
I think it's also worth noting, when introducing these topics, that there are Array methods that are mutable and others that are not (return new data structures). A lot of the ones that you've mentioned here mutate the original array. There are some tricky situations that people run into if they aren't aware of these behaviors. I know that you somewhat note that when presenting Array.prototype.filter , but it would be useful to show that the original array is left unchanged. I mention this because modern JavaScript adopts a lot of functional programming constructs, like immutable data types, that reduce bugs in code (e.g. React, Redux with ImmutableJS). To return a new data structure each time, I typically end up using Array.prototype.reduce . This is one of my most used functions or methods in various programming languages.
Otherwise, nice write-up! :)

- Location Ho Chi Minh City
- Work Software Developer at Aquipa
- Joined May 30, 2019
Awesome find, thanks for catching that error. Also thanks for the feedback, I'll try to expand on the explanation of each method :)

- Joined Oct 6, 2020
This has been very helpful. Especially this part "Replacing Items in an Array of Objects". I am building a project in React and I needed to set the state of an array of objects when one element changes
Awesome! I'm happy it helped

- Joined Jul 7, 2020
Array.prototype.splice is really cool. I think it's worth to mention that you actually can do a replace with a third argument:
const arr = [1,2,3,4,5]; arr.splice(1, 1, 0); arr; // [1,0,3,4,5]

- Location Nepal
- Joined Oct 25, 2019
"Array.pop" removes last or first? Please update the post, it's very hard to comprehend which one is which for a newbie like me. I figured out after going through MDN. Thanks for the effort.
You are correct, i should have spellchecked my article more thoroughly. The example was correct, the text was saying the opposite

- Joined May 6, 2020
Array.find will not return a Boolean value in your examples. Instead the value itself will be returned. Or the undefined, if there is no match.
You are perfectly right, i've updated the text

- Location Algeria
- Work teacher at high school
- Joined Nov 21, 2019
good job ...

- Joined Dec 19, 2020
I'm annoyed I had to create an account to help you guys out. That's not a knock on you but those who manage this site.
"Ass you can see," search for that in your article. I think you mean "As…"?

- Joined Feb 22, 2017
That's a nice one, I am late so only have origianl -> original
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
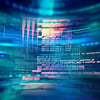
Build a Discord Bot with Discord.js V14: A Step-by-Step Guide
LordCodex - Apr 12
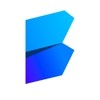
How to Use Intellicode in Visual Studio
ByteHide - Apr 12
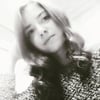
How I built CupBook
jolamemushaj - Apr 15
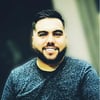
Resilience and Failure Management in DevOps
Allan Pablo - Apr 16
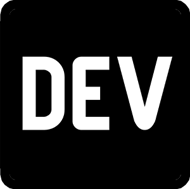
We're a place where coders share, stay up-to-date and grow their careers.

- Posted in in JavaScript
Replace Item in Array with JavaScript
- Posted by by Gaël Thomas
- 3 years ago
- Updated 1 year ago
Replace item in array using the index
One of the most common ways to replace an element in an array is to use its index.
If you know the index of the element you want to replace, you can update it.
Don’t worry if you don’t know the index of the item you want to replace. In the next part, I will show you how to find it.
Replace item in array using IndexOf
If you don’t know the index of the item you want to replace, you can use the JavaScript indexOf method to find it.
The indexOf function helps you find the book’s index in the example below.
If the element isn’t in the array, the index returned is -1 .
To perform an item replacement, you can use the returned index to update the element if it’s in the array.
If you want to have more information, you can read the indexOf documentation .
Replace item in array using Splice
Another way to replace an item in an array is by using the JavaScript splice method.
The splice function allows you to update an array’s content by removing or replacing existing elements.
As usual, if you want to replace an item, you will need its index.
Here are the parameters you will use with splice :
- index of the element to replace
- the number of elements to remove after the index (1 in your case)
- the value to add
If you read the return of splice , you will see the array’s deleted values. In your case, it will be the book at the specified index.
If you want to have more information, you can read the splice documentation .
Find and replace object in array
If you want to replace an object in an array, you can do the same as the previous ways. The main difference is the variable type. For example, you will not treat with strings but with objects.
It means that you will need to replace your value with an object.
If you want to replace an object in an array, you can find its index based on one of its property values. To do that, you can use the JavaScript findIndex method.
The findIndex function returns the index of the first element matching the condition. It also returns -1 if the condition is not met in the array.
If you want to have more information, you can read the findIndex documentation .
Join me on Twitter for daily doses of educational content to help you Unlock your Web Development skills! 🚀 From tips to tutorials, let’s learn & grow together! 📚 DMs are open, let’s connect! 🤝📬
Getting started on a solopreneur journey alongside my 9-5 🚧 Documenting my learnings and progress on Twitter (X) ✍️ Software Engineer 💻 My latest project: BooksByMood 📚

- April 24, 2021

- August 3, 2021
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Array.prototype.splice()
Baseline widely available.
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The splice() method of Array instances changes the contents of an array by removing or replacing existing elements and/or adding new elements in place .
To create a new array with a segment removed and/or replaced without mutating the original array, use toSpliced() . To access part of an array without modifying it, see slice() .
Zero-based index at which to start changing the array, converted to an integer .
- Negative index counts back from the end of the array — if -array.length <= start < 0 , start + array.length is used.
- If start < -array.length , 0 is used.
- If start >= array.length , no element will be deleted, but the method will behave as an adding function, adding as many elements as provided.
- If start is omitted (and splice() is called with no arguments), nothing is deleted. This is different from passing undefined , which is converted to 0 .
An integer indicating the number of elements in the array to remove from start .
If deleteCount is omitted, or if its value is greater than or equal to the number of elements after the position specified by start , then all the elements from start to the end of the array will be deleted. However, if you wish to pass any itemN parameter, you should pass Infinity as deleteCount to delete all elements after start , because an explicit undefined gets converted to 0 .
If deleteCount is 0 or negative, no elements are removed. In this case, you should specify at least one new element (see below).
The elements to add to the array, beginning from start .
If you do not specify any elements, splice() will only remove elements from the array.
Return value
An array containing the deleted elements.
If only one element is removed, an array of one element is returned.
If no elements are removed, an empty array is returned.
Description
The splice() method is a mutating method . It may change the content of this . If the specified number of elements to insert differs from the number of elements being removed, the array's length will be changed as well. At the same time, it uses @@species to create a new array instance to be returned.
If the deleted portion is sparse , the array returned by splice() is sparse as well, with those corresponding indices being empty slots.
The splice() method is generic . It only expects the this value to have a length property and integer-keyed properties. Although strings are also array-like, this method is not suitable to be applied on them, as strings are immutable.
Remove 0 (zero) elements before index 2, and insert "drum"
Remove 0 (zero) elements before index 2, and insert "drum" and "guitar", remove 0 (zero) elements at index 0, and insert "angel".
splice(0, 0, ...elements) inserts elements at the start of the array like unshift() .
Remove 0 (zero) elements at last index, and insert "sturgeon"
splice(array.length, 0, ...elements) inserts elements at the end of the array like push() .
Remove 1 element at index 3
Remove 1 element at index 2, and insert "trumpet", remove 2 elements from index 0, and insert "parrot", "anemone" and "blue", remove 2 elements, starting from index 2, remove 1 element from index -2, remove all elements, starting from index 2, using splice() on sparse arrays.
The splice() method preserves the array's sparseness.
Calling splice() on non-array objects
The splice() method reads the length property of this . It then updates the integer-keyed properties and the length property as needed.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Indexed collections guide
- Array.prototype.concat()
- Array.prototype.push()
- Array.prototype.pop()
- Array.prototype.shift()
- Array.prototype.slice()
- Array.prototype.toSpliced()
- Array.prototype.unshift()
Move an Array element from one Index to another in JS
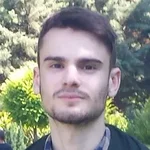
Last updated: Mar 1, 2024 Reading time · 4 min
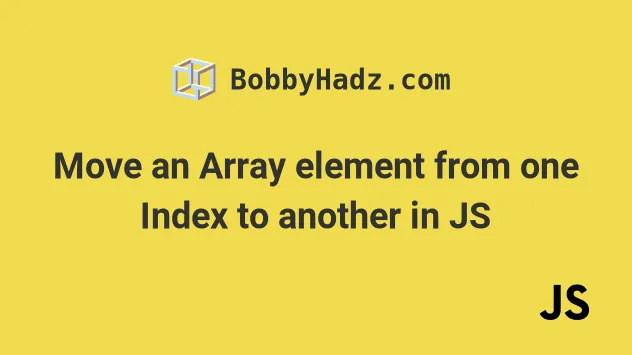
# Move an Array element from one Index to another in JS
To change the position of an element in an array:
- Use the splice() method to remove the element at the specified index from the array.
- Use the splice() method to insert the element at the new index in the array.
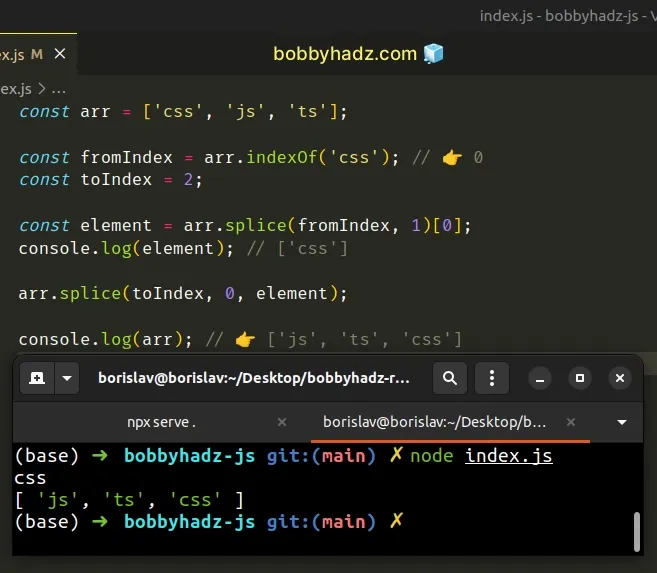
We changed the position of the array element with value css from index 0 to index 2 .
We first used the Array.indexOf() method to get the index of the element.
We then used the Array.splice() method, passing it the following 2 arguments:
- Start index - the index at which to start changing the array.
- Delete count - how many elements should be deleted from the array.
The splice() method returns an array containing the removed elements.
We know that we only deleted 1 element from the array, so we directly access the array element at index 0 to get the value of the element we will insert at the new position.
The 3rd argument we passed to the splice method is the element to add to the array.
We set the start index argument to the new position the element should be placed in.
Lastly, we set the delete count argument to 0 to denote that we don't want to remove any elements from the array.
We used the splice method to:
- Remove an element at a specific index from an array and get its value.
- Add an element to an array at a specific index.
I would have liked to have 2 separate built-in methods to achieve these 2 very different goals. However, this is the way to do it in JavaScript.
You can also shorten the code to a single line, but it becomes less readable.
# Creating a reusable function that moves an element
You can also create a reusable function that takes the array, the fromIndex and toIndex arguments and then moves the element.
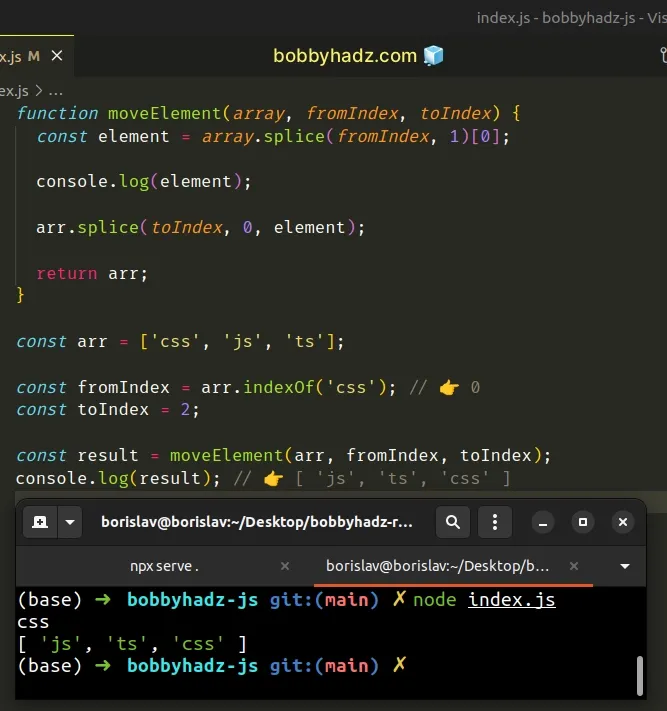
The function moves the array element in place and returns the array.
If you want to create a new array without changing the original, create a shallow copy.
We used the spread syntax (...) to create a shallow copy of the array and called the splice() method on the copy.
The moveElement() function doesn't change the original array, it returns a new array that reflects the changes.
Alternatively, you can use the array-move npm package.
# Change the Position of an Element in an Array using array-move
This is a two-step process:
- Open your terminal in your project's root directory (where your package.json file is) and install the array-move package.
- Import and use the functions the package exports to move array elements.
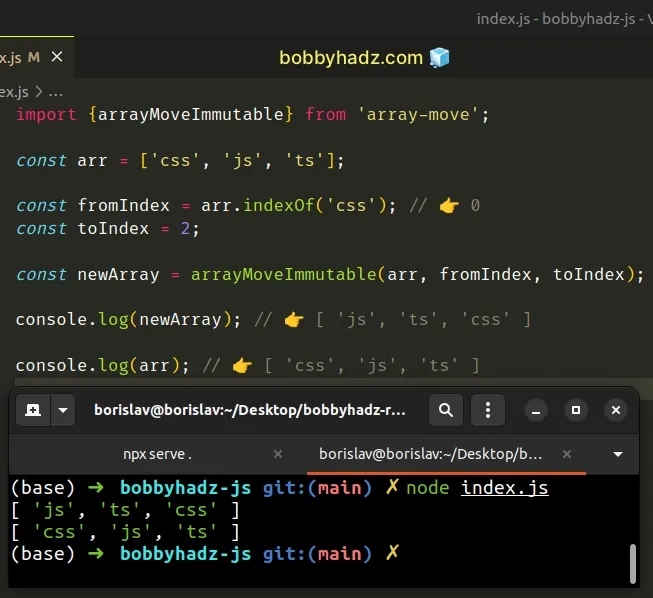
The arrayMoveImmutable function takes the array, the from index and the to index as arguments and returns a new array with the specified element moved.
The function doesn't mutate the original array in place.
If you want to change the original array in place, use the arrayMoveMutable() function.
The arrayMoveMutable() function takes the array, the from index and the to index as arguments, changes the array in place and returns undefined .
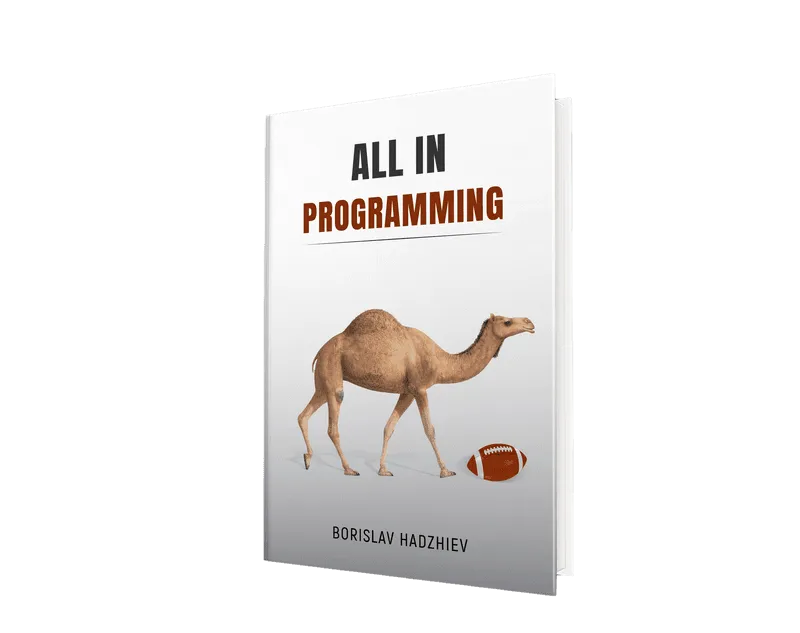
Borislav Hadzhiev
Web Developer
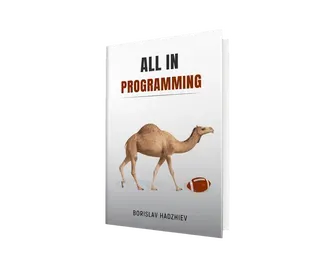
Copyright © 2024 Borislav Hadzhiev
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Program to Sort an Associative Array by its Values
- JavaScript Program to Find the First Non-Repeated Element in an Array
- JavaScript Program to Find Largest Subarray with a Sum Divisible by k
- Sparse Table Using JavaScript Array
- JavaScript Program to Search a Target Value in a Rotated Array
- JavaScript Program to Create an Array of Unique Values From Multiple Arrays Using Set Object
- JavaScript Program to Find k Most Occurrences in the Given Array
- JavaScript Program to Convert an Array into a String
- JavaScript Program to Find Median in a Stream of Integers (running integers) using Array
- JavaScript Program to Find Second Largest Element in an Array
- JavaScript Program to Find Sum of elements in a Matrix
- JavaScript Program to Find Index of an Object by Key and Value in an Array
- JavaScript Program to Create an Array with a Specific Length and Pre-filled Values
- JavaScript Program for Finding the Majority Element of an Array
- JavaScript Program for the Minimum Index of a Repeating Element in an Array
- JavaScript Program to find Maximum Distance Between two Occurrences of Same Element in Array
- JavaScript Program for Space Optimization Using bit Manipulations
- JavaScript Program to find the Longest Consecutive Sequence of Numbers in an Array
- JavaScript Program to Handle Number Overflow
JavaScript Program to change the Value of an Array Elements
In this article, we will learn how to change the value of an array element in JavaScript. Changing an element is done by using various approaches.
Changing the value of an array element in JavaScript is a common operation. Arrays in JavaScript are mutable, meaning you can modify their elements after they are created. You may want to change an element’s value based on its index or certain conditions. An item can be replaced in an array using the following approaches:
Table of Content
- Accessing Index
- Using Array Methods
- Using fill ( ) method
Method 1: Accessing Index
To change the value of an array element in JavaScript, simply access the element you want to change by its index and assign a new value to it.
Example : In this example, we will see how to change the value of array elements by Accessing its Index.
Method 2: Using Array Methods
JavaScript provides array methods like splice(), pop(), push(), shift(), and unshift(), which can be used to change elements based on specific requirements.
Example : In this example, we will see how to change the value of array elements by using array methods.
Method 3: Using fill() method
The fill() method in javascript is used to change the content of original array at specific index. It takes three parameter (element,startidx,endidx)
Example : In this example, we will see how to change the value of array elements by using fill( ) method.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- javascript-array
- JavaScript-Program
- Geeks Premier League
- Web Technologies
- What are Tiktok AI Avatars?
- Poe Introduces A Price-per-message Revenue Model For AI Bot Creators
- Truecaller For Web Now Available For Android Users In India
- Google Introduces New AI-powered Vids App
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript arrays.
An array is a special variable, which can hold more than one value:
Why Use Arrays?
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is an array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Creating an Array
Using an array literal is the easiest way to create a JavaScript Array.
It is a common practice to declare arrays with the const keyword.
Learn more about const with arrays in the chapter: JS Array Const .
Spaces and line breaks are not important. A declaration can span multiple lines:
You can also create an array, and then provide the elements:
Using the JavaScript Keyword new
The following example also creates an Array, and assigns values to it:
The two examples above do exactly the same.
There is no need to use new Array() .
For simplicity, readability and execution speed, use the array literal method.
Advertisement
Accessing Array Elements
You access an array element by referring to the index number :
Note: Array indexes start with 0.
[0] is the first element. [1] is the second element.
Changing an Array Element
This statement changes the value of the first element in cars :
Converting an Array to a String
The JavaScript method toString() converts an array to a string of (comma separated) array values.
Access the Full Array
With JavaScript, the full array can be accessed by referring to the array name:
Arrays are Objects
Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays.
But, JavaScript arrays are best described as arrays.
Arrays use numbers to access its "elements". In this example, person[0] returns John:
Objects use names to access its "members". In this example, person.firstName returns John:
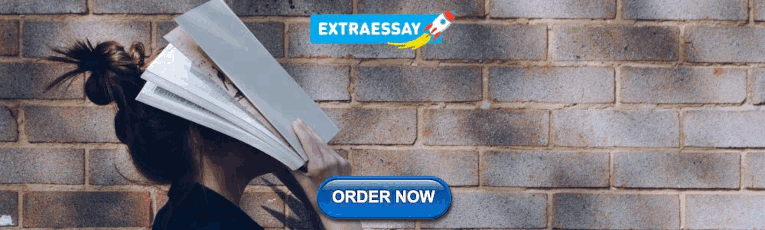
Array Elements Can Be Objects
JavaScript variables can be objects. Arrays are special kinds of objects.
Because of this, you can have variables of different types in the same Array.
You can have objects in an Array. You can have functions in an Array. You can have arrays in an Array:
Array Properties and Methods
The real strength of JavaScript arrays are the built-in array properties and methods:
Array methods are covered in the next chapters.
The length Property
The length property of an array returns the length of an array (the number of array elements).
The length property is always one more than the highest array index.
Accessing the First Array Element
Accessing the last array element, looping array elements.
One way to loop through an array, is using a for loop:
You can also use the Array.forEach() function:
Adding Array Elements
The easiest way to add a new element to an array is using the push() method:
New element can also be added to an array using the length property:
Adding elements with high indexes can create undefined "holes" in an array:
Associative Arrays
Many programming languages support arrays with named indexes.
Arrays with named indexes are called associative arrays (or hashes).
JavaScript does not support arrays with named indexes.
In JavaScript, arrays always use numbered indexes .
WARNING !! If you use named indexes, JavaScript will redefine the array to an object.
After that, some array methods and properties will produce incorrect results .
Example:
The difference between arrays and objects.
In JavaScript, arrays use numbered indexes .
In JavaScript, objects use named indexes .
Arrays are a special kind of objects, with numbered indexes.
When to Use Arrays. When to use Objects.
- JavaScript does not support associative arrays.
- You should use objects when you want the element names to be strings (text) .
- You should use arrays when you want the element names to be numbers .
JavaScript new Array()
JavaScript has a built-in array constructor new Array() .
But you can safely use [] instead.
These two different statements both create a new empty array named points:
These two different statements both create a new array containing 6 numbers:
The new keyword can produce some unexpected results:
A Common Error
is not the same as:
How to Recognize an Array
A common question is: How do I know if a variable is an array?
The problem is that the JavaScript operator typeof returns " object ":
The typeof operator returns object because a JavaScript array is an object.
Solution 1:
To solve this problem ECMAScript 5 (JavaScript 2009) defined a new method Array.isArray() :
Solution 2:
The instanceof operator returns true if an object is created by a given constructor:
Complete Array Reference
For a complete Array reference, go to our:
Complete JavaScript Array Reference .
The reference contains descriptions and examples of all Array properties and methods.
Test Yourself With Exercises
Get the value " Volvo " from the cars array.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
JavaScript Array Tutorial – Array Methods in JS

Arrays are data structures that are extremely useful and versatile. They're present in many programming languages, and they allow you to store multiple values in a single variable.
In this tutorial, we will explore how arrays work in JavaScript, their characteristics, and how to manipulate them using the most common array methods.
Table of Contents
How to create an array in javascript, array indexing, how to use the length property, multidimensional arrays, sparse arrays, how to compare arrays in javascript, the spread operator vs the rest parameter, destructuring assignment, how to add and remove elements from an array, how to combine arrays, how to convert an array into a string, how to compare arrays, how to copy an array, how to search inside an array, how to check if array elements meet a condition, how to sort an array, how to perform an operation on every array element, an introduction to arrays in js.
In JavaScript, an array is an object constituted by a group of items having a specific order . Arrays can hold values of mixed data types and their size is not fixed .
You can create an array using a literal syntax – specifying its content inside square brackets, with each item separated by a comma.
Let's create an array of strings, called nobleGases :
Alternatively, you can use the Array() constructor , passing the elements to put inside the array as arguments.
Each element inside an array is identified by its numeric index or position – starting from zero (not 1) in JavaScript, as in many programming languages. We can access elements through bracket notation , specifying the index inside square brackets.
When you try to access a value out of the index range, you get undefined as the return value. As you can see, in the example above no value is stored at index 5.
JavaScript arrays are not fixed in size . They can grow and shrink according to their content. You can easily verify this by trying to assign nobleGases[5] a value:
Now, nobleGases holds one more value, as you can see in the output.
You can check the number of elements contained inside an array using the length property, through dot notation :
The array length will be the value of the index of the last element inside the array + 1, since the indexing starts at zero.
JavaScript arrays can hold any allowed values, arrays included. An array inside another array is called a nested array. This situation creates the possibility of many array objects nested at different depths. Here's an example of a three-dimensional array:
You can access the different elements by repeating the bracket syntax with the indexes corresponding to the elements you are interested in, to go deeper and deeper. Like so:
Sparse arrays are arrays containing empty slots . For example, if you mistype two consecutive commas when creating an array, you will end up with a sparse array:
As you can see, between 'Na' and 'K' there is an empty value. This can be shown in different ways, depending on the coding environment. But it's not the same as having an undefined value.
Sparse arrays can also be created by directly changing the length property or by assignment to an index greater than the length:
Depending on the operation performed on a sparse array, empty slots can act as undefined or can be skipped .
JavaScript arrays are objects, and if you try to compare two objects, the comparison takes place considering their references – and not their actual values .
This means that you could try to compare two arrays containing the same elements – and so, that are apparently equal – like this:
But, according to JavaScript, they are not equal. And even the comparison of two empty arrays, no matter how they're created, would return the same result:
As I mentioned, this happens because object references are compared , and not their actual content. And each time you create a new array object, it will have a different reference in memory.
The only way to make this comparison evaluate to true is to make the two arrays point to the same reference. For example:
In the code above, let dough2 = dough1 does not mean you are making a copy of dough1 . It means the dough2 variable will point exactly to the same reference as dough1 . They are the same array object.
Having said that, if you want to compare two arrays, you will need to adopt a different strategy. A good approach would be iterating through the array and comparing each element one by one. You can do this with a for loop and some conditional statements:
In the code snippet above, you can see a function to check if the two arrays are equal.
- The first step is verifying if the arrays have the same length. If the length is different, they cannot be equal for sure:
- Then, a for loop iterates through the array and an if statement checks if each element of the first array is different from the element at the corresponding index in the second array:
- If no difference is caught, the arrays are equal and the function returns true .
Here's the result of comparing the two arrays from the beginning of this section with our function:
Note that we can apply this function only to an array containing primitive values . If an array contains objects, you should try to figure out the solution that fits your specific problem and deepen the check.
For example, if you know your arrays are nested, like these:
One possible solution would be the following:
With respect to the previous function, we added an additional for loop. This is enough to compare elements inside the inner arrays.
If you need to compare two arrays of objects:
You can do something like this:
- Again, the first step is verifying if the arrays have the same length. If the length is different, they cannot be equal.
- A for loop iterates through the array and an if statement checks if each object of the first array has a different length from the object at the corresponding index in the second array:
- Then a for ... in loop iterates through the properties of the i-th object of the first array. And an if statement checks if the value of each key is different from the value of the corresponding key in the i-th object of the other array:
In the end, the result would be:
Because the year value in the third object of albums2 is different. If we change it, the result will be true :
The spread operator and the rest parameter have similar syntax ( ... ) but they perform fundamentally different operations.
The spread operator enables you to expand an array – more generally an iterable object – into its elements. The rest parameter allows you to collect an undefined number of arguments into a single array .
How to Use the Spread Operator
Later on in this article, we will see some methods to copy an array or to merge different arrays. But using the spread operator is a valid alternative to do the same things.
In the example below, the alkali and alkEarth arrays are merged into a single array using the spread syntax. To do this, you need to list the arrays you want to merge between square brackets, prepending three dots to each one.
Also, you can use the same syntax with only one array, to create a copy of an array:
How to Use the Rest Parameter
The rest parameter allows you to collect an undefined number of elements into a single array. The rest parameter needs to be the last in a sequence of function parameters. Also, a function can have only one rest parameter.
In the example above, the f1 function is called with six string arguments. And the arguments after the third one are gathered inside the others array by using the rest syntax.
In general, the arguments passed to a function are collected in the arguments object, which is an array-like object and does not support the iterative methods we will see in the next section macro-section of this article.
So, the rest parameter provides a way to easily access the arguments passed to a function in array form, instead of using the arguments object:
In the example above, we have simply printed the args array, but the advantage here is being able to implement an iterative method on it.
The destructing syntax provides a simple way to assign values by unpacking them from an array object. Let's see a practical example:
The variables on the left side of the assignment operator are assigned to the value of the corresponding elements of the array on the right. You can skip array elements and go to the next ones by typing more than one comma between each variable name.
Common Array Methods in JS
In JavaScript, arrays are objects and possess properties and methods .
In this section, we will discuss some of the most common array methods you need to know to work efficiently with arrays in JavaScript.
In this section, you will see the most common ways to add and remove elements from an array in JavaScript. All the following methods mutate the original array.
How to Use the push() Method
Let's consider the example from the indexing section:
We have assigned Rn to index 5 of the nobleGases array using the bracket notation. At the end of the day, we have simply added Rn at the end of that array.
You can obtain the same result using the push() method, and you don't need to know the length of the array for that. You use the dot notation to call push() , indicating the element(s) to append inside the parenthesis. Like this:
The specified element will be added at the end of the array, returning the new array length. For example:
You can append multiple elements with push() , indicating their values separated by a comma:
How to Use the unshift() Method
Similar to push() , the unshift() method adds one or more elements to the beginning of an array and returns the length of the modified array.
For example:
How to Use the pop() Method
If you need to remove the last element of an array, you can use the pop() method.
It removes only the last element and returns it.
How to Use the shift() Method
Similarly, the shift() method removes the first element from an array and returns it.
Here's an example:
How to Use the splice() Method
If you need to remove one or more elements from a specific position of an array, you can use the splice() method.
The first parameter of splice() is the starting index , while the second is the number of items to remove from the array.
So .splice(1, 3) means "start at index = 1 and remove 3 elements". The method returns an array containing the elements removed from the original array.
If the second argument is not supplied, the elements are removed until the end.
Using splice() you can add elements, too.
If you specify additional arguments – after the starting index and the number of elements to remove – those will be inserted in the indicated position. For example:
Here, .splice(2, 1, 'Ar', 'Kr', 'Xn') means "start at index = 2 , remove 1 element and add the strings 'Ar' , 'Kr' , 'Xn' ". The array returned by the method contains the element 'Cl' , which was at index = 2 in the original array.
If you don't need to remove any elements from the array, you can simply use zero as the second argument. The elements will be added starting at the specified index, without removing any item:
How to Use the concat() Method
If you need to combine two or more arrays – that is create a single array containing each element of the arrays you want to merge – you can use the concat() method. This method does not change the original arrays and returns a new array.
You need to call .concat() on the array that should come first, passing as arguments the arrays you want it to merge with. The order will be reflected in the resulting array.
Here's an example of combining two and three arrays:
How to Use the push() Method & the Spread Operator
If you don't mind changing the original array you can combine a .push() call to the spread syntax ( ... ) to add all the elements in one or more arrays to the original array. For example:
You cannot use push() without the spread syntax in its arguments, unless you want to nest the whole array moreAlkali as the last element of alkali . In that case, the result would be ['Li', 'Na', 'K', ['Rb', 'Cs', 'Fr']] – an array composed of 4 elements with the last being an array.
Note that, as we have seen previously, the spread operator alone allows you to merge two or more arrays without causing any mutation. As a continuation of the previous example:
If you need to convert an array into a string, you have different options. And now, we are going to see some of them. Note that the following methods do not mutate the original array.
How to Use the toString() & join() Methods
These methods enable you to convert arrays into strings.
The toString() method is called without a parameter and returns a string representing the content of the array.
The join() method takes a separator as the argument, which is used to separate the array elements, in order to form the string.
These two methods have some limitations. If we consider the array in the following example, we can observe a couple of interesting things:
First, null and undefined result in the same string output (an empty substring).
Second, the string representation of an object is [object Object] . So if you are trying to convert an array containing objects into a string, you should employ another method. Otherwise, you will not be able to see the object content properly.
How to Use the JSON.stringify() Method
If you want to convert an array containing objects into a string, the JSON.stringify() method is what you need. Where the previous methods fail, JSON.stringify() enables you to handle objects properly.
This method takes a JavaScript value as the argument – in this case the albums array – and converts it to a JSON string.
As you can see, the square brackets are retained, so it is often desirable to use this method to create a string from an array.
Since arrays are objects, their comparison is based on references . Not on the actual values.
Before, we have seen some ways to compare arrays by looping through an array and comparing each element.
Another approach for comparing arrays is converting them into strings with one of the previous methods, and then comparing the string representations of the original arrays.
This is quite fast and easy, but sometimes it can lead to unexpected behavior. For example, when null and undefined values are compared.
You might think that the comparison between the string representation of a and b would return false , since null and undefined are not equal. But in practice, they are both stringified to null.
In light of this aspect, it would be better to use an iterative technique.
How to Use the every() Method
every() is an iterative method that verifies if all the elements in the array pass a condition implemented by a callback function and it returns true or false .
Among its many uses, you can build a simple function to compare arrays containing primitive values with every() , like this:
- First, the lengths are compared. If they are not equal, the arrays are not equal as well.
- Then, every() is called on the first array. The callback checks if every element of arr1 is equal to the element at the corresponding index in arr2 .
The AND operator ensures that true is returned only when both conditions are true.
Here's the function applied to the arrays from before:
All common operations to copy an array in JavaScript generate a shallow copy – instead of a deep copy – of the original array. This means that by mutating the copy, you can change the original array, too. We will see why this happens in a while.
How to Use the slice() Method
The slice() method allows you to copy an entire array – or just a portion of it – without mutating it.
As parameters, it takes the starting index and the final index (not included) to copy. When called without arguments, slice() create a duplicate of the whole array. For example:
If you try to change doughCopy in some way, for example, assigning doughCopy[1] a new value, you would see that no change is reflected in the original array:
This happens because the array is filled with primitive values. However, the story is quite different if you handle an array containing non-primitive values.
Let's consider the following array, with two objects:
You copy the array using the slice() method, like this:
Now, albumsCopy represents a shallow copy of albums and the elements inside each array point to the same objects. In other words, both albums[0] === albumsCopy[0] and albums[1] === albumsCopy[1] return true – remember that this comparison involves object references – because they are the very same objects.
If you change one of them by mutating a property value, the modification involves the other array, too.
Note that if you reassign an element to a different object – that is without mutating any of the existent objects – the modification does not involve the other array:
How to Use the map() Method
The map() method generates a new array containing the result of calling a callback function on every element of an array.
The function takes the current element, its index, and the array on which the method is called, as parameters.
You can use map() to copy an array by specifying a function that returns each array element:
How to Create a Deep Copy
If you want to create a deep clone of an array, you can convert the array into a string with JSON.stringify() and pass its return value to the JSON.parse() method.
In this way, the copy will be completely independent of the original array and you will not risk an unintentional modification.
Depending on what you are looking for, there are several ways to search inside an array. Let's explore some methods to search inside an array by index and by value.
How to Use the includes() Method
If you need to know whether a value is included in an array, you can call the includes() method on it, passing the value you are interested in as the argument.
This method returns true if the value is found. Otherwise, false .
It accepts also a second parameter, representing the index where to begin to search – the default is zero.
How to Use the indexOf() Method
If you need to know the index at which a specific value can be found in an array, you should use the indexOf() method.
It returns only the first index at which the specified value is found, otherwise, it returns -1. The second parameter is the index for where to start searching for the value – the default is zero.
How to Use the find() & findLast() Methods
find() and findLast() enable you to search for the first and the last element that satisfies a certain condition in an array, respectively.
They both accept a callback function, whose parameters are the current element, its index, and the array the method is called upon.
find() and findLast() return the first/last element that satisfies the function, or undefined when the no value matches the specified condition.
In the example above, only the first and the last objects containing 'Pigs' are found. The middle object {no: 3, track: 'Pigs (Three Different Ones)'} cannot be reached by these two methods.
How to Use the findIndex() & findLastIndex() Methods
The findIndex() and findLastIndex() methods work similarly to the previous ones.
But they return the index of the first and the last element that satisfies the provided condition, respectively, or undefined when the no value matches the specified condition.
How to Use the every() & some() Methods
Sometimes you want to verify if the elements inside an array satisfy a specific condition. We have already seen the every() method in a previous section. It loops through the array and returns true if all the elements meet the specified condition. Otherwise, it returns false .
The some() method is very similar. It iterates through the array, testing if some elements – not all of them – meet the requirements implemented by a callback function.
The last call returns false since none of the array elements is equal to the string 'Rn' .
How to Use the filter() Method
This method provides you a way to filter the array elements that satisfy a certain criterion.
filter() takes a callback function, whose parameters are the current element, its index, and the array the method is called upon.
It creates a shallow copy of the original array containing only the values for which the callback returns a truthy value, and it neglects the others.
Above, only the elements including 'Pigs' are inserted in the filtered array.
How to Use the sort() Method
If you want to sort an array, you can use sort() . This method sorts the array elements in place . It changes the array which it's acting on.
The default sorting procedure evaluates Unicode point values and sometimes may lead to unexpected outcomes. For this reason, it is better to pass sort() a callback function so that the elements can be sorted according to the return value of the callback.
The following table sums up the sorting criterion at the base of sort() .
The elements – represented by a and b parameters – are compared two at a time. If the return value is positive, a is placed after b. If it is negative, b is placed after a. While if the return value is zero the original order is kept.
Here's an example of sorting an array of strings in ascending and descending order:
The callback function is implemented by a ternary operator, in order to consider the three possible outcomes of the comparison.
Previously, we used map() to duplicate an array. But by using a different callback function you can perform many different operations.
In the example above, we have used map() to create an array populated with the values of the track key of each object in the animals array.
How to Use the forEach() Method
The forEach() method is similar to map() . It executes a function on every array element, but it has no return value. For this reason, a forEach() call can be used only at the end of a chain.
In the example below, forEach() is used to delete the no property from each array element:
How to Use the reduce() Method
The reduce() method accepts a callback function, which is executed on each array element. The callback takes an accumulator as the first parameter, followed by the current element, its index, and the array which the method is called on.
The return value of each iteration is passed to the next one. So that the array is reduced to a single value. The second parameter of reduce() is the starting value of the accumulator . If not specified, accumulator takes the first array value and the iteration starts at index 1.
In the example below, the reduce() method is used to count the number of tracks that include 'Pigs' in the title. The method iterates through the array, and when the track property includes 'Pigs', the value of count is incremented and passed to the next iteration.
In this case, it's important to specify the initial value as zero. Otherwise, the initial value will be the whole {no: 1, track: 'Pigs on the Wing (Part One)'} object, and this will lead to an unexpected result.
In JavaScript, arrays are data structures that contain multiple values in a specific order. They can hold values of different data types and they are re-sizable.
In this tutorial, we started with the basics of arrays in JavaScript and then we discussed some of the most common methods that allow you to manipulate arrays.
We have only begun to scratch the surface of this wide topic, but I hope this is a good starting point for you.
Thanks for reading, and happy coding.
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
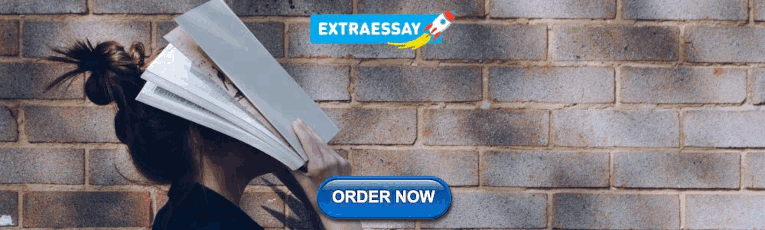
IMAGES
VIDEO
COMMENTS
To replace the first item (n=1) in the array, write: items[0] = Enter Your New Number; In your example, the number 3452 is in the second position (n=2). So the formula to determine the index number is 2-1 = 1. So write the following code to replace 3452 with 1010: items[1] = 1010; edited May 23, 2017 at 1:42. Jon Saw.
This quick and straightforward article shows you a couple of different ways to update or replace a specific element in an array in modern JavaScript. Sling S Academy A. ... with JS Disable Button After Click JS Auto-Save Form BOM The Modern JavaScript BOM Cheat Sheet Detect Dark/Light Mode Change Background Color on Scroll Get the Width ...
In practice, we remove the array element at the specified index and then insert a different value at the same index, so we end up replacing the array element. An alternative approach is to use a basic for loop. # Replace an Element in an Array using a for loop. This is a three-step process: Use a for loop to iterate for array.length iterations.
So for your code above, this means that each time the forEach () iterates, part is equal to the same value as arr[index], but not the same object. part = "four"; will change the part variable, but will leave arr alone. The following code will change the values you desire: var arr = ["one","two","three"];
Declaring an array: let myBox = []; // Initial Array declaration in JS. Arrays can contain multiple data types. let myBox = ['hello', 1, 2, 3, true, 'hi']; Arrays can be manipulated by using several actions known as methods. Some of these methods allow us to add, remove, modify and do lots more to arrays.
The function we passed to Array.map() gets called with each element in the array and its index. On each iteration, we modify the value of the current element using the index and return the result. The map() method returns a new array containing the values returned from the callback function. # Update all Elements in an Array using Array.reduce() This is a two-step process:
The array type in JavaScript provides us with the splice () method that helps us in order to replace the items of an existing array by removing and inserting new elements at the required/desired index. Syntax: Array.splice(start_index, delete_count, value1, value2, value3, ...) Note: Splice () method deletes zero or more elements of an array ...
Differently from Array.pop, Array.shift and Array.splice, Array.filter creates a new array with all the elements that pass the condition in the callback function so your original array won't get modified as you can see from the code above. In this case, our new Array consisted of all the elements of the original that are greater than 2.
Replace item in array using IndexOf. If you don't know the index of the item you want to replace, you can use the JavaScript indexOf method to find it. The indexOf function helps you find the book's index in the example below. If the element isn't in the array, the index returned is -1. const books = [. "You don't know JS", // Index 0.
The splice() method is a mutating method.It may change the content of this.If the specified number of elements to insert differs from the number of elements being removed, the array's length will be changed as well. At the same time, it uses @@species to create a new array instance to be returned.. If the deleted portion is sparse, the array returned by splice() is sparse as well, with those ...
Here is an example of an array with four elements: type Number, Boolean, String, and Object. const mixedTypedArray = [100, true, 'freeCodeCamp', {}]; The position of an element in the array is known as its index. In JavaScript, the array index starts with 0, and it increases by one with each element.
The JavaScript Console returns the same list of elements that you put in in the previous step. Change the value of the first element by entering this statement, and then press Return or Enter: people[0] = "Georgie";
We used the spread syntax (...) to create a shallow copy of the array and called the splice() method on the copy.. The moveElement() function doesn't change the original array, it returns a new array that reflects the changes.. Alternatively, you can use the array-move npm package. # Change the Position of an Element in an Array using array-move This is a two-step process:
Changing the value of an array element in JavaScript is a common operation. Arrays in JavaScript are mutable, meaning you can modify their elements after they are created. You may want to change an element's value based on its index or certain conditions. An item can be replaced in an array using the following approaches:
Try it Yourself ». The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice() method returns an array with the deleted items:
How to Swap Two Array Elements by Destructuring. A far better method you can use to swap array elements is destructuring, as it does the job in only one line of code. You just create a new array containing both elements in a particular order, then assign it to a new array that contains both elements in the reversed order.
Creating an Array. Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [ item1, item2, ... ]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const.
This means that splice() can be used to remove elements, add elements, or replace elements in an array, depending on the arguments you pass. Note that it returns an array of the removed elements. Something nice and generic would be: this.splice(to, 0, this.splice(from, 1)[0]); Then just use:
In this section, you will see the most common ways to add and remove elements from an array in JavaScript. All the following methods mutate the original array. ... of the original array. This means that by mutating the copy, you can change the original array, too. We will see why this happens in a while. How to Use the slice() Method.
How to change value of an array element through javascript? 1. How do I change the element value in an object array. 0. ... Change value of an object array element inside an array- js. Hot Network Questions Would medieval humans know if an ancient civilization had existed and been wiped out?
For example, say you've got an array of numbers and you want to square them all. Then you could do var squared = numbers.map(x => x * x). It creates a new array for each element in the original array, where the new value is what's returned from the function you passed it. - Mike Cluck. Mar 9, 2016 at 15:50.