Declaration
There are two syntaxes for creating an empty array:
Almost all the time, the second syntax is used. We can supply initial elements in the brackets:
Array elements are numbered, starting with zero.
We can get an element by its number in square brackets:
We can replace an element:
…Or add a new one to the array:
The total count of the elements in the array is its length :
We can also use alert to show the whole array.
An array can store elements of any type.
For instance:
An array, just like an object, may end with a comma:
The “trailing comma” style makes it easier to insert/remove items, because all lines become alike.
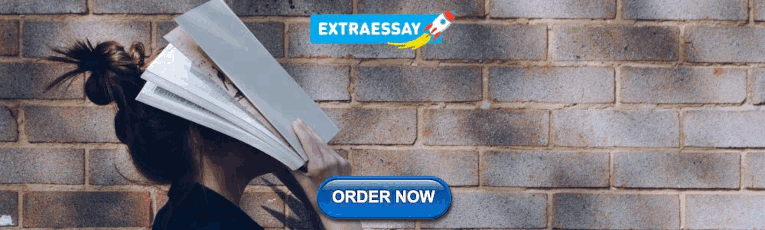
Get last elements with “at”
Let’s say we want the last element of the array.
Some programming languages allow the use of negative indexes for the same purpose, like fruits[-1] .
Although, in JavaScript it won’t work. The result will be undefined , because the index in square brackets is treated literally.
We can explicitly calculate the last element index and then access it: fruits[fruits.length - 1] .
A bit cumbersome, isn’t it? We need to write the variable name twice.
Luckily, there’s a shorter syntax: fruits.at(-1) :
In other words, arr.at(i) :
- is exactly the same as arr[i] , if i >= 0 .
- for negative values of i , it steps back from the end of the array.
Methods pop/push, shift/unshift
A queue is one of the most common uses of an array. In computer science, this means an ordered collection of elements which supports two operations:
- push appends an element to the end.
- shift get an element from the beginning, advancing the queue, so that the 2nd element becomes the 1st.
Arrays support both operations.
In practice we need it very often. For example, a queue of messages that need to be shown on-screen.
There’s another use case for arrays – the data structure named stack .
It supports two operations:
- push adds an element to the end.
- pop takes an element from the end.
So new elements are added or taken always from the “end”.
A stack is usually illustrated as a pack of cards: new cards are added to the top or taken from the top:
For stacks, the latest pushed item is received first, that’s also called LIFO (Last-In-First-Out) principle. For queues, we have FIFO (First-In-First-Out).
Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements, both to/from the beginning or the end.
In computer science, the data structure that allows this, is called deque .
Methods that work with the end of the array:
Extracts the last element of the array and returns it:
Both fruits.pop() and fruits.at(-1) return the last element of the array, but fruits.pop() also modifies the array by removing it.
Append the element to the end of the array:
The call fruits.push(...) is equal to fruits[fruits.length] = ... .
Methods that work with the beginning of the array:
Extracts the first element of the array and returns it:
Add the element to the beginning of the array:
Methods push and unshift can add multiple elements at once:
An array is a special kind of object. The square brackets used to access a property arr[0] actually come from the object syntax. That’s essentially the same as obj[key] , where arr is the object, while numbers are used as keys.
They extend objects providing special methods to work with ordered collections of data and also the length property. But at the core it’s still an object.
Remember, there are only eight basic data types in JavaScript (see the Data types chapter for more info). Array is an object and thus behaves like an object.
For instance, it is copied by reference:
…But what makes arrays really special is their internal representation. The engine tries to store its elements in the contiguous memory area, one after another, just as depicted on the illustrations in this chapter, and there are other optimizations as well, to make arrays work really fast.
But they all break if we quit working with an array as with an “ordered collection” and start working with it as if it were a regular object.
For instance, technically we can do this:
That’s possible, because arrays are objects at their base. We can add any properties to them.
But the engine will see that we’re working with the array as with a regular object. Array-specific optimizations are not suited for such cases and will be turned off, their benefits disappear.
The ways to misuse an array:
- Add a non-numeric property like arr.test = 5 .
- Make holes, like: add arr[0] and then arr[1000] (and nothing between them).
- Fill the array in the reverse order, like arr[1000] , arr[999] and so on.
Please think of arrays as special structures to work with the ordered data . They provide special methods for that. Arrays are carefully tuned inside JavaScript engines to work with contiguous ordered data, please use them this way. And if you need arbitrary keys, chances are high that you actually require a regular object {} .
Performance
Methods push/pop run fast, while shift/unshift are slow.
Why is it faster to work with the end of an array than with its beginning? Let’s see what happens during the execution:
It’s not enough to take and remove the element with the index 0 . Other elements need to be renumbered as well.
The shift operation must do 3 things:
- Remove the element with the index 0 .
- Move all elements to the left, renumber them from the index 1 to 0 , from 2 to 1 and so on.
- Update the length property.
The more elements in the array, the more time to move them, more in-memory operations.
The similar thing happens with unshift : to add an element to the beginning of the array, we need first to move existing elements to the right, increasing their indexes.
And what’s with push/pop ? They do not need to move anything. To extract an element from the end, the pop method cleans the index and shortens length .
The actions for the pop operation:
The pop method does not need to move anything, because other elements keep their indexes. That’s why it’s blazingly fast.
The similar thing with the push method.
One of the oldest ways to cycle array items is the for loop over indexes:
But for arrays there is another form of loop, for..of :
The for..of doesn’t give access to the number of the current element, just its value, but in most cases that’s enough. And it’s shorter.
Technically, because arrays are objects, it is also possible to use for..in :
But that’s actually a bad idea. There are potential problems with it:
The loop for..in iterates over all properties , not only the numeric ones.
There are so-called “array-like” objects in the browser and in other environments, that look like arrays . That is, they have length and indexes properties, but they may also have other non-numeric properties and methods, which we usually don’t need. The for..in loop will list them though. So if we need to work with array-like objects, then these “extra” properties can become a problem.
The for..in loop is optimized for generic objects, not arrays, and thus is 10-100 times slower. Of course, it’s still very fast. The speedup may only matter in bottlenecks. But still we should be aware of the difference.
Generally, we shouldn’t use for..in for arrays.
A word about “length”
The length property automatically updates when we modify the array. To be precise, it is actually not the count of values in the array, but the greatest numeric index plus one.
For instance, a single element with a large index gives a big length:
Note that we usually don’t use arrays like that.
Another interesting thing about the length property is that it’s writable.
If we increase it manually, nothing interesting happens. But if we decrease it, the array is truncated. The process is irreversible, here’s the example:
So, the simplest way to clear the array is: arr.length = 0; .
new Array()
There is one more syntax to create an array:
It’s rarely used, because square brackets [] are shorter. Also, there’s a tricky feature with it.
If new Array is called with a single argument which is a number, then it creates an array without items, but with the given length .
Let’s see how one can shoot themselves in the foot:
To avoid such surprises, we usually use square brackets, unless we really know what we’re doing.
Multidimensional arrays
Arrays can have items that are also arrays. We can use it for multidimensional arrays, for example to store matrices:
Arrays have their own implementation of toString method that returns a comma-separated list of elements.
Also, let’s try this:
Arrays do not have Symbol.toPrimitive , neither a viable valueOf , they implement only toString conversion, so here [] becomes an empty string, [1] becomes "1" and [1,2] becomes "1,2" .
When the binary plus "+" operator adds something to a string, it converts it to a string as well, so the next step looks like this:
Don’t compare arrays with ==
Arrays in JavaScript, unlike some other programming languages, shouldn’t be compared with operator == .
This operator has no special treatment for arrays, it works with them as with any objects.
Let’s recall the rules:
- Two objects are equal == only if they’re references to the same object.
- If one of the arguments of == is an object, and the other one is a primitive, then the object gets converted to primitive, as explained in the chapter Object to primitive conversion .
- …With an exception of null and undefined that equal == each other and nothing else.
The strict comparison === is even simpler, as it doesn’t convert types.
So, if we compare arrays with == , they are never the same, unless we compare two variables that reference exactly the same array.
For example:
These arrays are technically different objects. So they aren’t equal. The == operator doesn’t do item-by-item comparison.
Comparison with primitives may give seemingly strange results as well:
Here, in both cases, we compare a primitive with an array object. So the array [] gets converted to primitive for the purpose of comparison and becomes an empty string '' .
Then the comparison process goes on with the primitives, as described in the chapter Type Conversions :
So, how to compare arrays?
That’s simple: don’t use the == operator. Instead, compare them item-by-item in a loop or using iteration methods explained in the next chapter.
Array is a special kind of object, suited to storing and managing ordered data items.
The declaration:
The call to new Array(number) creates an array with the given length, but without elements.
- The length property is the array length or, to be precise, its last numeric index plus one. It is auto-adjusted by array methods.
- If we shorten length manually, the array is truncated.
Getting the elements:
- we can get element by its index, like arr[0]
- also we can use at(i) method that allows negative indexes. For negative values of i , it steps back from the end of the array. If i >= 0 , it works same as arr[i] .
We can use an array as a deque with the following operations:
- push(...items) adds items to the end.
- pop() removes the element from the end and returns it.
- shift() removes the element from the beginning and returns it.
- unshift(...items) adds items to the beginning.
To loop over the elements of the array:
- for (let i=0; i<arr.length; i++) – works fastest, old-browser-compatible.
- for (let item of arr) – the modern syntax for items only,
- for (let i in arr) – never use.
To compare arrays, don’t use the == operator (as well as > , < and others), as they have no special treatment for arrays. They handle them as any objects, and it’s not what we usually want.
Instead you can use for..of loop to compare arrays item-by-item.
We will continue with arrays and study more methods to add, remove, extract elements and sort arrays in the next chapter Array methods .
Is array copied?
What is this code going to show?
The result is 4 :
That’s because arrays are objects. So both shoppingCart and fruits are the references to the same array.
Array operations.
Let’s try 5 array operations.
- Create an array styles with items “Jazz” and “Blues”.
- Append “Rock-n-Roll” to the end.
- Replace the value in the middle with “Classics”. Your code for finding the middle value should work for any arrays with odd length.
- Strip off the first value of the array and show it.
- Prepend Rap and Reggae to the array.
The array in the process:
Calling in an array context
What is the result? Why?
The call arr[2]() is syntactically the good old obj[method]() , in the role of obj we have arr , and in the role of method we have 2 .
So we have a call of the function arr[2] as an object method. Naturally, it receives this referencing the object arr and outputs the array:
The array has 3 values: initially it had two, plus the function.
Sum input numbers
Write the function sumInput() that:
- Asks the user for values using prompt and stores the values in the array.
- Finishes asking when the user enters a non-numeric value, an empty string, or presses “Cancel”.
- Calculates and returns the sum of array items.
P.S. A zero 0 is a valid number, please don’t stop the input on zero.
Run the demo
Please note the subtle, but important detail of the solution. We don’t convert value to number instantly after prompt , because after value = +value we would not be able to tell an empty string (stop sign) from the zero (valid number). We do it later instead.
A maximal subarray
The input is an array of numbers, e.g. arr = [1, -2, 3, 4, -9, 6] .
The task is: find the contiguous subarray of arr with the maximal sum of items.
Write the function getMaxSubSum(arr) that will return that sum.
If all items are negative, it means that we take none (the subarray is empty), so the sum is zero:
Please try to think of a fast solution: O(n 2 ) or even O(n) if you can.
Open a sandbox with tests.
Slow solution
We can calculate all possible subsums.
The simplest way is to take every element and calculate sums of all subarrays starting from it.
For instance, for [-1, 2, 3, -9, 11] :
The code is actually a nested loop: the external loop over array elements, and the internal counts subsums starting with the current element.
The solution has a time complexity of O(n 2 ) . In other words, if we increase the array size 2 times, the algorithm will work 4 times longer.
For big arrays (1000, 10000 or more items) such algorithms can lead to serious sluggishness.
Fast solution
Let’s walk the array and keep the current partial sum of elements in the variable s . If s becomes negative at some point, then assign s=0 . The maximum of all such s will be the answer.
If the description is too vague, please see the code, it’s short enough:
The algorithm requires exactly 1 array pass, so the time complexity is O(n).
You can find more detailed information about the algorithm here: Maximum subarray problem . If it’s still not obvious why that works, then please trace the algorithm on the examples above, see how it works, that’s better than any words.
Open the solution with tests in a sandbox.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
- Overview: JavaScript first steps
In the final article of this module, we'll look at arrays — a neat way of storing a list of data items under a single variable name. Here we look at why this is useful, then explore how to create an array, retrieve, add, and remove items stored in an array, and more besides.
What is an array?
Arrays are generally described as "list-like objects"; they are basically single objects that contain multiple values stored in a list. Array objects can be stored in variables and dealt with in much the same way as any other type of value, the difference being that we can access each value inside the list individually, and do super useful and efficient things with the list, like loop through it and do the same thing to every value. Maybe we've got a series of product items and their prices stored in an array, and we want to loop through them all and print them out on an invoice, while totaling all the prices together and printing out the total price at the bottom.
If we didn't have arrays, we'd have to store every item in a separate variable, then call the code that does the printing and adding separately for each item. This would be much longer to write out, less efficient, and more error-prone. If we had 10 items to add to the invoice it would already be annoying, but what about 100 items, or 1000? We'll return to this example later on in the article.
As in previous articles, let's learn about the real basics of arrays by entering some examples into browser developer console .
Creating arrays
Arrays consist of square brackets and items that are separated by commas.
- Suppose we want to store a shopping list in an array. Paste the following code into the console: js const shopping = [ "bread" , "milk" , "cheese" , "hummus" , "noodles" ] ; console . log ( shopping ) ;
- In the above example, each item is a string, but in an array we can store various data types — strings, numbers, objects, and even other arrays. We can also mix data types in a single array — we do not have to limit ourselves to storing only numbers in one array, and in another only strings. For example: js const sequence = [ 1 , 1 , 2 , 3 , 5 , 8 , 13 ] ; const random = [ "tree" , 795 , [ 0 , 1 , 2 ] ] ;
- Before proceeding, create a few example arrays.
Finding the length of an array
You can find out the length of an array (how many items are in it) in exactly the same way as you find out the length (in characters) of a string — by using the length property. Try the following:
Accessing and modifying array items
Items in an array are numbered, starting from zero. This number is called the item's index . So the first item has index 0, the second has index 1, and so on. You can access individual items in the array using bracket notation and supplying the item's index, in the same way that you accessed the letters in a string .
- Enter the following into your console: js const shopping = [ "bread" , "milk" , "cheese" , "hummus" , "noodles" ] ; console . log ( shopping [ 0 ] ) ; // returns "bread"
Note: We've said it before, but just as a reminder — computers start counting from 0!
- Note that an array inside an array is called a multidimensional array. You can access an item inside an array that is itself inside another array by chaining two sets of square brackets together. For example, to access one of the items inside the array that is the third item inside the random array (see previous section), we could do something like this: js const random = [ "tree" , 795 , [ 0 , 1 , 2 ] ] ; random [ 2 ] [ 2 ] ;
- Try making some more modifications to your array examples before moving on. Play around a bit, and see what works and what doesn't.
Finding the index of items in an array
If you don't know the index of an item, you can use the indexOf() method. The indexOf() method takes an item as an argument and will either return the item's index or -1 if the item is not in the array:
Adding items
To add one or more items to the end of an array we can use push() . Note that you need to include one or more items that you want to add to the end of your array.
The new length of the array is returned when the method call completes. If you wanted to store the new array length in a variable, you could do something like this:
To add an item to the start of the array, use unshift() :
Removing items
To remove the last item from the array, use pop() .
The pop() method returns the item that was removed. To save that item in a new variable, you could do this:
To remove the first item from an array, use shift() :
If you know the index of an item, you can remove it from the array using splice() :
In this call to splice() , the first argument says where to start removing items, and the second argument says how many items should be removed. So you can remove more than one item:
Accessing every item
Very often you will want to access every item in the array. You can do this using the for...of statement:
Sometimes you will want to do the same thing to each item in an array, leaving you with an array containing the changed items. You can do this using map() . The code below takes an array of numbers and doubles each number:
We give a function to the map() , and map() calls the function once for each item in the array, passing in the item. It then adds the return value from each function call to a new array, and finally returns the new array.
Sometimes you'll want to create a new array containing only the items in the original array that match some test. You can do that using filter() . The code below takes an array of strings and returns an array containing just the strings that are greater than 8 characters long:
Like map() , we give a function to the filter() method, and filter() calls this function for every item in the array, passing in the item. If the function returns true , then the item is added to a new array. Finally it returns the new array.
Converting between strings and arrays
Often you'll be presented with some raw data contained in a big long string, and you might want to separate the useful items out into a more useful form and then do things to them, like display them in a data table. To do this, we can use the split() method. In its simplest form, this takes a single parameter, the character you want to separate the string at, and returns the substrings between the separator as items in an array.
Note: Okay, this is technically a string method, not an array method, but we've put it in with arrays as it goes well here.
- Let's play with this, to see how it works. First, create a string in your console: js const data = "Manchester,London,Liverpool,Birmingham,Leeds,Carlisle" ;
- Now let's split it at each comma: js const cities = data . split ( "," ) ; cities ;
- Finally, try finding the length of your new array, and retrieving some items from it: js cities . length ; cities [ 0 ] ; // the first item in the array cities [ 1 ] ; // the second item in the array cities [ cities . length - 1 ] ; // the last item in the array
- You can also go the opposite way using the join() method. Try the following: js const commaSeparated = cities . join ( "," ) ; commaSeparated ;
- Another way of converting an array to a string is to use the toString() method. toString() is arguably simpler than join() as it doesn't take a parameter, but more limiting. With join() you can specify different separators, whereas toString() always uses a comma. (Try running Step 4 with a different character than a comma.) js const dogNames = [ "Rocket" , "Flash" , "Bella" , "Slugger" ] ; dogNames . toString ( ) ; // Rocket,Flash,Bella,Slugger
Active learning: Printing those products
Let's return to the example we described earlier — printing out product names and prices on an invoice, then totaling the prices and printing them at the bottom. In the editable example below there are comments containing numbers — each of these marks a place where you have to add something to the code. They are as follows:
- Below the // number 1 comment are a number of strings, each one containing a product name and price separated by a colon. We'd like you to turn this into an array and store it in an array called products .
- Below the // number 2 comment, start a for...of() loop to go through every item in the products array.
- Below the // number 3 comment we want you to write a line of code that splits the current array item ( name:price ) into two separate items, one containing just the name and one containing just the price. If you are not sure how to do this, consult the Useful string methods article for some help, or even better, look at the Converting between strings and arrays section of this article.
- As part of the above line of code, you'll also want to convert the price from a string to a number. If you can't remember how to do this, check out the first strings article .
- There is a variable called total that is created and given a value of 0 at the top of the code. Inside the loop (below // number 4 ) we want you to add a line that adds the current item price to that total in each iteration of the loop, so that at the end of the code the correct total is printed onto the invoice. You might need an assignment operator to do this.
- We want you to change the line just below // number 5 so that the itemText variable is made equal to "current item name — $current item price", for example "Shoes — $23.99" in each case, so the correct information for each item is printed on the invoice. This is just simple string concatenation, which should be familiar to you.
- Finally, below the // number 6 comment, you'll need to add a } to mark the end of the for...of() loop.
Active learning: Top 5 searches
A good use for array methods like push() and pop() is when you are maintaining a record of currently active items in a web app. In an animated scene for example, you might have an array of objects representing the background graphics currently displayed, and you might only want 50 displayed at once, for performance or clutter reasons. As new objects are created and added to the array, older ones can be deleted from the array to maintain the desired number.
In this example we're going to show a much simpler use — here we're giving you a fake search site, with a search box. The idea is that when terms are entered in the search box, the top 5 previous search terms are displayed in the list. When the number of terms goes over 5, the last term starts being deleted each time a new term is added to the top, so the 5 previous terms are always displayed.
Note: In a real search app, you'd probably be able to click the previous search terms to return to previous searches, and it would display actual search results! We are just keeping it simple for now.
To complete the app, we need you to:
- Add a line below the // number 1 comment that adds the current value entered into the search input to the start of the array. This can be retrieved using searchInput.value .
- Add a line below the // number 2 comment that removes the value currently at the end of the array.
Test your skills!
You've reached the end of this article, but can you remember the most important information? You can find some further tests to verify that you've retained this information before you move on — see Test your skills: Arrays .
After reading through this article, we are sure you will agree that arrays seem pretty darn useful; you'll see them crop up everywhere in JavaScript, often in association with loops in order to do the same thing to every item in an array. We'll be teaching you all the useful basics there are to know about loops in the next module, but for now you should give yourself a clap and take a well-deserved break; you've worked through all the articles in this module!
The only thing left to do is work through this module's assessment, which will test your understanding of the articles that came before it.
- Indexed collections — an advanced level guide to arrays and their cousins, typed arrays.
- Array — the Array object reference page — for a detailed reference guide to the features discussed in this page, and many more.
Ace your Coding Interview
- DSA Problems
- Binary Tree
- Binary Search Tree
- Dynamic Programming
- Divide and Conquer
- Linked List
- Backtracking
Declare and Initialize arrays in JavaScript
This post will discuss how to declare and initialize arrays in JavaScript.
There are several ways to declare and initialize arrays in JavaScript. An array is a collection of values that can be accessed by an index. Here are some of the common functions:
1. Using an Array constructor
We can use the Array() constructor to create an array using the new keyword and the Array function. We can pass one or more values as arguments to the function. If only a single value is passed to the Array constructor, an empty array of that length is created, with all its elements undefined. However, if more than one values is passed to the Array constructor, the array is initialized with the given elements. The following example demonstrates this behavior:
Download Run Code
To declare and initialize the array with the specified value, we can use the Array constructor with the fill() function. The fill() function updates all element in an array to a given value and returns the modified array.
2. Using an array literal
An array literal is the easiest and most concise way to create an array. We can use square brackets [] to enclose a list of values separated by commas. For example:
3. Using Array.from() function
The Array.from() function creates a new array instance from the specified array and optionally map each array element to a new value using a mapping function. To create an array, we can pass an empty object with the length property defined. To initialize it with some value, map each element to a new value. For example:
4. Using Array.of() function
The Array.of() static function that creates a new array from a variable number of arguments. It works similarly to the Array constructor, but it always creates an array with the given arguments as elements, regardless of their type or number. For example:
That’s all about declaring and initializing arrays in JavaScript. Keep in mind that we can use the Array.map() function to create a new array from an old array, with each item of the old array transformed to a new value. The map() function accepts a callback function as an argument and gives back a new array with the results of applying the function to every array element. For example:
Join items of two arrays into a new array in JavaScript
Determine if two arrays are equal in JavaScript
Find set difference between two arrays in JavaScript
Rate this post
Average rating 4.95 /5. Vote count: 22
No votes so far! Be the first to rate this post.
We are sorry that this post was not useful for you!
Tell us how we can improve this post?
Thanks for reading.
To share your code in the comments, please use our online compiler that supports C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages.
Like us? Refer us to your friends and support our growth. Happy coding :)
Software Engineer | Content Writer | 12+ years experience
JavaScript Arrays: Create, Access, Add & Remove Elements
We have learned that a variable can hold only one value. We cannot assign multiple values to a single variable. JavaScript array is a special type of variable, which can store multiple values using a special syntax.
The following declares an array with five numeric values.
In the above array, numArr is the name of an array variable. Multiple values are assigned to it by separating them using a comma inside square brackets as [10, 20, 30, 40, 50] . Thus, the numArr variable stores five numeric values. The numArr array is created using the literal syntax and it is the preferred way of creating arrays.
Another way of creating arrays is using the Array() constructor, as shown below.
Every value is associated with a numeric index starting with 0. The following figure illustrates how an array stores values.
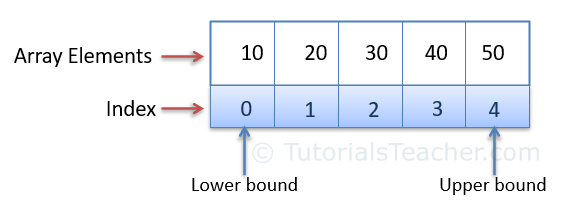
The following are some more examples of arrays that store different types of data.
It is not required to store the same type of values in an array. It can store values of different types as well.
Get Size of an Array
Use the length property to get the total number of elements in an array. It changes as and when you add or remove elements from the array.
Accessing Array Elements
Array elements (values) can be accessed using an index. Specify an index in square brackets with the array name to access the element at a particular index like arrayName[index] . Note that the index of an array starts from zero.
For the new browsers, you can use the arr.at(pos) method to get the element from the specified index. This is the same as arr[index] except that the at() returns an element from the last element if the specified index is negative.
You can iterate an array using Array.forEach() , for, for-of, and for-in loop, as shown below.
Update Array Elements
You can update the elements of an array at a particular index using arrayName[index] = new_value syntax.
Adding New Elements
You can add new elements using arrayName[index] = new_value syntax. Just make sure that the index is greater than the last index. If you specify an existing index then it will update the value.
In the above example, cities[9] = "Pune" adds "Pune" at 9th index and all other non-declared indexes as undefined.
The recommended way of adding elements at the end is using the push() method. It adds an element at the end of an array.
Use the unshift() method to add an element to the beginning of an array.
Remove Array Elements
The pop() method returns the last element and removes it from the array.
The shift() method returns the first element and removes it from the array.
You cannot remove middle elements from an array. You will have to create a new array from an existing array without the element you do not want, as shown below.
Learn about array methods and properties in the next chapter.
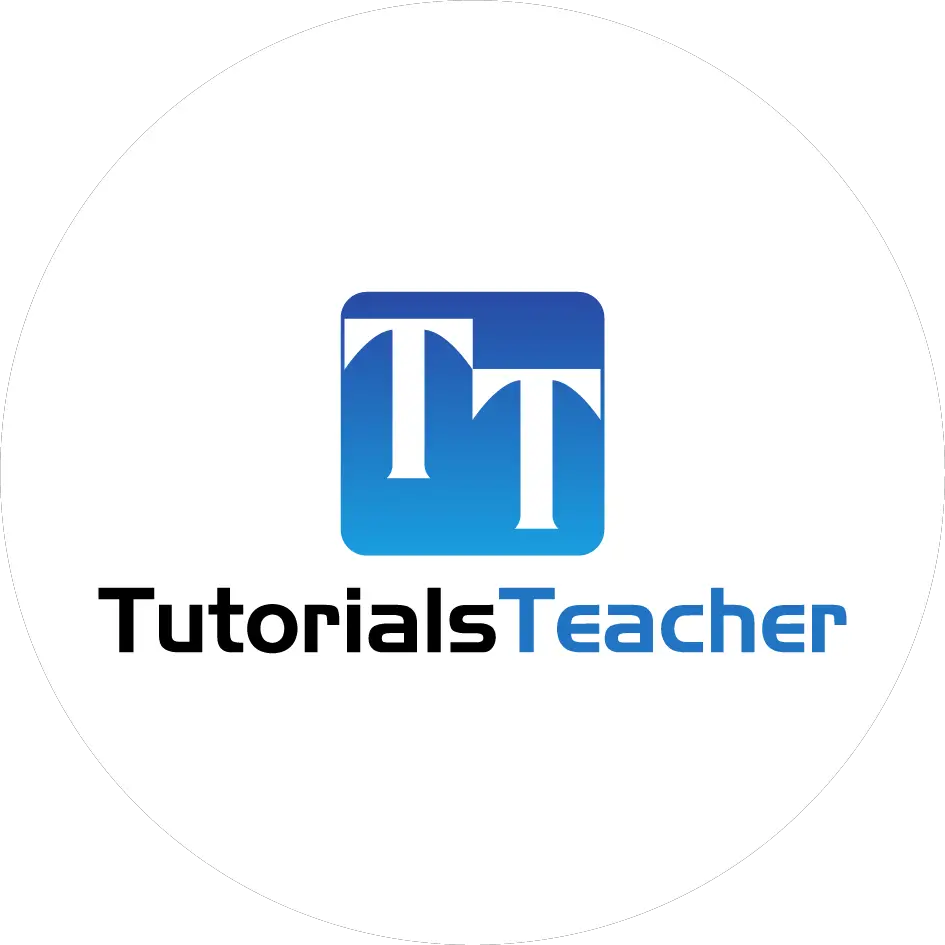
We are a team of passionate developers, educators, and technology enthusiasts who, with their combined expertise and experience, create in -depth, comprehensive, and easy to understand tutorials.We focus on a blend of theoretical explanations and practical examples to encourages hands - on learning. Visit About Us page for more information.
- JavaScript Minifier
- JSON Formatter
- XML Formatter
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Tutorial
JavaScript Basics
- Introduction to JavaScript
- JavaScript Versions
- How to Add JavaScript in HTML Document?
- JavaScript Statements
- JavaScript Syntax
- JavaScript Output
- JavaScript Comments
JS Variables & Datatypes
- Variables and Datatypes in JavaScript
- Global and Local variables in JavaScript
- JavaScript Let
- JavaScript Const
- JavaScript var
JS Operators
- JavaScript Operators
- Operator precedence in JavaScript
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
- JavaScript Bitwise Operators
- JavaScript Ternary Operator
- JavaScript Comma Operator
- JavaScript Unary Operators
- JavaScript Relational operators
- JavaScript String Operators
- JavaScript Loops
- 7 Loops of JavaScript
- JavaScript For Loop
- JavaScript While Loop
- JavaScript for-in Loop
- JavaScript for...of Loop
- JavaScript do...while Loop
JS Perfomance & Debugging
- JavaScript | Performance
- Debugging in JavaScript
- JavaScript Errors Throw and Try to Catch
- Objects in Javascript
- Introduction to Object Oriented Programming in JavaScript
- JavaScript Objects
- Creating objects in JavaScript (4 Different Ways)
- JavaScript JSON Objects
- JavaScript Object Reference
JS Function
- Functions in JavaScript
- How to write a function in JavaScript ?
- JavaScript Function Call
- Different ways of writing functions in JavaScript
- Difference between Methods and Functions in JavaScript
- Explain the Different Function States in JavaScript
- JavaScript Function Complete Reference
JavaScript Arrays
- JavaScript Array Methods
- Best-Known JavaScript Array Methods
- What are the Important Array Methods of JavaScript ?
- JavaScript Array Reference
- JavaScript Strings
- JavaScript String Methods
- JavaScript String Reference
- JavaScript Numbers
- How numbers are stored in JavaScript ?
- How to create a Number object using JavaScript ?
- JavaScript Number Reference
- JavaScript Math Object
- What is the use of Math object in JavaScript ?
- JavaScript Math Reference
- JavaScript Map
- What is JavaScript Map and how to use it ?
- JavaScript Map Reference
- Sets in JavaScript
- How are elements ordered in a Set in JavaScript ?
- How to iterate over Set elements in JavaScript ?
- How to sort a set in JavaScript ?
- JavaScript Set Reference
- JavaScript Date
- JavaScript Promise
- JavaScript BigInt
- JavaScript Boolean
- JavaScript Proxy/Handler
- JavaScript WeakMap
- JavaScript WeakSet
- JavaScript Function Generator
- JavaScript JSON
- Arrow functions in JavaScript
- JavaScript this Keyword
- Strict mode in JavaScript
- Introduction to ES6
- JavaScript Hoisting
- Async and Await in JavaScript
JavaScript Exercises
- JavaScript Exercises, Practice Questions and Solutions
- What is Array in JavaScript?
JavaScript Array is a data structure that allows you to store and organize multiple values within a single variable. It is a versatile and dynamic object. It can hold various data types, including numbers, strings, objects, and even other arrays. Arrays in JavaScript are zero-indexed i.e. the first element is accessed with an index 0, the second element with an index of 1, and so forth.
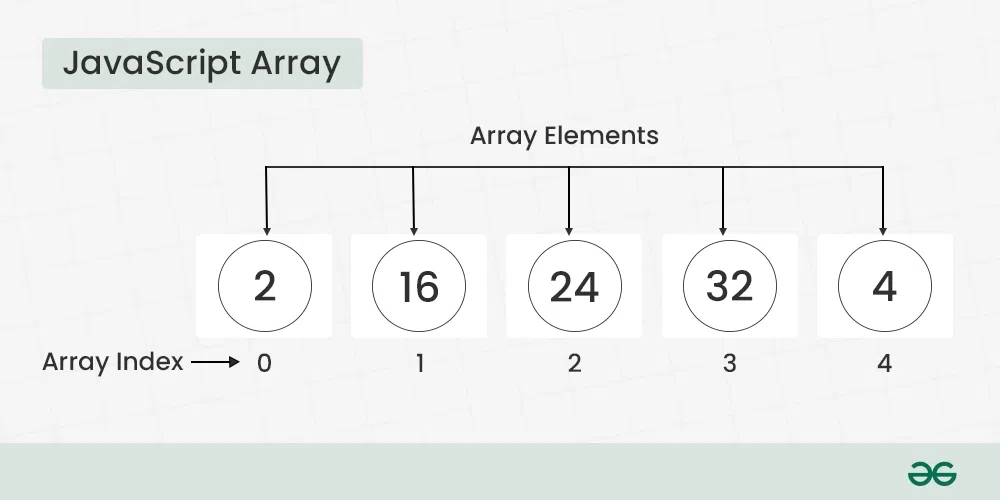
JavaScript Array
You can create JavaScript Arrays using the Array constructor or using the shorthand array literal syntax, which employs square brackets. Arrays can dynamically grow or shrink in size as elements are added or removed.
Table of Content
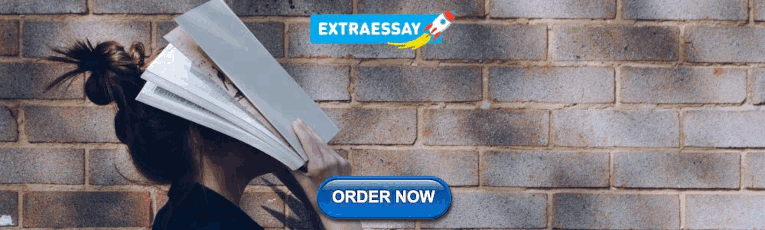
Basic Terminologies of JavaScript Array
Declaration of an array, basic operations on javascript arrays, difference between javascript arrays and objects, when to use javascript arrays and objects, recognizing a javascript array, javascript array complete reference, javascript array examples, javascript cheatsheet.
- Array: A data structure in JavaScript that allows you to store multiple values in a single variable.
- Array Element: Each value within an array is called an element. Elements are accessed by their index.
- Array Index: A numeric representation that indicates the position of an element in the array. JavaScript arrays are zero-indexed, meaning the first element is at index 0.
- Array Length: The number of elements in an array. It can be retrieved using the length property.
There are basically two ways to declare an array i.e. Array Literal and Array Constructor.
1. Creating an Array using Array Literal
Creating an array using array literal involves using square brackets [] to define and initialize the array. This method is concise and widely preferred for its simplicity.
2. Creating an Array using Array Constructor (JavaScript new Keyword)
The “Array Constructor” refers to a method of creating arrays by invoking the Array constructor function. This approach allows for dynamic initialization and can be used to create arrays with a specified length or elements.
Note: Both the above methods do exactly the same. Use the array literal method for efficiency, readability, and speed.
1. Accessing Elements of an Array
Any element in the array can be accessed using the index number. The index in the arrays starts with 0.
2. Accessing the First Element of an Array
The array indexing starts from 0, so we can access first element of array using the index number.
3. Accessing the Last Element of an Array
We can access the last array element using [array.length – 1] index number.
4. Modifying the Array Elements
Elements in an array can be modified by assigning a new value to their corresponding index.
5. Adding Elements to the Array
Elements can be added to the array using methods like push() and unshift().
6. Removing Elements from an Array
Remove elements using methods like pop(), shift(), or splice().
7. Array Length
Get the length of an array using the length property.
8. Increase and Decrease the Array Length
We can increase and decrease the array length using the JavaScript length property.
9. Iterating Through Array Elements
We can iterate array and access array elements using for and forEach loop.
Example: It is the example of for loop.
Example: It is the example of Array.forEach() loop.
10. Array Concatenation
Combine two or more arrays using the concat() method. Ir returns new array conaining joined arrays elements.
11. Conversion of an Array to String
We have a builtin method toString() to converts an array to a string.
12. Check the Type of an Arrays
The JavaScript typeof operator is used ot check the type of an array. It returns “object” for arrays.
- JavaScript arrays use indexes as numbers.
- objects use indexes as names..
- Arrays are used when we want element names to be numeric.
- Objects are used when we want element names to be strings.
There are two methods by which we can recognize a JavaScript array:
- By using Array.isArray() method
- By using instanceof method
Below is an example showing both approaches:
Note: A common error is faced while writing the arrays:
The above two statements are not the same.
Output: This statement creates an array with an element ” [5] “.
We have a complete list of Javascript Array, to check those please go through this JavaScript Array Reference article. It contains detailed description and examples of all Array Properties and Methods.
JavaScript Array examples contains list of questions that are majorily asked in interview. Please check this article JavaScript Array Examples for more detail.
We have a Cheat Sheet on Javascript where we have covered all the important topics of Javascript to check those please go through Javascript Cheat Sheet-A Basic guide to JavaScript .
Please Login to comment...
Similar reads.
- javascript-array
- Web Technologies

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript array const, ecmascript 2015 (es6).
In 2015, JavaScript introduced an important new keyword: const .
It has become a common practice to declare arrays using const :
Cannot be Reassigned
An array declared with const cannot be reassigned:
Arrays are Not Constants
The keyword const is a little misleading.
It does NOT define a constant array. It defines a constant reference to an array.
Because of this, we can still change the elements of a constant array.
Elements Can be Reassigned
You can change the elements of a constant array:
Browser Support
The const keyword is not supported in Internet Explorer 10 or earlier.
The following table defines the first browser versions with full support for the const keyword:
Assigned when Declared
Meaning: An array declared with const must be initialized when it is declared.
Using const without initializing the array is a syntax error:
This will not work:
Arrays declared with var can be initialized at any time.
You can even use the array before it is declared:
This is OK:
Const Block Scope
An array declared with const has Block Scope .
An array declared in a block is not the same as an array declared outside the block:
An array declared with var does not have block scope:
You can learn more about Block Scope in the chapter: JavaScript Scope .
Advertisement
Redeclaring Arrays
Redeclaring an array declared with var is allowed anywhere in a program:
Redeclaring or reassigning an array to const , in the same scope, or in the same block, is not allowed:
Redeclaring or reassigning an existing const array, in the same scope, or in the same block, is not allowed:
Redeclaring an array with const , in another scope, or in another block, is allowed:
Complete Array Reference
For a complete Array reference, go to our:
Complete JavaScript Array Reference .
The reference contains descriptions and examples of all Array properties and methods.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
JavaScript 2D Array – Two Dimensional Arrays in JS

In JavaScript programming, we are all used to creating and working with one-dimensional arrays. These are arrays that contain elements (elements of similar data types or multiple data types).
But it’s also good to know that two-dimensional arrays (2D arrays) exist in JS.
In this article, you will learn what two-dimensional arrays are and how they work in JavaScript. It is quite different from other programming languages because, technically, there is no two-dimensional array in JavaScript.
What is a Two-Dimensional Array?
A two-dimensional array, also known as a 2D array, is a collection of data elements arranged in a grid-like structure with rows and columns. Each element in the array is referred to as a cell and can be accessed by its row and column indices/indexes.
In JavaScript, there is no direct syntax for creating 2D arrays as with other commonly used programming languages like C, C++, and Java.
You can create two-dimensional arrays in JavaScript through jagged arrays — an array of arrays. For example, below is what a jagged array looks like:
But there is a limitation. It is important to note that two-dimensional arrays have a fixed size. This means that once they are created, the number of rows and columns should be fixed. Also, each row should have a similar number of elements (columns).
For example, the array below has three rows and four elements:
The limitation is that with jagged arrays, you don't get to specify a fixed row and column. This means that a jagged array could have m rows, each having different numbers of elements.
Why Use a 2D Array in JavaScript?
At this point, you may ask yourself about the importance of a 2D array, especially if this is your first time reading about 2D arrays.
In JavaScript, we use two-dimensional arrays, also known as matrices, to store and manipulate data in a structured and organized manner.
- They allow for the efficient storage and manipulation of large amounts of data, such as in image or video processing, scientific simulations, and spreadsheet applications.
- Two-dimensional arrays also enable the use of matrix operations, such as matrix multiplication and transposition, which can simplify complex calculations and make code more readable.
- Two-dimensional arrays can represent mathematical matrices in linear algebra and a wide range of data, such as graphs, maps, and tables.
- Two-dimensional arrays are commonly used in applications that involve data tables, image processing, and game development.
How to Access Elements in a JavaScript 2D Array
Before you learn how to create 2D arrays in JavaScript, let’s first learn how to access elements in 2D arrays.
You can access the elements of a two-dimensional array using two indices, one for the row and one for the column. Suppose you have the following two-dimensional array:
The above is a jagged array in which each element holds the student's name, test score, exam score, and grade. You can access specific elements using the row and column index as seen in the syntax below:
To better understand this, let’s convert the two-dimensional array above to a table using console.table(arrayName) .
Note: make sure that you replace arrayName with the name of your 2D array. In my case, it is MathScore .
You will get an output like this, showing the row and column index. Remember that arrays are zero-indexed, meaning items are referenced from 0, not 1.

Please note that the (index) column and row are for the illustration that indicates the indices/indexes of the inner array.
You use two square brackets to access an element of the two-dimensional or multi-dimensional array. The first accesses the rows, while the second accesses the element in the specified row.
How to access the first and last elements of a 2D array
Sometimes you might need to find the first and last elements of a two-dimensional array. You can do this using the first and last index of both the rows and columns.
The first element will always have the row and column index of 0, meaning you will use arrayName[0][0], .
The index of the last element can be tricky, however. For example, in the example above, the first element is ‘John Doe’ while the last is ‘B’:
How to add all elements of a 2D array
In some situations, all the elements of your 2D array can be numbers, so you may need to add them together and arrive at just one digit. You can do this using a nested loop. You will first loop through the rows, then, for each row, you loop through its elements.
How to Manipulate 2D Arrays in JavaScript
You can manipulate 2D arrays just like one-dimensional arrays using general array methods like pop, push, splice and lots more.
Let’s start by learning how to add/insert a new row and element to the 2D array.
How to insert an element into a 2D array
You can add an element or many elements to a 2D array with the push() and unshift() methods.
The push() method adds elements(s) to the end of the 2D array, while the unshift() method adds element(s) to the beginning of the 2D array.
When you console.log() or console.table() the array, you will see that the new rows have been added:
You can also add elements to the inner array, but it’s wrong to push to just one inner array without affecting all the array elements. This is because two-dimensional arrays are meant to have the same number of elements in each element array.
Instead of affecting one array element, you can add elements to all element arrays at once:
This will return:
You can also use the unshift() method to insert the element at the beginning and the splice() method to insert at the middle of the array:
In the above, 1 is the position where you want the new array to be inserted (remember it is zero-indexed), 0 is used so it removes no element, and then the third parameter is the array to be added.
This is the output:
How to remove an element from a 2D array
You can also remove element(s) from the beginning and end of a 2D array with the pop() and shift() methods. This is similar to how the push() and unshift() methods work, but you do not add any parameters to the methods this time.
When you console.log() or console.table() the array, you will see that the first and last array elements have been removed:
You can also remove elements from each array element:
You can also use the shift() method to remove the element at the beginning and the splice() method to remove array elements from specific positions:
In the code above, you remove one item from position index 2 of the MathScore 2D array. This will output:
Note: All JavaScript array methods will operate on a 2D array because it is an array of arrays. You only have to be mindful when adjusting individual elements in the elements array. You make a similar change on all elements by looping through, even though 2D arrays in JavaScript are not strict.
How to Create 2D Arrays in JavaScript
There are two options for creating a multi-dimensional array. You can create the array manually with the array literal notation, which uses square brackets [] to wrap a list of elements separated by commas. You can also use a nested loop.
How to create a 2D array using an array literal
This is just like the examples we have been considering using the following syntax:
For example, below is a 2D array that holds information about each student’s math score and grade:
How to create a 2D array using a nested for loop
There are many approaches to doing this. But generally, you create a nested loop where the first loop will loop through the rows while the second loop will loop through the elements in the inner array (columns):
In the code above, you will first loop through the rows. For each row, it will create an empty array within the original array declared and stored in variable arr . You will then create a nested loop to loop through the columns and add individual elements.
In this example, the index for j is used and will output:
You can decide to create a number, initialize with 0, and then increase as you loop through so you don’t have the same number for all elements:
You can also decide to create a function that accepts these values as arguments:
How to Update Elements in 2D Arrays in JavaScript
This is very similar to how you do it with one-dimensional arrays, where you can update an array’s value by using the index to assign another value.
You can use the same approach to change an entire row or even individual elements:
This will replace the value of index 1 with this new array:
You can update individual elements by tracking the row and column indices and updating their values:
This will change the name on the row with index 2 to “Jack Jim”, as seen below:
Wrapping Up
In this article, you have learned what two-dimensional arrays are and how they work in JavaScript.
It’s important to know that 2D arrays in JavaScript still work very much like one-dimensional arrays, so feel free to tweak and manipulate them with JavaScript methods .
Have fun coding!
You can access over 150 of my articles by visiting my website . You can also use the search field to see if I've written a specific article.
Frontend Developer & Technical Writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
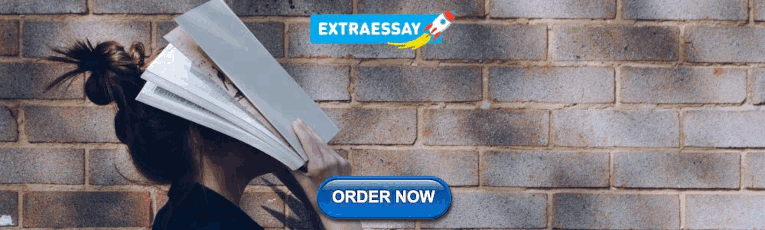
IMAGES
VIDEO
COMMENTS
Creating an Array. Using an array literal is the easiest way to create a JavaScript Array. Syntax: const array_name = [ item1, item2, ... ]; It is a common practice to declare arrays with the const keyword. Learn more about const with arrays in the chapter: JS Array Const.
8. There are a number of ways to create arrays. The traditional way of declaring and initializing an array looks like this: var a = new Array(5); // declare an array "a", of size 5. a = [0, 0, 0, 0, 0]; // initialize each of the array's elements to 0.
Arrays can be created using a constructor with a single number parameter. An array is created with its length property set to that number, and the array elements are empty slots. js. const arrayEmpty = new Array(2); console.log(arrayEmpty.length); // 2. console.log(arrayEmpty[0]); // undefined; actually, it is an empty slot.
Another way to create an array is to use the new keyword with the Array constructor. Here is the basic syntax: new Array(); If a number parameter is passed into the parenthesis, that will set the length for the new array. In this example, we are creating an array with a length of 3 empty slots. new Array(3)
The Difference Between Array() and [] Using Array literal notation if you put a number in the square brackets it will return the number while using new Array() if you pass a number to the constructor, you will get an array of that length.. you call the Array() constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument ...
How to Declare an Array with Literal Notation. This is the most popular and easiest way to create an array. It is a shorter and cleaner way of declaring an array. To declare an array with literal notation you just define a new array using empty brackets. It looks like this: let myArray = []; You will place all elements within the square ...
This example shows three ways to create new array: first using array literal notation, then using the Array() constructor, and finally using String.prototype.split() to build the array from a string. js. // 'fruits' array created using array literal notation. const fruits = ["Apple", "Banana"];
Arrays are carefully tuned inside JavaScript engines to work with contiguous ordered data, please use them this way. ... The call to new Array(number) creates an array with the given length, but without elements. The length property is the array length or, to be precise, its last numeric index plus one. It is auto-adjusted by array methods.
Arrays. In the final article of this module, we'll look at arrays — a neat way of storing a list of data items under a single variable name. Here we look at why this is useful, then explore how to create an array, retrieve, add, and remove items stored in an array, and more besides.
There are several ways to declare and initialize arrays in JavaScript. An array is a collection of values that can be accessed by an index. Here are some of the common functions: 1. Using an Array constructor. We can use the Array() constructor to create an array using the new keyword and the Array function. We can pass one or more values as ...
To declare arrays, we use the let or const keyword — like this: let arr = [1,2,3]; ... Another way to create an array is the new keyword, like this: let names = new Array('Bob','Jane', 'John'); ... To make manipulating arrays easy, the JavaScript's standard library contains lots of array methods that make manipulating arrays easy. There are ...
Approach 1: Using Array Literal. Array literals use square brackets to enclose comma-separated elements for initializing arrays in JavaScript. Syntax: const arrayName = [element1, element2, element3]; Example: This example shows the different types of array declaration. Javascript. // Number array. const numbers = [56, 74, 32, 45]; // String array.
In the above array, numArr is the name of an array variable. Multiple values are assigned to it by separating them using a comma inside square brackets as [10, 20, 30, 40, 50].Thus, the numArr variable stores five numeric values. The numArr array is created using the literal syntax and it is the preferred way of creating arrays.. Another way of creating arrays is using the Array() constructor ...
The "Array Constructor" refers to a method of creating arrays by invoking the Array constructor function. This approach allows for dynamic initialization and can be used to create arrays with a specified length or elements. Syntax: let arrayName = new Array(); Example: javascript. let names = new Array();
Try it Yourself ». The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice() method returns an array with the deleted items:
This said, for your needs you may just want to declare an array of a certain size: var foo = new Array(N); // where N is a positive integer /* this will create an array of size, N, primarily for memory allocation, but does not create any defined values foo.length // size of Array foo[ Math.floor(foo.length/2) ] = 'value' // places value in the ...
In 2015, JavaScript introduced an important new keyword: const. It has become a common practice to declare arrays using const: Example. const cars = ["Saab", "Volvo", "BMW"];
In JavaScript programming, we are all used to creating and working with one-dimensional arrays. These are arrays that contain elements (elements of similar data types or multiple data types). But it's also good to know that two-dimensional arrays (2D arrays) exist in JS. In this article, you will learn what
var sample = new Array(); sample[0] = new Object(); sample[1] = new Object(); This works fine, but I don't want to mention any index number. I want all elements of my array to be an object. How do I declare or initialize it? var sample = new Array(); sample[] = new Object(); I tried the above code but it doesn't work.
13. To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns. function Create2DArray(rows,columns) {. var x = new Array(rows); for (var i = 0; i < rows; i++) {.
When you define a = new Array(), you are defining an empty array. Note that there are no associative objects yet. When you define b = new Array(2), you are defining an array with two undefined locations. In both your examples of 'a' and 'b', you are adding associative properties i.e. objects to these arrays.