How to use window.location in JavaScript
The world of web development brims with tools and functions that enable developers to create seamless user experiences. Today, we delve into one of JavaScript’s essential objects: window.location . This object plays a pivotal role in managing the web page’s address and can be used for tasks such as page redirection, refreshing, and extracting URL information.
If you’re asking yourself, “What is window.location in JavaScript?” don’t worry. We’ve got you covered. Put simply, window.location is a property that gives you access to a Location object, containing information about the current URL and methods to manipulate it. Whether you want to redirect a user to a different page or just retrieve the current URL components, window.location is your go-to object in JavaScript.
In this article, we will walk you through the different aspects of window.location , including how to use it to interact with the web page’s URL. We will also take a look at its various properties and methods, ensuring you have all the information needed to leverage this powerful object in your projects.
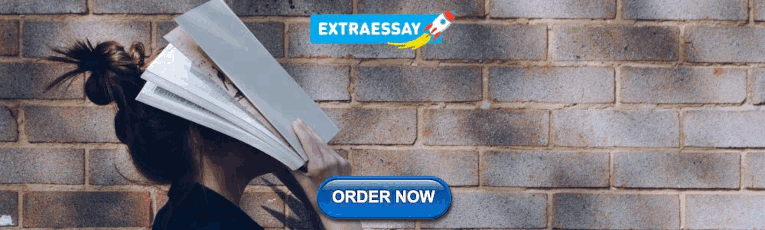
What is window.location?
window.location offers several properties and methods you can use to read and manipulate the current page URL. Whether it’s the protocol, hostname, port, pathname, or query string, window.location provides an easy interface to work with all these components.
For instance, you might want to retrieve the current page’s protocol using window.location.protocol or the hostname via window.location.hostname . These properties offer granular control over the URL, allowing you to tailor the user experience based on the current location or redirect users as needed.
How to Use window.location to Redirect to a New Page
One of the most common uses of window.location is to redirect the user to a different webpage. This can be achieved straightforwardly using:
This line of code instructs the browser to navigate to ' https://www.example.com' ; . But there’s more to redirection than just changing the href property. Let’s explore the other methods like assign() and replace() and when to use them.
Methods Available for window.location
Window.location.assign().
The assign() method is used to navigate to a new URL and is equivalent to setting the window.location.href property:
One unique feature of using assign() is that it keeps the originating page in session history. This means users can press the browser’s back button to return to the original page, which is crucial for ensuring a user-friendly navigation experience.
window.location.replace()
If you intend for a page redirect to be final, with no option for the user to return to the previous page using the back button, the replace() method is what you need:
This method replaces the current resource with the new one in a way that it is not possible to go back.
window.location.reload()
When you want to reload the current page, window.location.reload() comes in handy. By default, this method reloads the page from the browser’s cache, but you can force a server reload by passing true as an argument:
This forces the browser to fetch the latest version of the page from the server.
Accessing URL Components
Beyond redirection, window.location is also incredibly useful for breaking down the URL into readable parts. The properties available include:
- window.location.href – the entire URL
- window.location.protocol – the protocol scheme (e.g., http: or https: )
- window.location.host – the hostname and port number
- window.location.hostname – the domain name of the URL
- window.location.port – the port number if specified
- window.location.pathname – the path or file name after the domain name
- window.location.search – the query portion of the URL, beginning with a ?
- window.location.hash – the fragment identifier, including the #
- window.location.origin – the base URL (protocol plus hostname and port)
Each of these properties can be read and, in some cases, set to update the current URL without reloading the page.
Browser Compatibility and Potential Issues
When working with window.location , it’s crucial to keep in mind browser compatibility. While the object and its methods are widely supported, peculiarities in behavior across different browsers can occur. It is good practice to consult documentation and test across various platforms to ensure consistent functionality.
Conclusion and Call to Action
Understanding and effectively utilizing window.location can significantly enhance the way you interact with URLs in your web applications. By taking advantage of its properties and methods, you can direct users to different pages, refresh content, and dissect URLs to improve user experiences and meet your app’s navigational needs.
Ready to start experimenting with window.location ? Open up your code editor and try implementing some of the methods discussed in this article. Test out redirecting to a new page, parsing the current URL, or simply refreshing the page programmatically. As you become more familiar with window.location , youâll find it an indispensable tool in your JavaScript toolbox.
Related posts
# window.location Cheatsheet
Looking for a site's URL information, then the window.location object is for you! Use its properties to get information on the current page address or use its methods to do some page redirect or refresh đ«
https://www.samanthaming.com/tidbits/?filter=JS#2
- Difference between host vs hostname
- How to change URL properties
- window.location vs location
- window.location.toString
- assign vs replace
- replace vs assign vs href
- Scratch your own itch đ
- Community Input
# window.location Properties
# difference between host vs hostname.
In my above example, you will notice that host and hostname returns the value. So why do these properties. Well, it has do with the port number. Let's take a look.
URL without Port
https://www.samanthaming.com
URL with Port
https://www.samanthaming.com:8080
So host will include the port number, whereas hostname will only return the host name.
# How to change URL properties
Not only can you call these location properties to retrieve the URL information. You can use it to set new properties and change the URL. Let's see what I mean.
Here's the complete list of properties that you can change:
The only property you can't set is window.location.origin . This property is read-only.
# Location Object
The window.location returns a Location object. Which gives you information about the current location of the page. But you can also access the Location object in several ways.
The reason we can do this is because these are global variables in our browser.
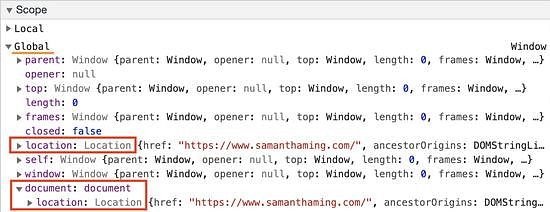
# window.location vs location
All 4 of these properties point at the same Location object. I personally prefer window.location and would actually avoid using location . Mainly because location reads more like a generic term and someone might accidentally name their variable that, which would override the global variable. Take for example:
I think that most developer is aware that window is a global variable. So you're less likely to cause confusion. To be honest, I had no idea location was a global variable until I wrote this post đ . So my recommendation is to be more explicit and use window.location instead đ
Here's my personal order of preference:
Of course, this is just my preference. You're the expert of your codebase, there is no best way, the best way is always the one that works best for you and your team đ€
# window.location Methods
# window.location.tostring.
This method returns the USVString of the URL. It is a read-only version of Location.href
In other words, you can use it to get the href value from the
on this đ. But I did find a performance test on the difference.
JSPerf: Location toString vs Location href
One thing I want to note about these speed tests is that it is browser specific. Different browser and versions will render different outcome. I'm using Chrome, so the href came out faster then the rest. So that's one I'll use. Also I think it reads more explicit then toString() . It is very obvious that href will provide the URL whereas toString seems like something it being converted to a string đ
# assign vs replace
Both of these methods will help you redirect or navigate to another URL. The difference is assign will save your current page in history, so your user can use the "back" button to navigate to it. Whereas with replace method, it doesn't save it. Confused? No problem, I was too. Let's walk through an example.
Current Page
I just need to emphasize the "current page" in the definition. It is the page right before you call assign or replace .
# How to Do a Page Redirect
By now, you know we can change the properties of the window.location by assigning a value using = . Similarly, there are methods we can access to do some actions. So in regards to "how to redirect to another page", well there are 3 ways.
# replace vs assign vs href
All three does redirect, the difference has to do with browser history. href and assign are the same here. It will save your current page in history, whereas replace won't. So if you prefer creating an experience where the navigation can't press back to the originating page, then use replace đ
So the question now is href vs assign . I guess this will come to personal preference. I like the assign better because it's a method so it feels like I'm performing some action. Also there's an added bonus of it being easier to test. I've been writing a lot of Jest tests, so by using a method, it makes it way easier to mock.
But for that that are rooting for href to do a page redirect. I found a performance test and running in my version of Chrome, it was faster. Again performance test ranges with browser and different versions, it may be faster now, but perhaps in future browsers, the places might be swapped.
JSPerf: href vs assign
# Scratch your own itch đ
Okay, a bit of a tangent and give you a glimpse of how this cheatsheet came to be. I was googling how to redirect to another page and encountered the window.location object. Sometimes I feel a developer is a journalist or detectiveâ-âthere's a lot of digging and combing through multiple sources for you to gather all the information available. Honestly, I was overwhelmed with the materials out there, they all covered different pieces, but I just wanted a single source. I couldn't find much, so I thought, I'll cover this in a tidbit cheatsheet! Scratch your own itch I always say đ
# Community Input
: This is awesome, Iâve used window.location.href in the past, but didnât realise how simple it is to access sections of the URL!
If you want to see a live-action of what James is talking about, check out the table of content at the top of this article. Click on it and it will scroll down to the specific section of the page.
# Resources
- Share to Twitter Twitter
- Share to Facebook Facebook
- Share to LinkedIn LinkedIn
- Share to Reddit Reddit
- Share to Hacker News Hacker News
- Email Email
Related Tidbits
Console.table to display data, colorful console message, window.location cheatsheet, fresh tidbits.
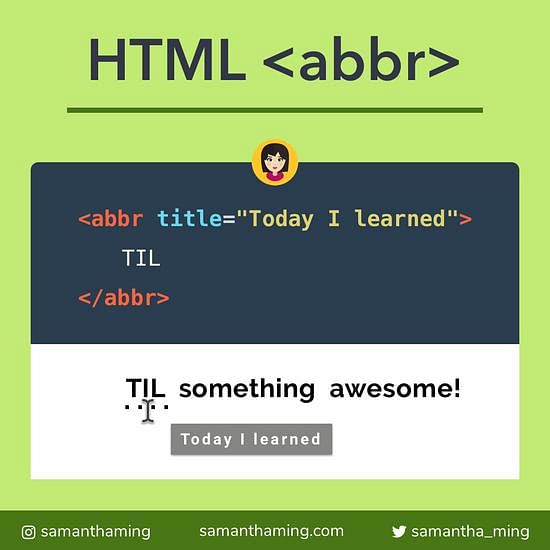
HTML abbr Tag
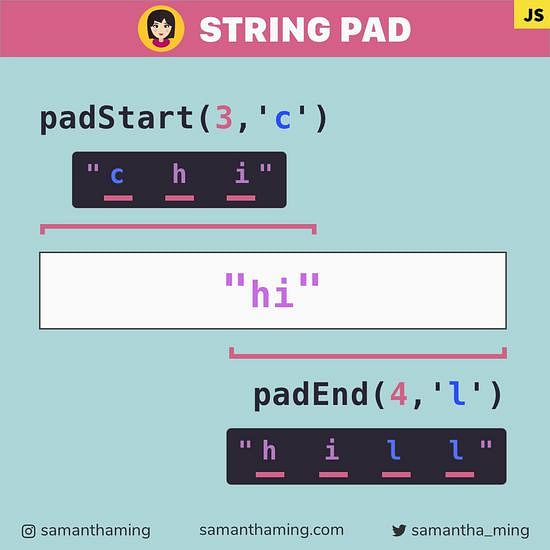
How to Pad a String with padStart and padEnd in JavaScript
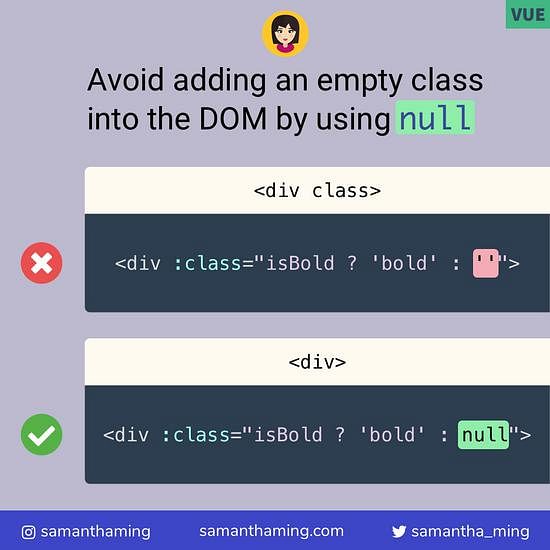
Avoid Empty Class in Vue with Null
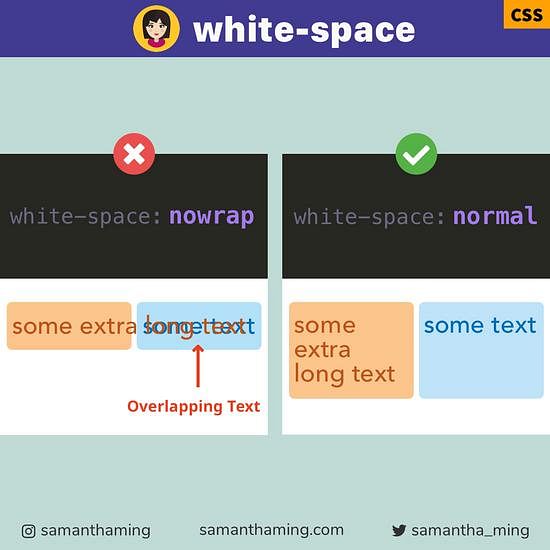
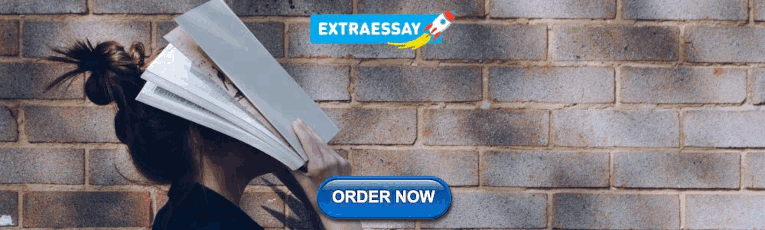
Fix Text Overlap with CSS white-space
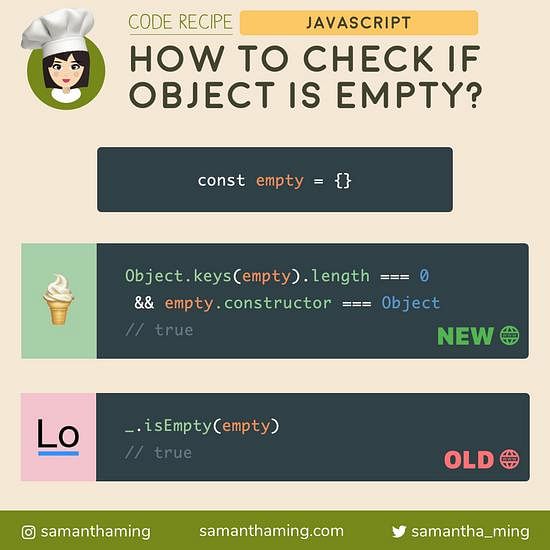
How to Check if Object is Empty in JavaScript
JavaScript Window Location Explained â Complete Guide self.__wrap_b=(t,n,e)=>{e=e||document.querySelector(`[data-br="${t}"]`);let a=e.parentElement,r=R=>e.style.maxWidth=R+"px";e.style.maxWidth="";let o=a.clientWidth,c=a.clientHeight,i=o/2-.25,l=o+.5,u;if(o){for(;i+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(a):process.env.NODE_ENV==="development"&&console.warn("The browser you are using does not support the ResizeObserver API. Please consider add polyfill for this API to avoid potential layout shifts or upgrade your browser. Read more: https://github.com/shuding/react-wrap-balancer#browser-support-information"))};self.__wrap_b(":R4mr36:",1)
Understanding the window.location Object
Using window.location in practice.

JavaScript is an essential programming language for web development, and one of its most powerful features is the ability to manipulate the browserâs window object. In this blog post, weâll dive into the window.location object and examine how it can be used to navigate, manipulate, and gather information about the browserâs current URL. By the end of this post, youâll have a complete understanding of the window.location object and its methods, properties, and use cases.
The window.location object is a part of the window object and provides information about the current documentâs URL. It also allows you to manipulate and navigate the browserâs history. The window.location object has several properties and methods that can be used to access and modify the current URL.
Properties of window.location
Letâs take a look at the properties of the window.location object and what information they provide:
- window.location.href : The full URL of the current document, including the protocol, domain, port, path, and query string. For example, if the current URL is https://codedamn.com/blog/javascript-window-location , the value of window.location.href would be the same.
- window.location.protocol : The protocol used by the current URL, such as http: or https: .
- window.location.host : The full domain and port of the current URL, such as codedamn.com:80 (where 80 is the default port number for HTTP).
- window.location.hostname : The domain name of the current URL, such as codedamn.com .
- window.location.port : The port number used by the current URL. If no port number is specified, this property will be an empty string.
- window.location.pathname : The path of the current URL, such as /blog/javascript-window-location .
- window.location.search : The query string of the current URL, including the leading question mark (?), such as ?id=123&name=John .
- window.location.hash : The URL fragment identifier, including the leading hash symbol (#), such as #section-1 .
Methods of window.location
The window.location object also provides several methods to manipulate and navigate the browserâs history:
- window.location.assign(url) : Loads a new document at the specified URL.
- window.location.replace(url) : Replaces the current document with a new one at the specified URL without adding an entry to the browserâs history.
- window.location.reload(forcedReload) : Reloads the current document. If the forcedReload parameter is set to true , the browser will bypass the cache and request the document from the server.
Now that weâve explored the properties and methods of the window.location object, letâs see how they can be used in practice.
Navigating to a New Page
To navigate to a new page, you can use the window.location.assign() method or simply set the window.location.href property to the desired URL. For example:
// Using window.location.href window . location . href = 'https://codedamn.com/learn/javascript' ;
Both of these methods have the same effect: they load the specified URL in the current browser window.
Reloading the Current Page
To reload the current page, you can use the window.location.reload() method:
// Force a reload, bypassing the browser cache window . location . reload ( true ) ;
Replacing the Current Page
If you want to navigate to a new page without adding an entry to the browserâs history, you can use the window.location.replace() method:
This method is useful when you want to prevent users from using the back button to return to the previous page.
Working with Query Strings
To access and manipulate the query string of the current URL, you can use the window.location.search property. For example, you can retrieve the query string and parse its key-value pairs:
// Parse the query string into an object const queryParams = new URLSearchParams ( queryString ) ;
// Access the values of the query parameters const id = queryParams . get ( 'id' ) ; // '123' const name = queryParams . get ( 'name' ) ; // 'John'
Q: Can I use window.location to navigate to a different domain?
A: Yes, you can use window.location.assign() or set window.location.href to a URL from a different domain. However, due to the same-origin policy, you wonât be able to access or manipulate the content of the other domainâs pages.
Q: How can I get only the domain and protocol of the current URL?
A: You can concatenate the window.location.protocol and window.location.hostname properties to get the full domain and protocol of the current URL, like this:
Q: How can I change only the hash part of the current URL?
A: You can set the window.location.hash property to the desired value:
This will update the URLâs hash without reloading the page.
Q: What is the difference between window.location.assign() and window.location.replace()?
A: The window.location.assign() method loads a new URL and adds it to the browserâs history, allowing the user to navigate back to the previous page using the back button. The window.location.replace() method loads a new URL and replaces the current page in the browserâs history, preventing the user from navigating back to the previous page.
We hope this guide has provided you with a comprehensive understanding of the JavaScript window.location object and its various properties and methods. As you continue to develop your JavaScript skills and work on web projects, youâll find that window.location is an essential tool for managing and navigating browser URLs.
Sharing is caring
Did you like what Mehul Mohan wrote? Thank them for their work by sharing it on social media.
No comment s so far
Curious about this topic? Continue your journey with these coding courses:
146 students learning
DINESH KUMAR REDDY
JavaScript from Zero to Hero with 5+ projects
106 students learning
Shubham Sarda
Mastering Advanced JavaScript
Robert Nana Sarpong
Getting Started With JavaScript

4 Ways to Use JavaScript to Redirect or Navigate to A URL or Refresh the Page
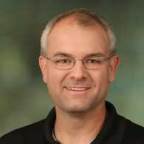
Last Updated - Wed Jan 20 2021
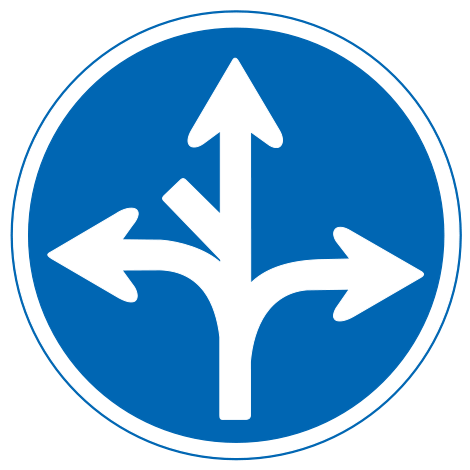
Many URLs are redirected to new locations, typically using a server configuration and HTTP header properties. But there are many scenarios where you may need to use JavaScript to redirect or navigate to another URL.
There are many reasons why you might want to redirect to a new page or URL.
- You changed your domain or URL structure
- You wan to redirect based on language, location or viewport
- The user has submitted a form and you want to direct them to the next page in the sequence
- The page may require authorization
- Redirect from HTTP to HTTPS
- You need to trigger a new page in a single page application
The window.location object manages the address loaded by the browser. Manipulating the location object is how you manage page redirects using JavaScript. The location object has properties and methods to trigger a JavaScript page redirect.
The location object properties you need to familiarize yourself with are:
- hash : used for single page apps and single page websites
- host : the URL's domain
- hostname : similar to host
- href: the full URL
- origin : the URL's protocol and domain
- pathname : the URL slug or page after the origin
- port : if a port is included in the URL
- protocol: http, https, ftp, ftps, etc
Each of these properties are strings and support all the standard string methods like replace, which we will use in this tutorial.
The location object methods are:
- assign: sets the location object to the new URL
- reload : forces the page to reload using the same URL
- replace: triggers a redirect, which we will explore in depth
- search : allows you to interrogate the queryString
Assign and replace look similar, but have a different constraints. The assign method loads the resource at the new URL and preserves the previous entry in the browser's navigation history.
This means the user can hit the back button and go to the original page.
The replace method does the same thing, except the original or 'current' resource is not retained in the browser's history.
This means the user cannot hit the back button to go to the original page.
Both may fail if there are security restrictions. For example, third party scripts cannot trigger a redirect. This is a good thing because so many sites use third party scripts without verifying their code.
Redirect to a New Address
Use javascript to refresh a page, redirect on a form submission, javascript redirect in a single page app (spa).
When you have changed an address, for example a new domain name, you should perform a 301 redirect. This is where the server sends an HTTP status code of 301, with the new address.
A 301 status code tells the user agent: i.e. the browser or search engine spider, there is a new address. This is sort of like when you move and submit an address change to the post office. Your mail is forwarded.
A 301 redirect allows you to keep the traffic and hopefully your search engine ranking.
Normally you would do this from the server. There are times when this is not possible and you would need to use a JavaScript redirect to a URL.
This is pretty simple, just include one of the following snippets:
I would recommend using replace because the original URL is not valid. So retaining it in their session history might confuse them and trigger an automatic redirect anyway.
location.replace is useful for any 301 redirect scenario, including when you need to redirect from HTTP to HTTPS. But a more succinct way to redirect from HTTP to HTTPS using JavaScript is the following code snippet:
I do recommend configuring your server to send the proper HTTP redirection headers because they are much more efficient. This applies to both new addresses and HTTP to HTTPS.
There are times when you will want to programatically refresh or reload a page using JavaScript. Typically you would place a button or action element on the page for the user to initiate the refresh. Automatically performing a page refresh can be a confusing and jarring experience for your user, so minimize that tactic.
The location.reload method reloads the current document, just like the user pressed F5 or the browser reload button.
The default setting reloads the page from browser cache, if it is available. You can force the method to bypass local cache and retrieve the document from the network by passing true to the method.
I think the best etiquette is to use JavaScript to refresh the page in response to a user action. This is typically in response to a click or touch event handler. I also think in this scenario you are also intending to retrieve from the network, so you will also want to force this action.
Here is an over simplified example:
You should be aware, if the user has scroll the page the browser may not retain their current scroll position. Most modern browsers seem to handle this well, but this is not always the case.
You are refreshing a page, and the content and document structure has most likely changed from what their originally rendered. In these cases the browser may not be able to determine where to reliably place the scroll position. In these cases the browser does not scroll the page and the positioning is the top.
There is also a security check in place with this call to prevent external scripts from using this method maliciously. This relates to the Same origin Policy. If this happens a DOMException is thrown with a SECURITY_ERROR.
Another time where using the location.replace method is useful is submitting forms.
This is not the right choice 100% of the time, but should be a consideration. A good example is checking out of a shopping cart.
Once the user clicks the button to submit payment it is typically not a good idea for them to go back to the cart. This can cause all sorts of confusion and potential duplicate orders.
In response to the cart or any form being submitted you need to add a click or pointer event handler to intercept the action. In the handler method you should include a return false to prevent the form from being submitted to the server.
You would do this normally when you create a client-side form handler, so don't forget this when you are adding JavaScript code to redirect to a new another page.
Just a helpful note to this process: You may want to include a queryString parameter in your URL to pass along a reference to the user's state. This might help you render or hydrate the new target URL.
A core principle of Single Page Web Applications (SPA) is things that used to live on the server now live in the browser. One of those responsibilities is managing redirections. For example when you make an AJAX call to a secure service and without a valid authorization token. The service should return a 401 status code. When receiving a 401 status code the application should redirect the user to the login address.
In classic web development the AJAX request would have been a page request or post back. The server recognizes the unauthorized state and performs the proper redirect server-side. In ASP.NET we do this with the response.redirect function.
In a SPA the client application's code needs to manage the redirect. I have encountered two common scenarios; redirecting to an external party or (secure token server) STS or to the application's login view. The internal redirection is fairly easy, change the hash fragment's route to the login view.
If you are familiar with single page applications you will recognize this as how to use JavaScript to redirect to another page.
Remember when redirecting to a new page due to stale or missing authentication token you are most likely using active authentication and need to persist the authentication token somewhere, like IndexedDB.
If you are using passive authentication the page will post back to the authentication server and the token returned, typically in the header.
Redirecting to an STS normally involves changing the browser's address from the SPA URL to the authentication URL. The STS URL is most likely on a completely different domain, server, etc.
Whatever the case may be the location must change. Most developers', me included, first reaction was to look for a location.redirect method.
It does not exist.
To change the browser's address you should use the location.href property. Changing this value triggers the browser to load the new URL.
Now the browser loads the new target. In this scenario the user then logins using the STS and return to the application authenticated.
Authentication is one scenario you might find yourself needing to programmatically redirecting the user. Of course there are many other scenarios where you might need to redirect a user either internally or externally in the course of normal application usage.
There are different reasons why you might need to redirect from one page to another. Try to configure these on the server as much as possible. But there are many common scenarios where you need to use JavaScript to perform a page redirect.
The window.location object has properties and methods you can manipulate using JavaScript to redirect to different URLs. The location.replace and location.assign can be very helpful. The replace method keeps the session history clean, and the assign method allows the user to back track through the URL history.

We use cookies to give you the best experience possible. By continuing, we'll assume you're cool with our cookie policy.
Install Love2Dev for quick, easy access from your homescreen or start menu.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Difference between window.location.href, window.location.replace and window.location.assign in JavaScript
- JavaScript | window.location and document.location Objects
- Differences between undeclared and undefined variables in JavaScript
- Explain the difference between undefined and not defined in JavaScript
- How to set location and location.href using JavaScript ?
- Difference between window.onkeypress and window.document.body.onkeypress
- How to replace a portion of strings with another value in JavaScript ?
- Difference between addEventListener and onclick in JavaScript
- How are the JavaScript window and JavaScript document different from one another?
- Difference between Function.prototype.apply and Function.prototype.call
- Difference between window.onload and body.onload
- What's the difference between JavaScript and JScript?
- What happen when we directly assign the variable without declaring it in JavaScript ?
- How to close window using JavaScript which is opened by the user with a URL ?
- What is the difference between anchor href vs angular routerlink in Angular ?
- What is the difference between call and apply in JavaScript ?
- Differences between Document and Window Objects
- How to replace an HTML element with another one using JavaScript ?
- Difference between Node.js and JavaScript
- How to remove hash from window.location with JavaScript without page refresh ?
Window.location is a property that returns a Location object with information about the document’s current location. This Location object represents the location (URL) of the object it is linked to i.e. holds all the information about the current document location (host, href, port, protocol, etc.)
All three commands are used to redirect the page to another page/website but differ in terms of their impact on the browser history.
window.location.href Property :
- The href property on the location object stores the URL of the current webpage.
- On changing the href property, a user can navigate to a new URL, i.e. go to a new webpage.
- It adds an item to the history list (so that when the user clicks the “Back” button, he/she can return to the current page).
- Updating the href property is considered to be faster than using the assign() function (as calling a function is slower than accessing the property).
Example: This example shows the use of the window.location.href property.
Note: The following 2 lines of code perform the same purpose.
window.location.replace Property :
- The replace function is used to navigate to a new URL without adding a new record to the history.
- As the name suggests, this function “replaces” the topmost entry from the history stack, i.e., removes the topmost entry from the history list, by overwriting it with a new entry.
- So, when the user clicks the “Back” button, he/she will not be able to return to the current page.
- Hence, the major difference between the assign() and replace() methods is that the replace() function will delete the current page from the session history.
- The replace function does not wipe out the entire page history, nor does it make the “Back” button non-functional.
Example: This example shows the use of the window.location.replace property.
window.location.assign Property :
- The assign function is similar to the href property as it is also used to navigate to a new URL.
- The assign method, however, does not show the current location, it is only used to go to a new location.
- Unlike the replace method, the assign method adds a new record to history (so that when the user clicks the “Back” button, he/she can return to the current page).
- However, rather than updating the href property, calling a function is considered safer and more readable.
- The assign() method is also preferred over href as it allows the user to mock the function and check the URL input parameters while testing.
Example: This example shows the use of the window.location.assign property.
Difference between window.location.replace, window.location.assign and window.location.href properties:
Please Login to comment...
Similar reads.
- JavaScript-Properties
- JavaScript-Questions
- Web Technologies - Difference Between
- Difference Between
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Location: replace() method
The replace() method of the Location interface replaces the current resource with the one at the provided URL. The difference from the assign() method is that after using replace() the current page will not be saved in session History , meaning the user won't be able to use the back button to navigate to it.
A string containing the URL of the page to navigate to.
Thrown if the provided url parameter is not a valid URL.
Return value
None ( undefined ).
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- The Location interface it belongs to.
- Similar methods: Location.assign() and Location.reload() .
JS Reference
Html events, html objects, other references, window location, the window location object.
The location object contains information about the current URL.
The location object is a property of the window object .
The location object is accessed with:
window.location or just location
Location Object Properties
Location object methods.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
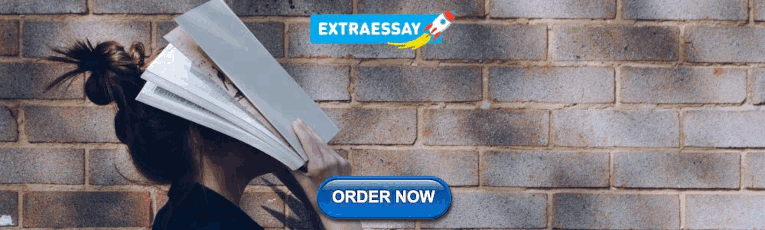
IMAGES
VIDEO
COMMENTS
26. I am setting up authentication for an app. After I make a post request to login, a JSON Web Token is sent in response. I am able to attach this to the header via Ajax. The problem is when using window.location.pathname to redirect after login, since it is not an Ajax request it does not have the token attached to the header.
The assign() method of the Location interface causes the window to load and display the document at the URL specified. After the navigation occurs, the user can navigate back to the page that called Location.assign() by pressing the "back" button.
Window: location property. The Window.location read-only property returns a Location object with information about the current location of the document. Though Window.location is a read-only Location object, you can also assign a string to it. This means that you can work with location as if it were a string in most cases: location = 'http ...
The window.location object can be written without the window prefix. Some examples: window.location.href returns the href (URL) of the current page. window.location.hostname returns the domain name of the web host. window.location.pathname returns the path and filename of the current page. window.location.protocol returns the web protocol used ...
See Also: The replace() Method. Note. The difference between assign() and replace(): replace() removes the current URL from the document history. With replace() it is not possible to use "back" to navigate back to the original document.
One of the most common uses of window.location is to redirect the user to a different webpage. This can be achieved straightforwardly using: window.location.href = 'https://www.example.com'; This line of code instructs the browser to navigate to ' https://www.example.com';. But there's more to redirection than just changing the href property.
Location.ancestorOrigins Read only . A static DOMStringList containing, in reverse order, the origins of all ancestor browsing contexts of the document associated with the given Location object.. Location.href. A stringifier that returns a string containing the entire URL. If changed, the associated document navigates to the new page. It can be set from a different origin than the associated ...
#replace vs assign vs href. All three does redirect, the difference has to do with browser history. href and assign are the same here. It will save your current page in history, whereas replace won't. So if you prefer creating an experience where the navigation can't press back to the originating page, then use replace đ. So the question now is href vs assign.
The window.location object is a part of the window object and provides information about the current document's URL. It also allows you to manipulate and navigate the browser's history. The window.location object has several properties and methods that can be used to access and modify the current URL. Properties of window.location.
Normally you would do this from the server. There are times when this is not possible and you would need to use a JavaScript redirect to a URL. This is pretty simple, just include one of the following snippets: window.location.assign("new target URL"); //or. window.location.replace("new target URL");
window.location.assign Property:. The assign function is similar to the href property as it is also used to navigate to a new URL.; The assign method, however, does not show the current location, it is only used to go to a new location. Unlike the replace method, the assign method adds a new record to history (so that when the user clicks the "Back" button, he/she can return to the current ...
The window.location.port property returns the port through which a web page is served to a user. Web browsers don't usually show the default port in the address bar for common web protocols, but window.location.port can access them. Sometimes, depending on a server configuration, window.location.port will return an empty string if the default ...
The href property of the Location interface is a stringifier that returns a string containing the whole URL, and allows the href to be updated.. Setting the value of href navigates to the provided URL. If you want redirection, use location.replace().The difference from setting the href property value is that when using the location.replace() method, after navigating to the given URL, the ...
Add Header to window.location.pathname. 5. How should I make sure the user accessing a backend rendered frontend route is authenticated? 4. Create an HTTP request, give it a header and open url in a new tab. 3. Add headers to a link in angular. 1. Jwt token authentication for direct URL hit in browser. 1.
Location: replace () method. The replace() method of the Location interface replaces the current resource with the one at the provided URL. The difference from the assign() method is that after using replace() the current page will not be saved in session History , meaning the user won't be able to use the back button to navigate to it.
The Window Location Object. The location object contains information about the current URL. The location object is a property of the window object. The location object is accessed with: window.location or just location.
The Window.location read-only property produces a Position object that contains information about the document's current location. There is only one value for using the Location HTTP Header. The value for using the Location HTTP Header is the URL. An example of a Location HTTP Header is given below.
I'm trying to use the window.location.assign to change my project page, but it's not working. The page I want to change is in the same folder. My Code: filterFunc (){ window.location.assig...
window.location.href = 'https://stackoverflow.com'; Whether to use PHP or JS to manage the redirection depends on what your code is doing and how. But if you're in a position to use PHP; that is, if you're going to be using PHP to send some JS code back to the browser that simply tells the browser to go somewhere else, then logic suggests that ...