JS Reference
Html events, html objects, other references, html dom document write().
Write some text directly to the HTML output:
Write some HTML elements directly to the HTML output:
Using document.write() after a document is loaded, deletes all existing HTML:
More examples below.
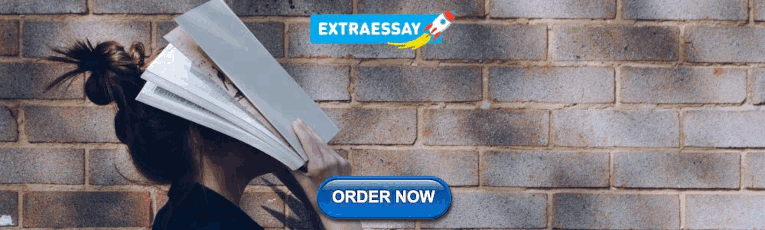
Description
The write() method writes directly to an open (HTML) document stream.
The write() method deletes all existing HTML when used on a loaded document.
The write() method cannot be used in XHTML or XML.
The write() method is most often used to write to output streams opened by the the open() method.
The Document open() Method
The Document close() Method
The Document writeln() Method
Return Value
More examples.
Write a date object directly to the HTML ouput:
Open an output stream, add some HTML, then close the output stream:
Open a new window and write some HTML into it:
The Difference Between write() and writeln()
It makes no sense to use writeln() in HTML.
It is only useful when writing to text documents (type=".txt").
Newline characters are ignored in HTML.
If you want new lines in HTML, you must use paragraphs or <br>:
Browser Support
document.write is supported in all browsers:

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- English (US)
Document: write() method
Warning: Use of the document.write() method is strongly discouraged.
As the HTML spec itself warns :
This method has very idiosyncratic behavior. In some cases, this method can affect the state of the HTML parser while the parser is running, resulting in a DOM that does not correspond to the source of the document (e.g. if the string written is the string " <plaintext> " or " <!-- "). In other cases, the call can clear the current page first, as if document.open() had been called. In yet more cases, the method is simply ignored, or throws an exception. Users agents are explicitly allowed to avoid executing script elements inserted via this method . And to make matters even worse, the exact behavior of this method can in some cases be dependent on network latency, which can lead to failures that are very hard to debug. For all these reasons, use of this method is strongly discouraged. Therefore, avoid using document.write() — and if possible, update any existing code that is still using it.
The document.write() method writes a string of text to a document stream opened by document.open() .
Note: Because document.write() writes to the document stream , calling document.write() on a closed (loaded) document automatically calls document.open() , which will clear the document .
A string containing the text to be written to the document.
Return value
None ( undefined ).
The text you write is parsed into the document's structure model. In the example above, the h1 element becomes a node in the document.
Writing to a document that has already loaded without calling document.open() will automatically call document.open() . After writing, call document.close() to tell the browser to finish loading the page.
If the document.write() call is embedded within an inline HTML <script> tag, then it will not call document.open() . For example:
Note: document.write() and document.writeln do not work in XHTML documents (you'll get an "Operation is not supported" [ NS_ERROR_DOM_NOT_SUPPORTED_ERR ] error in the error console). This happens when opening a local file with the .xhtml file extension or for any document served with an application/xhtml+xml MIME type . More information is available in the W3C XHTML FAQ .
Note: Using document.write() in deferred or asynchronous scripts will be ignored and you'll get a message like "A call to document.write() from an asynchronously-loaded external script was ignored" in the error console.
Note: In Edge only, calling document.write() more than once in an <iframe> causes the error "SCRIPT70: Permission denied".
Note: Starting with version 55, Chrome will not execute <script> elements injected via document.write() when specific conditions are met. For more information, refer to Intervening against document.write() .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- element.innerHTML
- document.createElement()
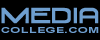
- Photography
JavaScript "document.write"
One of the most basic JavaScript commands is document.write . This simply prints the specified text to the page. To print text literally, enter the text in single quote marks inside parentheses like so:
The code above will cause the phrase "Hello World!" to appear on the page.
You can also use document.write to print variables. Enter the variable name without quotes, like so:
Note that if quote marks are placed around the variable name, the variable name itself will be printed (instead of the variable value). You can also combine variable values and text strings by using the + sign:
Notice the way the variable names are used literally as well as for passing the values. This will print the following:
Remember, text inside quotes will be printed literally, text with no quotes is assumed to be a variable.
How to display JavaScript variable value in HTML
by Nathan Sebhastian
Posted on Mar 28, 2021
Reading time: 3 minutes
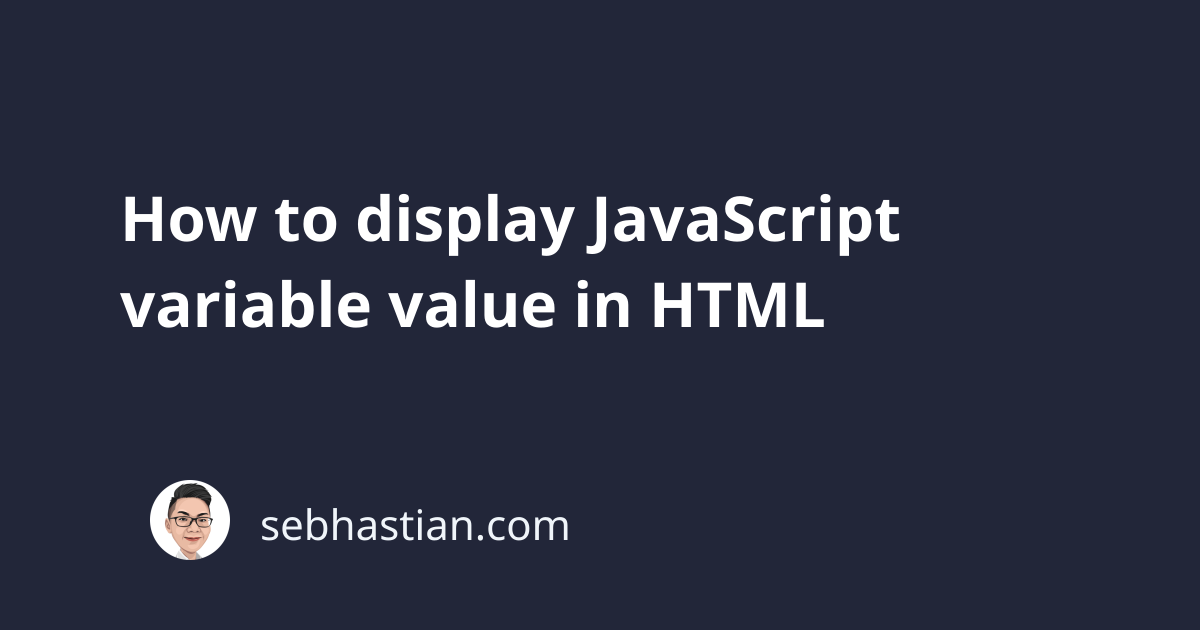
There are three ways to display JavaScript variable values in HTML pages:
- Display the variable using document.write() method
- Display the variable to an HTML element content using innerHTML property
- Display the variable using the window.alert() method
This tutorial will show you how to use all three ways to display JavaScript variables in HTML pages. Let’s start with using document.write() method.
Display JavaScript variable using document.write() method
The document.write() method allows you to replace the entire content of HTML <body> tag with HTML and JavaScript expressions that you want to be displayed inside the <body> tag. Suppose you have the following HTML element:
When you run document.write("Hello") method on the HTML piece above, the content of <body> will be replaced as follows:
Knowing this, you can display any JavaScript variable value by simply passing the variable name as the parameter to document.write() method:
The document.write() method is commonly used only for testing purposes because it will delete any existing HTML elements inside your <body> tag. Mostly, you would want to display a JavaScript variable beside your HTML elements. To do that, you need to use the next method.
Display JavaScript variable using innerHTML property.
Every single HTML element has the innerHTML property which holds the content of that element. The browser allows you to manipulate the innerHTML property by using JavaScript by simply assigning the property to a different value.
For example, imagine you have the following HTML <body> tag:
You can replace the content of <p> tag by first retrieving the element using its identifier. Since the element <p> has an id attribute with the value of greeting , you can use document.getElementById method to retrieve it and change its innerHTML property.
Here’s how you do it:
The content of <p> tag will be changed as follows:
Knowing this, you can simply wrap the space where you want your JavaScript variable to be displayed with a <span> element as follows:
The code above will output the following HTML:
And that’s how you can display JavaScript variable values using innerHTML property.
Display JavaScript variable using window.alert() method
The window.alert() method allows you to launch a dialog box at the front of your HTML page. For example, when you try running the following HTML page:
The following dialog box should appear in your browser:

The implementation for each browser will slightly vary, but they all work the same. Knowing this, you can easily use the dialog box to display the value of a JavaScript variable. Simply pass the variable name to the alert() method as follows:
The code above will launch a dialog box that displays the value of the name variable.
Displaying JavaScript variables in HTML pages is a common task for web developers. Modern browsers allow you to manipulate the HTML content by calling on exposed JavaScript API methods.
The most common way to display the value of a JavaScript variable is by manipulating the innerHTML property value, but when testing your variables, you can also use either document.write() or window.alert() methods. You are free to use the method that suits you best.
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:

JavaScript Document write: Examples, Syntax, and Benefits
One of the fundamental functions in Javascript is the document.write method , which enables developers to generate content dynamically and manipulate the DOM (Document Object Model) in real time.
This article will explore the depths of javascript document write, exploring its examples and application as well as its benefits.
What is JavaScript Document Write?
JavaScript’s document.write() function is used to dynamically generate and insert content into an HTML document while it is being parsed and loaded by a web browser.
It allows you to add text, HTML tags, or JavaScript code directly into the document.
When document.write() is called, the specified content is written to the document at the location where the script tag is placed.
This means that if document.write() is called after the document has finished loading, it will overwrite the entire content of the document, effectively replacing the existing content.
The parameter of the document.write() function is the content that you want to write to the document. It can be a string literal or a variable that holds a string value.
Return Value
The document.write() function does not have a return value. It is a void function, which means it does not return any specific value.
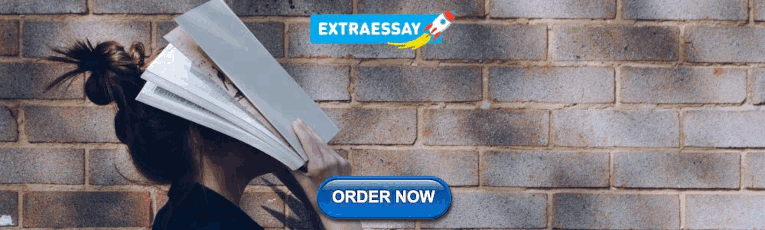
Example Programs of JavaScript document write
Example 1: Writing Text
This example shows how to use document.write() to write plain text to the document.
Example 2: Writing HTML Tags
In this example, document.write() is used to write HTML tags to the document.
Example 3: Writing JavaScript Code
document.write() can also be used to write JavaScript code for the document. In this example, a simple script that displays an alert is written using document.write().
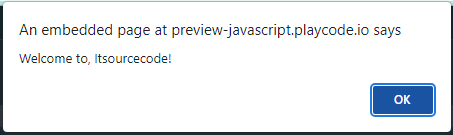
It’s important to note that these examples illustrate the usage of document.write(), but it is not typically recommended to use document.write() extensively in modern web development. Alternative approaches like manipulating the DOM directly or using frameworks and libraries provide more flexibility and control over the content of the document.
Benefits of using document.write in JavaScript
Using document.write offers several advantages when it comes to web development.
Let’s explore some of the key benefits:
1. Dynamic Content Generation
document.write empowers developers to generate content dynamically based on certain conditions or events.
This dynamic approach enables the creation of interactive and personalized web experiences for users.
2. Easy DOM Manipulation
With document.write , developers can effortlessly manipulate the DOM.
It allows the insertion of content at specific positions within the HTML document, making it possible to modify or extend the page’s structure and appearance dynamically.
3. Seamless Integration
The document.write method seamlessly integrates with other Javascript functions and frameworks.
It can be combined with event handlers, conditional statements, and loops to achieve complex interactions and behaviors on a webpage.
4. Quick Prototyping
document.write serves as a valuable tool for rapid prototyping.
It allows developers to quickly test and visualize ideas without the need for a complex setup or external libraries.
Alternative Approach for JavaScript document write
DOM Manipulation :
Instead of directly writing content to the document, you can create and modify elements in the Document Object Model (DOM).
For example, you can use methods like createElement, appendChild, or innerHTML to dynamically add or modify content on your web page.
innerHTML :
The innerHTML property allows you to set the HTML content of an element. You can use it to replace or add HTML content to an existing element.
textContent :
If you want to set or change only the text content of an element, you can use the textContent property.
These alternatives provide more flexibility and control over the content manipulation in the DOM compared to document.write.
Anyway here are some of the functions you might want to learn and can help you:
- Toarray JavaScript: Simplifying Array Manipulation
- JavaScript AssertEquals Explained: Ensuring Equality in Testing
Browsers Supports JavaScript document.write()
Most modern web browsers support JavaScript’s document.write() method.
Here are some popular web browsers that support document.write():
- Google Chrome
- Mozilla Firefox
- Apple Safari
- Microsoft Edge
It’s important to note that the usage of document.write() is generally discouraged in modern web development.
In conclusion, the document.write method in Javascript serves as a valuable tool for dynamic content generation and DOM manipulation. It offers developers the ability to create engaging and interactive web experiences.
By following best practices and understanding its capabilities, document.write can be harnessed effectively to enhance web development projects.
Leave a Comment Cancel reply
You must be logged in to post a comment.
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar
Actual Wizard
Great Content From Actual Wizards
How to use document.write() in JavaScript
November 17, 2020 by Kevin Marszalek
The document.write() function is commonly used when testing simple web applications or when learning JavaScript.
document.write() will print the contents of the parameter passed to it to the current node at execution time.
Here is a simple example:
Here is a more complex example that will demonstrate a major quirk of the document.write() method.
If the DOM tree has already been built, then calling the document.write() method will result in the entire body node of the DOM tree being replaced with whatever parameter is passed to it.
Note: Using document.write() in a production environment is frowned upon as the timing of the execution of the function call will determine its behavior. This makes the code much less useful than techniques that would interact with the DOM or use appendChild().
Alternatives to document.write()
The most commonly used alternative is console.log(), which will print the value of the parameter passed to it to the browser’s console, which can be accessed by pressing F12.
Another alternative is to use appendChild().
This technique is more complex but adequate for production code, as the output text can be controlled in a consistent manner.
Advanced Example:
document.appendchild() Demo
Text After the Script
Pressing This Button Will Output Text in a Predictable Way
Read More From Actual Wizard
- Understanding the Different Parts of an Email Address An email address has four parts; the recipient name, the @ symbol, the domain name, and the top-level domain. …
- How to Get the Last Element of an Array in JavaScript There are a number of different ways to get the last element of an array in JavaScript. Below we will explore …
- How to Open a New Tab with JavaScript Opening a new browser window in a new tab can be done easily in JavaScript. Modern web browsers provide a …
- The Difference Between a Franchisor and a Franchisee Explained The franchised business system has two sides that play different roles in the business. The franchisor …
JavaScript Document.write()
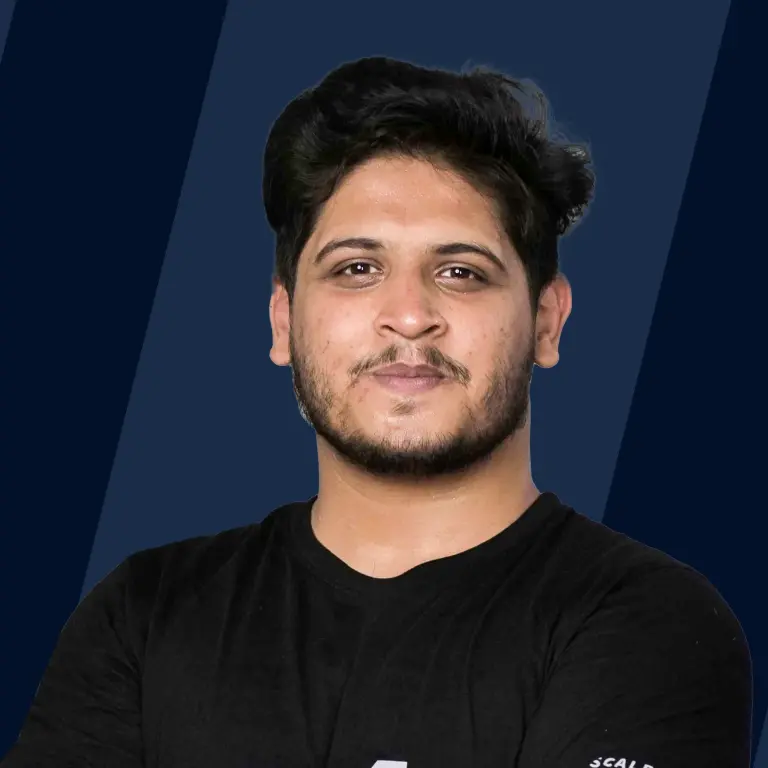
document.write in JavaScript is a function that is used to display some strings in the output of HTML web pages (Browser window). We know that in JavaScript, a name followed by parentheses "( )" is known as a method or a function . So, document.write in javascript is a method in JavaScript to display text in the browser window. Also for testing purposes, it is convenient to use document.write() function. When document.write() function is used after the HTML is fully loaded, it will delete all the contents of an existing HTML document and will show only contents that are inside the document.write() function.
Syntax of JavaScript Document.write()
Syntax of document.write in javascript is as follows:
Parameters of JavaScript Document.write()
By using document.write() method, we can pass an expression called as 'single argument' or can pass multiple values separated by commas "," known as 'multiple arguments'. These values can be displayed in sequential order as they are written in the method of document.write in javascript.
Return Value of JavaScript Document. write()
The return value of the document. write in the javascript in the is undefined . This variable has not been assigned any value.
Exceptions of JavaScript Document.write()
There are some exceptions when ere document.write in javascript method does not work. Let us see them one by one.
- document .write() in JavaScript does not work when your document is of 'XHTML' format. You will get an unsupported error like "Operation is not supported".
- If the document. write() in JavaScript method is used in a script where multiple codes are executed parallelly then the method will get ignored by the console. An error will be like this- "A call to document.write() from an asynchronously-loaded external script was ignored."
- If the internet is much slower, and a document. write in javascript tries to inject some content into the main document, it may cause too long to appear in the main page component. It is also possible that the web page will fail to load.
- After version 55 , Chrome is restricted to executing script elements if it is inserted via the document.write() method.
Let'sseee some examples for a better understanding.
Example 1: We can write a string into a web page.
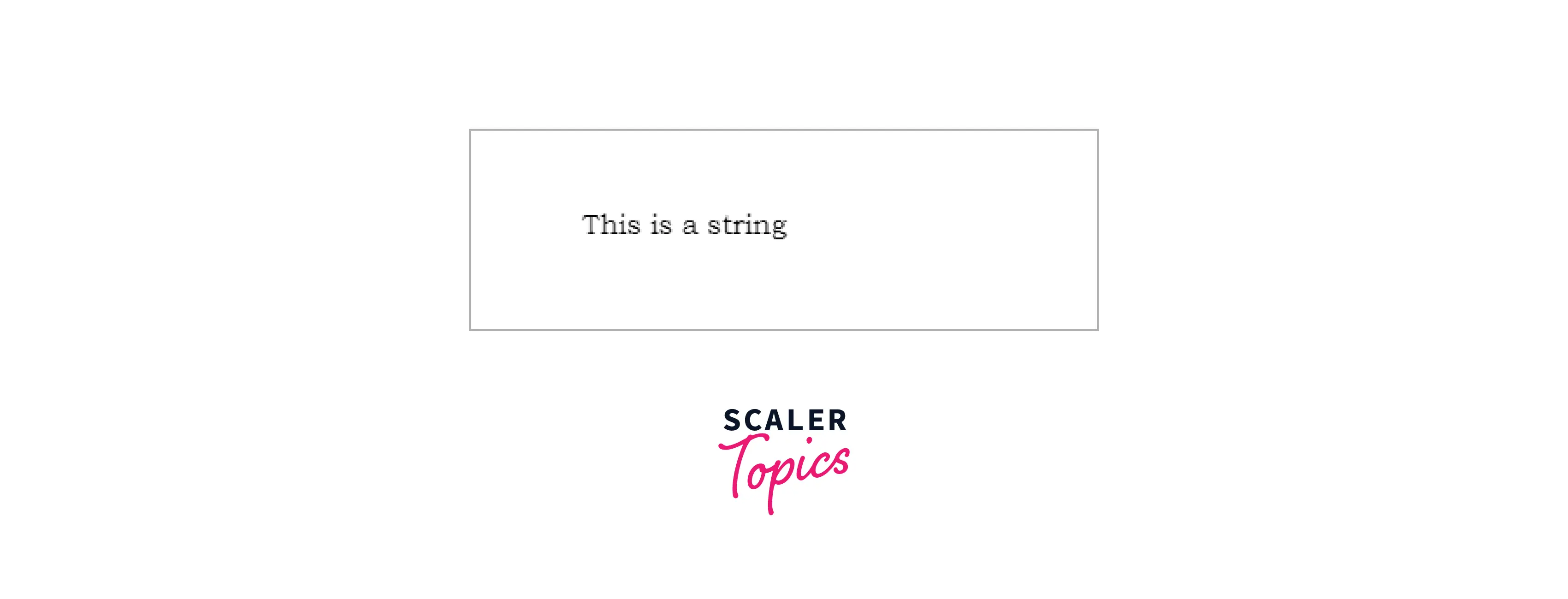
Example 2: We can also writHTMLml content and display them on a web page.
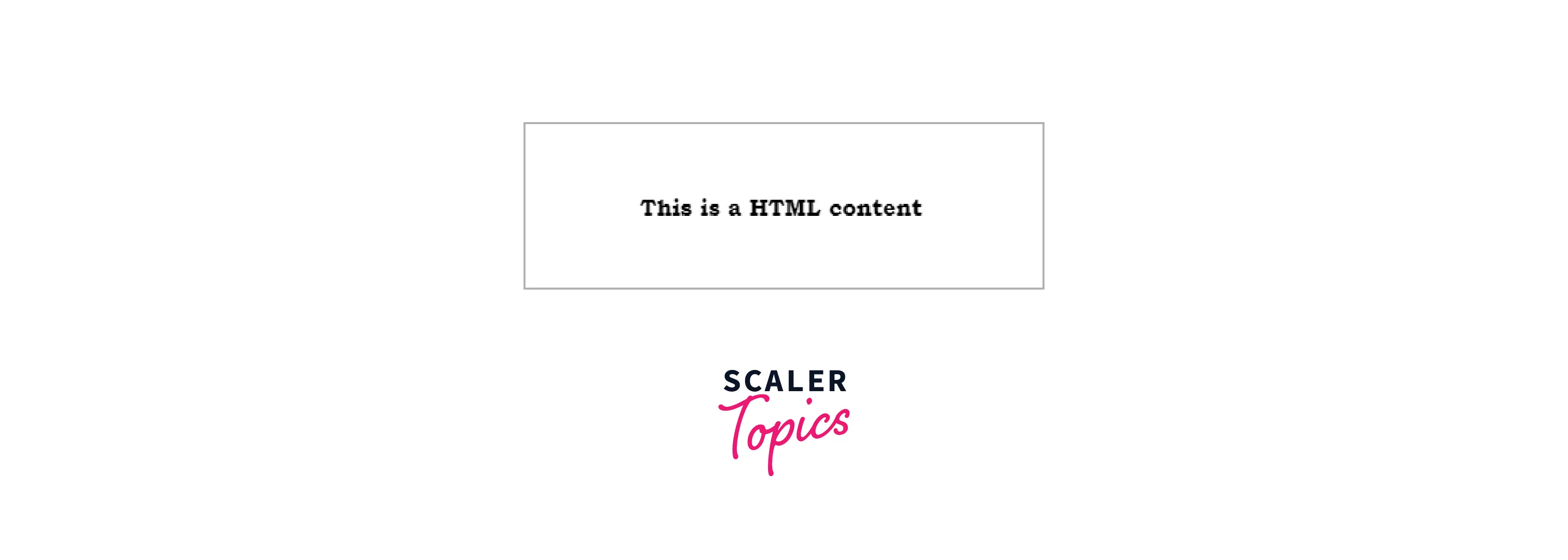
When the document.write() will execute, all the previous content of the web page will be erased and only content inside the method will be displayed on the web page.
How does JavaScript Document.write() Work?
The document.write() method aims to display some particular content in the browser window. This method is written inside the "script" tag. When this method is called, it clears all the previous content and prints the contents inside the method. There are some rules for executing the expressions inside this method which are as follows:
- () parentheses are evaluated from innermost to outermost.
- + operators are evaluated from left to right.
- ',' separators are evaluated from left to right.
More Examples
document.write() in JavaScript method can be used to display various types of strings, values, and operations on a web page. Multiple arguments can be passed using the document.write() method to display various types of content. Let us understand them using examples of each.
1. To display a simple string:
2. We can concatenate strings and display them as a single string:
Here we used the '+' operator and added '10' after the content inside the double quotation.
3. We can do athematic operations and display them in our result.
- Explanation : Inside the method, first we wrote the text that we need to display i.e.- "sum of 5+5" inside a double quotation. then we used the '+' operator to add the strings after that. But after '+', we need to do an operation that is an addition and display the result of that. So we expression that needs to be evaluated, we will again use parenthesis in which we will write the expression as shown in the example.
4. We can print variables in the output of web pages.
Explanation : Here, we created a variable "text1" and called it in document.write() method to print the text inside variable 'text1'.
5. We can also define multiple variables and concatenate them to print in the result using document.write() in JavaScript.
Explanation : In the above example, we defined two variables named 'text1' and 'text2' in which variables are stored. Then, we concatenated the two variables inside the method and printed them in the output.
6 . We can also print the date and time of the current time zone using this method.
Supported Browsers
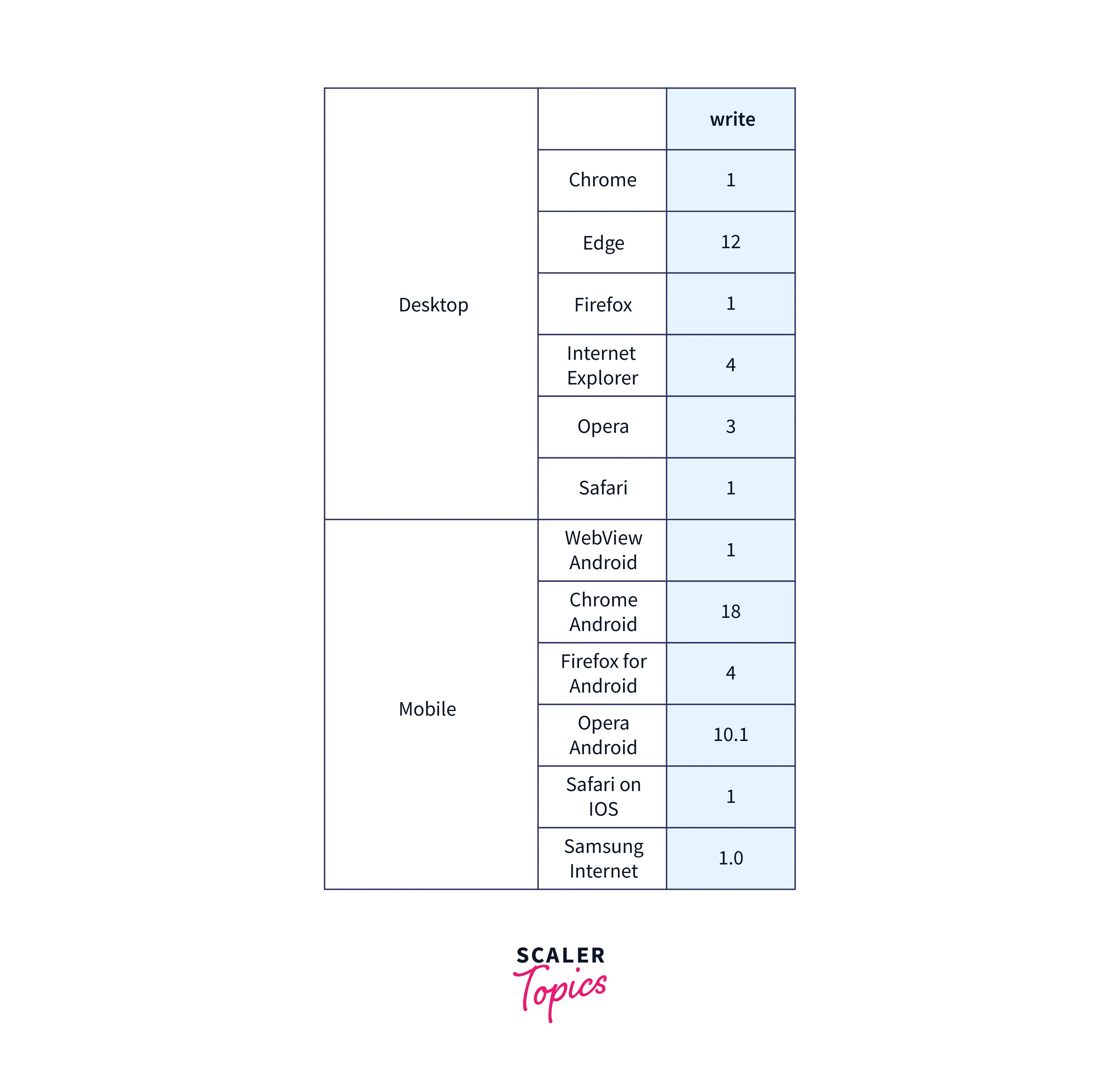
Conclusion:
- document.write() method in JavaScript is used to display some content on the web page.
- This method ends with parentheses.
- different strings, variables, and dates can be printed using the document.write() method.
- This method does not work in XHTML or XML.
- This method clears all the previous content if used after loading the existing page.
- document.write() method in JavaScript is supported in Chrome, IE, Firefox, Safari, and Opera browsers.
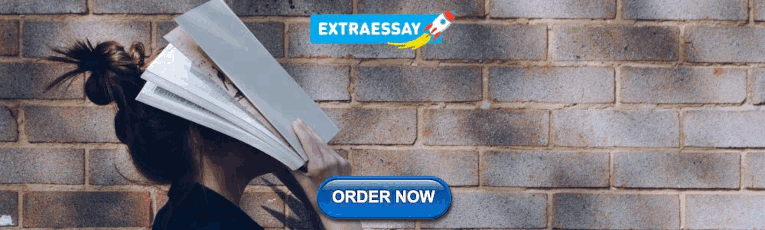
IMAGES
VIDEO
COMMENTS
How to use document.write in javascript to output variables in html? This question has been asked and answered by many developers on Stack Overflow, the largest online community for programmers. Learn from their examples and solutions, or post your own query if you need more help.
JavaScript can "display" data in different ways: Writing into an HTML element, using innerHTML. Writing into the HTML output using document.write(). Writing into an alert box, using window.alert(). Writing into the browser console, using console.log().
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
Warning: Use of the document.write() method is strongly discouraged. As the HTML spec itself warns:. This method has very idiosyncratic behavior. In some cases, this method can affect the state of the HTML parser while the parser is running, resulting in a DOM that does not correspond to the source of the document (e.g. if the string written is the string "<plaintext>" or "<!--
Enter the variable name without quotes, like so: var mytext = "Hello again"; document.write(mytext); Note that if quote marks are placed around the variable name, the variable name itself will be printed (instead of the variable value). You can also combine variable values and text strings by using the + sign:
Storing document.write in a variable not working in JavaScript. 1. javascript document.write variables. 0. How can I echo the value being used in document.write in ...
by Nathan Sebhastian. Posted on Mar 28, 2021. Reading time: 3 minutes. There are three ways to display JavaScript variable values in HTML pages: Display the variable using document.write() method. Display the variable to an HTML element content using innerHTML property. Display the variable using the window.alert() method.
document.write(content); Parameter. The parameter of the document.write() function is the content that you want to write to the document. It can be a string literal or a variable that holds a string value. Return Value. The document.write() function does not have a return value. It is a void function, which means it does not return any specific ...
document.write () will print the contents of the parameter passed to it to the current node at execution time. Here is a simple example: <script>document.write("Some Text")</script>. Here is a more complex example that will demonstrate a major quirk of the document.write () method. If the DOM tree has already been built, then calling the ...
document.write () method in JavaScript is used to display some content on the web page. This method ends with parentheses. different strings, variables, and dates can be printed using the document.write () method. This method does not work in XHTML or XML.