Octave Programming Tutorial/Vectors and matrices
- 1 Creating vectors and matrices
- 2.1 Element operations
- 4.1.1 Other matrices
- 4.2 Changing matrices
- 5 Linear algebra
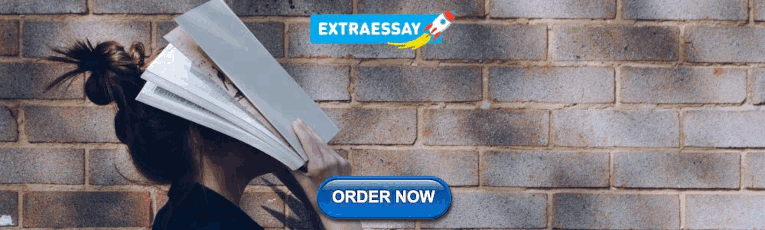
Creating vectors and matrices [ edit | edit source ]
Here is how we specify a row vector in Octave:
- the vector is enclosed in square brackets;
- each entry is separated by an optional comma. x = [1 3 2] results in the same row vector.
To specify a column vector, we simply replace the commas with semicolons:
From this you can see that we use a comma to go to the next column of a vector (or matrix) and a semicolon to go to the next row. So, to specify a matrix, type in the rows (separating each entry with a comma) and use a semicolon to go to the next row.
Operators [ edit | edit source ]
You can use the standard operators to
- subtract ( - ), and
- multiply ( * )
matrices, vectors and scalars with one another. Note that the matrices need to have matching dimensions (inner dimensions in the case of multiplication) for these operators to work.
- The transpose operator is the single quote: ' . To continue from the example in the previous section,
(Note: this is actually the complex conjugate transpose operator, but for real matrices this is the same as the transpose. To compute the transpose of a complex matrix, use the dot transpose ( .' ) operator.)
- The power operator ( ^ ) can also be used to compute real powers of square matrices.
Element operations [ edit | edit source ]
When you have two matrices of the same size, you can perform element by element operations on them. For example, the following divides each element of A by the corresponding element in B :
Note that you use the dot divide ( ./ ) operator to perform element by element division. There are similar operators for multiplication ( .* ) and exponentiation ( .^ ).
Let's introduce a scalar for future use.
The dot divide operators can also be used together with scalars in the following manner.
returns a matrix, C where each entry is defined by
i.e. a is divided by each entry in B . Similarly
return a matrix with
Indexing [ edit | edit source ]
You can work with parts of matrices and vectors by indexing into them. You use a vector of integers to tell Octave which elements of a vector or matrix to use. For example, we create a vector
Now, to see the second element of x , type
You can also view a list of elements as follows.
This last command displays the 1st, 3rd and 4th elements of the vector x .
To select rows and columns from a matrix, we use the same principle. Let's define a matrix
and select the 1st and 3rd rows and 2nd and 3rd columns:
The colon operator ( : ) can be used to select all rows or columns from a matrix. So, to select all the elements from the 2nd row, type
You can also use : like this to select all Matrix elements:
Ranges [ edit | edit source ]
We can also select a range of rows or columns from a matrix. We specify a range with
You can actually type ranges at the Octave prompt to see what the results are. For example,
The first number displayed was start , the second was start + step , the third, start + (2 * step) . And the last number was less than or equal to stop .
Often, you simply want the step size to be 1. In this case, you can leave out the step parameter and type
Finally, there is a keyword called end that can be used when indexing into a matrix or vector. It refers to the last element in the row or column. For example, to see the last column in a Matrix, you can use
Functions [ edit | edit source ]
The following functions can be used to create and manipulate matrices.
Creating matrices [ edit | edit source ]
- tril(A) returns the lower triangular part of A .
- triu(A) returns the upper triangular part of A .
- diag(x) or diag(A) . For a vector, x , this returns a square matrix with the elements of x on the diagonal and 0s everywhere else. For a matrix, A , this returns a vector containing the diagonal elements of A . For example,
- linspace(a, b, n) returns a vector with n values, such that the first element equals a , the last element equals b and the difference between consecutive elements is constant. The last argument, n , is optional with default value 100.
Other matrices [ edit | edit source ]
There are some more functions for creating special matrices. These are
- hankel ( Hankel matrix ),
- hilb ( Hilbert matrix ),
- invhilb (Inverse of a Hilbert matrix),
- sylvester_matrix ( Sylvester matrix ) - In v3.8.1 there is a warning: sylvester_matrix is obsolete and will be removed from a future version of Octave; please use hadamard(2^k) instead,
- toeplitz ( Toeplitz matrix ),
- vander ( Vandermonde matrix ).
Use help to find out more about how to use these functions.
Changing matrices [ edit | edit source ]
- fliplr(A) returns a copy of matrix A with the order of the columns reversed, for example,
- flipud(A) returns a copy of matrix A with the order of the rows reversed, for example,
- sort(x) returns a copy of the vector x with the elements sorted in increasing order.
Linear algebra [ edit | edit source ]
For a description of more operators and functions that can be used to manipulate vectors and matrices, find eigenvalues, etc., see the Linear algebra section.
Return to the Octave Programming Tutorial index
- Book:Octave Programming Tutorial
Navigation menu
Introduction to Octave: Arrays: Vectors and Matrices
1.1 creating, 1.2 indexing, 1.3 describing, 1.4 iterating over, 1.5 concatenating, 2.1 creating, 2.2 indexing, 2.3 describing, 2.4 iterating over, 2.5 finding, 3.1 scalar mathematics, 3.2 element-wise multiplication, 3.3 dot products, 3.4 inverse.
Vectors are 1-dimensional arrays of numbers (as opposed to scalars which are 0-dimensional and matrices which are 2-dimensional).
Vectors are created by using:
- Square brackets
- Commas or spaces between numbers (or a mixture of both)
When working with vectors the output can get quite large so we will use format compact to reduce the space used:
Vectors can also be created by specifying ranges of numbers:
The linspace() function can be used to create a vector of a defined length between a start and an end point with a consistent gap between successive elements:
Read values in a vector by using round brackets:
Use length() and size() to display details about your vector:
This tells us that our vector is 5 elements in length, with 1 row and 5 columns.
You can iterate over a vector using a for loop:
Add a vector onto the end of another vector using square brackets:
Matrices are much the same as vectors except they have two dimensions.
Just like vectors, matrices are created by using square brackets together with either commas or spaces (or both) except, this time, either semi-colons or line breaks are also used as these indicate the line breaks at the end of rows:
A matrix is also indexed using round brackets, but this time two numbers are needed (corresponding to the row and column of the element you want):
More advanced indexing is also possible, using vectors to define the rows and columns you want:
This has given us the odd-numbered rows (1 and 3) and the even-numbered (2 and 4) columns.
A colon : represents ‘all’ rows or columns:
This has given us the odd-numbered rows (1 and 3) and all columns.
The length() , size() , rows() and columns() functions all work for describing a matrix:
You can iterate over a matrix using two for loops:
To find a particular value in a matrix, use == to find which elements are equal to what you are looking for:
Then, use find() to get the indices of these locations (index numbers start at the top-left and increase going down the columns first, then along the rows):
So the number 3 is the second, third and sixth element of this matrix, counting from the top-left downwards and then to the right.
If you search for a two-element vector in a two-column matrix, Octave will search each column separately:
The first column was searched for the number 3 and it was found at indices 2 and 3. The second column was searched for the number 8 and it was found at index 8.
Alternatively, you can search only a subset of the matrix:
All rows and the first column of the matrix was searched for the number 3, and it was found at indices 2 and 3.
3 Array Operations
Addition, subtraction, multiplication and division of an array by a scalar simply applies the operation to all the elements in the array:
If you want to perform an operation between every element in one array and the corresponding elements in a second array, you can add a full stop before the operation:
This causes each element in A to be multiplied by the corresponding element in B . This is not the same as matrix multiplication (dot products and/or cross products). This element-wise mode of operation also applies to division ( ./ ) and exponentiation ( .^ ).
Given an \(r \times n\) matrix A and an \(n \times c\) matrix B the dot product will produce an \(r \times c\) matrix:
A \(3 \times 2\) matrix times a \(2 \times 3\) matrix produces a \(3 \times 3\) matrix.
The inverse of A is inv(A) or A^-1 :
Use this to solve for unknown variables in a system on linear equations: Ax = b
Using Octave
First, follow the installation instructions for:
- GNU/Linux and other Unix systems
- Microsoft Windows
or consult the GNU Octave manual to install GNU Octave on your system. Then, start the GNU Octave by clicking the icon in the programs menu or launch the interactive prompt by typing octave in a terminal. See the manual page on running Octave .
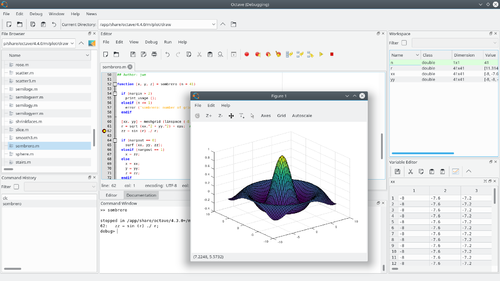
- 1 Variable Assignment
- 3 Command evaluation
- 4 Elementary math
- 6 Linear Algebra
- 7 Accessing Elements
- 8 Control flow with loops
- 9 Vectorization
- 10 Plotting
- 13 Getting Help
- 14 Octave packages
- 15 Octave User Codes
Variable Assignment [ edit ]
Assign values to variables with = (Note: assignment is pass-by-value ). Read more about variables .
Comments [ edit ]
# or % start a comment line, that continues to the end of the line. Read more about comments .
Command evaluation [ edit ]
The output of every command is printed to the console unless terminated with a semicolon ; . The disp command can be used to print output anywhere. Use exit or quit to quit the console. Read more about command evaluation .
Elementary math [ edit ]
Many mathematical operators are available in addition to the standard arithmetic. Operations are floating-point. Read more about elementary math .
Matrices [ edit ]
Arrays in Octave are called matrices. One-dimensional matrices are referred to as vectors. Use a space or a comma , to separate elements in a row and semicolon ; to start a new row. Read more about matrices .
Linear Algebra [ edit ]
Many common linear algebra operations are simple to program using Octave’s matrix syntax. Read more about linear algebra .
Accessing Elements [ edit ]
Octave is 1-indexed. Matrix elements are accessed as matrix(rowNum, columnNum) . Read more about accessing elements .
Control flow with loops [ edit ]
Octave supports for and while loops, as well as other control flow structures. Read more about control flow .
Vectorization [ edit ]
For-loops can often be replaced or simplified using vector syntax. The operators * , / , and ^ all support element-wise operations writing a dot . before the operators. Many other functions operate element-wise by default ( sin , + , - , etc.). Read more about vectorization .
Plotting [ edit ]
The function plot can be called with vector arguments to create 2D line and scatter plots. Read more about plotting .
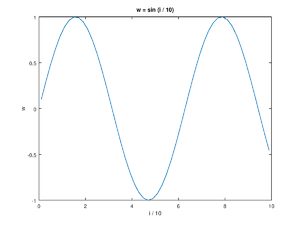
Strings [ edit ]
Strings are simply arrays of characters. Strings can be composed using C-style formatting with sprintf or fprintf . Read more about strings .
If-else [ edit ]
Conditional statements can be used to create branching logic in your code. Read more in the manual .
Getting Help [ edit ]
The help and doc commands can be invoked at the Octave prompt to print documentation for any function.
Octave packages [ edit ]
Community-developed packages can be added from the Octave Packages website to extend the functionality of Octave’s core library. (Matlab users: Packages act similarly to Matlab’s toolboxes.) The pkg command is used to manage these packages. For example, to use the image processing library visit its page on Octave Packages, copy the install command and run it in octave
Read more about packages .
Octave User Codes [ edit ]
There are also User Codes available for GNU Octave which are not part of the core program or any of the packages.
See Category User Codes .
Navigation menu
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
How to load and modify matrices and vectors in Octave?
- How to Convert matrix to a list of column vectors in R ?
- How to inverse a vector in MATLAB?
- How to find the transpose of a tensor in PyTorch?
- How to Permute the Rows and Columns in a Matrix on MATLAB?
- Turn a Matrix into a Row Vector in MATLAB
- Program for addition of two matrices
- How to solve Equality of Matrices?
- How to create matrix and vector from CSV file in R ?
- How to find length of matrix in R
- How to Initialize 3D Vector in C++ STL?
- C++ Program For Addition of Two Matrices
- Java Program to Multiply two Matrices of any size
- How to read a Matrix from user in Java?
- How to Find Indices and Values of Nonzero Elements in MATLAB?
- Addition of Two Matrices in PHP
- How to Select Random Rows from a Matrix in MATLAB?
- How to Convert Sparse Matrix to Dense Matrix in R?
- How to Set the Diagonal Elements of a Matrix to 1 in R?
- Reshaping array as a vector in Julia - vec() Method
In this article, we will see how to load and play with the data inside matrices and vectors in Octave. Here are some basic commands and function to regarding with matrices and vector in Octave : 1. The dimensions of the matrix : We can find the dimensions of a matrix or vector using the size() function.
2. Accessing the elements of the matrix : The elements of a matrix can be accessed by passing the location of the element in parentheses. In Octave, the indexing starts from 1.
3. Longest Dimension : length() function returns the size of the longest dimension of the matrix/vector.
4. Loading Data : First of all let us see how to identify the directories in Octave :
Now before loading the data, we need to change our present working directory to the location where our data is stored. We can do this with the cd command and then load it as follows :
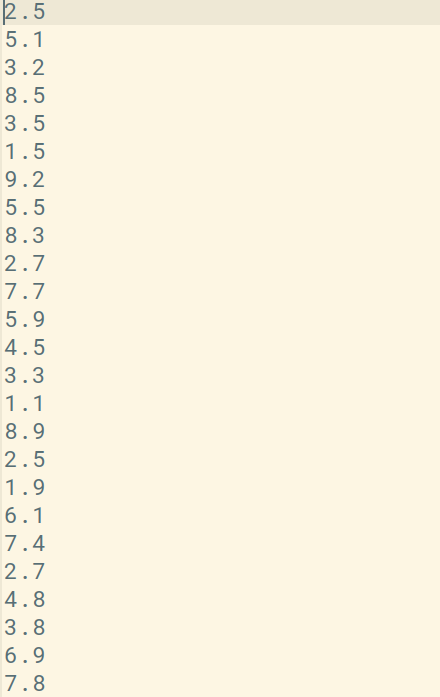
We can use who command for knowing the variables in our current Octave scope or whos for more a detailed description.
We can also select some of the rows from a loaded file, for example in our case 25 records data is present in Feature and target, we can create some other variable to store the trimmed data rows as shown below :
5. Modifying Data : Let us now see how to modify the data of matrices and vectors.
We can also append the new columns and rows in an existing matrix :
We can also concatenate 2 different matrices :
Please Login to comment...
Similar reads.
- Programming Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Scalar, Vector, and Matrix
A scalar is just a fancy word for a number; it is used to distinguish numbers from vectors or matrices.
Rows and Columns
You create a row matrix consisting of the numbers 4 5 6 7 by entering the numbers inside []-brackets and separating the numbers by a comma or a blank.
You create a column matrix consisting of the numbers 0.1 0.2 0.3 by entering the numbers inside []-brackets and separating the numbers by a semicolon.
Row and column matrices are sometimes called vectors.
You can combine row matrices or column matrices
The first number in a vector has index 1. You can get the number at a specified index.
You can use the same notation that creates numbers in for-statements for creating vectors.
You can use the same notation for extracting a vector from a vector.
You can turn a row into a column or the other way around by doing a transpose using the operator '.
Arithmetic with matrices and scalars
You can use the arithmetic operators + , - , * and / on a matrix and a scalar. The operation is applied to each element of the matrix.
Functions of matrices
You can apply functions on matrices, the function is then applied to each element of the matrix.
General matrices
A general matrix consists of n rows and m columns.
Try these commands:
Try the commands ones(3), zeros(4), eye(5) !
Element by Element
You can perform element by element operations on two matrices having the same dimension, i.e. having the same number of rows and columns respectively. When performing an element by element operation the result is a new matrix having the same dimension as the two operands.
When doing an element by element addition, the element on place (row, col) in the resulting matrix will be the sum of the two elements at (row, col) in the operand matrices.
The regular arithmetic operators will become element-by-element operators if you place a dot in front of them.
.+ .- .* ./ .^
When applying a function on a matrix the function is applied to each element of the matrix
Using element by element operations you can combine comparisons and arithmetic.
Regular linear algebra
In regular mathematics, matrix addition and subtraction are defined to be element by element operations. Since using the Octave operators without any dot means "regular" usage, there is no difference between + and .+ , or between - and .- . When it comes to multiplication, division and to-the-power-of, there is a difference between "regular" usage and the element-by-element usage; hence do not use these operators without a dot in front of them (unless you actually know linear algebra).
further info:
GNU Octave, 8.3 Arithmetic Operators by delorie software
by Malin Christersson under a Creative Commons Attribution-Noncommercial-Share Alike 2.5 Sweden License
www.malinc.se
Multivariate Statistical Techniques Matrix Operations in Octave
Multiplication by a scalar, matrix addition & subtraction, matrix multiplication, transpose of a matrix, common vectors, unit vector, common matrices, unit matrix, diagonal matrix, identity matrix, symmetric matrix, inverse of a matrix, inverse & determinant of a matrix, number of rows & columns, computing column & row sums, computing column & row means, horizontal concatenation, vertical concatenation (appending).
- MATLAB Answers
- File Exchange
- AI Chat Playground
- Discussions
- Communities
- Treasure Hunt
- Community Advisors
- Virtual Badges
- Trial software
You are now following this question
- You will see updates in your followed content feed .
- You may receive emails, depending on your communication preferences .
Assigning a vector to multiple rows of a matrix

Direct link to this question
https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix
0 Comments Show -2 older comments Hide -2 older comments
Sign in to comment.
Sign in to answer this question.
Accepted Answer

Direct link to this answer
https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#answer_137011
1 Comment Show -1 older comments Hide -1 older comments

Direct link to this comment
https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#comment_412934
More Answers (2)
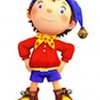
https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#answer_137020
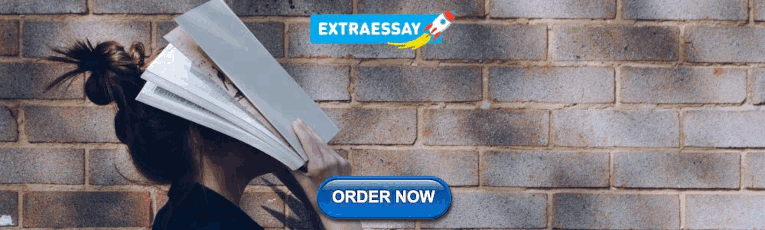
2 Comments Show None Hide None

https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#comment_770080

https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#comment_869511

https://www.mathworks.com/matlabcentral/answers/129805-assigning-a-vector-to-multiple-rows-of-a-matrix#answer_137012
- matrix assignment
Community Treasure Hunt
Find the treasures in MATLAB Central and discover how the community can help you!
An Error Occurred
Unable to complete the action because of changes made to the page. Reload the page to see its updated state.
Select a Web Site
Choose a web site to get translated content where available and see local events and offers. Based on your location, we recommend that you select: .
You can also select a web site from the following list
How to Get Best Site Performance
Select the China site (in Chinese or English) for best site performance. Other MathWorks country sites are not optimized for visits from your location.
- América Latina (Español)
- Canada (English)
- United States (English)
- Belgium (English)
- Denmark (English)
- Deutschland (Deutsch)
- España (Español)
- Finland (English)
- France (Français)
- Ireland (English)
- Italia (Italiano)
- Luxembourg (English)
- Netherlands (English)
- Norway (English)
- Österreich (Deutsch)
- Portugal (English)
- Sweden (English)
- United Kingdom (English)
Asia Pacific
- Australia (English)
- India (English)
- New Zealand (English)
- 简体中文 Chinese
- 日本 Japanese (日本語)
- 한국 Korean (한국어)
Contact your local office
PolyBrute 12
The art of expression
PolyBrute 12 is the most expressive synthesizer ever. With a FullTouch® keyboard, unrivaled sonic palette and advanced software companion - it offers more sonic possibilities than any other analog synthesizer.
Find a dealer near you →
- Sound Design
- Overview Details Resources Sound Design Start
If you thought you knew what a polysynth was capable of - think again. If you thought you knew what the interplay between human touch and analog synthesis could sound like - think again.
PolyBrute 12 takes in-the-moment sonic expression to a whole new level, with a groundbreaking FullTouch® keyboard that brings you into direct and tactile connection with your sound like never before.
A machine as sensitive as it is powerful, embodying a dynamic and expressive sound on par with acoustic instruments.
The next-generation keyboard; the 12-voice architecture; the modulation matrix; the Morphée pad; the ribbon control; the bi-timbral morphing presets - PolyBrute 12 unites them all so you can discover truly breathtaking composition textures.
The future of analog synthesis
Experience unprecedented tactile control while you play
Conduct an ensemble of parameters with every keystroke, feel the Brute force of PolyBrute’s analog VCO’s, weave a web of envelopes and LFO’s with the modulation matrix - discover a colossal, rich, luminous and infinitely mutable sound that will define a new era of expressive analog synthesis.
FullTouch® MPE keyboard
PolyBrute 12’s FullTouch® keyboard enables polyphonic aftertouch expression across the entire key movement range, inviting incredibly dynamic composition unmatched by any other synth. Choose between regular synth action aftertouch modes and FullTouch® modes for a cascade of modulation at your fingertips.
Limitless sound design
The 12-voice architecture of PolyBrute 12 combined with its analog oscillators, filters, advanced matrix and integrated FX allow for an infinite spectrum of sonic color to be explored. Choose between 480 dynamic presets or advanced end-to-end sound design and discover PolyBrute’s seismic sound.
Morphing & multi-axis control
Morph seamlessly between two states in a single preset and discover PolyBrute’s innovative Morphée controller which allows you to transition between 3 destination parameters. Combined with PolyBrute’s keyboard, discover truly limitless in-the-moment modulation while you play.
Seamless DAW integration
PolyBrute Connect provides a coherent bridge between PolyBrute and your virtual studio projects. Manage your patches and easily import new presets and sound banks. Control PolyBrute in real-time with every parameter change mirrored between synth and software.
Sound corner
A truly polyvalent synth, able to traverse vast sonic territory
From the most striking basses to glistening pads and utterly alive and undulating composition textures - PolyBrute 12’s sound transcends all boundaries.
Epic Journey
Michael geyre.
"Epic Journey" is an epic cinematic ambient track. All sounds come from the Polybrute 12.
Feather Wings
"Feather Wings" is a dark ambient cinematic track using seven sounds from the Polybrute 12.
Fluttering Away
Jean-michel blanchet.
"Fluttering Away" is a downtempo ambient track. All sounds come from the Polybrute 12 except the rim shot percussion. All the expressivity controllers available in the machine (Full Touch modes, Morphee, Ribbon controller) were used for this track.
Fortress of Feelings
Victor morello.
Fortress of Feelings is a dark cinematic track. All the sounds come from the PolyBrute 12 except the drums and percussions.
Maxime Desormieres
All in all, 22 presets are used during this demo while playing the poly aftertouch modes, Morphee & Ribbon controller, showing you the true potential of this synthesizer.
Lone Walker
Lone_Walker is a dark psy goa trance track. All the sounds come from the Polybrute 12.
The most expressive synthesizer ever
Discover an immense and animate sound that will send shivers up your spine.
At Arturia we believe that technology can enhance the connection between humans and sound. By engineering a machine to be as sensitive as it is powerful, where every subtle nuance and detail of performance is captured - the inner creative voice can be heard with more resolution than ever before.
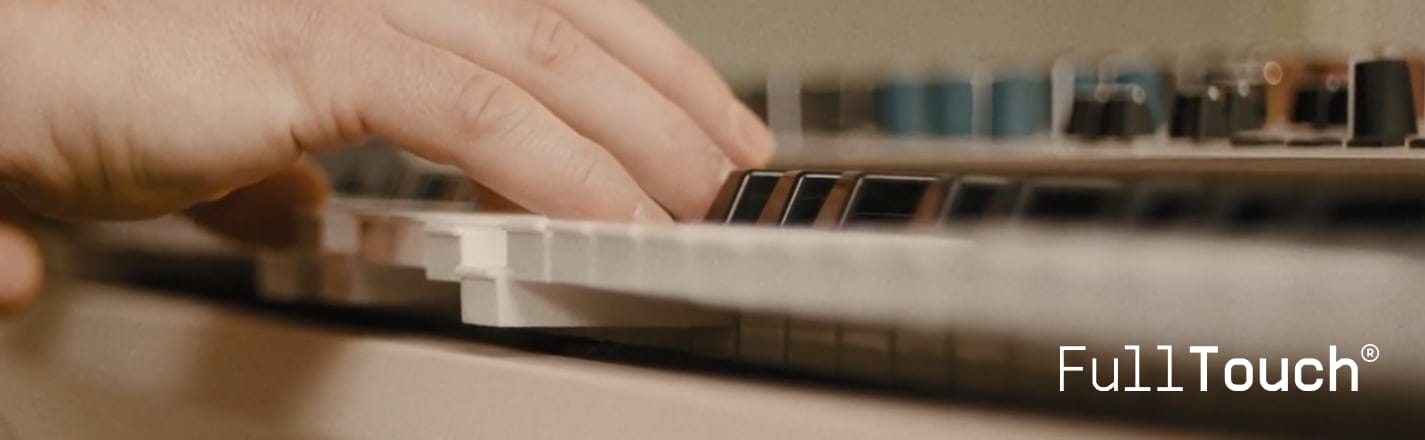
A patented keybed architecture that has been years in the making: FullTouch® describes the keyboards’ dynamic aftertouch responsiveness - able to capture the precise position and acceleration of each key across the entire movement range.
With three new aftertouch modes, PolyBrute 12 invites a playing style that evolves and breathes; allowing composers, artists and synthesists alike to express their inner creative voice with unparalleled delicacy and detail - for a sound that can go from incredibly subtle to infinitely expansive in one motion.
12-Voice Polyphony
Create complex and lush harmonic structures, huge unison effects, dynamic sonic layering and split keyboard performances with PolyBrute 12’s expanded voice architecture. Use each hand and finger to command a unique aftertouch input per key for never-before-heard composition textures.
Morphée controller
It does more than just put morphing at your fingertips; you can discreetly map the X and Y touch axes plus the Z pressure axis to bring a new form of control to any destination parameters available in the Mod Matrix. You can even use the Morphée to randomize your sequences or arpeggios with spice, dice, and ratcheting!
The ribbon controller
We’ve resurrected the coveted ribbon controller, once a staple on classic hardware synthesizers. Positioned immediately above the keybed, you can assign it to your chosen modulation destination and glide effortlessly up and down to alter your sound.
Unrivaled sonic palette
PolyBrute 12’s voices, signature Brute oscillators, dual analog filters and modulation matrix allow for more sonic possibilities than any other synth - from luminous pads to synth-string plucks.
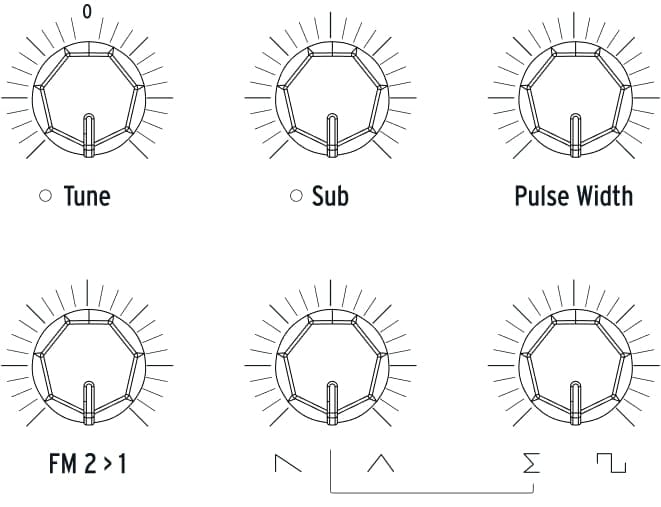
The Oscillators
It all begins with two powerful Brute analog VCOs that generate sawtooth, triangle, and square waves simultaneously. Add edge with the Metalizer and low-end depth with sub-oscillator, as well as having cross-mod & sync controls right at your fingertips.
The Steiner & Ladder Filters
Add color and shape with two classic filter designs, configurable in series, parallel, or continuously variable. The first, a Steiner 12db/octave filter with continuous slope type, enhanced with signature Brute Factor feedback; the second, the legendary 24db/octave Ladder filter for that essential low-pass smoothness, supercharged with biting distortion.
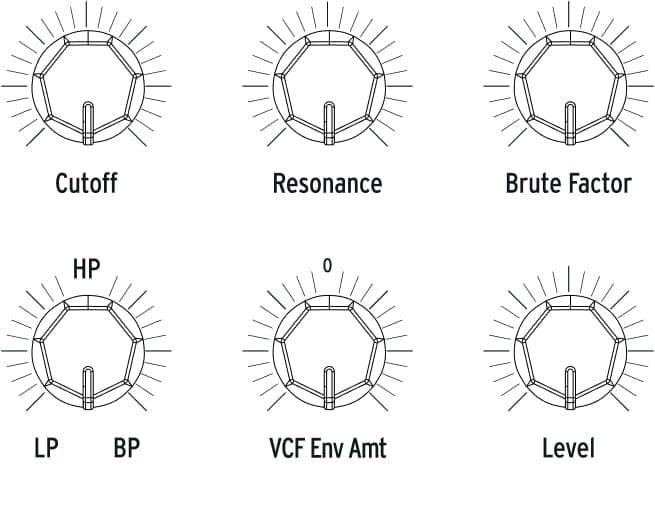
Modulation matrix & more
Create your own unique web of modulators and dive deeper into your sound design than ever before; the Matrix is where PolyBrute 12’s analog reactivity and digital precision collide.
Instant access to 480 presets and hundreds of preset slots, including exclusive patches from the greatest sound designers in the world.
2 Sequencer
A tactile visual interface with everything you need to create dynamic polyphonic sequences, arpeggios, parameter motion recording and more.
A 96-point digital patchbay with up to 32 destinations - easily route any of your modulation sources and controllers to influence dozens of PolyBrute 12’s parameters at once.
Adjust the modulation sources and destinations for the A and B states within each preset to deepen your morphing contrast and color.
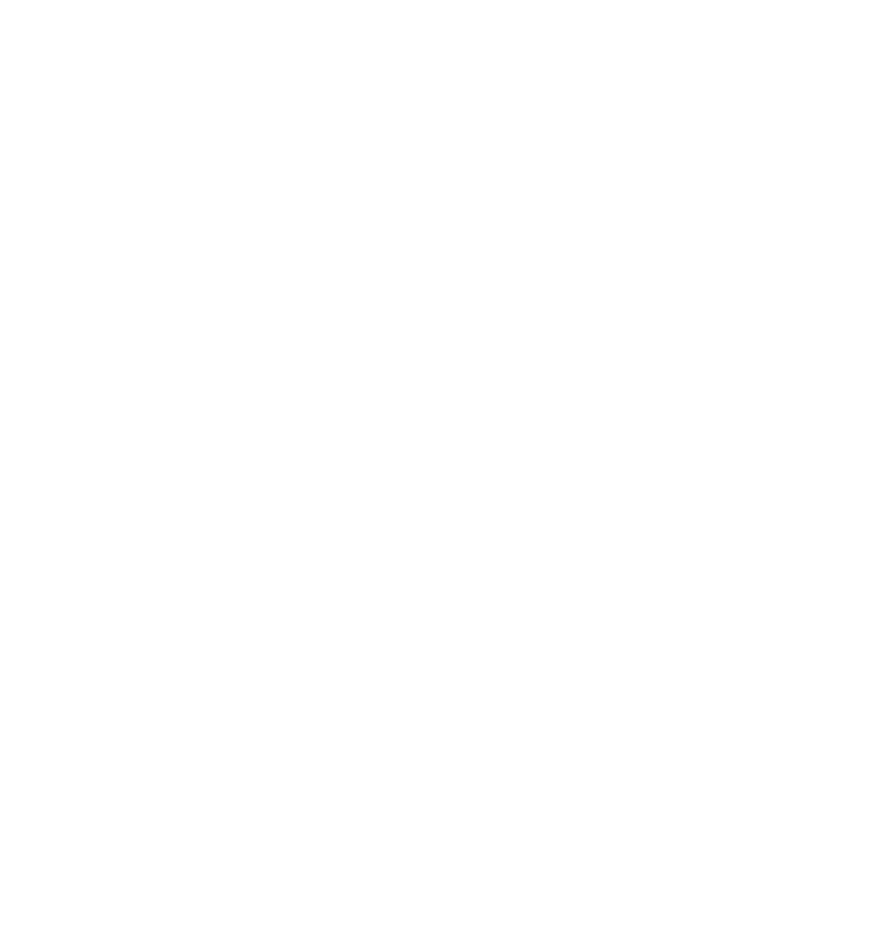
A closer look
Explore PolyBrute 12’s interface in detail, including its unique voice controls, its advanced Modulation Matrix, and its array of evolved expression tools.
PolyBrute 12 Front
Vcf 1 (steiner filter).
Classic 12db/octave multimode filter updated to be continuously variable between lowpass, highpass, bandpass and notch modes. Crank up the Brute Factor feedback control to add bark and bite as desired.
VCF 2 (Ladder Filter)
Legendary 24db/octave lowpass filter inspired by Dr. Bob Moog. Embellishments include distortion overdrive and high-resonance gain compensation.
Balances the levels of all three sound sources and determines the routing of each through one or both filters.
Master Cutoff Control
Big macro control knob for simultaneous control over the cutoff frequencies for both filters. Run PolyBrute 12's two filters in series to create unique filter characteristics, or in parallel for stereo synthesis with different sounds per channel. You can even craft a mix of both modes for yet another signature sound!
Dedicated easy-access amount controls for instant hardwired filter modulation. VCF 1 (Steiner) is modulated by VCO 2, and VCF 2 (Ladder) is modulated by the Noise generator.
3 Envelope Generators
Dedicated velocity-sensitive ADSR Envelope Generators for VCF and VCA - no Matrix assignment required. The freely assignable third EG complete with added delay stage is ready to shape whatever sonic parameter you want in the Matrix.
61-note MPE polyphonic keyboard that tracks position, pressure, and touch of every key. Up to 12-voice polyphony with split, layer and unison capabilities.
Multifunction Matrix
• Presets mode: Easily save and recall 768 memory locations - even more with the PolyBrute Connect software. PolyBrute 12 comes with 480 professionally designed patches to inspire you right out of the box. • Mods mode: Route any of PolyBrute 12's modulation sources to one or more destinations - almost any knob or fader - with positive or negative amounts set via the large encoder knob. With up to 32 modulation destinations and 64 total connections, what new sounds will you discover? • Sequencer mode: The Matrix is the perfect medium to visualize creating and editing polyphonic sequences. Button color intuitively represents step status including notes, ties, mutes and other nuances.
Ribbon Controller
Touch-sensitive strip immediately above the keyboard ready for you to slip, slide and tap to control pitch, volume or any Matrix destination you want to assign. This rare controller is positioned in such a way that you can even incorporate it in your keyboard fingering!
Output Section
Determines stereo placement of voices, overall fine tuning, headphone volume, master volume and more.
Digital Effects
This built-in array of high-quality stereo digital effects - chorus, phaser, flanger, delays, reverbs and more can also be modulated via the Matrix to become an integral part of your synth patch designs.
Motion Recorder
Simple means to instantly record your real-time performance of a single synth control, then play it back with every subsequent note trigger. Great for easily creating custom envelope curves for most PolyBrute parameters.
Sequencer/Arpeggiator
• Sequencer mode: Craft polyphonic sequences of up to 64 steps, including velocity, accents, slides and randomization. Three parallel modulation tracks can automate timbre changes along with triggered notes or serve alone as exotic envelopes. • Arpeggiator mode: Steps through your held notes in a variety of musically useful orders, optionally repeated in higher octaves. Matrix Arpeggiator mode: Advanced sequencer/arpeggiator hybrid that lets you construct a 16-step framework to generate complex arpeggios. This opens up a whole new realm of assisted performance.
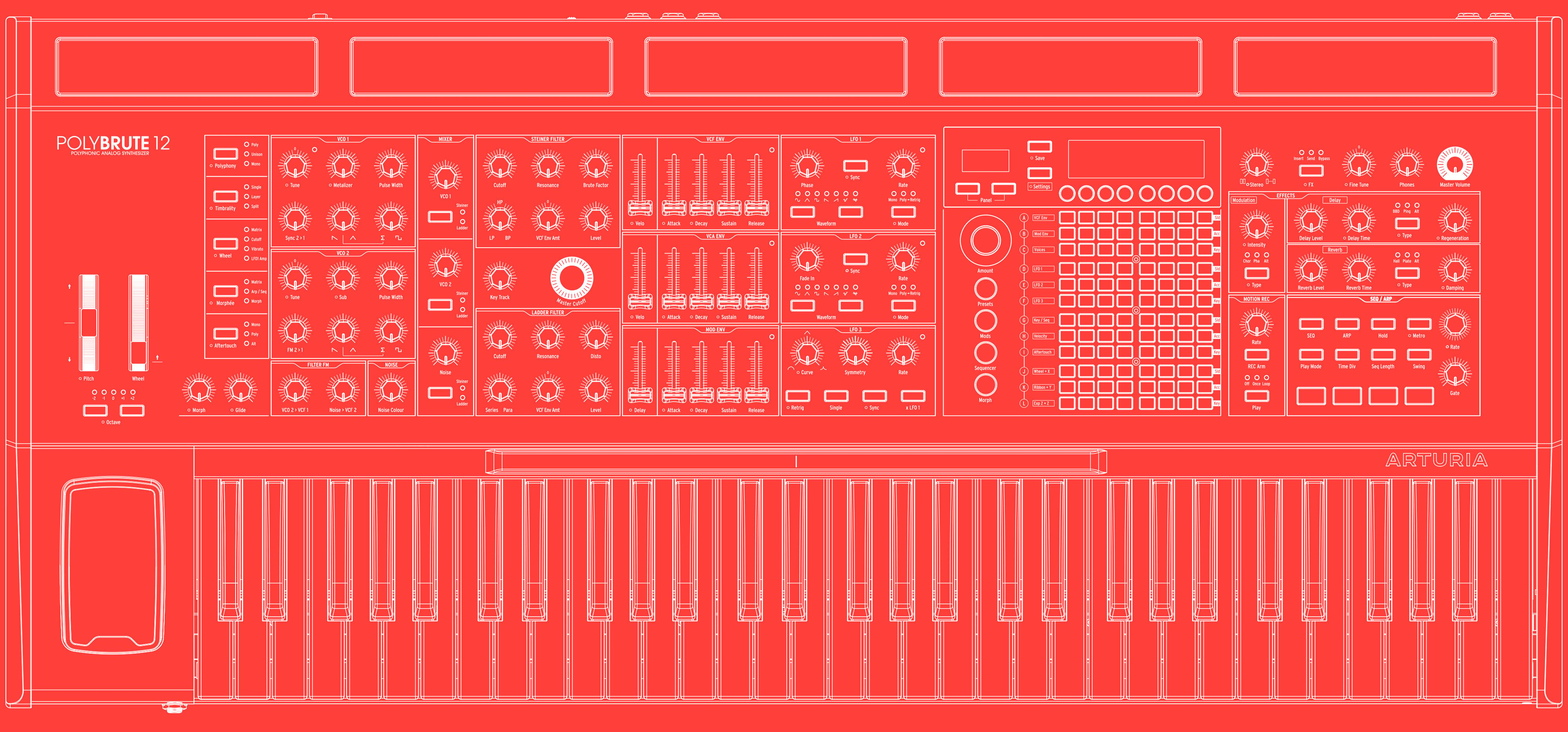
PolyBrute 12 Back
Capture the full sonic power and width of PolyBrute 12 with stereo audio output.
Analog clock
Keep things in sync and mediate timing or tempo between other analog gear and modular rigs with control voltage in/out.
1 sustain and 2 expression pedal ports enable advanced hands-free modulation.
Memory Protection On/Off
When On, prevents the PolyBrute 12's patch memories from being overwritten.
Connect MIDI In, Out or Thru to get PolyBrute 12 talking to your setup.
Connect to your computer for updates, preset management and using PolyBrute 12 with its companion software: PolyBrute Connect.
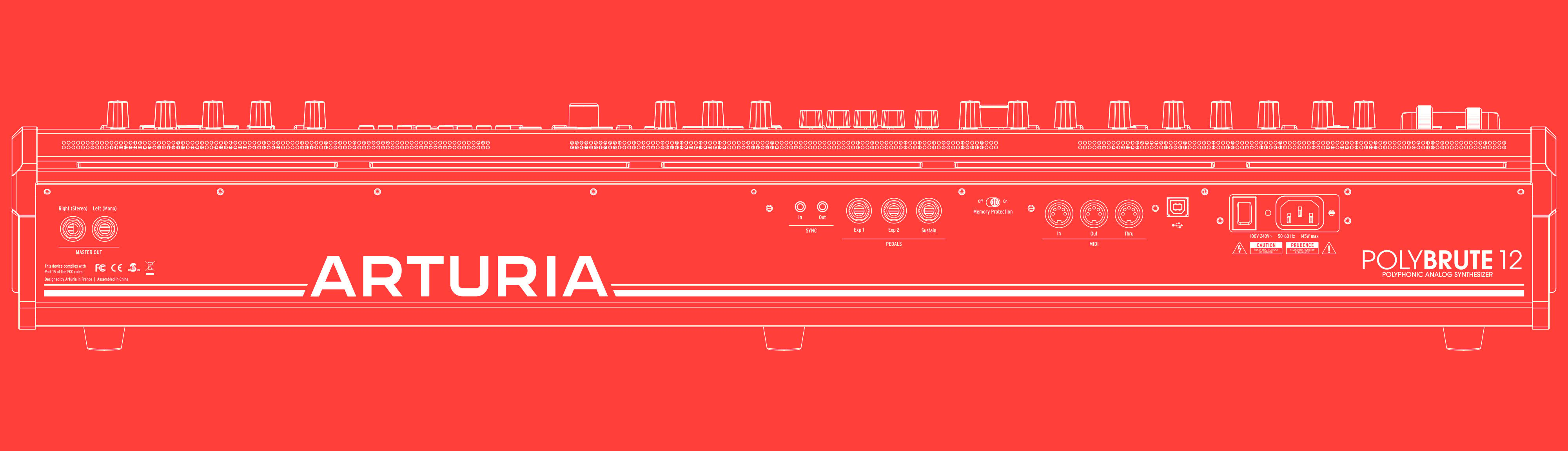
The most advanced studio integration
Combining the tactility of analog hardware with the convenience of virtual instrument flexibility, PolyBrute Connect replicates the entire front panel as a standalone Mac/PC app and a plugin for all major DAWs.
Craft sound
Explore the depths of PolyBrute’s advanced features with a like-for-like virtual interface.
Control in real-time
Every parameter change, either on synth or software, is mirrored by the other.
Manage your patches
Organize your favorite sounds with an intuitive drag-and-drop interface, and easily import new presets & sound banks.
Preview sounds from the store
Discover new sonic territories with the Sound Store and preview sound banks directly on PolyBrute.
Distinction in design
PolyBrute 12 represents a leap forward in analog synthesis, while remaining firmly rooted in synth design heritage.
PolyBrute enters a new era with its groundbreaking FullTouch® MPE keyboard combined with the same controls, buttons, and expressive components that are a delight to use. Crafted with the finest materials, including walnut wood and a striking aluminum exterior - PolyBrute 12 is designed to meet the demands of professional musicians as well as appeal to the enthusiasts. Its not just for looks either, the vents at the back provide passive cooling so PolyBrute can operate in complete silence.
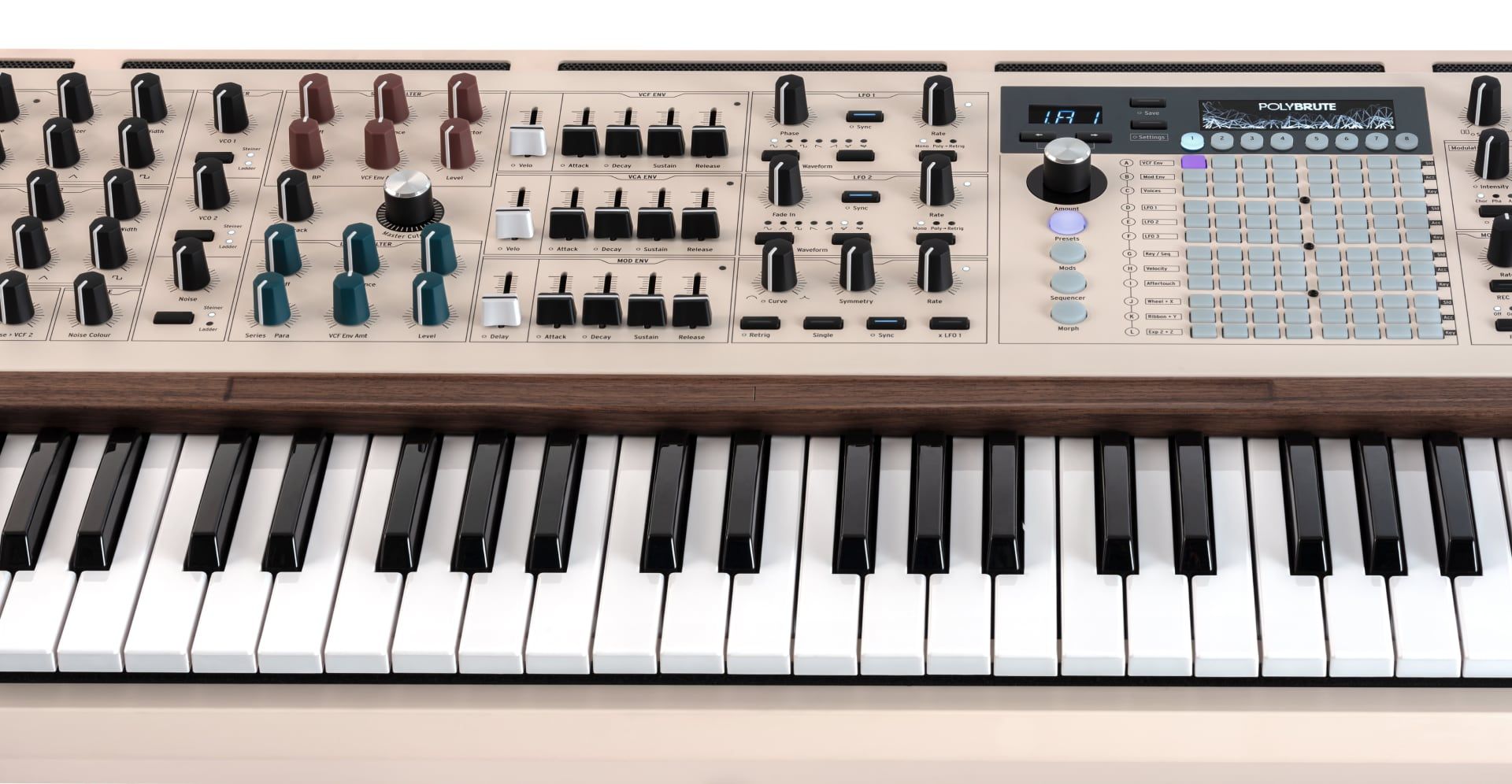
Main Features
Analog morphing synthesizer.
- 12 voices of Polyphony
- Mono, Unison, Poly voicing
- Single, Split, Layer modes
61-keys with FullTouch® MPE Technology keyboard
Pitch bend, mod wheel, ribbon controllers, morphée touch and pressure sensitive 3d controller, osc and noise mixer with filter routing, 12db/oct steiner parker filter.
- Continuous LP>Notch>HP>BP morphing
- Cutoff, Resonance, Brute Factor
24dB/Oct Ladder Filter with Distortion
Three envelopes.
- Looping capability
Three stereo digital effects
- Modulation FX : Chorus, Phaser, Flanger, Ring Modulation
- Delay : 9 algorithms including BBD, Digital delay
- Reverb : 9 algorithms including Hall, Plate, Spring, Shimmer
- Distortions : Subtle Tape, Classic Distortion, Soft Clip, Worn Out Tape, Germanium, BitCrusher, DownSampler
Arpeggiator, Matrix Arpeggiator, Sequencer
Stereo audio output, 2 expression and 1 sustain pedal inputs, two analog vco's.
- Triangle + Metalizer
- Square + Pulse Width
Noise Generator
- Continuous tone from rumble noise to white noise
- LFO1 and LFO2 with waveform selection
- LFO3 with waveform shaping using Shape and Symmetry
- Rate control & Tempo Sync
- Various retrig options
480 presets and 768 preset slots
12x32 modulation matrix.
- Notes, Accent, Slide per step
- 3 tracks of automation
MIDI and USB i/o + analog clock i/o
Platform specifications.
- Win 10+ (64bit)
- 4 cores CPU, 3.4 GHz (4.0 GHz Turbo-boost)
- 3GB free hard disk space
- OpenGL 2.0 compatible GPU
- ARM processors not supported on Windows
Required configuration
- Works in Standalone, VST, AAX, Audio Unit.

- 4 cores CPU, 3.4 GHz (4.0 GHz Turbo-boost) or M1 CPU
Work with ASC
- An elegant and simple solution to help you install, activate, and update your Arturia software instruments.
What’s in the box
Box content.
- PolyBrute 12 Unit
- User Manual
- Registration Card
Instrument dimensions
- Size : 38.3 x 17.1 x 6.1 inches (972 x 435 x 156mm)
- Weight : 50.7lbs (23kg)
Next: Increment Ops , Previous: Boolean Expressions , Up: Expressions [ Contents ][ Index ]
8.6 Assignment Expressions
An assignment is an expression that stores a new value into a variable. For example, the following expression assigns the value 1 to the variable z :
After this expression is executed, the variable z has the value 1. Whatever old value z had before the assignment is forgotten. The ‘ = ’ sign is called an assignment operator .
Assignments can store string values also. For example, the following expression would store the value "this food is good" in the variable message :
(This also illustrates concatenation of strings.)
Most operators (addition, concatenation, and so on) have no effect except to compute a value. If you ignore the value, you might as well not use the operator. An assignment operator is different. It does produce a value, but even if you ignore the value, the assignment still makes itself felt through the alteration of the variable. We call this a side effect .
The left-hand operand of an assignment need not be a variable (see Variables ). It can also be an element of a matrix (see Index Expressions ) or a list of return values (see Calling Functions ). These are all called lvalues , which means they can appear on the left-hand side of an assignment operator. The right-hand operand may be any expression. It produces the new value which the assignment stores in the specified variable, matrix element, or list of return values.
It is important to note that variables do not have permanent types. The type of a variable is simply the type of whatever value it happens to hold at the moment. In the following program fragment, the variable foo has a numeric value at first, and a string value later on:
When the second assignment gives foo a string value, the fact that it previously had a numeric value is forgotten.
Assignment of a scalar to an indexed matrix sets all of the elements that are referenced by the indices to the scalar value. For example, if a is a matrix with at least two columns,
sets all the elements in the second column of a to 5.
Assigning an empty matrix ‘ [] ’ works in most cases to allow you to delete rows or columns of matrices and vectors. See Empty Matrices . For example, given a 4 by 5 matrix A , the assignment
deletes the third row of A , and the assignment
deletes the first, third, and fifth columns.
An assignment is an expression, so it has a value. Thus, z = 1 as an expression has the value 1. One consequence of this is that you can write multiple assignments together:
stores the value 0 in all three variables. It does this because the value of z = 0 , which is 0, is stored into y , and then the value of y = z = 0 , which is 0, is stored into x .
This is also true of assignments to lists of values, so the following is a valid expression
that is exactly equivalent to
In expressions like this, the number of values in each part of the expression need not match. For example, the expression
is equivalent to
The number of values on the left side of the expression can, however, not exceed the number of values on the right side. For example, the following will produce an error.
The symbol ~ may be used as a placeholder in the list of lvalues, indicating that the corresponding return value should be ignored and not stored anywhere:
This is cleaner and more memory efficient than using a dummy variable. The nargout value for the right-hand side expression is not affected. If the assignment is used as an expression, the return value is a comma-separated list with the ignored values dropped.
A very common programming pattern is to increment an existing variable with a given value, like this
This can be written in a clearer and more condensed form using the += operator
Similar operators also exist for subtraction ( -= ), multiplication ( *= ), and division ( /= ). An expression of the form
is evaluated as
where op can be either + , - , * , or / , as long as expr2 is a simple expression with no side effects. If expr2 also contains an assignment operator, then this expression is evaluated as
where temp is a placeholder temporary value storing the computed result of evaluating expr2 . So, the expression
You can use an assignment anywhere an expression is called for. For example, it is valid to write x != (y = 1) to set y to 1 and then test whether x equals 1. But this style tends to make programs hard to read. Except in a one-shot program, you should rewrite it to get rid of such nesting of assignments. This is never very hard.
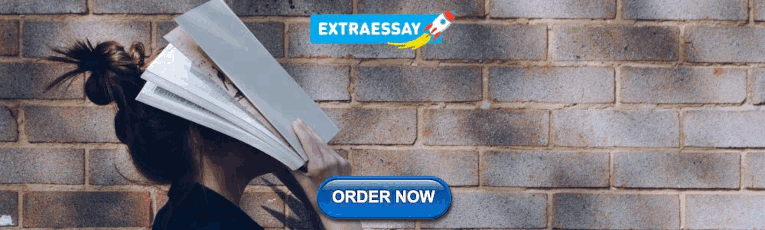
IMAGES
VIDEO
COMMENTS
When you type a matrix or the name of a variable whose value is a matrix, Octave responds by printing the matrix in with neatly aligned rows and columns. If the rows of the matrix are too large to fit on the screen, Octave splits the matrix and displays a header before each section to indicate which columns are being displayed.
1. I am trying to do something like this in Octave: Assign some variables to a matrix, do some operation on the matrix and then assign the members of the matrix back to the variables, e.g: x=1; y=2; d=[x y]; d=(d.^2)+1; [x y]=d; However, this does not work and only x is assigned the complete matrix.
18.2 Basic Matrix Functions. Balance the matrix A to reduce numerical errors in future calculations. Compute AA = DD \ A * DD in which AA is a matrix whose row and column norms are roughly equal in magnitude, and DD = P * D, in which P is a permutation matrix and D is a diagonal matrix of powers of two.
Creating vectors and matrices. Here is how we specify a row vector in Octave: octave:1> x = [1, 3, 2] x =. 1 3 2. Note that. the vector is enclosed in square brackets; each entry is separated by an optional comma. x = [1 3 2] results in the same row vector. To specify a column vector, we simply replace the commas with semicolons:
The 3-input form returns a diagonal matrix with vector v on the main diagonal and the resulting matrix being of size m rows x n columns. Given a matrix argument, instead of a vector, diag extracts the k-th diagonal of the matrix. Function File: blkdiag (A, B, C, …) Build a block diagonal matrix from A, B, C, …
1.1 Creating. Vectors are created by using: Square brackets. Commas or spaces between numbers (or a mixture of both) When working with vectors the output can get quite large so we will use format compact to reduce the space used: format compact # Commas between numbers x = [1, 3, 2] # Spaces between numbers x = [1 3 2] # A mixture of commas and ...
For a matrix argument, any returns a row vector of ones and zeros with each element indicating whether any of the elements of the corresponding column of the matrix are nonzero. For example, octave:13> any (eye (2, 4)) ans = 1 1 0 0 To see if any of the elements of a matrix are nonzero, you can use a statement like any (any (a)) For a matrix ...
Octave is a program specially designed for manipulating matrices. Simply speaking, an n by m matrix is a box of numbers with n rows and m columns. Vectors are a special case of a matrix where there is only one row (a row vector) or only one column (a column vector). Numbers are also a special case of a matrix as Octave regards them as 1 by 1 ...
18.2 Basic Matrix Functions AA = balance (A) AA = balance (A, opt) [DD, AA] = balance (A, opt)[D, P, AA] = balance (A, opt)[CC, DD, AA, BB] = balance (A, B, opt)Balance the matrix A to reduce numerical errors in future calculations.. Compute AA = DD \ A * DD in which AA is a matrix whose row and column norms are roughly equal in magnitude, and DD = P * D, in which P is a permutation matrix and ...
Assign values to variables with = (Note ... matrices. One-dimensional matrices are referred to as vectors. Use a space or a comma , to separate elements in a row and semicolon ; to start a ... Linear Algebra . Many common linear algebra operations are simple to program using Octave's matrix syntax. Read more about linear algebra ...
We can load the file with the load command in Octave, there are actually 2 ways to load the data either simply with load command or using the name of the file as a string in load (). We can use the name of the file like Feature or target to print its data. MATLAB. % loading Feature.dat.
You create a column matrix consisting of the numbers 0.1 0.2 0.3 by entering the numbers inside []-brackets and separating the numbers by a semicolon. Row and column matrices are sometimes called vectors. You can combine row matrices or column matrices. >>>error: number of rows must match (3 != 1) near line 43, column 16.
16 Matrix Manipulation. There are a number of functions available for checking to see if the elements of a matrix meet some condition, and for rearranging the elements of a matrix. For example, Octave can easily tell you if all the elements of a matrix are finite, or are less than some specified value. Octave can also rotate the elements ...
Inverse & Determinant of a Matrix octave: C = [2,1,6;1,3,4;6,4,-2] C = 2 1 6 1 3 4 6 4 -2 octave: CI = inv(C) CI = 0.215686 -0.254902 0.137255 -0.254902 0.392157 0.019608 0.137255 0.019608 -0.049020 octave: d = det(C) d = -102 ... Number of Rows & Columns octave: X = [3,2;2,-2;4,6;3,1] X = 3 2 2 -2 4 6 3 1 octave: r = rows(A) r = 3 octave: c ...
r = [1 3 5]; %and the corresponding columns: c = [1 2 3]; %now get your indices: idx = sub2ind (size (B),r,c); %and use these indices as follows: B (idx) = A (c); This assumes that you want your columns filled in the order that they are found in A, but you could just as easily make a different index into A to pick particular values from that ...
An index expression allows you to reference or extract selected elements of a matrix or vector. Indices may be scalars, vectors, ranges, or the special operator ': ', which may be used to select entire rows or columns. Vectors are indexed using a single index expression. Matrices (2-D) and higher multi-dimensional arrays are indexed using ...
Limitless sound design. The 12-voice architecture of PolyBrute 12 combined with its analog oscillators, filters, advanced matrix and integrated FX allow for an infinite spectrum of sonic color to be explored. Choose between 480 dynamic presets or advanced end-to-end sound design and discover PolyBrute's seismic sound.
Expected output is 3,4; 5,6 , I donot want output, I want to multiply the matrix p * q where q dimension is from (row+1,col). For accessing from (row+1,col) do i need to delete the first row and save it in another variable or can I just multipy by accessing from second row! -
a(:, 2) = 5. sets all the elements in the second column of a to 5. Assigning an empty matrix ' [] ' works in most cases to allow you to delete rows or columns of matrices and vectors. See Empty Matrices . For example, given a 4 by 5 matrix A, the assignment. A (3, :) = [] deletes the third row of A, and the assignment.
My Pythonic intuition wants to write something like a(:,1:) to indicate columns 1 to end of columns, but this does not compile. - user856358. Mar 12, 2021 at 16:30. 3. For anyone who had the same question I had: a(:,2:end) would be the syntax for an open-ended column slice for columns 2 onward! - user856358.